在C#编程中,有时我们需要实现一个功能,即让程序能够调用用户的默认浏览器来打开指定的网页。本文将详细探讨几种实现这一功能的方法,并提供相关的代码示例。以下是一些常用的技术途径: 1. 使用`Process.Start()`方法: 这是最简单且常见的方法,通过`System.Diagnostics`命名空间中的`Process`类启动一个新的进程,指定URL作为启动参数。例如: ```csharp using System.Diagnostics; // 调用默认浏览器打开网页 Process.Start("http://www.example.com"); ``` 此方法会自动使用用户系统设置的默认浏览器来打开链接。 2. 使用`ShellExecute()`函数(P/Invoke): 如果你希望有更多的控制,可以使用Windows API中的`ShellExecute()`函数。需要引入`DllImport`特性以导入该函数: ```csharp using System.Runtime.InteropServices; [DllImport("shell32.dll", CharSet = CharSet.Auto)] static extern bool ShellExecuteEx(ref SHELLEXECUTEINFO lpExecInfo); [StructLayout(LayoutKind.Sequential)] public struct SHELLEXECUTEINFO { public int cbSize; public uint fMask; public IntPtr hwnd; [MarshalAs(UnmanagedType.LPTStr)] public string lpVerb; [MarshalAs(UnmanagedType.LPTStr)] public string lpFile; [MarshalAs(UnmanagedType.LPTStr)] public string lpParameters; [MarshalAs(UnmanagedType.LPTStr)] public string lpDirectory; public int nShow; public IntPtr hInstApp; public IntPtr lpIDList; [MarshalAs(UnmanagedType.LPTStr)] public string lpClass; public IntPtr hIcon; public IntPtr hMonitor; } // 调用默认浏览器打开网页 public static void OpenUrlInDefaultBrowser(string url) { SHELLEXECUTEINFO info = new SHELLEXECUTEINFO(); info.cbSize = Marshal.SizeOf(info); info.lpVerb = "open"; info.lpFile = url; info.nShow = 1; // SW_SHOW (显示窗口) ShellExecuteEx(ref info); } ``` 在这个例子中,`lpVerb`参数设置为"open",这会告诉Windows打开指定的URL。 3. 使用`System.Windows.Forms.WebBrowser`控件: 虽然这不是直接调用默认浏览器,但如果你的应用程序需要在内部打开网页,可以使用`WebBrowser`控件。这将创建一个内嵌的浏览器窗口,显示指定的网页: ```csharp using System.Windows.Forms; // 创建WebBrowser控件 WebBrowser webBrowser = new WebBrowser(); // 设置URL webBrowser.Navigate("http://www.example.com"); // 显示控件(例如添加到Form) this.Controls.Add(webBrowser); ``` 请注意,这种方法需要用户界面支持,并且性能可能不如前两种方法。 4. 使用`msedge.exe`或`iexplore.exe`: 对于特定的浏览器,如Internet Explorer或Microsoft Edge,可以直接启动它们的可执行文件并传入URL作为参数。但是,这种方法受限于用户的默认浏览器,可能不总是有效: ```csharp // 对于Edge Process.Start("msedge.exe", "http://www.example.com"); // 对于旧版IE Process.Start("iexplore.exe", "http://www.example.com"); ``` 以上就是C#中调用默认浏览器打开网页的几种常见方法。实际应用中,可以根据需求和兼容性考虑选择合适的方式。提供的源代码文件"CSharp调用默认浏览器打开网页.sln"和"CSharp调用默认浏览器打开网页.suo"是Visual Studio解决方案和用户选项文件,可以用来查看和运行示例代码。
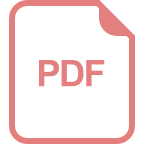
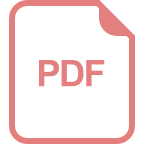
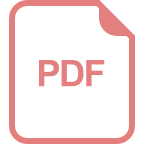
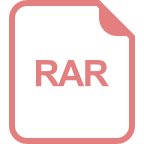
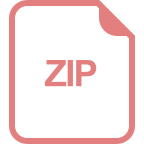
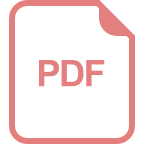
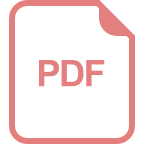
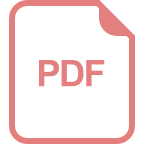
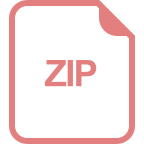
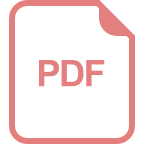
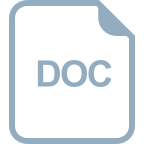

