# mysql
[![NPM Version][npm-version-image]][npm-url]
[![NPM Downloads][npm-downloads-image]][npm-url]
[![Node.js Version][node-image]][node-url]
[![Linux Build][travis-image]][travis-url]
[![Windows Build][appveyor-image]][appveyor-url]
[![Test Coverage][coveralls-image]][coveralls-url]
## Table of Contents
- [Install](#install)
- [Introduction](#introduction)
- [Contributors](#contributors)
- [Sponsors](#sponsors)
- [Community](#community)
- [Establishing connections](#establishing-connections)
- [Connection options](#connection-options)
- [SSL options](#ssl-options)
- [Connection flags](#connection-flags)
- [Terminating connections](#terminating-connections)
- [Pooling connections](#pooling-connections)
- [Pool options](#pool-options)
- [Pool events](#pool-events)
- [acquire](#acquire)
- [connection](#connection)
- [enqueue](#enqueue)
- [release](#release)
- [Closing all the connections in a pool](#closing-all-the-connections-in-a-pool)
- [PoolCluster](#poolcluster)
- [PoolCluster options](#poolcluster-options)
- [Switching users and altering connection state](#switching-users-and-altering-connection-state)
- [Server disconnects](#server-disconnects)
- [Performing queries](#performing-queries)
- [Escaping query values](#escaping-query-values)
- [Escaping query identifiers](#escaping-query-identifiers)
- [Preparing Queries](#preparing-queries)
- [Custom format](#custom-format)
- [Getting the id of an inserted row](#getting-the-id-of-an-inserted-row)
- [Getting the number of affected rows](#getting-the-number-of-affected-rows)
- [Getting the number of changed rows](#getting-the-number-of-changed-rows)
- [Getting the connection ID](#getting-the-connection-id)
- [Executing queries in parallel](#executing-queries-in-parallel)
- [Streaming query rows](#streaming-query-rows)
- [Piping results with Streams](#piping-results-with-streams)
- [Multiple statement queries](#multiple-statement-queries)
- [Stored procedures](#stored-procedures)
- [Joins with overlapping column names](#joins-with-overlapping-column-names)
- [Transactions](#transactions)
- [Ping](#ping)
- [Timeouts](#timeouts)
- [Error handling](#error-handling)
- [Exception Safety](#exception-safety)
- [Type casting](#type-casting)
- [Number](#number)
- [Date](#date)
- [Buffer](#buffer)
- [String](#string)
- [Custom type casting](#custom-type-casting)
- [Debugging and reporting problems](#debugging-and-reporting-problems)
- [Security issues](#security-issues)
- [Contributing](#contributing)
- [Running tests](#running-tests)
- [Running unit tests](#running-unit-tests)
- [Running integration tests](#running-integration-tests)
- [Todo](#todo)
## Install
This is a [Node.js](https://nodejs.org/en/) module available through the
[npm registry](https://www.npmjs.com/).
Before installing, [download and install Node.js](https://nodejs.org/en/download/).
Node.js 0.6 or higher is required.
Installation is done using the
[`npm install` command](https://docs.npmjs.com/getting-started/installing-npm-packages-locally):
```sh
$ npm install mysql
```
For information about the previous 0.9.x releases, visit the [v0.9 branch][].
Sometimes I may also ask you to install the latest version from Github to check
if a bugfix is working. In this case, please do:
```sh
$ npm install mysqljs/mysql
```
[v0.9 branch]: https://github.com/mysqljs/mysql/tree/v0.9
## Introduction
This is a node.js driver for mysql. It is written in JavaScript, does not
require compiling, and is 100% MIT licensed.
Here is an example on how to use it:
```js
var mysql = require('mysql');
var connection = mysql.createConnection({
host : 'localhost',
user : 'me',
password : 'secret',
database : 'my_db'
});
connection.connect();
connection.query('SELECT 1 + 1 AS solution', function (error, results, fields) {
if (error) throw error;
console.log('The solution is: ', results[0].solution);
});
connection.end();
```
From this example, you can learn the following:
* Every method you invoke on a connection is queued and executed in sequence.
