<?php
if( !class_exists('Fmfw_Theme_Setup')){
class Fmfw_Theme_Setup
{
private static $_instance;
protected $page_slug;
protected $page_url;
protected $steps;
protected $step;
protected $tgmpa_instance;
protected $theme;
protected $theme_version;
protected $tgmpa_menu_slug = 'tgmpa-install-plugins';
protected $tgmpa_url = 'themes.php?page=tgmpa-install-plugins';
protected $demo_host = 'cenos.familab.net';
protected $current_host;
private $delay_posts = array();
private $delay_meta = array();
public static function instance()
{
if (!isset(self::$_instance)) {
self::$_instance = new self();
}
return self::$_instance;
}
public function __construct()
{
global $current_theme;
$this->theme_version = $current_theme->get('Version');
$this->page_slug = 'cenos-setup';
$this->page_url = 'admin.php?page=' . $this->page_slug;
$site_url = get_site_url();
$site_url_data = parse_url($site_url);
$this->current_host = $site_url_data['host'];
add_filter('woocommerce_enable_setup_wizard','__return_false');
add_action('after_switch_theme', array($this, 'switch_theme'));
if (class_exists('TGM_Plugin_Activation') && isset($GLOBALS['tgmpa'])) {
add_action('init', array($this, 'get_tgmpa_instanse'), 30);
add_action('init', array($this, 'set_tgmpa_url'), 40);
}
add_action('admin_menu', array($this, 'admin_menus'), 30);
add_action('admin_init', array($this, 'skip_admin_redirects_started_page'), 9);
//maybe_redirect_to_getting_started
add_action('admin_init', array($this, 'admin_redirects'), 30);
add_action('admin_init', array($this, 'setup_wizard'), 30);
add_filter('tgmpa_load', array($this, 'tgmpa_load'), 10, 1);
