Android垂直滚动
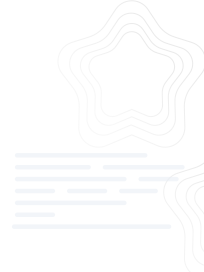


在Android开发中,垂直滚动是用户界面中常见的一种交互方式,尤其在长内容展示时尤为重要。本主题将深入探讨如何实现Android垂直滚动,主要聚焦于`ScrollView`组件的使用及其改写。 `ScrollView`是Android SDK提供的一种布局容器,它允许用户在单个屏幕内滚动查看超过屏幕高度的内容。它只能包含一个直接子视图,但这个子视图可以是一个复杂的布局,如`LinearLayout`或`RelativeLayout`,这些布局中可以包含多个子元素。 ### 1. ScrollView基本用法 创建`ScrollView`的基本步骤如下: 1. 在XML布局文件中添加`<ScrollView>`标签。 2. 将需要滚动的布局作为`ScrollView`的唯一子视图。 3. 设置子视图的宽度和高度为`match_parent`,以确保其能填充`ScrollView`。 例如: ```xml <ScrollView xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <!-- 这里放置需要滚动的子视图 --> </LinearLayout> </ScrollView> ``` ### 2. 自动滚动 在标题中提到的"AutoScroll"可能涉及到自动滚动功能。`ScrollView`本身并不支持自动滚动,但可以通过编程方式实现。例如,可以使用`postDelayed()`和`scrollBy()`方法来实现定时滚动: ```java final ScrollView scrollView = findViewById(R.id.scroll_view); final int scrollAmount = 5; // 每次滚动的距离 new Handler().post(new Runnable() { @Override public void run() { if (scrollView.getChildAt(0).getBottom() <= scrollView.getBottom()) { return; } scrollView.smoothScrollBy(scrollAmount, 0); scrollView.postDelayed(this, 100); // 每100毫秒滚动一次 } }); ``` ### 3. 改写ScrollView 在某些情况下,可能需要对`ScrollView`进行定制以满足特定需求,例如添加滚动监听、处理嵌套滚动等。这时,可以创建一个新的自定义`ScrollView`类,继承自`ScrollView`,然后重写需要的方法。 例如,如果你需要在滚动到底部时执行某操作,可以这样改写: ```java public class CustomScrollView extends ScrollView { public CustomScrollView(Context context) { super(context); } public CustomScrollView(Context context, AttributeSet attrs) { super(context, attrs); } public CustomScrollView(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); } @Override protected void onScrollChanged(int x, int y, int oldx, int oldy) { super.onScrollChanged(x, y, oldx, oldy); View child = getChildAt(getChildCount() - 1); int bottom = child.getBottom(); int height = getHeight() + getScrollY(); if (bottom == height) { // 滚动到底部,执行相应操作 Log.d("CustomScrollView", "Scrolled to bottom"); } } } ``` ### 4. 嵌套滚动与NestedScrollView 在现代的Android开发中,`NestedScrollView`是`ScrollView`的一个替代品,它支持嵌套滚动协议,能够与`CoordinatorLayout`等组件协同工作,适用于实现如抽屉布局、底部导航栏等复杂布局。 ### 5. 性能优化 虽然`ScrollView`提供了方便的滚动功能,但过度使用会导致性能问题。尽量减少嵌套滚动,避免在`ScrollView`内使用`ListView`或`RecyclerView`。如果需要显示大量可滚动数据,优先考虑使用`RecyclerView`,并利用其滚动优化功能。 总结来说,Android垂直滚动主要涉及`ScrollView`组件的使用,包括基本用法、自动滚动、改写以及与嵌套滚动相关的优化。通过理解并熟练掌握这些知识点,开发者可以构建出更加流畅、用户体验良好的Android应用。
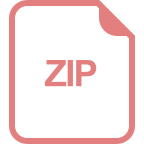
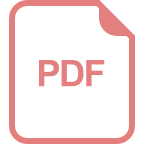
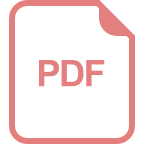
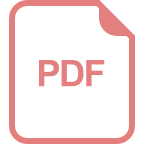
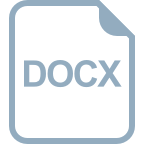
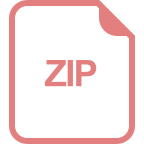
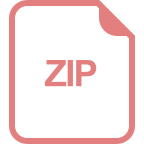
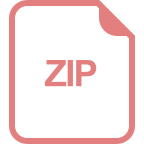
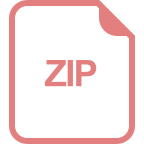
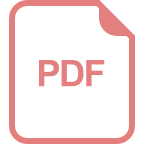
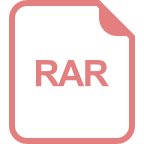
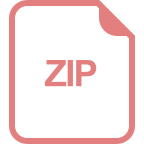
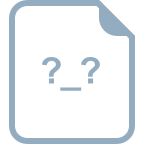



