RecyclerView 滚动到中间位置实例代码
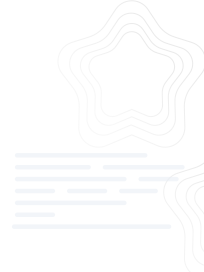

在Android开发中,RecyclerView是一个非常重要的组件,它用于展示可滚动的数据列表,具有高效和灵活的特点。本实例主要探讨如何实现RecyclerView滚动至指定项并使其居中显示,这对于创建诸如瀑布流、轮播效果等场景非常有用。我们将通过以下几个关键步骤来详细讲解这个功能的实现: 1. **设置RecyclerView** 我们需要在布局文件中添加RecyclerView,并在Activity或Fragment中初始化它。创建一个RecyclerView对象,设置布局管理器(通常为LinearLayoutManager或GridLayoutManager)以及适配器,以展示数据。 ```xml <androidx.recyclerview.widget.RecyclerView android:id="@+id/recyclerView" android:layout_width="match_parent" android:layout_height="match_parent" /> ``` ```java RecyclerView recyclerView = findViewById(R.id.recyclerView); recyclerView.setLayoutManager(new LinearLayoutManager(this)); // 或其他布局管理器 recyclerView.setAdapter(adapter); // 你的Adapter ``` 2. **监听点击事件** 为RecyclerView的每个条目设置点击监听器,以便在用户点击时获取选中的项。这可以通过在Adapter中实现或者在ViewHolder中设置点击监听器完成。 ```java // 在Adapter的getViewType方法内 holder.itemView.setOnClickListener(v -> { int position = holder.getAdapterPosition(); scrollToCenter(position); }); ``` 3. **滚动到中间** `scrollToCenter`方法是实现滚动到中间的关键。它接收点击的项的position作为参数,然后计算出需要滚动的距离,最后调用`scrollToPositionWithOffset`来实现滚动。 ```java private void scrollToCenter(int position) { RecyclerView.LayoutManager layoutManager = recyclerView.getLayoutManager(); int totalItemCount = layoutManager.getItemCount(); if (totalItemCount > 0) { int halfVisibleItems = (layoutManager instanceof GridLayoutManager) ? ((GridLayoutManager) layoutManager).getSpanCount() / 2 : 1; // 如果是LinearLayoutManager,只有一半的项可见 int targetPosition = position - halfVisibleItems; if (targetPosition < 0) { targetPosition = 0; } else if (targetPosition >= totalItemCount) { targetPosition = totalItemCount - 1; } int topBound = (int) layoutManager.getChildAt(0).getTop(); // 获取顶部item的顶部边界 int bottomBound = (int) layoutManager.getChildAt(layoutManager.getChildCount() - 1).getBottom(); // 获取底部item的底部边界 int height = recyclerView.getHeight(); int targetTop = layoutManager.getDecoratedTop(layoutManager.findViewByPosition(targetPosition)); int scrollY = (targetTop - (height / 2)) + (topBound - bottomBound) / 2; smoothScrollToPosition(recyclerView, layoutManager, targetPosition, scrollY); } } private void smoothScrollToPosition(RecyclerView recyclerView, RecyclerView.LayoutManager layoutManager, int position, int offset) { if (layoutManager instanceof LinearLayoutManager) { ((LinearLayoutManager) layoutManager).smoothScrollToPosition(recyclerView, null, position, offset); } else if (layoutManager instanceof GridLayoutManager) { ((GridLayoutManager) layoutManager).smoothScrollToPosition(recyclerView, null, position, offset); } } ``` 4. **延迟滚动** 为了在用户点击后4秒内没有其他操作时才滚动到中间,我们可以使用Handler和Runnable来实现这个延迟效果。 ```java private Handler handler = new Handler(); private Runnable scrollToRunnable = () -> scrollToCenter(selectedPosition); public void onItemClickListener(int position) { selectedPosition = position; handler.removeCallbacks(scrollToRunnable); handler.postDelayed(scrollToRunnable, 4000); // 4秒后执行 } @Override protected void onDestroy() { super.onDestroy(); handler.removeCallbacksAndMessages(null); // 防止内存泄漏 } ``` 以上代码实现了RecyclerView在用户点击某项后的延迟滚动功能,使被点击的项在4秒后自动滚动到屏幕中间。