<?php
/**
* Translator Revolution DropDown WP Plugin
* http://goo.gl/xrxDo
*
* LICENSE
*
* You need to buy a license if you want use this script.
* https://codecanyon.net/legal/market
*
* @package Translator Revolution WP Plugin
* @copyright Copyright (c) 2020, SurStudio, www.surstudio.net
* @license https://codecanyon.net/licenses/terms/regular
* @version 2.1
* @date 2020-10-25
*/
class SurStudioPluginTranslatorRevolutionDropDownConfig {
const NAME = 'TranslatorRevolutionDropDown';
const VERSION = '2.1';
const UI_NAME = 'Auto Translation';
const WIDGET_NAME = 'Translator Revolution DropDown';
const WELCOME_NAME = 'Dashboard';
const UI_WELCOME_NAME = 'Dashboard';
const SETTINGS_NAME = 'Settings';
const UI_SETTINGS_NAME = 'Settings';
const UI_TRANSLATIONS_NAME = 'Edit translations';
const ADMIN_TRANSLATIONS_NAME = 'surstudio-translator-revolution-dropdown-translations';
const WIDGET_INTERNAL_NAME = 'surstudio-translator-revolution-dropdown';
const ADMIN_LANGUAGE_DOMAIN = 'translator_revolution_dropdown';
const ADMIN_WELCOME_NAME = 'translator-revolution-dropdown-plugin-dashboard';
const ADMIN_SETTINGS_NAME = 'translator-revolution-dropdown-plugin-settings';
const ADMIN_SETTINGS_IMPORT_EXPORT_NAME = 'translator-revolution-dropdow-plugin-import-export-settings';
const DB_MAIN_NAME = 'translator-revolution-dropdown-main';
const DB_SETTINGS_NAME = 'translator-revolution-dropdown-settings';
const DB_CACHE_WRITABLE_FLAG_NAME = 'translator-revolution-dropdown-cache-validate';
const ENABLE_SUPPORT_TAB = true;
protected static $_settings = null;
protected static $_main_settings = null;
public static function getName($_to_lower=false, $_ui=false) {