* Closing the connection is done using `end()` which makes sure all remaining
queries are executed before sending a quit packet to the mysql server.
## Contributors
Thanks goes to the people who have contributed code to this module, see the
[GitHub Contributors page][].
[GitHub Contributors page]: https://github.com/mysqljs/mysql/graphs/contributors
Additionally I'd like to thank the following people:
* [Andrey Hristov][] (Oracle) - for helping me with protocol questions.
* [Ulf Wendel][] (Oracle) - for helping me with protocol questions.
[Ulf Wendel]: http://blog.ulf-wendel.de/
[Andrey Hristov]: http://andrey.hristov.com/
## Sponsors
The following companies have supported this project financially, allowing me to
spend more time on it (ordered by time of contribution):
* [Transloadit](http://transloadit.com) (my startup, we do file uploading &
video encoding as a service, check it out)
* [Joyent](http://www.joyent.com/)
* [pinkbike.com](http://pinkbike.com/)
* [Holiday Extras](http://www.holidayextras.co.uk/) (they are [hiring](http://join.holidayextras.co.uk/))
* [Newscope](http://newscope.com/) (they are [hiring](https://newscope.com/unternehmen/jobs/))
## Community
If you'd like to discuss this module, or ask questions about it, please use one
of the following:
* **Mailing list**: https://groups.google.com/forum/#!forum/node-mysql
* **IRC Channel**: #node.js (on freenode.net, I pay attention to any message
including the term `mysql`)
## Establishing connections
The recommended way to establish a connection is this:
```js
var mysql = require('mysql');
var connection = mysql.createConnection({
host : 'example.org',
user : 'bob',
password : 'secret'
});
connection.connect(function(err) {
if (err) {
console.error('error connecting: ' + err.stack);
return;
}
console.log('connected as id ' + connection.threadId);
});
```
However, a connection can also be implicitly established by invoking a query:
```js
var mysql = require('mysql');
var connection = mysql.createConnection(...);
connection.query('SELECT 1', function (error, results, fields) {
if (error) throw error;
// connected!
});
```
Depending on how you like to handle your errors, either method may be
appropriate. Any type of connection error (handshake or network) is considered
a fatal error, see the [Error Handling](#error-handling) section for more
information.
## Connection options
When establishing a connection, you can set the following options:
* `host`: The hostname of the database you are connecting to. (Default:
`localhost`)
* `port`: The port number to connect to. (Default: `3306`)
* `localAddress`: The source IP address to use for TCP connection. (Optional)
* `socketPath`: The path to a unix domain socket to connect to. When used `host`
and `port` are ignored.
* `user`: The MySQL user to authenticate as.
* `password`: The password of that MySQL user.
* `database`: Name of the database to use for this connection (Optional).
