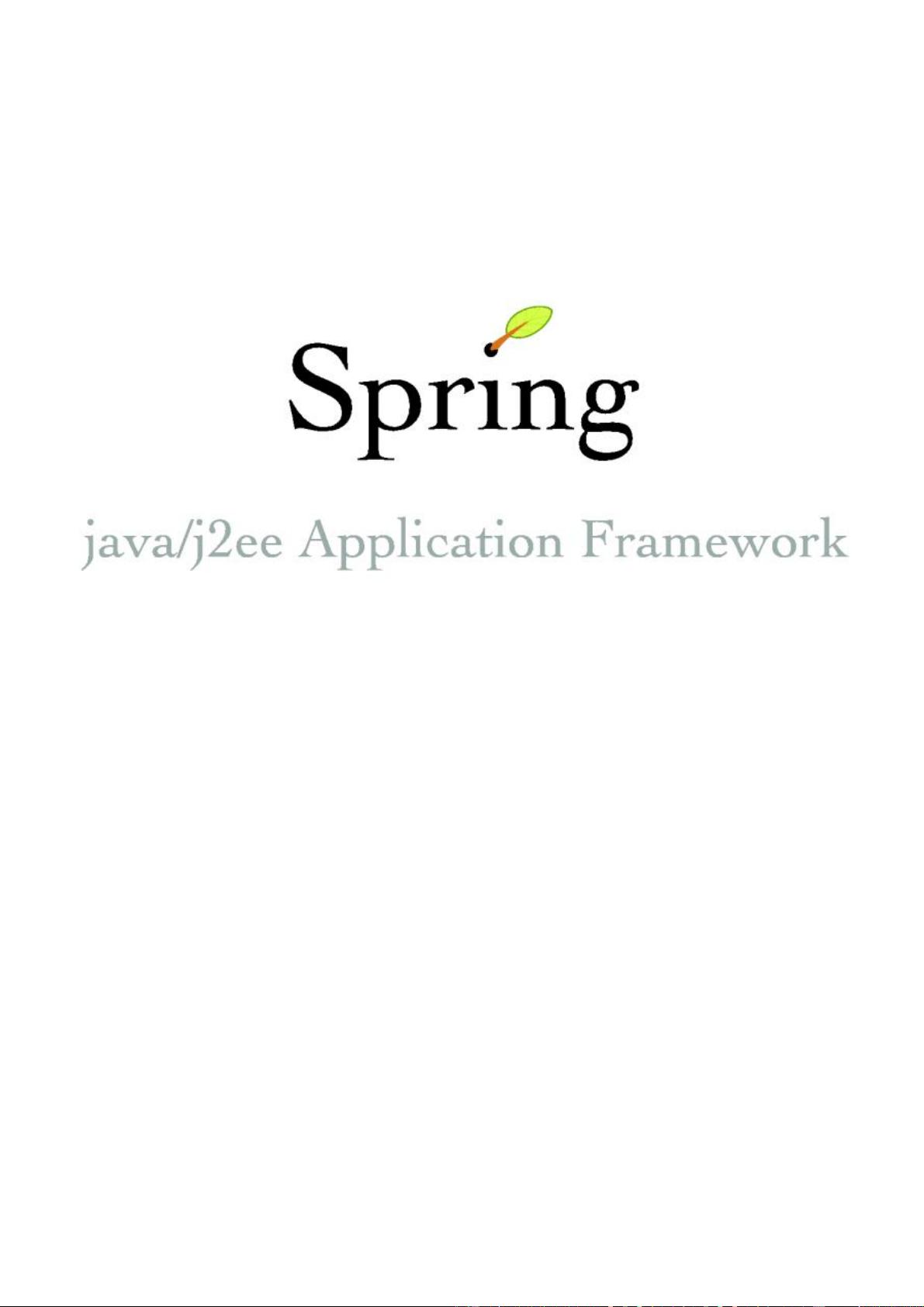
Reference Documentation
Version 1.2.9
(Work in progress)
Copyright (c) 2004-2007 Rod Johnson, Juergen Hoeller, Alef Arendsen, Colin Sampaleanu,
Rob Harrop, Thomas Risberg, Darren Davison, Dmitriy Kopylenko, Mark Pollack, Thierry
Templier, Erwin Vervaet
Copies of this document may be made for your own use and for distribution to others, provided that you do not
charge any fee for such copies and further provided that each copy contains this Copyright Notice, whether
distributed in print or electronically.
