/*
*********************************************************************************************************
* PC SUPPORT FUNCTIONS
*
* (c) Copyright 1992-2002, Jean J. Labrosse, Weston, FL
* All Rights Reserved
*
* File : PC.C
* By : Jean J. Labrosse
*********************************************************************************************************
*/
//change by cmj
#include "config.h"
//#include "includes.h"
/*
*********************************************************************************************************
* CONSTANTS
*********************************************************************************************************
*/
#define DISP_BASE 0xB800 /* Base segment of display (0xB800=VGA, 0xB000=Mono) */
#define DISP_MAX_X 80 /* Maximum number of columns */
#define DISP_MAX_Y 25 /* Maximum number of rows */
#define TICK_T0_8254_CWR 0x43 /* 8254 PIT Control Word Register address. */
#define TICK_T0_8254_CTR0 0x40 /* 8254 PIT Timer 0 Register address. */
#define TICK_T0_8254_CTR1 0x41 /* 8254 PIT Timer 1 Register address. */
#define TICK_T0_8254_CTR2 0x42 /* 8254 PIT Timer 2 Register address. */
#define TICK_T0_8254_CTR0_MODE3 0x36 /* 8254 PIT Binary Mode 3 for Counter 0 control word. */
#define TICK_T0_8254_CTR2_MODE0 0xB0 /* 8254 PIT Binary Mode 0 for Counter 2 control word. */
#define TICK_T0_8254_CTR2_LATCH 0x80 /* 8254 PIT Latch command control word */
#define VECT_TICK 0x08 /* Vector number for 82C54 timer tick */
#define VECT_DOS_CHAIN 0x81 /* Vector number used to chain DOS */
/*
*********************************************************************************************************
* LOCAL GLOBAL VARIABLES
*********************************************************************************************************
*/
static INT16U PC_ElapsedOverhead;
//static jmp_buf PC_JumpBuf; //del by cmj
//static BOOLEAN PC_ExitFlag; //del by cmj
//void (*PC_TickISR)(void); //del by cmj
/*$PAGE*/
/*
*********************************************************************************************************
* DISPLAY A SINGLE CHARACTER AT 'X' & 'Y' COORDINATE
*
* Description : This function writes a single character anywhere on the PC's screen. This function
* writes directly to video RAM instead of using the BIOS for speed reasons. It assumed
* that the video adapter is VGA compatible. Video RAM starts at absolute address
* 0x000B8000. Each character on the screen is composed of two bytes: the ASCII character
* to appear on the screen followed by a video attribute. An attribute of 0x07 displays
* the character in WHITE with a black background.
*
* Arguments : x corresponds to the desired column on the screen. Valid columns numbers are from
* 0 to 79. Column 0 corresponds to the leftmost column.
* y corresponds to the desired row on the screen. Valid row numbers are from 0 to 24.
* Line 0 corresponds to the topmost row.
* c Is the ASCII character to display. You can also specify a character with a
* numeric value higher than 128. In this case, special character based graphics
* will be displayed.
* color specifies the foreground/background color to use (see PC.H for available choices)
* and whether the character will blink or not.
*
* Returns : None
*********************************************************************************************************
*/
void Uart_SendChar(INT8U data)
{
while((U0LSR & 0x00000020) == 0);
U0THR = data;
}
//change by cmj
void PC_DispChar (INT8U x, INT8U y, INT8U c, INT8U color)
{
//OS_ENTER_CRITICAL();
OSSchedLock();
Uart_SendChar(0xff);
Uart_SendChar(x);
Uart_SendChar(y);
Uart_SendChar(c);
Uart_SendChar(color);
//OS_EXIT_CRITICAL();
OSSchedUnlock();
}
//void PC_DispChar (INT8U x, INT8U y, INT8U c, INT8U color)
//{
// INT8U far *pscr;
// INT16U offset;
// offset = (INT16U)y * DISP_MAX_X * 2 + (INT16U)x * 2; /* Calculate position on the screen */
// pscr = (INT8U far *)MK_FP(DISP_BASE, offset);
// *pscr++ = c; /* Put character in video RAM */
// *pscr = color; /* Put video attribute in video RAM */
//}
/*$PAGE*/
/*
*********************************************************************************************************
* CLEAR A COLUMN
*
* Description : This function clears one of the 80 columns on the PC's screen by directly accessing video
* RAM instead of using the BIOS. It assumed that the video adapter is VGA compatible.
