/*
Note: Before getting started, Basler recommends reading the "Programmer's Guide" topic
in the pylon C++ API documentation delivered with pylon.
If you are upgrading to a higher major version of pylon, Basler also
strongly recommends reading the "Migrating from Previous Versions" topic in the pylon C++ API documentation.
This sample illustrates the use of a MFC GUI together with the pylon C++ API to enumerate the attached cameras, to
configure a camera, to start and stop the grab and to display and store grabbed images.
It shows how to use GUI controls to display and modify camera parameters.
*/
#include "stdafx.h"
#include "GuiSample.h"
#include "GuiSampleDoc.h"
#include "AutoPacketSizeConfiguration.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#endif
std::string GetTimeStr()
{
SYSTEMTIME st;
GetLocalTime(&st);
int ih = st.wHour;
int im = st.wMinute;
int is = st.wSecond;
int id = st.wDay;
int imo = st.wMonth;
int iy = st.wYear;
int iss = st.wMilliseconds;
char ctmp[256];
sprintf(ctmp, "%04d%02d%02d_%02d%02d%02d%_04d", iy, imo, id, ih, im, is, iss);
return std::string(ctmp);
}
// CGuiSampleDoc
IMPLEMENT_DYNCREATE(CGuiSampleDoc, CDocument)
BEGIN_MESSAGE_MAP(CGuiSampleDoc, CDocument)
ON_COMMAND(ID_CAMERA_GRABONE, &CGuiSampleDoc::OnGrabOne)
ON_UPDATE_COMMAND_UI(ID_CAMERA_GRABONE, &CGuiSampleDoc::OnUpdateGrabOne)
ON_COMMAND(ID_CAMERA_STARTGRABBING, &CGuiSampleDoc::OnStartGrabbing)
ON_UPDATE_COMMAND_UI(ID_CAMERA_STARTGRABBING, &CGuiSampleDoc::OnUpdateStartGrabbing)
ON_COMMAND(ID_CAMERA_STOPGRAB, &CGuiSampleDoc::OnStopGrab)
ON_UPDATE_COMMAND_UI(ID_CAMERA_STOPGRAB, &CGuiSampleDoc::OnUpdateStopGrab)
ON_COMMAND(ID_NEW_GRABRESULT, &CGuiSampleDoc::OnNewGrabresult)
ON_COMMAND(ID_VIEW_REFRESH, &CGuiSampleDoc::OnViewRefresh)
ON_COMMAND(ID_FILE_SAVE_AS, &CGuiSampleDoc::OnFileSaveAs)
ON_COMMAND(ID_FILE_IMAGE_SAVE_AS, &CGuiSampleDoc::OnFileImageSaveAs)
ON_UPDATE_COMMAND_UI(ID_FILE_IMAGE_SAVE_AS, &CGuiSampleDoc::OnUpdateFileImageSaveAs)
ON_COMMAND( ID_UPDATE_NODES, &CGuiSampleDoc::OnUpdateNodes )
END_MESSAGE_MAP()
// CGuiSampleDoc construction/destruction
CGuiSampleDoc::CGuiSampleDoc()
: m_cntGrabbedImages(0)
, m_cntSkippedImages(0)
, m_cntGrabErrors(0)
, m_hTestImage(NULL)
, m_hGain(NULL)
, m_hExposureTime(NULL)
{
// TODO: add one-time construction code here
}
CGuiSampleDoc::~CGuiSampleDoc()
{
}
// Initial one-time initialization
BOOL CGuiSampleDoc::OnNewDocument()
{
if (!CDocument::OnNewDocument())
return FALSE;
try
{
// Register this object as an image event handler, so we will be notified of new new images
// See Pylon::CImageEventHandler for details
m_camera.RegisterImageEventHandler(this, Pylon::RegistrationMode_ReplaceAll, Pylon::Ownership_ExternalOwnership);
// Register this object as a configuration event handler, so we will be notified of camera state changes.
