# Customizer Library
A helpful library for working with the WordPress customizer.
## About
The customizer allows WordPress developers to add options for themes and plugins, but it should be easier to work with. This library abstracts out some of the complexity.
Instead of adding options to the $wp_customize object directly, developers can just define an array of controls and sections and pass it to the Customizer_Library class.
To see how this works in practice, please see the [Customizer Library Demo](https://github.com/devinsays/customizer-library-demo) theme.
The Customizer Library adds sections, settings and controls to the customizer based on the array that gets passed to it. There is default sanitization for all options (though you're still welcome to pass a sanitize_callback). All options are also saved by default as theme_mods (though look for a future update to make this more flexible).
At the moment there is only one custom control (for textarea), but look for additional controls as the library matures.
The Customizer Library includes additional classes and helper functions for creating inline styles and loading Google fonts. These functions and classes were developed by [The Theme Foundry](https://thethemefoundry.com/) for their theme [Make](https://thethemefoundry.com/wordpress-themes/make/) and I've found them quite useful in my own projects. However, I'm considering moving them into seperate modules in order to make the core library as focused as possible. Feedback on this is welcome.
## Installation
The [Customizer Library](https://github.com/devinsays/customizer-library) can be included in your own projects git submodule if you'd like to be able to pull down changes. To include it in your own projects the same way, navigate to the directory and use:
`git submodule add git@github.com:devinsays/customizer-library customizer-library`
## Options
The Customizer Library currently supports these options:
* Checkbox
* Select
* Radio
* Upload
* Image
* Color
* Text
* URL
* Range
* Textarea
* Select (Typography)
### Sections
Sections are convenient ways to group controls in the customizer.
Customizer Sections can be defined like this:
~~~php
// Example Section
$sections[] = array(
'id' => 'example', // Required
'title' => __( 'Example Section', 'textdomain' ), // Required
'priority' => '30', // Optional
'description' => 'Example description', // Optional
'panel' => 'panel_id' // optional, and it requires WP >= 4.0
);
~~~
### Panels
Panels are a convenient way to group your different sections.
Here's an example that adds a panel, a section to the panel, and then a text option to that section:
~~~php
// Panel Example
$panel = 'panel';
$panels[] = array(
'id' => $panel,
'title' => __( 'Panel Examples', 'demo' ),
'priority' => '100'
);
$section = 'panel-section';
$sections[] = array(
'id' => $section,
'title' => __( 'Panel Section', 'demo' ),
'priority' => '10',
'panel' => $panel
);
$options['example-panel-text'] = array(
'id' => 'example-panel-text',
'label' => __( 'Example Text Input', 'demo' ),
'section' => $section,
'type' => 'text',
);
~~~
The Customizer_Library uses the core function `$wp_customize->add_panel( $id, $args );` to add panels, and all the same $args are available. See [codex](https://developer.wordpress.org/reference/classes/wp_customize_manager/add_panel/).