* Video RAM starts at absolute address 0x000B8000. Each character on the screen is
* composed of two bytes: the ASCII character to appear on the screen followed by a video
* attribute. An attribute of 0x07 displays the character in WHITE with a black background.
*
* Arguments : x corresponds to the desired column to clear. Valid column numbers are from
* 0 to 79. Column 0 corresponds to the leftmost column.
*
* color specifies the foreground/background color combination to use
* (see PC.H for available choices)
*
* Returns : None
*********************************************************************************************************
*/
//change by cmj
void PC_DispClrCol (INT8U x, INT8U color)
{
INT8U i;
for (i = 0; i < DISP_MAX_Y; i++)
{
PC_DispChar(x, i, ' ', color);
}
}
//void PC_DispClrCol (INT8U x, INT8U color)
//{
// INT8U far *pscr;
// INT8U i;
// pscr = (INT8U far *)MK_FP(DISP_BASE, (INT16U)x * 2);
// for (i = 0; i < DISP_MAX_Y; i++) {
// *pscr++ = ' '; /* Put ' ' character in video RAM */
// *pscr-- = color; /* Put video attribute in video RAM */
// pscr = pscr + DISP_MAX_X * 2; /* Position on next row */
// }
//}
/*$PAGE*/
/*
*********************************************************************************************************
* CLEAR A ROW
*
* Description : This function clears one of the 25 lines on the PC's screen by directly accessing video
* RAM instead of using the BIOS. It assumed that the video adapter is VGA compatible.
* Video RAM starts at absolute address 0x000B8000. Each character on the screen is
* composed of two bytes: the ASCII character to appear on the screen followed by a video
* attribute. An attribute of 0x07 displays the character in WHITE with a black background.
*
* Arguments : y corresponds to the desired row to clear. Valid row numbers are from
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
DATASHEET 相关芯片手册及ARM参考资料 examples LPC2100系列ARM7微控制器功能部件相关实验程序及专用工程模板 easyarm_drive EasyJTAG仿真器驱动程序 sofware EasyARM软件及相关实验程序 lpc_can LPC2000的CAN接口参考资料 LPC210x ISP LPC210x的ISP软件 Ucosii 2.52 for lpc2100 uC/OS-II移植程序及相关中间件 LPC2114 component library LPC2114芯片SCH、PCB库 program file 开发板出厂时的编程文件 other ARM development board 其它ARM开发板简介
资源推荐
资源详情
资源评论
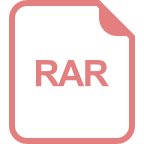
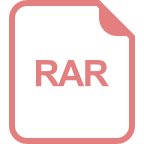
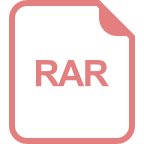
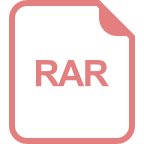
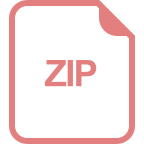