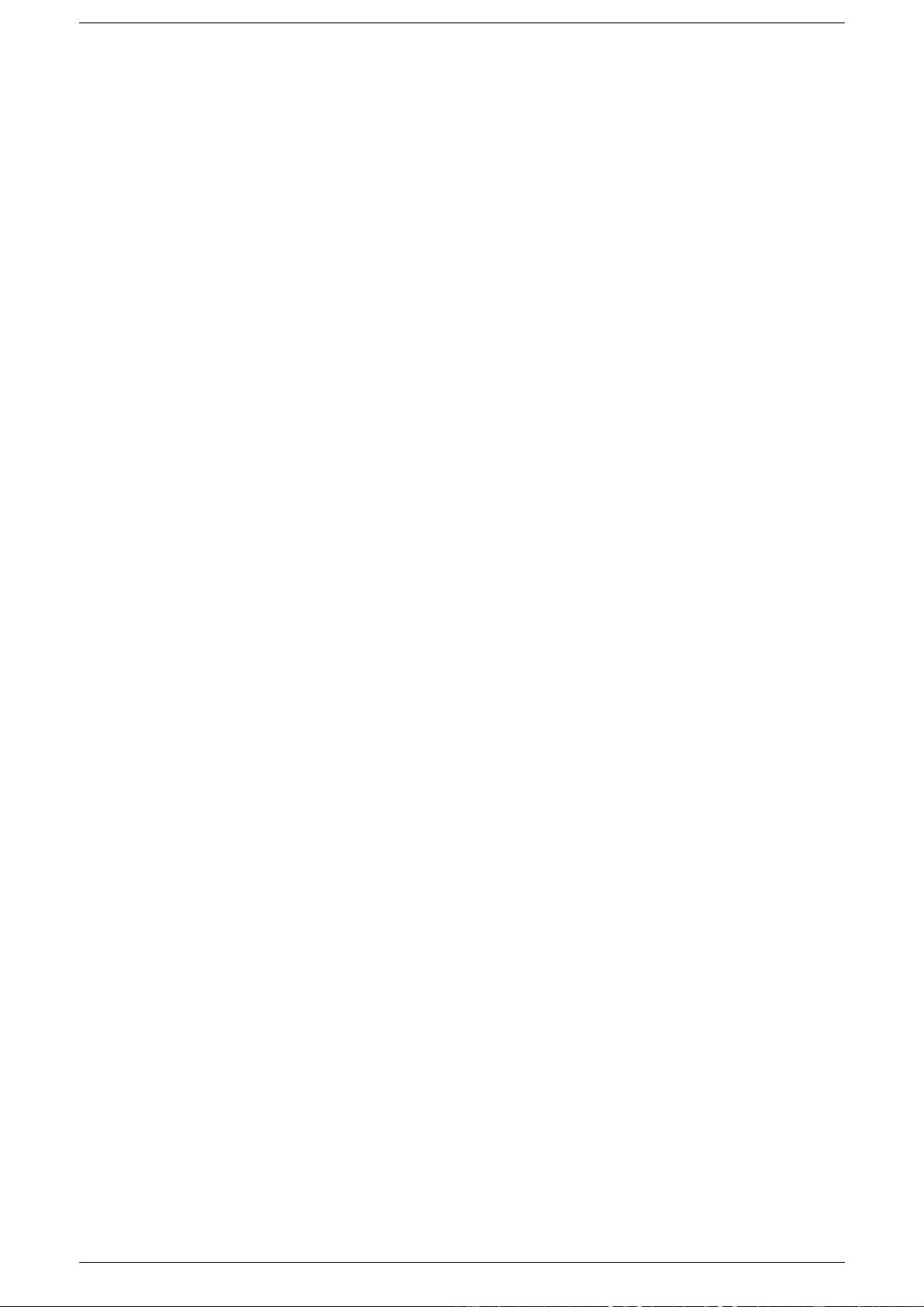
Preface ................................................................................................................................................ xi
1. Introduction .................................................................................................................................. 12
1.1. Overview ............................................................................................................................. 12
1.2. Usage scenarios .................................................................................................................... 13
2. Background information ............................................................................................................... 16
2.1. Inversion of Control / Dependency Injection .......................................................................... 16
3. Beans, BeanFactory and the ApplicationContext .......................................................................... 17
3.1. Introduction ......................................................................................................................... 17
3.2. BeanFactory and BeanDefinitions - the basics ........................................................................ 17
3.2.1. The BeanFactory ....................................................................................................... 17
3.2.2. The BeanDefinition ................................................................................................... 19
3.2.3. The bean class ........................................................................................................... 19
3.2.4. The bean identifiers (id and
name) ............................................................................................................................. 21
3.2.5. To singleton or not to singleton .................................................................................. 21
3.3. Properties, collaborators, autowiring and dependency
checking .................................................................................................................................. 22
3.3.1. Setting bean properties and collaborators .................................................................... 22
3.3.2. Constructor Argument Resolution ............................................................................... 25
3.3.3. Bean properties and constructor arguments detailed ..................................................... 26
3.3.4. Method Injection ....................................................................................................... 30
3.3.5. Using depends-on ..................................................................................................... 32
3.3.6. Autowiring collaborators ............................................................................................ 33
3.3.7. Checking for dependencies ......................................................................................... 34
3.4. Customizing the nature of a bean ........................................................................................... 34
3.4.1. Lifecycle interfaces ................................................................................................... 35
3.4.2. Knowing who you are ................................................................................................ 36
3.4.3. FactoryBean .............................................................................................................. 37
3.5. Abstract and child bean definitions ........................................................................................ 37
