/*******************************************************************************
*
* Copyright 2004-2016 NXP Semiconductor, Inc.
*
* This software is owned or controlled by NXP Semiconductor.
* Use of this software is governed by the NXP FreeMASTER License
* distributed with this Material.
* See the LICENSE file distributed for more details.
*
****************************************************************************//*!
*
* @brief FreeMASTER serial communication routines
*
*******************************************************************************/
#include "freemaster.h"
#include "freemaster_private.h"
#include "freemaster_protocol.h"
#if !(FMSTR_DISABLE)
#if FMSTR_USE_SERIAL
/***********************************
* local variables
***********************************/
/* Runtime base address used when constant address is not specified */
#if FMSTR_SCI_BASE_DYNAMIC
static FMSTR_ADDR pcm_pSciBaseAddr;
#define FMSTR_SCI_BASE pcm_pSciBaseAddr
#endif
#if FMSTR_USE_MBED
#include "serial_api.h"
serial_t* pSerialObj;
#endif
/* FreeMASTER communication buffer (in/out) plus the STS and LEN bytes */
static FMSTR_BCHR pcm_pCommBuffer[FMSTR_COMM_BUFFER_SIZE+3];
/* FreeMASTER runtime flags */
/*lint -e{960} using union */
typedef volatile union
{
FMSTR_FLAGS all;
struct
{
unsigned bTxActive : 1; /* response is being transmitted */
#if (FMSTR_USE_SCI) || (FMSTR_USE_ESCI) || (FMSTR_USE_LPUART)
unsigned bTxWaitTC : 1; /* response sent, wait for transmission complete */
#endif
unsigned bTxLastCharSOB : 1; /* last transmitted char was equal to SOB */
unsigned bRxLastCharSOB : 1; /* last received character was SOB */
unsigned bRxMsgLengthNext : 1; /* expect the length byte next time */
#if FMSTR_USE_JTAG
unsigned bJtagRIEPending : 1; /* JTAG RIE bit failed to be set, try again later */
#endif
#if (FMSTR_USE_USB_CDC) || (FMSTR_USE_MQX_IO) || (FMSTR_USE_JTAG)
unsigned bTxFirstSobSend : 1; /* to send SOB char at the begin of the packet */
#endif
#if FMSTR_USE_MQX_IO
unsigned bMqxReadyToSend : 1; /* to send next character in transmit routine */
#endif
#if FMSTR_USE_USB_CDC
unsigned bUsbCdcStartApp : 1; /* FreeMASTER USB CDC Application start Init Flag */
unsigned bUsbCdcStartTrans : 1; /* FreeMASTER USB CDC Application Carrier Activate Flag */
unsigned bUsbReadyToDecode : 1; /* FreeMASTER packet is received, ready to decode in Poll function in Short Interrupt mode */
#endif
} flg;
} FMSTR_SERIAL_FLAGS;
static FMSTR_SERIAL_FLAGS pcm_wFlags;
/* receive and transmit buffers and counters */
static FMSTR_SIZE8 pcm_nTxTodo; /* transmission to-do counter (0 when tx is idle) */
static FMSTR_SIZE8 pcm_nRxTodo; /* reception to-do counter (0 when rx is idle) */
static FMSTR_BPTR pcm_pTxBuff; /* pointer to next byte to transmit */
static FMSTR_BPTR pcm_pRxBuff; /* pointer to next free place in RX buffer */
static FMSTR_BCHR pcm_nRxCheckSum; /* checksum of data being received */
/* in older versions, the SCI flags were tested directly, new version is more general,
* but it remains backward compatible. If SCI flag test macros are not defined, use
* the equivalent of the old functionality. */
#ifndef FMSTR_SCI_TXFULL
#define FMSTR_SCI_TXFULL(wSciSR) (!((wSciSR) & FMSTR_SCISR_TDRE))
#endif
#ifndef FMSTR_SCI_TXEMPTY
#define FMSTR_SCI_TXEMPTY(wSciSR) ((wSciSR) & FMSTR_SCISR_TC)
#endif
#ifndef FMSTR_SCI_RXREADY
/* when overrun flag is defined, use it also to detect incoming character (and to
* make sure the OR flag is cleared in modules where RDRF and OR are independent */
#ifdef FMSTR_SCISR_OR
#define FMSTR_SCI_RXREADY(wSciSR) ((wSciSR) & (FMSTR_SCISR_RDRF | FMSTR_SCISR_OR))
#else
#define FMSTR_SCI_RXREADY(wSciSR) ((wSciSR) & (FMSTR_SCISR_RDRF))
#endif
#endif
#if FMSTR_DEBUG_TX
/* The poll counter is used to roughly measure duration of test frame transmission.