if ($_ui)
return $_to_lower ? strtolower(self::UI_NAME) : self::UI_NAME;
else
return $_to_lower ? strtolower(self::NAME) : self::NAME;
}
public static function getWelcomeName($_to_lower=false, $_ui=false) {
if ($_ui)
return $_to_lower ? strtolower(self::UI_WELCOME_NAME) : self::UI_WELCOME_NAME;
else
return $_to_lower ? strtolower(self::WELCOME_NAME) : self::WELCOME_NAME;
}
public static function getSettingsName($_to_lower=false, $_ui=false) {
if ($_ui)
return $_to_lower ? strtolower(self::UI_SETTINGS_NAME) : self::UI_SETTINGS_NAME;
else
return $_to_lower ? strtolower(self::SETTINGS_NAME) : self::SETTINGS_NAME;
}
public static function getTranslationsName() {
return self::UI_TRANSLATIONS_NAME;
}
public static function getTranslationsHandle() {
return self::ADMIN_TRANSLATIONS_NAME;
}
public static function getWidgetName($_internal=false) {
return $_internal ? self::WIDGET_INTERNAL_NAME : self::WIDGET_NAME;
}
public static function getVersion() {
return self::VERSION;
}
public static function getAdminLanguageDomain() {
return self::ADMIN_LANGUAGE_DOMAIN;
}
public static function getAdminPageTitle() {
return __('Plugin Settings', SURSTUDIO_TRD_TEXTDOMAIN);
}
public static function getWelcomeHandle() {
return self::ADMIN_WELCOME_NAME;
}
public static function getAdminHandle() {
return self::ADMIN_SETTINGS_NAME;
}
public static function getAdminImportExportHandle() {
return self::ADMIN_SETTINGS_IMPORT_EXPORT_NAME;
}
public static function isSupportTabEnabled() {
return self::ENABLE_SUPPORT_TAB;
}
public static function getDbCacheWritableName() {
return self::DB_CACHE_WRITABLE_FLAG_NAME;
}
public static function getDbMainName() {