3.6. Interacting with the BeanFactory ........................................................................................... 38
3.6.1. Obtaining a FactoryBean, not its product ..................................................................... 39
3.7. Customizing beans with BeanPostProcessors ......................................................................... 39
3.8. Customizing bean factories with BeanFactoryPostProcessors .................................................. 39
3.8.1. The PropertyPlaceholderConfigurer ....................................................................... 40
3.8.2. The PropertyOverrideConfigurer ............................................................................ 41
3.9. Registering additional custom PropertyEditors ....................................................................... 41
3.10. Using the alias element to add aliases for existing beans ....................................................... 42
3.11. Introduction to the ApplicationContext .............................................................................. 43
3.12. Added functionality of the
ApplicationContext ............................................................................................................... 43
3.12.1. Using the MessageSource ......................................................................................... 43
3.12.2. Propagating events ................................................................................................... 44
3.12.3. Low-level resources and the application context ........................................................ 45
3.13. Customized behavior in the ApplicationContext ................................................................... 46
3.13.1. ApplicationContextAware marker
interface ........................................................................................................................ 46
3.13.2. The BeanPostProcessor .......................................................................................... 46
3.13.3. The BeanFactoryPostProcessor .............................................................................. 46
3.13.4. The PropertyPlaceholderConfigurer ..................................................................... 47
3.14. Registering additional custom PropertyEditors ..................................................................... 47
3.15. Setting a bean property or constructor arg from a property
expression ............................................................................................................................... 48
Spring Framework Version 1.2.9 ii
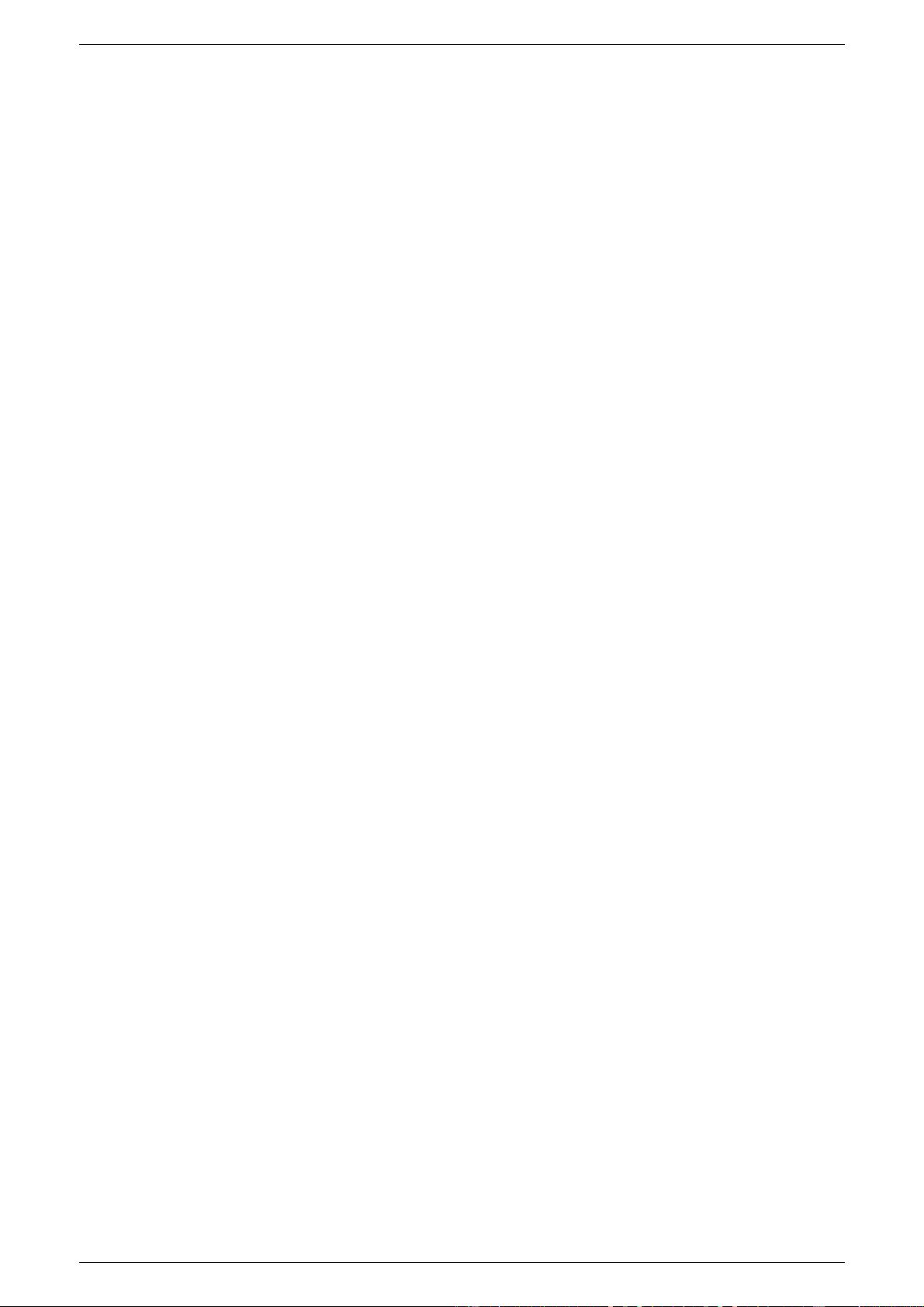
3.16. Setting a bean property or constructor arg from a field
value ....................................................................................................................................... 49
3.17. Invoking another method and optionally using the return
value. ...................................................................................................................................... 49
3.18. Importing Bean Definitions from One File Into Another ....................................................... 50
3.19. Creating an ApplicationContext from a web application ........................................................ 51
3.20. Glue code and the evil singleton .......................................................................................... 52
3.20.1. Using SingletonBeanFactoryLocator and
ContextSingletonBeanFactoryLocator ............................................................................. 52
4. Abstracting Access to Low-Level Resources .................................................................................. 54
