可用于STM开发基AD转换的C语言程序 Includes #include "stm8l15x adc h" @addtogroup STM8L15x StdPeriph Driver @{ Private typedef Private define Private macro Private variables Private function prototypes Private functions @addtogroup ADC Public Functions @{ @brief Deinitializes the ADC peripheral registers to their default reset values @param ADCx where x can be 1 to select the specified ADC peripheral @retval None void ADC DeInit ADC TypeDef ADCx { ADCx >CR1 ADC CR1 RESET VALUE; ADCx >CR2 ADC CR2 RESET VALUE; ADCx >CR3 ADC CR3 RESET VALUE; ADCx >SR uint8 t ADC SR RESET VALUE; ADCx >HTRH ADC HTRH RESET VALUE; ADCx >HTRL ADC HTRL RESET VALUE; ADCx >LTRH ADC LTRH RESET VALUE; ADCx >LTRL ADC LTRL RESET VALUE; ADCx >SQR[0] ADC SQR1 RESET VALUE; ADCx >SQR[1] ADC SQR2 RESET VALUE; ADCx >SQR[2] ADC SQR3 RESET VALUE; ADCx >SQR[3] ADC SQR4 RESET VALUE; ADCx >TRIGR[0] ADC TRIGR1 RESET VALUE; ADCx >TRIGR[1] ADC TRIGR2 RESET VALUE; ADCx >TRIGR[2] ADC TRIGR3 RESET VALUE; ADCx >TRIGR[3] ADC TRIGR4 RESET VALUE; } @brief Initializes the specified ADC peripheral according to the specified parameters @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC ConversionMode : specifies the ADC conversion mode This parameter can be one of the @ref ADC ConversionMode TypeDef @param ADC Resolution : specifies the ADC Data resolusion This parameter can be one of the @ref ADC Resolution TypeDef @param ADC Prescaler : specifies the ADC Prescaler This parameter can be one of the @ref ADC Prescaler TypeDef @retval None void ADC Init ADC TypeDef ADCx ADC ConversionMode TypeDef ADC ConversionMode ADC Resolution TypeDef ADC Resolution ADC Prescaler TypeDef ADC Prescaler { Check the parameters assert param IS ADC CONVERSION MODE ADC ConversionMode ; assert param IS ADC RESOLUTION ADC Resolution ; assert param IS ADC PRESCALER ADC Prescaler ; clear CR1 register ADCx >CR1 & uint8 t ADC CR1 CONT | ADC CR1 RES ; set the resolution and the conversion mode ADCx >CR1 | uint8 t ADC ConversionMode | ADC Resolution ; clear CR2 register ADCx >CR2 & uint8 t ADC CR2 PRESC ; set the Prescaler ADCx >CR2 | uint8 t ADC Prescaler; } @brief Enables or disables the selected ADC channel s @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC Channels: specifies the ADC channels to be initialized This parameter can be a value of @ref ADC Channel TypeDef or a combination of values as follows: 1st combination : channels from ADC Channel 0 to ADC Channel 7 2nd combination : channels from ADC Channel 8 to ADC Channel 15 3rd combination : channels from ADC Channel 16 to ADC Channel 23 @param NewState : new state of the specified ADC channel s This parameter can be: ENABLE or DISABLE @retval None void ADC ChannelCmd ADC TypeDef ADCx ADC Channel TypeDef ADC Channels FunctionalState NewState { uint8 t regindex 0; Check the parameters assert param IS FUNCTIONAL STATE NewState ; regindex uint8 t ADC Channels >> 8 ; if NewState DISABLE { ADCx >SQR[regindex] | uint8 t ADC Channels ; } else { ADCx >SQR[regindex] & uint8 t ADC Channels ; } } @brief Enables or disables the selected ADC peripheral @param ADCx where x can be 1 to select the specified ADC peripheral @param NewState : new state of the specified ADC peripheral This parameter can be: ENABLE or DISABLE @retval None void ADC Cmd ADC TypeDef ADCx FunctionalState NewState { Check the parameters assert param IS FUNCTIONAL STATE NewState ; if NewState DISABLE { Set the ADON bit to wake up the specified ADC from power down mode ADCx >CR1 | ADC CR1 ADON; } else { Disable the selected ADC peripheral ADCx >CR1 & uint8 t ADC CR1 ADON; } } @brief Enables or disables the specified ADC interrupts @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC IT : specifies the ADC interrupt sources to be enabled or disabled This parameter can be any