package cli // import "github.com/urfave/cli/v2"
Package cli provides a minimal framework for creating and organizing command
line Go applications. cli is designed to be easy to understand and write,
the most simple cli application can be written as follows:
func main() {
(&cli.App{}).Run(os.Args)
}
Of course this application does not do much, so let's make this an actual
application:
func main() {
app := &cli.App{
Name: "greet",
Usage: "say a greeting",
Action: func(c *cli.Context) error {
fmt.Println("Greetings")
return nil
},
}
app.Run(os.Args)
}
VARIABLES
var (
SuggestFlag SuggestFlagFunc = suggestFlag
SuggestCommand SuggestCommandFunc = suggestCommand
SuggestDidYouMeanTemplate string = suggestDidYouMeanTemplate
)
var AppHelpTemplate = `NAME:
{{$v := offset .Name 6}}{{wrap .Name 3}}{{if .Usage}} - {{wrap .Usage $v}}{{end}}
USAGE:
{{if .UsageText}}{{wrap .UsageText 3}}{{else}}{{.HelpName}} {{if .VisibleFlags}}[global options]{{end}}{{if .Commands}} command [command options]{{end}} {{if .ArgsUsage}}{{.ArgsUsage}}{{else}}[arguments...]{{end}}{{end}}{{if .Version}}{{if not .HideVersion}}
VERSION:
{{.Version}}{{end}}{{end}}{{if .Description}}
DESCRIPTION:
{{wrap .Description 3}}{{end}}{{if len .Authors}}
AUTHOR{{with $length := len .Authors}}{{if ne 1 $length}}S{{end}}{{end}}:
{{range $index, $author := .Authors}}{{if $index}}
{{end}}{{$author}}{{end}}{{end}}{{if .VisibleCommands}}
COMMANDS:{{range .VisibleCategories}}{{if .Name}}
{{.Name}}:{{range .VisibleCommands}}
{{join .Names ", "}}{{"\t"}}{{.Usage}}{{end}}{{else}}{{range .VisibleCommands}}
{{join .Names ", "}}{{"\t"}}{{.Usage}}{{end}}{{end}}{{end}}{{end}}{{if .VisibleFlagCategories}}
GLOBAL OPTIONS:{{range .VisibleFlagCategories}}
{{if .Name}}{{.Name}}
{{end}}{{range .Flags}}{{.}}
{{end}}{{end}}{{else}}{{if .VisibleFlags}}
GLOBAL OPTIONS:
{{range $index, $option := .VisibleFlags}}{{if $index}}
{{end}}{{wrap $option.String 6}}{{end}}{{end}}{{end}}{{if .Copyright}}
COPYRIGHT:
{{wrap .Copyright 3}}{{end}}
`
AppHelpTemplate is the text template for the Default help topic. cli.go uses
text/template to render templates. You can render custom help text by
setting this variable.
var CommandHelpTemplate = `NAME:
{{$v := offset .HelpName 6}}{{wrap .HelpName 3}}{{if .Usage}} - {{wrap .Usage $v}}{{end}}
USAGE:
{{if .UsageText}}{{wrap .UsageText 3}}{{else}}{{.HelpName}}{{if .VisibleFlags}} [command options]{{end}} {{if .ArgsUsage}}{{.ArgsUsage}}{{else}}[arguments...]{{end}}{{end}}{{if .Category}}
CATEGORY:
{{.Category}}{{end}}{{if .Description}}
DESCRIPTION:
{{wrap .Description 3}}{{end}}{{if .VisibleFlagCategories}}
OPTIONS:{{range .VisibleFlagCategories}}
{{if .Name}}{{.Name}}
{{end}}{{range .Flags}}{{.}}
{{end}}{{end}}{{else}}{{if .VisibleFlags}}
OPTIONS:
{{range .VisibleFlags}}{{.}}
{{end}}{{end}}{{end}}
`
CommandHelpTemplate is the text template for the command help topic. cli.go
uses text/template to render templates. You can render custom help text by
setting this variable.
