/*!
* fancyBox - jQuery Plugin
* version: 2.1.5 (Fri, 14 Jun 2013)
* @requires jQuery v1.6 or later
*
* Examples at http://fancyapps.com/fancybox/
* License: www.fancyapps.com/fancybox/#license
*
* Copyright 2012 Janis Skarnelis - janis@fancyapps.com
*
*/
var basePath = "H:/workspace/test/WebContent/";
var cssBasePath = `${basePath}static/css/`;
(function (window, document, $, undefined) {
"use strict";
var H = $("html"),
W = $(window),
D = $(document),
F = $.fancybox = function () {
F.open.apply( this, arguments );
},
IE = navigator.userAgent.match(/msie/i),
didUpdate = null,
isTouch = document.createTouch !== undefined,
isQuery = function(obj) {
return obj && obj.hasOwnProperty && obj instanceof $;
},
isString = function(str) {
return str && $.type(str) === "string";
},
isPercentage = function(str) {
return isString(str) && str.indexOf('%') > 0;
},
isScrollable = function(el) {
return (el && !(el.style.overflow && el.style.overflow === 'hidden') && ((el.clientWidth && el.scrollWidth > el.clientWidth) || (el.clientHeight && el.scrollHeight > el.clientHeight)));
},
getScalar = function(orig, dim) {
var value = parseInt(orig, 10) || 0;
if (dim && isPercentage(orig)) {
value = F.getViewport()[ dim ] / 100 * value;
}
return Math.ceil(value);
},
getValue = function(value, dim) {
return getScalar(value, dim) + 'px';
};
$.extend(F, {
// The current version of fancyBox
version: '2.1.5',
defaults: {
padding : 15,
margin : 20,
width : 800,
height : 600,
minWidth : 40,
minHeight : 40,
maxWidth : 4000,
maxHeight : 4000,
pixelRatio: 1, // Set to 2 for retina display support
autoSize : true,
autoHeight : false,
autoWidth : false,
autoResize : true,
autoCenter : !isTouch,
fitToView : true,
aspectRatio : false,
topRatio : 0.5,
leftRatio : 0.5,
scrolling : 'auto', // 'auto', 'yes' or 'no'
wrapCSS : '',
arrows : true,
closeBtn : true,
closeClick : false,
nextClick : false,
mouseWheel : true,
autoPlay : false,
playSpeed : 3000,
preload : 3,
modal : false,
loop : true,
ajax : {
dataType : 'html',
headers : { 'X-fancyBox': true }
},
iframe : {
scrolling : 'auto',
preload : true
},
swf : {
wmode: 'transparent',
allowfullscreen : 'true',
allowscriptaccess : 'always'
},
keys : {
next : {
13 : 'left', // enter
34 : 'up', // page down
39 : 'left', // right arrow
40 : 'up' // down arrow
},
prev : {
8 : 'right', // backspace
33 : 'down', // page up
37 : 'right', // left arrow
38 : 'down' // up arrow
},
close : [27], // escape key
play : [32], // space - start/stop slideshow
toggle : [70] // letter "f" - toggle fullscreen
},
direction : {
next : 'left',
prev : 'right'
},
scrollOutside : true,
// Override some properties
index : 0,
type : null,
href : null,
content : null,
title : null,
// HTML templates
tpl: {
wrap : '<div class="fancybox-wrap" onclick="xuanzhuan(this)" tabIndex="-1"><div class="fancybox-skin"><div class="fancybox-outer"><div class="fancybox-inner"></div></div></div></div>',
image : '<img class="fancybox-image" src="{href}" alt="" /><script> var current = 0;function xuanzhuan(obj) {current = (current+90)%360;obj.style.transform = \'rotate(\'+current+\'deg)\';}</script>',
iframe : '<iframe id="fancybox-frame{rnd}" name="fancybox-frame{rnd}" class="fancybox-iframe" frameborder="0" vspace="0" hspace="0" webkitAllowFullScreen mozallowfullscreen allowFullScreen' + (IE ? ' allowtransparency="true"' : '') + '></iframe>',
error : '<p class="fancybox-error">The requested content cannot be loaded.<br/>Please try again later.</p>',
closeBtn : '<a title="Close" class="fancybox-item fancybox-close" href="javascript:;"></a>',
next : '<a title="Next" class="fancybox-nav fancybox-next" href="javascript:;"><span></span></a>',
prev : '<a title="Previous" class="fancybox-nav fancybox-prev" href="javascript:;"><span></span></a>'
},
// Properties for each animation type
// Opening fancyBox
openEffect : 'fade', // 'elastic', 'fade' or 'none'
openSpeed : 250,
openEasing : 'swing',
openOpacity : true,
openMethod : 'zoomIn',
// Closing fancyBox