注意,这只是一个基础示例,实际项目中可能需要根据具体需求进行调整,例如考虑不同设备的屏幕尺寸、滚动速度等因素。同时,对于复杂布局,可能需要对`scrollToCenter`方法进行优化,以确保滚动到正确的位置。
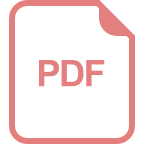
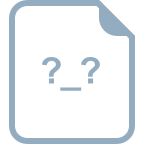
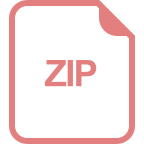
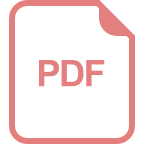
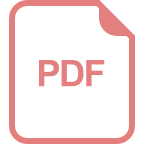
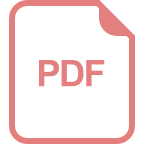
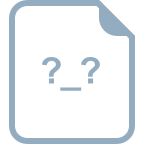
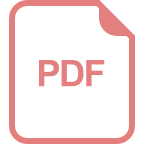
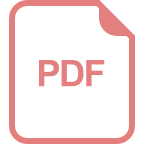
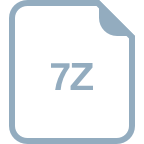
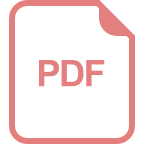
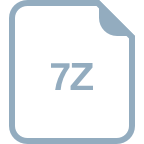
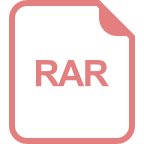
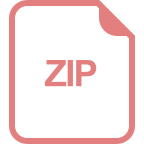
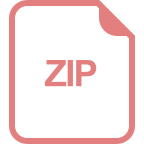
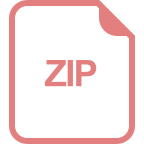
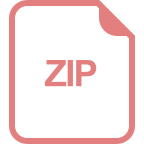

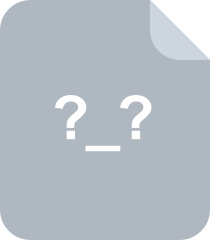
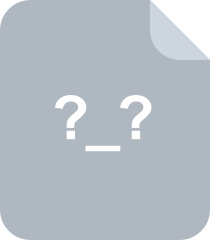
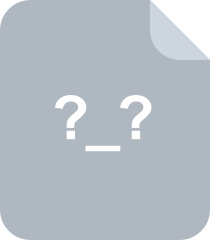
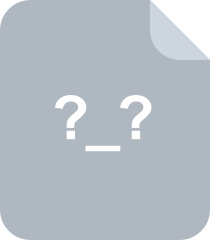
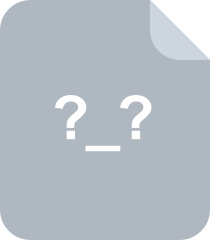
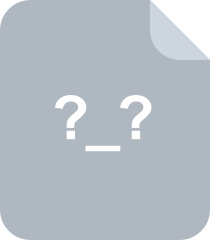
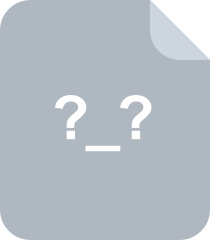
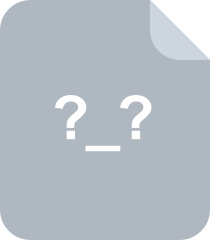
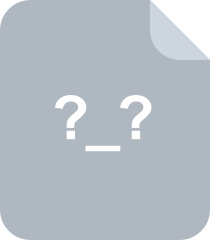
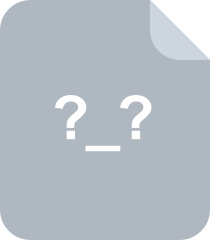
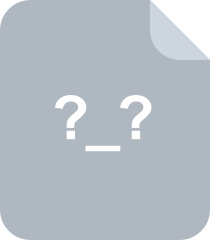
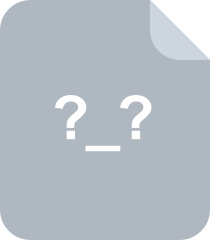
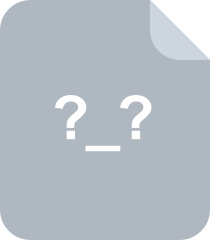
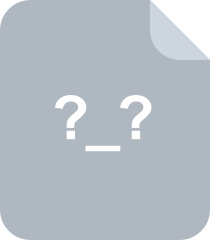
