<?php
/**
* PHPMailer - PHP email creation and transport class.
* PHP Version 5.5.
*
* @see https://github.com/PHPMailer/PHPMailer/ The PHPMailer GitHub project
*
* @author Marcus Bointon (Synchro/coolbru) <phpmailer@synchromedia.co.uk>
* @author Jim Jagielski (jimjag) <jimjag@gmail.com>
* @author Andy Prevost (codeworxtech) <codeworxtech@users.sourceforge.net>
* @author Brent R. Matzelle (original founder)
* @copyright 2012 - 2017 Marcus Bointon
* @copyright 2010 - 2012 Jim Jagielski
* @copyright 2004 - 2009 Andy Prevost
* @license http://www.gnu.org/copyleft/lesser.html GNU Lesser General Public License
* @note This program is distributed in the hope that it will be useful - WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
* FITNESS FOR A PARTICULAR PURPOSE.
*/
namespace PHPMailer\PHPMailer;
/**
* PHPMailer - PHP email creation and transport class.
*
* @author Marcus Bointon (Synchro/coolbru) <phpmailer@synchromedia.co.uk>
* @author Jim Jagielski (jimjag) <jimjag@gmail.com>
* @author Andy Prevost (codeworxtech) <codeworxtech@users.sourceforge.net>
* @author Brent R. Matzelle (original founder)
*/
class PHPMailer
{
/**
* Email priority.
* Options: null (default), 1 = High, 3 = Normal, 5 = low.
* When null, the header is not set at all.
*
* @var int
*/
public $Priority;
/**
* The character set of the message.
*
* @var string
*/
public $CharSet = 'iso-8859-1';
/**
* The MIME Content-type of the message.
*
* @var string
*/
public $ContentType = 'text/plain';
/**
* The message encoding.
* Options: "8bit", "7bit", "binary", "base64", and "quoted-printable".
*
* @var string
*/
public $Encoding = '8bit';
/**
* Holds the most recent mailer error message.
*
* @var string
*/
public $ErrorInfo = '';
/**
* The From email address for the message.
*
* @var string
*/
public $From = 'root@localhost';
/**
* The From name of the message.
*
* @var string
*/
public $FromName = 'Root User';
/**
* The envelope sender of the message.
* This will usually be turned into a Return-Path header by the receiver,
* and is the address that bounces will be sent to.
* If not empty, will be passed via `-f` to sendmail or as the 'MAIL FROM' value over SMTP.
*
* @var string
*/
public $Sender = '';
/**
* The Subject of the message.
*
* @var string
*/
public $Subject = '';
/**
* An HTML or plain text message body.
* If HTML then call isHTML(true).
*
* @var string
*/
public $Body = '';
/**
* The plain-text message body.
* This body can be read by mail clients that do not have HTML email
* capability such as mutt & Eudora.
* Clients that can read HTML will view the normal Body.
*
* @var string
*/
public $AltBody = '';
/**
* An iCal message part body.
* Only supported in simple alt or alt_inline message types
* To generate iCal event structures, use classes like EasyPeasyICS or iCalcreator.
*
* @see http://sprain.ch/blog/downloads/php-class-easypeasyics-create-ical-files-with-php/
* @see http://kigkonsult.se/iCalcreator/
*
* @var string
*/
public $Ical = '';
/**
* The complete compiled MIME message body.
*
* @var string
*/
protected $MIMEBody = '';
/**
* The complete compiled MIME message headers.
*
* @var string
*/
protected $MIMEHeader = '';
/**
* Extra headers that createHeader() doesn't fold in.
*
* @var string
*/
protected $mailHeader = '';
/**
* Word-wrap the message body to this number of chars.
* Set to 0 to not wrap. A useful value here is 78, for RFC2822 section 2.1.1 compliance.
*
* @see static::STD_LINE_LENGTH
*
* @var int
*/
public $WordWrap = 0;
/**
* Which method to use to send mail.
* Options: "mail", "sendmail", or "smtp".
*
* @var string
*/
public $Mailer = 'mail';
/**
* The path to the sendmail program.
*
* @var string
*/
public $Sendmail = '/usr/sbin/sendmail';
/**
* Whether mail() uses a fully sendmail-compatible MTA.
* One which supports sendmail's "-oi -f" options.