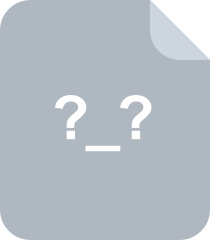



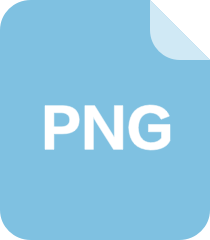

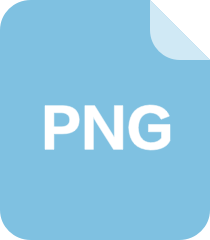

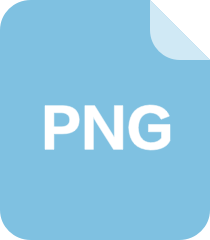

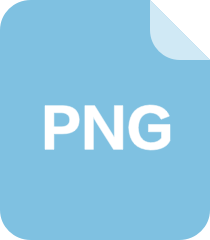
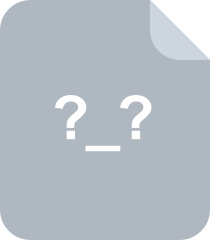

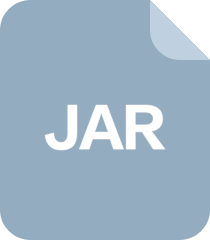




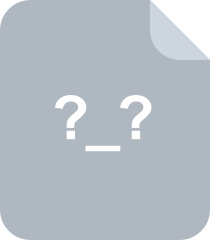
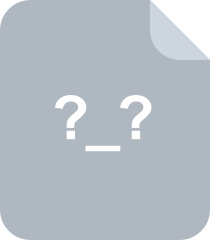
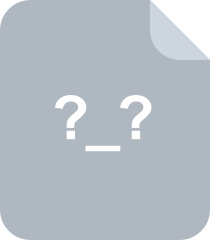
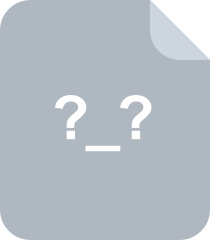
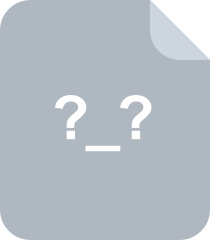
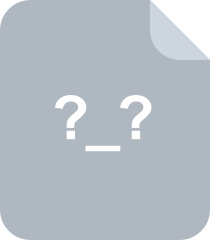
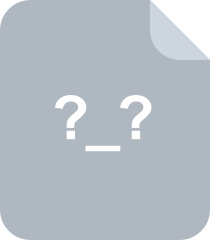
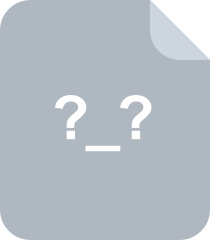
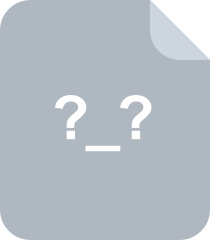
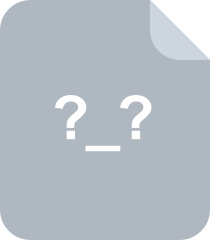