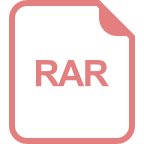
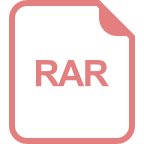
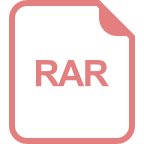
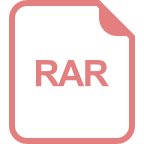
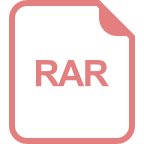
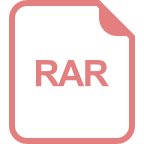
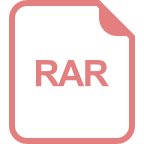
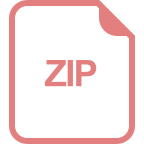
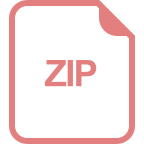
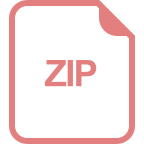
收起资源包目录

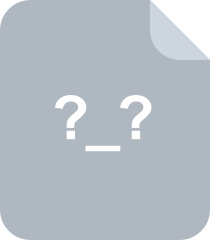
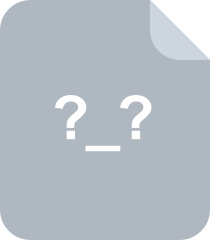
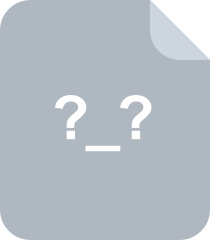
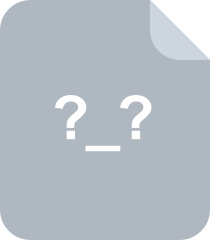
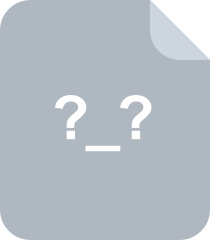
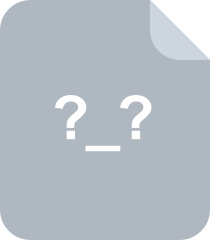
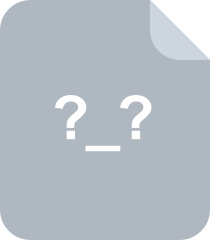
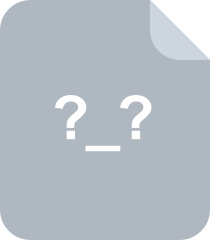
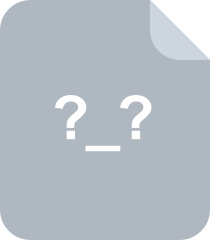
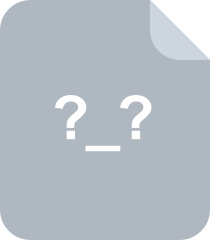
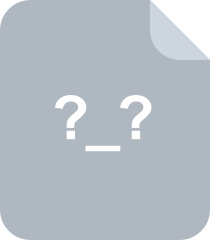
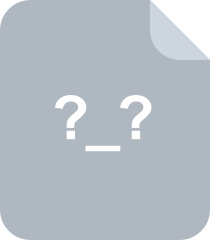
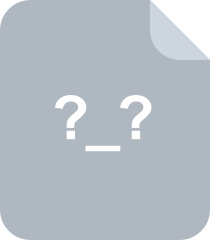
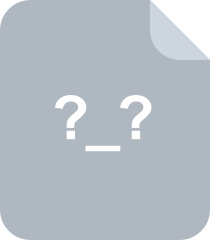
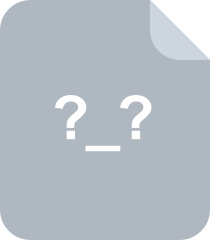
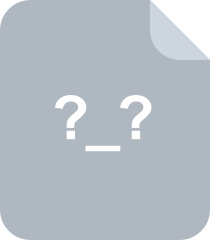
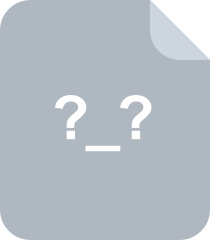
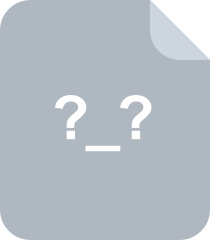
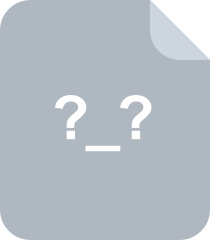
