在Java开发中,XML(eXtensible Markup Language)是一种常用的数据交换格式,它具有良好的结构和可读性。为了方便地将XML数据与Java对象(POJO,Plain Old Java Object)之间进行转换,XStream库应运而生。本项目提供了一个完整的Java工程示例,演示了如何使用XStream实现XML到POJO以及POJO到XML的双向转化,并包含了必要的jar包和测试类,可以直接运行。 XStream是JVM平台上的一个开源库,由XStream Software公司开发,它通过简单的API将Java对象序列化为XML,同时也能将XML反序列化回Java对象。这个过程非常便捷,使得开发者可以快速地处理XML数据,而无需手动编写大量的XML解析代码。 以下是使用XStream解析XML和POJO双向转化的基本步骤: 1. 引入XStream库:在Java工程中,需要添加XStream的jar包,通常可以通过Maven或Gradle等构建工具管理依赖。在这个项目中,"lib"目录下应该包含XStream的jar文件。 2. 创建POJO类:根据XML数据的结构,定义对应的Java类。例如,如果XML文件有一个`employee`元素,那么可以创建一个`Employee`类,包含`name`、`age`等属性。 3. 初始化XStream实例:在代码中,首先需要创建一个XStream实例,可以设置命名空间、转换器等配置。 4. 序列化:将Java对象转化为XML字符串。使用`XStream.toXML()`方法,传入要转化的对象即可。 5. 反序列化:将XML字符串转化为Java对象。调用`XStream.fromXML()`方法,传入XML字符串,返回对应类型的Java对象。 6. 测试:在"src"目录下的测试类中,可以编写单元测试,验证XML到POJO和POJO到XML的转化是否正确。通常会使用JUnit或TestNG等测试框架。 在这个项目中,`.classpath`和`.project`文件是Eclipse或类似的IDE的工作区配置,它们定义了项目的构建路径和编译设置。`.settings`目录可能包含项目特定的Eclipse设置。"bin"目录通常是编译后的Java类文件所在的地方。 通过这个工程,开发者可以学习并实践XStream的使用,了解XML与Java对象之间的转化机制。同时,由于包含了测试类和所需jar包,可以直接运行和调试,这对于初学者或者需要快速实现XML处理功能的开发者来说,是一个非常实用的资源。
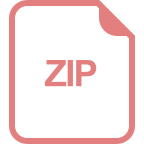
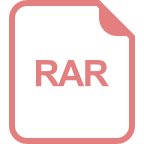
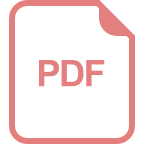
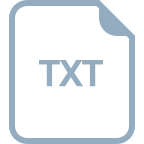
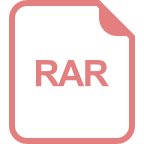
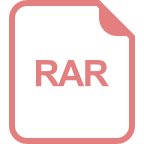
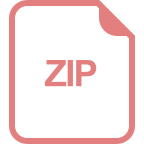
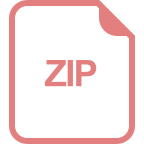
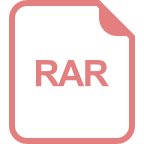
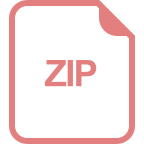
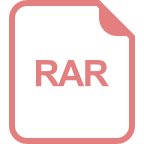
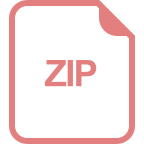
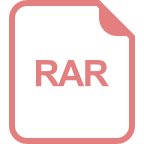
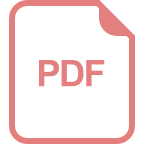
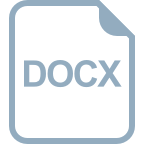
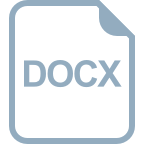
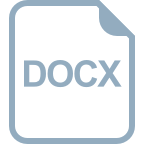

