/*
+---------------------------------------------------------------------------+
| OpenX v2.8 |
| ========= |
| |
| Copyright (c) 2003-2009 OpenX Limited |
| For contact details, see: http://www.openx.org/ |
| |
| This program is free software; you can redistribute it and/or modify |
| it under the terms of the GNU General Public License as published by |
| the Free Software Foundation; either version 2 of the License, or |
| (at your option) any later version. |
| |
| This program is distributed in the hope that it will be useful, |
| but WITHOUT ANY WARRANTY; without even the implied warranty of |
| MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the |
| GNU General Public License for more details. |
| |
| You should have received a copy of the GNU General Public License |
| along with this program; if not, write to the Free Software |
| Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA |
+---------------------------------------------------------------------------+
$Id:$
*/
package org.openads.proxy;
import java.io.IOException;
import java.net.URL;
import java.util.Date;
import java.util.Map;
import org.apache.xmlrpc.XmlRpcException;
/**
* The Class OpenAdsApiXmlRpcProxy.
*
* @author Andriy Petlyovanyy <apetlyovanyy@lohika.com>
*/
public class OpenAdsApiXmlRpcProxy {
private String host;
private String basepath;
private int port;
private boolean ssl;
private String sessionId;
private LogonService logonService;
private AgencyService agencyService;
private AdvertiserService advertiserService;
private CampaignService campaignService;
private BannerService bannerService;
private PublisherService publisherService;
private ZoneService zoneService;
/**
* Instantiates a new open ads api xml rpc proxy.
*
* @param host
* the host
* @param basepath
* the basepath
* @param port
* the port
* @param ssl
* the ssl
*/
public OpenAdsApiXmlRpcProxy(String host, String basepath, int port,
boolean ssl) {
this.host = host;
this.basepath = basepath;
this.port = port;
this.ssl = ssl;
try {
String protocol = this.ssl ? "https" : "http";
URL url = new URL(protocol, this.host, this.port, "/"
+ this.basepath);
logonService = new LogonService(url.toString());
agencyService = new AgencyService(url.toString());
advertiserService = new AdvertiserService(url.toString());
campaignService = new CampaignService(url.toString());
bannerService = new BannerService(url.toString());
publisherService = new PublisherService(url.toString());
zoneService = new ZoneService(url.toString());
} catch (Exception e) {
throw new RuntimeException(e.getMessage());
}
}
/**
* Instantiates a new open ads api xml rpc proxy.
*
* @param host
* the host
* @param basepath
* the basepath
*/
public OpenAdsApiXmlRpcProxy(String host, String basepath) {
this(host, basepath, -1, false);
}
/**
* This method is used to initialize session of user.
*
* @param username
* the username
* @param password
* the password
*
* @return True if the operation was successful
*
* @throws XmlRpcException, IOException
* the xml rpc exception
*/
public Boolean logon(String username, String password)
throws XmlRpcException, IOException {
try {
sessionId = logonService.logon(username, password);
if (isValidSessionId()) {
logonService.setSessionId(sessionId);
agencyService.setSessionId(sessionId);
advertiserService.setSessionId(sessionId);
campaignService.setSessionId(sessionId);
bannerService.setSessionId(sessionId);
publisherService.setSessionId(sessionId);
zoneService.setSessionId(sessionId);
return true;
} else {
return false;
}
} catch (XmlRpcException e) {
sessionId = null;
throw e;
}
}
/**
* Checks if is valid session id.
*
* @return true, if is valid session id
*/
private boolean isValidSessionId() {
return (sessionId != null);
}
/**
* Verify logon.
*/
private void verifyLogon() {
if (!isValidSessionId())
throw new IllegalArgumentException("Not logined to server");
}
/**
* This method is used to abandon user session.
*
* @return True if the operation was successful
*
* @throws XmlRpcException, IOException
* the xml rpc exception
*/
public Boolean logoff() throws XmlRpcException, IOException {
verifyLogon();
Boolean result = logonService.logoff();
if (result)
sessionId = null;
return result;
}
/**
* This method adds an agency to the system.
