package net.livebookstore.dao.jdbc;
import static org.junit.Assert.*;
import java.io.InputStream;
import java.io.Reader;
import java.math.BigDecimal;
import java.net.URL;
import java.sql.*;
import java.util.Calendar;
import java.util.Map;
import javax.persistence.*;
import org.junit.Test;
public class AbstractRowMapperTest {
private static final DomainObject DOMAIN_OBJECT;
static {
DOMAIN_OBJECT = new DomainObject();
DOMAIN_OBJECT.setId(new Integer(1024));
DOMAIN_OBJECT.setUsername("reg_user");
DOMAIN_OBJECT.setPassword("test*12345");
DOMAIN_OBJECT.setGender(true);
DOMAIN_OBJECT.setBonus(1982.0214f);
DOMAIN_OBJECT.setPub(new java.util.Date());
}
@Test
public void mapRow() throws SQLException {
DomainObjectRowMapper mapper = new DomainObjectRowMapper();
assertEquals("T_Domain", mapper.getTable());
ResultSetImpl rs = new ResultSetImpl(DOMAIN_OBJECT);
DomainObject domain = (DomainObject)mapper.mapRow(rs, 1);
assertEquals(DOMAIN_OBJECT.getUsername(), domain.getUsername());
assertEquals(DOMAIN_OBJECT.getPassword(), domain.getPassword());
assertTrue(domain.getGender());
assertEquals(DOMAIN_OBJECT.getBonus(), domain.getBonus(), 0.00001f);
assertEquals(DOMAIN_OBJECT.getId(), domain.getId());
}
}
@Table(name="T_Domain")
class DomainObject {
private Integer id;
private String username;
private String password;
private boolean gender;
private float bonus;
private java.util.Date pub;
@Id
public Integer getId() { return id; }
protected void setId(Integer id) { this.id = id; }
@Column(name="username")
public String getUsername() { return username; }
public void setUsername(String username) { this.username = username; }
@Column(name="password")
public String getPassword() { return password; }
