/*********************************************************************
* INCLUDES
*/
#include "OSAL.h"
#include "ZGlobals.h"
#include "AF.h"
#include "aps_groups.h"
#include "ZDApp.h"
#include "SampleApp.h"
#include "SampleAppHw.h"
#include "OnBoard.h"
/* HAL */
#include "hal_lcd.h"
#include "hal_led.h"
#include "hal_key.h"
#include "MT_UART.h"
#include "MT_APP.h"
#include "MT.h"
// This list should be filled with Application specific Cluster IDs.
const cId_t SampleApp_ClusterList[SAMPLEAPP_MAX_CLUSTERS] =
{
SAMPLEAPP_PERIODIC_CLUSTERID,
SAMPLEAPP_FLASH_CLUSTERID
};
const SimpleDescriptionFormat_t SampleApp_SimpleDesc =
{
SAMPLEAPP_ENDPOINT, // int Endpoint;
SAMPLEAPP_PROFID, // uint16 AppProfId[2];
SAMPLEAPP_DEVICEID, // uint16 AppDeviceId[2];
SAMPLEAPP_DEVICE_VERSION, // int AppDevVer:4;
SAMPLEAPP_FLAGS, // int AppFlags:4;
SAMPLEAPP_MAX_CLUSTERS, // uint8 AppNumInClusters;
(cId_t *)SampleApp_ClusterList, // uint8 *pAppInClusterList;
SAMPLEAPP_MAX_CLUSTERS, // uint8 AppNumInClusters;
(cId_t *)SampleApp_ClusterList // uint8 *pAppInClusterList;
};
// This is the Endpoint/Interface description. It is defined here, but
// filled-in in SampleApp_Init(). Another way to go would be to fill
// in the structure here and make it a "const" (in code space). The
// way it's defined in this sample app it is define in RAM.
endPointDesc_t SampleApp_epDesc;
/*********************************************************************
* LOCAL VARIABLES
*/
uint8 SampleApp_TaskID; // Task ID for internal task/event processing
// This variable will be received when
// SampleApp_Init() is called.
devStates_t SampleApp_NwkState;
uint8 SampleApp_TransID; // This is the unique message ID (counter)
afAddrType_t SampleApp_Periodic_DstAddr;
afAddrType_t SampleApp_Flash_DstAddr;
afAddrType_t Point_To_Point_DstAddr;//网蜂点对点通信定义
afAddrType_t Group_DstAddr; //网蜂组1播通信定义
afAddrType_t Group_DstAddr1; //网蜂组2播通信定义
aps_Group_t WEBEE_Group; //分组内容
aps_Group_t WEBEE_Group1; //分组内容
aps_Group_t SampleApp_Group; //默认组
uint8 SampleAppPeriodicCounter = 0;
uint8 SampleAppFlashCounter = 0;
/*********************************************************************
* LOCAL FUNCTIONS
*/
void SampleApp_HandleKeys( uint8 shift, uint8 keys );
void SampleApp_MessageMSGCB( afIncomingMSGPacket_t *pckt );
void SampleApp_SendPeriodicMessage( void );
void SampleApp_SendFlashMessage( uint16 flashTime );
void SampleApp_Init( uint8 task_id )//任务初始化
{
SampleApp_TaskID = task_id;
SampleApp_NwkState = DEV_INIT;
SampleApp_TransID = 0;
/***********串口初始化************/
MT_UartInit(); //初始化
MT_UartRegisterTaskID(task_id);//登记任务号
HalUARTWrite(0,"Hello World\n",12);
// 组一定义
Group_DstAddr.addrMode = (afAddrMode_t)afAddrGroup;
Group_DstAddr.endPoint = SAMPLEAPP_ENDPOINT;
Group_DstAddr.addr.shortAddr = WEBEE_GROUP; //组一,协调,终端
// 组二定义
Group_DstAddr1.addrMode = (afAddrMode_t)afAddrGroup;
Group_DstAddr1.endPoint = SAMPLEAPP_ENDPOINT;
Group_DstAddr1.addr.shortAddr = WEBEE_GROUP1;//组二,路由
// 填充描述符
SampleApp_epDesc.endPoint = SAMPLEAPP_ENDPOINT;
SampleApp_epDesc.task_id = &SampleApp_TaskID;
SampleApp_epDesc.simpleDesc
= (SimpleDescriptionFormat_t *)&SampleApp_SimpleDesc;
SampleApp_epDesc.latencyReq = noLatencyReqs;
//注册描述符
afRegister( &SampleApp_epDesc );
//处理所有按键
RegisterForKeys( SampleApp_TaskID );
WEBEE_Group.ID = 0x0002; // 组ID
WEBEE_Group1.ID = 0x0003; // 组ID
//不清楚为什么下面的有的不改都能正常运行。
osal_memcpy( WEBEE_Group1.name, "Group 3", 7 );//组名称
aps_AddGroup( SAMPLEAPP_ENDPOINT, &WEBEE_Group1);
}
//任务,事件处理函数,所有处理都在这里
uint16 SampleApp_ProcessEvent( uint8 task_id, uint16 events )
{
afIncomingMSGPacket_t *MSGpkt;
(void)task_id; // Intentionally unreferenced parameter
if ( events & SYS_EVENT_MSG ) //SYS_EVENT_MSG(0x8000)接收系统消息
{
//接收属于本应用任务SampleApp的消息(SampleApp_TaskID标记。
MSGpkt = (afIncomingMSGPacket_t *)osal_msg_receive( SampleApp_TaskID );
while ( MSGpkt )//接收到消息
{
switch ( MSGpkt->hdr.event )//系统消息的进一步判断
{
// Received when a key is pressed
case KEY_CHANGE: //如果一个OSAL任务已经被登记注册,那么任何键盘事件都将接受一个KEY_CHANGE事件信息。
HalUARTWrite(0,"123\n",4);
SampleApp_HandleKeys( ((keyChange_t *)MSGpkt)->state, ((keyChange_t *)MSGpkt)->keys );
break;
// Received when a messages is received (OTA) for this endpoint
case AF_INCOMING_MSG_CMD:
HalUARTWrite(0,"rec ok\n",7);
SampleApp_MessageMSGCB( MSGpkt );
break;
// Received whenever the device changes state in the network
case ZDO_STATE_CHANGE://??只要网络状态发生改变,就通过ZDO_STATE_CHANGE事件通知所有的任务,注意,是所有任务都会收到这消息。
SampleApp_NwkState = (devStates_t)(MSGpkt->hdr.status);
if (// (SampleApp_NwkState == DEV_ZB_COORD)||//协调器不能给自己点播
(SampleApp_NwkState == DEV_ROUTER)
|| (SampleApp_NwkState == DEV_END_DEVICE) )
{
// Start sending the periodic message in a regular interval.