* The test frame will be sent once per N.times this measured period. */
static FMSTR_S32 pcm_nDebugTxPollCount;
/* the N factor for multiplying the transmission time to get the wait time */
#define FMSTR_DEBUG_TX_POLLCNT_XFACTOR 32
#define FMSTR_DEBUG_TX_POLLCNT_MIN (-1*0x4000000L)
#endif
/***********************************
* local function prototypes
***********************************/
static void FMSTR_Listen(void);
static void FMSTR_SendError(FMSTR_BCHR nErrCode);
#else /* FMSTR_USE_SERIAL */
/*lint -efile(766, freemaster_protocol.h) include file is not used in this case */
#endif /* FMSTR_USE_SERIAL */
#if (FMSTR_USE_SCI) || (FMSTR_USE_ESCI) || (FMSTR_USE_LPUART) || (FMSTR_USE_JTAG)
/***********************************
* local variables
***********************************/
/* SHORT_INTR receive queue (circular buffer) */
#if FMSTR_SHORT_INTR
static FMSTR_BCHR pcm_pRQueueBuffer[FMSTR_COMM_RQUEUE_SIZE];
static FMSTR_BPTR pcm_pRQueueRP; /* SHORT_INTR queue read-pointer */
static FMSTR_BPTR pcm_pRQueueWP; /* SHORT_INTR queue write-pointer */
#endif
/***********************************
* local function prototypes
***********************************/
#if FMSTR_SHORT_INTR
static void FMSTR_RxQueue(FMSTR_BCHR nRxChar);
static void FMSTR_RxDequeue(void);
#endif
/*lint -esym(752,FMSTR_RxQueue) this may be unreferenced in some cases */
/*lint -esym(752,FMSTR_RxDequeue) this may be unreferenced in some cases */
/*******************************************************************************
*
* @brief Routine to quick-receive a character (put to a queue only)
*
* This function puts received character into a queue and exits as soon as possible.
*
*******************************************************************************/
#if FMSTR_SHORT_INTR
static void FMSTR_RxQueue(FMSTR_BCHR nRxChar)
{
/* future value of write pointer */
FMSTR_BPTR wpnext = pcm_pRQueueWP + 1;
/*lint -e{946} pointer arithmetic is okay here (same array) */
if(wpnext >= (pcm_pRQueueBuffer + FMSTR_COMM_RQUEUE_SIZE))
{
wpnext = pcm_pRQueueBuffer;
}
/* any space in queue? */
if(wpnext != pcm_pRQueueRP)
{
*pcm_pRQueueWP = (FMSTR_U8) nRxChar;
pcm_pRQueueWP = wpnext;
}
}
#endif /* FMSTR_SHORT_INTR */
/*******************************************************************************
*
* @brief Late processing of queued characters
*
* This function takes the queued characters and calls FMSTR_Rx() for each of them,
* just like as the characters would be received from SCI one by one.