public void setPassword(String password) { this.password = password; }
@Column(name="gender")
public boolean getGender() { return gender; }
public void setGender(boolean gender) { this.gender = gender; }
@Column(name="bonus")
public float getBonus() { return bonus; }
public void setBonus(float bonus) { this.bonus = bonus; }
@Column(name="publish")
public java.util.Date getPub() { return pub; }
public void setPub(java.util.Date pub) { this.pub = pub; }
@Transient
public String getDisplayName() { return "Guest"; }
}
class DomainObjectRowMapper extends DomainRowMapper<DomainObject> {
public DomainObjectRowMapper() {
super(DomainObject.class);
}
}
class ResultSetImpl extends ResultSetAdapter {
private DomainObject row;
public ResultSetImpl(DomainObject row) {
this.row = row;
}
@Override
public boolean getBoolean(String columnName) throws SQLException {
if(columnName.equals("gender"))
return row.getGender();
throw new SQLException("Not found");
}
@Override
public Timestamp getTimestamp(String columnName) throws SQLException {
if(columnName.equals("publish"))
return new Timestamp(row.getPub().getTime());
throw new SQLException("Not found");
}
@Override
public float getFloat(String columnName) throws SQLException {
if(columnName.equals("bonus"))
return row.getBonus();
throw new SQLException("Not found");
}
@Override
public int getInt(String columnName) throws SQLException {
if(columnName.equals("id"))
return row.getId().intValue();
throw new SQLException("Not found");
}
@Override
public String getString(String columnName) throws SQLException {
if(columnName.equals("username"))
return row.getUsername();
if(columnName.equals("password"))
return row.getPassword();
throw new SQLException("Not found");
}
}
class ResultSetAdapter implements ResultSet {