### Text
~~~php
$options['example-text'] = array(
'id' => 'example-text',
'label' => __( 'Example Text Input', 'textdomain' ),
'section' => $section,
'type' => 'text',
);
~~~
### URL
~~~php
$options['example-url'] = array(
'id' => 'example-url',
'label' => __( 'Example URL Input', 'textdomain' ),
'section' => $section,
'type' => 'url',
);
~~~
### Checkbox
~~~php
$options['example-checkbox'] = array(
'id' => 'example-checkbox',
'label' => __( 'Example Checkbox', 'textdomain' ),
'section' => $section,
'type' => 'checkbox',
'default' => 0,
);
~~~
### Select
~~~php
$choices = array(
'choice-1' => 'Choice One',
'choice-2' => 'Choice Two',
'choice-3' => 'Choice Three'
);
$options['example-select'] = array(
'id' => 'example-select',
'label' => __( 'Example Select', 'textdomain' ),
'section' => $section,
'type' => 'select',
'choices' => $choices,
'default' => 'choice-1'
);
~~~
### Drop Down Pages
$options['example-dropdown-pages'] = array(
'id' => 'example-dropdown-pages',
'label' => __( 'Example Drop Down Pages', 'textdomain' ),
'section' => $section,
'type' => 'dropdown-pages',
'default' => ''
);
~~~
### Radio
~~~php
$choices = array(
'choice-1' => 'Choice One',
'choice-2' => 'Choice Two',
'choice-3' => 'Choice Three'
);
$options['example-radio'] = array(
'id' => 'example-radio',
'label' => __( 'Example Radio', 'textdomain' ),
'section' => $section,
'type' => 'radio',
'choices' => $choices,
'default' => 'choice-1'
);
~~~
### Upload
~~~php
$options['example-upload'] = array(
'id' => 'example-upload',
'label' => __( 'Example Upload', 'demo' ),
'section' => $section,
'type' => 'upload',
'default' => '',
);
~~~
### Color
~~~php
$options['example-color'] = array(
'id' => 'example-color',
'label' => __( 'Example Color', 'demo' ),
'section' => $section,
'type' => 'color',
'default' => $color // hex
);
~~~
### Textarea
~~~php
$options['example-textarea'] = array(
'id' => 'example-textarea',
'label' => __( 'Example Textarea', 'demo' ),
'section' => $section,
'type' => 'textarea',
'default' => __( 'Example textarea text.', 'demo'),
);
~~~
### Select (Typography)
~~~php
$options['example-font'] = array(
'id' => 'example-font',
'label' => __( 'Example Font', 'demo' ),
'section' => $section,
'type' => 'select',
'choices' => customizer_library_get_font_choices(),
'default' => 'Monoton'
);
~~~
### Range
~~~php
$options['example-range'] = array(
'id' => 'example-range',
'label' => __( 'Example Range Input', 'demo' ),
'section' => $section,
'type' => 'range',
'input_attrs' => array(
'min' => 0,
'max' => 10,
'step' => 1,
'style' => 'color: #0a0',
)
);
~~~
### Content
~~~php
$options['example-content'] = array(
'id' => 'example-content',
'label' => __( 'Example Content', 'textdomain' ),
'section' => $section,
'type' => 'content',
'content' => '<p>' . __( 'Content to output. Use <a href="#">HTML</a> if you like.', 'textdomain' ) . '</p>',
'description' => __( 'Optional: Example Description.', 'textdomain' )
);
~~~
### Pass $options to Customizer Library
After all the options and sections are defined, load them with the Customizer Library:
~~~php
// Adds the sections to the $options array
$options['sections'] = $sections;
$customizer_library = Customizer_Library::Instance();
$customizer_library->add_options( $options );
~~~
### Demo
A full working example can be found here:
https://github.com/devinsays/customizer-library-demo/blob/master/inc/customizer-options.php
## Styles
The Customizer Library has a helper class to output inline styles. This code was originally developed by [The Theme Foundry](https://thethemefoundry.com/) for use in [Make](https://thethemefoundry.com/wordpress-themes/make/). To see how it works, see "inc/styles.php".
CSS selector(s) and value are passed to Customizer_Library_Styles class like this:
~~~php
Customizer_Library_Styles()->add( array(
'selectors' => array(
'.primary'
),
'declarations' => array(
'color' => $color
)
) );
~~~
#### Media Queries
Media queries can also be be used with Customizer_Library_Styles. Here's an example for outputting logo-image-2x on high resolution devices.
~~~php
$setting = 'logo-image-2x';
$mod = get_theme_mod( $setting, false );
if ( $mod ) {
Customizer_Library_Styles()->add( array(
'selectors' => array(
'.logo'
),
'declarations' => array(
'background-image' => 'url(' . $mod . ')'
),
'media' => '(-webkit-min-device-pixel-ratio: 1.3),(-o-min-device-pixel-ratio: 2.