*
*******************************************************************************/
#if FMSTR_SHORT_INTR
static void FMSTR_RxDequeue(void)
{
FMSTR_BCHR nChar = 0U;
/* get all queued characters */
while(pcm_pRQueueRP != pcm_pRQueueWP)
{
nChar = *pcm_pRQueueRP++;
/*lint -e{946} pointer arithmetic is okay here (same array) */
if(pcm_pRQueueRP >= (pcm_pRQueueBuffer + FMSTR_COMM_RQUEUE_SIZE))
{
pcm_pRQueueRP = pcm_pRQueueBuffer;
}
/* emulate the SCI receive event */
if(!pcm_wFlags.flg.bTxActive)
{
(void)FMSTR_Rx(nChar);
}
}
}
#endif /* FMSTR_SHORT_INTR */
#endif /* (FMSTR_USE_SCI) || (FMSTR_USE_ESCI) || (FMSTR_USE_LPUART) || (FMSTR_USE_JTAG) */
#if (FMSTR_USE_SCI) || (FMSTR_USE_LPUART) || (FMSTR_USE_ESCI)
/**************************************************************************//*!
*
* @brief Handle SCI communication (both TX and RX)
*
* This function checks the SCI flags and calls the Rx and/or Tx functions
*
* @note This function can be called either from SCI ISR or from the polling routine
*
********************
没有合适的资源?快使用搜索试试~ 我知道了~
MPC5744P FreeMaster下位机示例
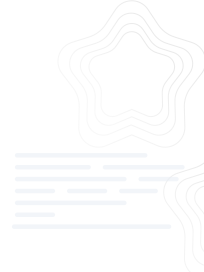
共124个文件
args:25个
o:21个
c:19个

需积分: 50 28 下载量 162 浏览量