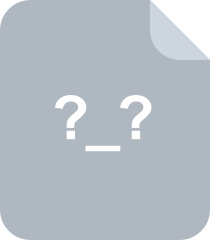
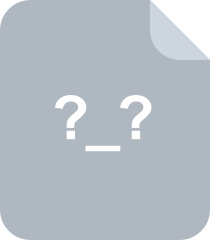
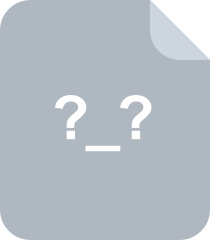
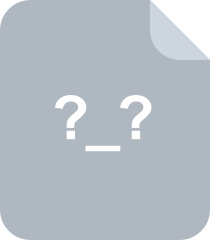
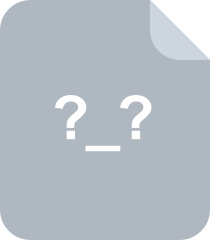
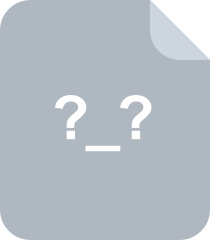
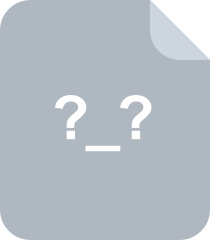
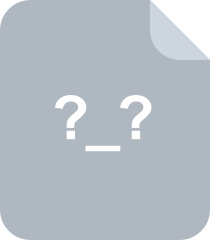
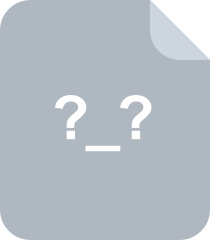
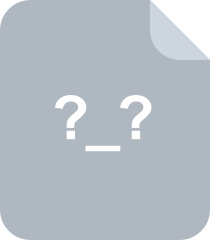
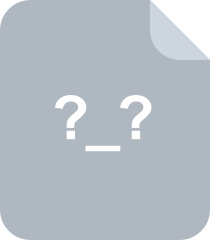
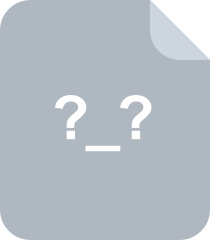
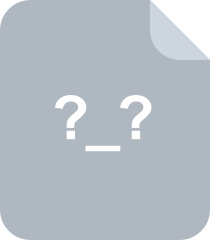
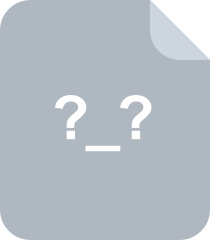
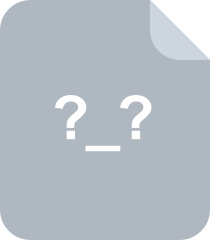
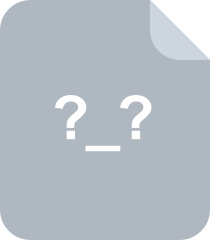
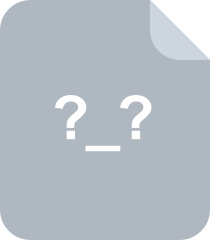
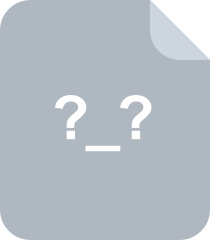
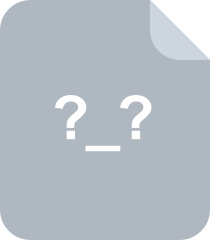
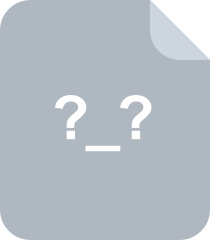
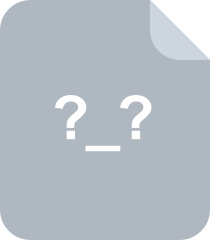
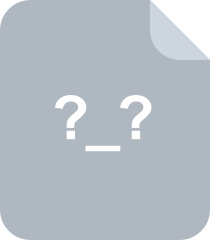
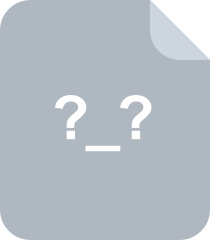
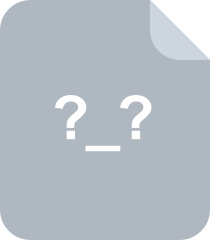
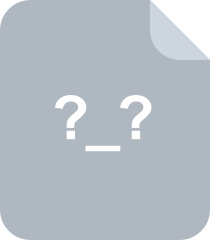
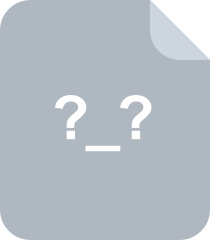
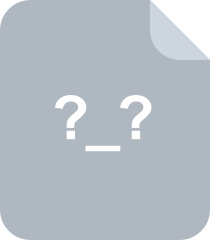
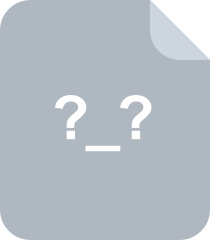
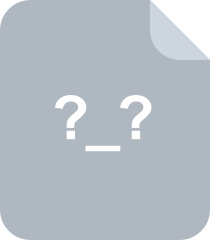
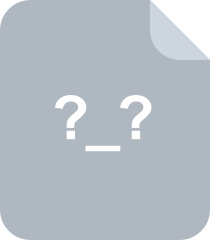
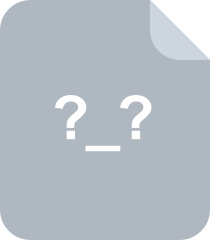
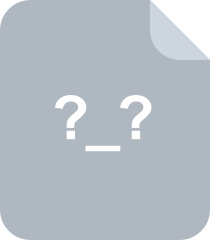
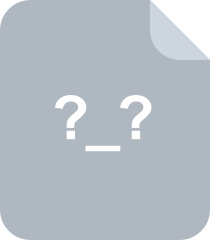
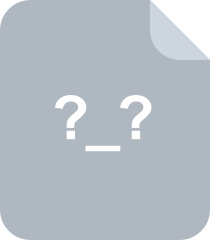
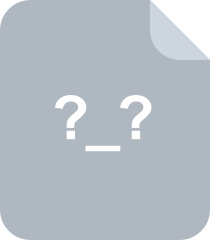
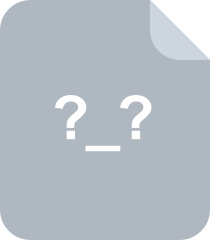
