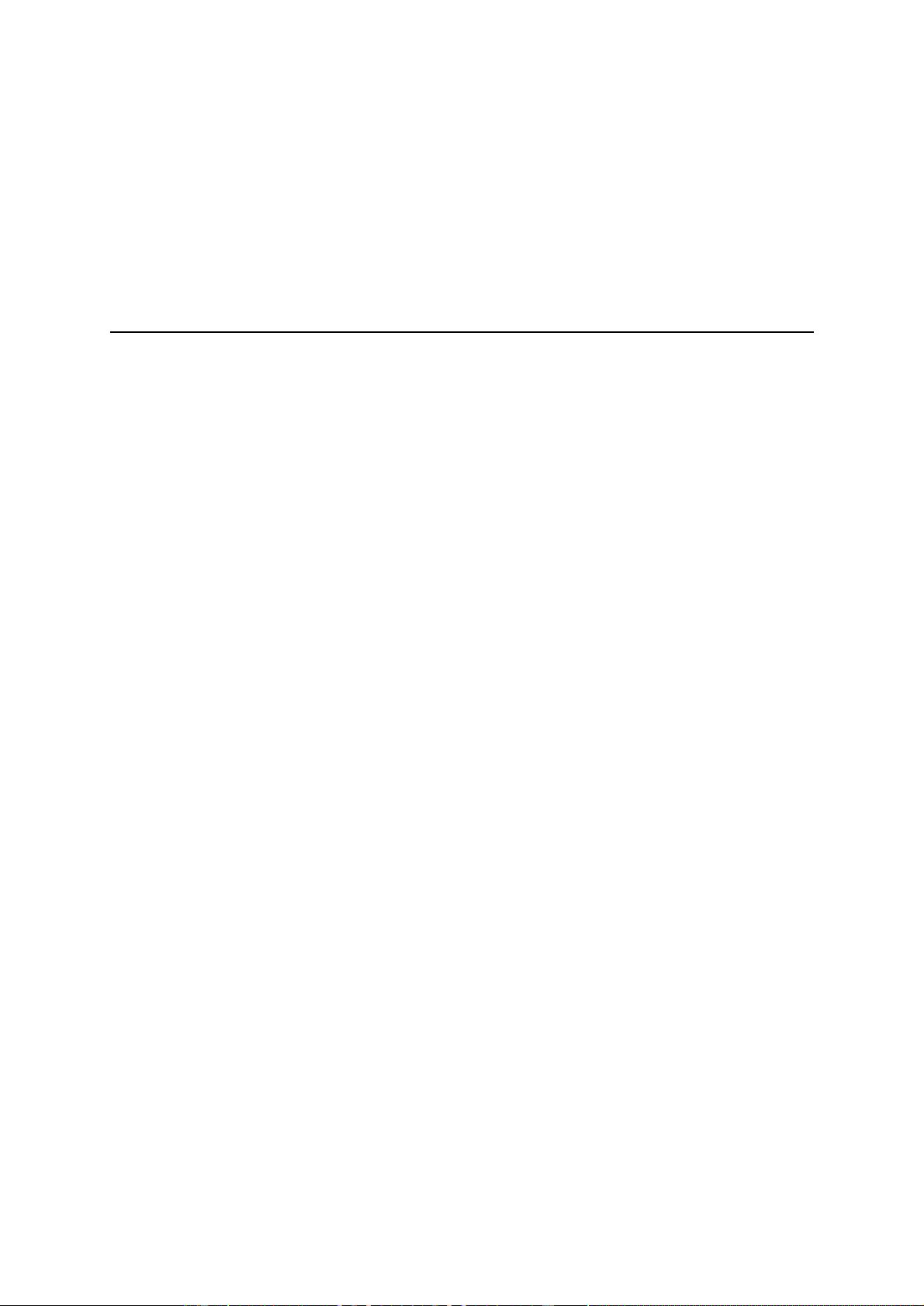
Developing WML applications using
PHP
Presented by developerWorks, your source for great tutorials
ibm.com/developerWorks
Table of Contents
If you're viewing this document online, you can click any of the topics below to link directly to that section.
