### C语言实现多项式的加减乘操作 #### 一、背景与目标 在数学和计算机科学领域,多项式是常见的代数表达式之一。对于计算机程序来说,处理多项式的加法、减法以及乘法是一种基本的需求。本篇文章将详细介绍如何使用C语言中的动态链表来实现多项式的加减乘功能。 #### 二、动态链表的基本概念 动态链表是一种数据结构,它允许我们在运行时动态地增加或删除元素。在C语言中,动态链表通常由一系列指向下一个元素的指针组成。这种数据结构非常适合用来表示多项式,因为多项式可以看作是一系列项的集合,每一项都有一个系数和一个指数。 #### 三、定义多项式节点 为了表示多项式的每个项,我们需要定义一个结构体类型,该结构体包含两个字段:一个表示系数(`coef`),另一个表示指数(`expn`)。此外,我们还需要一个指向下一个节点的指针,以便链接多个项。以下是一个示例定义: ```c typedef struct node { float coef; // 系数 int expn; // 指数 struct node* next; // 指向下一个节点 } POLY; ``` #### 四、创建多项式 创建多项式可以通过读取用户输入来完成。用户会输入多项式的各个项,包括系数和指数。我们可以定义一个函数 `creat` 来创建多项式链表。这个函数接收一个字符作为参数,用于区分不同的多项式,比如 `f(x)` 或者 `g(x)`。 ```c POLY* creat(char ch) { POLY* head, *inpt; float x; int y; head = (POLY*)malloc(sizeof(POLY)); head->next = NULL; printf("请输入多项式 %c 的各项(输入格式为系数 指数, 例如 5 3 表示 5x^3, 输入0 0结束):\n", ch); scanf("%f%d", &x, &y); while (x != 0) { inpt = (POLY*)malloc(sizeof(POLY)); inpt->coef = x; inpt->expn = y; inpt->next = NULL; insert(head, inpt); printf("继续输入多项式 %c 的下一项(0 0结束):\n", ch); scanf("%f%d", &x, &y); } return head; } ``` 这里还定义了一个 `insert` 函数,用于将新节点插入到链表中。插入时需要考虑当前节点的指数大小,以保持链表按照指数降序排列。 #### 五、实现多项式加法 多项式的加法可以通过遍历两个多项式的链表并合并它们来实现。我们需要比较两个多项式的指数,如果相同,则合并系数;如果不同,则将较大的指数项添加到结果链表中。 ```c POLY* addPoly(POLY* p1, POLY* p2) { POLY* result, *temp1, *temp2, *newNode; temp1 = p1; temp2 = p2; result = NULL; while (temp1 != NULL && temp2 != NULL) { if (temp1->expn == temp2->expn) { if (temp1->coef + temp2->coef != 0) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp1->coef + temp2->coef; newNode->expn = temp1->expn; newNode->next = result; result = newNode; } temp1 = temp1->next; temp2 = temp2->next; } else if (temp1->expn > temp2->expn) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp1->coef; newNode->expn = temp1->expn; newNode->next = result; result = newNode; temp1 = temp1->next; } else { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp2->coef; newNode->expn = temp2->expn; newNode->next = result; result = newNode; temp2 = temp2->next; } } while (temp1 != NULL) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp1->coef; newNode->expn = temp1->expn; newNode->next = result; result = newNode; temp1 = temp1->next; } while (temp2 != NULL) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp2->coef; newNode->expn = temp2->expn; newNode->next = result; result = newNode; temp2 = temp2->next; } return result; } ``` #### 六、实现多项式减法 多项式的减法类似于加法,只是在合并相同指数的项时需要将系数相减。 ```c POLY* minusPoly(POLY* p1, POLY* p2) { POLY* result, *temp1, *temp2, *newNode; temp1 = p1; temp2 = p2; result = NULL; while (temp1 != NULL && temp2 != NULL) { if (temp1->expn == temp2->expn) { if (temp1->coef - temp2->coef != 0) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp1->coef - temp2->coef; newNode->expn = temp1->expn; newNode->next = result; result = newNode; } temp1 = temp1->next; temp2 = temp2->next; } else if (temp1->expn > temp2->expn) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp1->coef; newNode->expn = temp1->expn; newNode->next = result; result = newNode; temp1 = temp1->next; } else { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = -temp2->coef; newNode->expn = temp2->expn; newNode->next = result; result = newNode; temp2 = temp2->next; } } while (temp1 != NULL) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp1->coef; newNode->expn = temp1->expn; newNode->next = result; result = newNode; temp1 = temp1->next; } while (temp2 != NULL) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = -temp2->coef; newNode->expn = temp2->expn; newNode->next = result; result = newNode; temp2 = temp2->next; } return result; } ``` #### 七、实现多项式乘法 多项式的乘法则较为复杂,需要对每一个项进行相乘,然后将结果合并。这涉及到遍历两个链表,并且对每一个节点进行相乘操作。 ```c POLY* multiplyPoly(POLY* p1, POLY* p2) { POLY* result, *temp1, *temp2, *newNode; temp1 = p1; result = NULL; while (temp1 != NULL) { temp2 = p2; while (temp2 != NULL) { newNode = (POLY*)malloc(sizeof(POLY)); newNode->coef = temp1->coef * temp2->coef; newNode->expn = temp1->expn + temp2->expn; insert(result, newNode); temp2 = temp2->next; } temp1 = temp1->next; } return result; } ``` #### 八、总结 本文详细介绍了如何使用C语言中的动态链表来实现多项式的加法、减法和乘法。通过定义一个多项式的节点结构,我们可以方便地创建、插入和操作这些节点。这些方法不仅能够处理基本的多项式运算,而且还可以进一步扩展来支持更复杂的多项式操作。 以上就是使用C语言实现多项式的加减乘功能的详细过程。希望这篇文章能对你有所帮助!
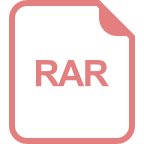
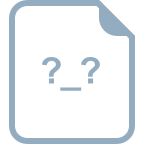
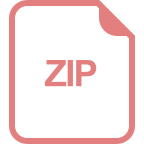
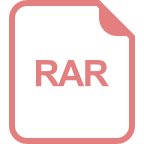
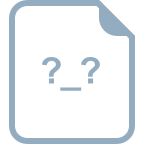
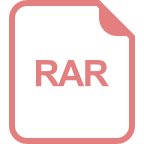
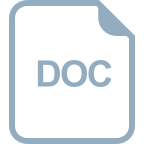
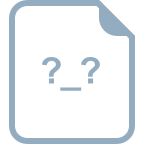
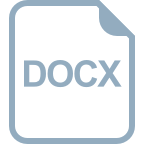
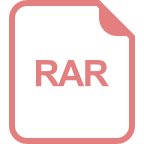
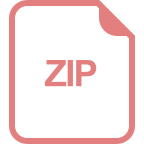
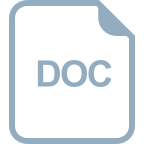
#include<malloc.h>
typedef struct node{//定义节点类型
float coef;
int expn;
struct node * next;
}PLOY;
void start()//用户选择界面
{
printf("************************************\n");
printf(" 两个一元多项式的相加/相减,相乘:\n");
printf("************************************\n");
printf("请选择操作:\n");
printf("0.退出\n");
printf("1.两个一元多项式相加\n");
printf("2.两个一元多项式相乘\n");
printf("3.两个一元多项式相减\n");
}
void insert(PLOY *head,PLOY *inpt)//查找位置插入新链节程序
{
PLOY *pre,*now;
int signal=0;
pre=head;//pre定义为现在的前一个链节
if(pre->next==NULL) {pre->next=inpt;}
else {now=pre->next;
while(signal==0)
{
if(inpt->expn<now->expn)//当新链节小于现在的连接时向后移一个链节
if(now->next==NULL)
{
now->next=inpt;
signal=1;
}
else
{
pre=now;
now=pre->next;
}
}
else
if(inpt->expn>now->expn)//如果发现比现在的链节大了就插入到这个连接的前面
{
inpt->next=now;
pre->next=inpt;
signal=1;
}
else
{
now->coef=now->coef+inpt->coef;
signal=1;
free(inpt);//与当前链节相等指数
if(now->coef==0)
{
pre->next=now->next;
free(now);
}
}
剩余8页未读,继续阅读
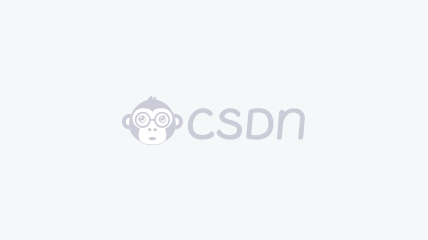

- 粉丝: 173
- 资源: 13
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

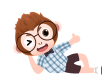
最新资源

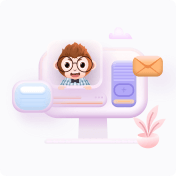