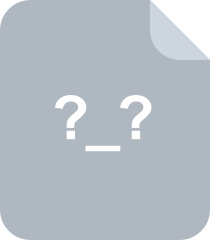
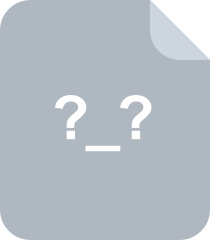
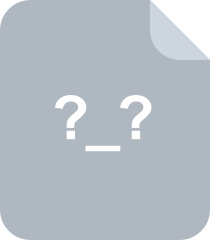
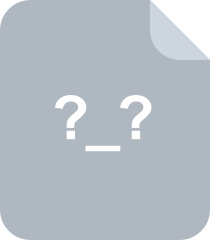
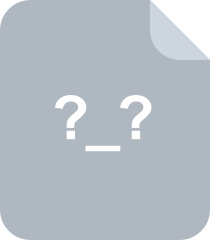
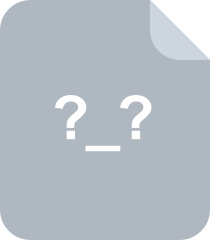
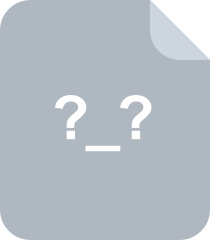
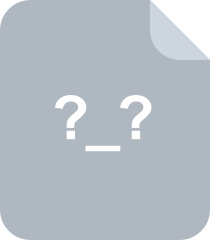
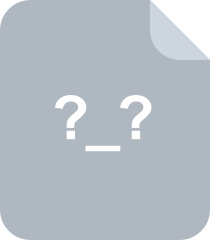
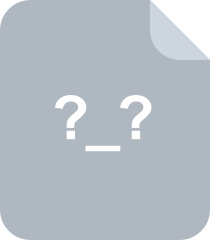
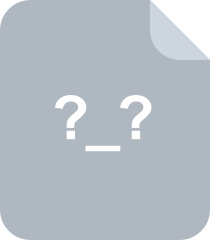
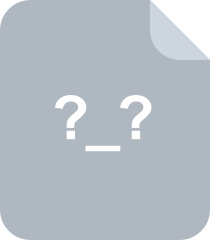
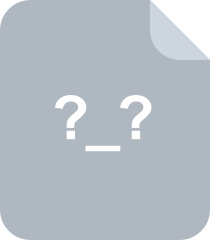
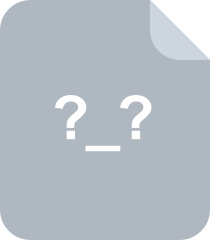
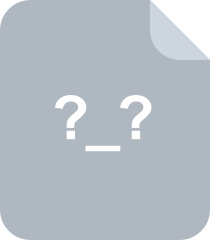
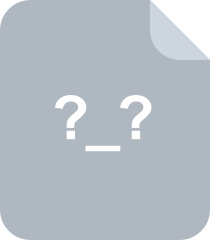
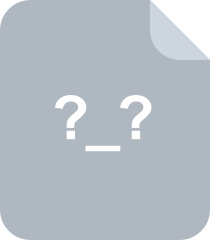
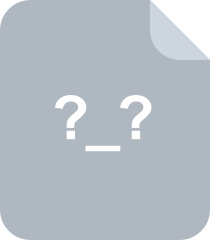
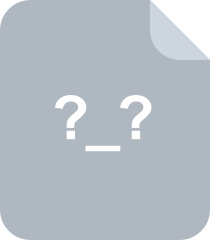
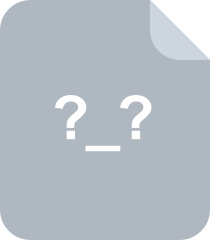
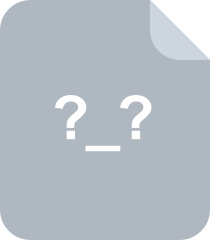
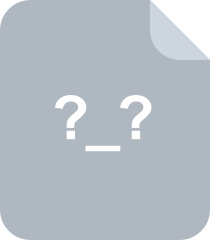
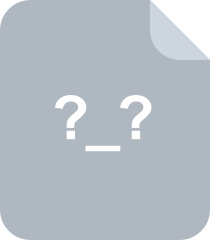
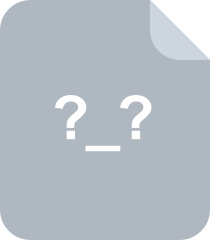
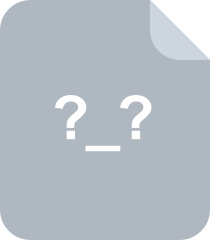
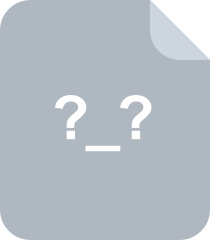
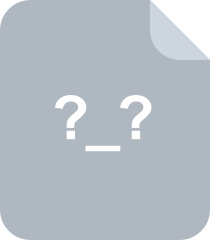
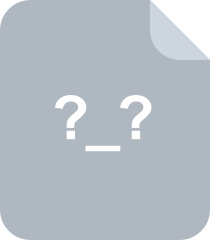
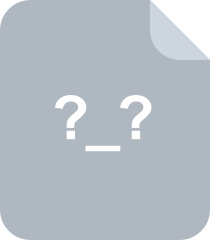
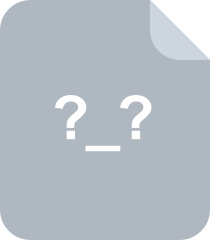
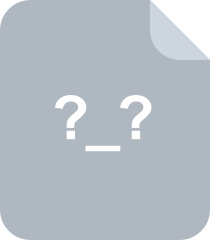
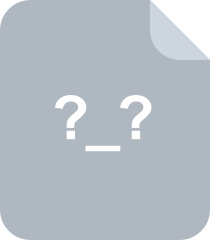
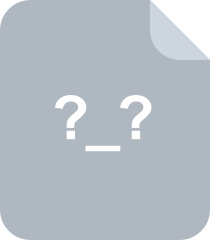
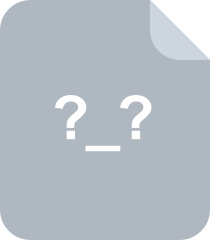
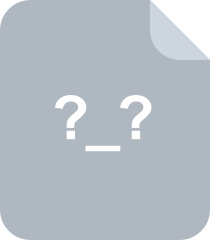
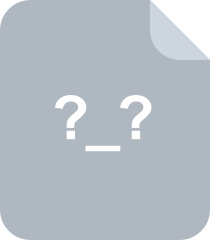
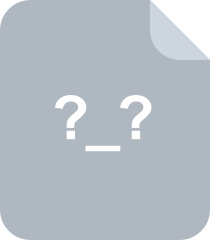