1. About this tutorial....................................................... 2
2. Wireless Markup Language (WML) ................................. 3
3. PHP:Hypertext Preprocessor (PHP) ................................ 6
4. Generating Dynamic WML using PHP.............................. 9
5. WML and PHP - an example......................................... 11
6. Feedback ................................................................ 15
Developing WML applications using PHP Page 1 of 15
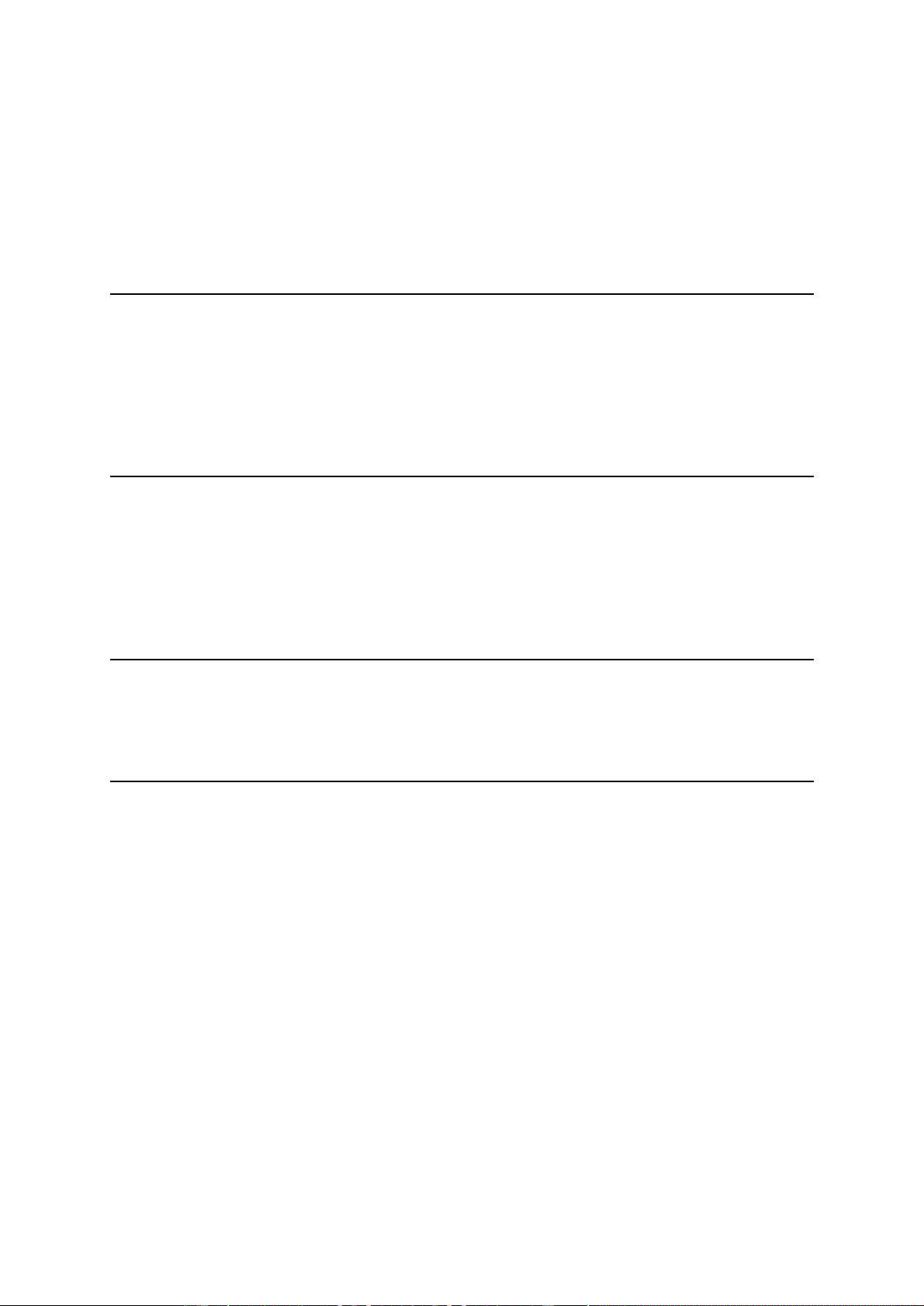
Section 1. About this tutorial
Who should take this tutorial?
The course is intended for developers and technical managers who want to get an
overview and understanding of WML application development using PHP.
About the author
Vivek Malhotra is a wireless technology expert based in the Washington D.C. area.
Vivek has several years of experience developing and implementing wireless
applications and has spoken on expert panels focusing on the wireless industry. You
can contact Vivek with any questions you might have about the content of this tutorial.
