/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
!function(t,e){"object"==typeof exports&&"undefined"!=typeof module?e(exports):"function"==typeof define&&define.amd?define(["exports"],e):e((t="undefined"!=typeof globalThis?globalThis:t||self).echarts={})}(this,(function(t){"use strict";
/*! *****************************************************************************
Copyright (c) Microsoft Corporation.
Permission to use, copy, modify, and/or distribute this software for any
purpose with or without fee is hereby granted.
THE SOFTWARE IS PROVIDED "AS IS" AND THE AUTHOR DISCLAIMS ALL WARRANTIES WITH
REGARD TO THIS SOFTWARE INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY
AND FITNESS. IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY SPECIAL, DIRECT,
INDIRECT, OR CONSEQUENTIAL DAMAGES OR ANY DAMAGES WHATSOEVER RESULTING FROM
LOSS OF USE, DATA OR PROFITS, WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR
OTHER TORTIOUS ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR
PERFORMANCE OF THIS SOFTWARE.
***************************************************************************** */var e=function(t,n){return(e=Object.setPrototypeOf||{__proto__:[]}instanceof Array&&function(t,e){t.__proto__=e}||function(t,e){for(var n in e)Object.prototype.hasOwnProperty.call(e,n)&&(t[n]=e[n])})(t,n)};function n(t,n){function i(){this.constructor=t}e(t,n),t.prototype=null===n?Object.create(n):(i.prototype=n.prototype,new i)}var i=function(){return(i=Object.assign||function(t){for(var e,n=1,i=arguments.length;n<i;n++)for(var r in e=arguments[n])Object.prototype.hasOwnProperty.call(e,r)&&(t[r]=e[r]);return