osal_start_timerEx( SampleApp_TaskID,
SAMPLEAPP_SEND_PERIODIC_MSG_EVT,
SAMPLEAPP_SEND_PERIODIC_MSG_TIMEOUT );
}
else
{
// Device is no longer in the network
}
break;
default:
break;
}
// Release the memory
osal_msg_deallocate( (uint8 *)MSGpkt );
// Next - if one is available
MSGpkt = (afIncomingMSGPacket_t *)osal_msg_receive( SampleApp_TaskID );
}
// return unprocessed events
return (events ^ SYS_EVENT_MSG);
}
if ( events & SAMPLEAPP_SEND_PERIODIC_MSG_EVT )//向外发送消息
{
// osal_start_timerEx( SampleApp_TaskID, SAMPLEAPP_SEND_PERIODIC_MSG_EVT,
// (SAMPLEAPP_SEND_PERIODIC_MSG_TIMEOUT + (osal_rand() & 0x00FF)) );
// return unprocessed events
return (events ^ SAMPLEAPP_SEND_PERIODIC_MSG_EVT);
}
// Discard unknown events
return 0;
}
//按键处理函数
void SampleApp_HandleKeys( uint8 shift, uint8 keys )
{
(void)shift; // Intentionally unreferenced parameter
if(ss1) //按键p0.4被按下
{
ss1 = 0;
uint8 buffer[3];
buffer[0] = (uint8)(SampleAppFlashCounter++);
buffer[1] = LO_UINT16( SAMPLEAPP_FLASH_DURATION ); //低八位
buffer[2] = HI_UINT16( SAMPLEAPP_FLASH_DURATION ); //高八位
//AF_DataRequest为主要的发送数据函数,发送时需设置各参数
if ( AF_DataRequest( &Group_DstAddr1, &SampleApp_epDesc,
SAMPLEAPP_FLASH_CLUSTERID,//对应-> AF_INCOMING_MSG_CMD ->SAMPLEAPP_FLASH_CLUSTERID
3,
buffer,
&SampleApp_TransID,
AF_DISCV_ROUTE,
AF_DEFAULT_RADIUS ) == afStatus_SUCCESS )
{
HalUARTWrite(0,"group1\n",7);
}
else
{
// Error occurred in request to send.
}
}
if(ss2) //按键p0.4被按下
{
ss2 = 0;
uint8 buffer[3];
buffer[0] = (uint8)(SampleAppFlashCounter++);
buffer[1] = LO_UINT16( SAMPLEAPP_FLASH_DURATION ); //低八位
buffer[2] = HI_UINT16( SAMPLEAPP_FLASH_DURATION ); //高八位
//AF_DataRequest为主要的发送数据函数,发送时需设置各参数
if ( AF_DataRequest( &Group_DstAddr, &SampleApp_epDesc,
SAMPLEAPP_FLASH_CLUSTERID,//对应->


俯仰一世_1
- 粉丝: 420
- 资源: 28
最新资源
- mamba 2 windows (cuda12.4)安装包,包括causal-conv1d,mamba-ssm
- simulink风储调频,风机混合储能电池超级电容储能联合一次调频,系统频率特性如下 电池储能采用双闭环pwm设计,频率控制环节为下垂控制 超级电容采用恒流充放电,降低电压释放存储在电容器中的能量
- OPC用户使用操作使用说明
- 0java实验报告.7z
- 两层液压梯程序,使用三菱FX1N系列PLC,已在设备上正常使用,非常稳定可靠,包含PLC程序
- zb25电气调车参考文档
- C++、基于MFC图像处理系统-2025
- JAVA基础编程练习:涵盖条件语句、循环结构、类和对象、继承多态及异常处理的应用案例集合
- zb45电气调车参考文档
- C++期末大作业-MFC 开发的Windows标准计算器和程序员计算器-2025
- Java编程实践:多位数字解析、文本检索、格式校验及其他基础练习
- python 的虚拟环境 原理
- C++-MFC框架为基础,结合opencv来对图像做处理-2025
- LSSVM,SSA-LSSVM,VMD-LSSVM,VMD-SSA-LSSVM四种算法做短期电力负荷预测,做对比 结果分析-lssvm 均方根误差(RMSE):0.79172 平均绝对误差(MAE)
- JAVA面向对象编程与异常处理核心技术解析及实际应用
- ZB45电气简化图册1
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


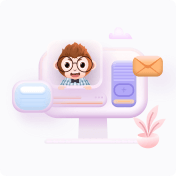