Introduction to the tutorial
PHP: Hypertext Preprocessor (PHP) is an open source server-side scripting language
that can be used to dynamically create Wireless Markup Language (WML) based
applications. This tutorial provides an introduction to developing dynamic WML
applications using PHP to serve WAP-enabled wireless devices.
Prerequisites
You should be familiar with basic PHP, WML, and MySQL.
Resources
For additional information and resources, refer to the following sites:
* The Official PHP Language Web site at http://www.php.net/
* The WAP Forum Web site at http://www.wapforum.org
* Get information on MySQL at http://www.mysql.com
* Download the UP SDK and the phone emulator at the Openwave Web site
(http://www.openwave.com/)
* Read the developerWorks article "Developing Wireless Applications"
* IBM and Wireless: Lotus Mobile Services for Domino has been enhanced by the
new Domino Everyplace.
Presented by developerWorks, your source for great tutorials
ibm.com/developerWorks
Developing WML applications using PHP Page 2 of 15
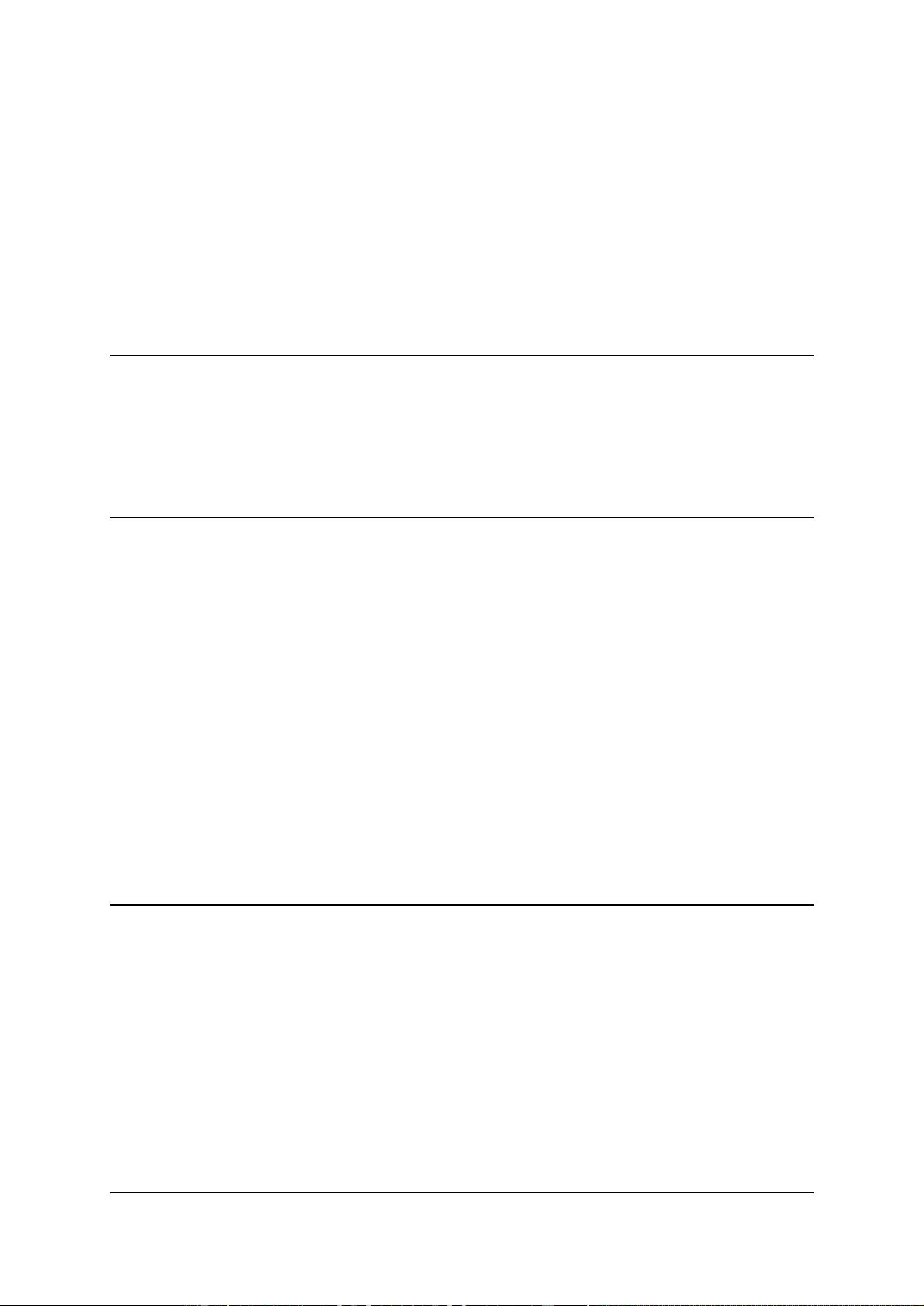
Section 2. Wireless Markup Language (WML)
What is WML?