4.1. Overview ............................................................................................................................. 54
4.2. The Resource interface ......................................................................................................... 54
4.3. Built-in Resource implementations ........................................................................................ 55
4.3.1. UrlResource ............................................................................................................. 55
4.3.2. ClassPathResource ................................................................................................... 55
4.3.3. FileSystemResource ................................................................................................. 56
4.3.4. ServletContextResource .......................................................................................... 56
4.3.5. InputStreamResource ............................................................................................... 56
4.3.6. ByteArrayResource ................................................................................................... 56
4.4. The ResourceLoader Interface .............................................................................................. 56
4.5. The ResourceLoaderAware interface ..................................................................................... 57
4.6. Setting Resources as properties ............................................................................................. 57
4.7. Application contexts and Resource paths ............................................................................... 58
4.7.1. Constructing application contexts ............................................................................... 58
4.7.2. The classpath*: prefix ............................................................................................. 58
4.7.3. Unexpected application context handling of FileSystemResource
absolute paths ................................................................................................................ 59
5. PropertyEditors, data binding, validation and the BeanWrapper ................................................. 61
5.1. Introduction ......................................................................................................................... 61
5.2. Binding data using the DataBinder ........................................................................................ 61
5.3. Bean manipulation and the BeanWrapper ................................................................................ 61
5.3.1. Setting and getting basic and nested properties ............................................................ 62
5.3.2. Built-in PropertyEditors, converting
types ............................................................................................................................. 63
5.3.3. Other features worth mentioning ................................................................................. 65
5.4. Validation using Spring's Validator interface .......................................................................... 65
5.5. The Errors interface .............................................................................................................. 66
5.6. Resolving codes to error messages ......................................................................................... 66
6. Spring AOP: Aspect Oriented Programming with Spring ............................................................. 67
6.1. Concepts .............................................................................................................................. 67