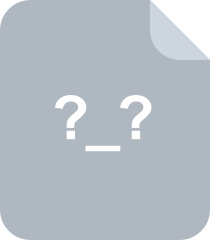
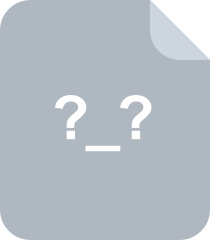
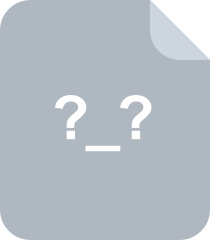
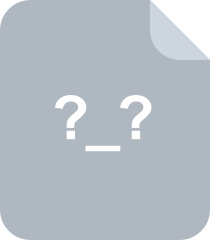
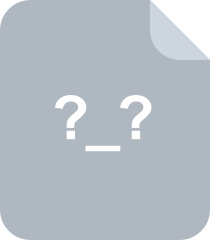
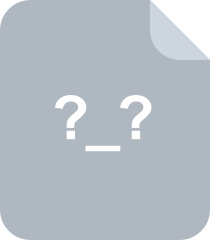
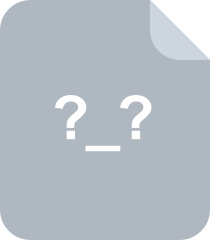
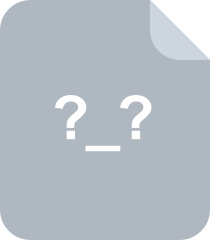
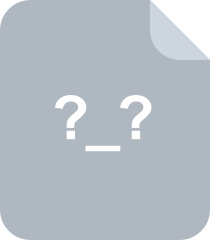
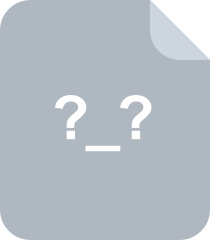
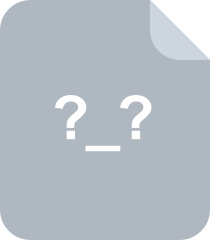
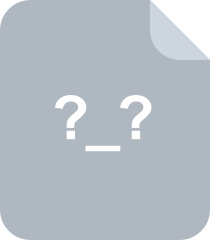
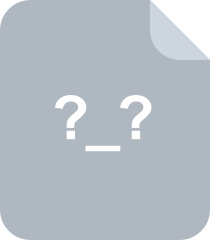
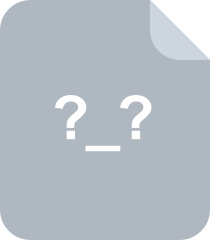
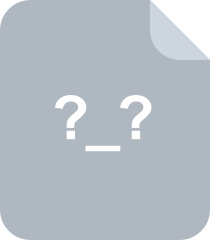
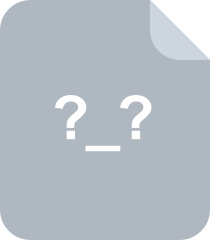
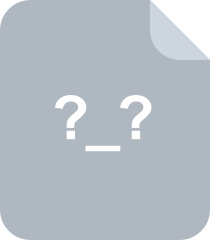
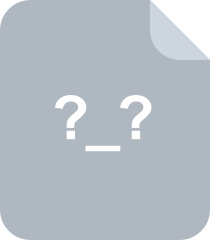
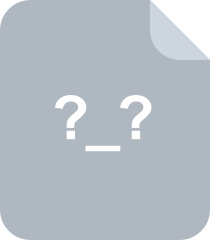
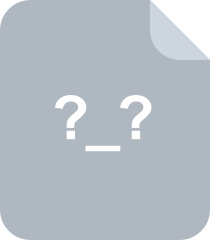
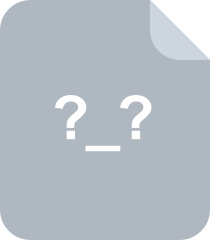
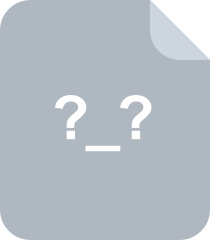
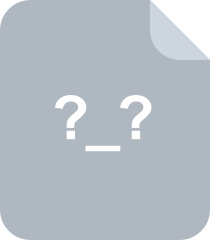
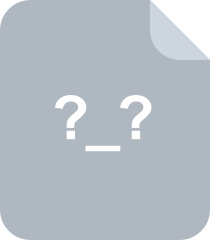
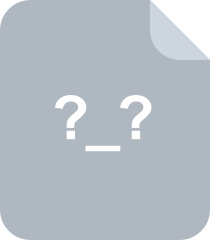
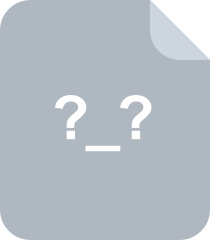
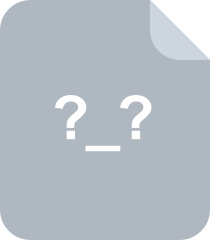
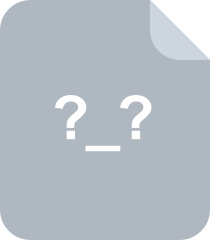
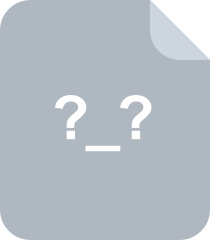
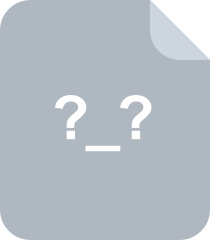
