import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.Toolkit;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import javax.swing.JCheckBoxMenuItem;
import javax.swing.JColorChooser;
import javax.swing.JFrame;
import javax.swing.JMenu;
import javax.swing.JMenuBar;
import javax.swing.JMenuItem;
import javax.swing.JOptionPane;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
public class ErsBlocksGame extends JFrame{
public final static int PER_LINE_SCORE=100;
public final static int PER_LEVEL_SCORE=PER_LINE_SCORE*20;
public final static int MAX_LEVEL=10;
public final static int DEFAULT_LEVEL =5;
private GameCanvas canvas;
private ErsBlock block;
private ControlPanel ctrlPanel;
private boolean playing = false;
private JMenuBar bar =new JMenuBar();
private JMenu
mGame=new JMenu("游戏"),
mControl =new JMenu("控制"),
mWindowStyle = new JMenu("窗口风格"),
mInfo = new JMenu("帮助");
private JMenuItem
miNewGame = new JMenuItem("新游戏"),
miSetBlockColor = new JMenuItem("设置方块颜色"),
miSetBackColor = new JMenuItem("设置背景颜色"),
miTurnHarder = new JMenuItem("增加难度"),
miTurnEasier = new JMenuItem("降低难度"),
miExit = new JMenuItem("退出"),
miPlay = new JMenuItem("开始"),
miPause = new JMenuItem("暂停"),
miResume = new JMenuItem("继续"),
miStop = new JMenuItem("停止"),
miAuthor = new JMenuItem("作者:clarsue"),
miSourceInfo = new JMenuItem("版本:1.0");
private JCheckBoxMenuItem
miAsWindows = new JCheckBoxMenuItem("windows"),
miAsMotif = new JCheckBoxMenuItem("Motif"),
miAsMetal = new JCheckBoxMenuItem("Metal",true);
public ErsBlocksGame(String title){
super(title);
setSize(315,392);
Dimension scrSize = Toolkit.getDefaultToolkit().getScreenSize();
setLocation((scrSize.width-getSize().width)/2,(scrSize.height-getSize().height)/2);
createMenu();
Container container=getContentPane();
container.setLayout(new BorderLayout(6,0));
canvas = new GameCanvas(20,12);
ctrlPanel = new ControlPanel(this);
container.add(canvas,BorderLayout.CENTER);
container.add(ctrlPanel,BorderLayout.EAST);
miNewGame.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
stopGame();
reset();
setLevel(DEFAULT_LEVEL);
}
});
miTurnHarder.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
int curLevel = getLevel();
if(curLevel < MAX_LEVEL) setLevel(curLevel+1);
}
});
miTurnEasier.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
int curLevel = getLevel();
if(curLevel >0) setLevel(curLevel-1);
}
});
miExit.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
System.exit(0);
}
});
miPlay.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
playGame();
}
});
miPause.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
pauseGame();
}
});
miResume.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
resumeGame();
}
});
miStop.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
stopGame();
}
});
miAsWindows.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
String plaf="com.sun.java.swing.plaf.windows.WindowsLookAndFeel";
setWindowStyle(plaf);
canvas.fanning();
ctrlPanel.fanning();
miAsWindows.setState(true);
miAsMotif.setSelected(false);
miAsMetal.setState(false);
}
});
miAsMotif.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
String plaf="com.sun.java.swing.plaf.motif.MotifLookAndFeel";
setWindowStyle(plaf);
canvas.fanning();
ctrlPanel.fanning();
miAsWindows.setState(false);
miAsMotif.setSelected(true);
miAsMetal.setState(false);
}
});
miAsMetal.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
String plaf="javax.swing.plaf.metal.MetalLookAndFeel";
setWindowStyle(plaf);
canvas.fanning();
ctrlPanel.fanning();
miAsWindows.setState(false);
miAsMotif.setSelected(false);
miAsMetal.setState(true);
}
});
miSetBlockColor.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
Color newColor = JColorChooser.showDialog(ErsBlocksGame.this,"设置方块颜色",canvas.getFrontColor());
if(newColor != null)
canvas.setFrontColor(newColor);
}
});
miSetBackColor.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
Color newColor = JColorChooser.showDialog(ErsBlocksGame.this,"设置背景颜色",canvas.getBackColor());
if(newColor != null)
canvas.setBackColor(newColor);
}
});
miAuthor.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
JOptionPane.showMessageDialog(ErsBlocksGame.this, "作者:clarksue\n"+"邮箱:clarksue@163.com\n");
}
});
miSourceInfo.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent ae){
JOptionPane.showMessageDialog(ErsBlocksGame.this, "版本:1.0\n"+"时间:2009/01/17\n");
}
});
addWindowListener(new WindowAdapter(){
public void windowClosing(WindowEvent we){
stopGame();
System.exit(0);
}
});
addComponentListener(new ComponentAdapter(){
public void componentResized(ComponentEvent ce){
canvas.fanning();
}
});
setVisible(true);
canvas.fanning();
}
public void reset(){
ctrlPanel.reset();
canvas.reset();
}
public boolean isPlaying(){
return playing;
}
public ErsBlock getCurBlock(){
return block;
}
public GameCanvas getCanvas(){
return canvas;
}
public void playGame(){
play();
ctrlPanel.setPlayButtonEnable(false);
miPlay.setEnabled(false);
ctrlPanel.requestFocus();
}
public void pauseGame(){
if(block!=null) block.pauseMove();
ctrlPanel.setPauseButtonEnable(false);
miPause.setEnabled(false);
miResume.setEnabled(false);
ctrlPanel.requestFocus();
}
public void resumeGame(){
if(block!=null) block.resumeMove();
ctrlPanel.setPauseButtonEnable(true);
miPause.setEnabled(true);
miResume.setEnabled(false);
ctrlPanel.requestFocus();
}
public void stopGame(){
playing = false;
if(block!= null) block.stopMove();
miPlay.setEnabled(true);
miPause.setEnabled(true);
miResume.setEnabled(false);
ctrlPanel.setPlayButtonEnable(true);
ctrlPanel.setPauseButtonEnable(true);
}
public int getLevel(){
return ctrlPanel.getLevel();
}
public void setLevel(int level){
if(level<11&&level>0) ctrlPanel.setLevel(level);
}
public int getScore(){
if(canvas !=null) return canvas.getScore();
return 0;
}
public int getScoreForLevelUpdate(){
if(canvas!=null) return canvas.getScoreForLevelUpdate();
return 0;
}
public boolean levelUpdate(){
int curLevel = getLevel();
if(curLevel<MAX_LEVEL){
setLevel(curLevel+1);
canvas.resetScoreForLevelUpdate();
return true;
}
return false;
}
private void play(){
reset();
playing=true;
Thread thread = new Thread(new Game());
thread.start();
}
private void reportGameOver(){
JOptionPane.showMessageDialog(this, "游戏结束");
}
private void createMenu(){
bar.add(mGame);
bar.add(mCo
没有合适的资源?快使用搜索试试~ 我知道了~
俄罗斯方块JAVA源代码
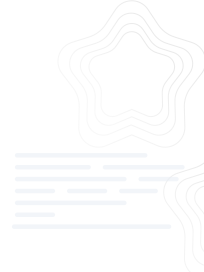
共39个文件
class:32个
java:5个
classpath:1个