* `charset`: The charset for the connection. This is called "collation" in the SQL-level
of MySQL (like `utf8_general_ci`). If a SQL-level charset is specified (like `utf8mb4`)
then the default collation for that charset is used. (Default: `'UTF8_GENERAL_CI'`)
* `timezone`: The timezone configured on the MySQL server. This is used to type cast server date/time values to JavaScript `Date` object and vice versa. This can be `'local'`, `'Z'`, or an offset in the form `+HH:MM` or `-HH:MM`. (Default: `'local'`)
* `connectTimeout`: The milliseconds before a timeout occurs during the initial connection
to the MySQL server. (Default: `10000`)
* `stringifyObjects`: Stringify objects instead of converting to values. See
issue [#501](https://github.com/mysqljs/mysql/issues/501). (Default: `false`)
* `insecureAuth`: Allow connecting to MySQL instances that ask for the old
(insecure) authentica
没有合适的资源?快使用搜索试试~ 我知道了~
mysql+node.js+express 实现登录功能
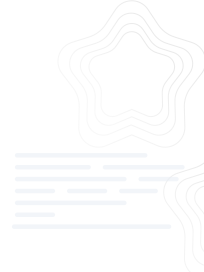
共1645个文件
js:289个
md:150个
json:111个

需积分: 0 1 下载量 40 浏览量
2024-12-25
15:51:43
上传
评论
收藏 5.15MB ZIP 举报
温馨提示
mysql+node.js+express 实现登录功能
资源推荐
资源详情
资源评论
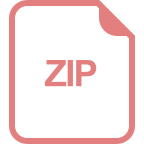
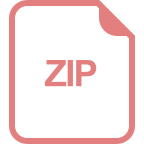