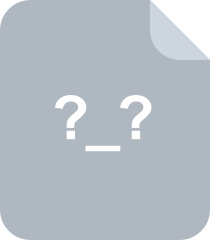
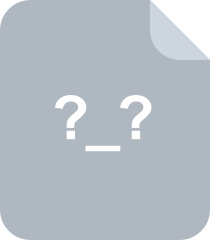
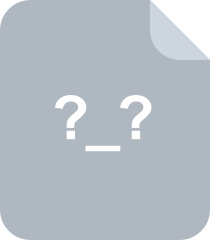
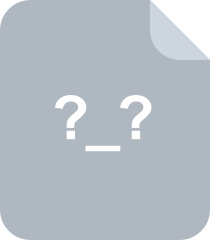
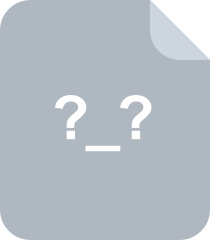



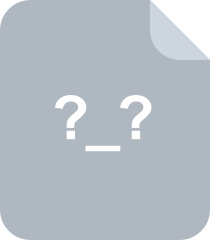

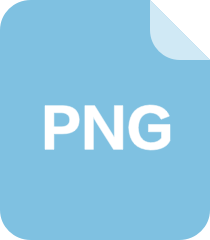
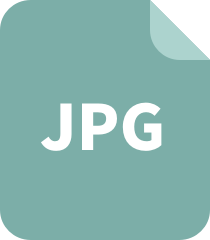
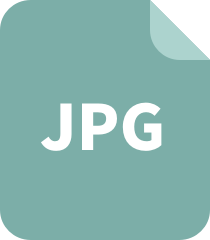

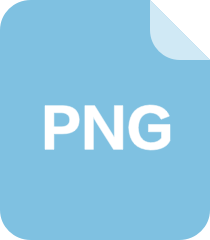

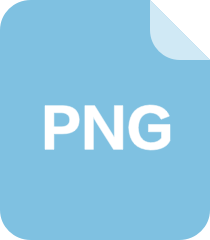

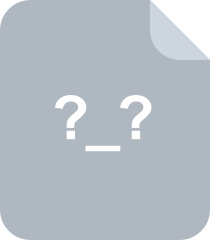
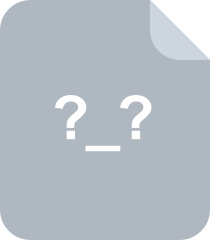
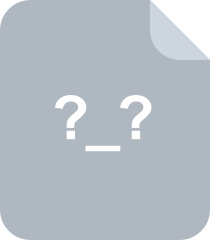

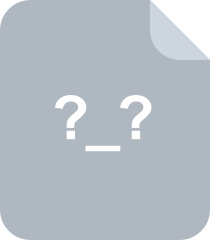

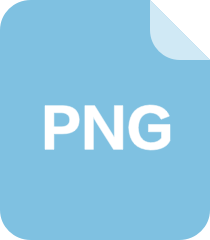

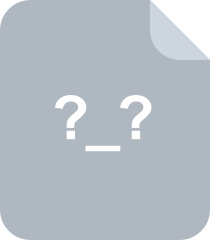

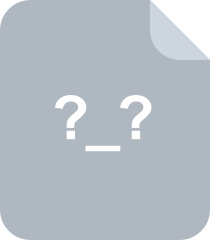
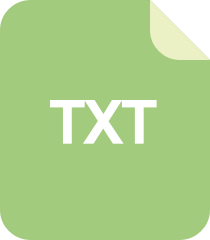
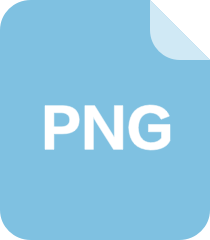