return self::DB_MAIN_NAME;
}
public static function getDbSettingsName() {
return self::DB_SETTINGS_NAME;
}
public static function setCacheFlag($_value) {
$name = SurStudioPluginTranslatorRevolutionDropDownConfig::getDbCacheWritableName();
$value = $_value ? 'true' : 'false';
if (!get_option($name))
add_option($name, $value);
else
update_option($name, $value);
}
public static function getCacheFlag() {
$option = get_option(SurStudioPluginTranslatorRevolutionDropDownConfig::getDbCacheWritableName());
if (!$option)
return false;
return $option == 'true';
}
public static function _get_main_settings() {
$option = get_option(SurStudioPluginTranslatorRevolutionDropDownConfig::getDbMainName());
return !$option ? array() : $option;
}
public static function _get_settings() {
$option = get_option(SurStudioPluginTranslatorRevolutionDropDownConfig::getDbSettingsName());
return !$option ? array() : $option;
}
public static function getMainSettings($_force=false) {
if (is_array(self::$_main_settings) && $_force == false)
return self::$_main_settings;
$defaults = self::getMainDefaults();
$current = self::_get_main_settings();
$result = SurStudioPluginTranslatorRevolutionDropDownCommon::mergeArrays($defaults, $current);
return self::$_settings = $result;
}
public static function getSettings($_force=false) {
if (is_array(self::$_settings) && $_force == false)
return self::$_settings;
$defaults = self::getDefaults();
$current = self::_get_settings();
$result = SurStudioPluginTranslatorRevolutionDropDownCommon::mergeArrays($defaults, $current);
$result = self::_adjust_languages($result, $current);
return self::$_settings = $result;
