<?php
/**
* 招募小程序模块小程序接口定义
*
* @author weixinmao
* @url
*/
defined('IN_IA') or exit('Access Denied');
class Weixinmao_zpModuleWxapp extends WeModuleWxapp
{
public function doPageSavepubmsg()
{
global $_GPC, $_W;
$uid = $_GPC['uid'];
$uploadimagelist_str = $_GPC['uploadimagelist_str'];
$imagelistarray = explode('@', $uploadimagelist_str);
foreach ($imagelistarray as $k => $v) {
if ($v != "") {
$imagelist[] = $v;
}
}
$account_data = array('uniacid' => $_W['uniacid'],
'name' => $_GPC['name'],
'tel' => $_GPC['tel'],
'title' => $_GPC['title'],
'content' => $_GPC['content'],
'status' => 0,
'createtime' => TIMESTAMP,
'uid' => $uid,
'piclist' => serialize($imagelist)
);
pdo_insert('weixinmao_zp_pubmsglist', $account_data);
$data = array('error' => 0, 'msg' => '提交成功,请耐心等待审核');
return $this->result(0, 'success', $data);
}
public function doPageGetbanner()
{
global $_GPC, $_W;
//$siteurl = $this->GetSiteUrl();
$list = pdo_fetchall("SELECT * FROM " . tablename('weixinmao_zp_adv') . "WHERE enabled =1 AND weid=:weid ORDER BY displayorder DESC ", array(":weid" => $_W['uniacid']));
if ($list) {
foreach ($list as $k => $v) {
$list[$k]['thumb'] = tomedia($v['thumb']);
}
}
return $this->result(0, 'success', $list);
}
public function doPageUpdatesecret()
{
global $_GPC, $_W;
$uid = $_GPC['uid'];
$tel = $_GPC['tel'];
$code = $_GPC['code'];
$is_code = pdo_fetch("SELECT id FROM " . tablename('weixinmao_zp_mobile_verify_code') . " WHERE uniacid=:uniacid AND verify_code=:code AND mobile=" . $tel, array(":uniacid" => $_W['uniacid'], ':code' => $code));
if (!$is_code) {
$list = array('msg' => '验证码错误', 'error' => 1);
return $this->result(0, 'success', $list);
}
$companyinfo = pdo_get('weixinmao_zp_company', array('uniacid' => $_W['uniacid'], 'tel' => $tel));
if ($companyinfo) {
$account_data = array('uniacid' => $_W['uniacid'],
'password' => md5($_GPC['password'])
);
pdo_update('weixinmao_zp_companyaccount', $account_data, array('companyid' => $companyinfo['id'], 'uniacid' => $_W['uniacid']));
$data = array('msg' => '修改成功', 'error' => 0);
} else {
$data = array('msg' => '机构信息不存在', 'error' => 1);
}
return $this->result(0, 'success', $data);
}
public function doPageUploadvideo()
{
global $_GPC, $_W;
load()->func('file');
$log = json_encode($_FILES);
$res = file_upload($_FILES['file'], 'video');
$data = array(
'weid' => $_W['uniacid'],
//'content'=>json_encode($res)
'content' => $log
);
pdo_insert('weixinmao_house_log', $data);
return $this->result(0, 'success', $res);
}
public function doPageUploaddoc()
{
global $_GPC, $_W;
load()->func('file');
$log = json_encode($_FILES);
$res = file_upload($_FILES['file'], 'file');
$data = array(
'weid' => $_W['uniacid'],