t}).apply(this,arguments)};function r(){for(var t=0,e=0,n=arguments.length;e<n;e++)t+=arguments[e].length;var i=Array(t),r=0;for(e=0;e<n;e++)for(var o=arguments[e],a=0,s=o.length;a<s;a++,r++)i[r]=o[a];return i}var o=function(){this.firefox=!1,this.ie=!1,this.edge=!1,this.newEdge=!1,this.weChat=!1},a=new function(){this.browser=new o,this.node=!1,this.wxa=!1,this.worker=!1,this.canvasSupported=!1,this.svgSupported=!1,this.touchEventsSupported=!1,this.pointerEventsSupported=!1,this.domSupported=!1};"object"==typeof wx&&"function"==typeof wx.getSystemInfoSync?(a.wxa=!0,a.canvasSupported=!0,a.touchEventsSupported=!0):"undefined"==typeof document&&"undefined"!=typeof self?(a.worker=!0,a.canvasSupported=!0):"undefined"==typeof navigator?(a.node=!0,a.canvasSupported=!0,a.svgSupported=!0):function(t,e){var n=e.browser,i=t.match(/Firefox\/([\d.]+)/),r=t.match(/MSIE\s([\d.]+)/)||t.match(/Trident\/.+?rv:(([\d.]+))/),o=t.match(/Edge?\/([\d.]+)/),a=/micromessenger/i.test(t);i&&(n.firefox=!0,n.version=i[1]);r&&(n.ie=!0,n.version=r[1]);o&&(n.edge=!0,n.version=o[1],n.newEdge=+o[1].split(".")[0]>18);a&&(n.weChat=!0);e.canvasSupported=!!document.createElement("canvas").getContext,e.svgSupported="undefined"!=typeof SVGRect,e.touchEventsSupported="ontouchstart"in window&&!n.ie&&!n.edge,e.pointerEventsSupported="onpointerdown"in window&&(n.edge||n.ie&&+n.version>=11),e.domSupported="undefined"!