2018-12-05
08:11:11
上传
评论 6
收藏 407KB 7Z 举报
温馨提示
MPC5744P FreeMaster下位机示例,可以使用PEMicro作为通信接口,详细请参考文章:https://blog.csdn.net/u010875635/article/details/84789579
资源推荐
资源详情
资源评论
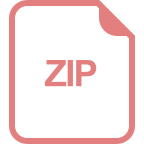
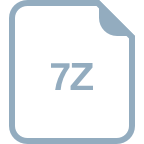
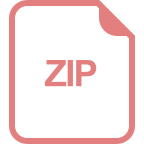
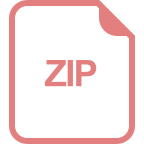
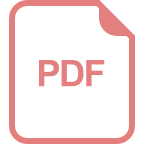
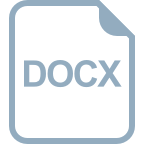
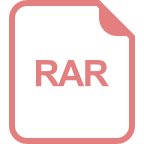
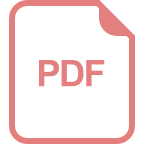
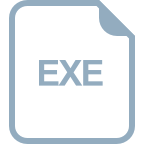
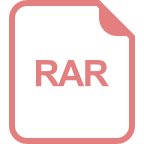
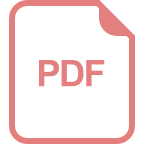
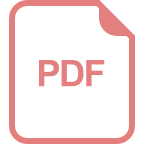
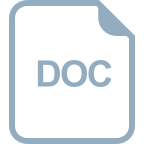
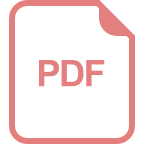
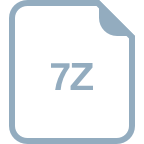
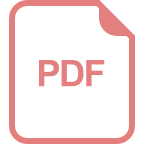
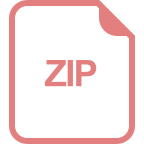
收起资源包目录

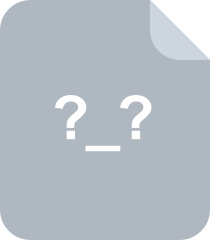
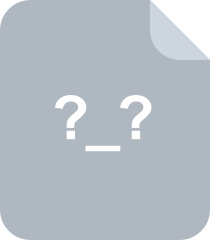
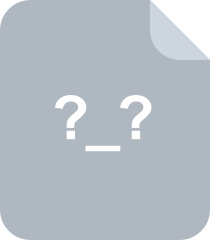
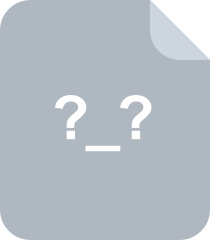
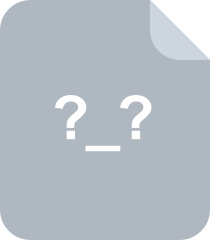
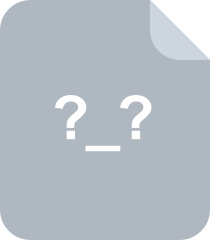
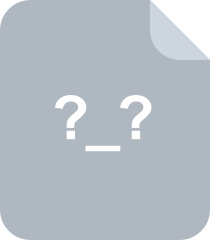