}
protected static function _adjust_languages($_settings, $_current) {
$result = $_settings;
if (array_key_exists('languages', $_current))
$result['languages']['value'] = $_current['languages']['value'];
return $result;
}
public static function getMainSetting($_name, $_force=false) {
$settings = self::getMainSettings($_force);
return array_key_exists($_name, $settings) ? $settings[$_name] : null;
}
public static function getSetting($_name, $_force=false) {
$settings = self::getSettings($_force);
return array_key_exists($_name, $settings) ? $settings[$_name] : null;
}
protected static function _compare_settings($_id, $_setting_1, $_setting_2) {
if (SurStudioPluginTranslatorRevolutionDropDownCommon::endsWith($_id, '_template'))
return SurStudioPluginTranslatorRevolutionDropDownCommon::stripBreakLinesAndTabs($_setting_1['value']) == SurStudioPluginTranslatorRevolutionDropDownCommon::stripBreakLinesAndTabs($_setting_2['value']);
if ($_id == 'override')
if ($_setting_1['value'] != $_setting_2['value'] && SurStudioPluginTranslatorRevolutionDropDownValidator::isEmpty($_setting_1['value']))
return true;
if ($_id == 'languages')
return $_setting_1['value'] === $_setting_2['value'];
return $_setting_1['value'] == $_setting_2['value'];
}
protected static function _get_settings_values_for_export() {
$settings = self::_get_settings();
return count($settings) > 0 ? base64_encode(serialize($settings)) : __('No settings to export. The current settings are the default ones.', SURSTUDIO_TRD_TEXTDOMAIN);
}
public static function getSettingsValues($_force=false, $_new=true) {
$result = array();
$settings = self::getSettings($_force);