*
* @param params -
* Structure with the following fields:
* <ol>
* <li> agencyName String (255) The name of the agency
* <li> contactName String (255) The name of the contact
* <li> emailAddress String (64) The email address of the contact
* <li> username String (64) The username of the contact used to
* log into OA
* <li> password String (64) The password of the contact used to
* log into OA
* <ol>
*
* @return The ID of the newly created agency
*
* @throws XmlRpcException, IOException
* the xml rpc exception
*
* @see Webservices API
*/
public Integer addAgency(Map params) throws XmlRpcException, IOException {
verifyLogon();
return agencyService.addAgency(params);
}
/**
* This method modifies an existing agency.
*
* @param params
* Structure with the following fields:
* <ol>
* <li> agencyId integer The ID of the agency
* <li> agencyName String (255) The name of the agency
* <li> contactName String (255) The name of the contact
* <li> emailAddress String (64) The email address of the contact
* <li> username String (64) The username of the contact used to
* log into OA
* <li> password String (64) The password of the contact used to
* log into OA
* <ol>
*
* @return True if the operation was successful
*
* @throws XmlRpcException, IOException
* the xml rpc exception
*
*
*/
public Boolean modifyAgency(Map params) throws XmlRpcException, IOException {
verifyLogon();
return agencyService.modifyAgency(params);
}
/**
* Delete agency.
*
* @param id
* the id
*
* @return True if the operation was successful
*
* @throws XmlRpcException, IOException
* the xml rpc exception
*/
public Boolean deleteAgency(Integer id) throws XmlRpcException, IOException {
verifyLogon();
return agencyService.deleteAgency(id);
}
/**
* Gets the agency.
*
* @param id
* the id
*
* @return map agency whith key:
*
* <ol>
* <li> agencyName String (255) The name of the agency
* <li> contactName String (255) The name of the contact
* <li> emailAddress String (64) The email address of the contact
* <li> username String (64) The username of the contact used to log into OA
* <li> password String (64) The password of the contact used to log into OA
* </ol>
*
* @throws XmlRpcException, IOException
* the xml rpc exception
*/
public Map getAgency(Integ
没有合适的资源?快使用搜索试试~ 我知道了~
开源广告管理平台openx 多国语言

温馨提示
开源广告管理平台开源广告管理平台开源广告管理平台开源广告管理平台
资源推荐
资源详情
资源评论
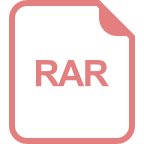
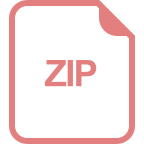
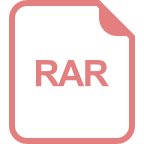
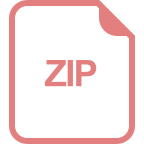
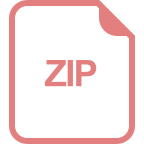
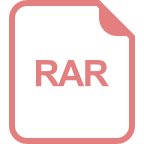
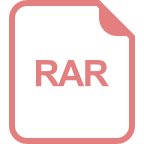
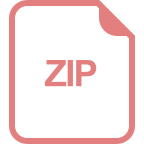