*
* @var bool
*/
public $UseSendmailOptions = true;
/**
* The email address that a reading confirmation should be sent to, also known as read receipt.
*
* @var string
*/
public $ConfirmReadingTo = '';
/**
* The hostname to use in the Message-ID header and as default HELO string.
* If empty, PHPMailer attempts to find one with, in order,
* $_SERVER['SERVER_NAME'], gethostname(), php_uname('n'), or the value
* 'localhost.localdomain'.
*
* @var string
*/
public $Hostname = '';
/**
* An ID to be used in the Message-ID header.
* If empty, a unique id will be generated.
* You can set your own, but it must be in the format "<id@domain>",
* as defined in RFC5322 section 3.6.4 or it will be ignored.
*
* @see https://tools.ietf.org/html/rfc5322#section-3.6.4
*
* @var string
*/
public $MessageID = '';
/**
* The message Date to be used in the Date header.
* If empty, the current date will be added.
*
* @var string
*/
public $MessageDate = '';
/**
* SMTP hosts.
* Either a single hostname or multiple semicolon-delimited hostnames.
* You can also specify a different port
* for each host by using this format: [hostname:port]
* (e.g. "smtp1.example.com:25;smtp2.example.com").
* You can also specify encryption type, for example:
* (e.g. "tls://smtp1.example.com:587;ssl://smtp2.example.com:465").
* Hosts will be tried in order.
*
* @var string
*/
public $Host = 'localhost';
/**
* The default SMTP server port.
*
* @var int
*/
public $Port = 25;
/**
* The SMTP HELO of the message.
* Default is $Hostname. If $Hostname is empty, PHPMailer attempts to find
* one with the same method described above for $Hostname.
*
* @see PHPMailer::$Hostname
*
* @var string
*/
public $Helo = '';
/**
* What kind of encryption to use on the SMTP connection.
* Options: '', 'ssl' or 'tls'.
*
* @var string
*/
public $SMTPSecure = '';
/**
* Whether to enable TLS encryption automatically if a server supports it,
* even if `SMTPSecure` is not set to 'tls'.
* Be aware that in PHP >= 5.6 this requires that the server's certificates are valid.
*
* @var bool
*/
public $SMTPAutoTLS = true;
/**
* Whether to use SMTP authentication.
* Uses the Username and Password properties.
*
* @see PHPMailer::$Username
* @see PHPMailer::$Password
*
* @var bool
*/
public $SMTPAuth = false;
/**
* Options array passed to stream_context_create when connecting via SMTP.
*
* @var array
*/
public $SMTPOptions = [];
/**
* SMTP username.
*
* @var string
*/
public $Username = '';
/**
* SMTP password.
*
* @var string
*/
public $Password = '';
/**
* SMTP auth type.
* Options are CRAM-MD5, LOGIN, PLAIN, XOAUTH2, attempted in that order if not specified.
*
* @var string
*/
public $AuthType = '';
/**
* An instance of the PHPMailer OAuth class.
*
* @var OAuth
*/
protected $oauth;
/**
* The SMTP server timeout in seconds.