$defaults = self::getDefaults();
foreach ($settings as $key => $setting)
if ($_new == false || !self::_compare_settings($key, $setting, $defaults[$key]))
$result[$key] = array(
'value' => $setting['value'],
'option_id' => array_key_exists('option_id', $setting) ? $setting['option_id'] : null
);
return $result;
}
public static function getMainSettingValue($_name, $_force=false) {
$setting = self::getMainSetting($_name, $_force);
$result = $setting['value'];
if (SurStudioPluginTranslatorRevolutionDropDownValidator::isBool($result))
$result = $result == 'true' || $result === true;
return $result;
}
public static function getSettingValue($_name, $_force=false) {
$setting = self::getSetting($_name, $_force);
if (is_null($setting))
return $setting;
$result = $setting['value'];
if (SurStudi
没有合适的资源?快使用搜索试试~ 我知道了~
【WordPress插件】2022年最新版完整功能demo+插件v2.1 Nulled.zip
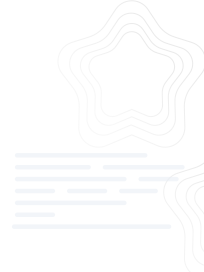
共177个文件
gif:111个
tpl:40个
png:8个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 155 浏览量
2022-04-17
18:30:14
上传
评论
收藏 259KB ZIP 举报
温馨提示
"【WordPress插件】2022年最新版完整功能demo+插件v2.1 Nulled Ajax Translator Revolution DropDown WP Plugin Ajax翻译转速下拉WP插件" ---------- 泰森云每天更新发布最新WordPress主题、HTML主题、WordPress插件、shopify主题、opencart主题、PHP项目源码、安卓项目源码、ios项目源码,更有超10000个资源可供选择,如有需要请站内联系。
资源推荐
资源详情
资源评论
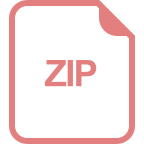
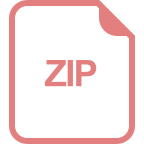
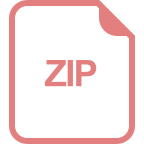
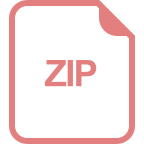
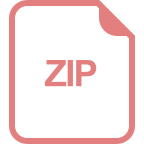
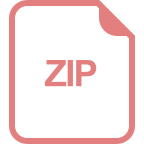
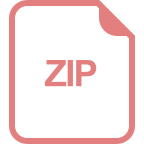
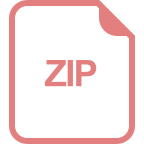
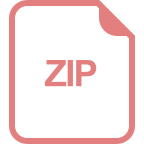