=typeof document}(navigator.userAgent,a);var s={"[object Function]":!0,"[object RegExp]":!0,"[object Date]":!0,"[object Error]":!0,"[object CanvasGradient]":!0,"[object CanvasPattern]":!0,"[object Image]":!0,"[object Canvas]":!0},l={"[object Int8Array]":!0,"[object Uint8Array]":!0,"[object Uint8ClampedArray]":!0,"[object Int16Array]":!0,"[object Uint16Array]":!0,"[object Int32Array]":!0,"[object Uint32Array]":!0,"[object Float32Array]":!0,"[object Float64Array]":!0},u=Object.prototype.toString,h=Array.prototype,c=h.forEach,p=h.filter,d=h.slice,f=h.map,g=function(){}.constructor,y=g?g.prototype:null,v={};function m(t,e){v[t]=e}var _=2311;function x(){return _++}function b(){for(var t=[],e=0;e<arguments.length;e++)t[e]=arguments[e];"undefined"!=typeof console&&console.error.apply(console,t)}function w(t){if(null==t||"object"!=typeof t)return t;var e=t,n=u.call(t);if("[object Array]"===n){if(!lt(t)){e=[];for(var i=0,r=t.length;i<r;i++)e[i]=w(t[i])}}else if(l[n]){if(!lt(t)){var o=t.constructor;if(o.from)e=o.from(t);else{e=new o(t.length);for(i=0,r=t.length;i<r;i++)e[i]=w(t[i])}}}else if(!s[n]&&!lt(t)&&!j(t))for(var a in e={},t)t.hasOwnProperty(a)&&(e[a]=w(t[a]));return e}function S(t,e,n){if(!X(e)||!X(t))return n?w(e):t;for(var i in e)if(e.hasOwnProperty(i)){var r=t[i],o=e[i];!X(o)||!X(r)||F(o)||F(r)||j(o)||j(r)||U(o)||U(r)||lt(o)||lt(r)?!n&&i in t||(t[i]=w(e[i])):S(r,o,n)}return t}function M(t,e){for(var n=t[0],i=1,r=t.length;i<r;i++)n=S(n,t[i],e);return n}function I(t,e){if(Object.assign)Object.assign(t,e);else for(var n in e)e.hasOwnProperty(n)&&(t[n]=e[n]);return t}function T(t,e,n){for(var i=z(e),r=0;r<i.length;r++){var o=i[r];(n?null!