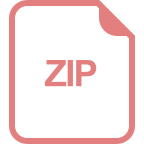
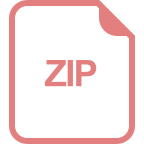
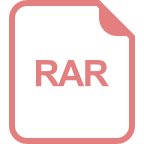
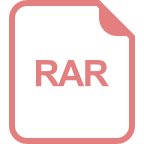
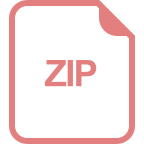
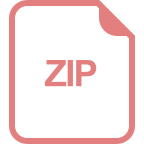
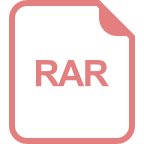
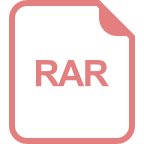
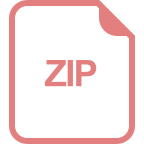
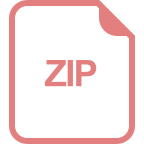
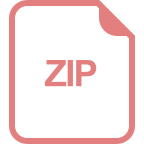
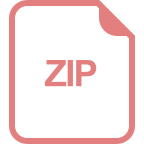
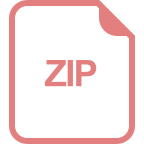
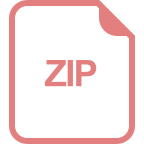
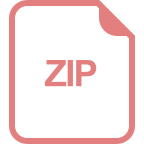
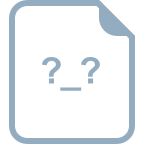
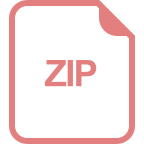
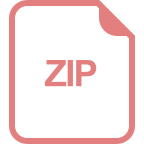
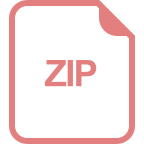
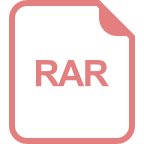
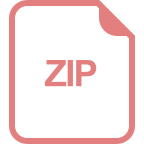
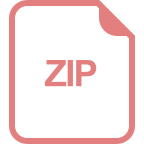
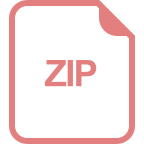
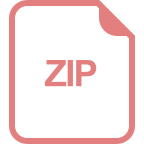
收起资源包目录

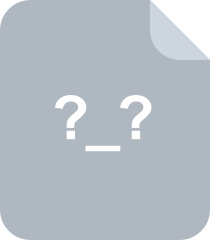
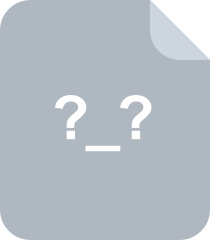
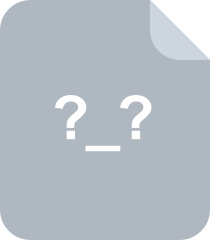
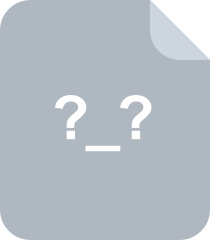
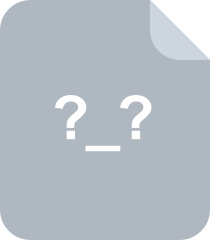