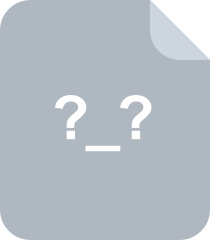

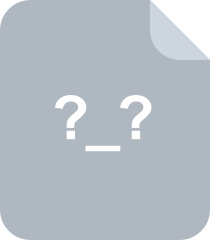
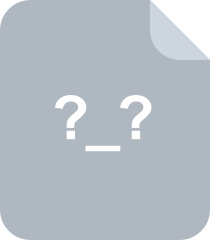
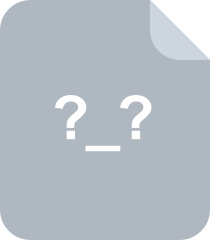

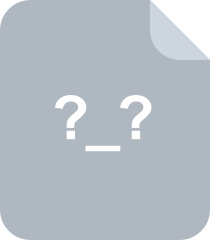
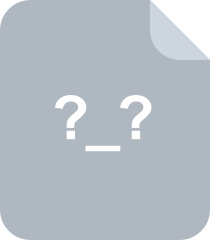
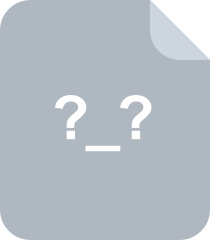
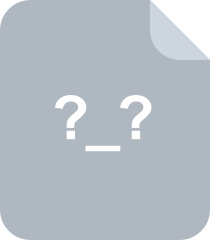
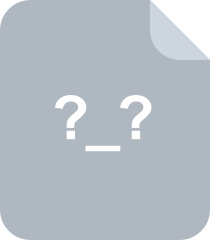
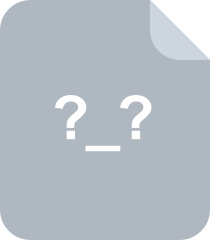




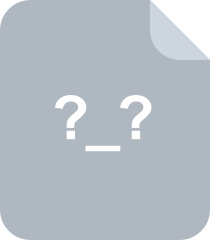
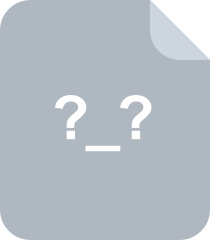
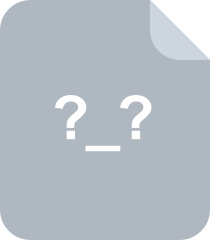


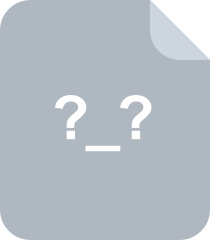
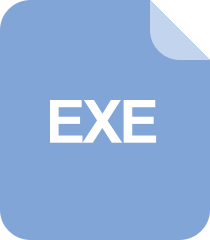
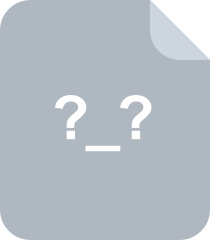
- 1

- 粉丝: 1w+
- 资源: 94
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

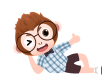
最新资源
- 此存储库收集了所有有趣的 Python 单行代码 欢迎随意提交你的代码!.zip
- 高考志愿智能推荐-JAVA-基于springBoot高考志愿智能推荐系统设计与实现
- 标准 Python 记录器的 Json 格式化程序.zip
- kernel-5.15-rc7.zip
- 来自我在 Udemy 上的完整 Python 课程的代码库 .zip
- 来自微软的免费 Edx 课程.zip
- c++小游戏猜数字(基础)
- 金铲铲S13双城之战自动拿牌助手
- x64dbg-development-2022-09-07-14-52.zip
- 多彩吉安红色旅游网站-JAVA-基于springBoot多彩吉安红色旅游网站的设计与实现

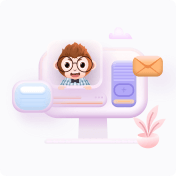

- 1
- 2
- 3
- 4
前往页