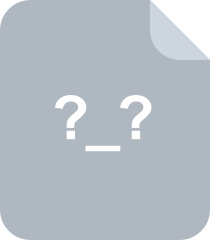

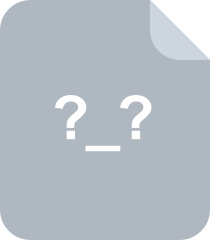
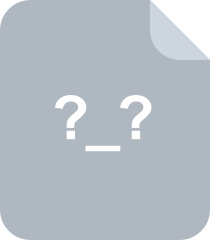
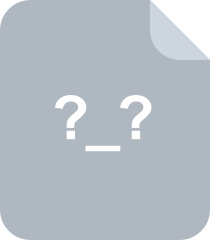

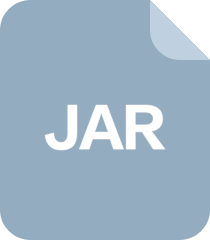
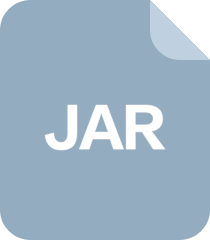
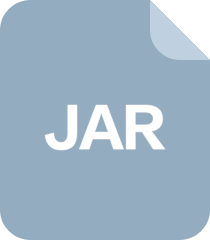

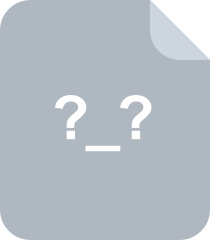
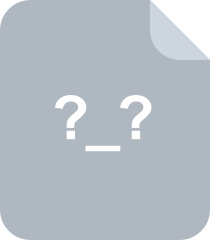

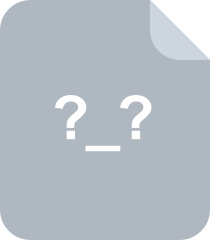
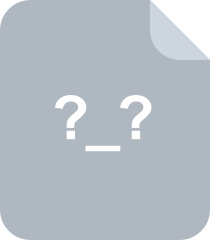
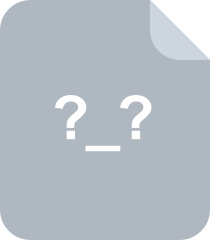
- 1
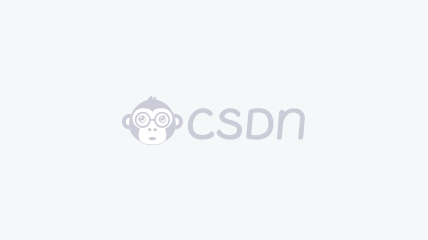
- yh_ma2013-11-18写得很一般。
- Earl_Lee2014-05-02还不错 给了我点儿思路
- antzou2014-10-08還行,可以用
- qyc31155352014-05-21借鉴了思路
- kenn06262013-11-16还不错。就是格式有点死板

- 粉丝: 7
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

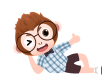
最新资源
- 橙色扁平风格的汽车经销商店企业网站源码下载.zip
- 橙色扁平风格的云端服务器租赁整站网站模板.zip
- 橙色扁平风格的图片日志信息源码下载.zip
- 基于ssm的数学课程评价系统的设计与开发源码(java毕业设计完整源码+LW).zip
- 橙色扁平风格的徒步旅行企业网站源码下载.zip
- 橙色扁平化风格的HTML商务人士网站模板.zip
- 橙色扁平风格的自行车运动企业网站源码下载.zip
- 橙色扁平风格的在线红酒电商网站源码下载.zip
- 橙色扁平化风格的咖啡休闲屋HTML网站模板.zip
- 橙色扁平化风格的军事新闻企业网站模板下载.zip
- 橙色扁平化风格的巴西特色美食模板下载.zip
- 橙色扁平化风格的企业公司模板下载.zip
- 橙色扁平化风格的农业致富项目网站模板下载.zip
- 橙色扁平化风格的商务活动模板下载.zip
- 橙色扁平化风格的托管提供商整站网站模板.zip
- 橙色扁平化风格的视差滚动设计公司模板.zip

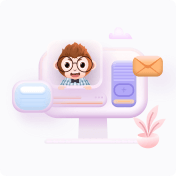
