/*
* thr_queue.cpp
*
* Created on: 2010-4-21
* Author: hollow
*/
#include <stdlib.h> // malloc
#include "thr_queue.h"
struct thr_queue_s {
void ** _queue;
size_t _max_size;
size_t _cur_size;
size_t _head;
size_t _tail;
pthread_mutex_t _mutex;
pthread_cond_t _cond_avail; // signal when queue is not full, can push more
pthread_cond_t _cond_have; // signal when queue is not empty, can pop
};
// ---------------- Create/Destroy -------------------
int thr_queue_create(thr_queue_t *q, size_t max_size)
{
struct thr_queue_s *queue = (struct thr_queue_s *) malloc(sizeof(struct thr_queue_s));
if (queue == NULL) {
return -1;
}
queue->_queue = (void **) malloc(max_size * sizeof(void *));
if (queue->_queue == NULL) {
free(queue);
queue = NULL;
return -1;
}
queue->_max_size = max_size;
queue->_cur_size = queue->_head = queue->_tail = 0;
pthread_mutex_init(&queue->_mutex, NULL);
pthread_cond_init(&queue->_cond_avail, NULL);
pthread_cond_init(&queue->_cond_have, NULL);
*q = queue;
return 0;
}
void thr_queue_destroy(thr_queue_t *q)
{
struct thr_queue_s *queue;
if ( q == NULL || *q == NULL )
return;
queue = *q;
pthread_cond_destroy(&queue->_cond_have);
pthread_cond_destroy(&queue->_cond_avail);
pthread_mutex_destroy(&queue->_mutex);
free(queue->_queue);
free(queue);
}
// ---------------- push/pop -------------------
size_t thr_queue_size(const thr_queue_t *self)
{
return (*self)->_cur_size;
}
size_t thr_queue_capacity(const thr_queue_t *self)
{
return (*self)->_max_size;
}
void thr_queue_push(thr_queue_t *q, void *in)
{
struct thr_queue_s *self = *q;
pthread_mutex_lock(&self->_mutex);
while (self->_cur_size == self->_max_size)
{
// full? wait it
pthread_cond_wait(&self->_cond_avail, &self->_mutex);
}
self->_queue[self->_tail++] = in;
if (self->_tail == self->_max_size)
{
self->_tail = 0;
}
if (self->_cur_size++ == 0)
{
// not empty? wakeup pop()
pthread_cond_signal(&self->_cond_have);
}
pthread_mutex_unlock(&self->_mutex);
}
void thr_queue_pop(thr_queue_t *q, void **out)
{
struct thr_queue_s *self = *q;
pthread_mutex_lock(&self->_mutex);
while (self->_cur_size == 0)
{
// empty? wait
pthread_cond_wait(&self->_cond_have, &self->_mutex);
}
if (out)
{
*out = self->_queue[self->_head];
}
self->_head++;
if (self->_head == self->_max_size)
{
self->_head = 0;
}
if (self->_cur_size-- == self->_max_size)
{
// not full? wakeup push()
pthread_cond_signal(&self->_cond_avail);
}
pthread_mutex_unlock(&self->_mutex);
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
前段时间整理一下代码,仿照java的mina自己做了一套C++的异步socket IO 框架。 编译环境: fedora 10 / cenos 5.4 / cygwin gcc version 4.3.2 其他linux环境没试过,不过应该也没啥问题。 使用到的库: 如果光使用socket 只需要 apr-1 库即可, 我用的版本是 0.3.8 代码中集成了 config++ 一个读配置文件的库。 log4cxx log4j 的 C++ 版本,写日志的。 Postgresql开发 使用了 pqxx 库 这是基于 pq库(postgresql 的 c 语言api ) 的c++库 工程 core : 库工程 工程 tools: 例子 motcp socket存储转发功能,可以将通信的包以十六进制的格式打印出来,调试网络程序的好帮手 echoserv echo 服务器,把客户端的数据不更改地返回。 编译,提供两种编译模式,windows的cygwin环境,还有linux环境。不用配置,直接make即可。 先编译core工程,在core工程下直接执行make指令 然后编译tools 工程 , 在工程下直接执行 make 指令 开发环境 eclipse cdt
资源推荐
资源详情
资源评论
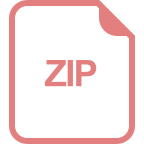
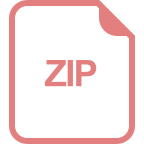
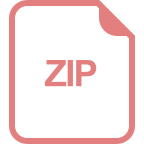
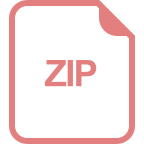
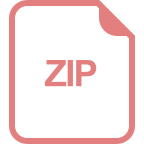
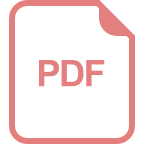
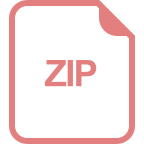
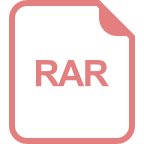
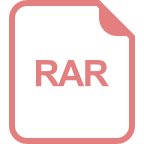
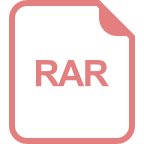
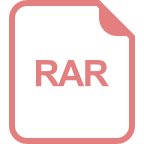
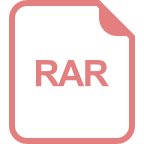
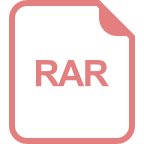
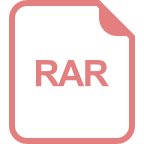
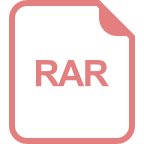
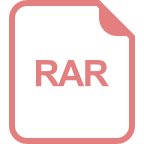
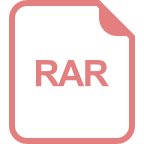
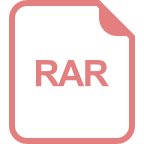
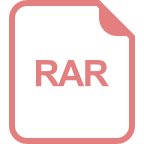
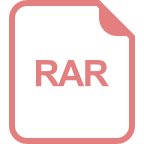
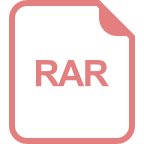
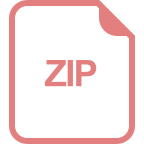
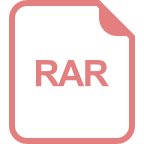
收起资源包目录