// See Pylon::CConfigurationEventHandler for details
m_camera.RegisterConfiguration(this, Pylon::RegistrationMode_ReplaceAll, Pylon::Ownership_ExternalOwnership);
}
catch (const Pylon::GenericException& e)
{
TRACE(CUtf82W(e.what()));
return FALSE;
UNUSED(e);
}
return TRUE;
}
// Some features of USB cameras are of type float (i.e. Gain).
// To use the value in Windows controls, we need to convert it to an integer.
// This conversion can be done by the GenICam.
// All float features have a so-called "alias feature" representing the float value
// converted to an integer. This function retrieves the alias feature for the feature passed.
// If the feature passed is already of type integer, the function returns the
// value passed converted to IInteger.
// If the feature passed doesn't have an alias and is not an integer, the function returns an
// empty parameter.
Pylon::CIntegerParameter CGuiSampleDoc::GetIntegerParameter(GenApi::INode* pNode) const
{
Pylon::CFloatParameter parameterFloat(pNode);
Pylon::CIntegerParameter parameterInteger;
// Is this a float feature?
if (parameterFloat.IsValid())
{
// Get the alias.
// If it doesn't exist, return an empty parameter.
parameterFloat.GetAlternativeIntegerRepresentation(parameterInteger);
}
else
{
// Convert the node to an integer parameter.
// If conversion isn't possible, return an empty parameter.
parameterInteger.Attach(pNode);
}
return parameterInteger;
}
// Called when a node was possibly changed
void CGuiSampleDoc::OnNodeChanged(GenApi::INode* pNode)
{
if (pNode == NULL)
{
return;
}
// Uncomment the following line if you want to see which nodes are getting callbacks.
//TRACE(_T("Node changed: %s\n"), (LPCWSTR)CUtf82W(pNode->GetName().c_str()));
// Tell the document that some camera features must be updated.
CWnd* pWnd = AfxGetApp()->GetMainWnd();
// When the application shuts down the windows may already be gone.
if (pWnd != NULL)
{
// You must use PostMessage here to separate the grab thread from the GUI thread.
pWnd->PostMessage( WM_COMMAND, MAKEWPARAM( ID_UPDATE_NODES, 0 ), 0 );
}
}
// CGuiSampleDoc serialization
void CGuiSampleDoc::Serialize(CArchive& ar)
{
ASSERT(FALSE && "serialization is not supported");
}
// CGuiSampleDoc diagnostics
#ifdef _DEBUG
void CGuiSampleDoc::AssertValid() const
{
CDocument::AssertValid();
}
void CGuiSampleDoc::Dump(CDumpContext& dc) const
{
CDocument::Dump(dc);
}
#endif //_DEBUG
// This will be called by MFC before a new document is created
// or when the application is shutting down.
// Perform all cleanup here.
void CGuiSampleDoc::DeleteContents()
{
// Make sure the device is not grabbing.
OnStopGrab();
// Free the grab result, if present.
m_bitmapImage.Release();
m_ptrGrabResult.Release();
// Perform cleanup.
if (m_camera.IsPylonDeviceAttached())
{
try
{
// Deregister the node callbacks.
if (m_hExposureTime)
{
GenApi::Deregister( m_hExposureTime );
m_hExposureTime = NULL;
}
if (m_hGain)
{
GenApi::Deregister( m_hGain );
m_hGain = NULL;
}
if (m_hPixelFormat)
{
GenApi::Deregister( m_hPixelFormat );
m_hPixelFormat = NULL;
}
if (m_hTestImage)
{
GenApi::Deregister( m_hTestImage );
m_hTestImage = NULL;
}
// Clear the pointer to the features.
m_exposureTime.Release();
m_gain.Release();
m_testImage.Release();
m_pixelFormat.Release();
// Close camera.
// This will also stop the grab.
m_camera.Close();
// Free the camera.
// This will also stop the grab and close the camera.
m_camera.DestroyDevice();
// Tell all the views that there is no camera anymore.