=e[o]:null==t[o])&&(t[o]=e[o])}return t}var C=function(){return v.createCanvas()};function A(t,e){if(t){if(t.indexOf)return t.indexOf(e);for(var n=0,i=t.length;n<i;n++)if(t[n]===e)return n}return-1}function D(t,e){var n=t.prototype;function i(){}for(var r in i.prototype=e.prototype,t.prototype=new i,n)n.hasOwnProperty(r)&&(t.prototype[r]=n[r]);t.prototype.constructor=t,t.superClass=e}function L(t,e,n){if(t="prototype"in t?t.prototype:t,e="prototype"in e?e.prototype:e,Object.getOwnPropertyNames)for(var i=Object.getOwnPropertyNames(e),r=0;r<i.length;r++){var o=i[r];"constructor"!==o&&(n?null!=e[o]:null==t[o])&&(t[o]=e[o])}else T(t,e,n)}function k(t){return!!t&&("string"!=typeof t&&"number"==typeof t.length)}function P(t,e,n){if(t&&e)if(t.forEach&&t.forEach===c)t.forEach(e,n);else if(t.length===+t.length)for(var i=0,r=t.length;i<r;i++)e.call(n,t[i],i,t);else for(var o in t)t.hasOwnProperty(o)&&e.call(n,t[o],o,t)}function O(t,e,n){if(!t)return[];if(!e)return nt(t);if(t.map&&t.map===f)return t.map(e,n);for(var i=[],r=0,o=t.length;r<o;r++)i.push(e.call(n,t[r],r,t));return i}function R(t,e,n,i){if(t&&e){for(var r=0,o=t.length;r<o;r++)n=e.call(i,n,t[r],r,t);return n}}function N(t,e,n){if(!t)return[];if(!e)return nt(t);if(t.filter&&t.filter===p)return t.filter(e,n);for(var i=[],r=0,o=t.length;r<o;r++)e.call(n,t[r],r,t)&&i.push(t[r]);return i}function E(t,e,n){if(t&&e)for(var i=0,r=t.length;i<r;i++)if(e.call(n,t[i],i,t))return t[i]}function z(t){if(!t)return[];if(Object.keys)return Object.keys(t);var e=[];for(var n in t)t.hasOwnProperty(n)&&e.push(n);return e}v.createCanvas=function(){return document.createElement("canvas")};var B=y&&G(y.bind)?y.call.bind(y.bind):function(t,e){for(var n=[],i=2;i<arguments.length;i++)n[i-2]=arguments[i];return function(){return t.apply(e,n.concat(d.call(arguments)))}};function