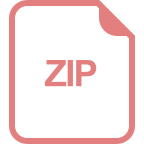
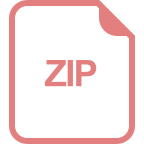
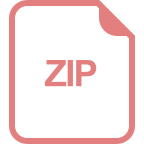
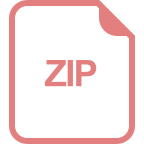
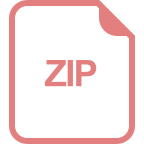
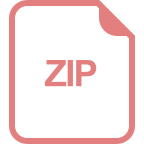
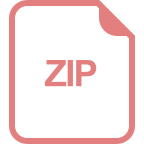
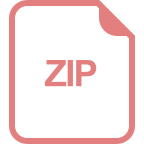
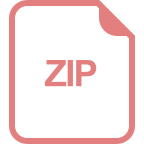
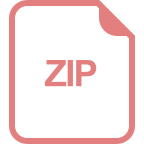
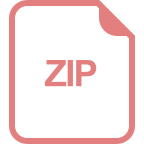
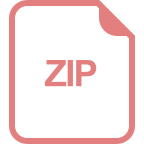
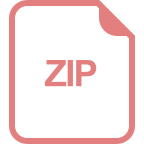
收起资源包目录

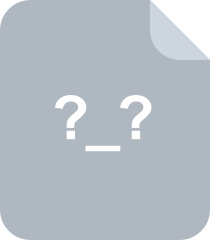
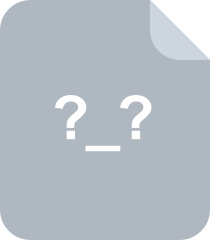
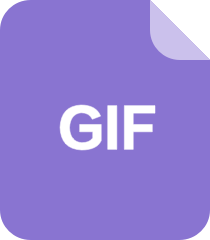
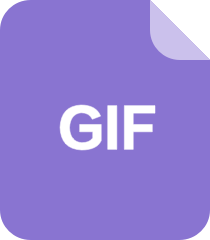
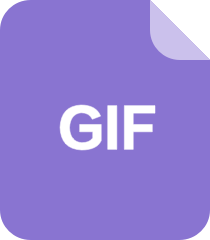
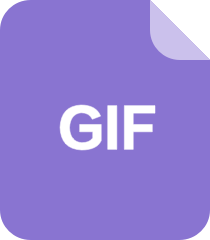
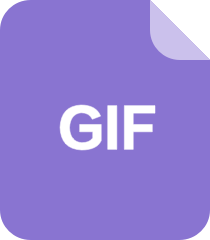
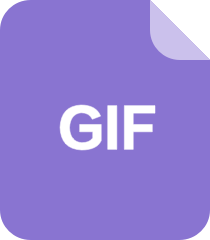
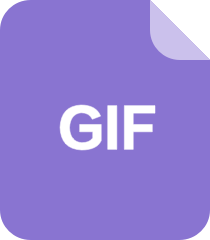
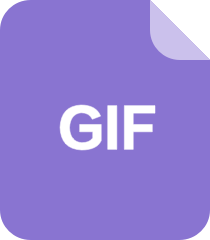
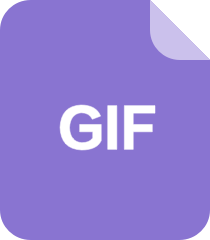
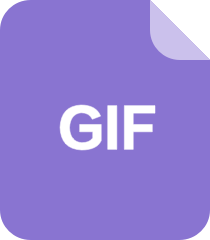
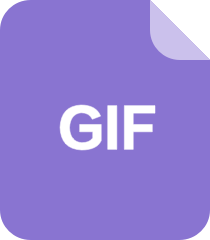
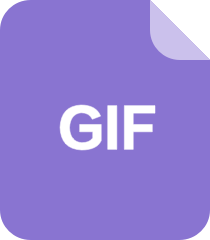
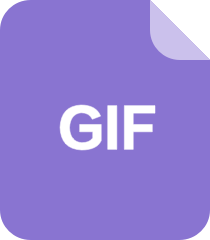
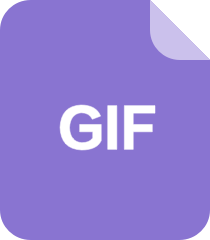
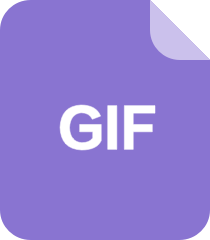
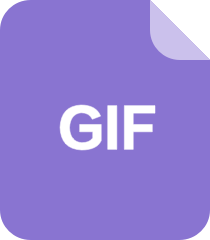
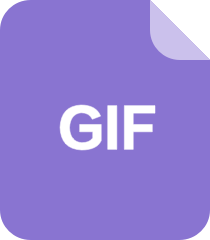
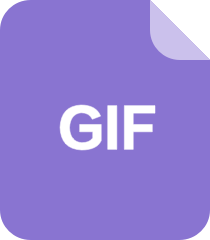
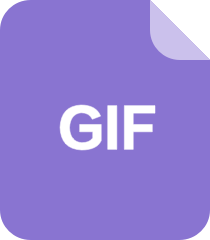
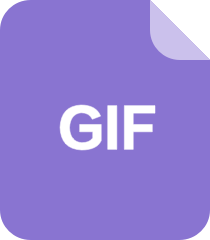
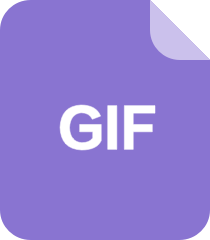
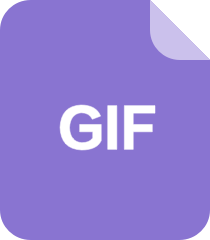
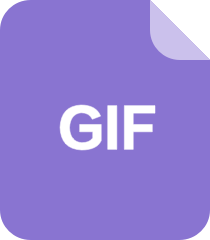
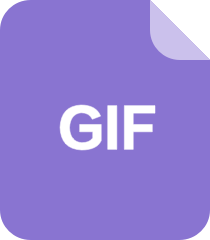
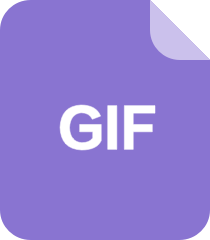