UpdateAllViews(NULL, UpdateHint_All);
}
catch (const Pylon::GenericException& e)
{
TRACE(_T("Error during cleanup: %s"), (LPCWSTR)CUtf82W(e.what()) );
UNUSED(e);
}
}
// Call the base class.
CDocument::DeleteContents();
}
// Enumerate devices.
void CGuiSampleDoc::OnViewRefresh()
{
try
{
// Refresh the list of all attached cameras.
theApp.EnumerateDevices();
// Update the GUI.
// Always update the device list and the
没有合适的资源?快使用搜索试试~ 我知道了~
基于basler巴斯勒工业相机驱动5.2内的C++ MFC的demo,在连续采集图像时,加入了本地存储环节,保存了时间戳
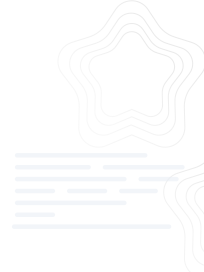
共78个文件
tlog:19个
h:9个
cpp:7个

需积分: 3 0 下载量 32 浏览量
2024-06-14
14:23:18
上传
评论
收藏 30.19MB ZIP 举报
温馨提示
主要用于工业相机保存图像,实现存储时间戳的功能,配合demo自带存储功能,实现实时采集的图像存储,所见即所得,所得即时图像。 可根据需要修改代码,调整存储格式,demo支持bmp、tiff、jpeg、png、raw; 可以根据需要,修改存储间隔、存储时长,配合自带相机驱动调节帧率,设置自动存储;定时存储等功能。
资源推荐
资源详情
资源评论
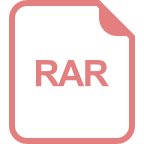
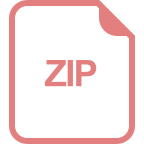
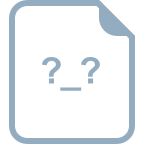
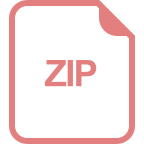
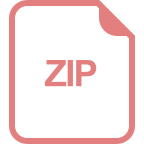
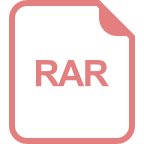
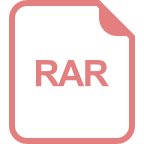
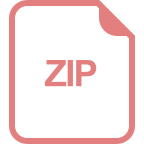
