RC4是一种流式加密算法,由Ron Rivest在1987年设计,因其简单、高效而被广泛应用。在.NET框架中,虽然没有内置的RC4类,但我们可以使用System.Security.Cryptography命名空间中的SymmetricAlgorithm类作为基础,自定义一个RC4加密解密的实现。以下是对`c#实现Rc4加密`这一主题的详细解析。 RC4算法的核心在于两个主要步骤:Key Scheduling Algorithm (KSA) 和 Pseudo-Random Generation Algorithm (PRGA)。KSA用于初始化状态数组S,而PRGA则根据S生成伪随机序列,用于加密或解密数据。 在C#中,我们首先需要创建一个继承自SymmetricAlgorithm的类,例如命名为RC4Cipher。在这个类中,我们需要实现几个关键方法: 1. **Initialize**: 这是初始化算法的关键,其中包含KSA。在这个方法中,我们将密钥转换为一个特定长度的数组(RC4通常使用256位),并填充状态数组S。 2. **GenerateIV**: RC4不使用初始化向量(IV),所以这个方法可以返回一个空数组。 3. **TransformBlock/TransformFinalBlock**: 这两个方法实现了PRGA。每次调用时,它们会生成新的伪随机字节,与明文或密文进行异或操作,从而完成加密或解密。 以下是一个简单的RC4Cipher类实现示例: ```csharp using System; using System.IO; using System.Security.Cryptography; public class RC4Cipher : SymmetricAlgorithm { private byte[] key; private byte[] s; public RC4Cipher(byte[] key) { KeySizeValue = 256; BlockSizeValue = 8; FeedbackSizeValue = 8; Mode = CipherMode.ECB; Padding = PaddingMode.None; this.key = key; Initialize(); } protected override void Dispose(bool disposing) { if (disposing) { if (s != null) { Array.Clear(s, 0, s.Length); s = null; } } base.Dispose(disposing); } public override ICryptoTransform CreateEncryptor(byte[] rgbKey, byte[] rgbIV) { return new RC4Transform(this, true); } public override ICryptoTransform CreateDecryptor(byte[] rgbKey, byte[] rgbIV) { return new RC4Transform(this, false); } public override void GenerateIV() { } private void Initialize() { // KSA int j = 0; s = new byte[256]; for (int i = 0; i < 256; i++) { s[i] = (byte)i; } for (int i = 0; i < 256; i++) { j = (j + s[i] + key[i % key.Length]) & 0xFF; Swap(s, i, j); } } private static void Swap(byte[] arr, int i, int j) { byte temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } private class RC4Transform : ICryptoTransform { private RC4Cipher cipher; private bool encrypting; private int index1 = 0; private int index2 = 0; public RC4Transform(RC4Cipher cipher, bool encrypting) { this.cipher = cipher; this.encrypting = encrypting; } public override bool CanReuseTransform => false; public override bool CanTransformMultipleBlocks => true; public override int InputBlockSize => 1; public override int OutputBlockSize => 1; public override int TransformBlock(byte[] inputBuffer, int inputOffset, int inputCount, byte[] outputBuffer, int outputOffset) { for (int i = 0; i < inputCount; i++) { index1 = (index1 + 1) & 0xFF; index2 = (index2 + s[index1]) & 0xFF; Swap(cipher.s, index1, index2); int t = cipher.s[(cipher.s[index1] + cipher.s[index2]) & 0xFF]; if (encrypting) outputBuffer[outputOffset + i] = (byte)(inputBuffer[inputOffset + i] ^ t); else outputBuffer[outputOffset + i] = (byte)(inputBuffer[inputOffset + i] ^ cipher.s[t]); } return inputCount; } public override byte[] TransformFinalBlock(byte[] inputBuffer, int inputOffset, int inputCount) { var result = new byte[inputCount]; TransformBlock(inputBuffer, inputOffset, inputCount, result, 0); return result; } } } ``` 使用这个类进行加密和解密操作就像使用其他.NET加密类一样: ```csharp byte[] key = Encoding.ASCII.GetBytes("密钥"); byte[] data = File.ReadAllBytes("data.txt"); using (var rc4 = new RC4Cipher(key)) { byte[] encryptedData = rc4.CreateEncryptor().TransformFinalBlock(data, 0, data.Length); File.WriteAllBytes("encrypted_data.txt", encryptedData); byte[] decryptedData = rc4.CreateDecryptor().TransformFinalBlock(encryptedData, 0, encryptedData.Length); File.WriteAllBytes("decrypted_data.txt", decryptedData); } ``` 请注意,RC4的安全性已经受到质疑,特别是在某些特定的攻击场景下。现代的应用场景更倾向于使用更安全的算法,如AES。然而,对于学习和理解加密算法,RC4仍然是一个很好的实例。
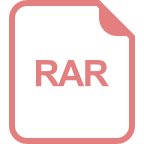
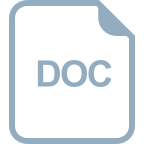
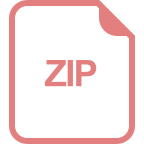
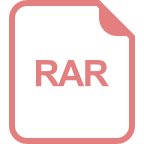
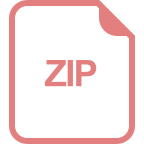
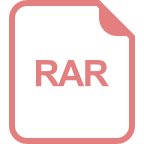
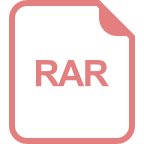
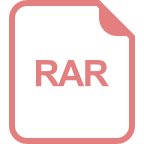
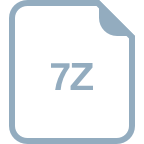
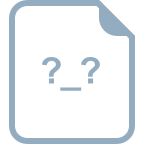
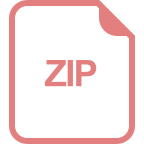
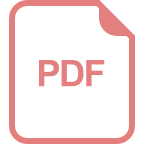
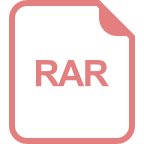
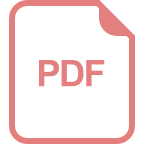
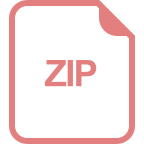
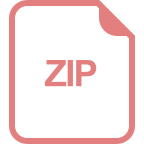

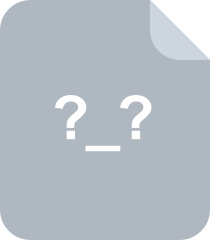
- 1
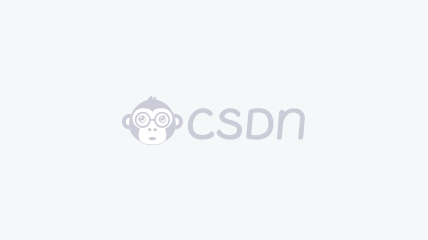

- 粉丝: 3
- 资源: 16
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

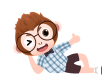
最新资源

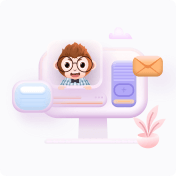