public boolean absolute(int row) throws SQLException {
throw new UnsupportedOperationException();
}
public void afterLast() throws SQLException {
throw new UnsupportedOperationException();
}
public void beforeFirst() throws SQLException {
throw new UnsupportedOperationException();
}
public void cancelRowUpdates() throws SQLException {
throw new UnsupportedOperationException();
}
public void clearWarnings() throws SQLException {
throw new UnsupportedOperationException();
}
public void close() throws SQLException {
throw new UnsupportedOperationException();
}
public void deleteRow() throws SQLException {
throw new UnsupportedOperationException();
}
public int findColumn(String columnName) throws SQLException {
throw new UnsupportedOperationException();
}
public boolean first() throws SQLException {
throw new UnsupportedOperationException();
}
public Array getArray(int i) throws SQLException {
throw new UnsupportedOperationException();
}
public Array getArray(String colName) throws SQLException {
throw new UnsupportedOperationException();
}
public InputStream getAsciiStream(int columnIndex) throws SQLException {
throw new UnsupportedOperationException();
}
public InputStream getAsciiStream(String columnName) throws SQLException {
throw new UnsupportedOperationException();
}
public BigDecimal getBigDecimal(int columnIndex, int scale) throws SQLException {
throw new UnsupportedOperationException();
}
public BigDecimal getBigDecimal(int columnIndex) throws SQLException {
throw new UnsupportedOperationException();
}
public BigDecimal getBigDecimal(String columnName, int scale) throws SQLException {
throw new UnsupportedOperationException();
}
public BigDecimal getBigDecimal(String columnName) throws SQLException {
