CSS实现圆角,三角,五角星,五边形,爱心,12角星,8角星,圆,椭圆,圆圈,八卦等
### CSS 实现几何图形 #### 一、概述 在网页设计与开发中,利用 CSS 来绘制各种形状是非常常见的需求之一。通过简单的 CSS 属性设置,可以轻松地创建出多种多样的几何图形,如圆角矩形、三角形、圆形等。本文将详细介绍如何用 CSS 实现圆角、三角形、五角星、五边形、爱心、十二角星、八角星、圆形、椭圆形、圆圈以及八卦等图形,并提供具体的代码示例。 #### 二、基础几何图形 ##### 1. 长方形 ```css #Rectangle { width: 200px; height: 50px; background-color: red; color: white; text-align: center; } ``` - **属性解析**: - `width` 和 `height` 设置了长方形的宽度和高度。 - `background-color` 设定了背景颜色为红色。 - `color` 指定了文字的颜色。 - `text-align` 使文本居中显示。 ##### 2. 正方形 ```css #square { width: 200px; height: 200px; background-color: red; color: white; text-align: center; } ``` - **属性解析**:与长方形类似,这里设置了相同的宽度和高度来创建一个正方形。 ##### 3. 实心圆 ```css #perfect-circle { width: 200px; height: 200px; background-color: red; color: white; text-align: center; border-radius: 100%; /*-webkit-border-radius: 100%;*/ /*-moz-border-radius: 100%;*/ } ``` - **属性解析**: - 使用 `border-radius: 100%;` 可以将正方形变为圆形。 - `-webkit-` 和 `-moz-` 前缀是为了兼容不同的浏览器引擎。 ##### 4. 圆圈(空心) ```css #circle { width: 200px; height: 200px; background-color: white; color: black; text-align: center; border-radius: 100%; /*-webkit-border-radius: 100%;*/ /*-moz-border-radius: 100%;*/ border: 3px solid red; } ``` - **属性解析**:与实心圆相似,但增加了 `border` 属性以创建一个空心圆。 ##### 5. 椭圆 ```css #ellipse { width: 200px; height: 100px; background-color: red; color: white; text-align: center; border-radius: 100px / 50px; /*-webkit-border-radius: 100px / 50px;*/ /*-moz-border-radius: 100px / 50px;*/ } ``` - **属性解析**: - 使用 `border-radius: 100px / 50px;` 可以创建一个椭圆形,这里的数值代表了水平半径和垂直半径的比例。 ##### 6. 上三角形 ```css #triangle-up { width: 0px; height: 0px; color: white; text-align: center; border-left: 50px solid transparent; border-right: 50px solid transparent; border-bottom: 100px solid red; /*-webkit-border-top-left-radius: 50px solid transparent;*/ /*-webkit-border-top-right-radius: 50px solid transparent;*/ /*-webkit-border-bottom-left-radius: 100px solid red;*/ /*-webkit-border-bottom-right-radius: 100px solid red;*/ } ``` - **属性解析**: - 通过设置 `border` 的不同部分为透明和红色,可以创建出一个向上指的三角形。 ##### 7. 下三角形 ```css #triangle-down { width: 0px; height: 0px; color: white; text-align: center; border-top: 100px solid red; border-left: 50px solid transparent; border-right: 50px solid transparent; } ``` - **属性解析**:与上三角形类似,只是通过改变 `border` 的设置方向来创建一个向下指的三角形。 ##### 8. 左三角形 ```css #triangle-left { width: 0px; height: 0px; color: white; text-align: center; border-top: 50px solid transparent; border-left: 100px solid red; border-bottom: 50px solid transparent; } ``` - **属性解析**:通过改变 `border` 的设置来创建一个向左指的三角形。 ##### 9. 右三角形 ```css #triangle-right { width: 0px; height: 0px; color: white; text-align: center; border-top: 50px solid transparent; border-right: 100px solid red; border-bottom: 50px solid transparent; } ``` - **属性解析**:类似于左三角形,但是改变了 `border` 的设置以创建一个向右指的三角形。 #### 三、复杂图形 接下来我们将进一步探索如何使用 CSS 创建更加复杂的图形,如五角星、爱心、多角星等。 ##### 10. 五角星 创建一个五角星较为复杂,可以通过组合多个三角形来实现,也可以使用伪元素来简化代码: ```css .star { position: relative; display: inline-block; color: #f00; font-size: 40px; width: 0; height: 0; margin: 20px; } .star:before, .star:after { position: absolute; content: ''; display: block; width: 0; height: 0; border-left: 15px solid transparent; border-right: 15px solid transparent; } .star:before { border-bottom: 80px solid #f00; top: -40px; } .star:after { width: 30px; border-top: 50px solid #f00; border-left: 15px solid transparent; border-right: 15px solid transparent; top: 10px; left: -15px; } ``` - **属性解析**: - 使用伪元素 `:before` 和 `:after` 来创建五角星的不同部分。 - 通过调整边框的宽度和位置来形成完整的五角星形状。 ##### 11. 爱心 爱心的绘制可以通过两个叠加的圆形和一个底部的三角形来完成: ```css .heart { position: relative; width: 100px; height: 90px; } .heart::before, .heart::after { content: ""; position: absolute; top: 40px; width: 52px; height: 80px; background: red; border-radius: 50px 50px 0 0; } .heart::before { left: 50px; transform: rotate(-45deg); transform-origin: 0 100%; } .heart::after { left: 0; transform: rotate(45deg); transform-origin: 100% 100%; } .heart::after { bottom: 0; left: 25px; width: 0; height: 0; border-style: solid; border-width: 50px 30px 0 30px; border-color: red transparent transparent transparent; } ``` - **属性解析**: - 使用伪元素 `::before` 和 `::after` 来创建爱心的两个圆形部分。 - 通过旋转和定位来形成爱心的形状。 - 底部的三角形通过设置 `border` 来完成。 ##### 12. 八卦 八卦图可以视为两个叠加的圆形和其中的阴鱼和阳鱼。可以通过 SVG 或者 CSS 来实现,这里给出一个简单的 CSS 示例: ```css .guabao { width: 100px; height: 100px; position: relative; } .guabao .yin { position: absolute; width: 100%; height: 100%; clip: rect(0, 50px, 100px, 0); background: #000; border-radius: 50%; } .guabao .yang { position: absolute; width: 100%; height: 100%; clip: rect(0, 100px, 100px, 