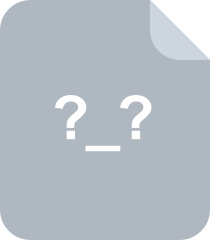
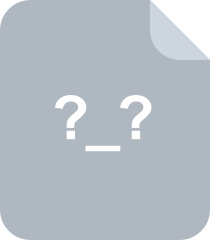
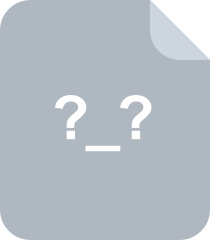
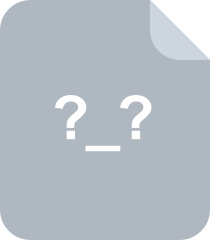
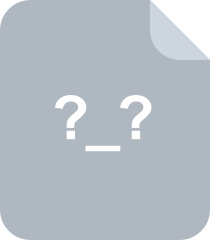
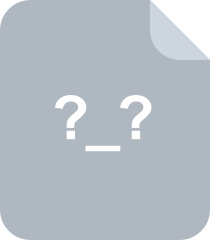
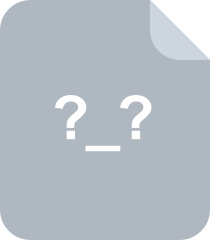
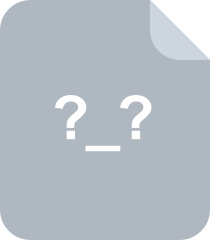
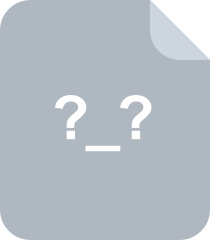
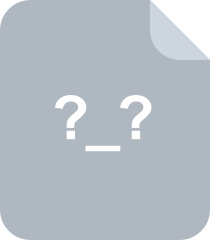
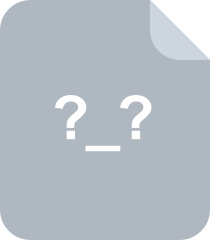
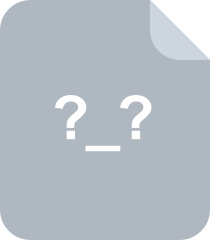
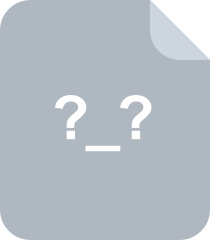
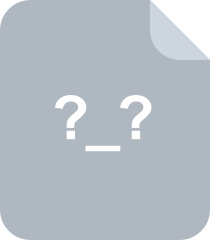
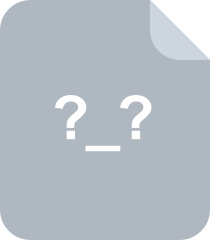
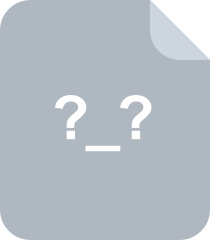
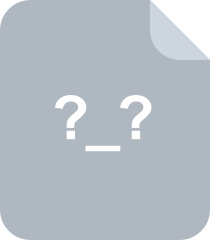
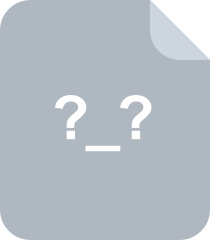
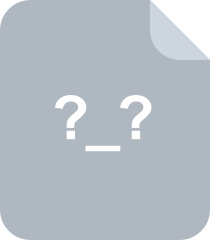
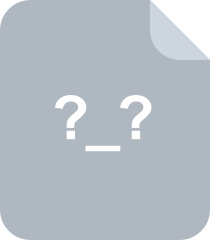
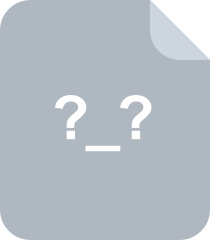
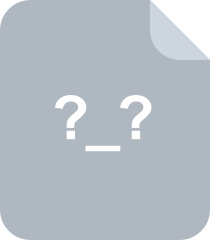
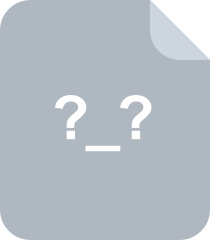
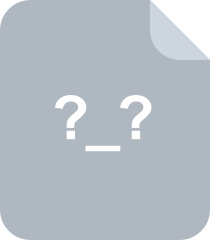
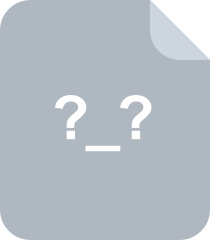
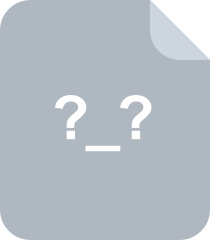
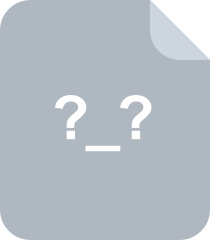
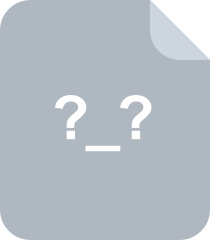
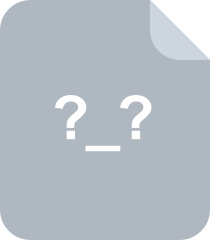