没有合适的资源?快使用搜索试试~ 我知道了~
【WordPress主题】2022年最新版完整功能demo+插件v1.2.0.zip
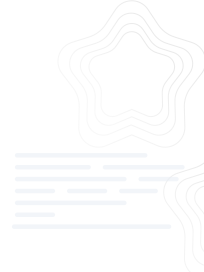
共103个文件
php:75个
css:6个
js:5个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 131 浏览量
2022-04-03
11:51:11
上传
评论
收藏 482KB ZIP 举报
温馨提示
"【WordPress主题】2022年最新版完整功能demo+插件v1.2.0 Newkarma - A special wordpress theme for magazine, news or news Indonesia websites Newkarma - 用于杂志,新闻或新闻印度尼西亚网站的特殊Wordpress主题" ---------- 泰森云每天更新发布最新WordPress主题、HTML主题、WordPress插件、shopify主题、opencart主题、PHP项目源码、安卓项目源码、ios项目源码,更有超10000个资源可供选择,如有需要请站内联系。
资源推荐
资源详情
资源评论
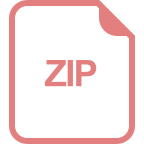
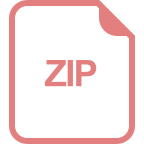
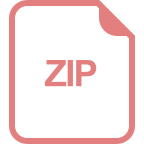
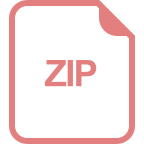
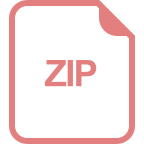
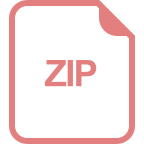
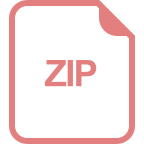
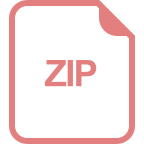
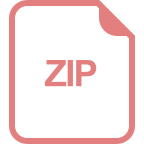
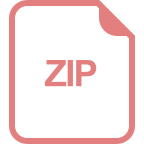
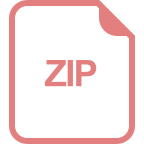
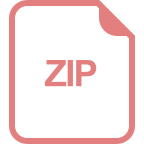
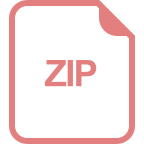