add_action('wp_ajax_cenos_setup_plugins', array($this, 'ajax_plugins'));
add_action('wp_ajax_cenos_setup_content', array($this, 'ajax_content'));
}
//define theme data for Import.
public function theme_preset_data() {
return [
[
'slug'=> 'home-01',//page slug
'thumb' => 'home1.jpg',
'header' => 'header_home_01'// header preset style
],
[
'slug'=> 'home-02',
'thumb' => 'home2.jpg',
'header' => 'header_home_02'
],
[
'slug'=> 'home-03',//page slug
'thumb' => 'home3.jpg',
'header' => 'header_home_03'// header preset style
],
[
'slug'=> 'home-04',//page slug
'thumb' => 'home4.jpg',
'header' => 'header_home_04'// header preset style
],
[
'slug'=> 'home-05',//page slug
'thumb' => 'home5.jpg',
'header' => 'header_home_05'// header preset style
],
[
'slug'=> 'home-06',//page slug
'thumb' => 'home6.jpg',
'header' => 'header_home_06'// header preset style
],
[
'slug'=> 'home-07',//page slug
'thumb' => 'home7.jpg',
'header' => 'header_home_07'// header preset style
],
[
'slug'=> 'home-09',//page slug
'thumb' => 'home9.jpg',
'header' => 'header_home_02'// header preset style
],
[
'slug'=> 'home-10',//page slug
'thumb' => 'home10.jpg',
'header' => 'header_home_10'// header preset style
],
[
'slug'=> 'home-11',//page slug
'thumb' => 'home11.jpg',
'header' => 'header_home_11'// header preset style
],
[
'slug'=> 'home-12',//page slug
'thumb' => 'home12.jpg',
'header' => 'header_home_12'// header preset style
],
[
'slug'=> 'home-13',//page slug
'thumb' => 'home13.jpg',
'header' => 'header_home_05'// header preset style
],
[
'slug'=> 'home-14',//page slug
'thumb' => 'home14.jpg',
'header' => 'header_home_14'// header preset style
]
];
}