温馨提示
俄罗斯方块游戏的JAVA源代码,用eclipse编写。 版本:1.0 待改进功能: 1.方块颜色彩色功能(现为单色)。 2.可增加随机生成方块以增加难度功能。 3.可增加背景音乐功能。 4.可扩展为双人对战版本。并增加聊天功能。
资源推荐
资源详情
资源评论
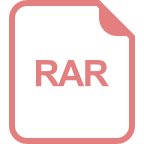
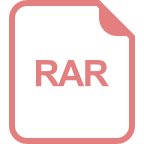
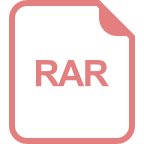
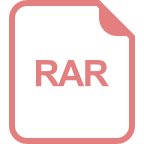
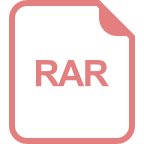
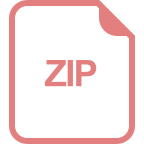
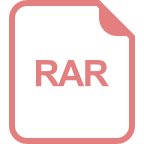
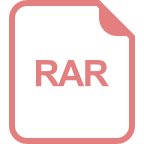
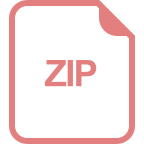
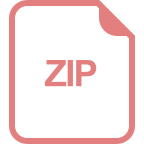
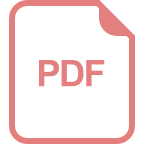
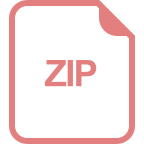
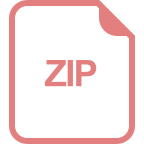
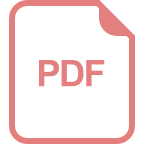
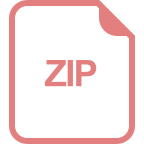
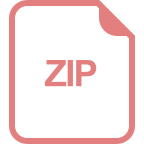
收起资源包目录