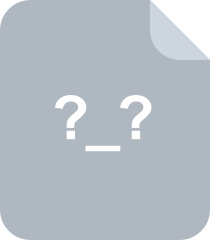
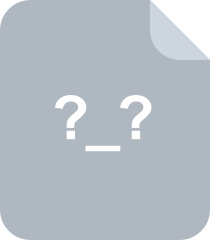

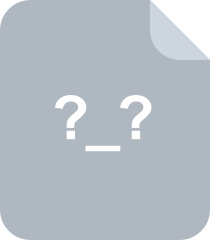
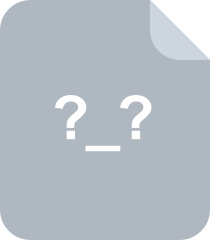
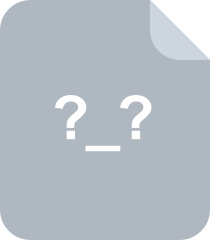
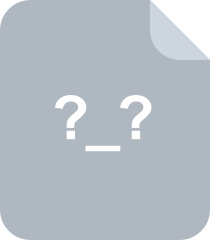

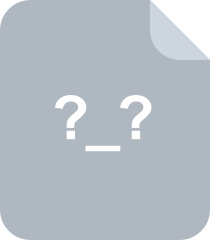
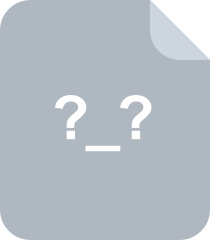