Wireless Markup Language (WML) is a markup language, based on XML, that allows
information to be presented to WAP-enabled devices. WML specifications are
developed by the WAP Forum, a consortium founded by Nokia, Phone.com (now called
Openwave), Motorola, and Ericsson. The current version of the WAP specifications is
2.0.
Cards and decks
A deck is a single WML page that contains information with which a user interacts. A
card is part of a deck that contains formatting information, content, and processing
instructions. A deck can be made up of one or more cards.
Basic structure of a WML deck
Below is a the basic structure of a WML deck:
<?xml version="1.0"?>
<!DOCTYPE wml PUBLIC "-//WAPFORUM//DTD WML 1.1//EN"
"http://www.wapforum.org/DTD/wml_1.1.xml">
<wml>
<head>
...
</head>
<template>
...
</template>
<card>
...
</card>
</wml>
Hello World! Example in WML
Below is an example of a basic WML application:
<?xml version="1.0"?>
<!DOCTYPE wml PUBLIC "-//WAPFORUM//DTD WML 1.1//EN"
"http://www.wapforum.org/DTD/wml_1.1.xml">
<wml>
<card>
<p>Hello World!</p>
</card>
</wml>
Presented by developerWorks, your source for great tutorials
ibm.com/developerWorks
Developing WML applications using PHP Page 3 of 15
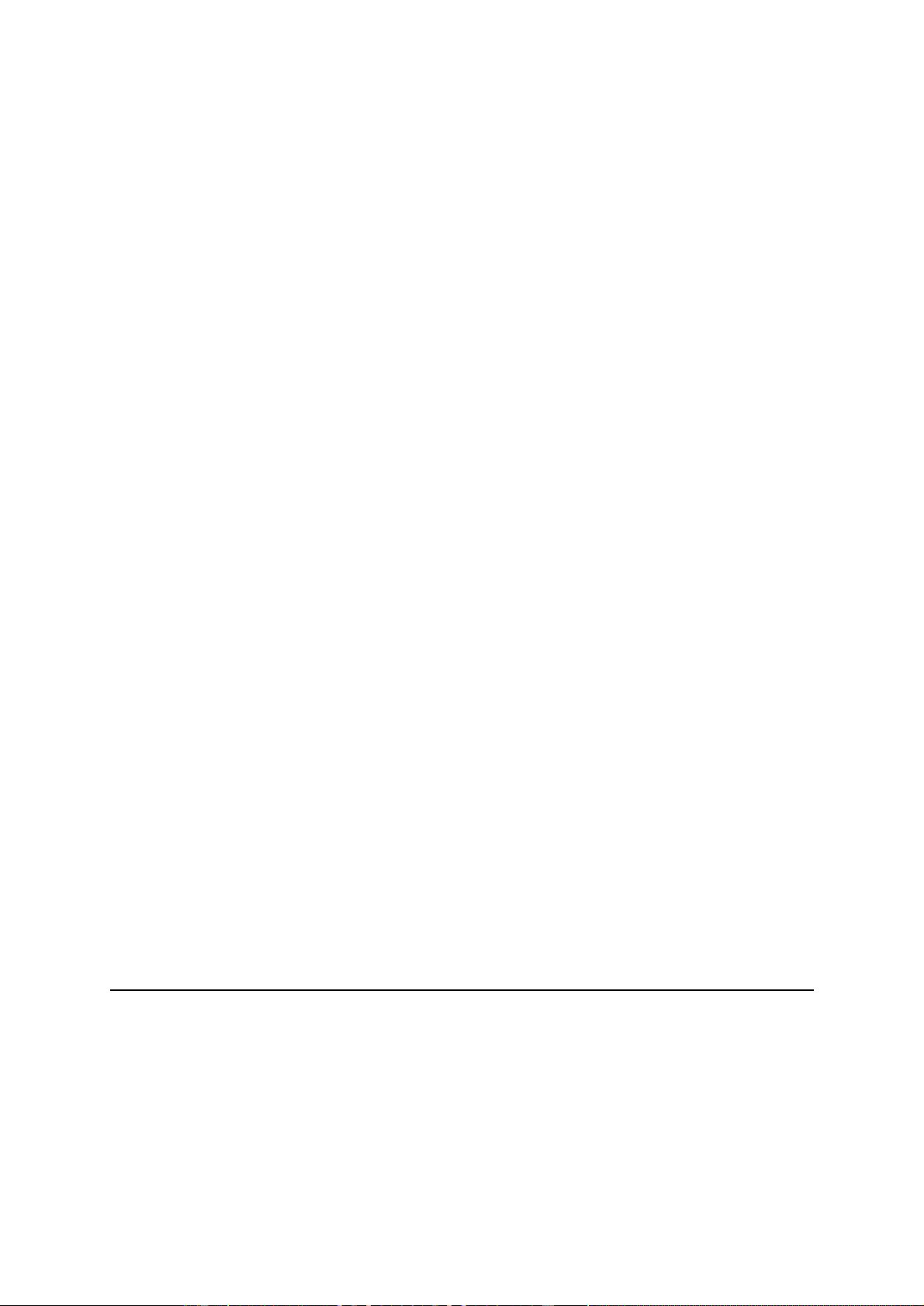
Displaying text
This section describes displaying text to a WAP client. You can display text on a WAP
client that is either formatted or unformatted. The <br/> element -- also called the break
