#!/bin/bash
#####################################################################################################
# Script_Name : xrdp-installer-1.2.2.sh
# Description : Perform xRDP installation on Ubuntu 16.04,18.04,20.4,20.10 and perform
# additional post configuration to improve end user experience
# Date : December 2020
# written by : Griffon
# WebSite :http://www.c-nergy.be - http://www.c-nergy.be/blog
# Version : 1.2.2
# History : 1.2.2 - Changing Ubuntu repository from be.archive.ubuntu.com to archive.ubuntu.com
# - Bug Fixing - /etc/xrdp/xrdp-installer-check.log not deleted when remove option
# selected in the script - Force Deletion (Thanks to Hiero for this input)
# - Bug Fixing - Grab automatically xrdp/xorgxrdp package version to avoid
# issues when upgrade operation performed (Thanks to Hiero for this input)
# : 1.2.1 - Adding Support to Ubuntu 20.10 + Removed support for Ubuntu 19.10
# 1.2 - Adding Support to Ubuntu 20.04 + Removed support for Ubuntu 19.04
# - Stricter Check for HWE Package (thanks to Andrej Gantvorg)
# - Changed code in checking where to copy image for login screen customization
# - Fixed Bug checking SSL group membership
# - Updating background color xrdp login screen
# - Updating pkgversion to x.13 for checkinstall process
# : 1.1 - Tackling multiple run of the script
# - Improved checkinstall method/check ssl group memberhsip
# - Replaced ~/Downloads by a variable
# : 1.0 - Added remove option + Final optimization
# : 0.9 - updated compile section to use checkinstall
# : 0.8 - Updated the fix_theme function to add support for Ubuntu 16.04
# : 0.7 - Updated prereqs function to add support for Ubuntu 16.04
# : 0.6 - Adding logic to detect Ubuntu version for U16.04
# : 0.5 - Adding env variable Fix
# : 0.4 - Adding SSL Fix
# : 0.3 - Adding custom login screen option
# : 0.2 - Adding new code for passing parameters
# : 0.1 - Initial Script (merging custom & Std)
# Disclaimer : Script provided AS IS. Use it at your own risk....
# You can use this script and distribute it as long as credits are kept
# in place and unchanged
####################################################################################################
#--------------------------------------------------------------------------#
# -----------------------Function Section - DO NOT MODIFY -----------------#
#--------------------------------------------------------------------------#
############################################################################
# DEFAULT INSTALLATION MODE : STANDARD INSTALLATION
############################################################################
#---------------------------------------------------#
# Function 1 - check xserver-xorg-core package....
#---------------------------------------------------#
check_hwe()
{
Release=$(lsb_release -sr)
echo
/bin/echo -e "\e[1;33m |-| Detecting xserver-xorg-core package installed \e[0m"
xorg_no_hwe_install_status=$(dpkg-query -W -f ='${Status}\n' xserver-xorg-core 2>/dev/null)
xorg_hwe_install_status=$(dpkg-query -W -f ='${Status}\n' xserver-xorg-core-hwe-$Release 2>/dev/null)
if [[ "$xorg_hwe_install_status" =~ \ installed$ ]]
then
# – hwe version is installed on the system
/bin/echo -e "\e[1;32m |-| xorg package version: xserver-xorg-core-hwe \e[0m"
HWE="yes"
elif [[ "$xorg_no_hwe_install_status" =~ \ installed$ ]]
then
/bin/echo -e "\e[1;32m |-| xorg package version: xserver-xorg-core \e[0m"
HWE="no"
else
/bin/echo -e "\e[1;31m |-| Error checking xserver-xorg-core flavour \e[0m"
exit 1
fi
}
#---------------------------------------------------#
# Function 2 - Install xRDP Software....
#---------------------------------------------------#
install_xrdp()
{
echo
/bin/echo -e "\e[1;33m !---------------------------------------------!\e[0m"
/bin/echo -e "\e[1;33m ! Installing XRDP Packages...Proceeding... !\e[0m"
/bin/echo -e "\e[1;33m !---------------------------------------------!\e[0m"
echo
if [[ $HWE = "yes" ]] && [[ "$version" = *"Ubuntu 18.04"* ]];