* Default of 5 minutes (300sec) is from RFC2821 section 4.5.3.2.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
APP内部管理系统-APP解析部分(PHP版本) 本系统功能说明: 1. 支持安卓、苹果APP包上传后自动解析读取包信息,减少用户手动录入的繁琐。 2. 支持苹果APP开发包上传后,自动打包企业认证,提供在线安装IPA包功能。方便公司内部各种历史版本上传就可以安装,减少手工打包的繁琐。
资源推荐
资源详情
资源评论
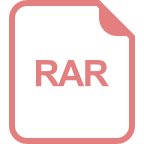
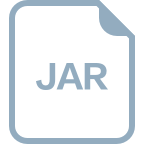
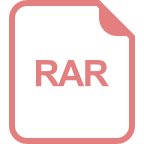
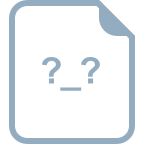
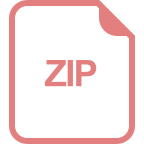
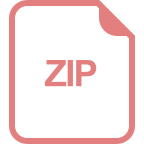
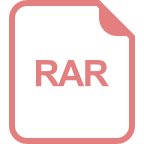
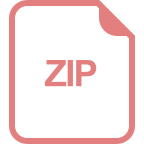
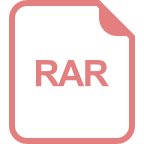
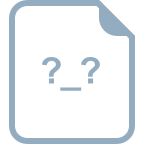
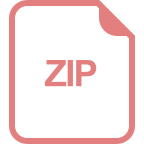
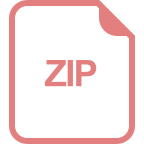
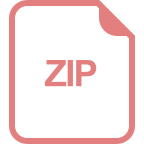
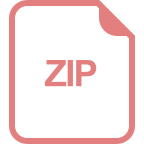
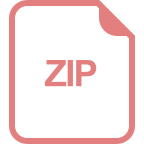
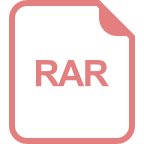
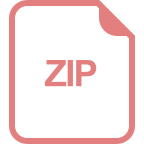
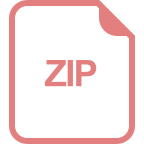
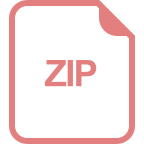
收起资源包目录

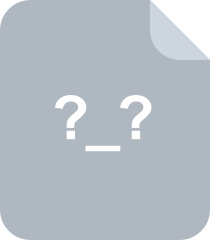
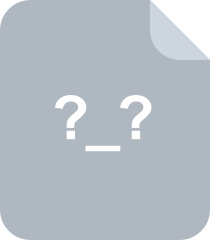
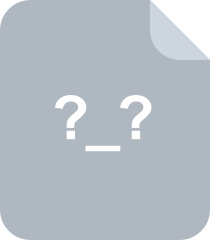