throw new UnsupportedOperationException();
}
public InputStream getBinaryStream(int columnIndex) throws SQLException {
throw new UnsupportedOperationException();
}
public InputStream getBinaryStream(String columnName) throws SQLException {
throw new UnsupportedOperationException();
}
public Blob getBlob(int i) throws SQLException {
throw new UnsupportedOperationException();
}
public Blob getBlob(String colName) throws SQLException {
throw new UnsupportedOperationException();
}
public boolean getBoolean(int columnIndex) throws SQLException {
throw new UnsupportedOperationException();
}
public boolean getBoolean(String columnName) throws SQLException {
throw new UnsupportedOperationException();
}
public byte getByte(int columnIndex) throws SQLException {
throw new UnsupportedOperationException();
}
public byte getByte(String columnName) throws SQLException {
throw new UnsupportedOperationException();
}
public byte[] getBytes(int columnIndex) throws SQLException {
throw new UnsupportedOperationException();
}
public byte[] getBytes(String columnName) throws SQLException {
throw new UnsupportedOperationException();
}
public Reader getCharacterStream(int columnIndex) throws SQLException {
throw new UnsupportedOperationException();
}
public Reader getCharacterStream(String columnName) throws SQLException {
throw new UnsupportedOperationException();
}
public Clob getClob(int i) throws SQLException {
throw new UnsupportedOperationException();
}
public Cl
没有合适的资源?快使用搜索试试~ 我知道了~
Live在线书店
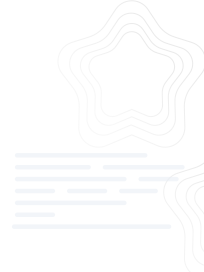
共547个文件
html:192个
java:133个
jpg:88个

需积分: 10 211 下载量 10 浏览量
2008-08-05
11:48:24
上传
评论 1
收藏 9.64MB RAR 举报
温馨提示