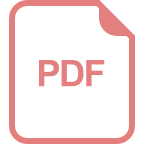
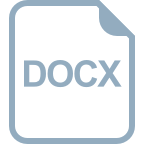
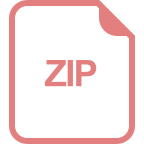
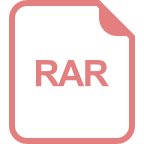
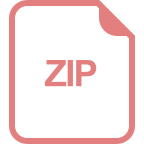
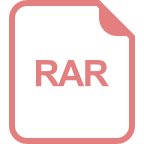
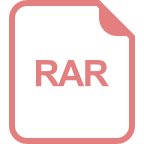
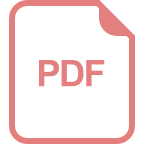
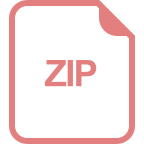
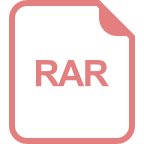
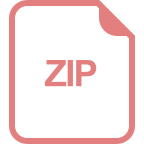
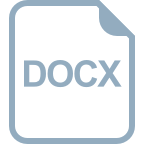
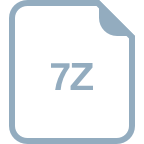
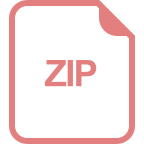
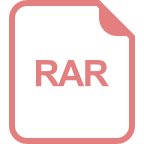
收起资源包目录


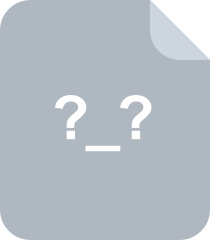
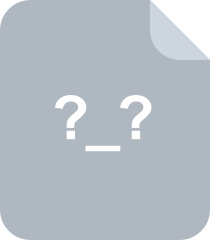
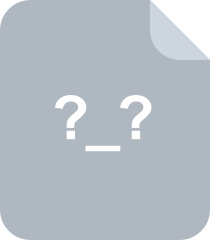
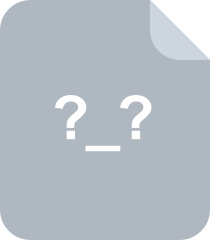
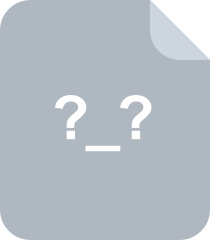
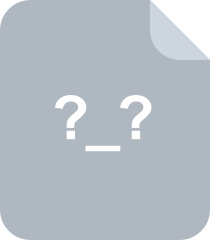
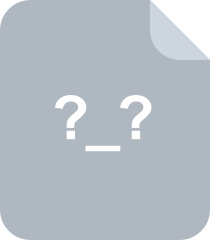
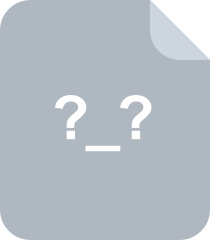
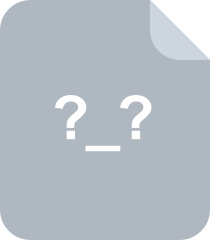
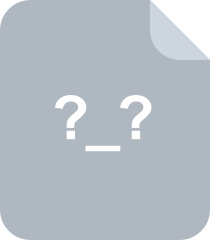
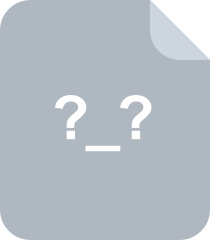
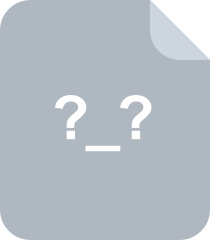