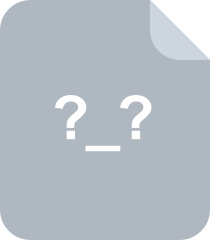
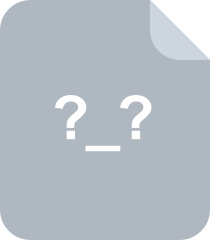
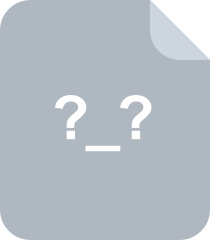
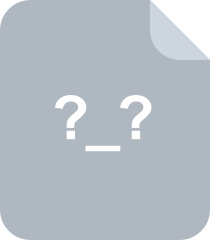
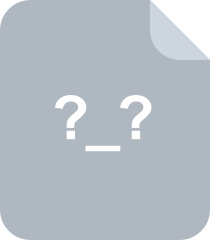
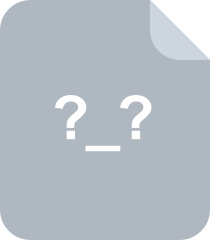
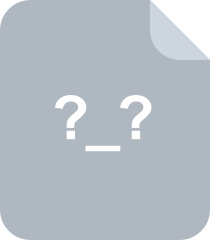
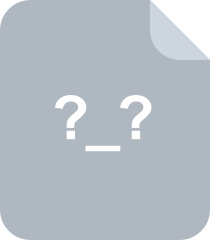
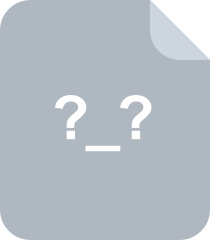
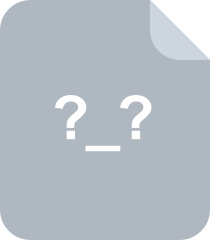
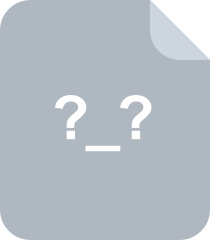
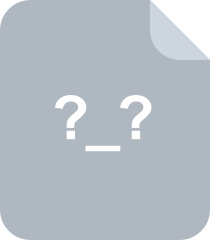
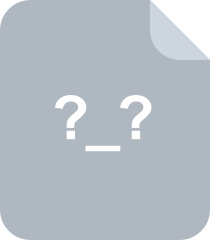
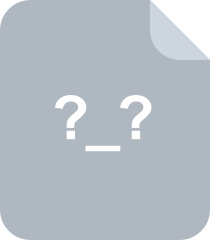
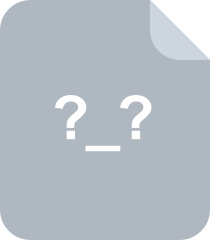
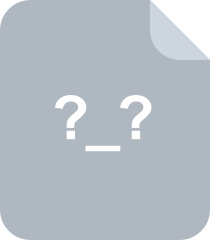
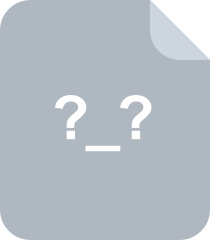
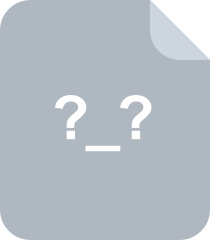
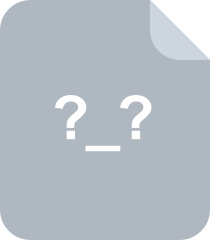
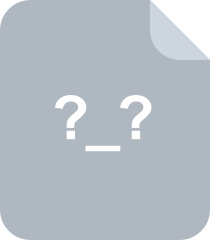
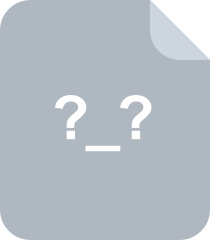
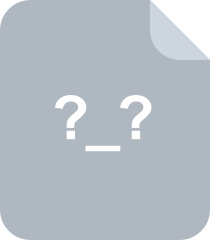
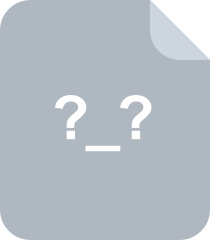
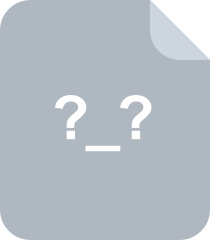
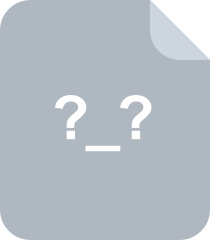
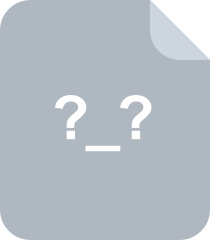
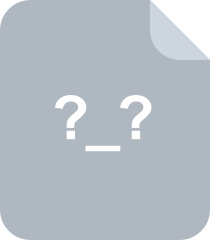
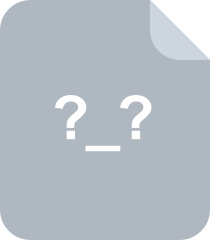