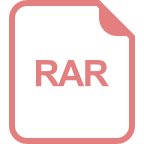
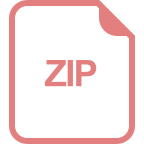
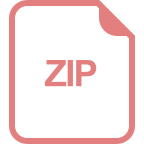
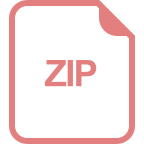
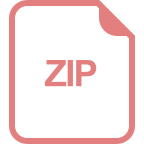
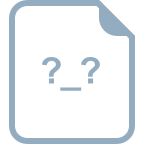
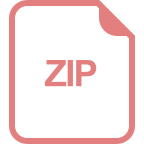
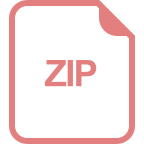
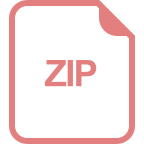
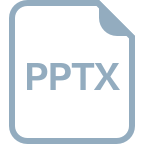
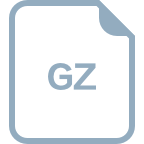
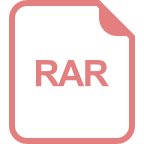
收起资源包目录

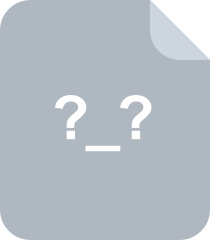
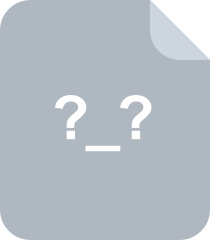
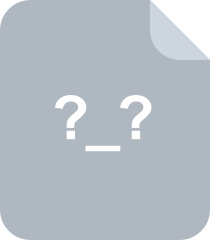
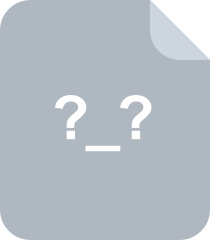
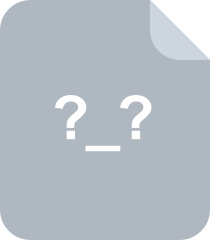
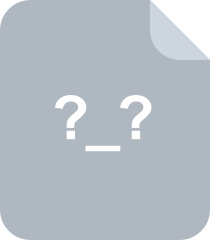
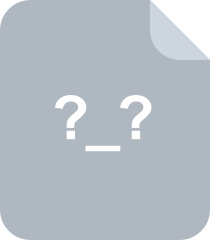
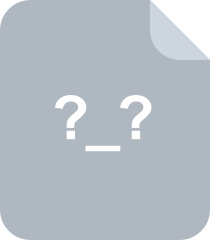
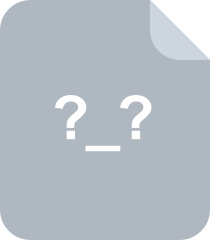
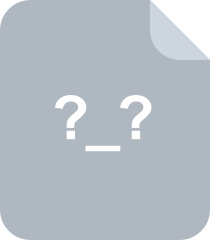
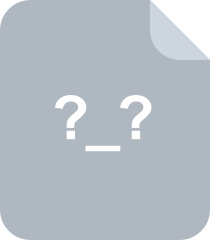
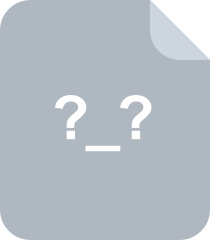
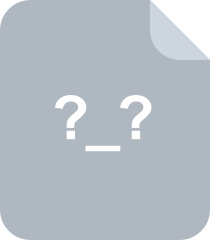
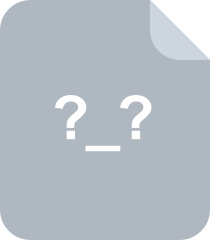
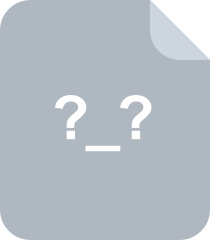
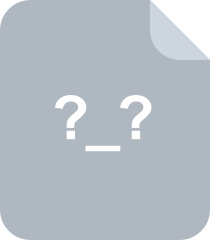
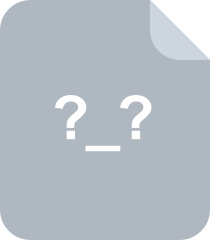
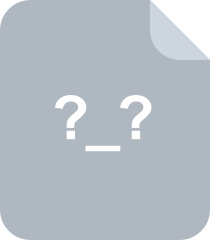
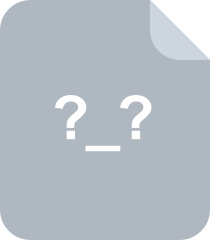
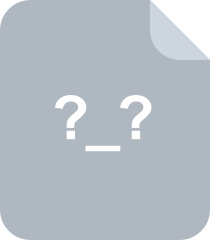
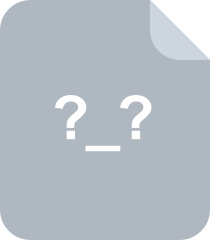