public function theme_slider_packages(){
return ['slide-home-3','slide-home-4','slide-home-5','slide-home-6','slide-home-7','slide-home-9','slide-home-12','slide-home-13'];
}
public function tgmpa_load($status)
{
return is_admin() || current_user_can('install_themes');
}
public function switch_theme()
{
set_transient('_cenos_activation_redirect', 1);
}
public function skip_admin_redirects_started_page(){
delete_option('activate-woo-variation-swatches');
delete_transient('elementor_activation_redirect');
delete_transient('_tinvwl_activation_redirect');
}
public function admin_redirects()
{
if (!get_transient('_cenos_activation_redirect') || get_option('cenos_theme_setup_complete', false)) {
return;
}
delete_transient('_cenos_activation_redirect');
wp_safe_redirect(admin_url($this->page_url));
exit;
}
public function get_tgmpa_instanse()
{
$this->tgmpa_instance = call_user_func(array(get_class($GLOBALS['tgmpa']), 'get_instance'));
}
public function set_tgmpa_url()
{
$this->tgmpa_menu_slug = (property_exists($this->tgmpa_instance, 'menu')) ? $this->tgmpa_instance->menu : $this->tgmpa_menu_slug;
$tgmpa_parent_slug = (property_exists($this->tgmpa_instance, 'parent_slug') && $this->tgmpa_instance->parent_slug !== 'themes.php') ? 'admin.php' : 'themes.php';
$this->tgmpa_url = $tgmpa_parent_slug . '?page=' . $this->tgmpa_menu_slug;
}
public function admin_menus()
{
add_submenu_page(
'themes.php',
'Theme Setup Wizard',
'Theme Setup Wizard',
'manage_options',
$this->page_slug,
[$this, $this->page_slug]
);
}
public function init_wizard_steps()
{
$this->steps = [
'introduction' => [
'name' => 'Introduction',
'view' => [$this, 'theme_setup_introduction'],