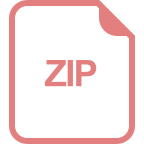
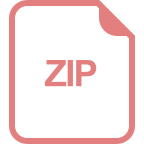
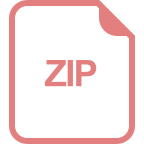
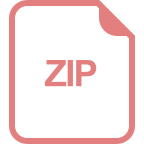
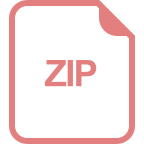
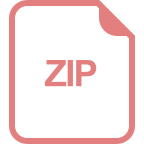
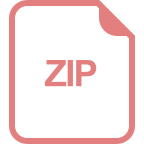
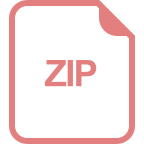
收起资源包目录

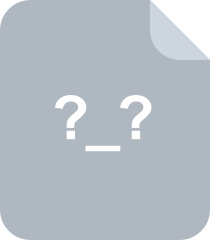
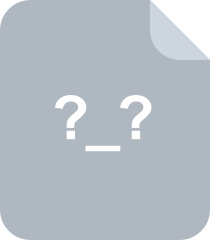
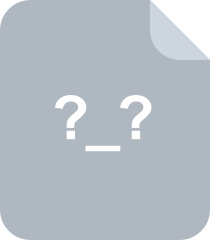
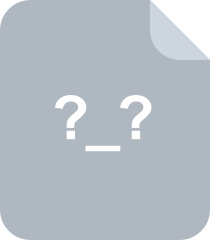
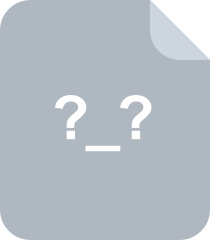
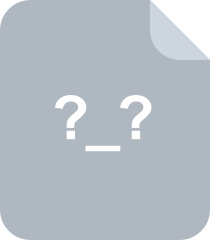
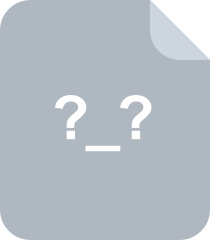
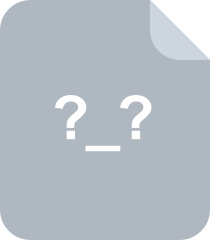
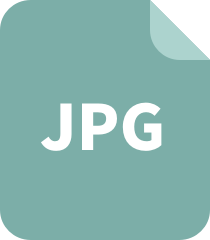
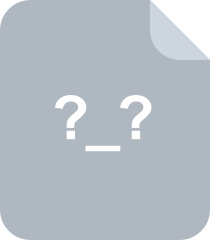
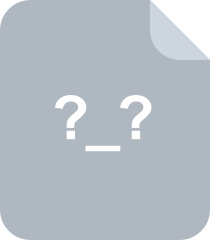
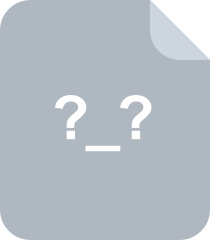
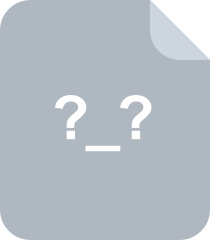
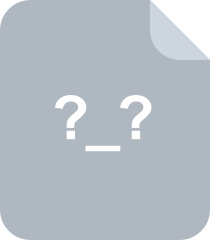
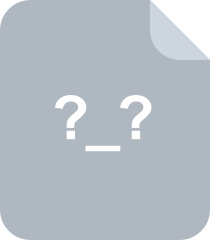
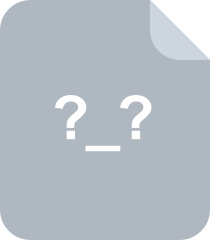
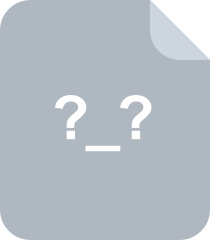
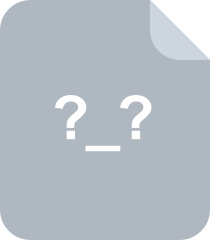