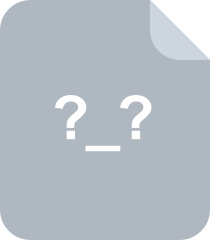
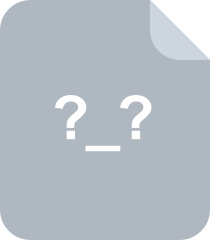
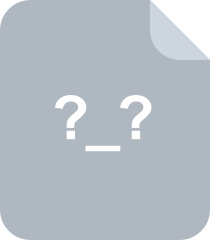
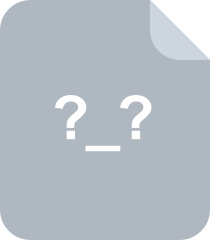
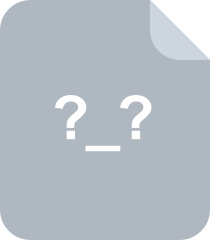
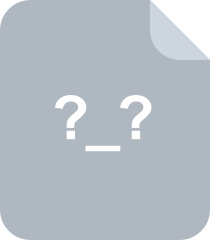
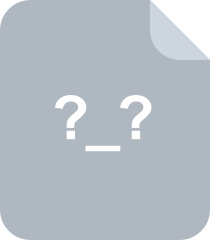
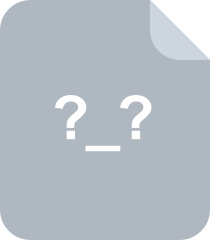
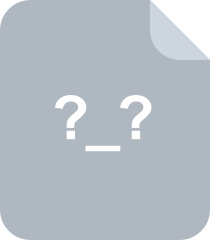
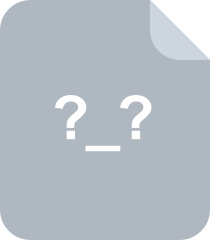
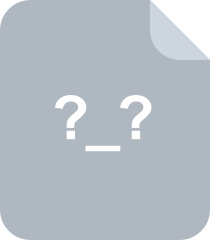
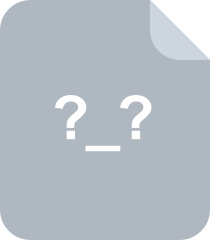
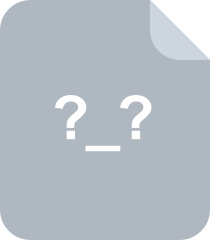
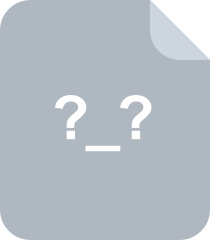
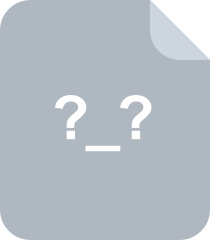
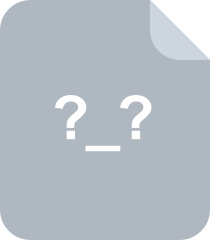
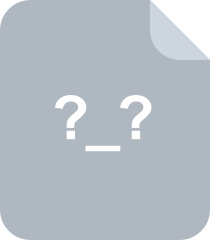
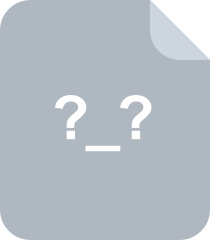
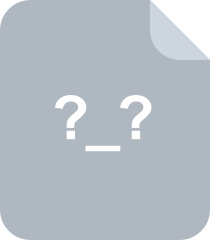
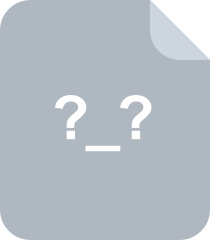
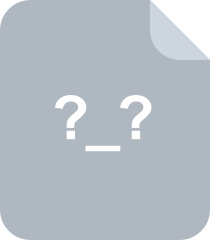
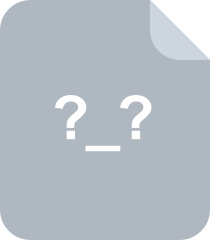
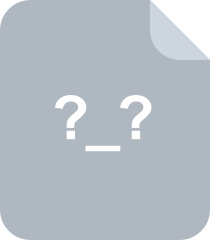
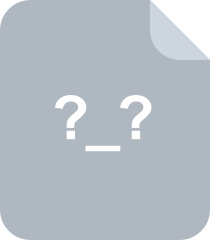
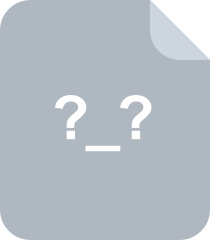
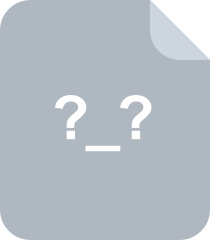
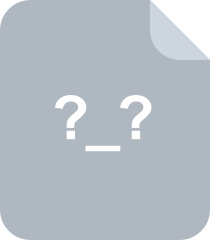
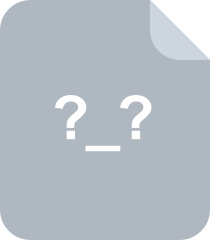
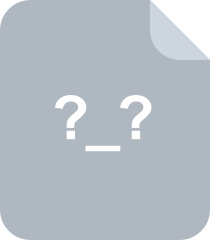
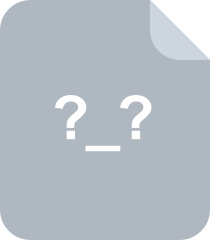
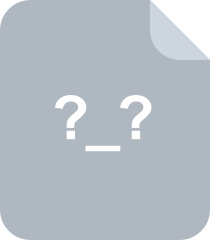
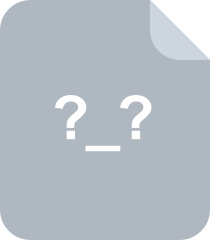
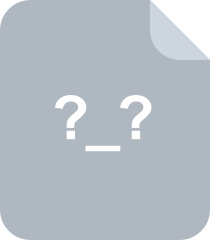