'content' => json_encode($res)
// 'content'=>$log
);
pdo_insert('weixinmao_house_log', $data);
return $this->result(0, 'success', $res);
}
public function doPageSaveFormId()
{
global $_GPC, $_W;
$uid = $_GPC['uid'];
if (!$uid || $uid <= 0) {
return $this->result(1, '用户未授权');
}
$msgdata = array(
'uniacid' => $_W['uniacid'],
'uid' => $uid,
'form_id' => $_GPC['form_id'],
'status' => 0,
'createtime' => TIMESTAMP
);
pdo_insert('weixinmao_zp_msgidlist', $msgdata);
$this->dealMsglist($uid);
$list = array('msg' => '提交成功', 'error' => 0, 'msgcount' => $msgcount);
return $this->result(0, 'success', $list);
}
public function dealMsglist($uid)
{
global $_GPC, $_W;
$uid = $uid;
$msglist = pdo_fetchall("SELECT id,createtime FROM " . tablename('weixinmao_zp_msgidlist') . " WHERE uniacid=:weid AND status = 0 AND uid=" . $uid, array(":weid" => $_W['uniacid']));
$time7 = 60 * 60 * 24 * 7;
foreach ($msglist as $k => $v) {
$currenttime = time() - $v['createtime'];
if ($currenttime >= $time7) {
pdo_delete('weixinmao_zp_msgidlist', array('id' => $v['id']));
}
}
return;
}
public function doPageGetphone()
{
global $_GPC, $_W;
include "inc/wxBizDataCrypt.php";
$iv = $_GPC['iv'];
$encryptedData = $_GPC['encryptedData'];
$appid = $_W['uniaccount']['key'];;//小程序唯一标识 (在微信小程序管理后台获取)
$appsecret = $_W['uniaccount']['secret'];//小程序的 app secret (在微信小程序管理后台获取)
$pc = new WXBizDataCrypt($appid, $_SESSION['session_key']);
$errCode = $pc->decryptData($encryptedData, $iv, $data);
$obj = json_decode($data);
$uid = $_GPC['uid'];
$tel = $obj->phoneNumber;
pdo_update('weixinmao_zp_userinfo', array('tel' => $tel), array('uid' => $uid));
return $this->result(0, 'success', array());
}
public function doPageIntro()
{
global $_GPC, $_W;
//$siteurl = $this->GetSiteUrl();
$list = pdo_fetch("SELECT * FROM " . tablename('weixinmao_zp_intro') . " WHERE uniacid=:uniacid", array(":uniacid" => $_W['uniacid']));
$list['logo'] = tomedia($list['logo']);
$list['description'] = html_entity_decode($list['content']);
$list['content'] = trim(html_entity_decode(strip_tags($list['content'])), chr(0xc2) . chr(0xa0));
$map = $this->Convert_BD09_To_GCJ02($list['lat'], $list['lng']);
$list['lat'] = $map['lat'];
$list['lng'] = $map['lng'];
$list['intro'] = $list;
return $this->result(0, 'success', $list);
}
public function doPageUserInit()
{
global $_GPC, $_W;
//$siteurl = $this->GetSiteUrl();
$list = pdo_fetch("SELECT * FROM " . tablename('weixinmao_zp_intro') . " WHERE uniacid=:uniacid", array(":uniacid" => $_W['uniacid']));
$list['logo'] = tomedia($list['logo']);
$list['description'] = html_entity_decode($list['content']);
$list['content'] = trim(html_entity_decode(strip_tags($list['content'])), chr(0xc2) . chr(0xa0));