closeEffect : 'fade', // 'elastic', 'fade' or 'none'
closeSpeed : 250,
closeEasing : 'swing',
closeOpacity : true,
closeMethod : 'zoomOut',
// Changing next gallery item
nextEffect : 'elastic', // 'elastic', 'fade' or 'none'
nextSpeed : 250,
nextEasing : 'swing',
nextMethod : 'changeIn',
// Changing previous gallery item
prevEffect : 'elastic', // 'elastic', 'fade' or 'none'
prevSpeed : 250,
prevEasing : 'swing',
prevMethod : 'changeOut',
// Enable default helpers
helpers : {
overlay : true,
title : true
},
// errorMessage
errorMessage : {
image:'The picture can not open',
content:'This content can not be resolved'
},
// Callbacks
onCancel : $.noop, // If canceling
beforeLoad : $.noop, // Before loading
afterLoad : $.noop, // After loading
beforeShow : $.noop, // Before changing in current item
afterShow : $.noop, // After opening
beforeChange : $.noop, // Before changing gallery item
beforeClose : $.noop, // Before closing
afterClose : $.noop // After closing
},
//Current state
group : {}, // Selected group
opts : {}, // Group options
previous : null, // Previous element
coming : null, // Element being loaded
current : null, // Currently loaded element
isActive : false, // Is activated
isOpen : false, // Is currently open
isOpened : false, // Have been fully opened at least once
wrap : null,
skin : null,
outer : null,
inner : null,
player : {
timer : null,
isActive : false
},
// Loaders
ajaxLoad : null,
imgPreload : null,
// Some collections
transitions : {},
helpers : {},
/*
* Static methods
*/
open: function (group, opts) {
if (!group) {
return;
}
if (!$.isPlainObject(opts)) {
opts = {};
}
// Close if already active
if (false === F.close(true)) {
return;
}
// Normalize group
if (!$.isArray(group)) {
group = isQuery(group) ? $(group).get() : [group];
}
// Recheck if the type of each element is `object` and set content type (image, ajax, etc)
$.each(group, function(i, element) {
var obj = {},
href,
title,
content,
type,
rez,
hrefParts,
selector;
if ($.type(element) === "object") {
// Check if is DOM element
if (element.nodeType) {
element = $(element);
}
if (isQuery(element)) {
obj = {
href : element.data('fancybox-href') || element.attr('href'),
title : element.data('fancybox-title') || element.attr('title'),
isDom : true,
element : element
};
if ($.metadata) {
$.extend(true, obj, element.metadata());
}
} else {
obj = element;
}
}
href = opts.href || obj.href || (isString(element) ? element : null);
title = opts.title !== undefined ? opts.title : obj.title || '';
content = opts.content || obj.content;
type = content ? 'html' : (opts.type || obj.type);
if (!type && obj.isDom) {
type = element.data('fancybox-type');
if (!type) {
rez = element.prop('class').match(/fancybox\.(\w+)/);
type = rez ? rez[1] : null;
}
}
if (isString(href)) {
// Try to guess the content type
if (!type) {
if (F.isImage(href)) {
type = 'image';
} else if (F.isSWF(href)) {
type = 'swf';
} else if (href
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
原生jQuery-fancybox不支持Base64的图片,改资源已经过改造,支持Base64格式图片(需要满足正则【data:image\/.*,】的前缀以识别Base64编码),但暂时对IE8支持不好。 之前的资源【JQuery-fancybox(支持Base64)静态资源文件】的代码存在问题,以该资源取代之。
资源推荐
资源详情
资源评论
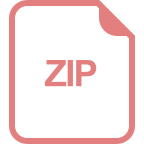
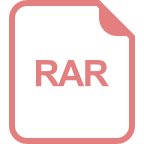
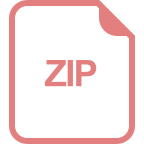
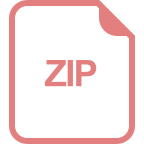
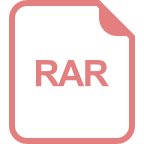
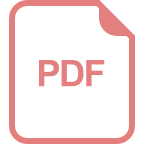
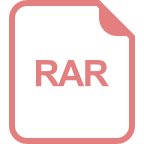
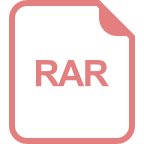
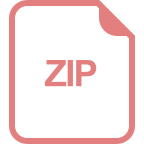
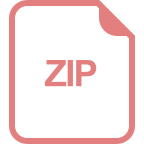
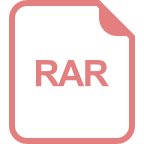
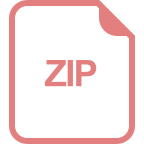
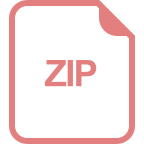
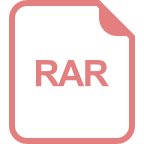
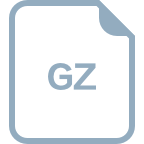
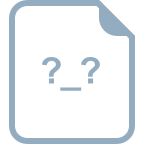
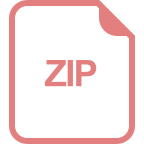
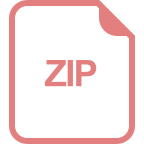
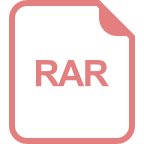
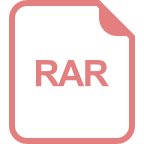
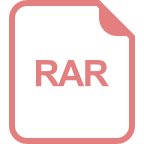
收起资源包目录