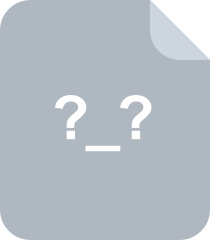
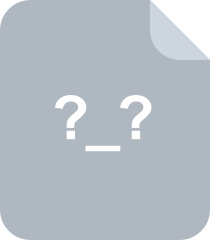
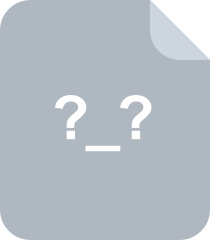
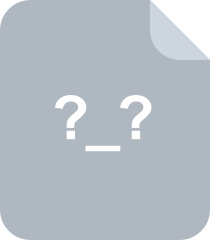
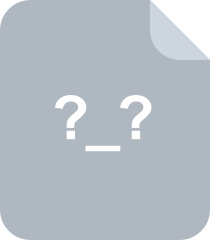
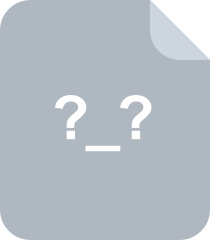
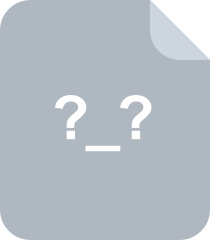
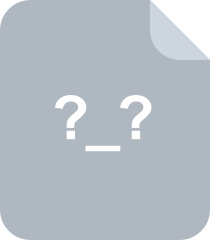
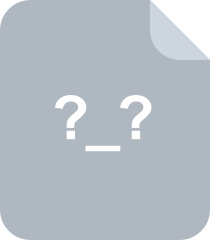
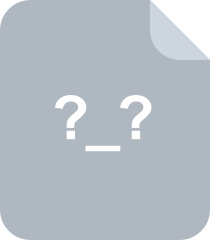
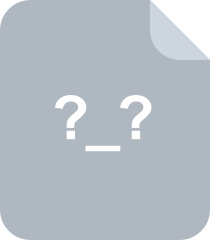
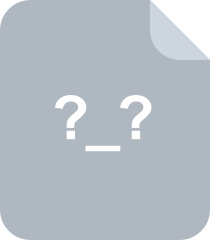
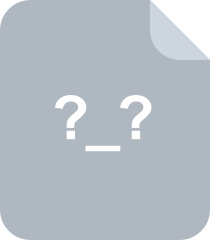
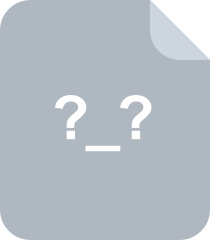
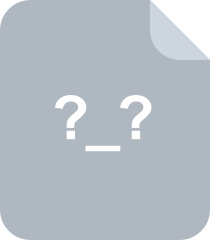
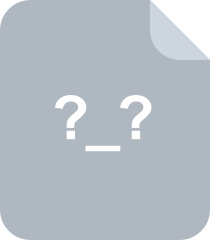
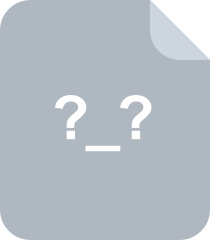
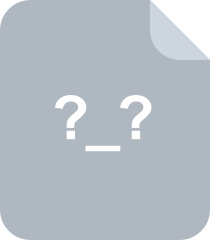
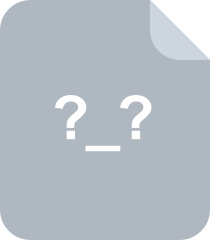
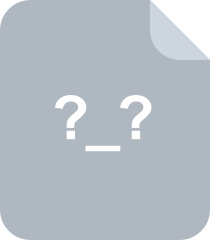
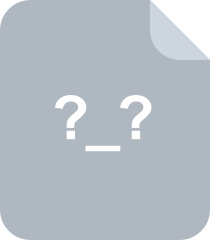
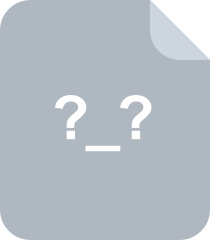
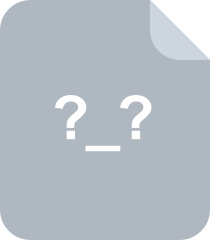
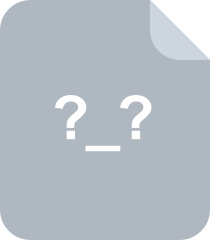
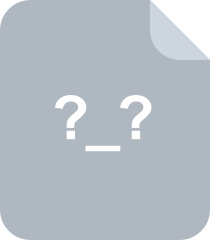
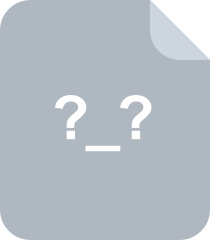
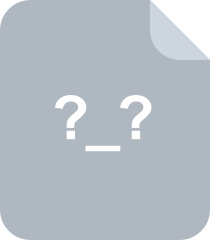
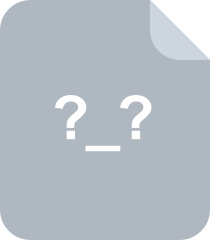
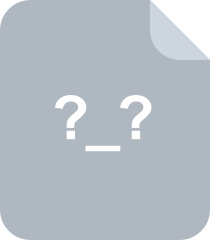