50px); background: #fff; border-radius: 50%; } .guabao .yin-circle { position: absolute; width: 30px; height: 30px; top: 35px; left: 35px; background: #fff; border-radius: 50%; } .guabao .yang-circle { position: absolute; width: 30px; height: 30px; top: 35px; right: 35px; background: #000; border-radius: 50%; } ``` - **属性解析**: - 通过设置 `clip` 属性来裁剪出半圆形状。 - 通过定位和圆形元素的叠加来形成八卦图。 #### 四、总结 以上就是利用 CSS 实现圆角、三角形、五角星、五边形、爱心、多角星、圆形、椭圆形、圆圈以及八卦等图形的方法。这些基本形状的创建为更复杂的网页布局提供了强大的支持,同时也为网页设计师提供了无限的创意空间。通过掌握这些基本技巧,你可以轻松地为你的网站添加各种视觉效果。
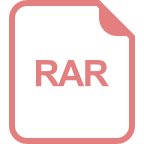
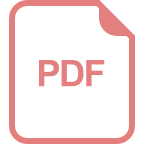
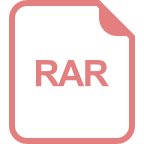
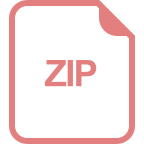
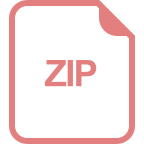
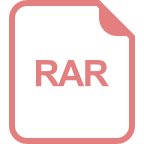
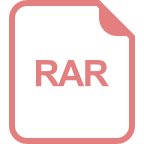
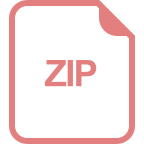
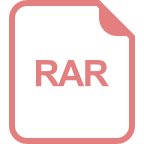
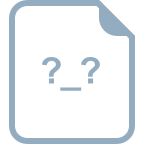