6.1.1. AOP concepts ........................................................................................................... 67
6.1.2. Spring AOP capabilities and goals .............................................................................. 68
6.1.3. AOP Proxies in Spring ............................................................................................... 69
6.2. Pointcuts in Spring ............................................................................................................... 69
6.2.1. Concepts ................................................................................................................... 70
6.2.2. Operations on pointcuts ............................................................................................. 70
6.2.3. Convenience pointcut implementations ....................................................................... 71
6.2.4. Pointcut superclasses ................................................................................................. 72
6.2.5. Custom pointcuts ....................................................................................................... 73
6.3. Advice types in Spring .......................................................................................................... 73
6.3.1. Advice lifecycles ....................................................................................................... 73
6.3.2. Advice types in Spring ............................................................................................... 73
Spring - Java/J2EE Application Framework
Spring Framework Version 1.2.9 iii
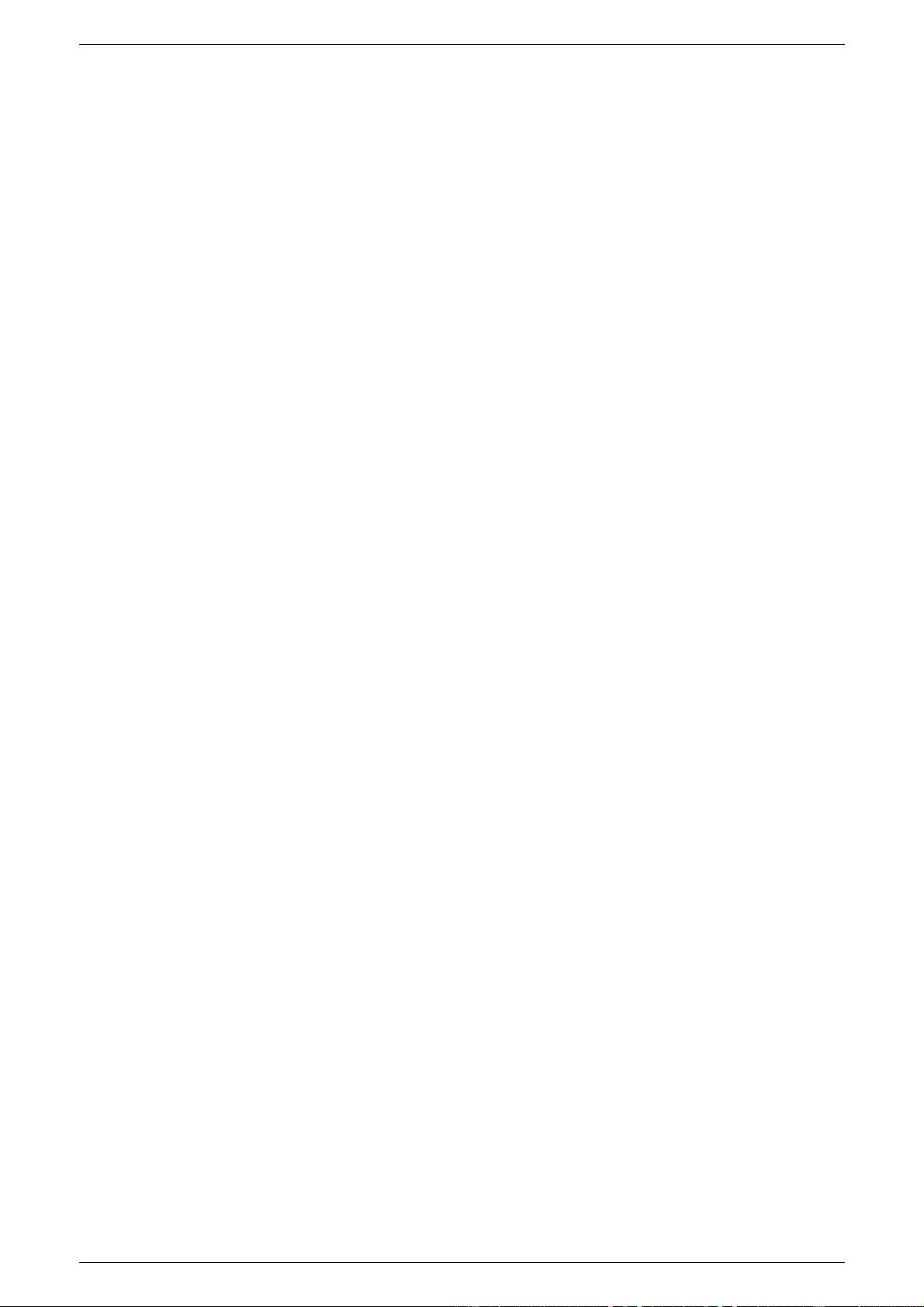
6.4. Advisors in Spring ................................................................................................................ 78
6.5. Using the ProxyFactoryBean to create AOP proxies ............................................................... 78
6.5.1. Basics ....................................................................................................................... 78
6.5.2. JavaBean properties ................................................................................................... 79
6.5.3. Proxying interfaces .................................................................................................... 80
6.5.4. Proxying classes ........................................................................................................ 81
6.5.5. Using 'global' advisors ............................................................................................... 82
6.6. Convenient proxy creation .................................................................................................... 82
6.6.1. TransactionProxyFactoryBean .................................................................................... 82
6.6.2. EJB proxies ............................................................................................................... 83
6.7. Concise proxy definitions ..................................................................................................... 84
6.8. Creating AOP proxies programmatically with the ProxyFactory .............................................. 85
6.9. Manipulating advised objects ................................................................................................ 85
6.10. Using the "autoproxy" facility ............................................................................................. 86
6.10.1. Autoproxy bean definitions ...................................................................................... 87
6.10.2. Using metadata-driven auto-proxying ....................................................................... 88