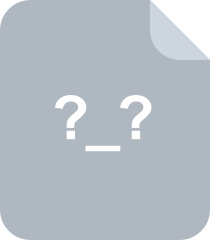
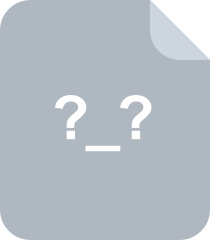
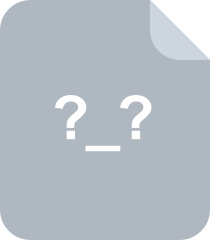
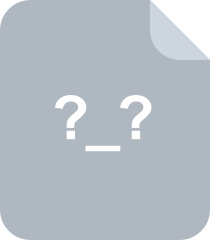
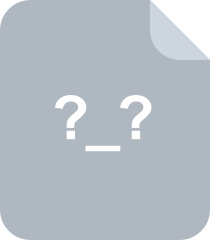
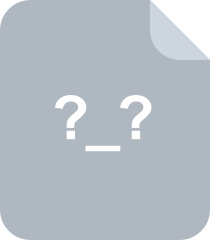
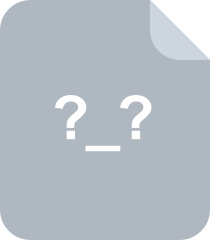
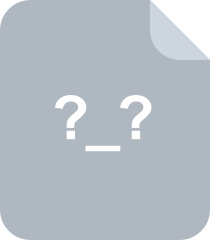
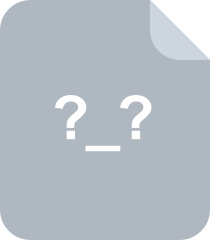
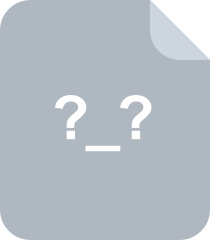
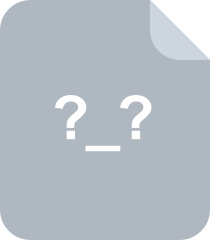
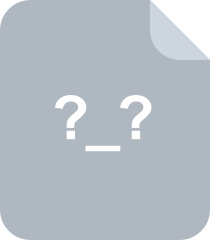
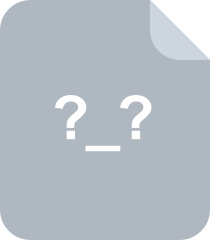
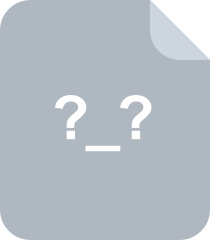
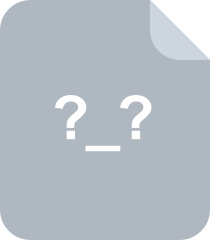
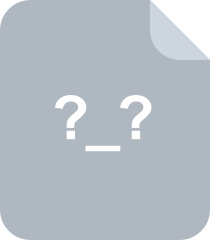
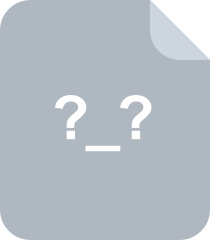
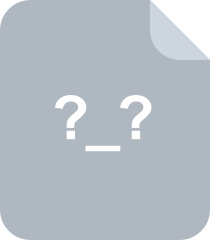
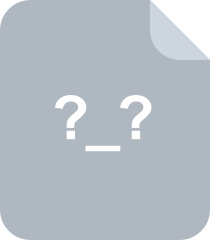
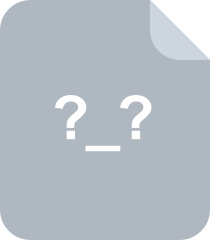
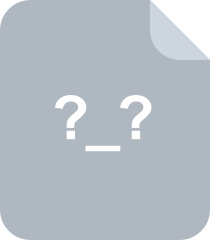
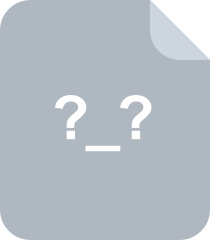
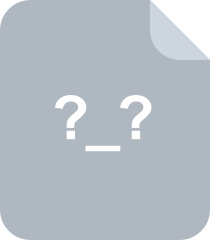
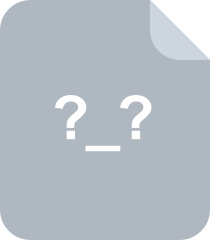
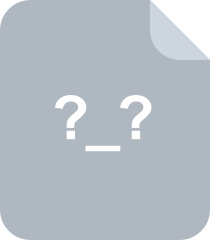
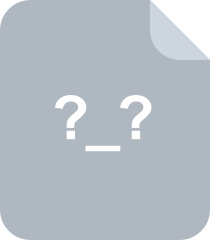
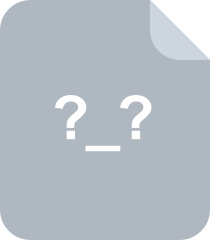
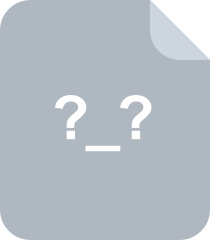
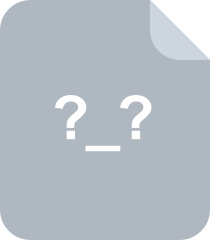