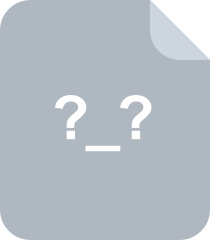
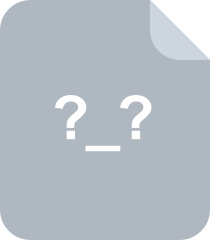
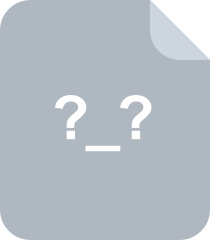
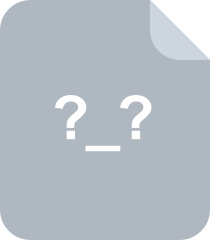
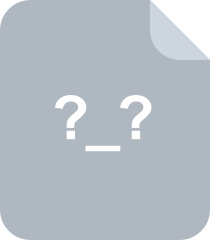
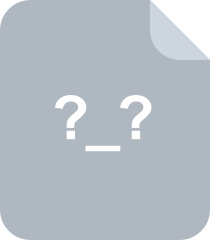
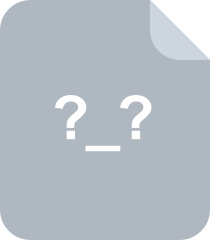
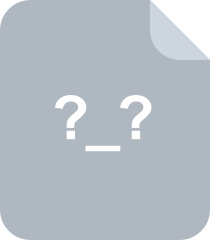
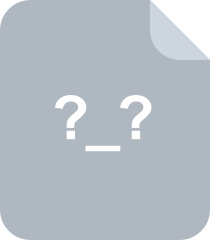
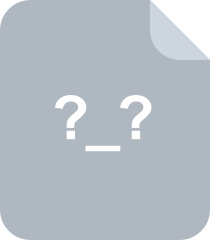
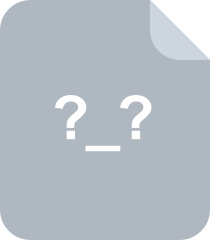
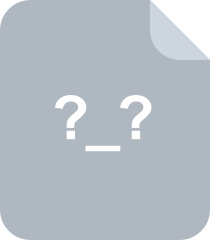
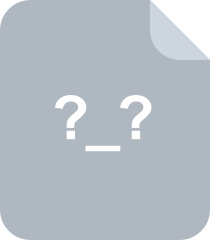
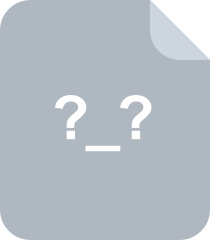
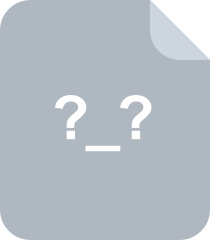
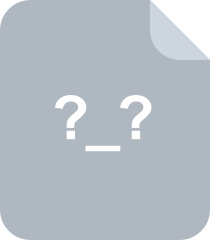
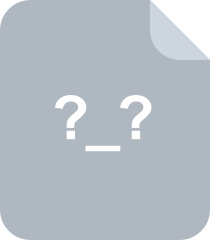
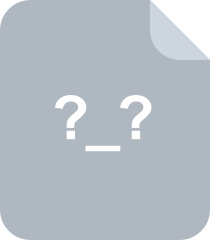
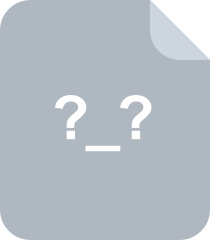
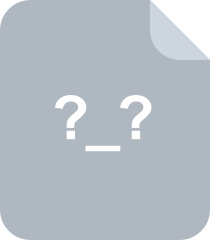
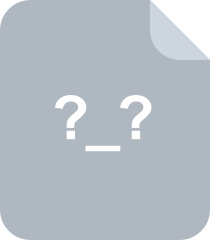
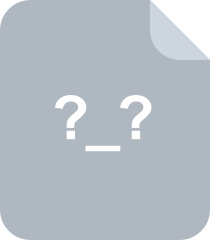
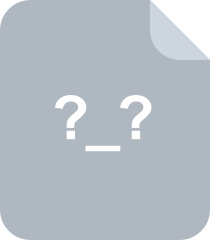
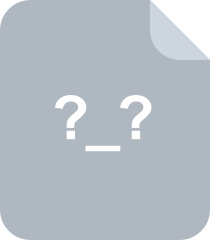
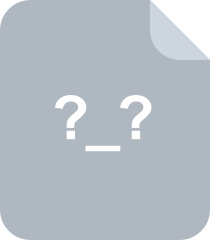
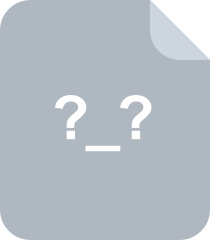
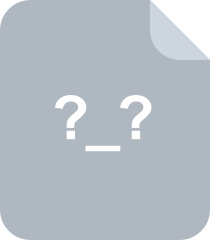
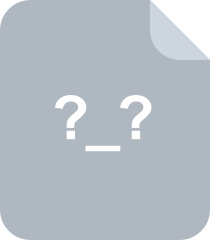
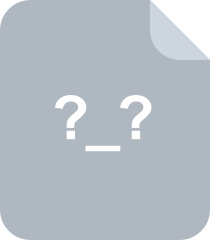
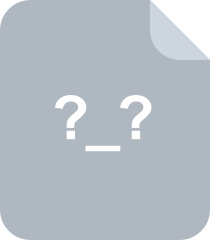
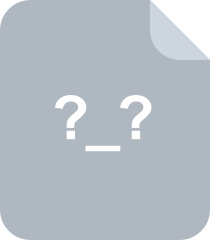
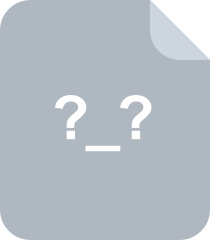
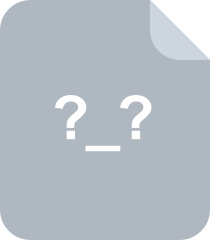