'handler' => '',
],
];
if (class_exists('TGM_Plugin_Activation') && isset($GLOBALS['tgmpa'])) {
$this->steps['default_plugins'] = [
'name' => 'Plugins',
'view' => [$this, 'theme_setup_default_plugins'],
'handler' => '',
];
}
$this->steps['updates'] = [
'name' => 'Activate',
'view' => [$this, 'theme_setup_updates'],
'handler' => '',
];
没有合适的资源?快使用搜索试试~ 我知道了~
【WordPress主题】2022年最新版完整功能demo+插件1.2.1.zip
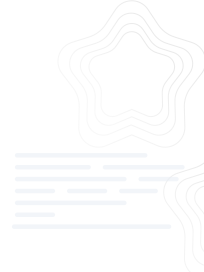
共514个文件
php:211个
jpg:158个
json:39个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 12 浏览量
2022-04-01
11:52:59
上传
评论
收藏 5.29MB ZIP 举报
温馨提示
"【WordPress主题】2022年最新版完整功能demo+插件1.2.1 Cenos - Modern Furniture WooCommerce Theme CENOS - 现代家具Woocommerce主题" ---------- 泰森云每天更新发布最新WordPress主题、HTML主题、WordPress插件、shopify主题、opencart主题、PHP项目源码、安卓项目源码、ios项目源码,更有超10000个资源可供选择,如有需要请站内联系。
资源详情
资源评论
资源推荐
收起资源包目录

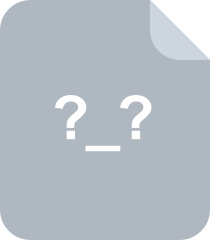
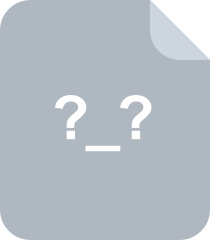
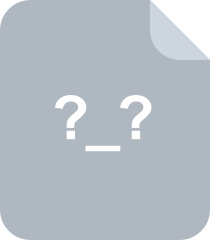
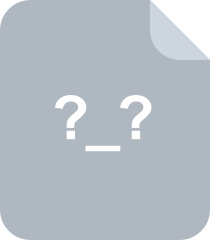
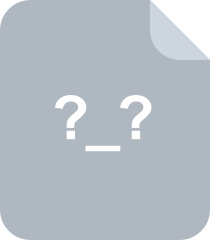
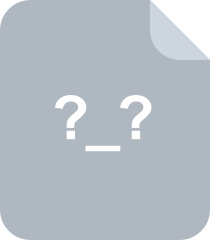
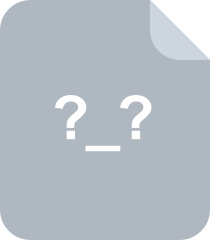
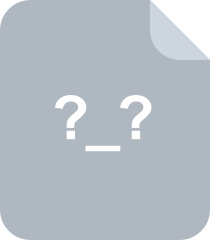
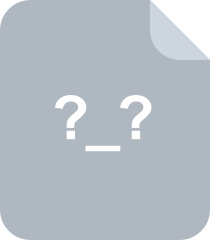
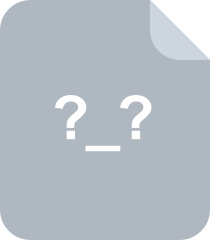
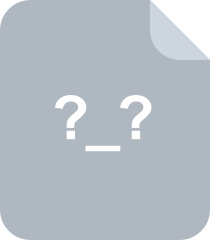
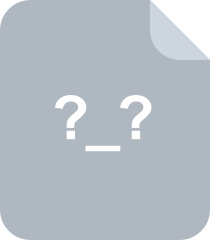
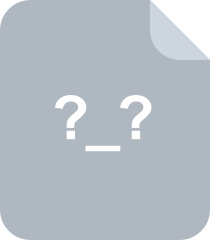
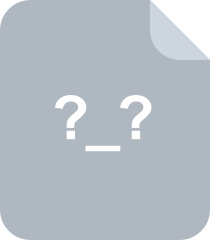
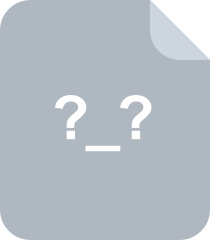
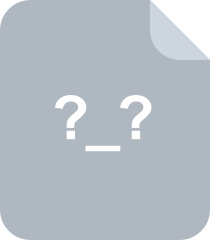
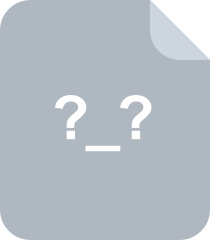
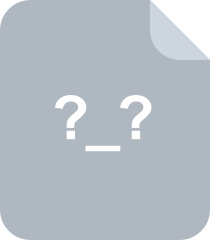
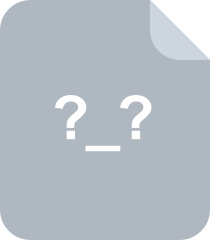