combination of the @ref ADC IT TypeDef @param NewState : new state of the specified ADC interrupts This parameter can be: ENABLE or DISABLE @retval None void ADC ITConfig ADC TypeDef ADCx ADC IT TypeDef ADC IT FunctionalState NewState { Check the parameters assert param IS FUNCTIONAL STATE NewState ; assert param IS ADC IT ADC IT ; if NewState DISABLE { Enable the selected ADC interrupts ADCx >CR1 | uint8 t ADC IT; } else { Disable the selected ADC interrupts ADCx >CR1 & uint8 t ADC IT ; } } @brief Enables or disables the specified ADC DMA request @param ADCx where x can be 1 to select the specified ADC peripheral @param NewState : new state of the specified ADC DMA transfer This parameter can be: ENABLE or DISABLE @retval None void ADC DMACmd ADC TypeDef ADCx FunctionalState NewState { Check the parameters assert param IS FUNCTIONAL STATE NewState ; if NewState DISABLE { Enable the specified ADC DMA request ADCx >SQR[0] & uint8 t ADC SQR1 DMAOFF; } else { Disable the specified ADC DMA request ADCx >SQR[0] | ADC SQR1 DMAOFF; } } @brief Enables or disables the Temperature sensor internal reference @param NewState : new state of the Temperature sensor internal reference This parameter can be: ENABLE or DISABLE @retval None void ADC TempSensorCmd FunctionalState NewState { Check the parameters assert param IS FUNCTIONAL STATE NewState ; if NewState DISABLE { ADC1 >TRIGR[0] | uint8 t ADC TRIGR1 TSON ; } else { ADC1 >TRIGR[0] & uint8 t ADC TRIGR1 TSON ; } } @brief Enables or disables the Internal Voltage reference @param NewState : new state of the Internal Voltage reference This parameter can be: ENABLE or DISABLE @retval None void ADC VrefintCmd FunctionalState NewState { Check the parameters assert param IS FUNCTIONAL STATE NewState ; if NewState DISABLE { ADC1 >TRIGR[0] | uint8 t ADC TRIGR1 VREFINTON ; } else { ADC1 >TRIGR[0] & uint8 t ADC TRIGR1 VREFINTON ; } } @brief Starts ADC conversion by software trigger @param ADCx where x can be 1 to select the specified ADC peripheral @retval None void ADC SoftwareStartConv ADC TypeDef ADCx { Start the ADC software conversion ADCx >CR1 | ADC CR1 START; } @brief Configures the sampling time for the selected ADC channel group @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC GroupChannels : ADC channel group to configure This parameter can be a value of @ref ADC Group TypeDef @param ADC SamplingTimeCycles : Specifies the sample time value This parameter can be a value of @ref ADC SamplingTime TypeDef @retval None void ADC SamplingTimeConfig ADC TypeDef ADCx ADC Group TypeDef ADC GroupChannels ADC SamplingTime TypeDef ADC SamplingTimeCycles { Check the parameters assert param IS ADC GROUP ADC GroupChannels ; assert param IS ADC SAMPLING TIME CYCLES ADC SamplingTimeCycles ; if ADC GroupChannels ADC Group SlowChannels { ADCx >CR3 & uint8 t ADC CR3 SMPT2; ADCx >CR3 | uint8 t ADC SamplingTimeCycles << 5 ; } else { ADCx >CR2 & uint8 t ADC CR2 SMPT1; ADCx >CR2 | uint8 t ADC SamplingTimeCycles; } } @brief Configures the status of the Schmitt Trigger for the selected ADC channel s @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC Channels: specifies the ADC channels to be initialized This parameter can be a value of @ref ADC Channel TypeDef or a combination of values as follows: 1st combination : channels from ADC Channel 0 to ADC Channel 7 2nd combination : channels from ADC Channel 8 to ADC Channel 15 3rd combination : channels from ADC Channel 16 to ADC Channel 23 @param NewState : new state of the Schmitt Trigger This parameter can be: ENABLE or DISABLE @retval None void ADC SchmittTriggerConfig ADC TypeDef ADCx ADC Channel TypeDef ADC Channels FunctionalState NewState { uint8 t regindex 0; Check the parameters assert param IS FUNCTIONAL STATE NewState ; regindex