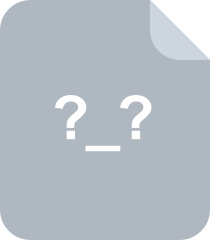
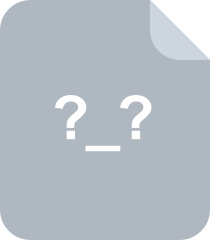
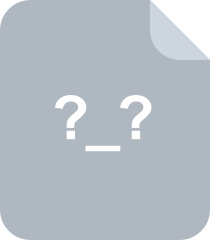
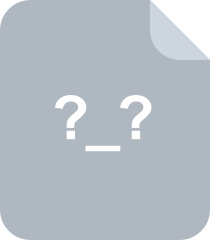
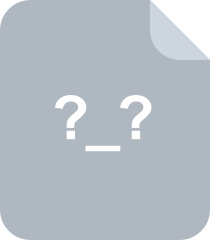
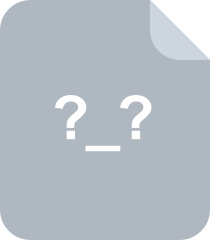
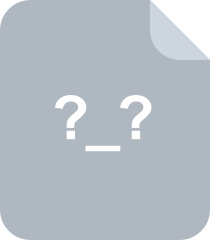
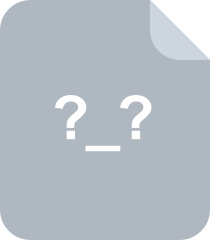
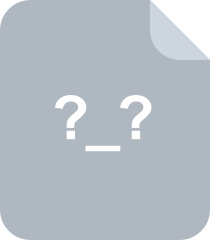
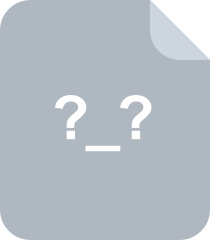
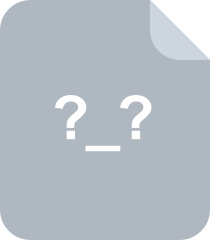
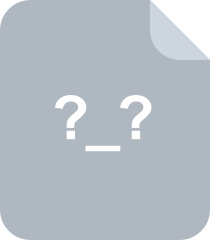
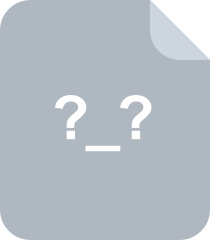
共 3363 条
- 1
- 2
- 3
- 4
- 5
- 6
- 34
资源评论
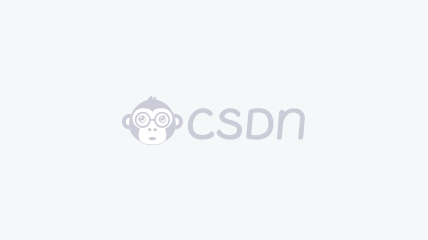
- 达夫_zh2014-02-25中文有乱码,怎么回事呢?
- nsu0022014-08-19有乱码 再看看怎么弄
- faryoungchen2014-08-28不错,但是略微简单了点儿
- andy_melody2012-10-09不错,但是略微简单了点儿

top369ying
- 粉丝: 0
- 资源: 13
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

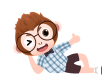
最新资源
- GigaDevice.GD32F4xx-DFP.2.1.0 器件安装包
- 智慧校园数字孪生,三维可视化
- 多种土地使用类型图像分类数据集【已标注,约30,000张数据】
- 3.0(1).docx
- 国产文本编辑器:EverEdit用户手册 1.1.0
- 多边形框架物体检测27-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 基于stm32风速风向测量仪V2.0
- 高效排序算法:快速排序Java与Python实现详解
- Metropolis-Hastings算法和吉布斯采样(Gibbs sampling)算法Python代码实现
- IP网络的仿真及实验.doc
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


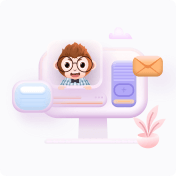
安全验证
文档复制为VIP权益,开通VIP直接复制
