// VC6DemoDlg.cpp : implementation file
//
#include "stdafx.h"
#include "VC6Demo.h"
#include "VC6DemoDlg.h"
#include "GetWordInterface.h"
#include "OnlineTranslate.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
//#define MAX_OUTPUT_LEN 4096 // the max length of the point-capturing output string
//#define MAX_RECT_OUTPUT_LEN 81920 // the max length of the rectangle-capturing output string
//#define MOUSEHOOK_CAPTURE_OK_MSG _T("MOUSEHOOK_CAPTUREOK_MSG-")##LICENSEID
//#define HIGHLIGHT_CAPTURE_OK_MSG _T("HIGHLIGHT_CAPTUREOK_MSG_XUFU_V1-")##LICENSEID
//#define HOTKEY_CAPTURE 1000 // the hotkey message
#define ID_HOTKEY 1002 // Enable highlighted capture
//UINT MOUSEHOOK_CAPTURE_OK = 0;
//UINT HIGHLIGHT_CAPTURE_OK = 0;
//#define TYPE_PROCESS 0x00000000
//#define TYPE_WND 0x10000000
//#define TYPE_MOUSE 0x20000000
//#define TYPE_KEYBOARD 0x30000000
//#define TYPE_ACTIVATE 0x40000000
#define UM_TRANSLATE (WM_USER+0x10)
#define WM_POST_INIT (WM_USER+0x11)
#define WM_MOUSEMOVE_EX (WM_USER+0x12)
#define BKG_COLOR RGB(249, 249, 249)
/////////////////////////////////////////////////////////////////////////////
// CAboutDlg dialog used for App About
CRITICAL_SECTION spinlock = {0};
CVC6DemoDlg* maindlg = 0;
/////////////////////////////////////////////////////////////////////////////
// CVC6DemoDlg dialog
CVC6DemoDlg::CVC6DemoDlg(CWnd* pParent /*=NULL*/)
: CDialog(CVC6DemoDlg::IDD, pParent)
{
//{{AFX_DATA_INIT(CVC6DemoDlg)
//m_bEnableMouseCap = FALSE;
m_bEnableHighlight = FALSE;
//}}AFX_DATA_INIT
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
m_uCheckTimerID = 1;
textEmpty = 1;
hasTitle = 1;
minimized = 1;
// hook相关 用于判定鼠标位置
maindlg = this;
InitializeCriticalSection(&spinlock);
m_brush.CreateSolidBrush( BKG_COLOR ); // 背景色
m_brushBorder.CreateSolidBrush( RGB(100,200,200) );
font = new CFont;
font->CreateFont(16,8,0,0,0,0,0,0,DEFAULT_CHARSET,0,0,0,0,0);
}
void CVC6DemoDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CVC6DemoDlg)
DDX_Check(pDX, IDC_CHECK_HIGHLIGHT, m_bEnableHighlight);
DDX_Control(pDX, IDC_EDIT_HIGHLIGHT, m_editHighlight);
DDX_Control(pDX, IDC_EDIT_RECT, m_editRect);
//}}AFX_DATA_MAP
DDX_Control(pDX, IDC_CHECK_CLEAR, m_chkClear);
DDX_Control(pDX, IDC_COMBO1, dstCode);
}
BEGIN_MESSAGE_MAP(CVC6DemoDlg, CDialog)
//{{AFX_MSG_MAP(CVC6DemoDlg)
ON_WM_CREATE()
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
ON_WM_DESTROY()
ON_WM_CLOSE()
ON_WM_SIZE()
ON_WM_CTLCOLOR()
ON_WM_HOTKEY()
ON_BN_CLICKED(IDC_CHECK_HIGHLIGHT, OnCheckHighlight)
//}}AFX_MSG_MAP
//user defined
ON_MESSAGE(UM_TRANSLATE, OnTranslate)
ON_MESSAGE(WM_MOUSEMOVE_EX, OnMouseMoveEx)
ON_EN_CHANGE(IDC_EDIT_HIGHLIGHT, &CVC6DemoDlg::OnEnChangeEditHighlight)
ON_BN_CLICKED(IDC_CHECK_CLEAR, &CVC6DemoDlg::OnBnClickedCheckClear)
ON_CBN_SELCHANGE(IDC_COMBO1, &CVC6DemoDlg::OnCbnSelchangeLangCode)
ON_CBN_EDITCHANGE(IDC_COMBO1, &CVC6DemoDlg::OnCbnEditchangeLangCode)
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CVC6DemoDlg message handlers
const wchar_t* langCode[] = // 目标语言代码
{L"auto",L"zh",L"jp",L"spa",L"th",L"ru",L"yue",L"zh",L"de",L"nl",L"en",L"kor",L"fra",L"ara",L"pt",L"wyw",L"it",L"el",};
HRESULT WINAPI NotifyCallBackC (long wParam, long lParam)
{
//wParam lParam 永远是1,0 没用
maindlg->CaptureTextStub(wParam, lParam);
return 0;
}
HRESULT WINAPI NotifyCallBackH (long wParam, long lParam)
{
// not capture self
if (maindlg->IsSelfSubWin((HWND)lParam) ) {return 1;}
maindlg->CaptureHighlightTextStub(wParam, lParam);
return 0;
}
BOOL CVC6DemoDlg::OnInitDialog()
{
CDialog::OnInitDialog();
SetWindowText(VC6DEMO_TITLE);
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
dstCode.SetCurSel(0);
GetClientRect(&m_clRect);
// set the window to the topmost
//CRect rect;