uint8 t ADC Channels >> 8 ; if NewState DISABLE { ADCx >TRIGR[regindex] & uint8 t ADC Channels ; } else { ADCx >TRIGR[regindex] | uint8 t ADC Channels ; } } @brief Configures the ADC conversion through external trigger @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC ExtEventSelection : Specifies the external trigger This parameter can be a value of @ref ADC ExtEventSelection TypeDef @param ADC ExtTRGSensitivity : Specifies the external trigger sensitivity This parameter can be a value of @ref ADC ExtTRGSensitivity TypeDef @retval None void ADC ExternalTrigConfig ADC TypeDef ADCx ADC ExtEventSelection TypeDef ADC ExtEventSelection ADC ExtTRGSensitivity TypeDef ADC ExtTRGSensitivity { Check the parameters assert param IS ADC EXT EVENT SELECTION ADC ExtEventSelection ; assert param IS ADC EXT TRG SENSITIVITY ADC ExtTRGSensitivity ; clear old config in CR2 register ADCx >CR2 & uint8 t ADC CR2 TRIGEDGE | ADC CR2 EXTSEL ; set the External Trigger Edge Sensitivity and the external event selection ADCx >CR2 | uint8 t ADC ExtTRGSensitivity | ADC ExtEventSelection ; } @brief Returns the last ADC converted data @param ADCx where x can be 1 to select the specified ADC peripheral @retval The Data conversion value uint16 t ADC GetConversionValue ADC TypeDef ADCx { uint16 t tmpreg 0; tmpreg uint16 t ADCx >DRH ; tmpreg uint16 t tmpreg << 8 | ADCx >DRL ; Return the selected ADC conversion value return uint16 t tmpreg ; } @brief Configures the channel to be checked by the Analog watchdog @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC AnalogWatchdogSelection : Specifies the channel to be checked by by the Analog watchdog This parameter can be a value of @ref ADC AnalogWatchdogSelection TypeDef @retval None void ADC AnalogWatchdogChannelSelect ADC TypeDef ADCx ADC AnalogWatchdogSelection TypeDef ADC AnalogWatchdogSelection { Check the parameters assert param IS ADC ANALOGWATCHDOG SELECTION ADC AnalogWatchdogSelection ; reset the CHSEL bits ADCx >CR3 & uint8 t ADC CR3 CHSEL ; ADCx >CR3 | uint8 t ADC AnalogWatchdogSelection; } @brief Configures the high and low thresholds of the Analog watchdog @param ADCx where x can be 1 to select the specified ADC peripheral @param HighThreshold: Analog watchdog High threshold value This parameter must be a 12bit value @param LowThreshold: Analog watchdog Low threshold value This parameter must be a 12bit value @retval None void ADC AnalogWatchdogThresholdsConfig ADC TypeDef ADCx uint16 t HighThreshold uint16 t LowThreshold { Check the parameters assert param IS ADC THRESHOLD HighThreshold ; assert param IS ADC THRESHOLD LowThreshold ; Set the ADC high threshold ADCx >HTRH uint8 t HighThreshold >> 8 ; ADCx >HTRL uint8 t HighThreshold ; Set the ADC low threshold ADCx >LTRH uint8 t LowThreshold >> 8 ; ADCx >LTRL uint8 t LowThreshold ; } @brief Configures the Analog watchdog @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC AnalogWatchdogSelection : Specifies the channel to be checked by by the Analog watchdog This parameter can be a value of @ref ADC AnalogWatchdogSelection TypeDef @param HighThreshold: Analog watchdog High threshold value This parameter must be a 12bit value @param LowThreshold: Analog watchdog Low threshold value This parameter must be a 12bit value @retval None void ADC AnalogWatchdogConfig ADC TypeDef ADCx ADC AnalogWatchdogSelection TypeDef ADC AnalogWatchdogSelection uint16 t HighThreshold uint16 t LowThreshold { Check the parameters assert param IS ADC ANALOGWATCHDOG SELECTION ADC AnalogWatchdogSelection ; assert param IS ADC THRESHOLD HighThreshold ; assert param IS ADC THRESHOLD LowThreshold ; reset the CHSEL bits ADCx >CR3 & uint8 t ADC CR3 CHSEL ; ADCx >CR3 | uint8 t ADC AnalogWatchdogSelection; Set the ADC high threshold ADCx >HTRH uint8 