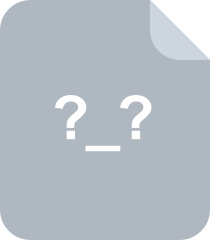
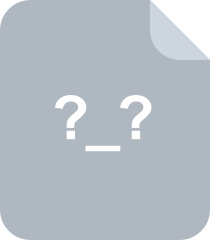
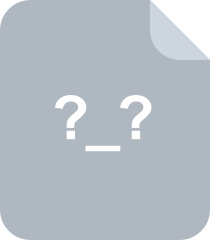
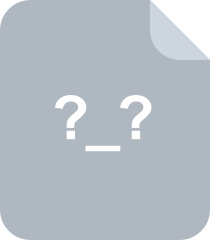
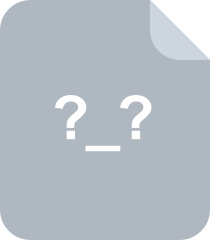
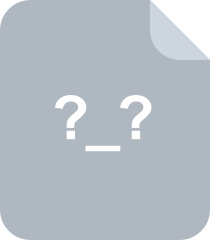
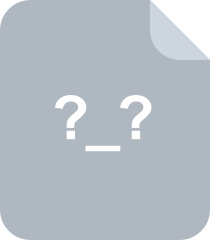
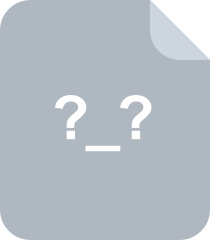
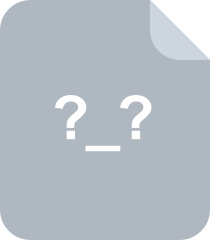
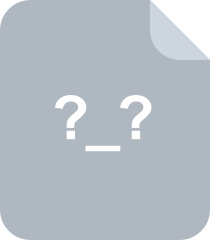
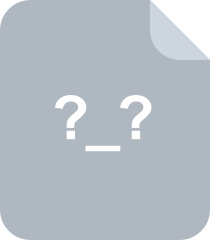
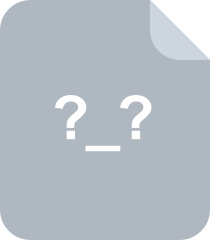
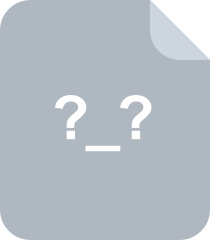
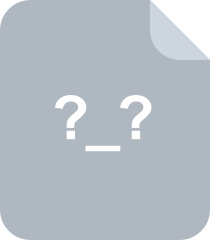
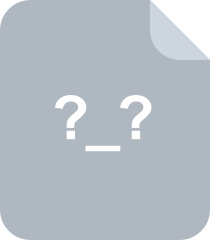
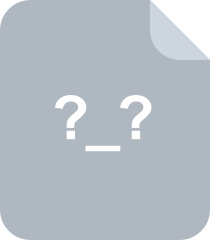
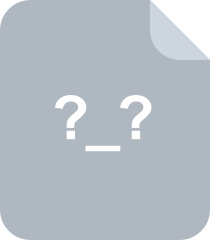
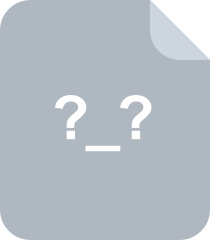
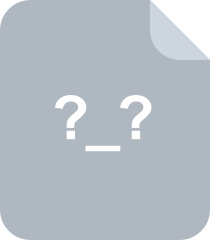
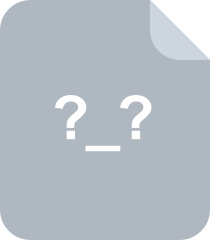
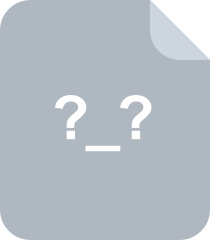
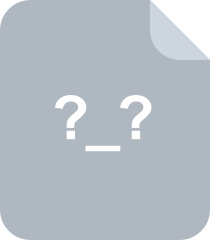
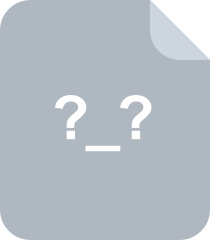
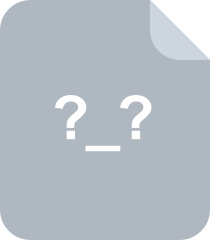
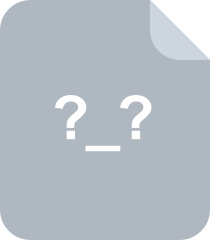
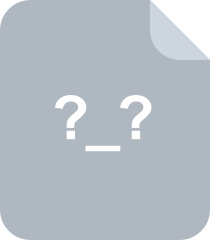
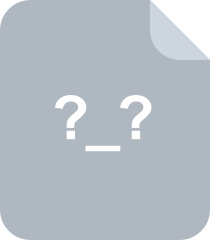
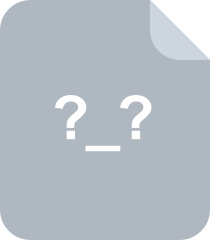
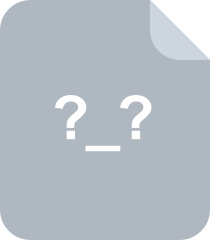