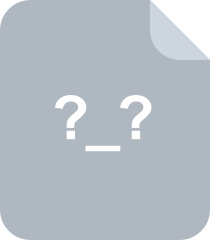
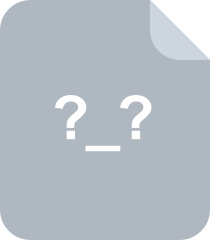
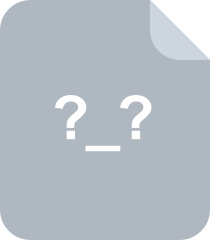
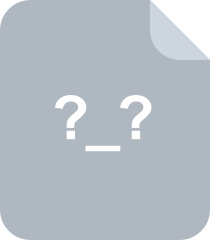
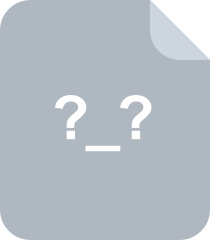
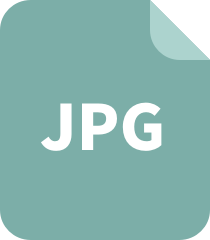
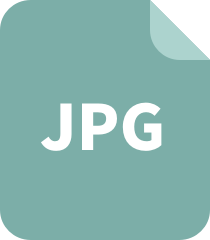
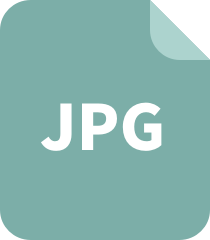
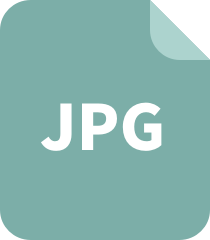
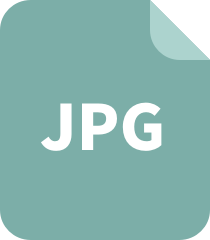
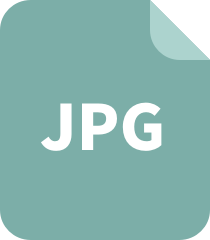
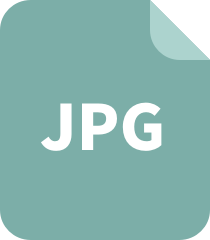
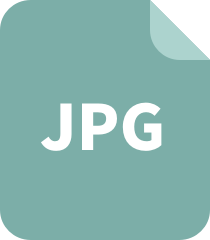
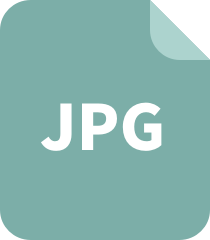
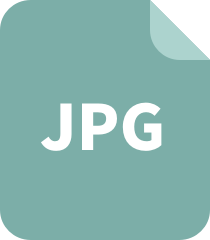
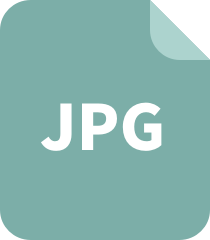
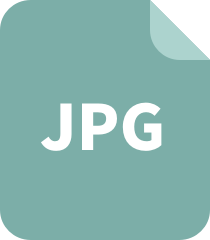
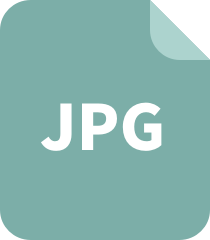
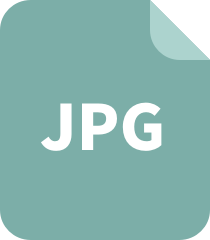
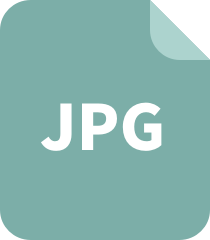
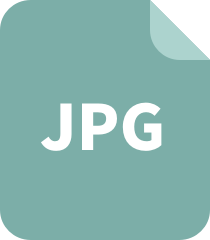
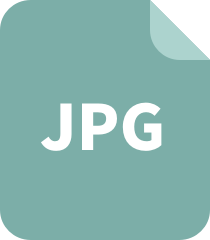
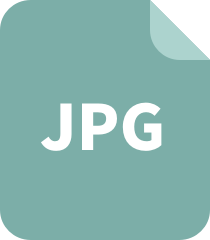
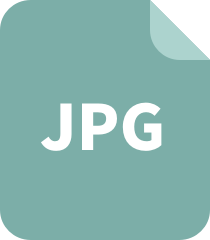
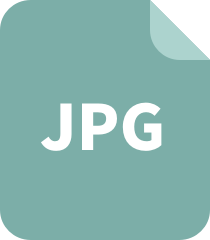
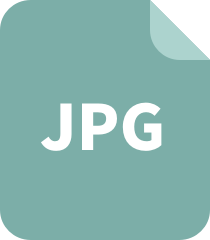
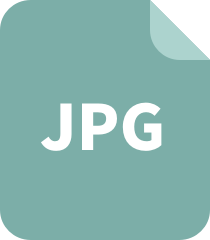
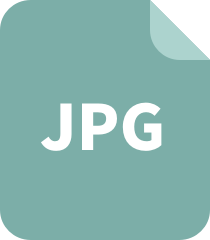
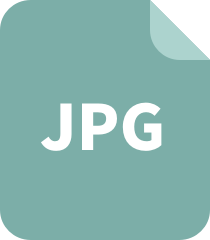
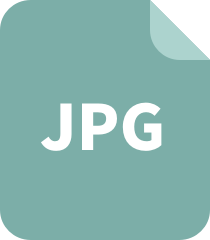
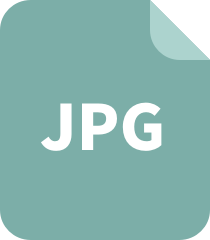
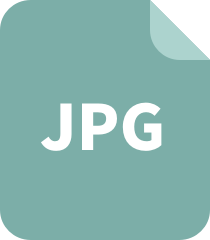
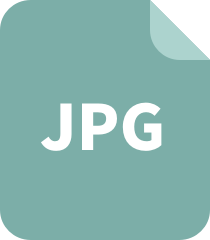
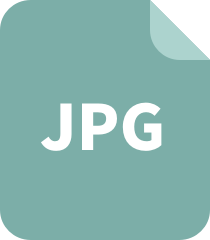
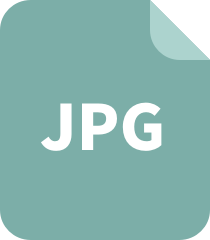
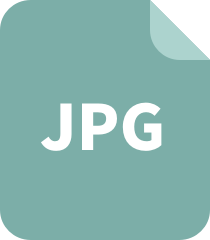
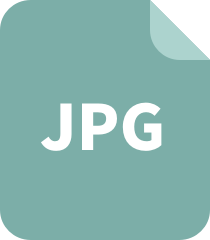
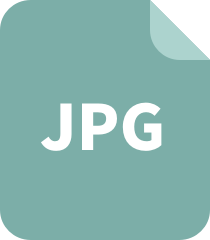
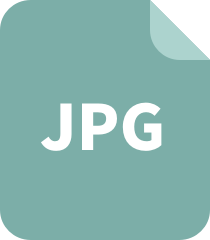
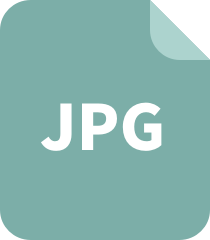