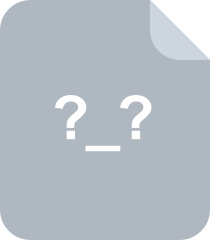

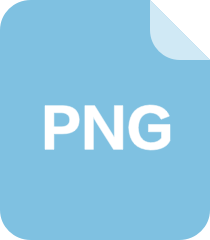
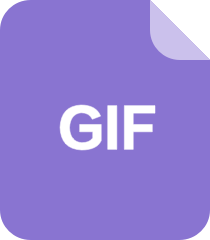
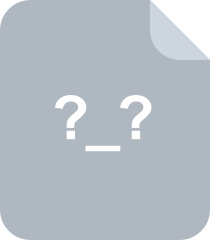
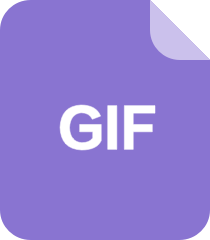
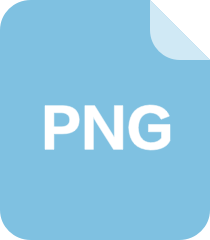
共 6 条
- 1
资源评论
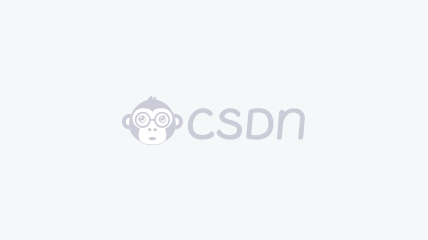

TyphoonBull
- 粉丝: 0
- 资源: 4
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

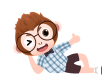
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


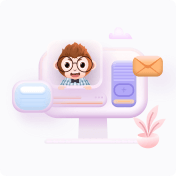
安全验证
文档复制为VIP权益,开通VIP直接复制