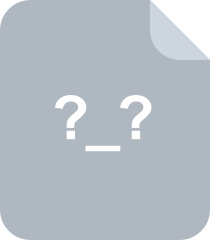

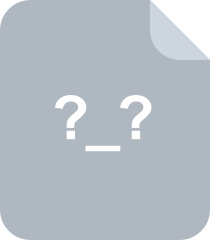
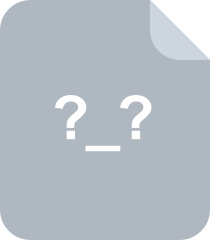
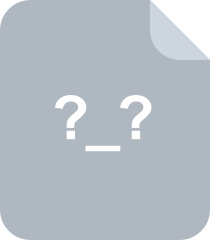
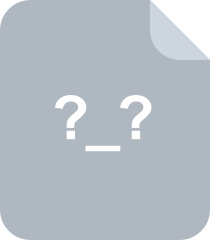
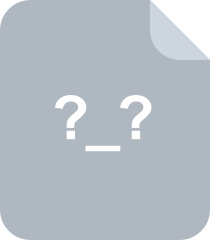
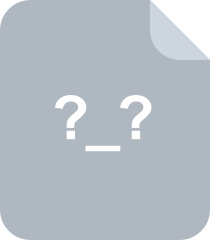
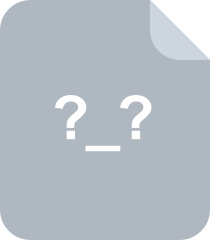
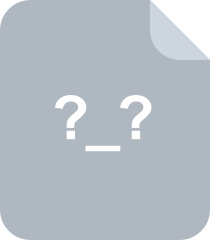
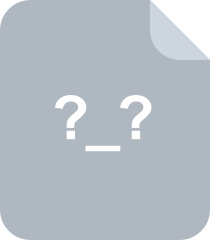
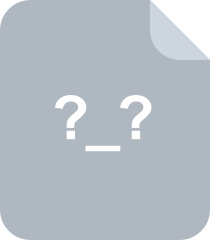
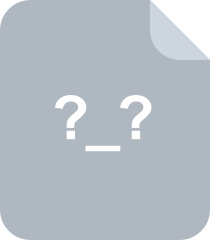
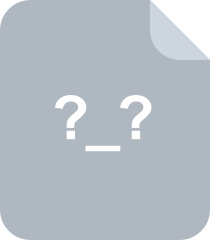
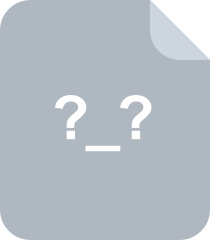
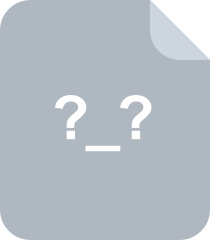
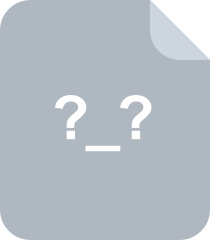
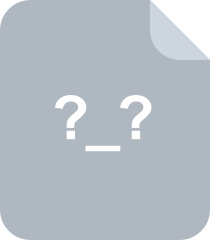
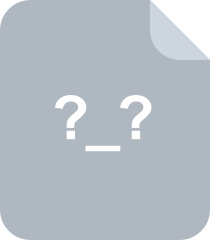
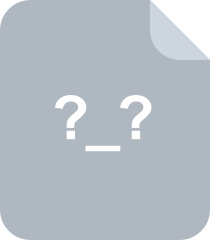
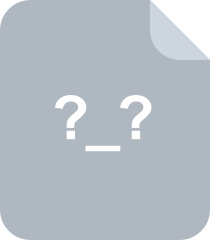
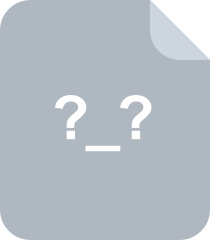
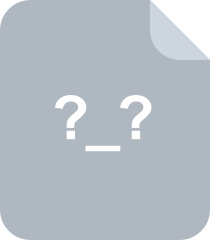
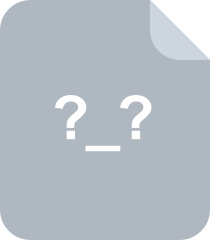
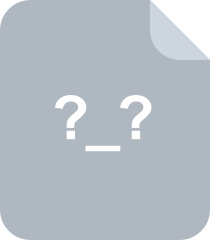
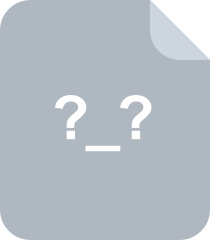
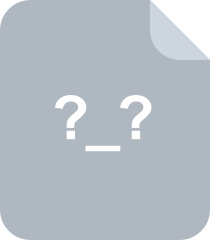
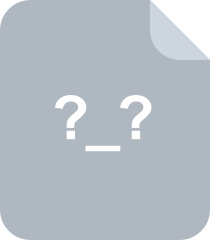
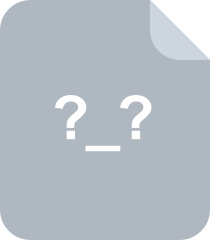
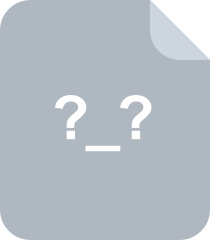
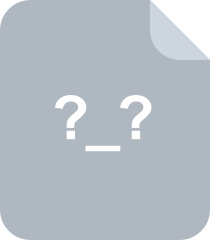
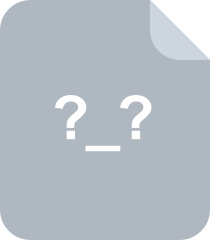
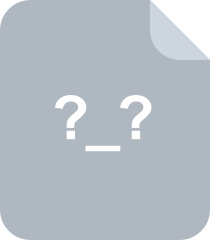
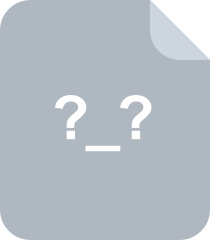