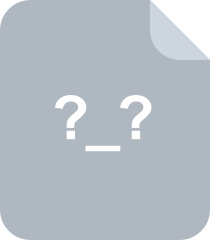
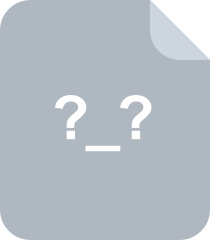
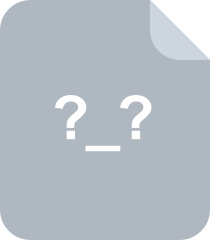
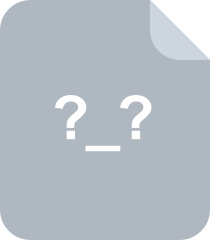
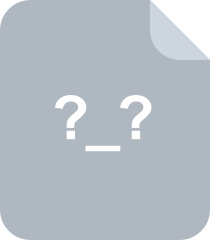
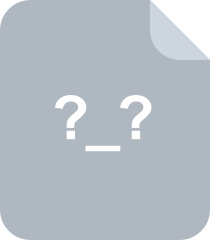
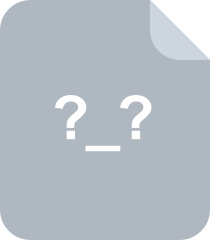
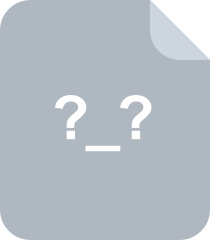
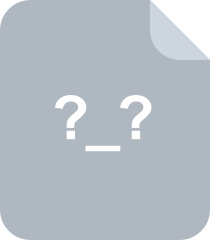
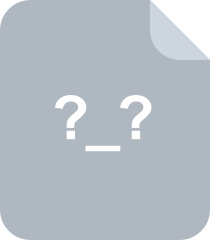
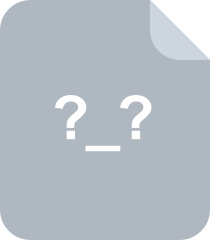
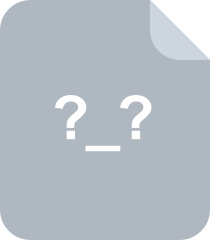
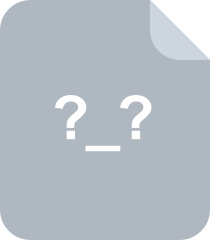
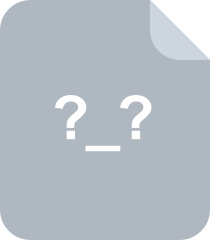
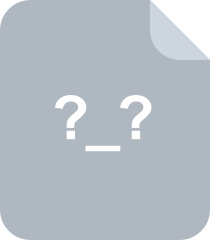
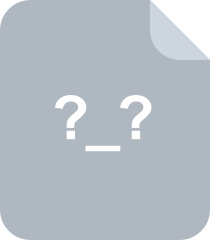
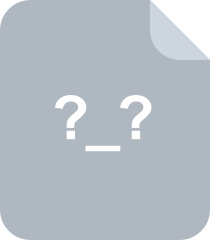
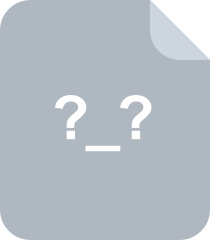
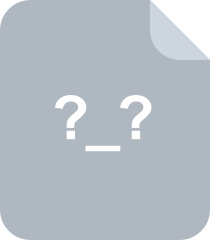
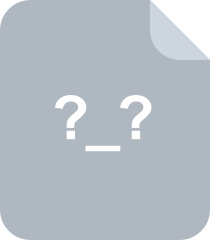
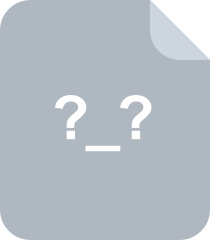
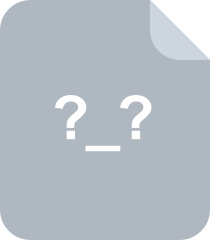
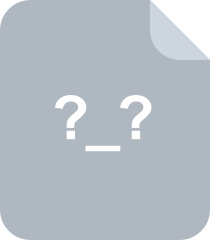
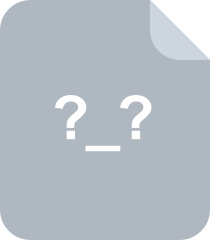
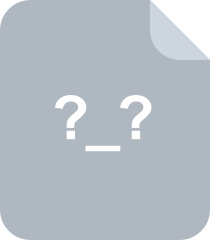
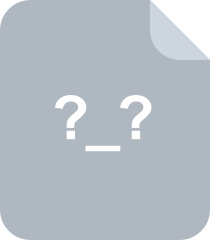
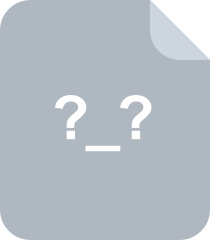
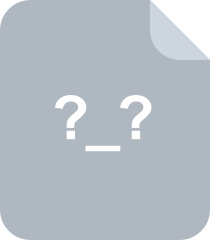
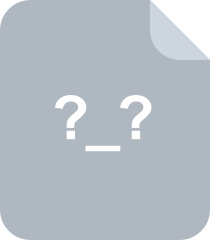