V(t){for(var e=[],n=1;n<arguments.length;n++)e[n-1]=arguments[n];return function(){return t.apply(this,e.concat(d.call(arguments)))}}function F(t){return Array.isArray?Array.isArray(t):"[object Array]"===u.call(t)}function G(t){return"function"==typeof t}function H(t){return"string"==typeof t}function W(t){return"[object String]"===u.call(t)}function Y(t){return"number"==typeof t}function X(t){var e=typeof t;return"function"===e||!!t&&"object"===e}function U(t){return!!s[u.call(t)]}function Z(t){return!!l[u.call(t)]}function j(t){return"object"==typeof t&&"number"==typeof t.nodeType&&"object"==typeof t.ownerDocument}function q(t){return null!=t.colorStops}function K(t){return null!=t.image}function $(t
没有合适的资源?快使用搜索试试~ 我知道了~
网格回测工具1.2.6.1
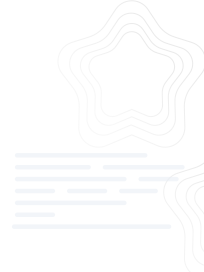
共94个文件
ini:66个
dll:10个
dat:6个


温馨提示
升级1.2.6.1 1、更新了跳转算法; 2、改进了登录失败的验证算法; 3、对采集功能做了升级; 简介:网格回测工具是一款用来回测网格历史数据的软件,看是否匹配自己的预测和结果,对自己决策做出辅助。 本软件是一款高效的网格数据回测工具,可以对5,10,30,60,1日等多种模式做回测。同时支持成本计算,并可以同长期持有模式进行比对,数据准确,高效稳定。
资源推荐
资源详情
资源评论
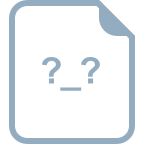
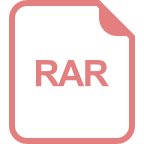
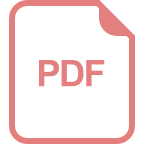
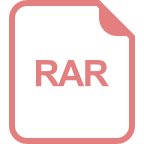
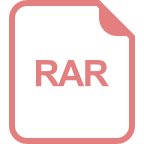
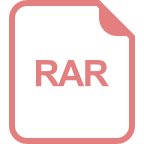
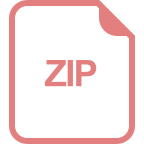
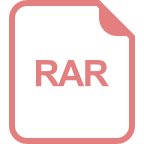
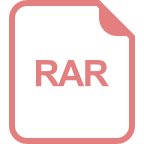
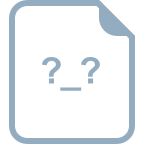
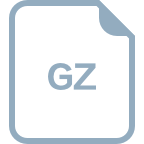
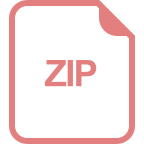
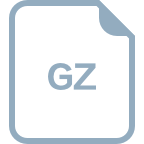
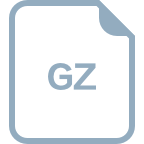