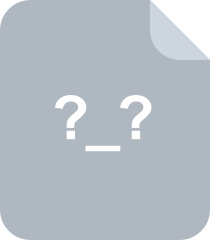
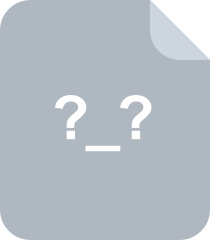
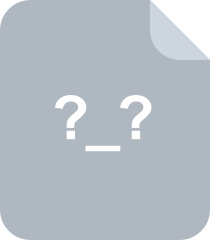
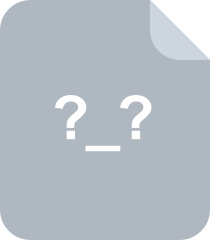
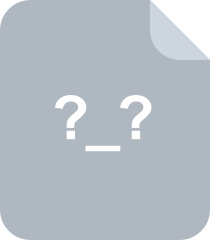
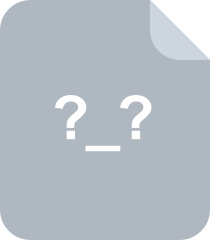
共 39 条
- 1
资源评论
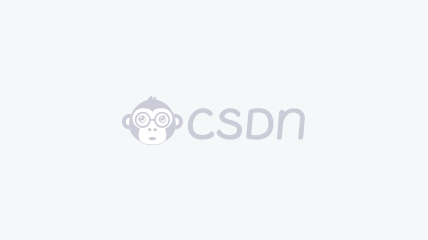
- 战歌IT2014-08-10还可以,就是要成功运行还需要自己的修改。 ..
- Hben1232012-06-20修改一些地方还是可以的
- fyanli8882012-05-18还可以,就是要成功运行还需要自己的修改。
- 弹你个脑蹦瓜2014-06-08做的还是不错的

暗夜精灵Ne
- 粉丝: 0
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

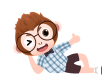
最新资源
- Simulink&Stateflow
- selective-scan-0.0.2-cp310-cp310-win-amd64.whl.zip(包含core)
- Lazarus,FPC 的 DirectX 示例.zip
- SPI软件模拟读写W25Q128
- springboot入门.docx
- selective-scan-0.0.2-cp310-cp310-win-amd64.whl.zip(不含core)
- KatanaZero DirectX MFC工具.zip
- 捕食者直升机无人机3D
- Johnny Chung Lee 的 Wii Remote Head Tracking 代码的 git 存储库(不是我的项目).zip
- 离线下,给用户机器封U盘口 可以自定义密码,原始密码6个1 该工具权限>本地组策略等
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


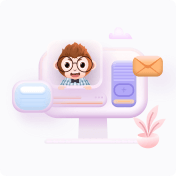
安全验证
文档复制为VIP权益,开通VIP直接复制