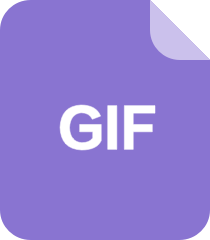
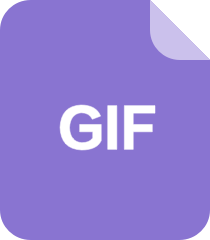
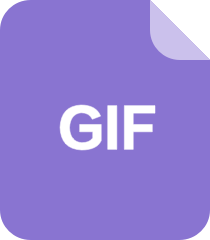
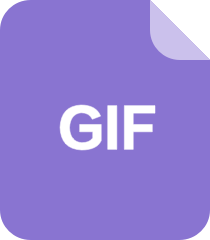
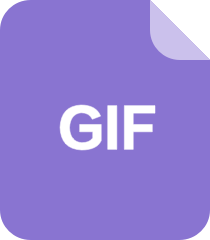
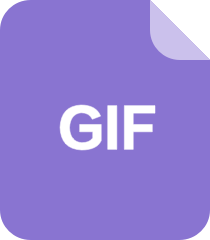
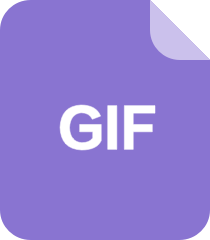
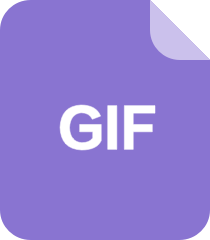
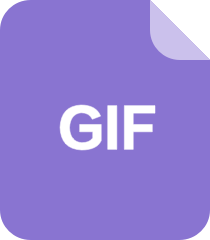
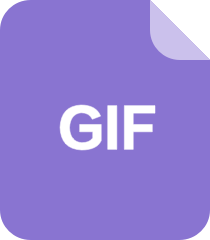
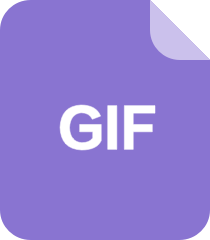
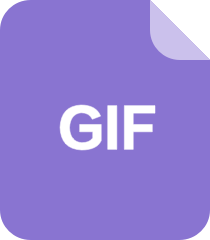
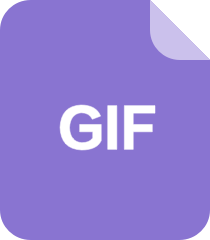
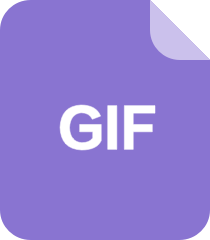
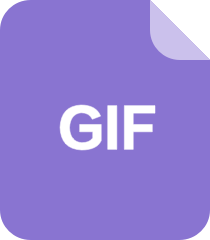
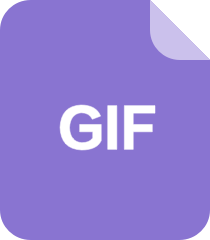
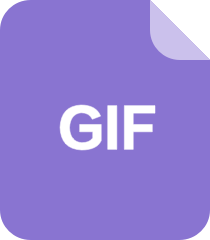
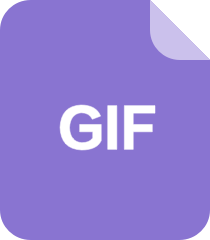
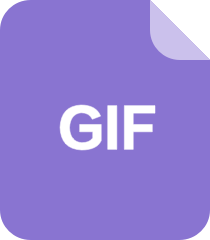
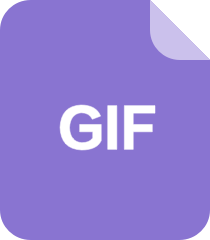
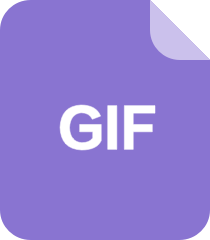
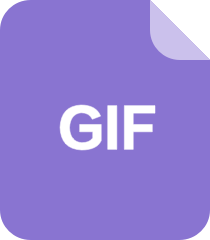
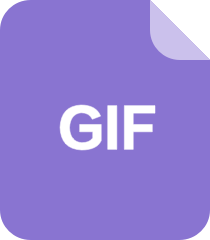
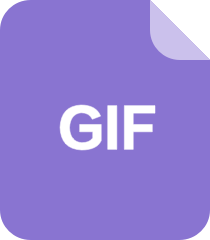
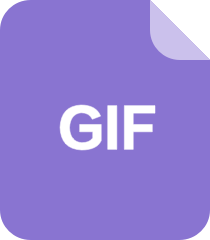
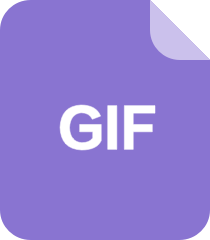
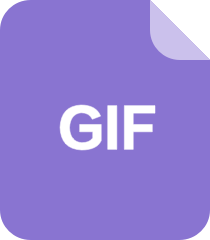
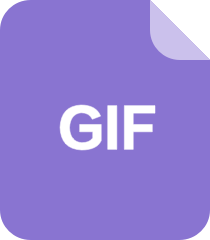
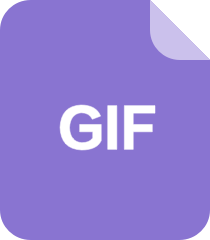
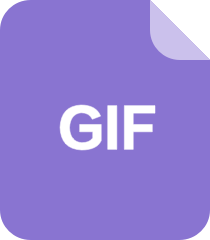
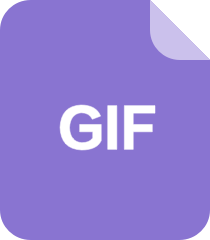
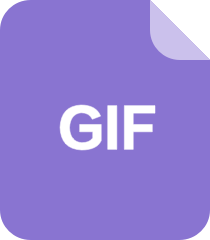
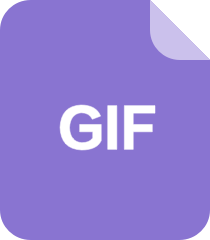
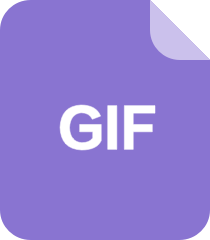
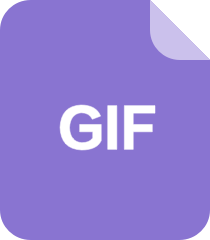
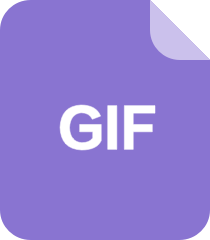
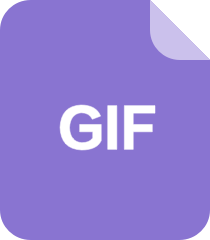
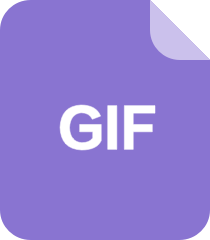
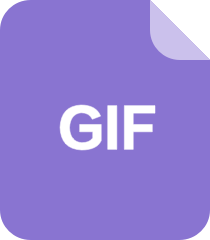
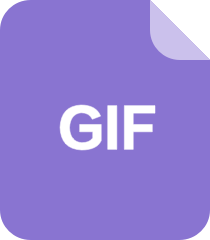
