在Java编程环境中,控制Windows操作系统中的鼠标和键盘是一项常见的需求,尤其在自动化测试、远程桌面控制或者模拟用户交互等场景中。本项目名为"java控制windows鼠标键盘",旨在提供一个工具箱,允许开发者通过调用内部代码来实现对Windows系统鼠标和键盘的精确控制。 在Java中,我们可以使用Java AWT(Abstract Window Toolkit)和SWT(Standard Widget Toolkit)库来实现这些功能。AWT提供了诸如`Robot`类,它允许我们模拟鼠标和键盘事件。例如,`Robot.createRobot()`方法用于创建一个Robot实例,之后可以调用`mouseMove(int x, int y)`来移动鼠标,`mousePress(int buttonMask)`和`mouseRelease(int buttonMask)`来模拟鼠标点击,以及`keyPress(int keyCode)`和`keyRelease(int keyCode)`来模拟键盘按键。 SWT则更接近于原生的窗口和控件操作,适用于需要更高精度或与GUI组件交互的情况。SWT提供了`Display`和`Shell`对象,以及各种`Widget`,如`Button`, `Text`等,它们都有对应的事件处理机制,可以通过监听和响应这些事件来实现键盘和鼠标的控制。 在"Touch 控制鼠标 键盘 控制"这个标签中,我们可以理解到项目可能还支持触摸屏设备的控制。在Windows环境下,Java可以通过`WinAPI`或者`JNA (Java Native Access)`库来访问底层系统API,从而实现对触摸事件的支持。例如,通过JNA调用Windows的`GetMessage`和`TranslateMessage`函数,可以捕获并处理触摸输入。 在压缩包文件"TouchBox"中,可能包含了项目的主要源代码和资源文件。通常,这样的项目会有一个主程序类,负责初始化和运行整个工具箱。此外,还可能包含多个子类或接口,分别实现鼠标和键盘的控制逻辑,以及触摸事件的处理。项目的结构可能如下: 1. 主程序类(如`TouchBoxMain.java`):启动点,初始化并加载所有功能模块。 2. 鼠标控制类(如`MouseController.java`):实现`Robot`类的功能,提供API供其他部分调用。 3. 键盘控制类(如`KeyboardController.java`):同样基于`Robot`类,实现键盘输入的模拟。 4. 触摸事件处理器(如`TouchEventHandler.java`):使用JNA或其它方式处理触摸屏输入。 5. 工具箱接口(如`Toolbox.java`):定义了控制键盘、鼠标和触摸的基本方法,供外部调用。 这个项目可能会提供一个图形化界面,用户可以直接在界面上选择执行相应的控制操作,也可以通过编写代码调用工具箱提供的API,实现自动化控制。 "java控制windows鼠标键盘"项目结合了Java的AWT/SWT库以及可能的WinAPI/JNA技术,为开发者提供了一个全面的解决方案,用于在Windows环境下实现鼠标、键盘和触摸设备的控制。通过这个工具箱,开发者可以轻松地进行自动化测试、用户界面模拟或其他需要交互控制的场景。
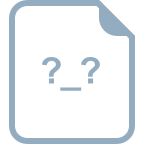
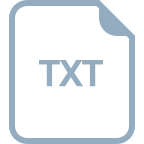
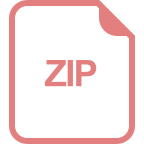
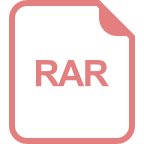
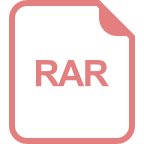
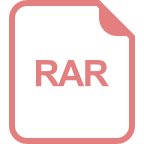
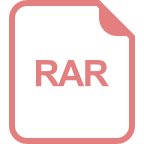
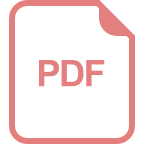
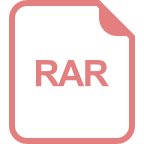
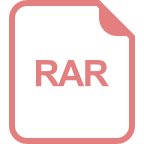
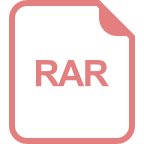
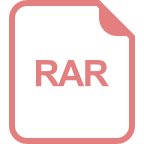
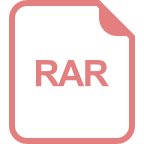