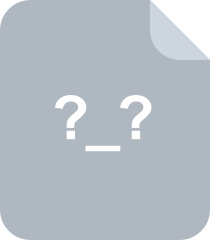
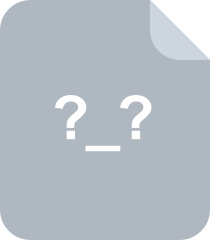
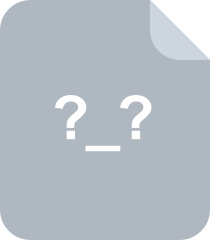
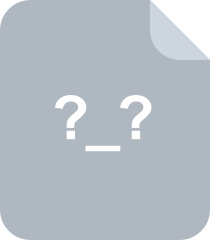
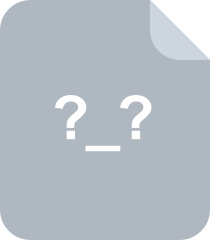
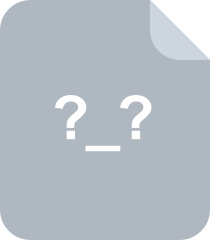
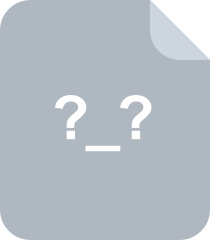
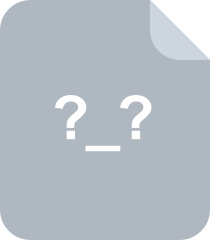
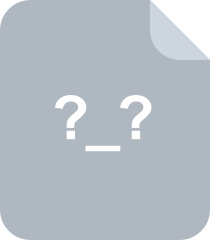
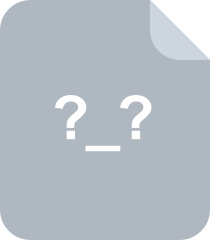
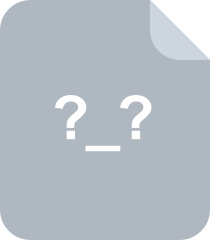
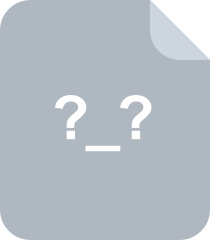
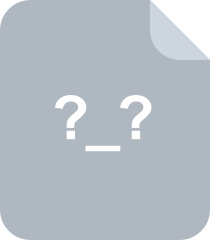
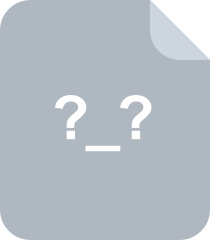
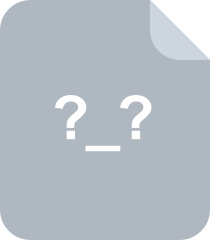
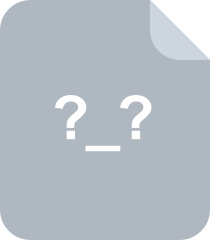
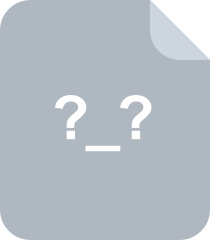
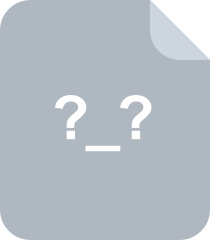
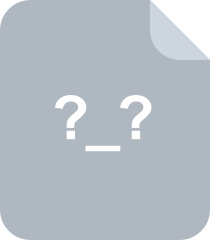
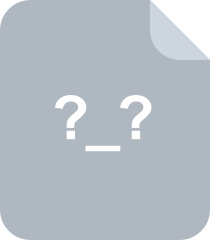
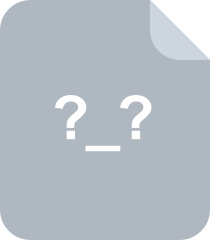
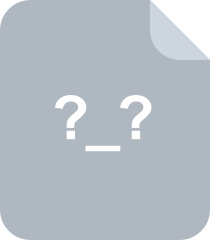
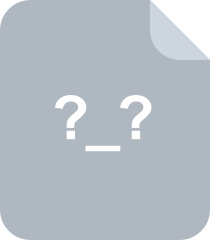
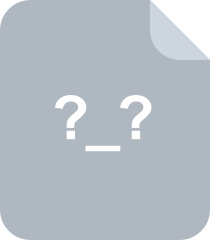
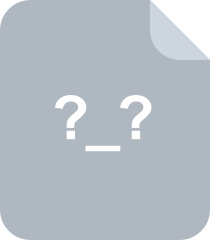
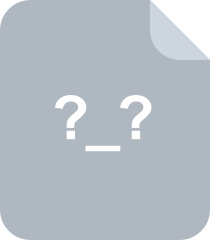
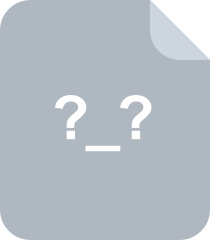
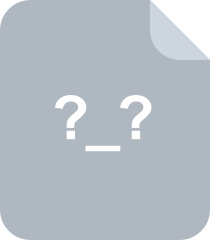
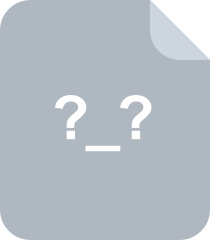