var ErrWriter io.Writer = os.Stderr
ErrWriter is used to write errors to the user. This can be anything
implementing the io.Writer interface and defaults to os.Stderr.
var FishCompletionTemplate = `# {{ .App.Name }} fish shell completion
function __fish_{{ .App.Name }}_no_subcommand --description 'Test if there has been any subcommand yet'
for i in (commandline -opc)
if contains -- $i{{ range $v := .AllCommands }} {{ $v }}{{ end }}
return 1
end
end
return 0
end
{{ range $v := .Completions }}{{ $v }}
{{ end }}`
var MarkdownDocTemplate = `{{if gt .SectionNum 0}}% {{ .App.Name }} {{ .SectionNum }}
{{end}}# NAME
{{ .App.Name }}{{ if .App.Usage }} - {{ .App.Usage }}{{ end }}
# SYNOPSIS
{{ .App.Name }}
{{ if .SynopsisArgs }}
` + "```" + `
{{ range $v := .SynopsisArgs }}{{ $v }}{{ end }}` + "```" + `
{{ end }}{{ if .App.Description }}
# DESCRIPTION
{{ .App.Description }}
{{ end }}
**Usage**:
` + "```" + `{{ if .App.UsageText }}
{{ .App.UsageText }}
{{ else }}
{{ .App.Name }} [GLOBAL OPTIONS] command [COMMAND OPTIONS] [ARGUMENTS...]
{{ end }}` + "```" + `
{{ if .GlobalArgs }}
# GLOBAL OPTIONS
{{ range $v := .GlobalArgs }}
{{ $v }}{{ end }}
{{ end }}{{ if .Commands }}
# COMMANDS
{{ range $v := .Commands }}
{{ $v }}{{ end }}{{ end }}`
var OsExiter = os.Exit
OsExiter is the function used when the app exits. If not set defaults to
os.Exit.
var SubcommandHelpTemplate = `NAME:
{{.HelpName}} - {{.Usage}}
USAGE:
{{if .UsageText}}{{wrap .UsageText 3}}{{else}}{{.HelpName}} command{{if .VisibleFlags}} [command options]{{end}} {{if .ArgsUsage}}{{.ArgsUsage}}{{else}}[arguments...]{{end}}{{end}}{{if .Description}}
DESCRIPTION:
{{wrap .Description 3}}{{end}}
COMMANDS:{{range .VisibleCategories}}{{if .Name}}
{{.Name}}:{{range .VisibleCommands}}
{{join .Names ", "}}{{"\t"}}{{.Usage}}{{end}}{{else}}{{range .VisibleCommands}}
{{join .Names ", "}}{{"\t"}}{{.Usage}}{{end}}{{end}}{{end}}{{if .VisibleFlags}}
OPTIONS:
{{range .VisibleFlags}}{{.}}
{{end}}{{end}}
`
SubcommandHelpTemplate is the text template for the subcommand help topic.
cli.go uses text/template to render templates. You can render custom help
text by setting this variable.
var VersionPrinter = printVersion
VersionPrinter prints the version for the App
var HelpPrinter helpPrinter = printHelp
HelpPrinter is a function that writes the help output. If not set
explicitly, this calls HelpPrinterCustom using only the default template
functions.
If custom logic for printing help is required, this function can be
overridden. If the ExtraInfo field is defined on an App, this function
should not be modified, as HelpPrinterCustom will be used directly in order
to capture the extra information.
var HelpPrinterCustom helpPrinterCustom = printHelpCustom
HelpPrinterCustom is a function that writes the help output. It is used as
the default implementation of HelpPrinter, and may be called directly if the
ExtraInfo field is set on an App.
In the default implementation, if the customFuncs argument contains a
"wrapAt" key, which is a function which takes no arguments and returns an
int, this int value will be used to produce a "wrap" function used by the
default template to wrap long lines.
