<?php
/**
*
* Idiorm
*
* http://github.com/j4mie/idiorm/
*
* A single-class super-simple database abstraction layer for PHP.
* Provides (nearly) zero-configuration object-relational mapping
* and a fluent interface for building basic, commonly-used queries.
*
* BSD Licensed.
*
* Copyright (c) 2010, Jamie Matthews
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
*
* * Redistributions of source code must retain the above copyright notice, this
* list of conditions and the following disclaimer.
*
* * Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
*/
class ORM {
// ----------------------- //
// --- CLASS CONSTANTS --- //
// ----------------------- //
// Where condition array keys
const WHERE_FRAGMENT = 0;
const WHERE_VALUES = 1;
// ------------------------ //
// --- CLASS PROPERTIES --- //
// ------------------------ //
// Class configuration
protected static $_config = array(
'connection_string' => 'sqlite::memory:',
'id_column' => 'id',
'id_column_overrides' => array(),
'error_mode' => PDO::ERRMODE_EXCEPTION,
'username' => null,
'password' => null,
'driver_options' => null,
'identifier_quote_character' => null, // if this is null, will be autodetected
'logging' => true,
'caching' => false,
);
// Database connection, instance of the PDO class
protected static $_db;
// Last query run, only populated if logging is enabled
protected static $_last_query;
// Log of all queries run, only populated if logging is enabled
protected static $_query_log = array();
// Query cache, only used if query caching is enabled
protected static $_query_cache = array();
// --------------------------- //
// --- INSTANCE PROPERTIES --- //
// --------------------------- //
// The name of the table the current ORM instance is associated with
protected $_table_name;
// Alias for the table to be used in SELECT queries
protected $_table_alias = null;
// Values to be bound to the query
protected $_values = array();
// Columns to select in the result
protected $_result_columns = array('*');
// Are we using the default result column or have these been manually changed?
protected $_using_default_result_columns = true;
// Join sources
protected $_join_sources = array();
// Should the query include a DISTINCT keyword?
protected $_distinct = false;
// Is this a raw query?
protected $_is_raw_query = false;
// The raw query
protected $_raw_query = '';
// The raw query parameters
protected $_raw_parameters = array();
// Array of WHERE clauses
protected $_where_conditions = array();
// LIMIT
protected $_limit = null;
// OFFSET
protected $_offset = null;
// ORDER BY
protected $_order_by = array();
// GROUP BY
protected $_group_by = array();
// The data for a hydrated instance of the class
protected $_data = array();
// Fields that have been modified during the
// lifetime of the object
protected $_dirty_fields = array();
// Fields that are to be inserted in the DB raw
protected $_expr_fields = array();
// Is this a new object (has create() been called)?
protected $_is_new = false;
// Name of the column to use as the primary key for
// this instance only. Overrides the config settings.
protected $_instance_id_column = null;
// ---------------------- //
// --- STATIC METHODS --- //
// ---------------------- //
/**
* Pass configuration settings to the class in the form of
* key/value pairs. As a shortcut, if the second argument
* is omitted and the key is a string, the setting is
* assumed to be the DSN string used by PDO to connect
* to the database (often, this will be the only configuration
* required to use Idiorm). If you have more than one setting
* you wish to configure, another shortcut is to pass an array
* of settings (and omit the second argument).
*/
public static function configure($key, $value=null) {
if (is_array($key)) {
// Shortcut: If only one array argument is passed,
// assume it's an array of configuration settings
foreach ($key as $conf_key => $conf_value) {
self::configure($conf_key, $conf_value);
}
} else {
if (is_null($value)) {
// Shortcut: If only one string argument is passed,
// assume it's a connection string
$value = $key;
$key = 'connection_string';
}
self::$_config[$key] = $value;
}
}
/**
* Despite its slightly odd name, this is actually the factory
* method used to acquire instances of the class. It is named
* this way for the sake of a readable interface, ie
* ORM::for_table('table_name')->find_one()-> etc. As such,
* this will normally be the first method called in a chain.
*/
public static function for_table($table_name) {
self::_setup_db();
return new self($table_name);
}
/**
* Set up the database connection used by the class.
*/
protected static function _setup_db() {
if (!is_object(self::$_db)) {
$connection_string = self::$_config['connection_string'];
$username = self::$_config['username'];
$password = self::$_config['password'];
$driver_options = self::$_config['driver_options'];
$db = new PDO($connection_string, $username, $password, $driver_options);
$db->setAttribute(PDO::ATTR_ERRMODE, self::$_config['error_mode']);
self::set_db($db);
}
}
/**
* Set the PDO object used by Idiorm to communicate with the database.
* This is public in case the ORM should use a ready-instantiated
* PDO object as its database connection.
*/
public static function set_db($db) {
self::$_db = $db;
self::_setup_identifier_quote_character();
}
/**

大可v
- 粉丝: 0
- 资源: 7
最新资源
- 全球前8GDP数据图(python动态柱状图)
- 汽车检测7-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 检测高压线电线-YOLO(v5至v9)、COCO、Darknet、VOC数据集合集.rar
- 检测行路中的人脸-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- Image_17083039753012.jpg
- 检测生锈铁片生锈部分-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- 检测桌面物体-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 基于Java实现的动态操作实体属性及数据类型转换的设计源码
- x32dbg-And-x64dbg-for-windows逆向调试
- 检测是否佩戴口罩-YOLO(v5至v9)、Paligemma、TFRecord、VOC数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


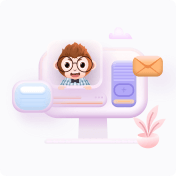