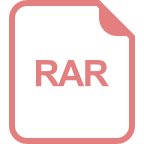
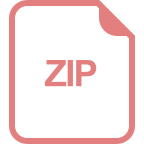
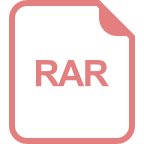
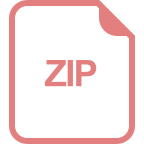
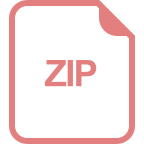
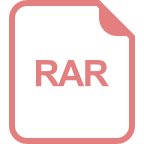
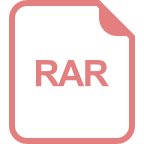
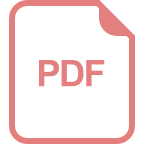
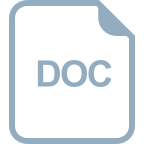
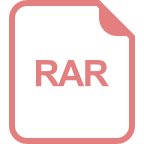
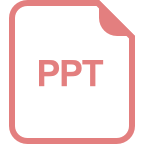
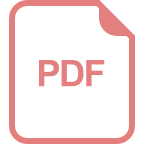
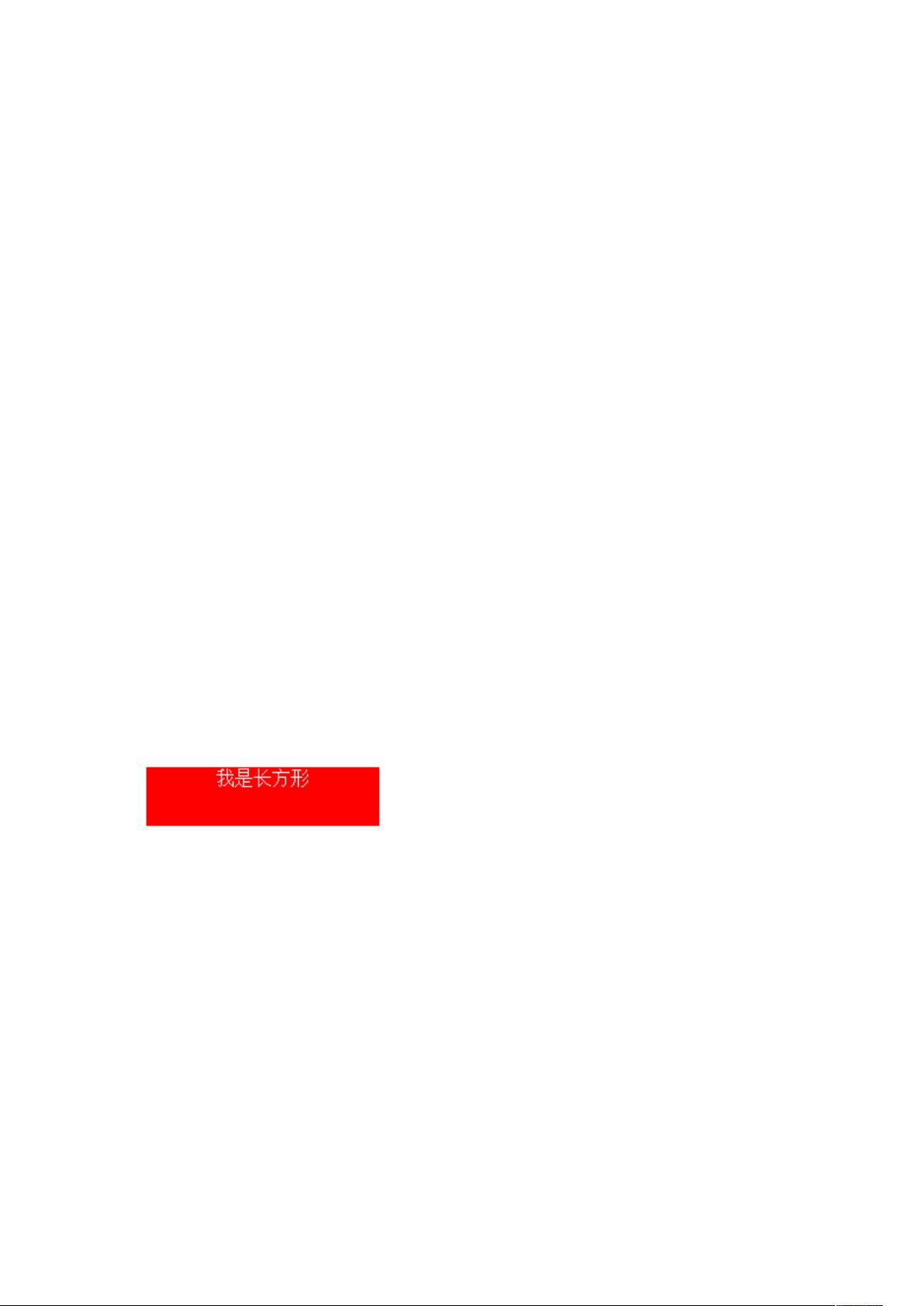
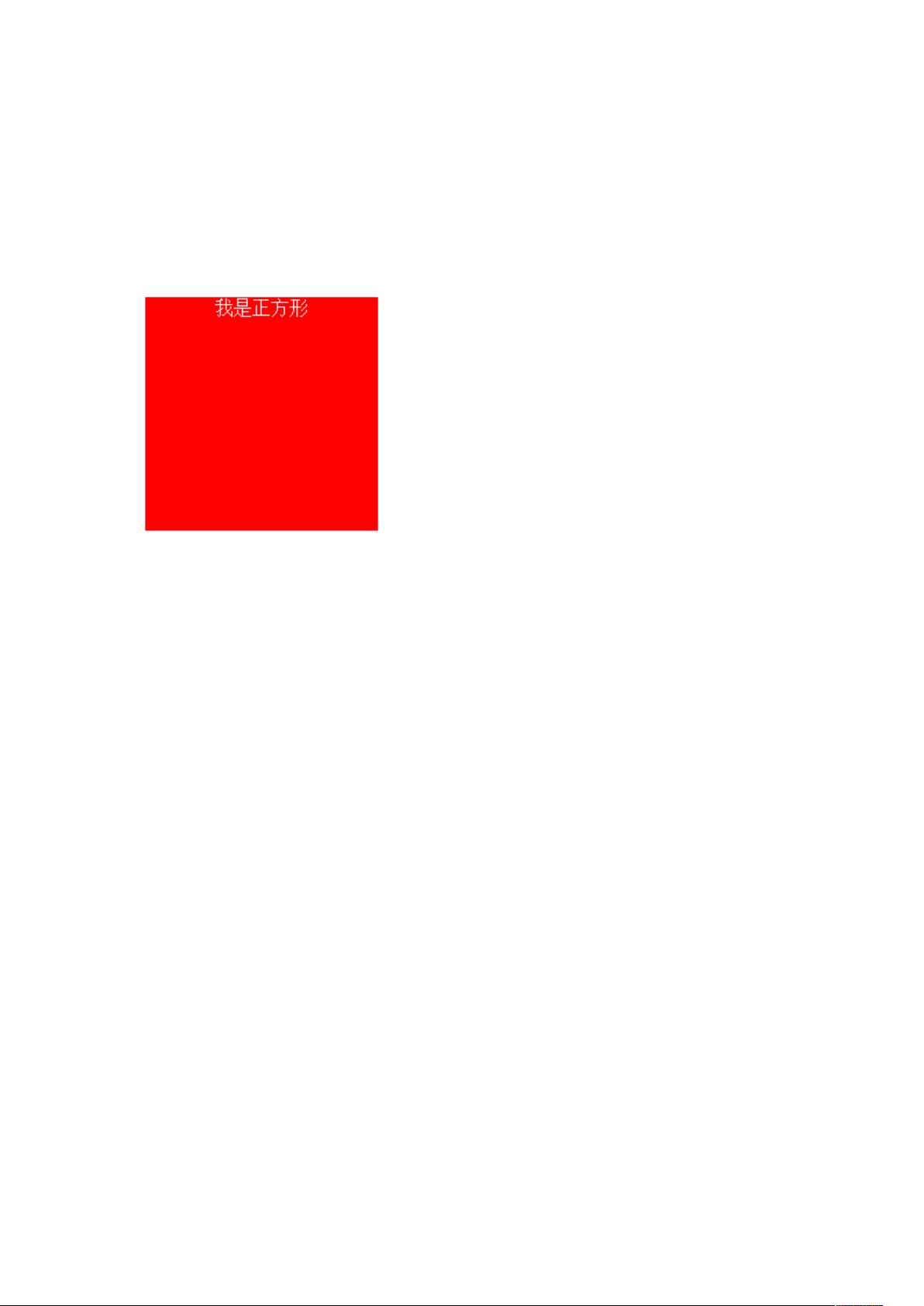
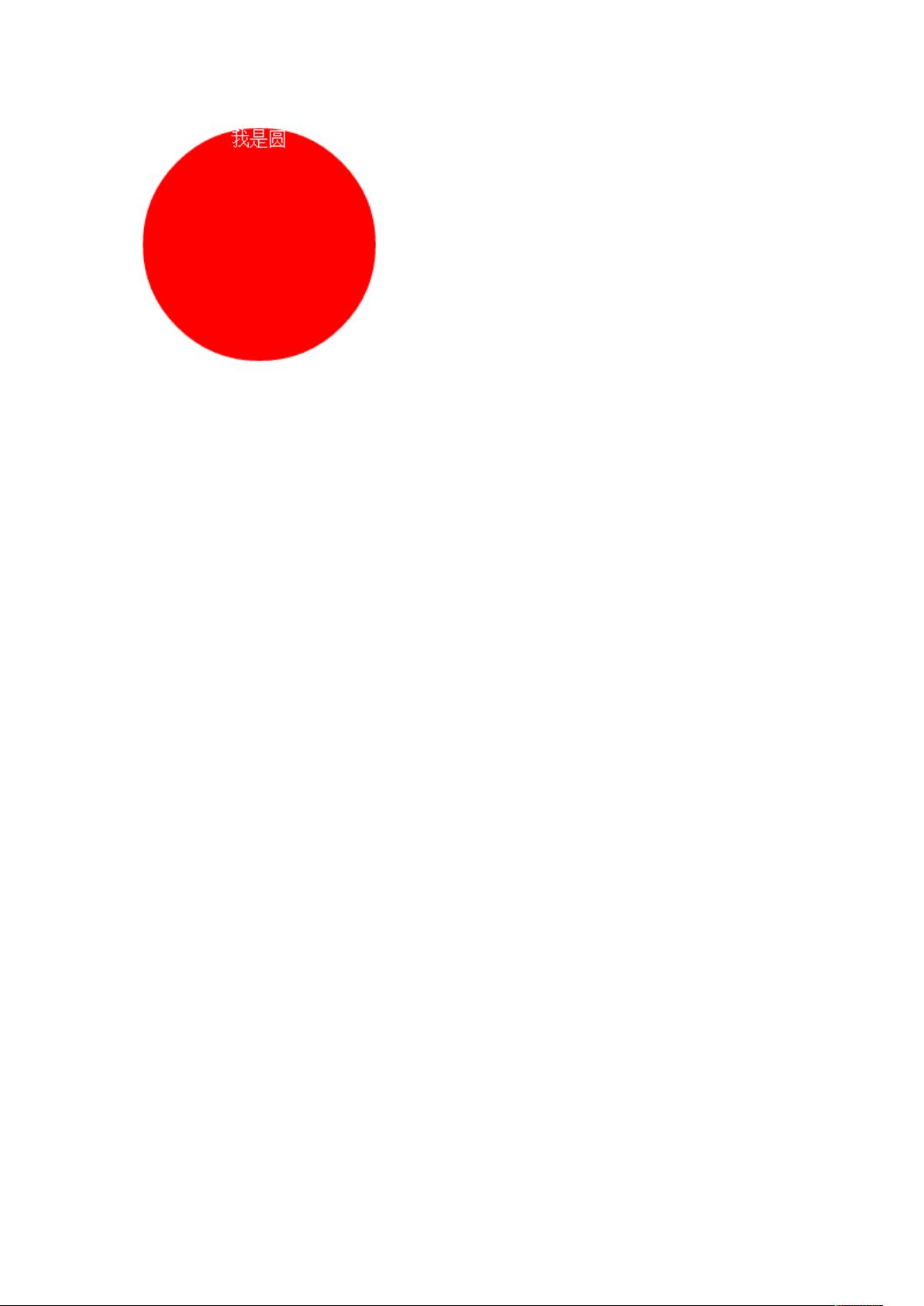
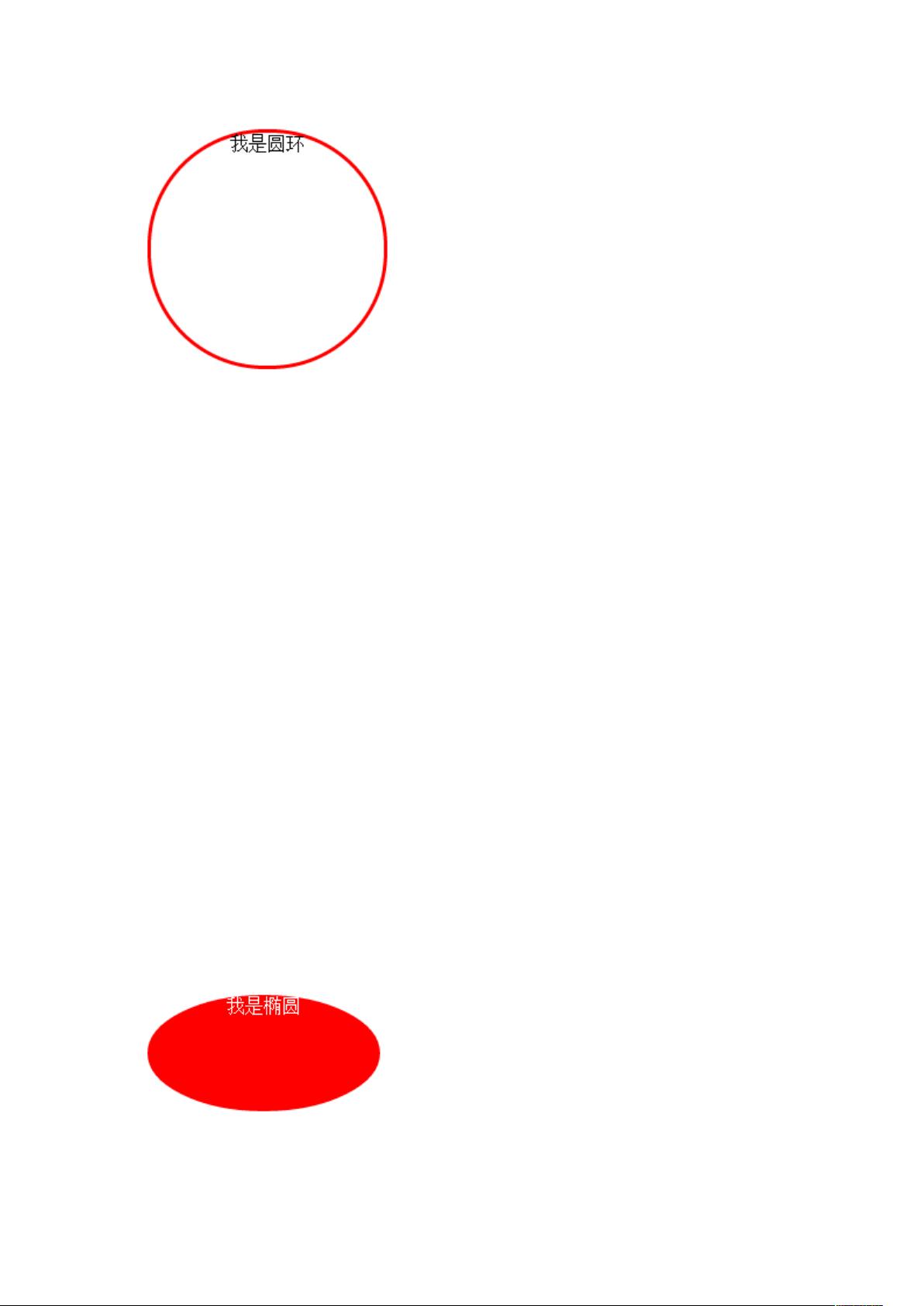
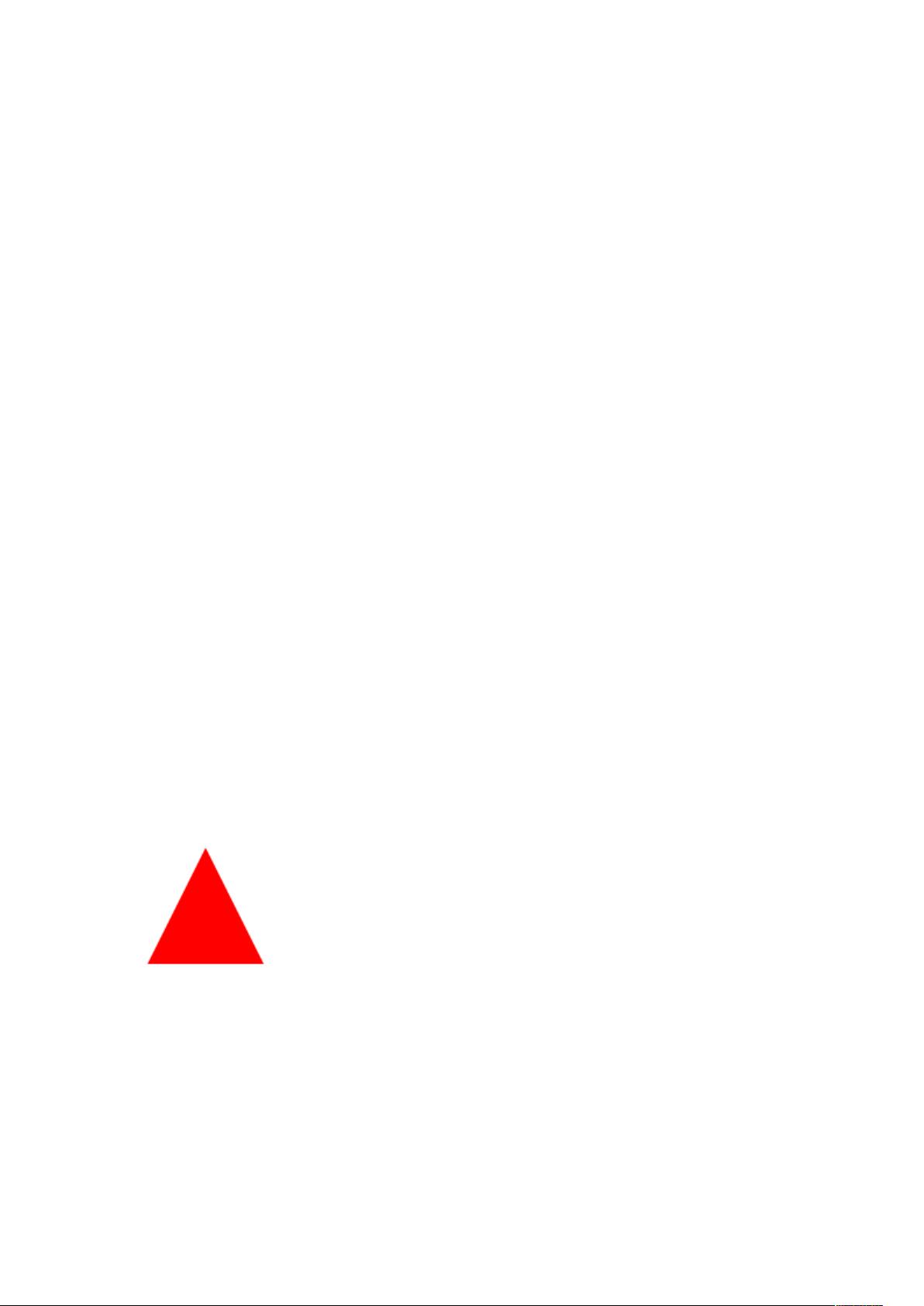
剩余29页未读,继续阅读
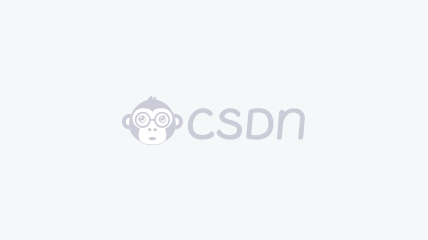

- 粉丝: 29
- 资源: 41
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

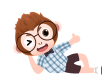
最新资源

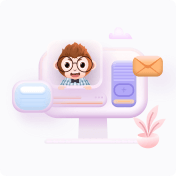