FUNCTIONS
func DefaultAppComplete(cCtx *Context)
DefaultAppComplete prints the list of subcommands as the default app
completion method
func DefaultCompleteWithFlags(cmd *Command) func(cCtx *Context)
func FlagNames(name string, aliases []string) []string
func HandleAction(action interface{}, cCtx *Context) (err error)
HandleAction attempts to figure out which Action signature was used. If it's
an ActionFunc or a func with the legacy signature for Action, the func is
run!
func HandleExitCoder(err error)
HandleExitCoder handles errors implementing ExitCoder by printing their
message and calling OsExiter with the given exit code.
If the given error instead implements MultiError, each error will be checked
for the ExitCoder interface, and OsExiter will be called with the last exit
code found, or exit code 1 if no ExitCoder is found.
This function is the default error-handling behavior for an App.
func ShowAppHelp(cCtx *Context) error
ShowAppHelp is an action that displays the help.
func ShowAppHelpAndExit(c *Context, exitCode int)
ShowAppHelpAndExit - Prints the list of subcommands for the app and exits
with exit code.
func ShowCommandCompletions(ctx *Context, command string)
ShowCommandCompletions prints the custom completions for a given command
func ShowCommandHelp(ctx *Context, command string) error
ShowCommandHelp prints help f
没有合适的资源?快使用搜索试试~ 我知道了~
cri-tools-1.25.0.tar.gz
需积分: 9 4 下载量 197 浏览量
2022-11-26
15:12:03
上传
评论
收藏 7.54MB GZ 举报
温馨提示
通常,容器引擎会提供一个命令行工具来帮助用户调试容器应用并简化故障排错。比如使用 Docker 作为容器运行时的时候,可以使用 docker 命令来查看容器和镜像的状态,并验证容器的配置是否正确。但在使用其他容器引擎时,推荐使用 crictl 来替代 docker 工具。 crictl 是 cri-tools 的一部分,它提供了类似于 docker 的命令行工具,不需要通过 Kubelet 就可以通过 CRI 跟容器运行时通信。它是专门为 Kubernetes 设计的,提供了Pod、容器和镜像等资源的管理命令,可以帮助用户和开发者调试容器应用或者排查异常问题。crictl 可以用于所有实现了 CRI 接口的容器运行时。
资源推荐
资源详情
资源评论
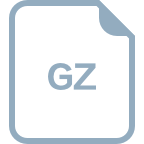
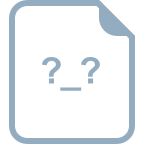
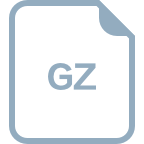
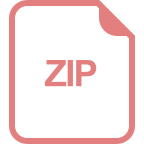
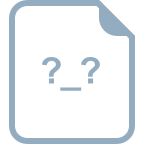
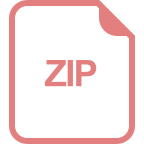
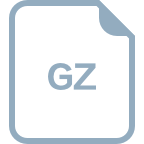
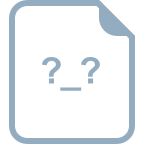
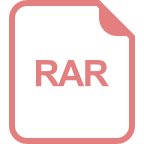
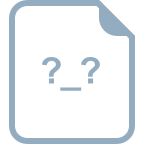
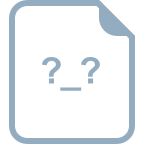
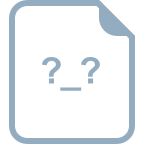
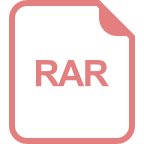
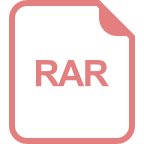
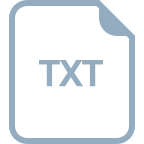