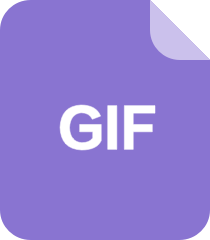
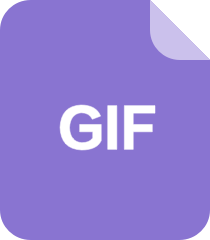
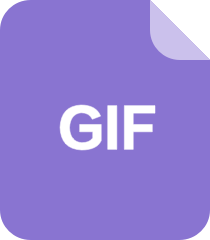
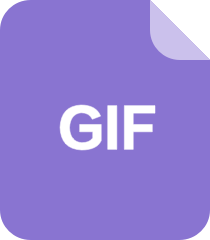
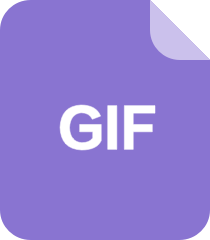
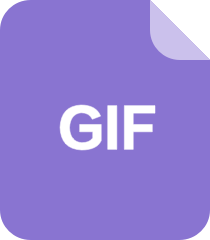
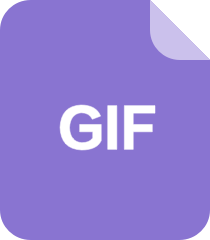
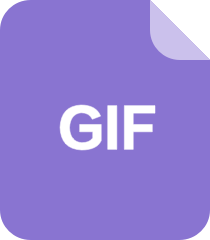
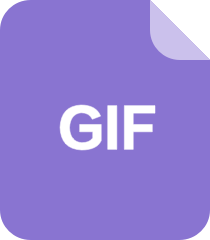
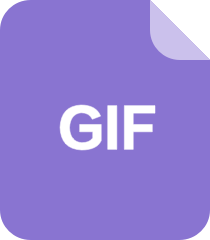
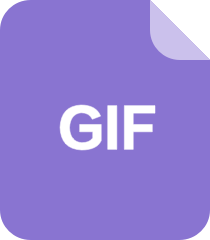
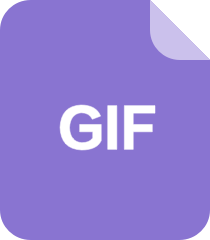
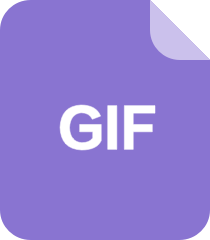
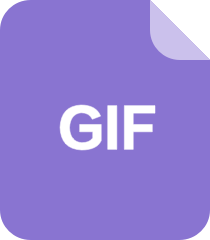
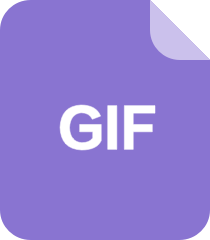
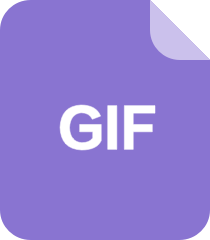
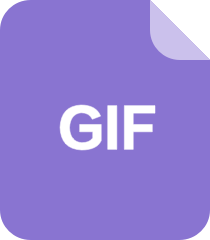
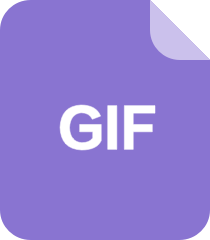
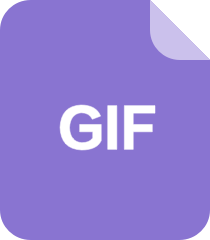
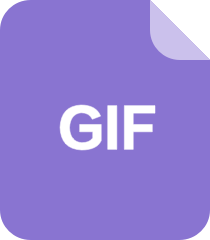
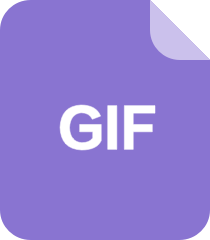
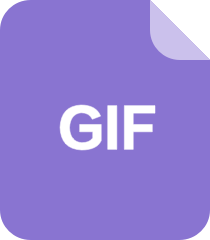
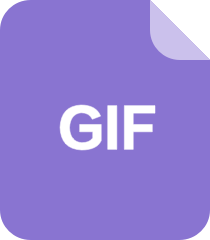
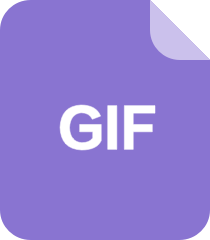
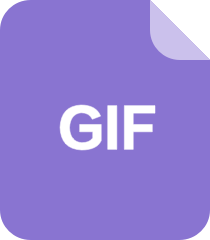
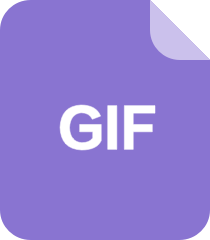
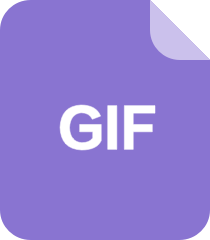
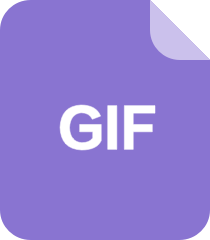
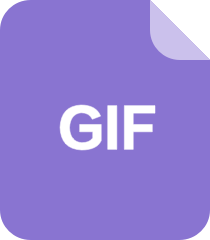
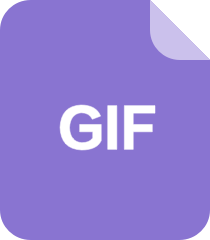
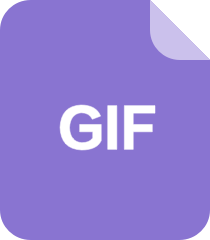
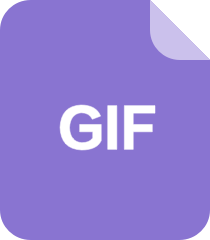
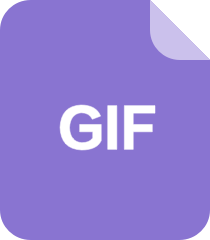
共 177 条
- 1
- 2
资源评论
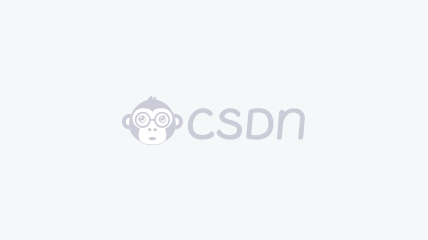

Lee达森
- 粉丝: 1515
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

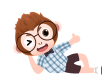
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


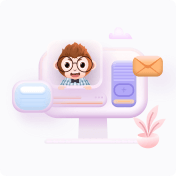
安全验证
文档复制为VIP权益,开通VIP直接复制