《Spring 2.0核心技术与最佳实践》一书由浅入深,详细介绍了Spring 2.0框架的几乎全部内容,并重点突出2.0版本的新特性,是一本注重实践而又深入理论的书,力图为读者展示如何应用Spring 2.0框架创建灵活高效的J2EE应用程序,并提供了一个真正可直接部署的完整的Web应用——Live在线书店(见下图),读者可以从中学习设计并实现一个基于Spring 2.0的完整的多层轻量级J2EE应用程序的方法,积累大量有用的经验和技巧。
资源推荐
资源详情
资源评论
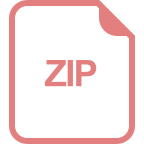
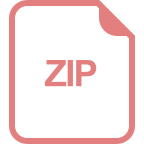
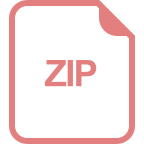
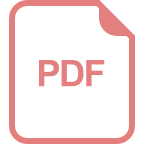
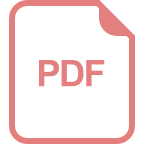
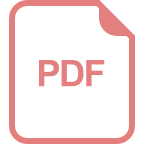
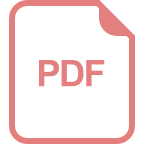
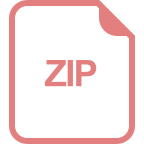
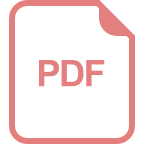
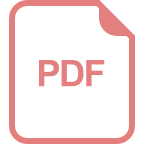
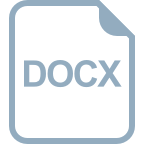
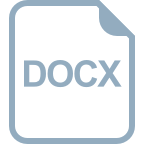
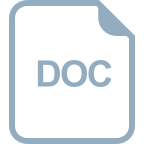
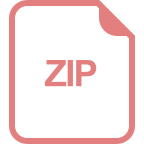
收起资源包目录

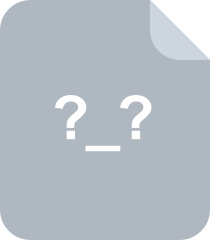
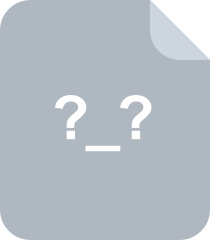
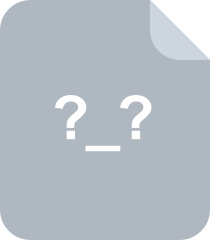
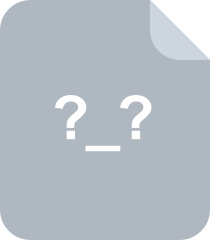
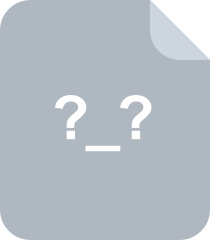
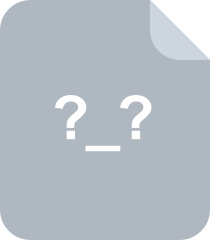
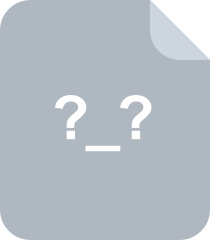
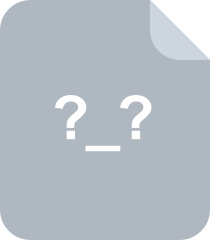
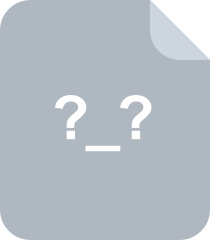
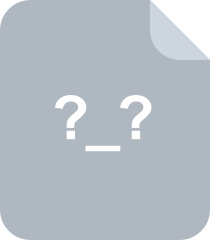
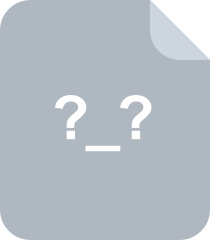
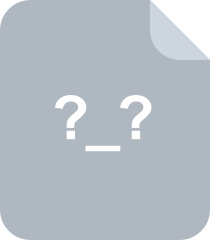
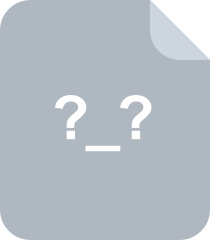
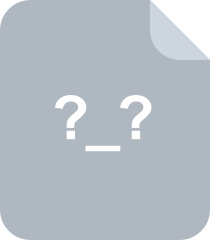
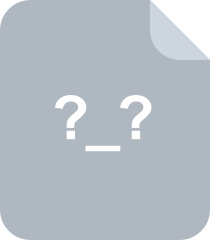
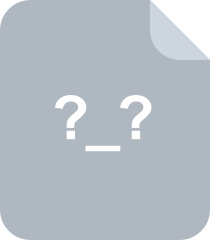
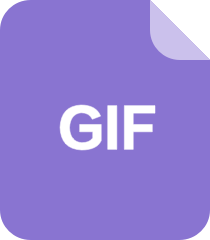
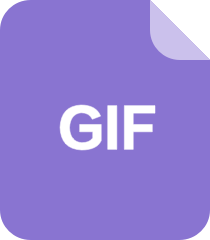
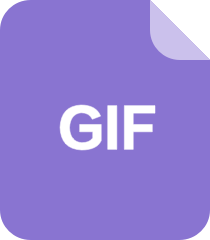
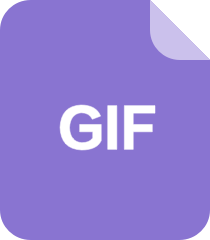
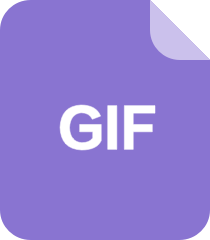
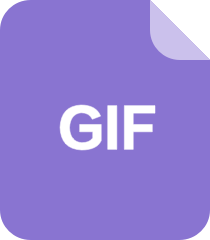
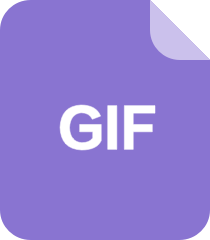
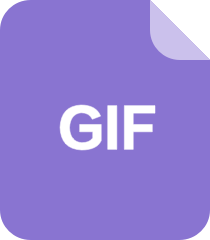