收起资源包目录

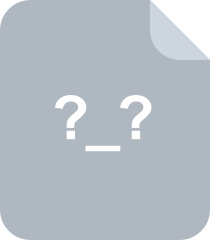
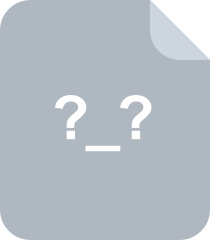
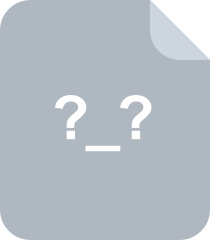
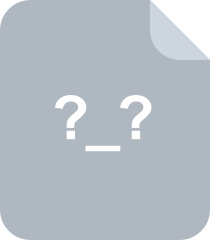
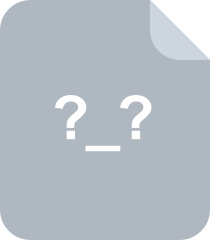
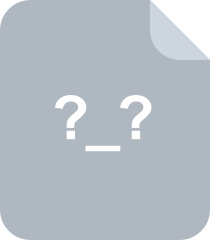
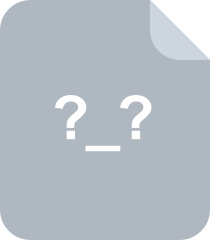
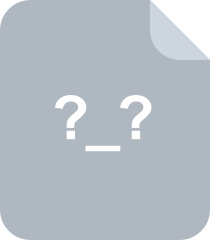
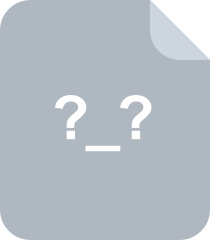
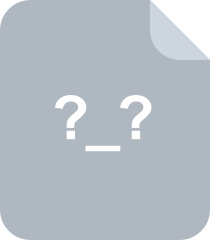
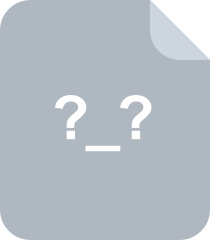
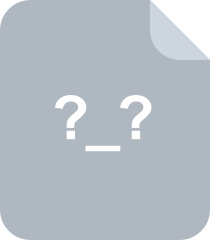
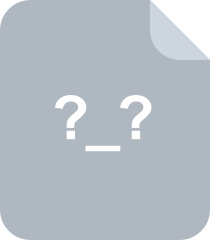
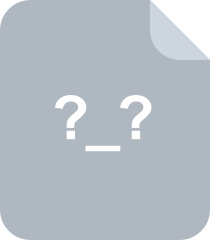
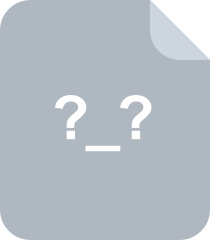
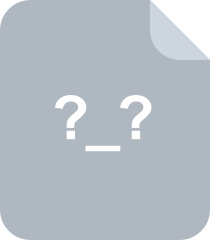
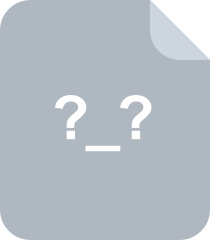
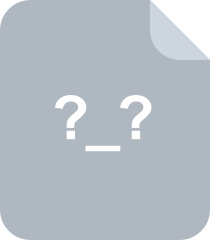
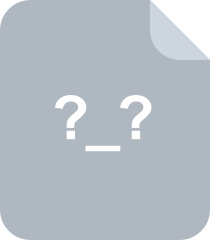
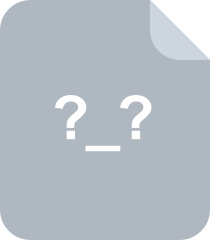
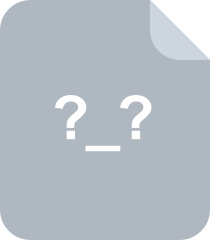
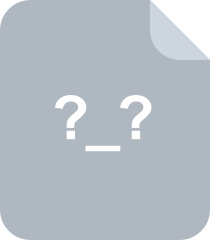
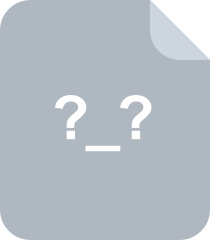
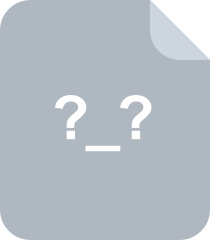
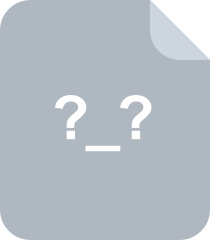
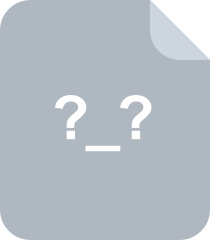
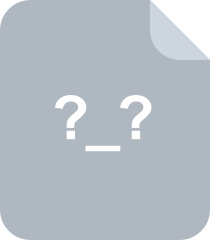
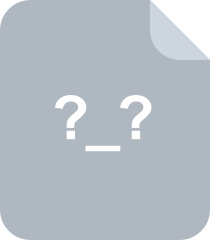
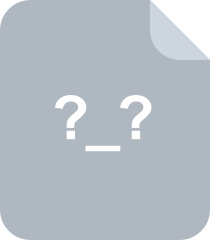