6.11. Using TargetSources ........................................................................................................... 90
6.11.1. Hot swappable target sources .................................................................................... 90
6.11.2. Pooling target sources .............................................................................................. 91
6.11.3. Prototype target sources ........................................................................................... 92
6.11.4. ThreadLocal target sources ....................................................................................... 92
6.12. Defining new Advice types ................................................................................................. 93
6.13. Further reading and resources .............................................................................................. 93
7. AspectJ Integration ....................................................................................................................... 94
7.1. Overview ............................................................................................................................. 94
7.2. Configuring AspectJ aspects using Spring IoC ....................................................................... 94
7.2.1. "Singleton" aspects .................................................................................................... 94
7.2.2. Non-singleton aspects ................................................................................................ 95
7.2.3. Gotchas .................................................................................................................... 95
7.3. Using AspectJ pointcuts to target Spring advice ..................................................................... 95
7.4. Spring aspects for AspectJ .................................................................................................... 96
8. Transaction management .............................................................................................................. 97
8.1. The Spring transaction abstraction ......................................................................................... 97
8.2. Transaction strategies ........................................................................................................... 98
8.3. Resource synchronization with transactions ........................................................................... 100
8.3.1. High-level approach .................................................................................................. 100
8.3.2. Low-level approach ................................................................................................... 100
8.3.3. TransactionAwareDataSourceProxy ............................................................................ 101
8.4. Programmatic transaction management .................................................................................. 101
8.4.1. Using the TransactionTemplate ................................................................................ 102
8.4.2. Using the PlatformTransactionManager .................................................................... 102
8.5. Declarative transaction management ...................................................................................... 103
8.5.1. Source Annotations for Transaction Demarcation ........................................................ 105
8.5.2. BeanNameAutoProxyCreator,
another declarative approach ........................................................................................... 108