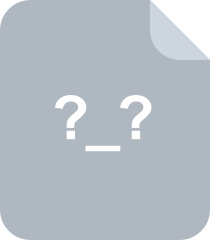
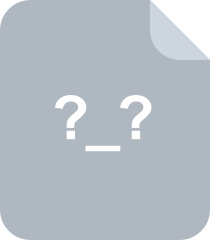
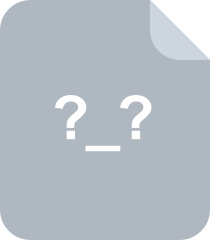
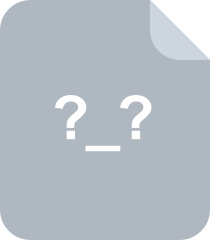
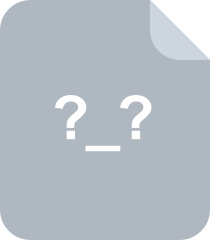
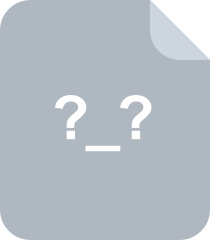
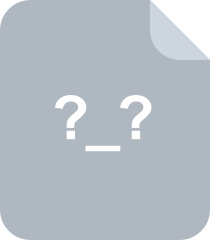
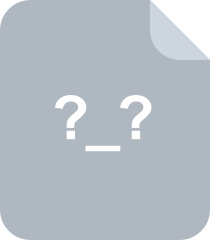
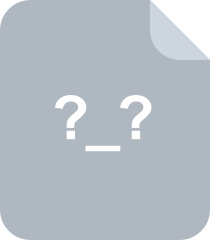
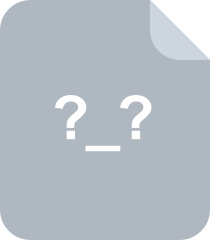
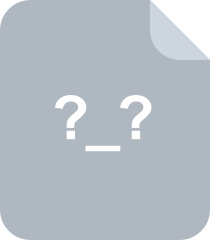
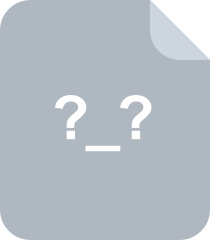
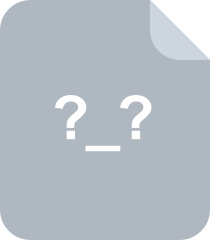
- 1
- 2
- 3
- 4
- 5
- 6
- 16
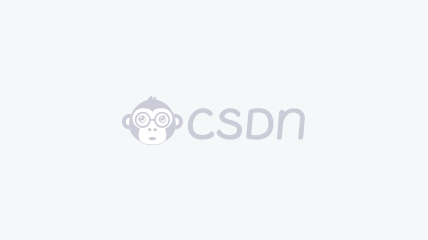

- 粉丝: 2
- 资源: 8
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

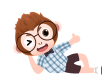
最新资源
- 基于java的图书管理系统+jsp源码(java毕业设计完整源码+LW).zip
- 扫描全能王 2024-12-31 00.01.pdf
- 基于java的物流管理系统设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 工业焊接机器sw18可编辑全套技术资料100%好用.zip
- 基于java的汽车养护管理系统+jsp源码(java毕业设计完整源码+LW).zip
- 12基于java的汽车租赁系统设计新版源码+数据库+说明
- 工件定位小型流水线sw18全套技术资料100%好用.zip
- 基于Java的菜匣子优选系统设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 滚刀式裁切机sw18可编辑+cad全套技术资料100%好用.zip
- 管状输送带sw18可编辑全套技术资料100%好用.zip
- 基于java的安徽新华学院实验中心管理系统的设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 海绵条贴附装置(sw18可编辑+工程图)全套技术资料100%好用.zip
- 基于java的在线云音乐系统的设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 红外线流水线烤箱方案(sw18可编辑+cad)全套技术资料100%好用.zip
- 基于java的龙腾公司员工信息管理系统的设计与实现+jsp源码(java毕业设计完整源码+LW).zip
- 回形传送线sw20可编辑全套技术资料100%好用.zip

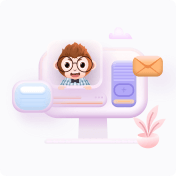