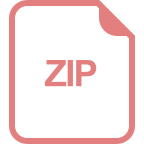
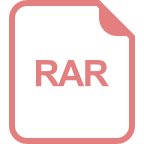
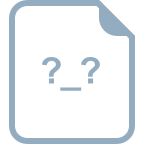
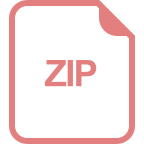
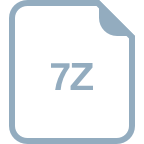
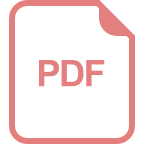
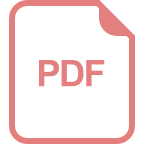
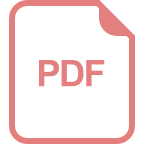
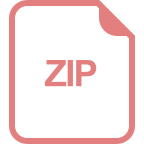
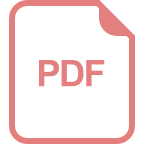
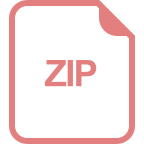
收起资源包目录

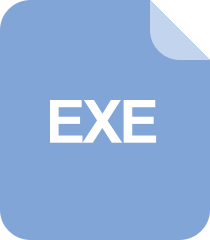
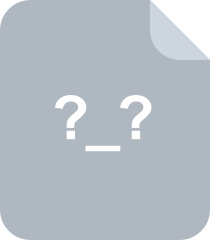
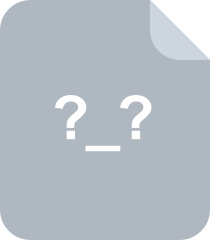
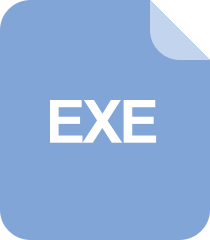
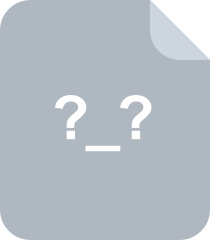
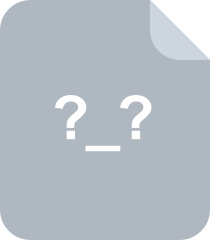

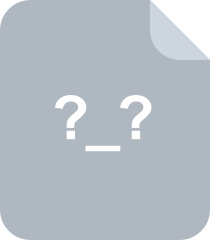
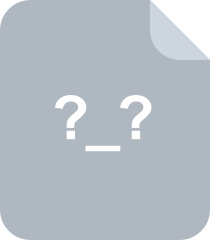
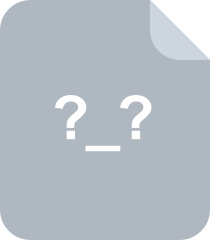
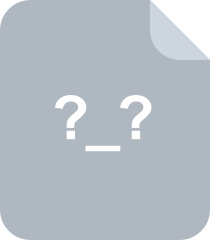
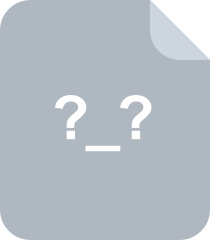
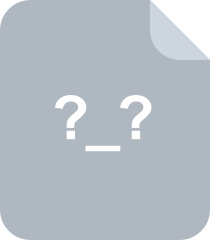
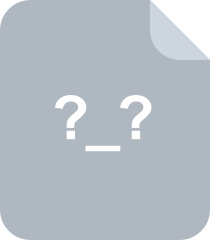
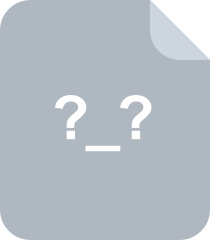
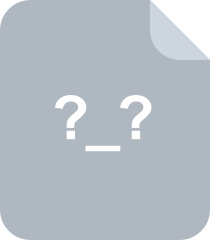
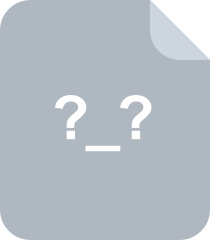
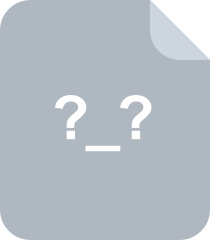
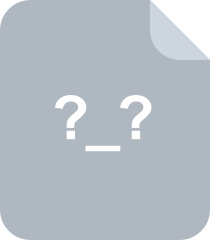
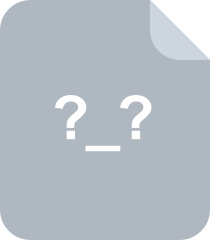
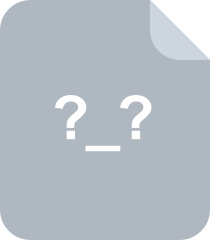
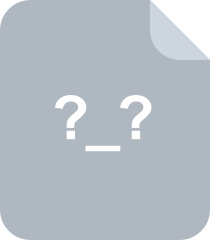
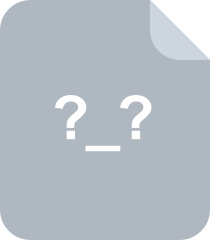
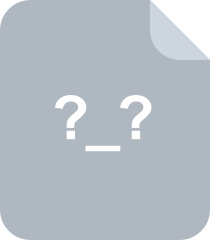
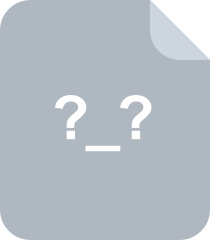
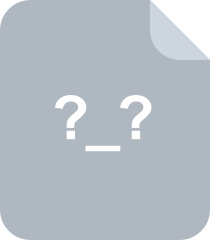
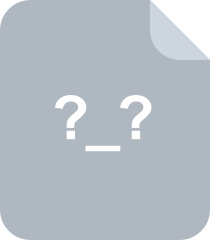
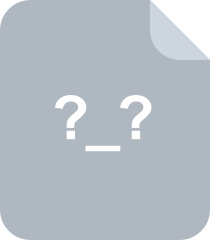
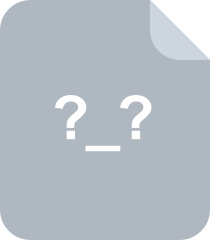
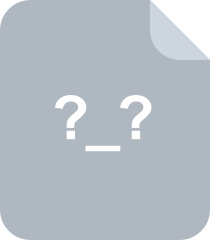
