
tomjun1986
- 粉丝: 1
- 资源: 5
最新资源
- 【创新发文】秃鹰算法BES-DELM预测(多输入单输出)【含Matlab源码 6953期】.zip
- 【创新发文】向量加权平均算法INFO-DELM预测(多输入单输出)【含Matlab源码 6955期】.zip
- 【创新发文】向量加权平均算法INFO-DELM预测(多输入单输出)【含Matlab源码 6955期】.zip
- 【创新发文】星雀算法NOA-DELM预测(多输入单输出)【含Matlab源码 6956期】.zip
- 【创新发文】星雀算法NOA-DELM预测(多输入单输出)【含Matlab源码 6956期】.zip
- 【创新发文】雪融算法SAO-DELM预测(多输入单输出)【含Matlab源码 6957期】.zip
- 【创新发文】雪融算法SAO-DELM预测(多输入单输出)【含Matlab源码 6957期】.zip
- 【创新发文】天鹰算法AO-DELM预测(多输入单输出)【含Matlab源码 6952期】.zip
- 【创新发文】天鹰算法AO-DELM预测(多输入单输出)【含Matlab源码 6952期】.zip
- 【创新发文】雾凇算法RIME-DELM预测(多输入单输出)【含Matlab源码 6954期】.zip
- 【创新发文】雾凇算法RIME-DELM预测(多输入单输出)【含Matlab源码 6954期】.zip
- 【创新发文】遗传算法GA-DELM预测(多输入单输出)【含Matlab源码 6958期】.zip
- 【创新发文】遗传算法GA-DELM预测(多输入单输出)【含Matlab源码 6958期】.zip
- 【创新发文】蚁狮算法ALO-DELM预测(多输入单输出)【含Matlab源码 6959期】.zip
- 【创新发文】蚁狮算法ALO-DELM预测(多输入单输出)【含Matlab源码 6959期】.zip
- 【创新发文】引力搜索算法GSA-DELM预测(多输入单输出)【含Matlab源码 6960期】.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


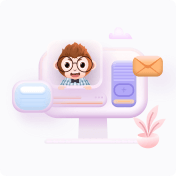