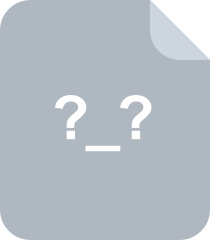
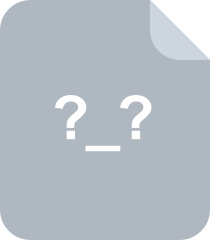
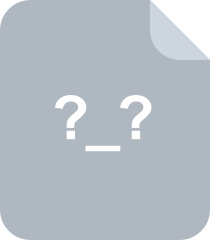
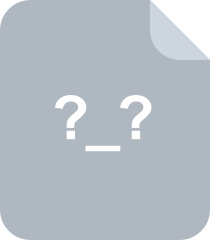
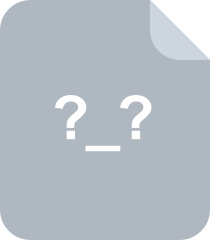
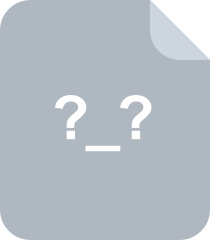
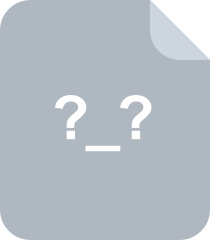
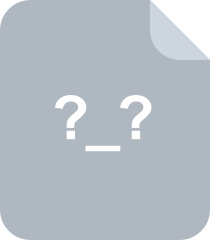
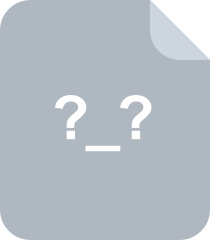
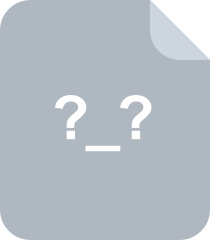
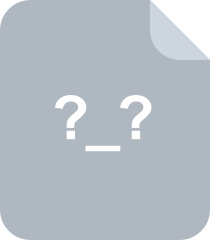
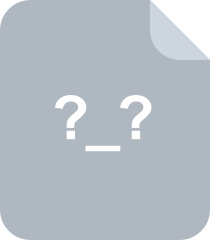
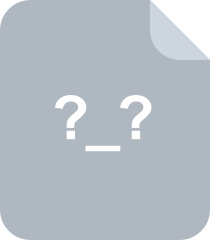
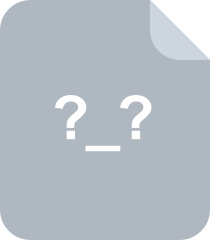
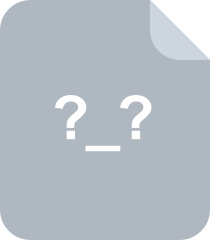
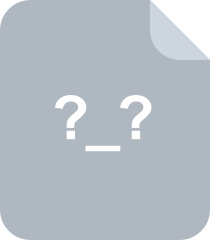
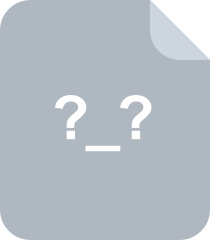
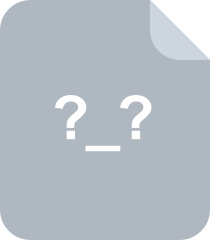
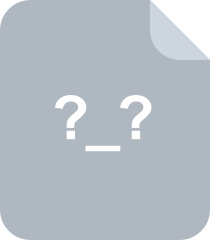
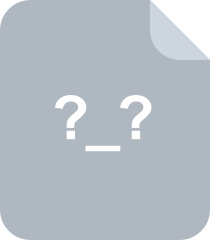
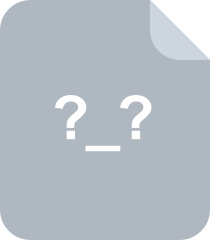
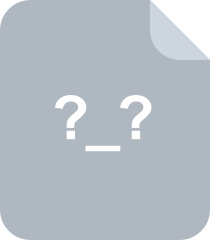
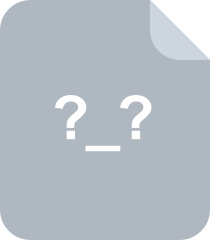
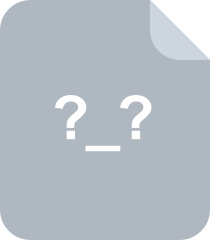
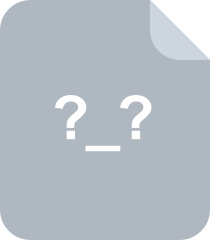
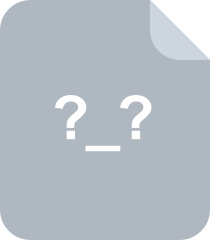