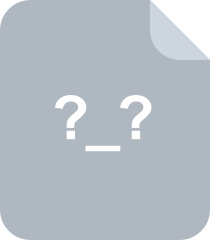
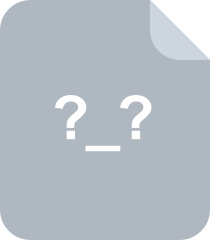
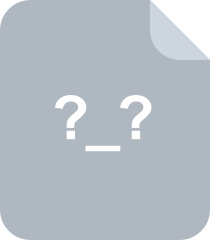
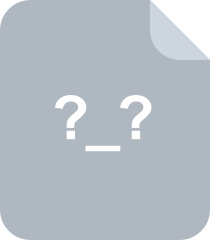
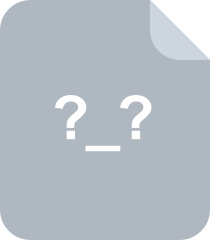
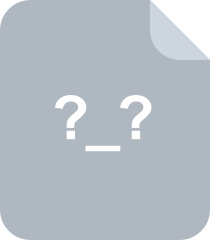
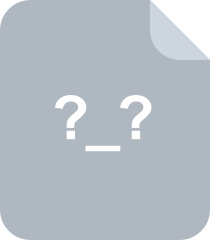
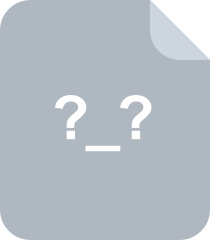
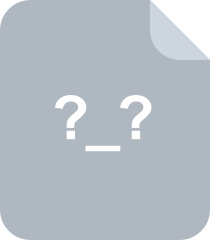
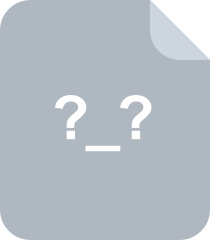
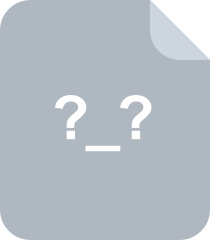
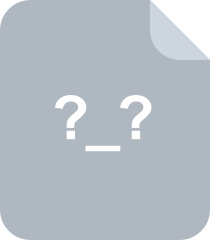
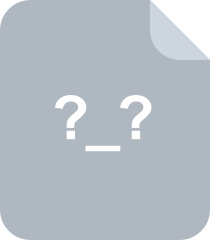
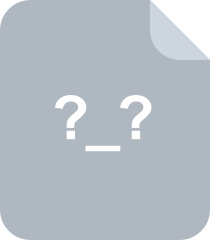
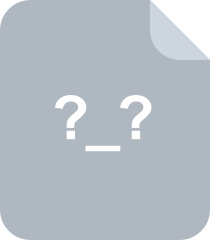
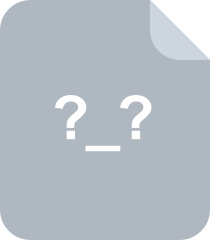
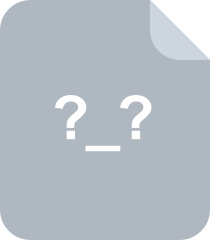
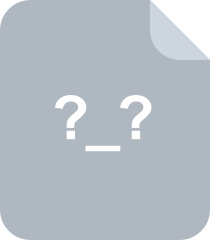
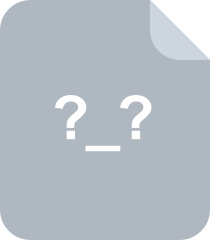
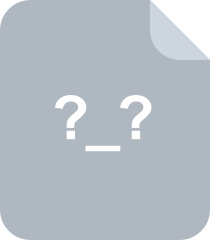
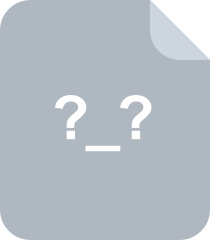
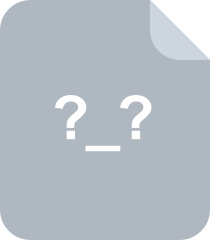
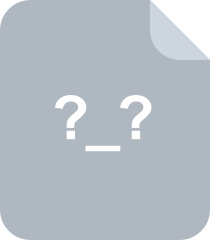
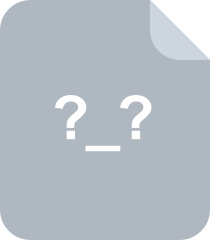
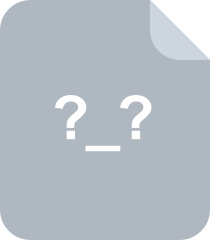
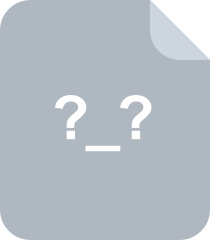
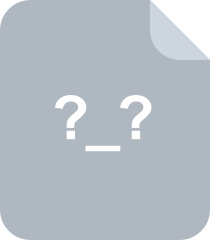
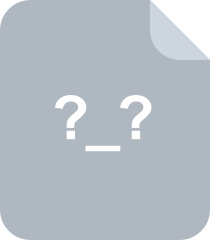
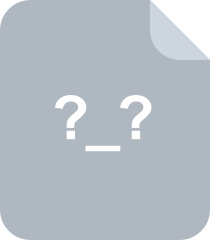
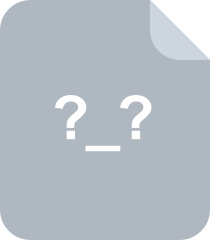
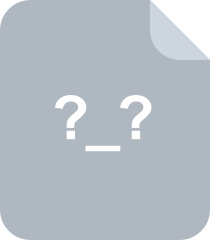
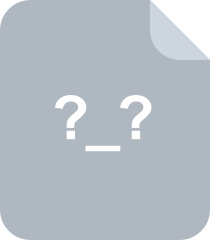
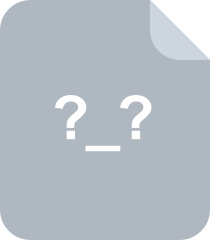
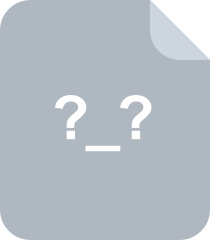
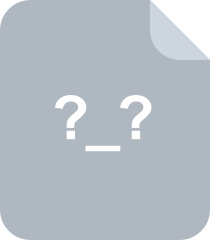
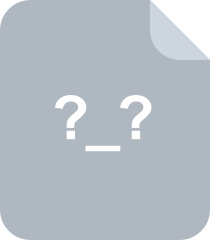
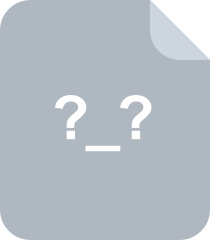
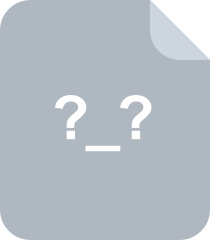
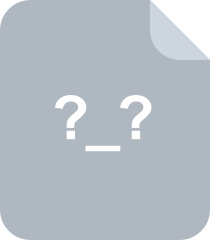
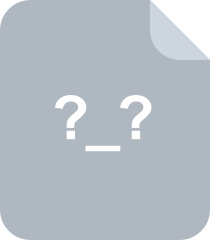