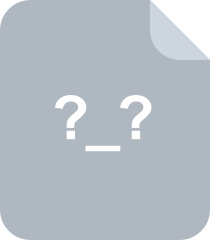
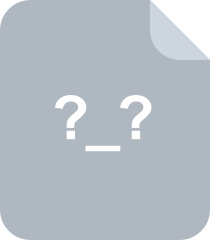
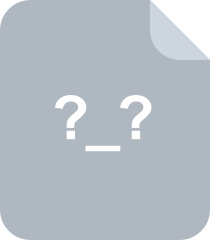
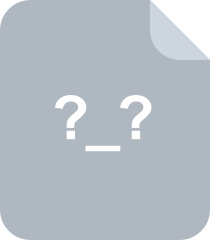
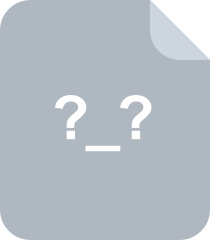
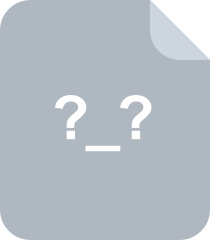
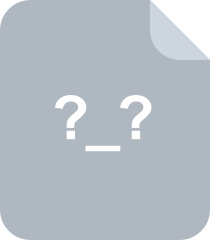
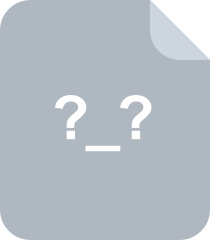
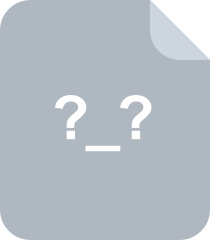
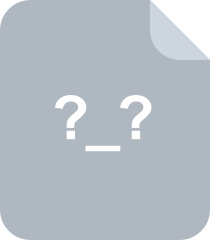
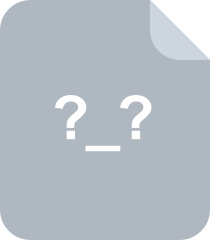
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
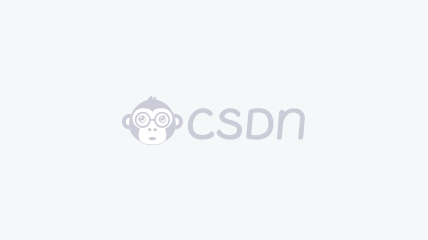

新视点心理
- 粉丝: 5
- 资源: 5
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

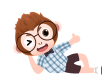
最新资源
- C/C++基本框架及解释
- 使用OpenGL实现透明效果
- java房屋租赁系统源码 房屋房源出租管理系统源码数据库 MySQL源码类型 WebForm
- JAVA的Springboot博客网站源码数据库 MySQL源码类型 WebForm
- c++数字雨实现 c++
- 如何制作MC(需要下载海龟编辑器2.0,下载pyglet==1.5.15)
- JAVA的Springboot小区物业管理系统源码数据库 MySQL源码类型 WebForm
- IMG_20241103_153322.jpg
- Screenshot_2024-11-10-20-33-57-639_com.tencent.tmgp.pubgmhd.jpg
- C#商家会员管理系统源码带微信功能数据库 SQL2008源码类型 WebForm
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


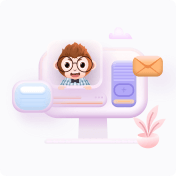
安全验证
文档复制为VIP权益,开通VIP直接复制