//GetWindowRect(&rect);
//height = rect.Height();
//width = rect.Width();
m_editHighlight.SetFont(font);
m_editRect.SetFont(font);
//nRight = GetSystemMetrics(SM_CXFULLSCREEN ); //SM_CXSCREEN
//nBottom = GetSystemMetrics(SM_CYFULLSCREEN );
//nCapHeight = GetSystemMetrics(SM_CYCAPTION);
hCheckHighlight = GetDlgItem(IDC_CHECK_HIGHLIGHT)->GetSafeHwnd();
hCheckClear = GetDlgItem(IDC_CHECK_CLEAR)->GetSafeHwnd();
SetWindowPos( &wndTopMost,
nRight-width,
nBottom-height+nCapHeight,
0,0, SWP_NOSIZE|SWP_SHOWWINDOW);
SetCaptureReadyCallback( NotifyCallBackC );
SetHighlightReadyCallback( NotifyCallBackH );
::RegisterHotKey(GetSafeHwnd(),ID_HOTKEY, MOD_CONTROL, VK_F9);
m_editHighlight.SetFocus();
return 0; // return TRUE unless you set the focus to a control
}
void CVC6DemoDlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND, (WPARAM) dc.GetSafeHdc(), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
HCURSOR CVC6DemoDlg::OnQueryDragIcon()
{
return (HCURSOR) m_hIcon;
}
void CVC6DemoDlg::OnDestroy()
{
// remove the global mouse hook
RemoveHighlightReadyCallback( NotifyCallBackH );
RemoveCaptureReadyCallback( NotifyCallBackC );
CDialog::OnDestroy();
}
void CVC6DemoDlg::OnCheckHighlight()
{
UpdateData(TRUE);
EnableHighlightCapture( m_bEnableHighlight );
EnableCursorCapture( m_bEnableHighlight );
}
void CVC6DemoDlg::CaptureTextStub(WPARAM wParam, LPARAM lParam)
{
// get the window handle at the cursor point
POINT ptCursor;
::GetCursorPos(&ptCursor);
CRect rect;
GetWindowRect(&rect);
// Self
if ( rect.PtInRect(ptCursor) ) {return ;}
// nCursorPos is the zero-based index in the string bstr
// if the cursor is not in the string bstr, nCursorPos is -1
long nCursorPos = -1;
BOOL bOK = FALSE;
BSTR wstrOut;
bOK = GetString(ptCursor.x, ptCursor.y, &wstrOut, &nCursorPos);
if (bOK) // successfully get the words
{
CString strTotal(wstrOut);
FreeString(&wstrOut);
if (!strTotal.IsEmpty())
{
if ( textEmpty)
{
m_editHighlight.SetEmpty(0);
m_editHighlight.SetWindowText(strTotal);
BeginTranslate(strTotal);
}
}
}
}
bool CVC6DemoDlg::IsSelfSubWin(HWND hwnd)
{
return
hwnd== m_hWnd
||hwnd == m_editHighlight.m_hWnd
||hwnd == m_editRect.m_hWnd
||hwnd == hCheckClear
||hwnd == hCheckHighlight;
}
void CVC6DemoDlg::CaptureHighlightTextStub(WPARAM wParam, LPARAM lParam)
{
CString strText;
POINT ptCursor;
::GetCursorPos(&ptCursor);
BOOL bOK = FALSE;
BSTR bstr = NULL;
bOK = GetHighlightText2(ptCursor.x, ptCursor.y, &bstr);
if (bOK)
{
strText = bstr;
FreeString(&bstr);
}
if (strText.IsEmpty())
{
//textEmpty = 1;
} else {
textEmpty = 0;
m_editHighlight.SetEmpty(0);
m_editHighlight.SetWindowTextW(strText);
BeginTranslate(strText);
}
}
afx_msg LRESULT CVC6DemoDlg::OnTranslate(WPARAM wParam, LPARAM lParam)
{
TranslationInfo * info = (TranslationInfo*)lParam;
if (m_curInfo == info)
{
m_editRect.SetWindowText(info->dst);
}
delete (TranslationInfo*)lParam;
return 0;
}
int CVC6DemoDlg::BeginTranslate(const CString& strText)
{
if (strText.IsEmpty()) { m_curInfo = 0; return 0;}
int index = dstCode.GetCurSel();
if (index < 0) {index=0;} // 未选中 置为auto
TranslationInfo *info = new TranslationInfo;
info->hwnd = GetSafeHwnd();

tiankong_bear
- 粉丝: 31
- 资源: 15
最新资源
- HAL库驱动TCS3200颜色识别模块-STM32F103ZET6
- boost电路参数详细计算.xls
- HTML+CSS+JavaScript实现带飘雪花效果的圣诞树
- 实习实训大作业-基于python的电商产品评论数据情感分析源码+说明(高分项目)
- HTML与CSS创建圣诞树及动态雪花效果
- 数据结构与算法:Python递归实现计算二叉树的深度
- 前端开发中的平安夜贺卡HTML代码示例
- C# WPF一个测弹力,显示曲线的工具 .zip
- 本地磁盘学习使用仅供参考
- 本地磁盘学习使用仅供参考
- 基于Kaggle数据集的泰坦尼克号幸存者预测机器学习实践
- 本地磁盘学习使用仅供参考
- 视频游戏人物检测35-YOLO(v5至v9)、COCO、CreateML、Paligemma、TFRecord数据集合集.rar
- 本地磁盘学习使用仅供参考
- 本地磁盘学习使用仅供参考
- HTML、CSS与JavaScript实现圣诞节雪花飘落效果
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


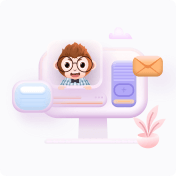