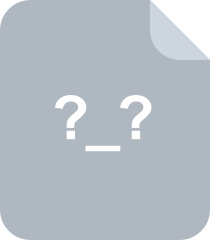

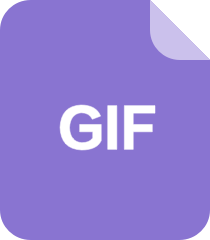
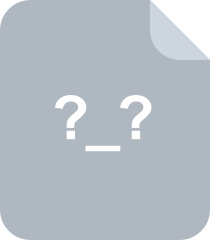
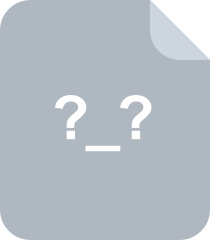
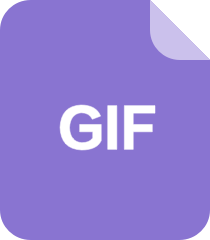

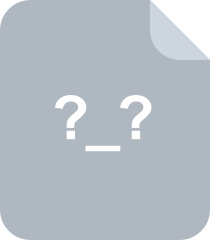
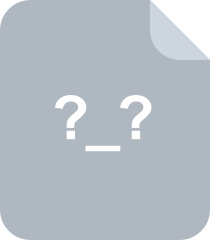
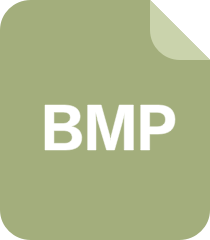
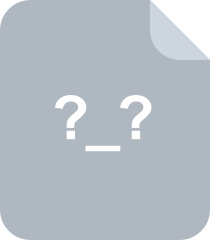
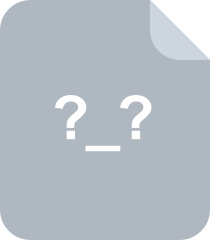
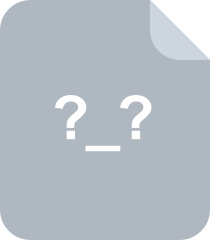
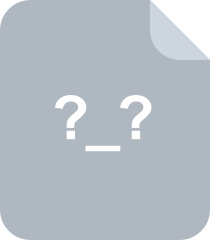
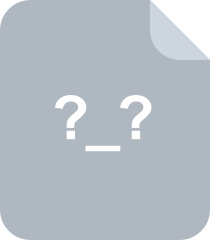
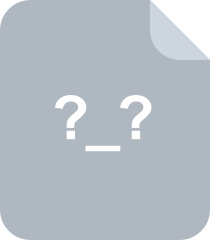
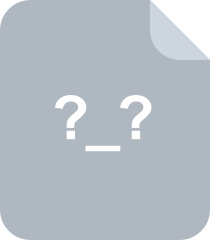
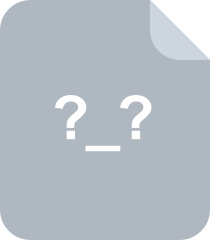
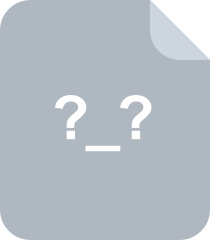


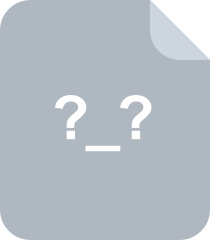
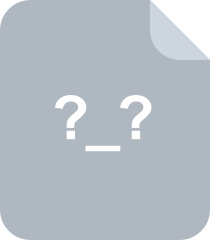
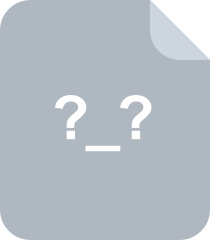
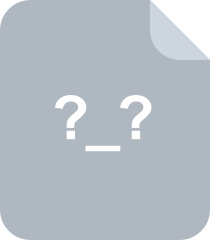
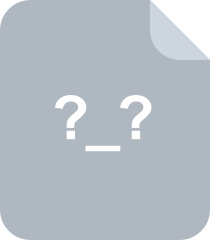
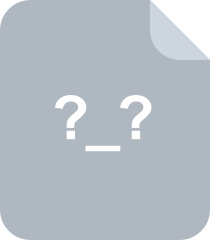
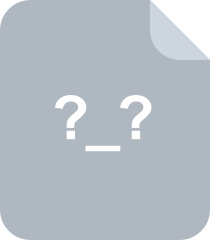

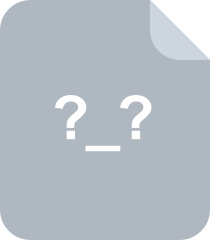
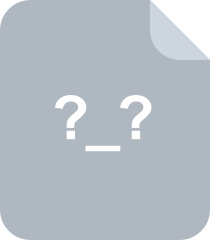
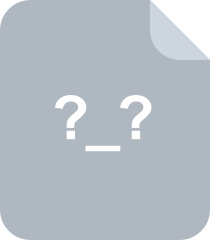
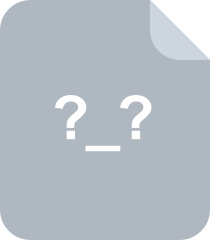