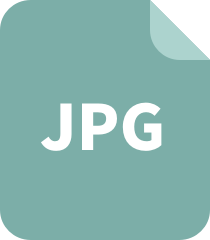
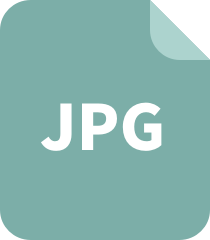
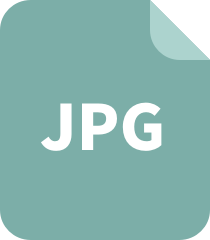
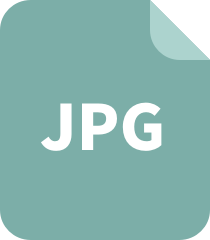
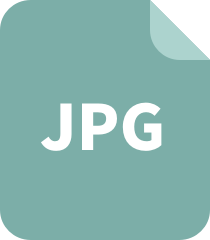
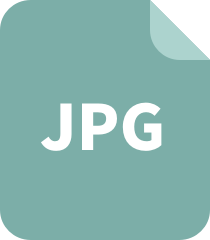
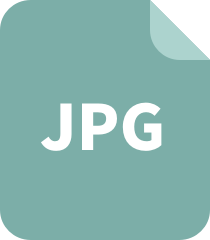
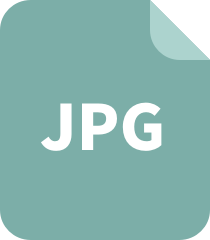
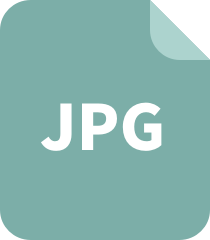
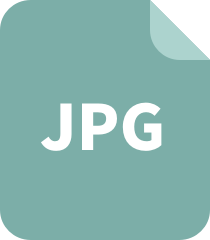
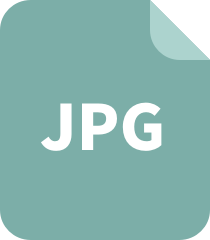
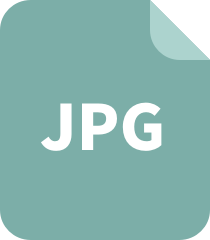
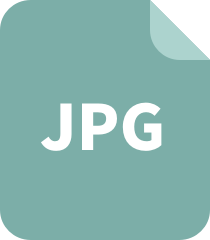
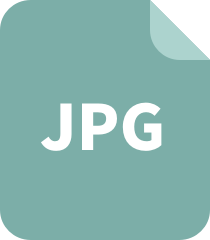
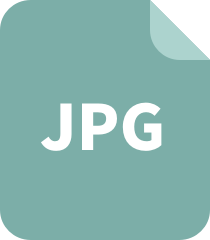
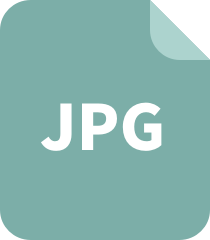
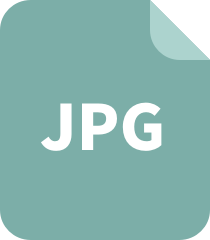
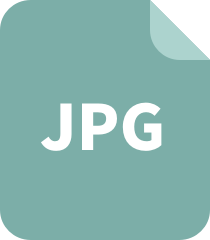
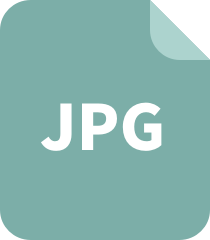
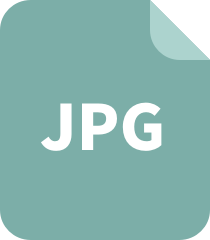
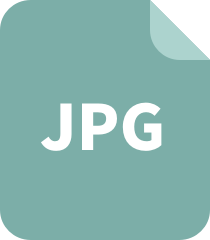
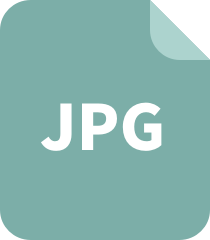
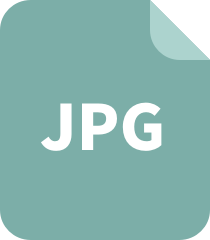
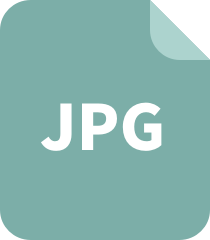
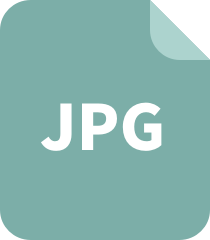
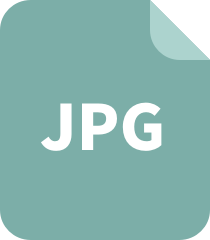
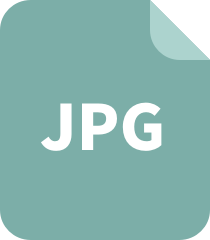
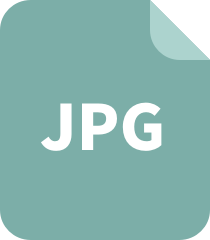
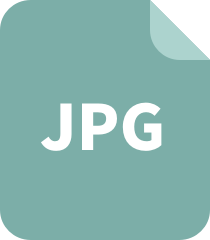
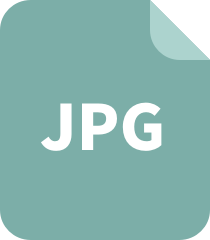
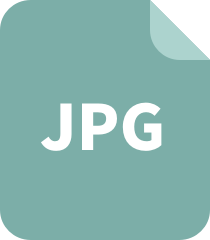
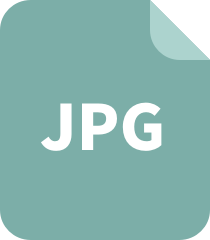
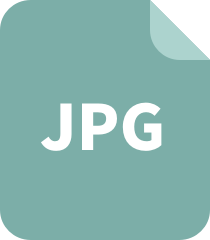
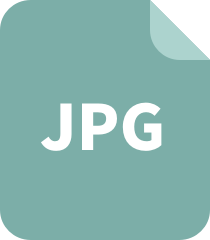
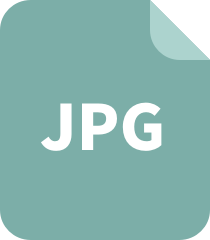
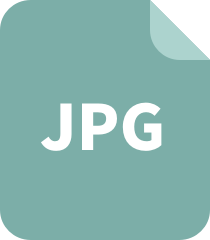
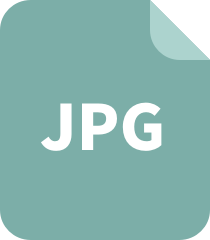
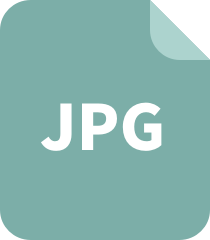
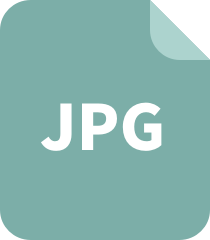
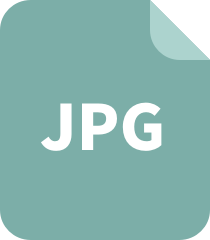