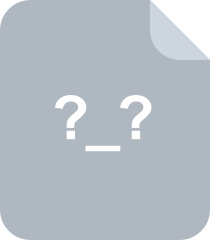
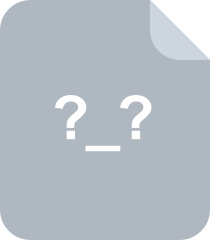

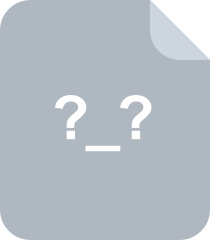
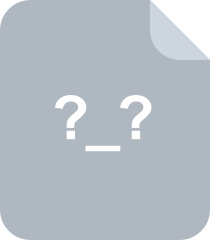




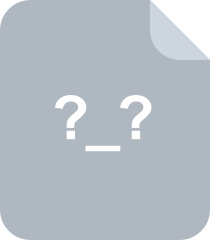
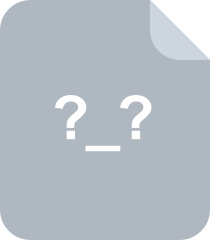
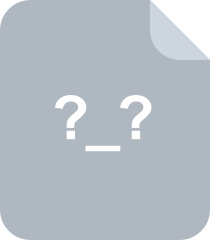
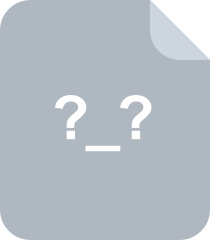
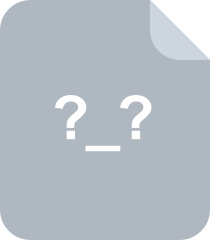
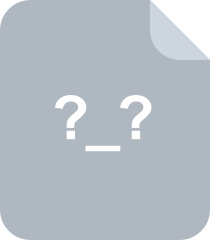

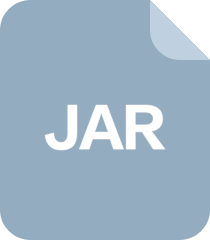
- 1
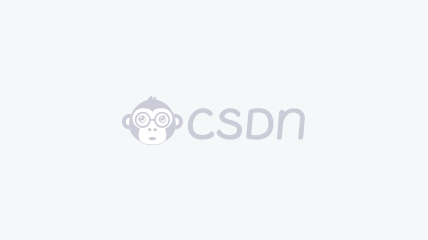
- 我就是我zx2015-11-11效果还可以,实用
- liumeng9202015-12-01还不错,可以借鉴.
- ligen68853942015-11-04挺好的,很实用,
- argive2016-03-22有点颤抖,不能点击。

- 粉丝: 6
- 资源: 17
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

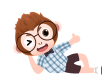
最新资源
- 5G SRM815模组原理框图.jpg
- T型3电平逆变器,lcl滤波器滤波器参数计算,半导体损耗计算,逆变电感参数设计损耗计算 mathcad格式输出,方便修改 同时支持plecs损耗仿真,基于plecs的闭环仿真,电压外环,电流内环
- 毒舌(解锁版).apk
- 显示HEX、S19、Bin、VBF等其他汽车制造商特定的文件格式
- 8bit逐次逼近型SAR ADC电路设计成品 入门时期的第三款sarADC,适合新手学习等 包括电路文件和详细设计文档 smic0.18工艺,单端结构,3.3V供电 整体采样率500k,可实现基
- 操作系统实验 ucorelab4内核线程管理
- 脉冲注入法,持续注入,启动低速运行过程中注入,电感法,ipd,力矩保持,无霍尔无感方案,媲美有霍尔效果 bldc控制器方案,无刷电机 提供源码,原理图
- Matlab Simulink#直驱永磁风电机组并网仿真模型 基于永磁直驱式风机并网仿真模型 采用背靠背双PWM变流器,先整流,再逆变 不仅实现电机侧的有功、无功功率的解耦控制和转速调节,而且能实
- 157389节奏盒子地狱模式第三阶段7.apk
- 操作系统实验ucore lab3

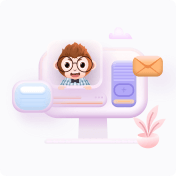