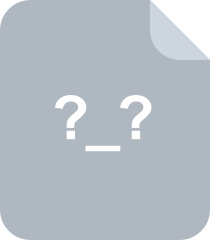
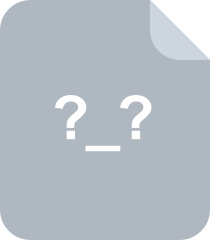
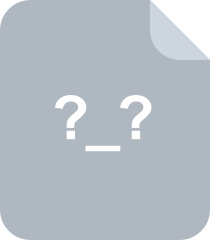
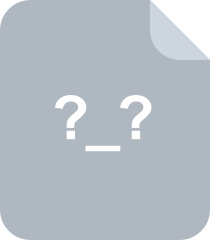
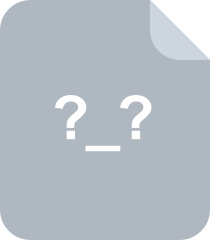
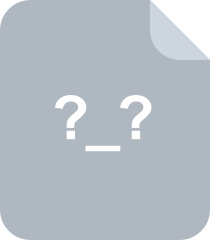
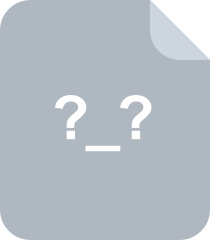
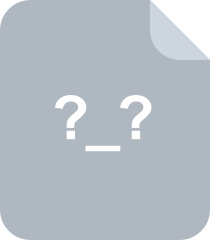
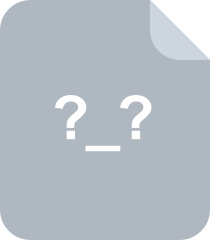
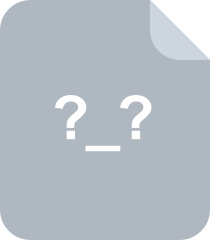
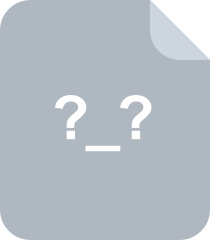
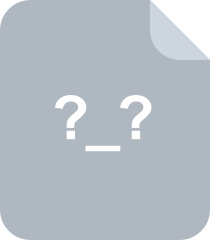
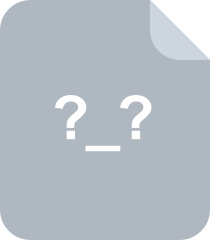
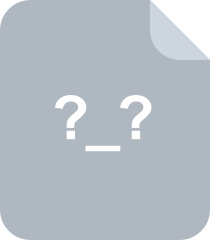
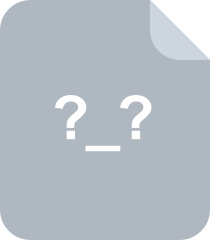
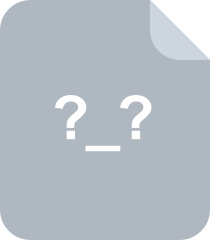
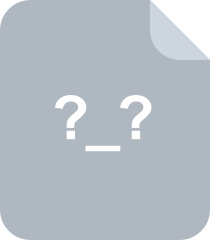
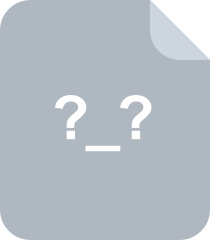
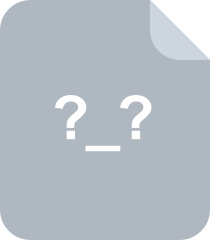
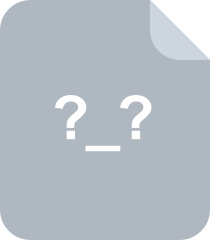
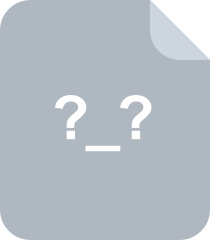
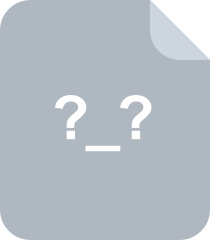
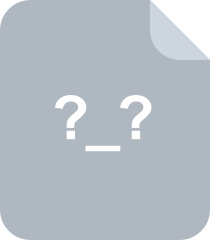
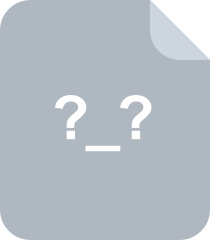
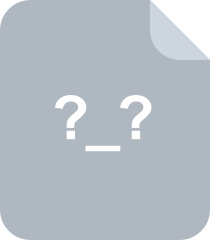
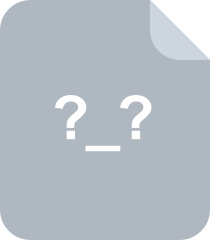
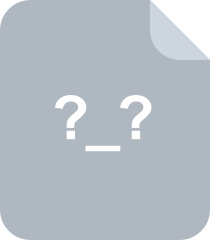
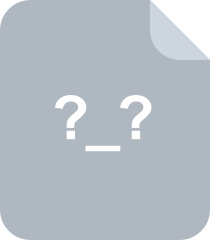