element -- is used for starting a new line of text. The <p> element, or paragraph
element, without any attributes displays unformatted text using the various attributes
supported by the <p> element and can be used for aligning the text. For example,
<?xml version="1.0"?>
<!DOCTYPE wml PUBLIC "-//WAPFORUM//DTD WML 1.1//EN"
"http://www.wapforum.org/DTD/wml_1.1.xml">
<wml>
<card>
<p>
This is a Hello World! application.
This text is unformatted with white space removed
and text wrapped.</p></card>
</wml>
will display text that is unformatted, and
<?xml version="1.0"?>
<!DOCTYPE wml PUBLIC "-//WAPFORUM//DTD
WML1.1//EN"
"http://www.wapforum.org/DTD/wml_1.1.xml">
<wml>
<card>
<p align="left" mode="wrap">
This is a Hello World! application.
This text is left justified with white
space removed and wrapping of text enabled.</p>
</card>
</wml>
will display text that is aligned as left justified with wrapping of text enabled. Tables in
WML can be created using the <table>, <td>, and <tr> elements. Overall alignment of
the table can be controlled, but not individual cells within the table. Here is how you
would create a WML table:
<?xml version="1.0"?>
<!DOCTYPE wml PUBLIC "-//WAPFORUM//DTD WML 1.1//EN"
"http://www.wapforum.org/DTD/wml_1.1.xml">
<wml>
<card>
<p><table columns="2">
<tr><td>col1</td><td>col2</td
></tr></table></p></card></wml>
Links and navigation
The <go> and <anchor> elements allow for navigation between WML cards and decks.