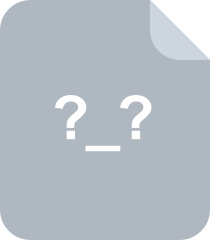
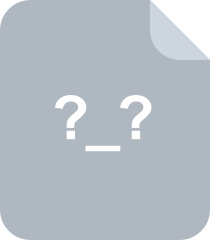
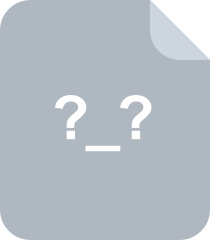

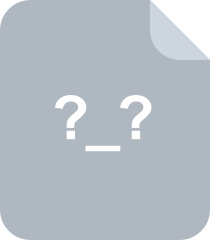
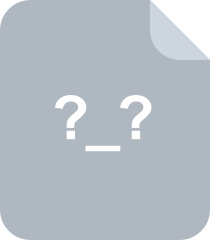
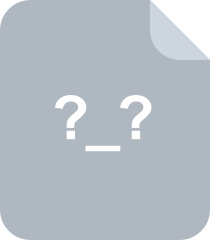
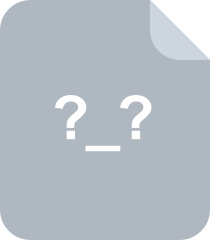
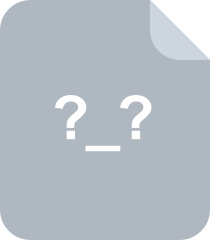
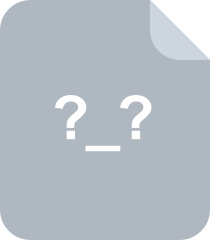

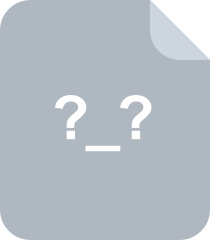
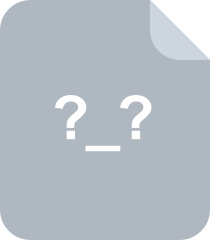

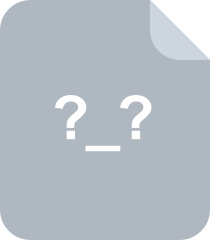
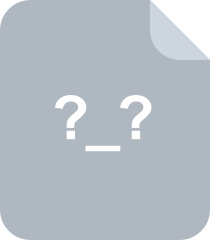
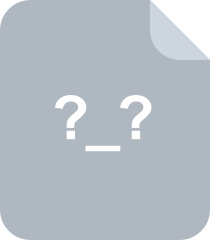
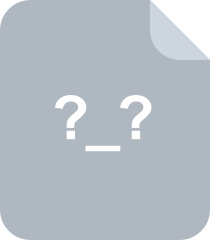
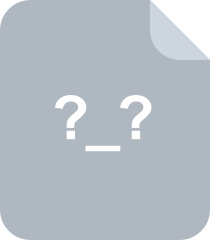
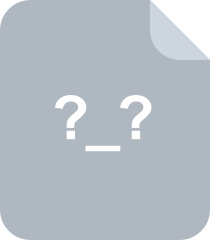
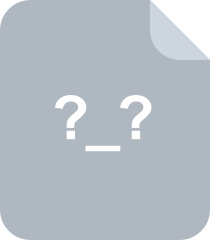
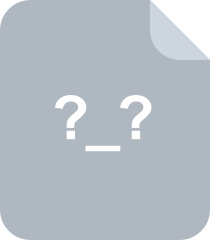
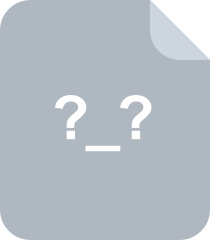
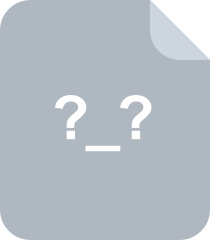
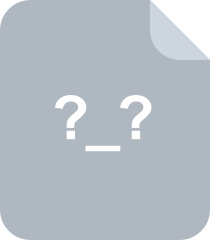
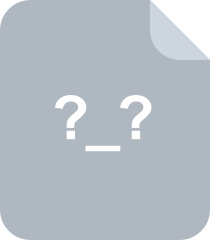
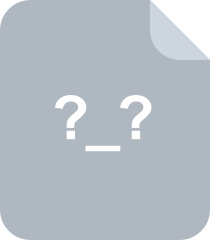
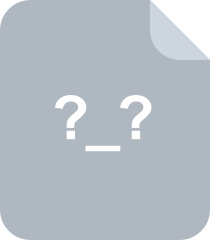
共 94 条
- 1
资源评论
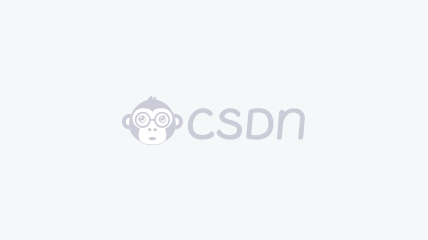
- 陌陌的日记2023-07-25这个网格回测工具在回测速度上表现出色,我可以快速地对不同策略进行测试,提高了工作效率。
- 顾露2023-07-25使用这个网格回测工具,我发现它的界面简洁明了,操作也相对简单,非常适合新手使用。
- 西门镜湖2023-07-25使用这个网格回测工具,我能够对交易策略进行有效地优化,找到更合适的投资策略。
- 深层动力2023-07-25这个网格回测工具提供了丰富的数据分析功能,让我可以深入了解我的交易策略的盈利潜力。
- Msura2023-07-25这个网格回测工具能够有效地分析交易策略,给出合理的回测结果。

topgun2
- 粉丝: 19
- 资源: 266
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

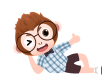
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


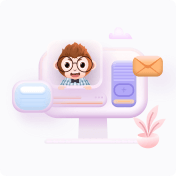
安全验证
文档复制为VIP权益,开通VIP直接复制