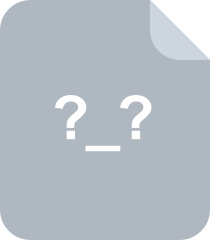
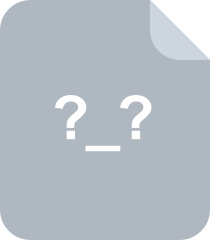
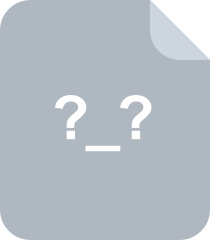
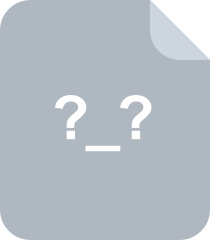
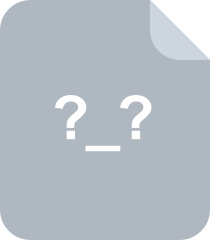
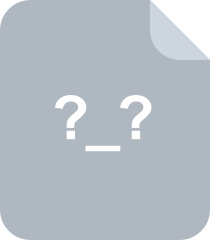
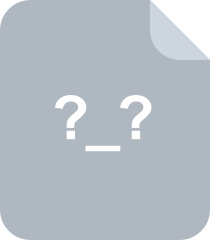
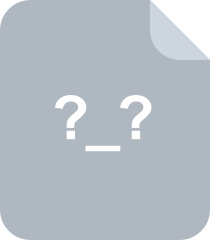
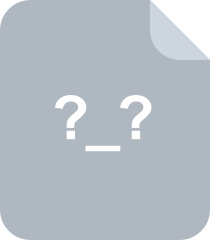
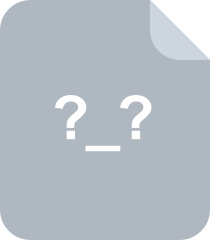
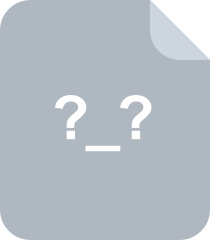
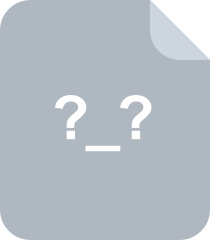
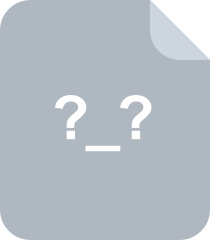
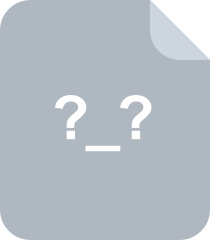
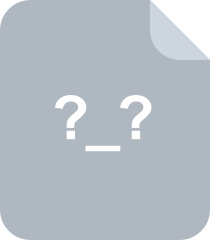
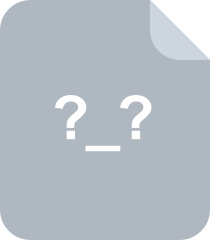
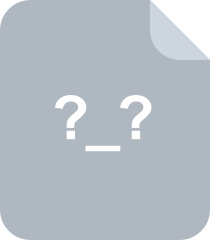
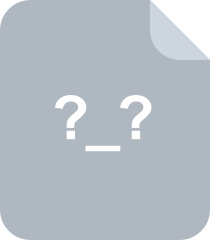
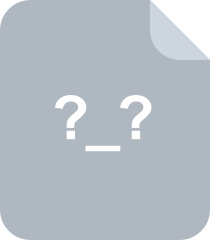
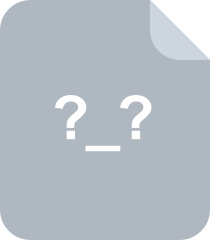
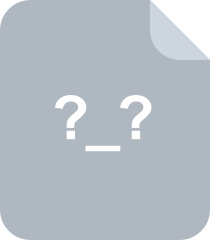
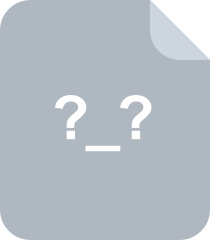
共 1605 条
- 1
- 2
- 3
- 4
- 5
- 6
- 17
资源评论
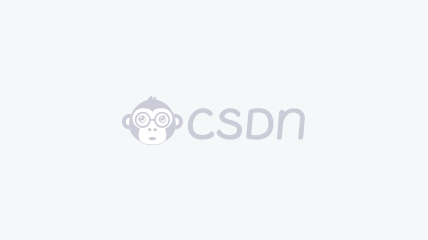

teliduxingdeji
- 粉丝: 5
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

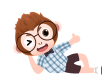
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


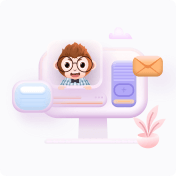
安全验证
文档复制为VIP权益,开通VIP直接复制
