单例模式和工厂模式代码
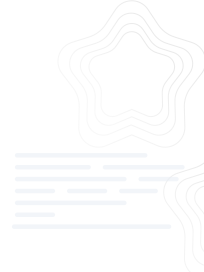


单例模式和工厂模式是两种常见的软件设计模式,在面向对象编程中扮演着重要的角色。它们都是为了解决特定的问题而提出的解决方案,但有着不同的应用场景和设计思路。 **单例模式** 是一种限制类实例化次数的模式,确保一个类在整个程序运行过程中只有一个实例存在。这种模式通常用于管理共享资源或者需要全局访问的对象,例如数据库连接、线程池或缓存。在Java中,实现单例模式有多种方法,包括懒汉式(线程不安全)、饿汉式(静态常量)、双检锁(DCL)和枚举单例。其中,双检锁和枚举单例是线程安全的,推荐在多线程环境下使用。 ```java // 双检锁/双重校验锁(DCL,即 double-checked locking) public class Singleton { private volatile static Singleton instance; private Singleton() {} public static Singleton getInstance() { if (instance == null) { synchronized (Singleton.class) { if (instance == null) { instance = new Singleton(); } } } return instance; } } ``` **工厂模式** 是一种对象创建型设计模式,它提供了一种创建对象的最佳方式。在工厂模式中,当创建对象时不会直接实例化,而是通过调用一个公共的工厂方法来完成。这样做的好处是将对象的创建过程与使用对象的代码解耦,使得代码更加灵活,易于扩展。工厂模式分为简单工厂模式、工厂方法模式和抽象工厂模式。在Java中,工厂方法模式可以通过定义接口或抽象类,让子类决定实例化哪个类。 ```java // 工厂方法模式示例 interface Shape { void draw(); } class Circle implements Shape { @Override public void draw() { System.out.println("Drawing a circle..."); } } class Square implements Shape { @Override public void draw() { System.out.println("Drawing a square..."); } // 创建工厂类 class ShapeFactory { public static Shape getShape(String type) { if ("Circle".equalsIgnoreCase(type)) { return new Circle(); } else if ("Square".equalsIgnoreCase(type)) { return new Square(); } return null; } } } public class FactoryPatternDemo { public static void main(String[] args) { Shape circle = ShapeFactory.getShape("Circle"); circle.draw(); Shape square = ShapeFactory.getShape("Square"); square.draw(); } } ``` 单例模式关注的是控制类的实例化过程,确保其唯一性;而工厂模式则关注于对象的创建,通过提供一个统一的接口来生产不同类型的对象,降低了系统的耦合度。在实际开发中,这两种模式常常结合使用,例如,单例模式中的单例对象可以通过工厂模式来创建,以提高代码的可读性和可维护性。通过深入理解和熟练运用这些设计模式,开发者可以编写出更高效、更易于维护的代码。
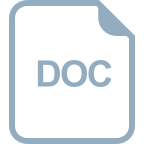
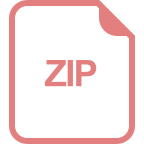
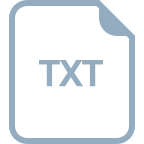
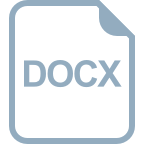
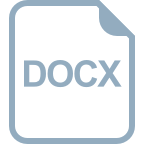
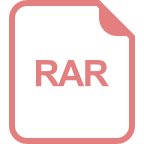
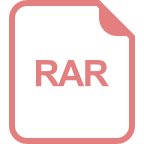
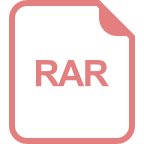
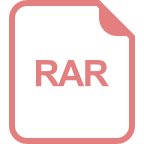
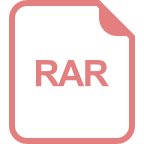
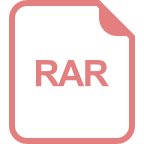






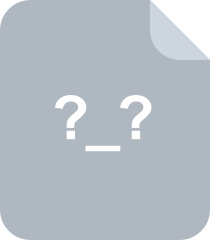
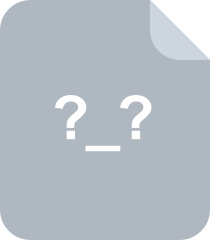
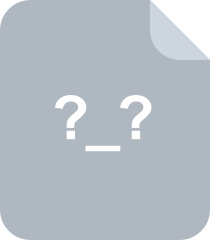




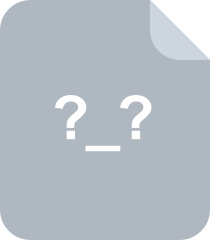
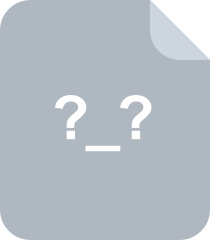
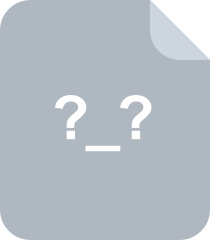
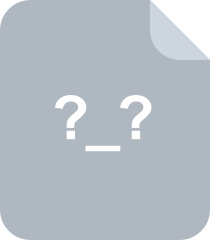
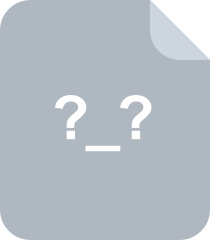
- 1
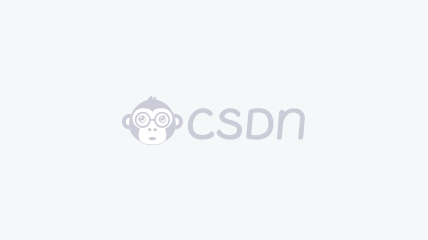
- leapple198709102012-12-26很实用,推荐下载


- 粉丝: 96
- 资源: 38
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

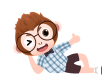