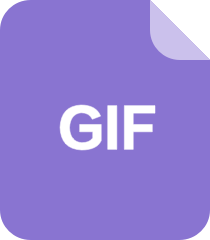
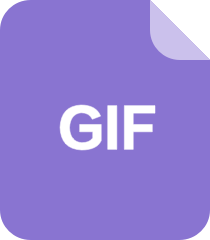
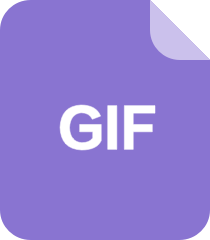
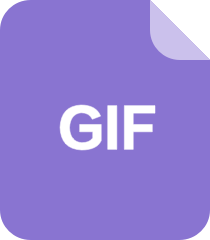
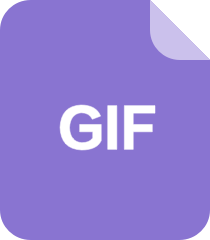
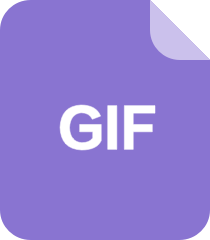
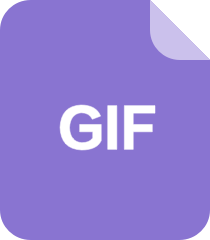
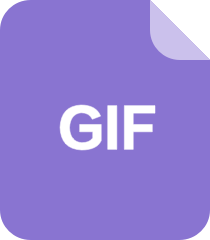
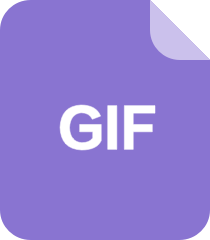
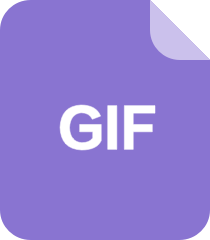
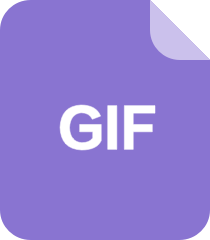
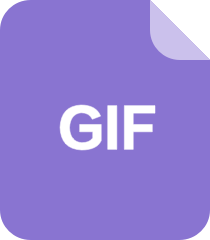
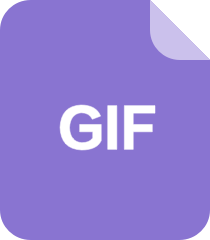
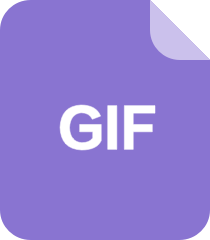
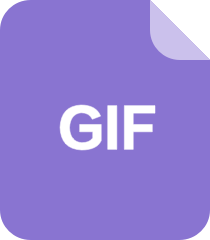
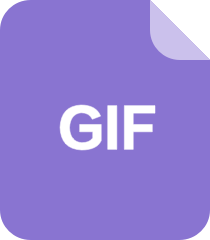
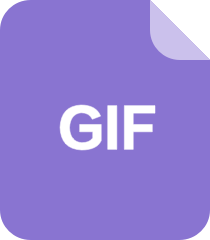
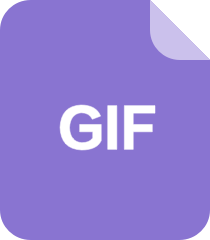
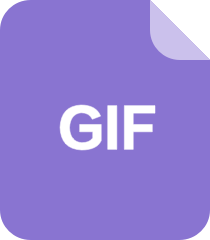
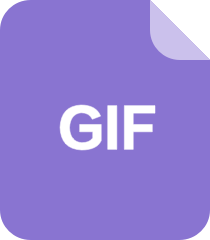
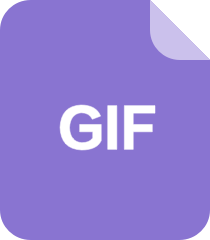
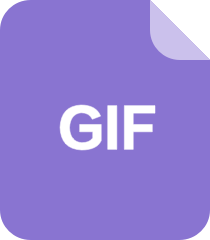
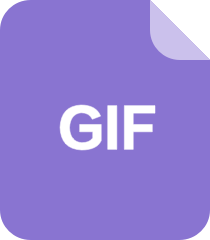
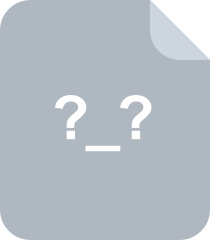
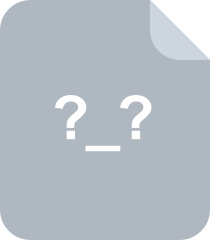
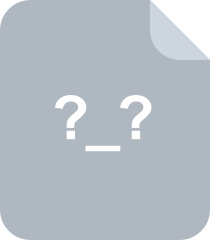
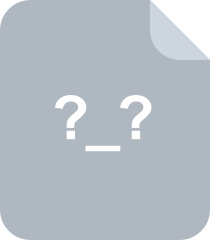
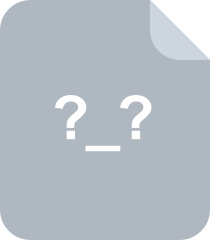
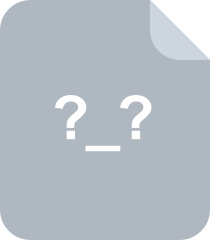
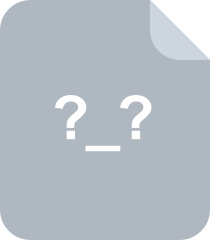
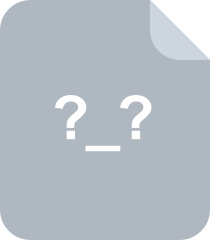
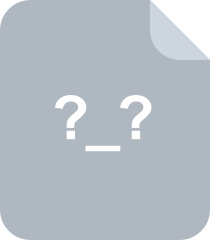
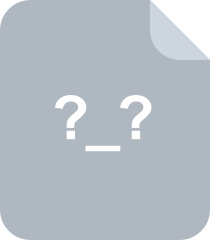
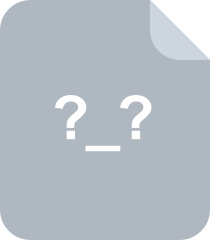
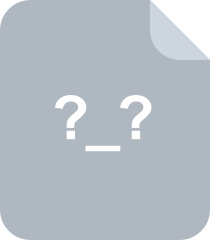
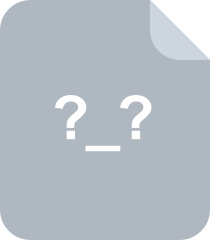
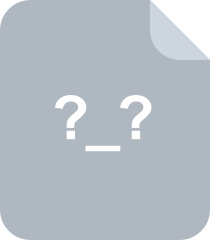
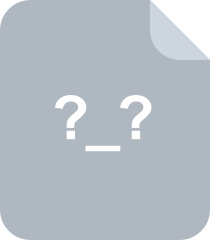
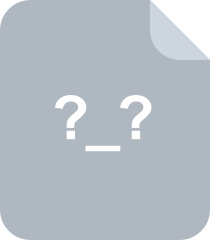
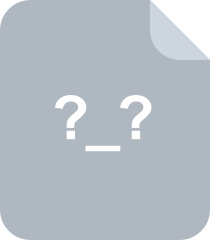
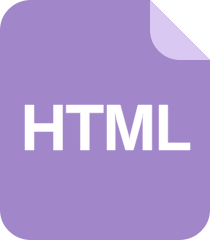
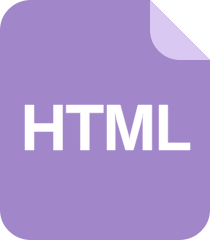