then
sudo apt-get install xrdp -y
sudo apt-get install xorgxrdp-hwe-18.04
else
sudo apt-get install xrdp -y
fi
}
############################################################################
# ADVANCED INSTALLATION MODE : CUSTOM INSTALLATION
############################################################################
#---------------------------------------------------#
# Function 0 - Install Prereqs...
#---------------------------------------------------#
install_prereqs() {
echo
Release=$(lsb_release -sr)
/bin/echo -e "\e[1;33m !---------------------------------------------!\e[0m"
/bin/echo -e "\e[1;33m ! Installing PreReqs packages..Proceeding. ! \e[0m"
/bin/echo -e "\e[1;33m !---------------------------------------------!\e[0m"
echo
sudo apt-get -y install libx11-dev libxfixes-dev libssl-dev libpam0g-dev libtool libjpeg-dev flex bison gettext autoconf libxml-parser-perl libfuse-dev xsltproc libxrandr-dev python-libxml2 nasm fuse pkg-config git intltool checkinstall
echo
if [ $HWE = "yes" ];
then
# - xorg-hwe-* to be installed
/bin/echo -e "\e[1;32m |-| xorg package version: xserver-xorg-core-hwe-$Release \e[0m"
sudo apt-get install -y xserver-xorg-dev-hwe-$Release xserver-xorg-core-hwe-$Release
else
#-no-hwe
/bin/echo -e "\e[1;32m |-| xorg package version: xserver-xorg-core \e[0m"
echo
sudo apt-get install -y xserver-xorg-dev xserver-xorg-core
fi
}
#---------------------------------------------------#
# Function 1 - Download XRDP Binaries...
#---------------------------------------------------#
get_binaries() {
echo
/bin/echo -e "\e[1;33m !---------------------------------------------!\e[0m"
/bin/echo -e "\e[1;33m ! Download xRDP Binaries.......Proceeding. ! \e[0m"
/bin/echo -e "\e[1;33m !---------------------------------------------!\e[0m"
echo
#cd ~/Downloads
Dwnload=$(xdg-user-dir DOWNLOAD)
cd $Dwnload
## -- Download the xrdp latest files
echo
/bin/echo -e "\e[1;32m !---------------------------------------------!\e[0m"
/bin/echo -e "\e[1;32m ! Preparing download xrdp package !\e[0m"
/bin/echo -e "\e[1;32m !---------------------------------------------!\e[0m"
echo
git clone https://github.com/neutrinolabs/xrdp.git
echo
/bin/echo -e "\e[1;32m !---------------------------------------------!\e[0m"
/bin/echo -e "\e[1;32m ! Preparing download xorgxrdp package !\e[0m"
/bin/echo -e "\e[1;32m !---------------------------------------------!\e[0m"
echo
git clone https://github.com/neutrinolabs/xorgxrdp.git
}
#---------------------------------------------------#
# Function 2 - compiling xrdp...
#---------------------------------------------------#
compile_source() {
echo
/bin/echo -e "\e[1;33m !---------------------------------------------!\e[0m"
/bin/echo -e "\e[1;33m ! Compile xRDP packages .......Proceeding. !\e[0m"
/bin/echo -e "\e[1;33m !---------------------------------------------!\e[0m"
echo
#cd ~/Downloads/xrdp
cd $Dwnload/xrdp
#Get the release version automatically
pkgver=$(git describe --abbrev=0 --tags | cut -dv -f2)
sudo ./bootstrap
sudo ./configure --enable-fuse --enable-jpeg --enable-rfxcodec
sudo make
#-- check if no error during compilation
if [ $? -eq 0 ]
then
/bin/echo -e "\e[1;33m |-| Make Operation Completed successfully \e[0m"
else
echo
echo
/bin/echo -e "\e[1;31m !---------------------------------------------!\e[0m"
/bin/echo -e "\e[1;31m ! Error while Executing make !\e[0m"
/bin/echo -e "\e[1;31m ! The Script is exiting....

喝下影响力
- 粉丝: 3
- 资源: 32
最新资源
- 适用于 Android、Java 和 Kotlin Multiplatform 的现代 I,O 库 .zip
- 高通TWS蓝牙规格书,做HIFI级别的耳机用
- Qt读写Usb设备的数据
- 这个存储库适合初学者从 Scratch 开始学习 JavaScript.zip
- AUTOSAR 4.4.0版本Rte模块标准文档
- 25考研冲刺快速复习经验.pptx
- MATLAB使用教程-初步入门大全
- 该存储库旨在为 Web 上的语言提供新信息 .zip
- 考研冲刺的实用经验与技巧.pptx
- Nvidia GeForce GT 1030-GeForce Studio For Win10&Win11(Win10&Win11 GeForce GT 1030显卡驱动)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


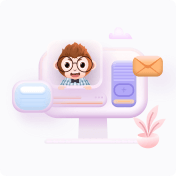
评论0