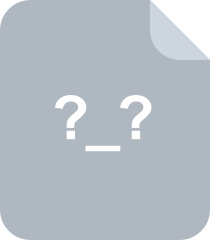
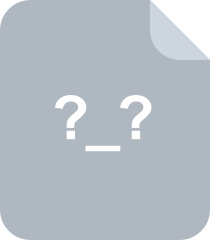
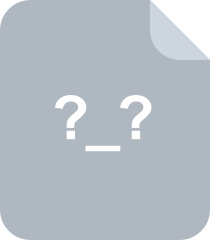
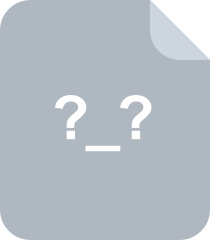
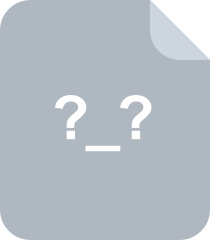
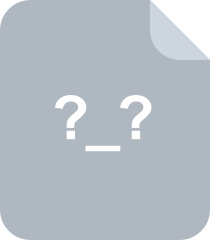
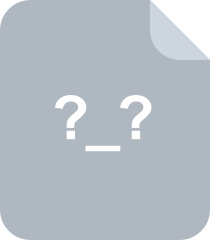
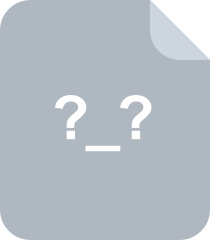
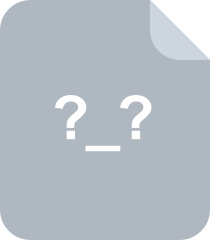
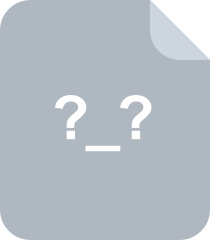
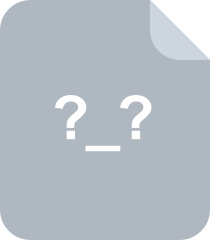
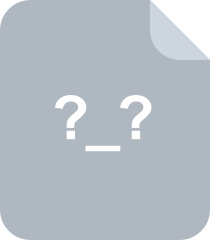
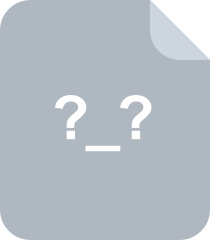
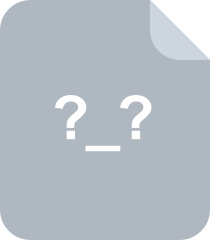
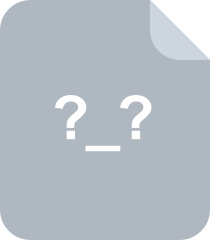
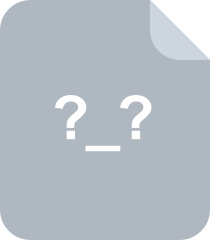
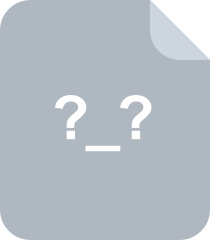
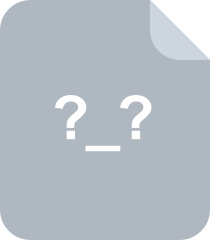
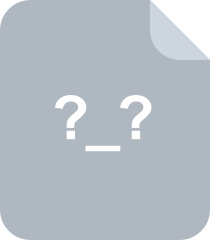
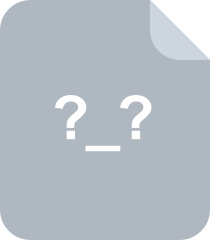
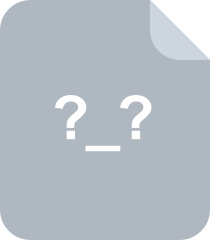
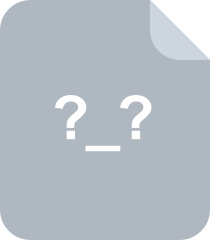
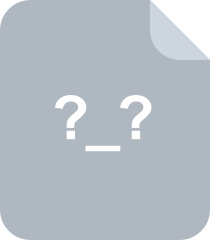
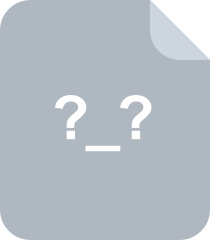
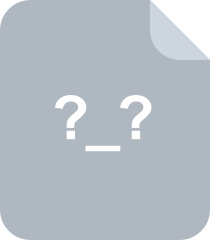
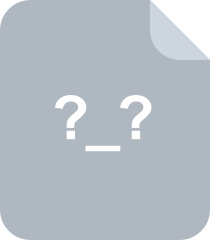