8.5.3. AOP and Transactions ............................................................................................... 110
8.6. Choosing between programmatic and declarative transaction
management ............................................................................................................................ 110
8.7. Do you need an application server for transaction
management? ........................................................................................................................... 110
8.8. AppServer-specific integration .............................................................................................. 110
8.8.1. BEA WebLogic ......................................................................................................... 111
Spring - Java/J2EE Application Framework
Spring Framework Version 1.2.9 iv
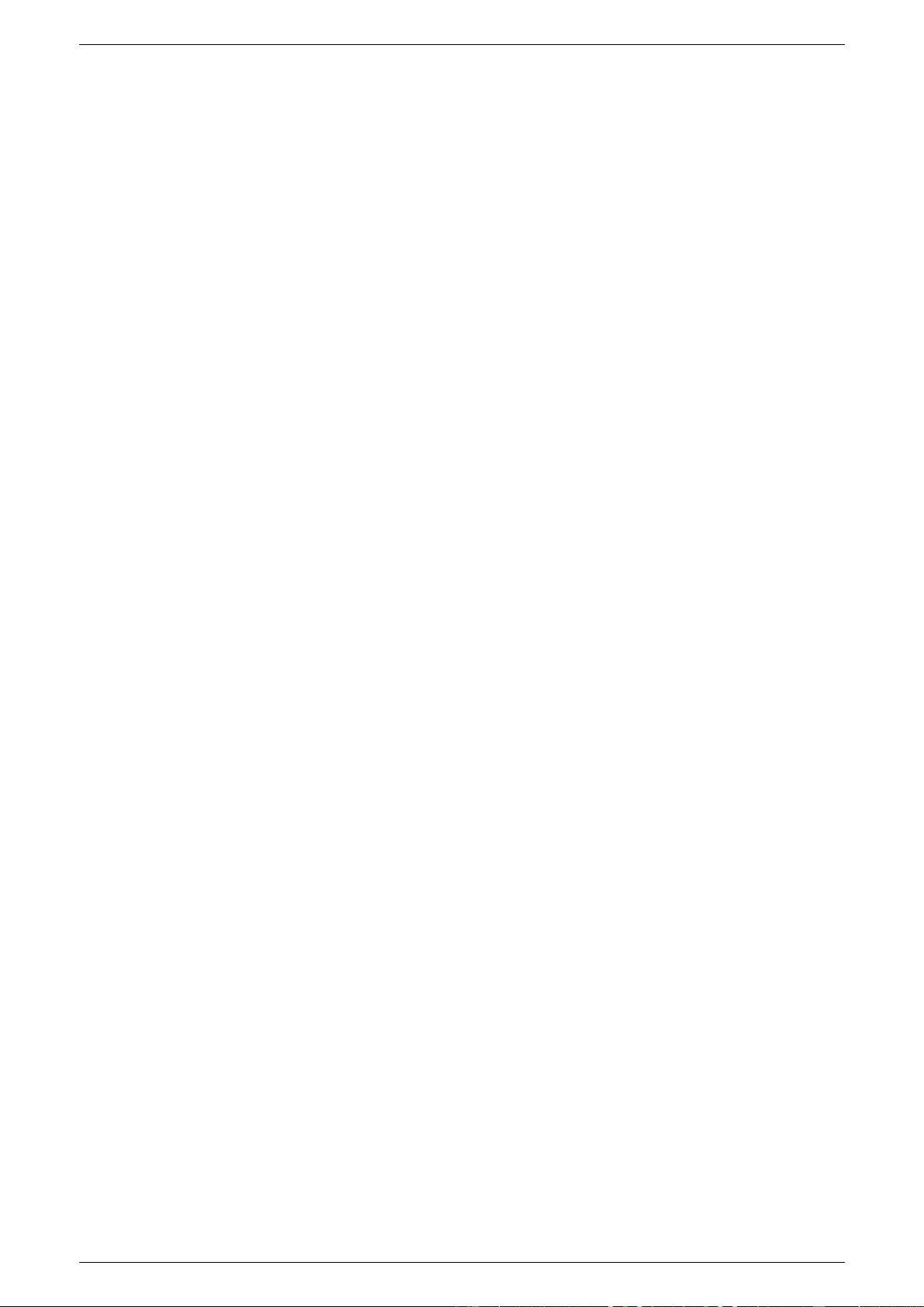
8.8.2. IBM WebSphere ........................................................................................................ 111
8.9. Common problems ............................................................................................................... 111
8.9.1. Use of the wrong transaction manager for a specific
DataSource .................................................................................................................... 111
8.9.2. Spurious AppServer warnings about the transaction or DataSource
no longer being active .................................................................................................... 111
9. Source Level Metadata Support .................................................................................................... 113
9.1. Source-level metadata ........................................................................................................... 113
9.2. Spring's metadata support ..................................................................................................... 114
9.3. Integration with Jakarta Commons Attributes ......................................................................... 115
9.4. Metadata and Spring AOP autoproxying ................................................................................ 116
9.4.1. Fundamentals ............................................................................................................ 116
9.4.2. Declarative transaction management ........................................................................... 117
9.4.3. Pooling ..................................................................................................................... 117
9.4.4. Custom metadata ....................................................................................................... 118
9.5. Using attributes to minimize MVC web tier configuration ....................................................... 119
9.6. Other uses of metadata attributes ........................................................................................... 121
9.7. Adding support for additional metadata APIs ......................................................................... 121
10. DAO support ............................................................................................................................... 122
10.1. Introduction ....................................................................................................................... 122
10.2. Consistent Exception Hierarchy ........................................................................................... 122
10.3. Consistent Abstract Classes for DAO Support ...................................................................... 123
11. Data Access using JDBC .............................................................................................................. 124