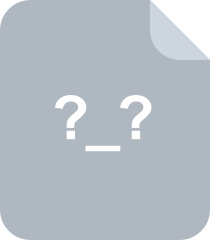
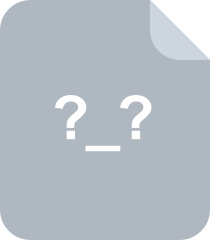
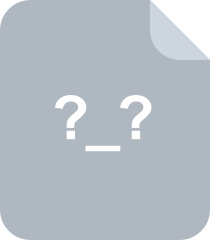
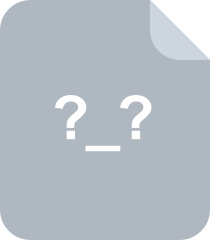
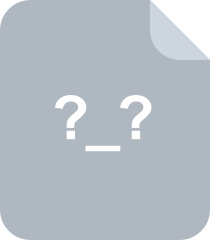
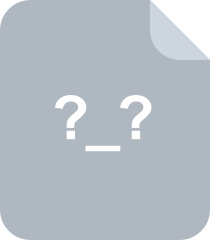
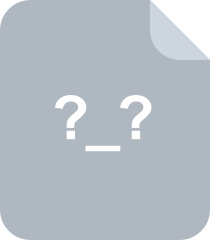
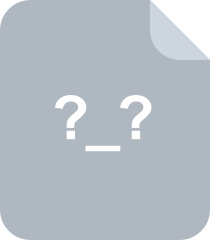
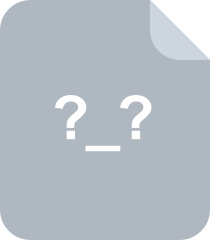
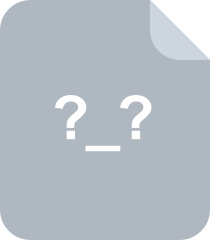
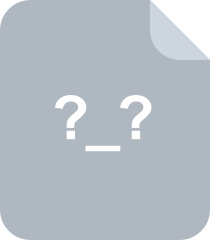
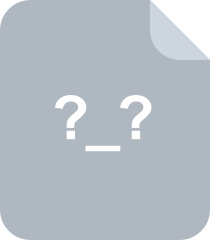
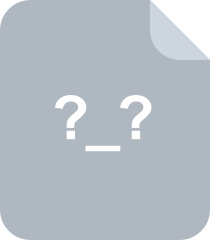
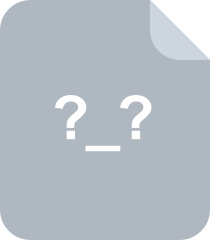
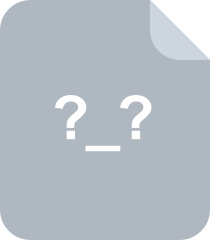
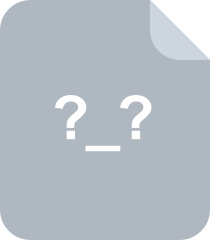
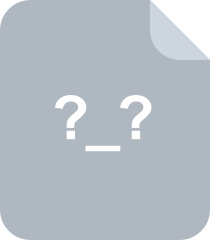
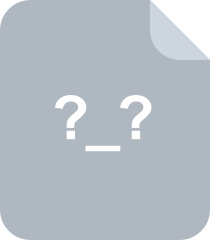
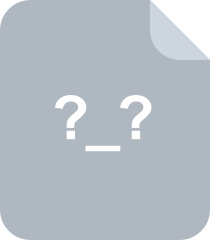
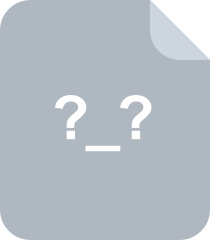
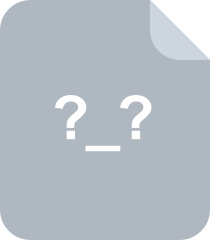
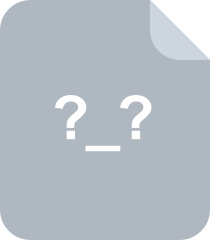
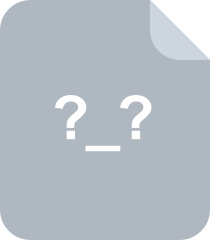
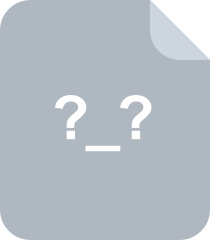
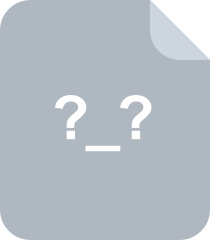
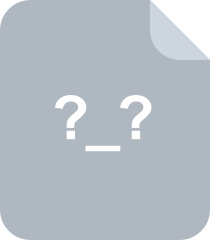
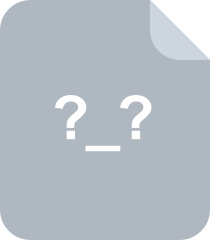
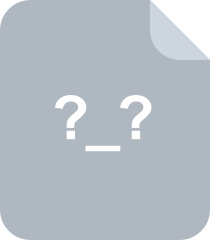
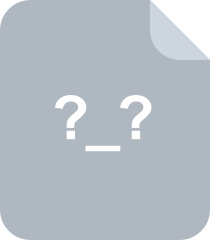
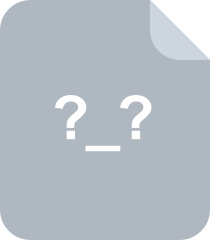