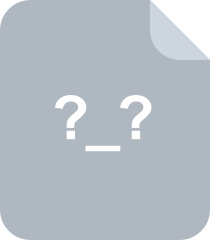
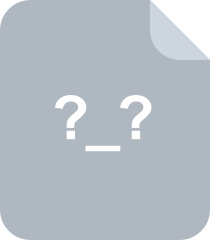
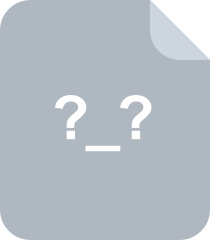
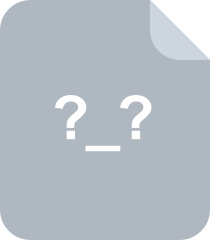
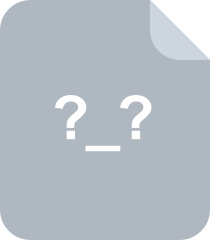
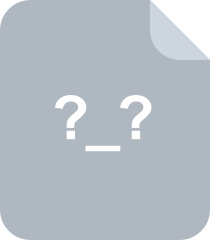
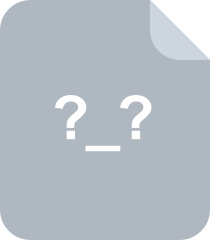
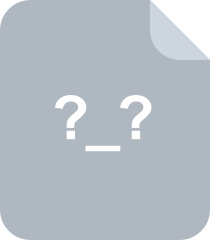
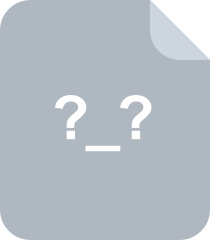
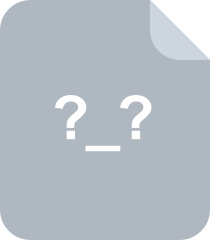
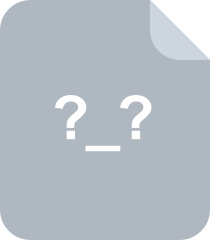
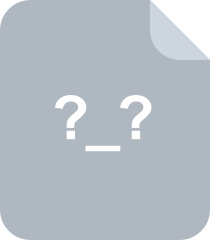
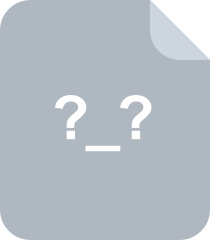
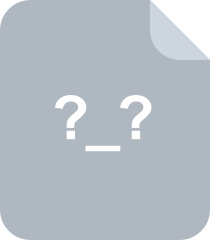
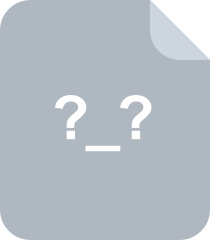
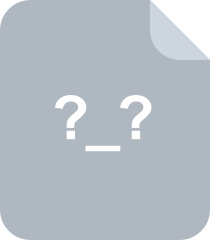
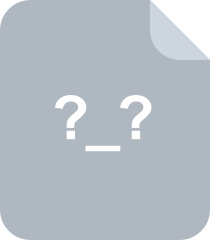
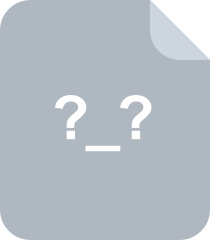
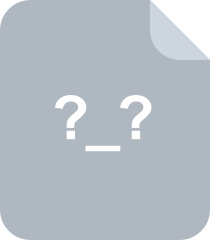
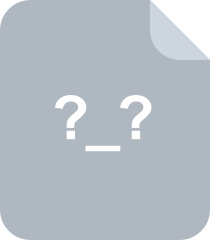
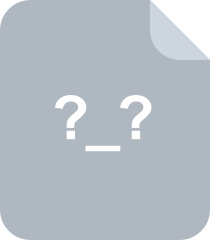
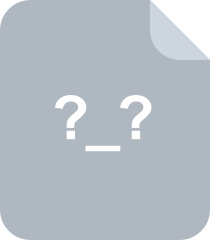
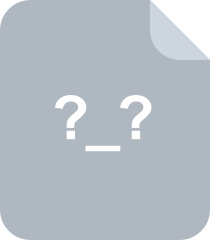
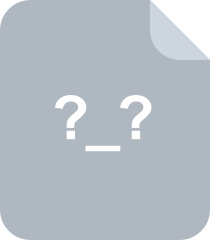
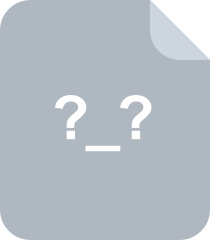
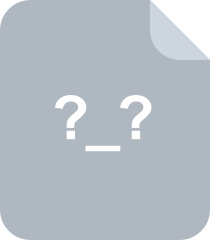
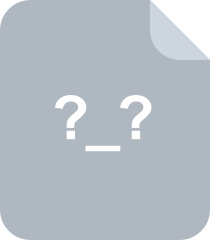
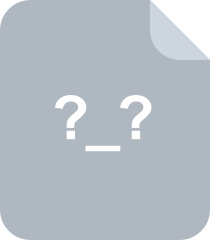
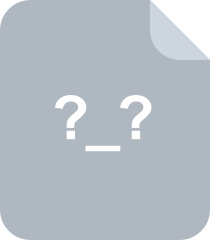