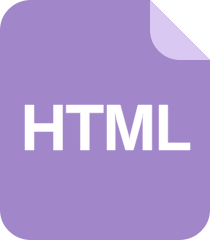
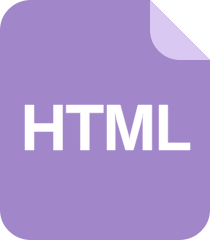
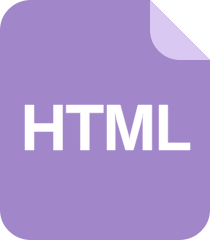
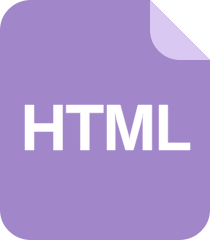
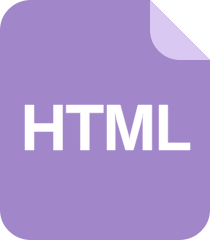
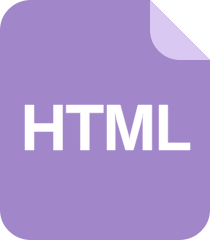
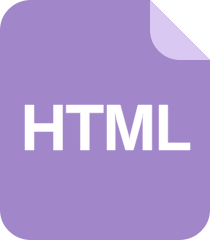
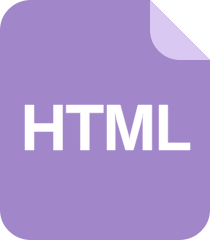
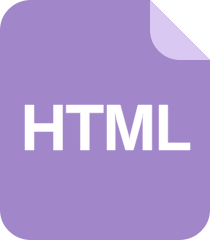
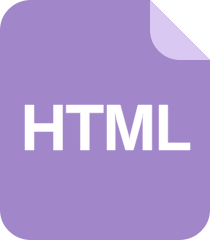
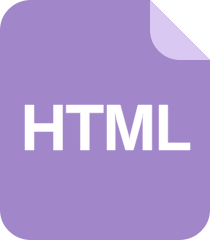
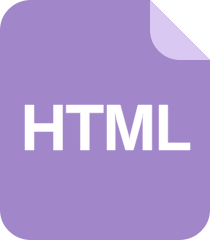
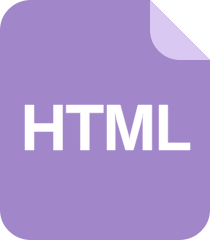
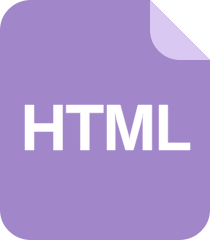
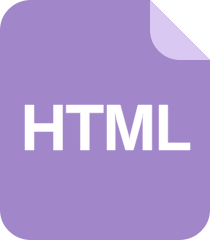
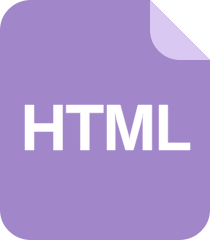
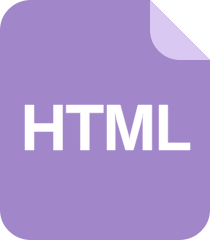
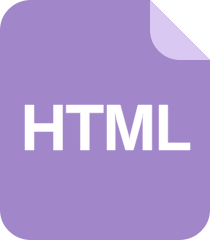
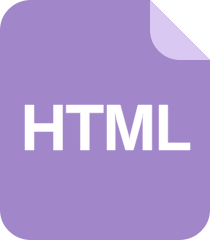
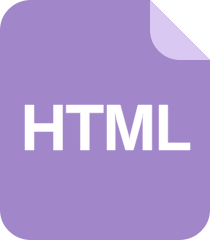
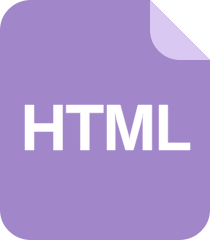
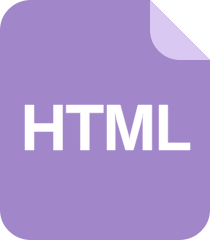
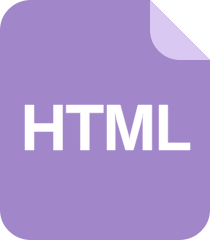
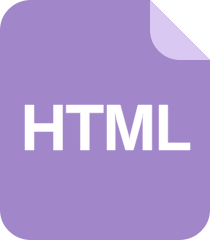
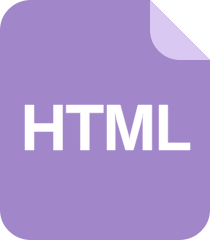
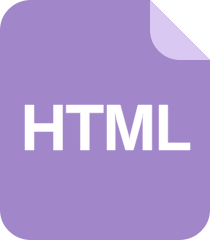
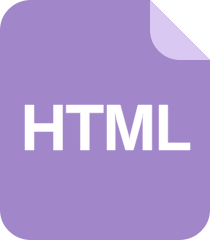
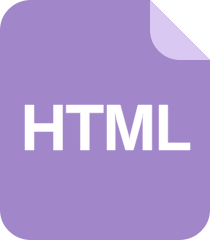
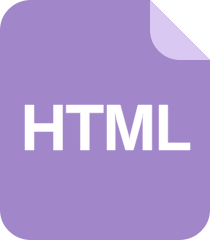
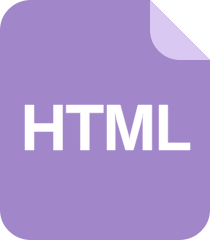
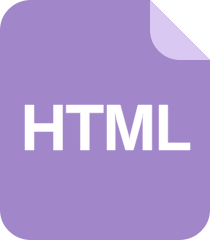
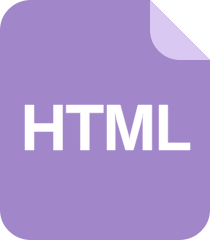
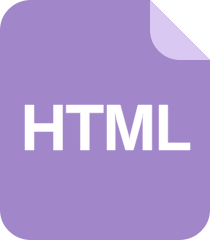
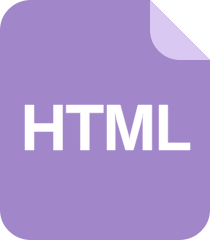
共 547 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
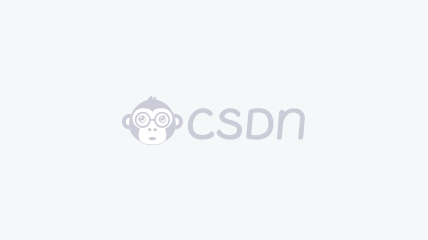

呵呵俊俊
- 粉丝: 0
- 资源: 8
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

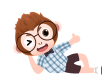
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


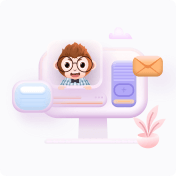
安全验证
文档复制为VIP权益,开通VIP直接复制