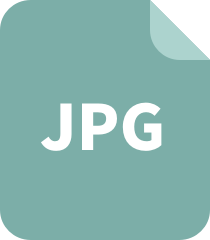
共 514 条
- 1
- 2
- 3
- 4
- 5
- 6
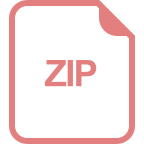
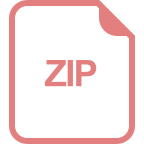
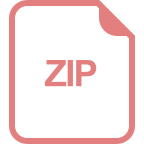
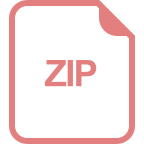
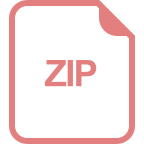
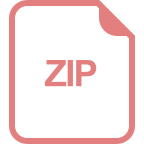
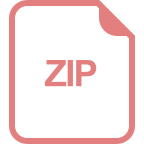
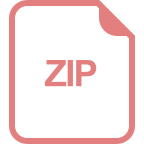
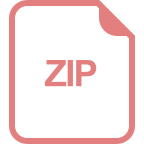
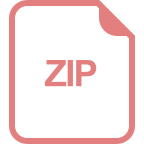
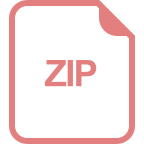
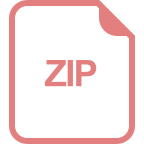
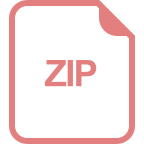
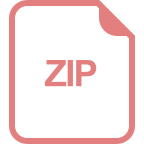
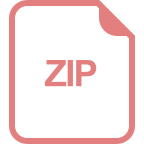
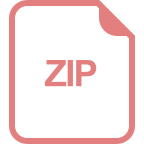
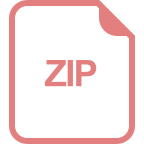
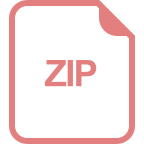
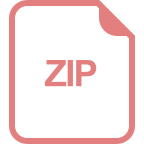
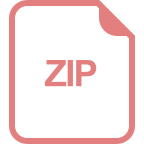
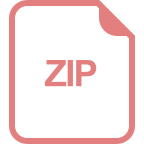
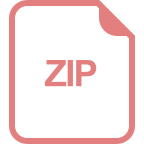
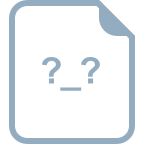

Lee达森
- 粉丝: 1501
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

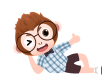
最新资源
- java销售数据决策管理系统源码数据库 MySQL源码类型 WebForm
- getchar() 函数在 C 语言中的用法.pdf
- (源码)基于JavaSwing和MySQL的航班管理系统.zip
- (源码)基于C语言的试卷管理系统.zip
- 云开发介绍与发展场景,分享给有需要的人,仅供参考
- (源码)基于Arduino框架的呼吸机控制系统.zip
- 基于Yolov5的区域人流量检测平台(源码)
- (源码)基于Arduino架构的LilyGoTTWatch智能手表系统.zip
- C# NetWorkHelper.dll,C#基于Socket封装的高性能TCP/UDP客户端服务端组件
- (源码)基于MPU9250和RTOS的自行车计算机系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


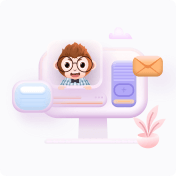
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0