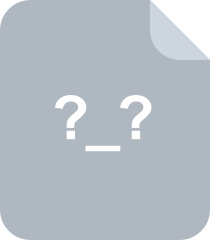
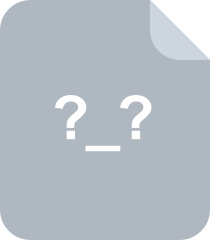
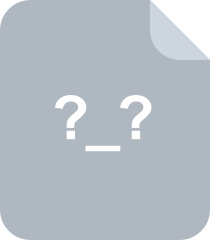
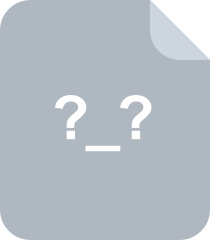
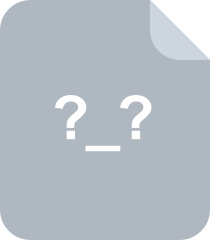
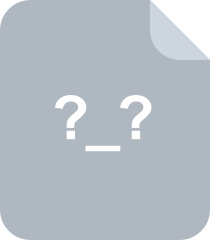
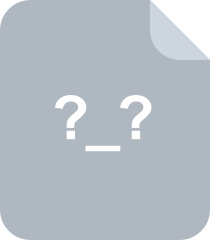
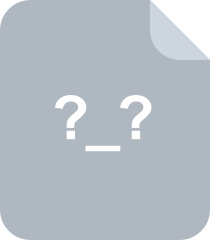
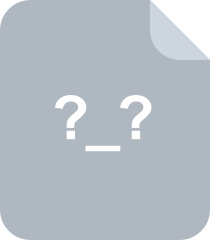
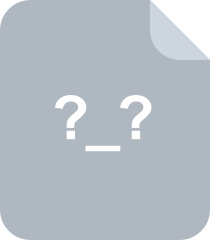
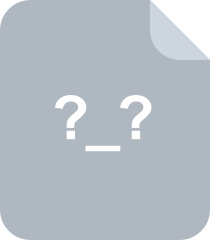
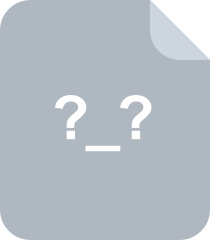
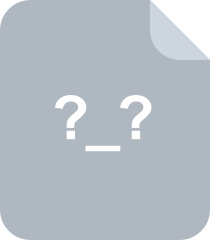
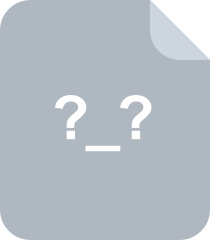
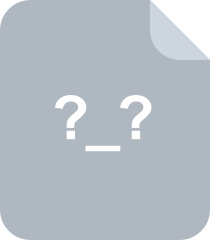
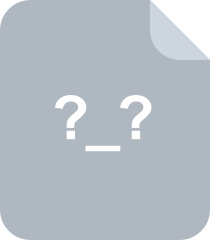
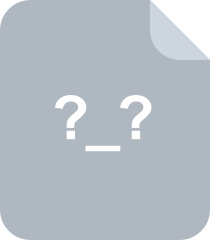
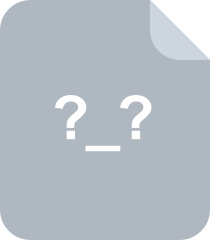
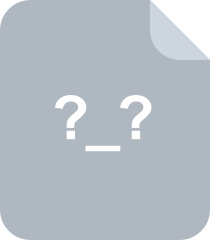
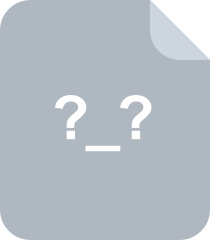
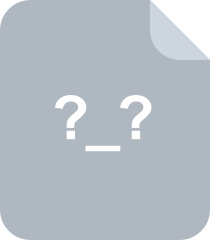
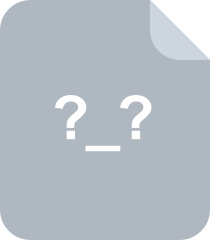
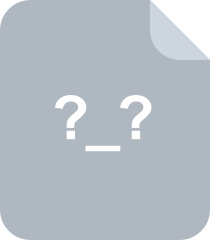
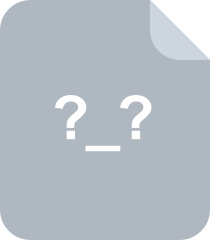
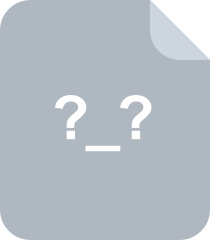
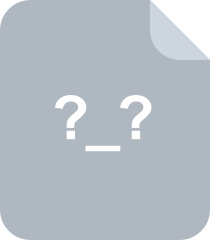
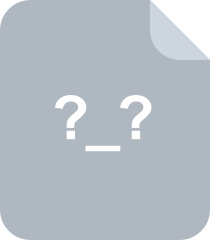
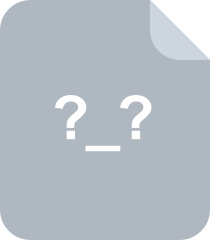
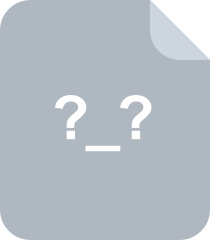
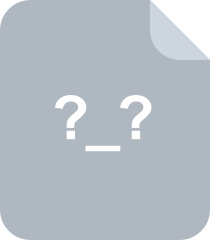