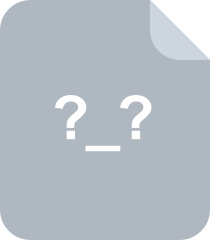
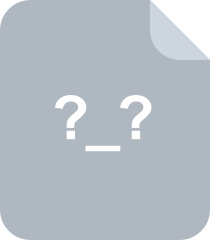
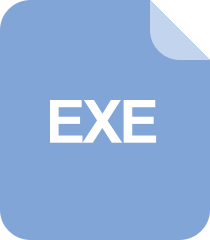
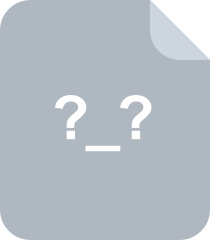
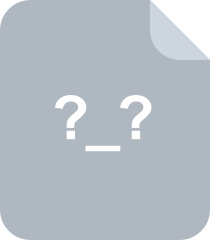
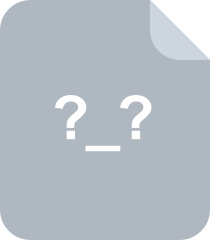
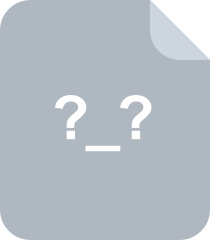
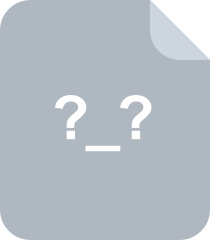
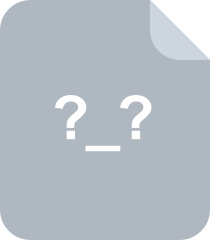
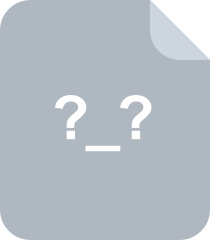
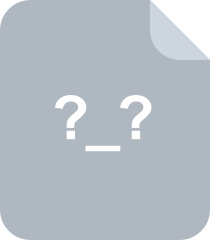
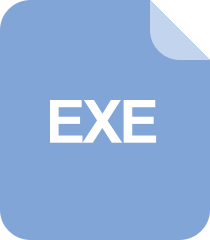
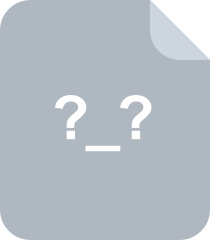
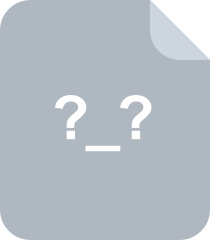
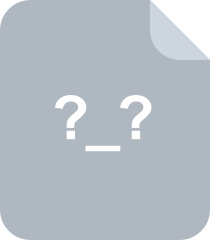
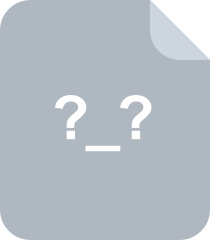
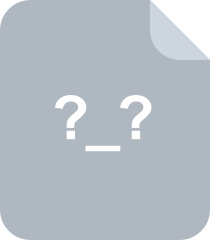
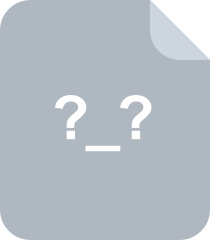
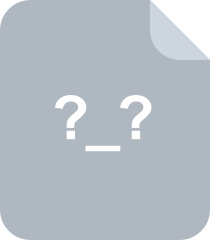
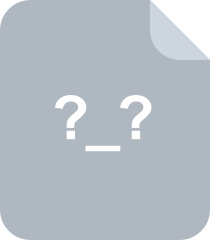
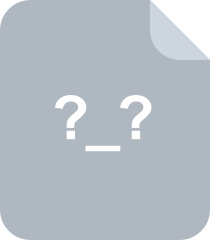
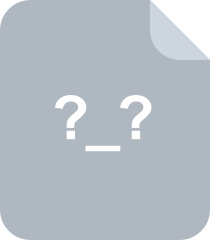
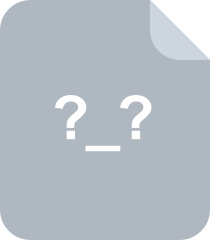
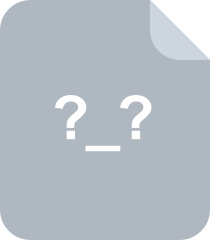
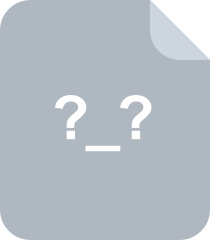
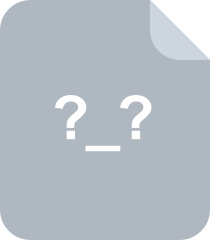
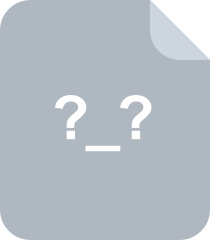