t HighThreshold >> 8 ; ADCx >HTRL uint8 t HighThreshold ; Set the ADC low threshold ADCx >LTRH uint8 t LowThreshold >> 8 ; ADCx >LTRL uint8 t LowThreshold; } @brief Checks whether the specified ADC flag is set or not @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC FLAG: specifies the flag to check This parameter can be a value of @ref ADC FLAG TypeDef @retval The new state of ADC FLAG SET or RESET FlagStatus ADC GetFlagStatus ADC TypeDef ADCx ADC FLAG TypeDef ADC FLAG { FlagStatus bitstatus RESET; Check the parameters assert param IS ADC GET FLAG ADC FLAG ; Check the status of the specified ADC flag if ADCx >SR & ADC FLAG uint8 t RESET { ADC FLAG is set bitstatus SET; } else { ADC FLAG is reset bitstatus RESET; } Return the ADC FLAG status return bitstatus; } @brief Clears the ADC"s pending flags @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC FLAG: specifies the flag to clear This parameter can be a value of @ref ADC FLAG TypeDef @retval None void ADC ClearFlag ADC TypeDef ADCx ADC FLAG TypeDef ADC FLAG { Check the parameters assert param IS ADC CLEAR FLAG ADC FLAG ; Clear the selected ADC flags ADCx >SR uint8 t ADC FLAG; } @brief Checks whether the specified ADC interrupt has occurred or not @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC IT: specifies the ADC interrupt source to check This parameter can be a value of @ref ADC IT TypeDef @retval Status of ADC IT SET or RESET ITStatus ADC GetITStatus ADC TypeDef ADCx ADC IT TypeDef ADC IT { ITStatus bitstatus RESET; uint8 t itmask 0 enablestatus 0; Check the parameters assert param IS ADC GET IT ADC IT ; Get the ADC IT index itmask uint8 t ADC IT >> 3 ; itmask uint8 t uint8 t uint8 t itmask & 0x10 >> 2 | uint8 t itmask & 0x03 ; Get the ADC IT enable bit status enablestatus uint8 t ADCx >CR1 & uint8 t ADC IT ; Check the status of the specified ADC interrupt if ADCx >SR & itmask uint8 t RESET && enablestatus { ADC IT is set bitstatus SET; } else { ADC IT is reset bitstatus RESET; } Return the ADC IT status return bitstatus; } @brief Clears the ADC抯 interrupt pending bits @param ADCx where x can be 1 to select the specified ADC peripheral @param ADC IT: specifies the ADC interrupt pending bit to clear This parameter can be a value of @ref ADC IT TypeDef @retval None void ADC ClearITPendingBit ADC TypeDef ADCx ADC IT TypeDef ADC IT { uint8 t itmask 0; Check the parameters assert param IS ADC IT ADC IT ; Get the ADC IT index itmask uint8 t ADC IT >> 3 ; itmask uint8 t uint8 t itmask & 0x10 >> 2 | uint8 t itmask & 0x03 ; Clear the selected ADC interrupt pending bits ADCx >SR uint8 t itmask; }">可用于STM开发基AD转换的C语言程序 Includes #include "stm8l15x adc h" @addtogroup STM8L15x StdPeriph Driver @{ Private typedef [更多]
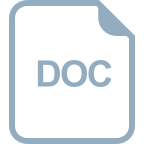
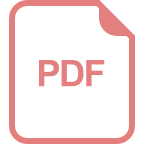
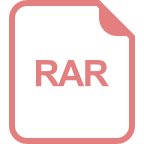
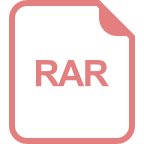
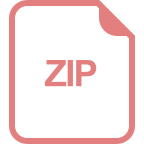
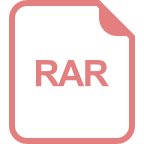
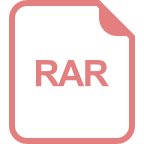
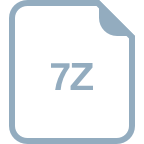
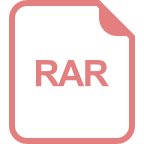
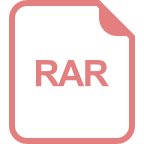
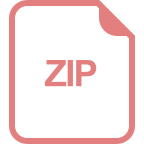
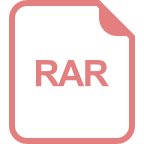
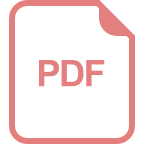
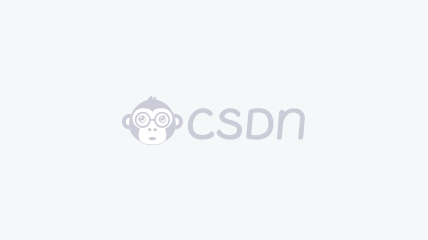

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

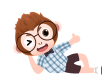
最新资源
- java毕业设计-基于SSM框架的传统服饰文化体验平台【代码+部署教程】
- 优化领域的模拟退火算法详解与实战
- NewFileTime-x64.zip.fgpg
- 基于Python和HTML的Chinese-estate-helper房地产爬虫及可视化设计源码
- 基于SpringBoot2.7.7的当当书城Java后端设计源码
- 基于Python和Go语言的开发工具集成与验证设计源码
- 基于Python与JavaScript的国内供应商管理系统设计源码
- aspose.words-20.12-jdk17
- 基于czsc库的Python时间序列分析设计源码
- 基于Java、CSS、JavaScript、HTML的跨语言智联平台设计源码

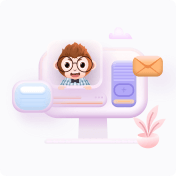