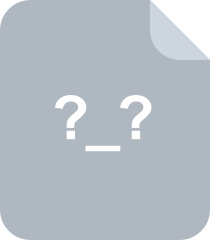


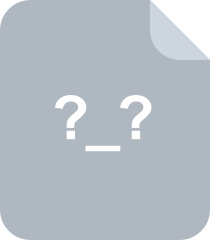
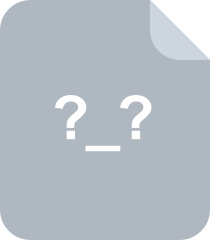
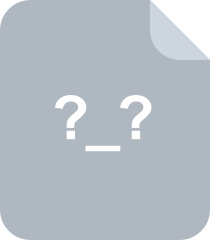
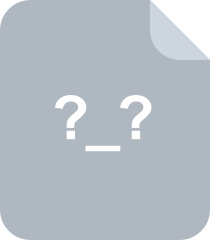
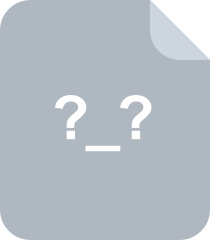

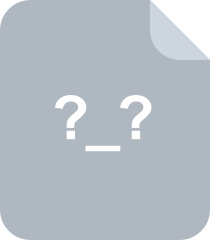

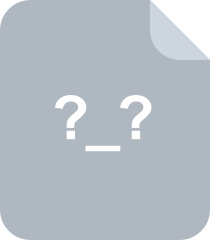
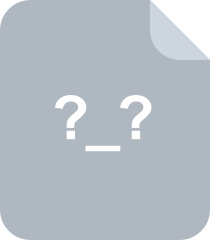


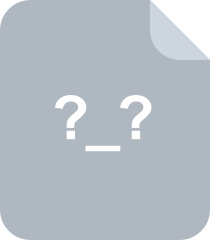
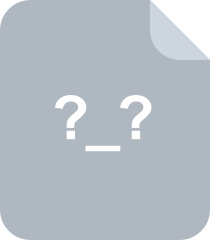
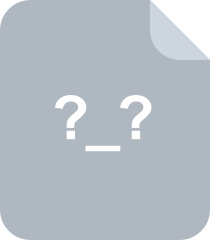
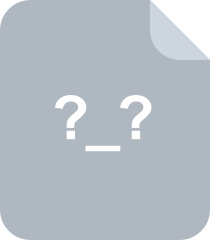
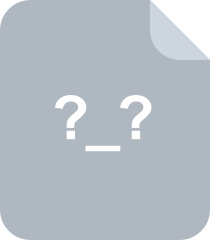

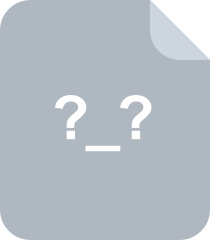
- 1
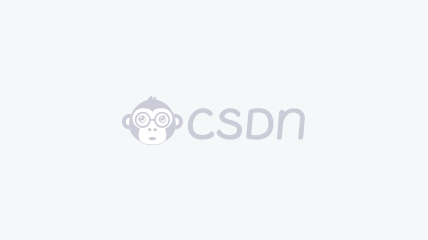
- zhangxiaoqi1990110722018-10-01有些帮助的
- cainiaoliulanggou2014-12-22没有看太懂

- 粉丝: 24
- 资源: 8
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

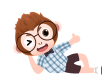
最新资源
- C语言-leetcode题解之70-climbing-stairs.c
- C语言-leetcode题解之68-text-justification.c
- C语言-leetcode题解之66-plus-one.c
- C语言-leetcode题解之64-minimum-path-sum.c
- C语言-leetcode题解之63-unique-paths-ii.c
- C语言-leetcode题解之62-unique-paths.c
- C语言-leetcode题解之61-rotate-list.c
- C语言-leetcode题解之59-spiral-matrix-ii.c
- C语言-leetcode题解之58-length-of-last-word.c
- 计算机编程课程设计基础教程

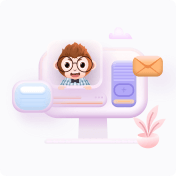