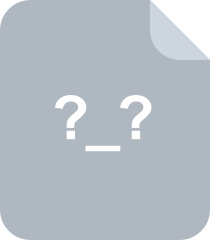
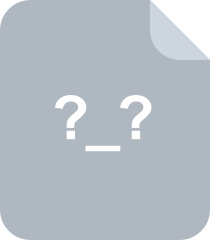
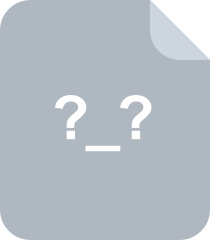
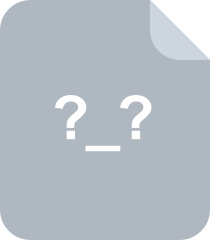
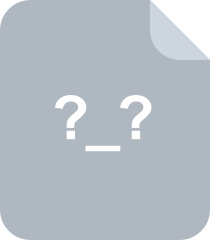
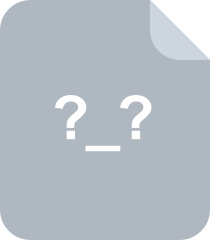
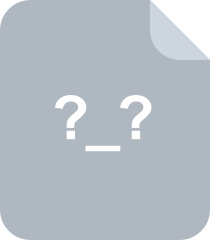
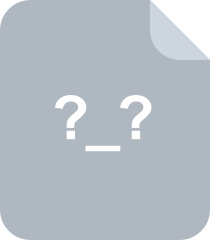
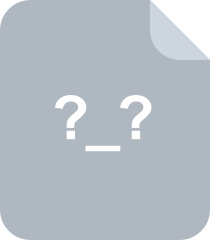
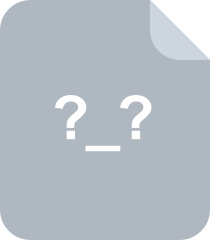
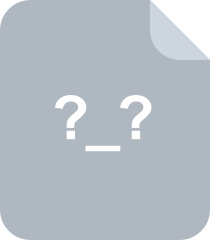
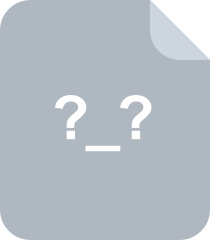
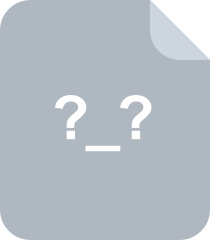
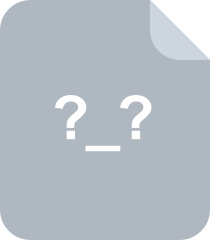
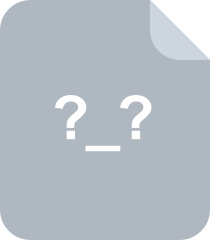
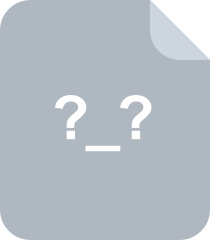
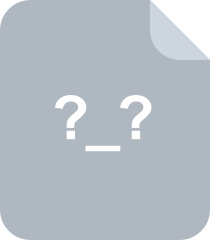
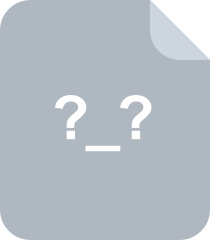
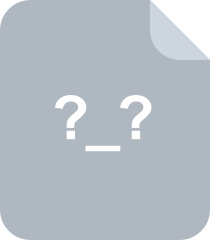
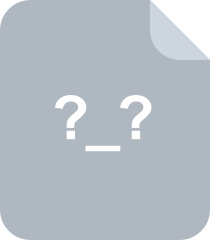
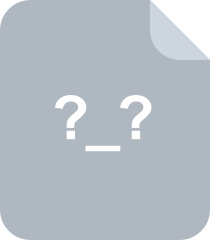
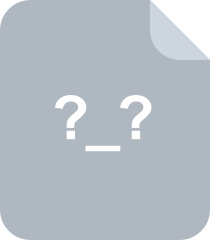
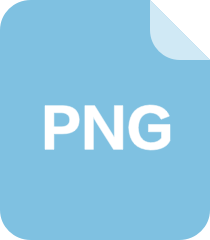
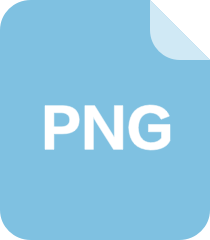
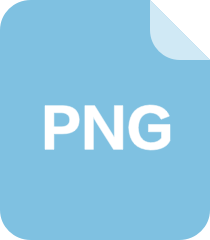
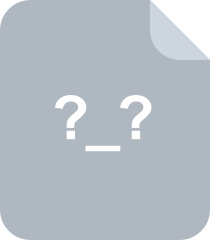