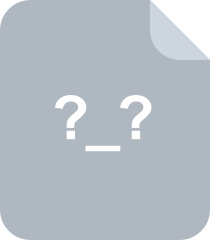
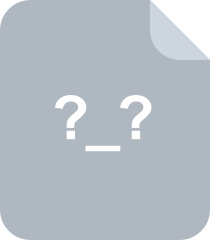
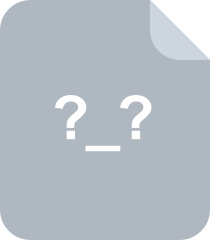
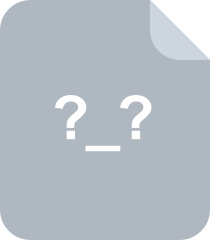
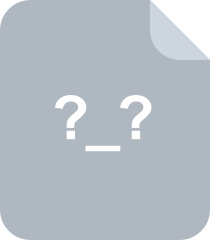
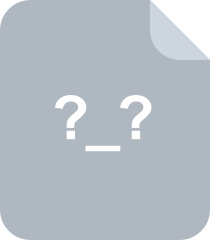
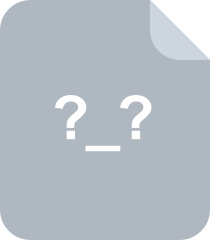
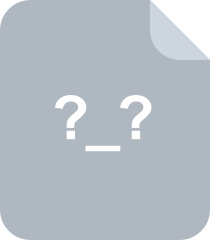
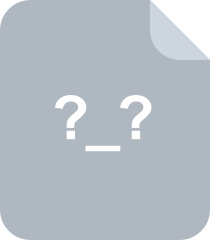
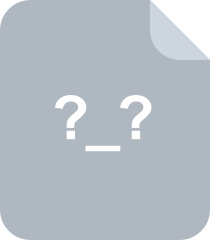
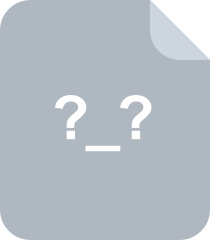
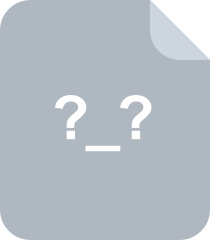
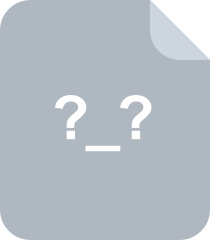
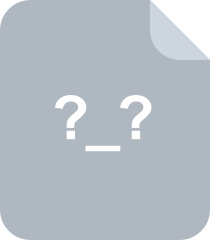
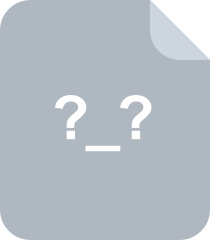
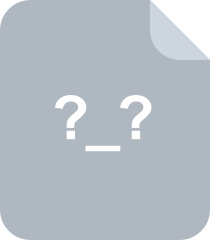
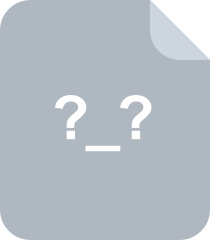
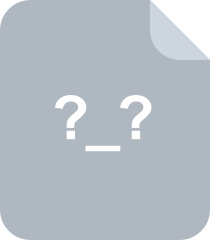
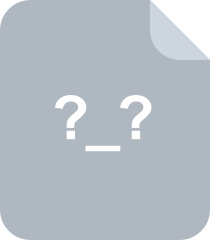
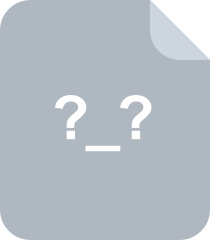
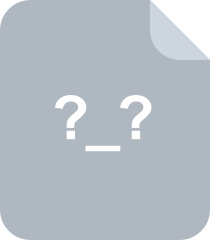
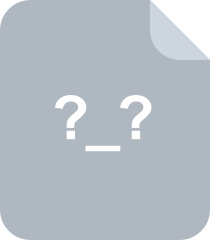
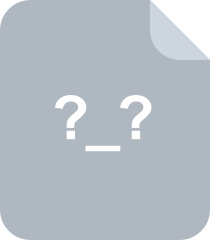
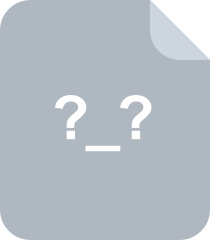
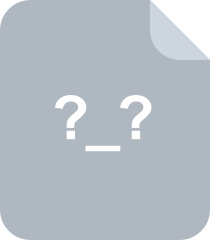
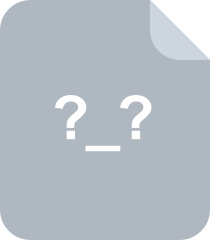
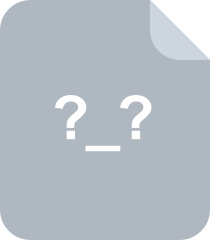
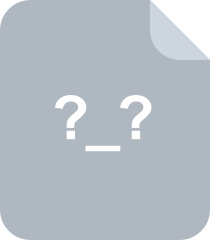
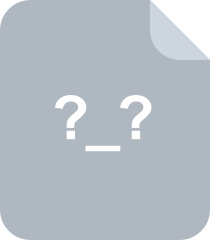