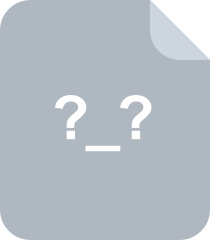
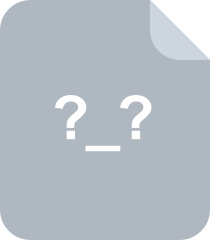
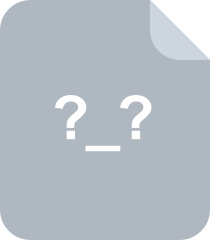
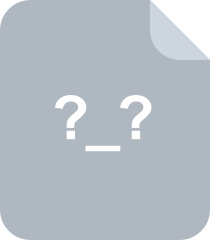
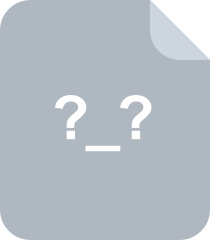
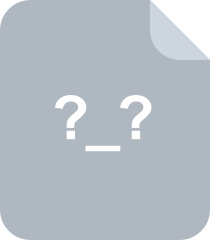
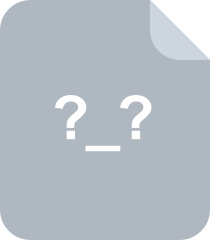
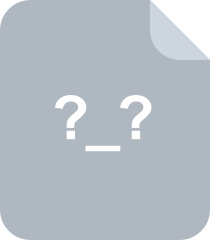
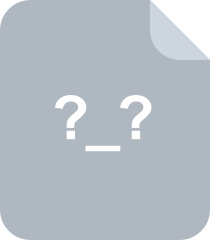
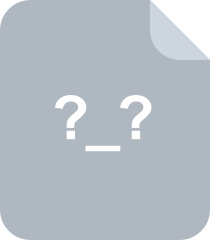
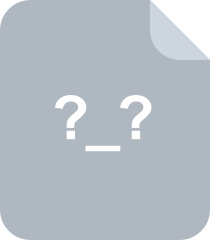

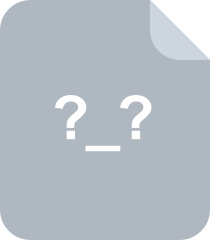
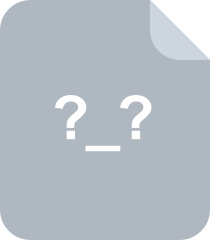
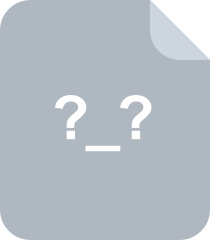
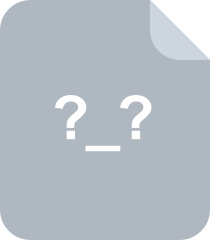
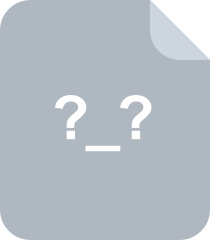
共 24 条
- 1

teadust
- 粉丝: 2
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

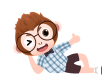
最新资源
- 5G建设和AI技术推动下,中证5G通信ETF的投资价值探讨
- Python项目之淘宝模拟登录.zip
- 课程设计项目:python+QT实现的小型编译器.zip
- (源码)基于AVR ATmega644的智能卡AES解密系统.zip
- (源码)基于C++插件框架的计算与打印系统.zip
- (源码)基于Spring Boot和Vue的苍穹外卖管理系统.zip
- (源码)基于wxWidgets库的QMiniIDE游戏开发环境管理系统.zip
- 通过C++实现原型模式(Prototype Pattern).rar
- 学习记录111111111111111111111111
- 通过java实现原型模式(Prototype Pattern).rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


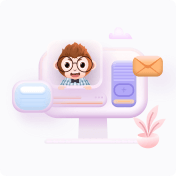
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页