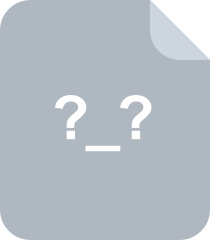
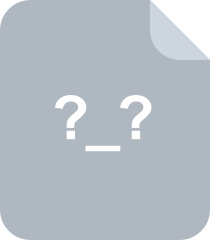
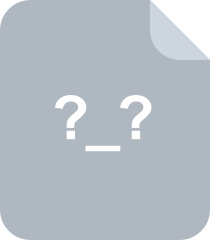
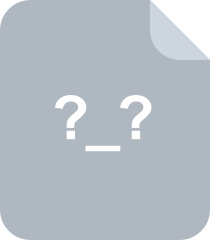
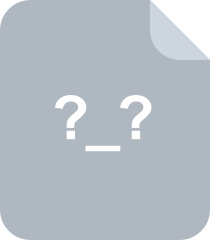
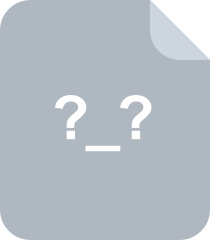
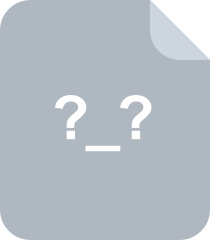
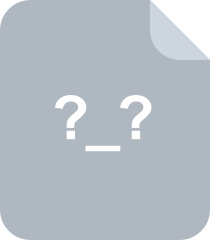
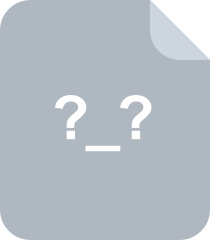
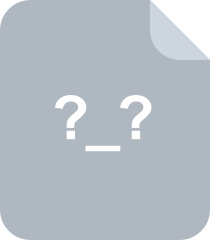
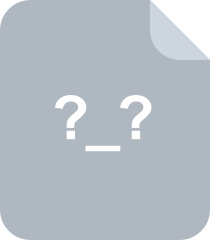
共 78 条
- 1
资源评论
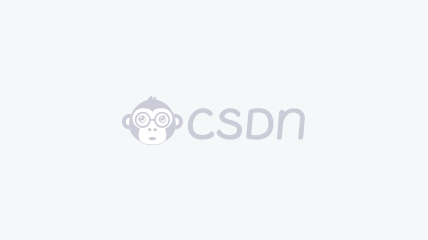

BFSTL
- 粉丝: 81
- 资源: 57
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

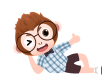
最新资源
- 55555555555555555555555555555
- 三菱旋切飞剪,用的是运动控制器Q172DSCPU做的飞剪控制,凸轮曲线的由来是分析计算出来的 其中文件是一个程序+一个文档说明
- java基于ssm+vue游泳会员管理系统源码 带毕业论文
- mqttfx-5.3.0-windows-x64
- gitlab搭建与日常使用
- 【最新python必过毕设选题推荐】基于python+Django的电影数据爬取与数据分析(包含源码+万字LW+答辩PPT
- tts-vue-main
- 发那科FANUC电路板图纸 全套驱动图纸 原理图 电源图,维修人员必备电路图
- 西门子SMART200程序 PID的控制写法,突破8路,PID直接做成子程序,无密码,直接调用
- java基于ssm+vue物流配送人员车辆调度管理系统源码 带毕业论文
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


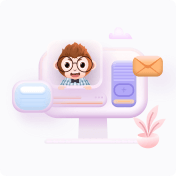
安全验证
文档复制为VIP权益,开通VIP直接复制