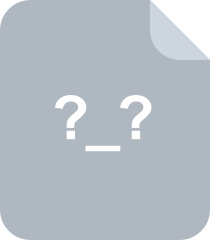
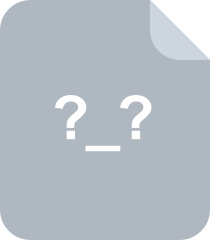
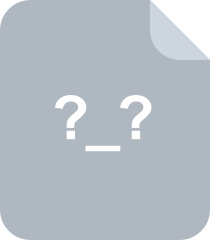
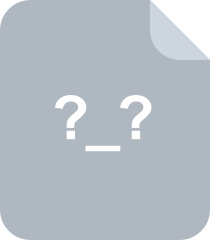
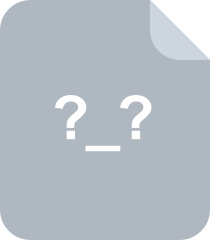
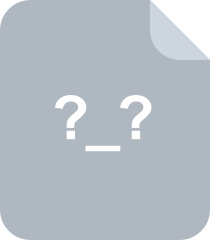
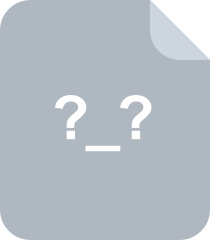
共 1645 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
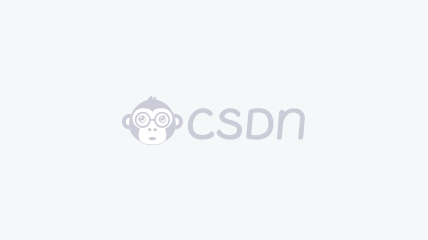

u010009053
- 粉丝: 10
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

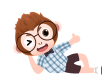
最新资源
- 使用归一化互信息对图像进行刚性(平移和旋转)自动配准Maatlab代码.rar
- 使用各向异性扩散过滤进行高级 2D_3D 噪声去除和边缘增强 ( Weickert )Matlab代码.rar
- wqeqweqeqwe
- igh ethercat kingseng robottt
- 学习threejs,导入babylon格式的模型
- 听力提升播客《Listening Time》第1集:英语学习者的听力训练指南
- CDN:加速全球互联网内容的关键技术及其应用场景与未来趋势
- 使用最佳 Gamma 校正和加权和进行图像对比度增强亮度保留Matlab代码.rar
- 使用自定义组织回声图模拟伪 B 型超声图像Matlab代码.rar
- 使用中等光谱相关性和一致的边缘图进行联合去马赛克和缩放Matlab代码.rar
- 使用坐标信息进行 3D 重建Matlab代码.rar
- 适用于 MATLAB 的简单图像堆栈可视化工具MATLAB代码.rar
- 适用于 MATLAB 的 Elastix 包装器Matlab代码.rar
- 数字图像的插值Matlab代码.rar
- 它读取 3D 原始图像并显示原始体积图像的中间横截面MATLAB代码.rar
- 手术前后的整形手术Matlab代码.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


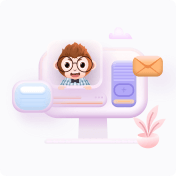
安全验证
文档复制为VIP权益,开通VIP直接复制