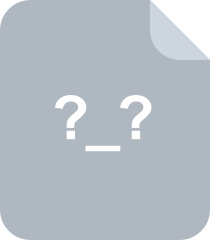
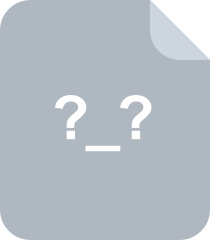
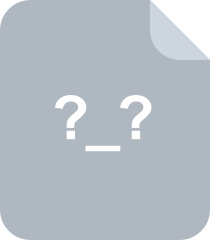
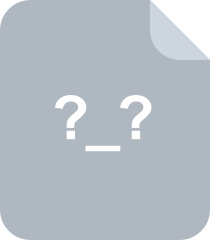
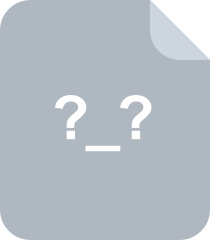
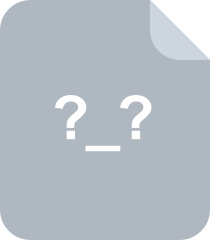
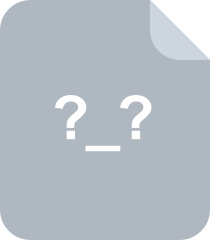
共 124 条
- 1
- 2
资源评论
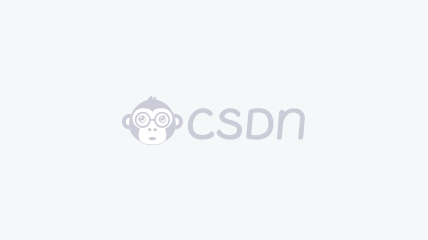

Beatfan_N
- 粉丝: 702
- 资源: 28
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

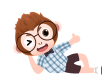
最新资源
- 线性块码实现汉明码(7,4)Matlab代码.rar
- 相位偏移键控调制 8PSK附matlab代码.rar
- 相移键控 8PSK 调制Matlab代码.rar
- 信道编码中使用的两种卷积码的误码率(BER)比较Matlab代码.rar
- 研究正交幅度调制(QAM)中的相位误差检测.rar
- 一个用于FSK调制和解调方案的MATLAB代码.rar
- 一个模拟Alamouti空间时间码的Matlab函数.rar
- 循环前缀和直接序列扩频用于BPSK、QPSK和16QAM调制Matlab代码.rar
- 选择性无线信道中模拟了OFDM系统。同时模拟了相干和非相干情况Matlab代码.rar
- 选择性无线信道中模拟了OFDM系统。同时模拟了相干和非相干情况Matlanb代码.rar
- 硬决策块码BPSK的BER曲线Matlab代码.rar
- 用于 ASK 调制和解调的 MATLAB 代码.rar
- 医学影像阅读器和查看器Matlab代码.rar
- 用于 BPSK、QPSK 和 16QAM 调制的直接序列扩频 (DSSS)Matlab代码.rar
- 用于 FSK 调制和解调的 MATLAB 代码.rar
- 用于 MIMO 仿真的空间信道模型。基于 3GPP TR 25.996 v.6.1.0 的基于 Ray 的模拟器Matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


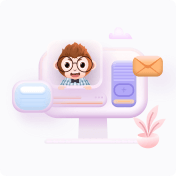
安全验证
文档复制为VIP权益,开通VIP直接复制
