<?php
if(!class_exists('pwaforwp_ptafp')){
require_once PWAFORWP_PTAFP_PLUGIN_DIR."/inc/common_authentication.php";
class pwaforwp_ptafp{
public $settings = array();
public $ptap_authentication;
public function __construct(){
add_action("admin_init", array( $this, 'init' ) );
add_action( 'plugin_action_links_' . plugin_basename( PWAFORWP_PTAFP_DIR_NAME_FILE ), array($this, 'plugin_action_links') );
if(function_exists('pwaforwp_defaultSettings')){
$this->settings = pwaforwp_defaultSettings();
}else{ $this->settings = array(); }
$this->ptap_authentication = new ptap_common_authentication();
add_action("admin_enqueue_scripts", array($this , 'script_enqueue'));
//on save
add_action( "update_option_pwaforwp_settings", array($this, 'on_options_update') ,10,3);
add_action("wp_ajax_pwa_to_apk_plugin_caller", array($this, "pwa_to_apk_plugin_gen"));
add_action("wp_ajax_pwa_to_apk_plugin_project_caller", array($this, "pwa_to_apk_plugin_project"));
add_action("wp_ajax_pwa_to_apk_assets_deeeplink", array($this, "pwa_to_apk_assets_deeeplink"));
add_action("wp_ajax_pwaforwp_apk_keystore_upload", array($this, "pwaforwp_apk_keystore_upload"));
add_action("wp_ajax_pwa_to_apk_assets_removeold", array($this, "pwa_to_apk_assets_removeold"));
}
public function on_options_update($old_value, $value, $option){
$pwa_pwatapk = get_option("pwa_pwatapk", true);
if(!is_array($pwa_pwatapk)){ $pwa_pwatapk = array(); }
if(isset($pwa_pwatapk["user_authentication"]) && $pwa_pwatapk["user_authentication"]==1){
return true;
}
if( (isset($value['ptafp_addon_license_key'])
&& isset($value['ptafp_addon_license_key_status'])
&& $value['ptafp_addon_license_key_status'] == 'active'
) && isset($value['pwa_to_apk_plugin'])
){
/*$data = array(
'license_key' => $value['ptafp_addon_license_key'],
'license_status'=> $value['ptafp_addon_license_key_status'],
'referrer'=> get_home_url()
);
$response = $this->ptap_authentication->sender_request("registerSiteUser", $data);
if($response){*/
$pwa_pwatapk["user_authentication"] = 1;
update_option("pwa_pwatapk", $pwa_pwatapk);
//}
}
}
function pwa_to_apk_plugin_gen(){
$nonce = $_POST['ref'];
if(!wp_verify_nonce($nonce, 'pwa_pwatapk_nonce_gen')){
echo json_encode(array("status"=>400, "message"=>"Request not verified"));die;
}
$pwa_pwatapk = get_option("pwa_pwatapk", true);
if( (isset($pwa_pwatapk["user_authentication"]) && $pwa_pwatapk["user_authentication"]!=1)){
echo json_encode(array("status"=>400, "message"=>"Plugin not activated"));die;
}
//PWA settings
$defaults = pwaforwp_defaultSettings();
$signingMode = 'new'; $keyPassword = $keystorePassword = ''; $keystorefile = null;
$appVersionCode = 1;
$version = "1.0.0";
if(isset($defaults['apk_type']) && $defaults['apk_type']=='mine'){
$signingMode = 'mine';
$keyPassword = isset($defaults['apk_key_password'])? $defaults['apk_key_password']: '';
$keystorePassword = isset($defaults['apk_key_store_password'])? $defaults['apk_key_store_password']: '';
if(!file_exists(PWAFORWP_PTAFP_PLUGIN_DIR."/keystore/signing.keystore")){
echo json_encode(array("status"=>400, "message"=>"Keystore File not found"));die;
}else{
$keystorefile = 'data:application/octet-stream;base64,'.base64_encode(file_get_contents(PWAFORWP_PTAFP_PLUGIN_DIR."/keystore/signing.keystore"));
}
if( empty($keyPassword) ){
echo json_encode(array("status"=>400, "message"=>"Key password is empty"));die;
}
if( empty($keystorePassword) ){
echo json_encode(array("status"=>400, "message"=>"Key store password is empty"));die;
}
if(isset($defaults['apk_app_version']) && !empty($defaults['apk_app_version'])){
$version = $defaults['apk_app_version'];
}else{
echo json_encode(array("status"=>400, "message"=>"Last APK version is empty"));die;
}
if( isset($defaults['apk_app_code']) && !empty($defaults['apk_app_code']) ) {
$appVersionCode = $defaults['apk_app_code']+1;
}else{
echo json_encode(array("status"=>400, "message"=>"Last APK code is empty, ex: 1"));die;
}
if(version_compare ( $version , "1.0.0" , '>=' ) ){