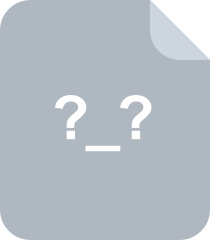
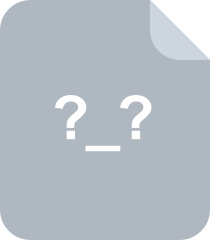
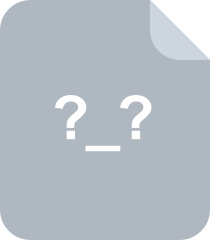
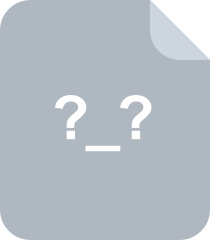
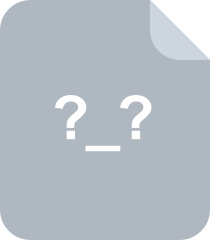
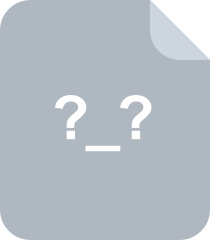
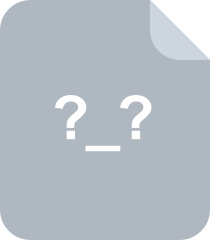
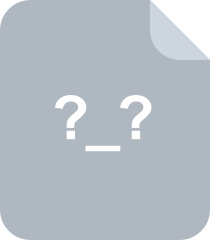
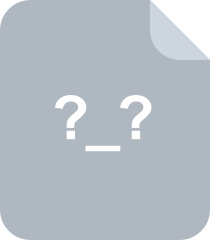
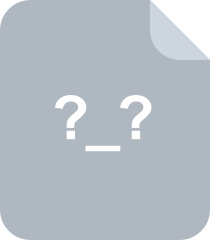
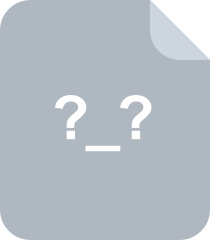
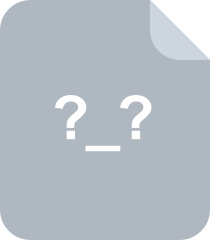
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
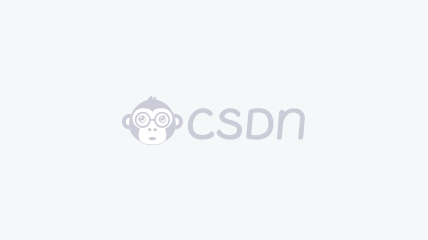

雪饮大侠
- 粉丝: 2
- 资源: 53
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

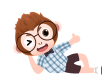
最新资源
- 该存储库将演示如何使用 OpenVINO 运行时 API 部署官方 YOLOv7 预训练模型.zip
- 该存储库包含使用 YOLOv9 对象检测模型和 DeepSORT 算法在视频中进行对象检测和跟踪的代码 .zip
- 论文《YOLO-ReT在边缘 GPU 上实现高精度实时物体检测》的实现.zip
- 让yolov6可以更方便的改变网络结构.zip
- springboot0桂林旅游景点导游平台(代码+数据库+LW)
- mmexportf3d00a398950f9982c0f132475da3f26_1732379945062.jpeg
- mmexport1732556836794.jpg
- 12月考核变动点.wps
- 自定义数据集上的实现.zip
- 891833097559212数据恢复大师_3.8会员版.apk
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


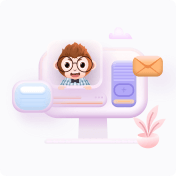
安全验证
文档复制为VIP权益,开通VIP直接复制