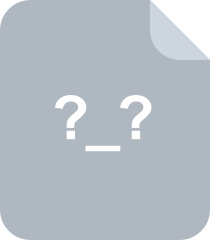
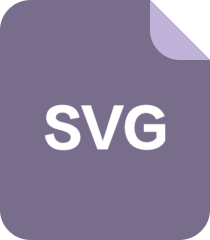
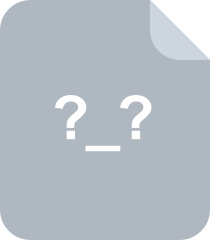
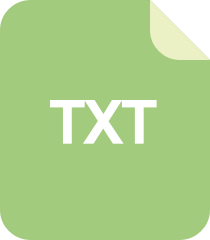
共 103 条
- 1
- 2
资源评论
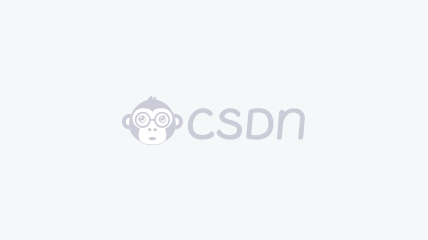

Lee达森
- 粉丝: 1556
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

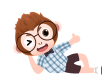
最新资源
- Oracle10gDBA学习手册中文PDF清晰版最新版本
- 扒网站数据软件项目全套技术资料100%好用.zip
- AI爬虫项目全套技术资料100%好用.zip
- 倪海厦讲义及笔记,易学数据测算
- 智能图书管理系统项目全套技术资料.zip
- 基于java写的爬虫项目全套技术资料.zip
- 218) Leverage - 创意机构与作品集 WordPress 主题 2.2.7.zip
- 220) Vinkmag - 多概念创意报纸新闻杂志 WordPress v5.0.zip
- 219) Axtra - 数字机构创意作品集主题 v2.0.zip
- 217) Voice - 清洁新闻 - 杂志 WordPress 主题 v3.0.3.zip
- 215) Classiera – 分类广告 WordPress 主题 v4.0.28.zip
- 216) Creote - 企业与咨询业务 WordPress 主题 v2.7.8.zip
- 212) Outgrid - 多用途 Elementor WordPress 主题 v2.0.0.zip
- 213) Blacksilver - 摄影 WordPress 主题 v9.4.zip
- 214) Nokri - 招聘板 WordPress 主题 v1.5.9.zip
- 211) TopDeal - 多供应商市场 WordPress 主题(移动布局就绪) v2.3.15.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


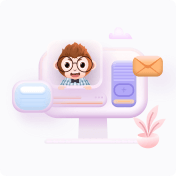
安全验证
文档复制为VIP权益,开通VIP直接复制