11.1. Introduction ....................................................................................................................... 124
11.2. Using the JDBC Core classes to control basic JDBC processing and
error handling .......................................................................................................................... 124
11.2.1. JdbcTemplate .......................................................................................................... 124
11.2.2. DataSource .............................................................................................................. 125
11.2.3. SQLExceptionTranslator .......................................................................................... 125
11.2.4. Executing Statements ............................................................................................... 126
11.2.5. Running Queries ...................................................................................................... 126
11.2.6. Updating the database .............................................................................................. 127
11.3. Controlling how we connect to the database ......................................................................... 128
11.3.1. DataSourceUtils ...................................................................................................... 128
11.3.2. SmartDataSource ..................................................................................................... 128
11.3.3. AbstractDataSource ................................................................................................. 128
11.3.4. SingleConnectionDataSource ................................................................................... 128
11.3.5. DriverManagerDataSource ....................................................................................... 128
11.3.6. TransactionAwareDataSourceProxy .......................................................................... 129
11.3.7. DataSourceTransactionManager ............................................................................... 129
11.4. Modeling JDBC operations as Java objects .......................................................................... 129
11.4.1. SqlQuery ................................................................................................................. 129
11.4.2. MappingSqlQuery ................................................................................................... 130
11.4.3. SqlUpdate ............................................................................................................... 131
11.4.4. StoredProcedure ...................................................................................................... 131
11.4.5. SqlFunction ............................................................................................................. 132
12. Data Access using O/R Mappers .................................................................................................. 133
12.1. Introduction ....................................................................................................................... 133
12.2. Hibernate ........................................................................................................................... 134
12.2.1. Resource management ............................................................................................. 134
12.2.2. SessionFactory setup in a Spring application context .................................................. 135
12.2.3. Inversion of Control: HibernateTemplate and
Spring - Java/J2EE Application Framework
Spring Framework Version 1.2.9 v