//$version = $pwa_pwatapk['last_version'];
$verArr = explode(".", $version);
if($verArr[count($verArr)-1]<=9){
$verArr[count($verArr)-1] = $verArr[count($verArr)-1]+1;
}elseif($verArr[count($verArr)-2]<=9){
$verArr[count($verArr)-1] = 0;
$verArr[count($verArr)-2] = $verArr[count($verArr)-2]+1;
}elseif($verArr[count($verArr)-3]<=9){
$verArr[count($verArr)-1] = 0;
$verArr[count($verArr)-2] = 0;
$verArr[count($verArr)-3] = $verArr[count($verArr)-3]+1;
}
$version = implode(".", $verArr);
}
}
$host = pwaforwp_home_url();
/*$host = parse_url(pwaforwp_home_url(), PHP_URL_HOST);
if(strpos($host, 'www')!==false){
$host = str_replace("www.", "", $host);
} */
$packageId = $defaults['pta_package_id'];
$data = array(
"packageId"=> $packageId,
"host"=> $host,
"name"=> esc_attr($defaults['app_blog_name']),
"launcherName"=> esc_attr($defaults['app_blog_short_name']),
"themeColor"=> sanitize_hex_color($defaults['theme_color']),
"navigationColor"=> sanitize_hex_color($defaults['background_color']),
"backgroundColor"=> sanitize_hex_color($defaults['background_color']),
"startUrl"=> "/",
"webManifestUrl"=>esc_url( pwaforwp_manifest_json_url() ),
"iconUrl"=> esc_url(pwaforwp_https($defaults['icon'])),
"maskableIconUrl"=> esc_url(pwaforwp_https($defaults['splash_icon'])),
"appVersion"=> $version,
"appVersionCode"=> $appVersionCode,
"useBrowserOnChromeOS"=> true,
"splashScreenFadeOutDuration"=> 300,
"enableNotifications"=> true,
"display"=> esc_attr($defaults['display']),
"shortcuts"=> array(array(
"name"=> esc_attr($defaults['app_blog_name']),
"short_name"=> esc_attr($defaults['app_blog_short_name']),
"url"=> "/?shortcut",
"icons"=> array( /*array(
"sizes"=> "128x128",
"src"=> "/favicon.png",
) */)
)),
"signingMode"=> $signingMode,
"signing"=> array(
"file"=> $keystorefile,
"alias"=> "my-key-alias",
"fullName"=> "PWA for wp",
"organization"=> "MAGAZINE3",
"organizationalUnit"=> "Development",
"countryCode"=> "IN",
"keyPassword"=> $keyPassword,
"storePassword"=> $keystorePassword
),
"fallbackType"=> "customtabs",
);
$headers_data = array('request_url'=>esc_url(get_home_url()), 'request_license'=>sanitize_text_field($this->settings['ptafp_addon_license_key']));
set_time_limit(500);
$response = $this->ptap_authentication->sender_request("generateApkZip", $data, $headers_data, true);
if($response){
if( $response['status'] == 200){
$host = parse_url(pwaforwp_home_url(), PHP_URL_HOST);
if(strpos($host, 'www')!==false){
$host = str_replace("www.", "", $host);
}
$upload_path = wp_upload_dir();
$file_path = $upload_path['basedir']."/pwa_to_app/";
wp_mkdir_p($file_path);
$file_name = $host."-".$version."-".$this->generate_random_string().'.zip';
$file_name_path = $file_path.'/'.$file_name;
$fp = file_put_contents($file_name_path, $response['body']);
$pwa_pwatapk["user_authentication"] = 1;
$pwa_pwatapk["last_version"] = $version;
$pwa_pwatapk["old_files"][] = array('name'=>$file_name_path, 'fname'=>$file_name, 'version'=>$version, 'time'=> time());
update_option("pwa_pwatapk", $pwa_pwatapk);
$this->pwa_to_apk_assets_deeeplink($version);
$fileUrl = $upload_path['baseurl']."/pwa_to_app/".$file_name;
echo json_encode(array("status"=>200, "message"=> "apk downloaded <a href

Lee达森
- 粉丝: 1559
- 资源: 1万+
最新资源
- 基于CNN卷积神经网络的网络入侵检测python源码+全部数据(高分毕业设计)
- 2-菜单栏系统状态监视工具Stats v2.11.9,支持监测内存、硬盘、网络等状态
- 火焰烟雾检测测试视频,fire-detect-video
- SAM实验.........
- boost序列化x86和x64兼容
- 脉振方波高频注入代码+增强型滑膜esmo代码,永磁同步电机高频注入程序 资料为C代码一份,大厂代码,可运行,经典流传; 配套一篇代码对应的说明文档,详细算法说明; 脉振方波注入方法相对于脉振正弦信号注
- SQLite数据库浏览器可视化工具-v3.13.1-win32
- 2024年福建省村级(居委会)行政区划shp数据集
- 基于pytorch实现minist手写数字识别源码+数据集(高分项目).zip
- 数字图像处理系统的Python实现:集成功能模块及人脸识别
- 编程直接实现HTML网页燃放烟花效果的代码
- 基于机器学习CNN卷积神经网络的网络入侵检测python源码+文档说明+全部数据
- 基于深度学习的VVC帧内编码中快速QTMT编码单元划分方法
- 15款L1218L1258L1259L3218L3219L3251L3253L3255L3256L3258L3266L3267L3268L3269L5298清零软 图解
- comsol枝晶生长 模型包括:典型,形状成核,随机成核,均匀沉积,雪花晶形成过程 适用于电池,电化学沉积,催化的模拟学习
- 基于javaweb实现的SQL基于JSP的学生信息管理系统源码+数据库.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


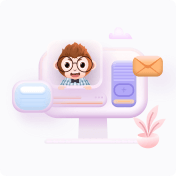