Below is an example of how you would use the <go> element to navigate to specified
URL:
<card id="name">
<do type="type" label="label">
<go href="url"/>
</do>
Presented by developerWorks, your source for great tutorials
ibm.com/developerWorks
Developing WML applications using PHP Page 4 of 15
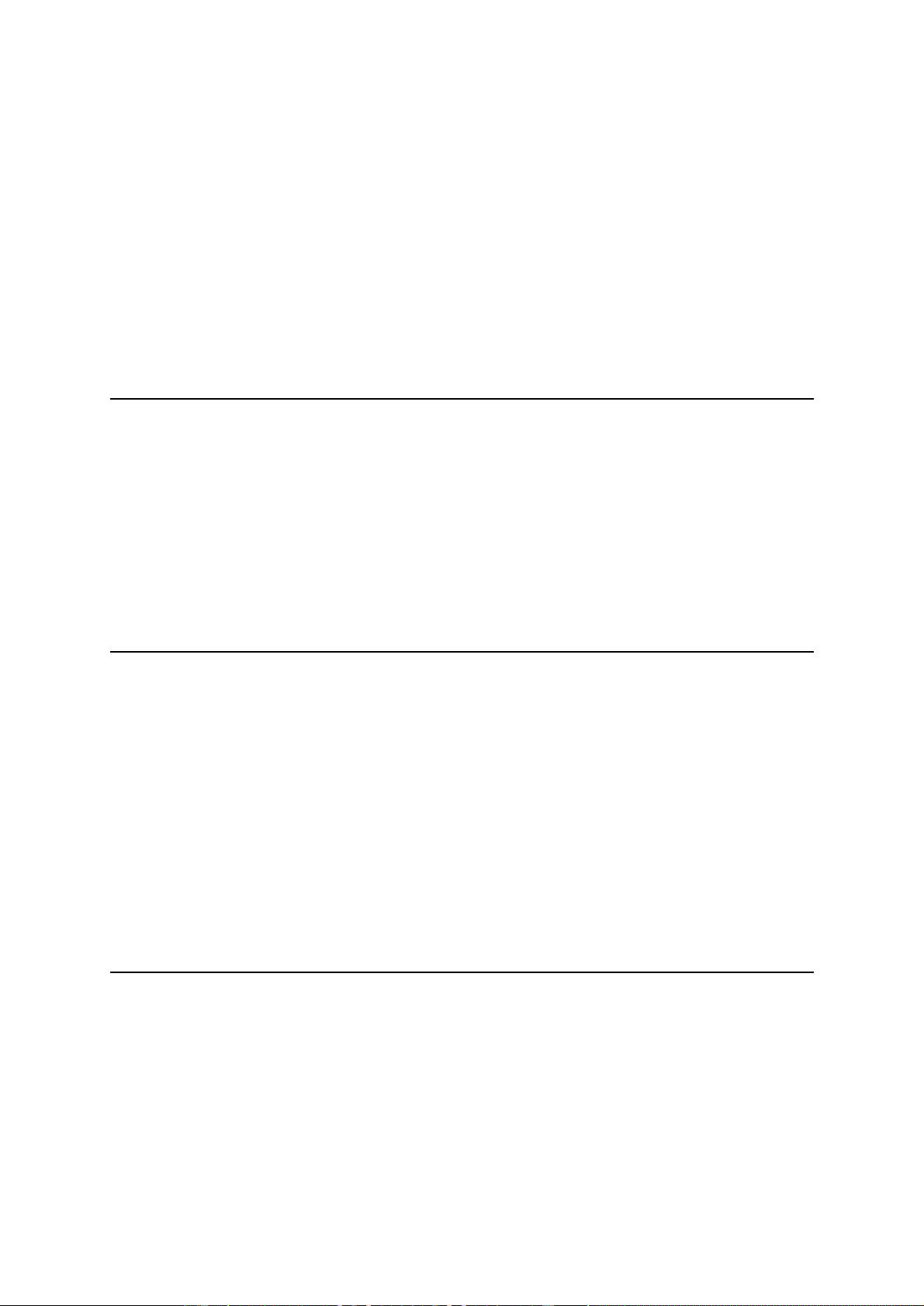
<p>
...
</p>
</card>
where url specifies where to go next. The <anchor> element provides another way to
navigate between cards and decks. By pressing the ACCEPT key the task associated
with the anchored link is invoked. Below is the use of the <anchor> element:
<anchor title="Link1"><go href="#sports"/>Sports</anchor>
<br/>
In this case the link is to the sports card.
User input
The <input> element prompts the user to input text in string or number format. The
following is how you would use the <input> element: Please input your name:
<input name="variable" title="label" format="specifier" maxlength="n"
emptyok="boolean"/>
where name stores the input value, title is the label for the input item, format is the type
of input, maxlength specifies the maximum number of characters a user can enter, and
emptyok specifies whether the field is optional.
Selecting from a list
The <select> element prompts the user to select from a list. The following is how you
would use the <select> element: Please select from the following list:
<select title="label" name="variable" ivalue="default">
<option value="value">content</option>
<option value="value">content</option>
...
</select>
where title is the label for the selected item, name stores the value of selected item,
ivalue is the default item selected if name isn't set to anything. The <option> creates
the list of items where value stores the value of list and content is the list item.
Variables
The <setvar> element is used to set a variables name and assign it a value. Note that
variable names are case sensitive. Here is how you would create a variable and assign
it a value:
<setvar name="id" value="123"/>
where the variable name is id and 123 is its value. The id variable can be referenced by
$id.
Presented by developerWorks, your source for great tutorials
ibm.com/developerWorks
Developing WML applications using PHP Page 5 of 15