$map = $this->Convert_BD09_To_GCJ02($list['lat'], $list['lng']);
$list['lat'] = $map['lat'];
$list['lng'] = $map['lng'];
//$list['intro'] = $list;
if ($list['ischeck'] == 0) {
$title = array('简历编辑', '我的接单', '任务匹配', '分享收益', '推荐简历有奖', '接单通知', '机构邀请', '我的颜值', '我的直推', '购买VIP套餐', '经纪人中心', '刷新简历');
} else {
$title = array();
}
$data = array('intro' => $list, 'title' => $title);
return $this->result(0, 'success', $data);
}
public function doPagePubmsgInit()
{
global $_GPC, $_W;
$list = pdo_fetch("SELECT * FROM " . tablename('weixinmao_zp_in
没有合适的资源?快使用搜索试试~ 我知道了~
招聘求职小程序V4.1.87源码 类似58同城、前程无忧、智联招聘等招聘类小程序模块
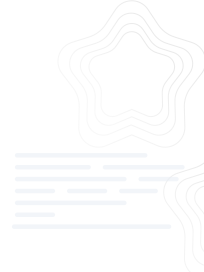
共632个文件
js:134个
wxss:108个
wxml:107个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 144 浏览量
2022-07-02
22:25:15
上传
评论
收藏 1.42MB ZIP 举报
温馨提示
简介: 测试环境:系统环境:CentOS Linux 7.6.1810 (Core) 运行环境:宝塔 Linux v7.0.3(专业版) 网站环境:Nginx 1.15.10 + MySQL 5.6.46 + PHP-7.1 / PHP-5.6 常见插件:ionCube ;Fileinfo ; redis ; Swoole ; sg11 求职招聘小程序是一款类似58同城、前程无忧、智联招聘等招聘类小程序模块。 支持功能 : 1、职位版块 2、人才版块 3、招聘会(支持企业在线报名参加招聘会) 4、职场资讯 5、企业登录(在手机端可操作企业信息编辑、发布职位、查收简历、通知面试) 6、会员中心(在手机端 投送简历、收藏职位、填写简历、面试通知、刷新简历等功能) 7、查看简历收费 8、消息通知 9、企业购买查看简历次数(支持企业初次注册送企业查看次数功能) 重新更新功能通知: 1、三级分销上线啦,申请经纪人帮平台招人、企业入驻即可获取佣金! 2、企业推广职位二维码上线啦,企业分享职位用户投简历可获取查看职位次数! 3、颜值招聘功能上线啦,为企业提供有形象
资源推荐
资源详情
资源评论
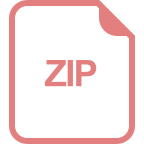
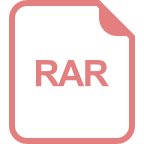
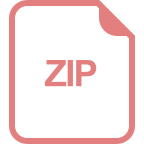
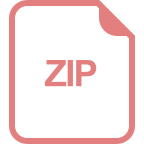
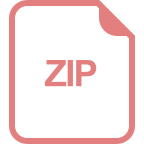
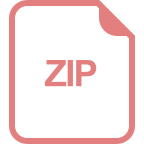
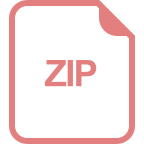
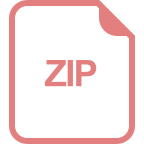
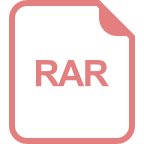
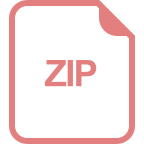
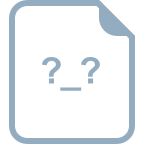
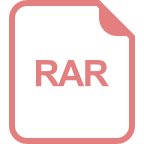
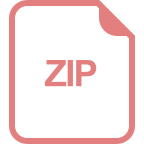
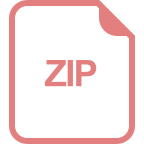
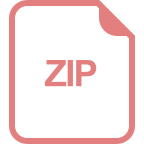
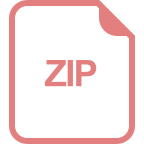
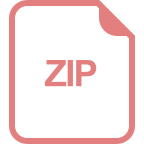
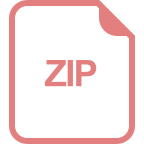
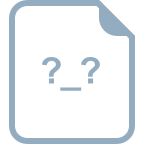
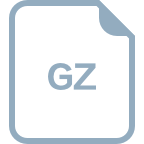
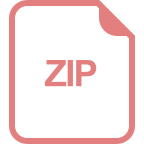
收起资源包目录

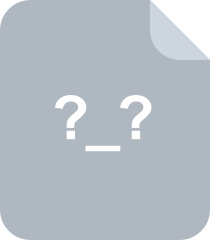
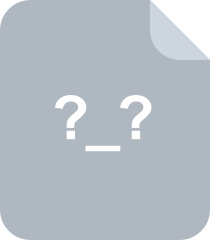
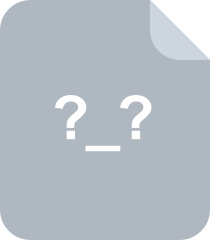
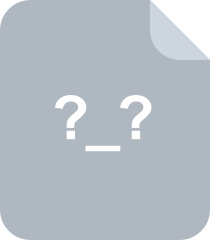
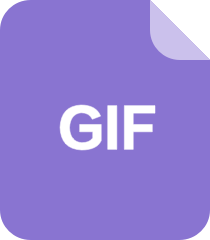
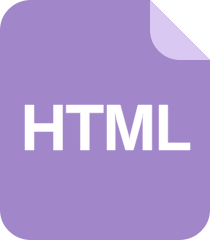
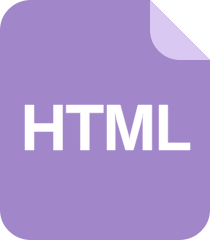
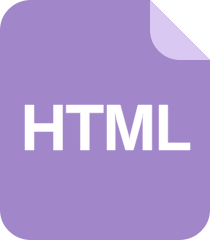
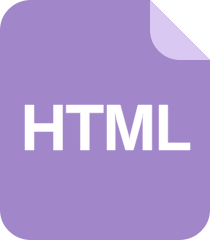
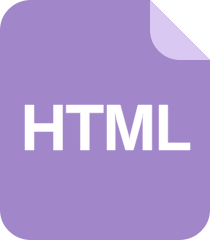
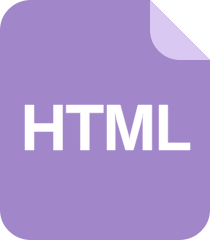
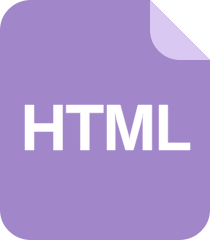
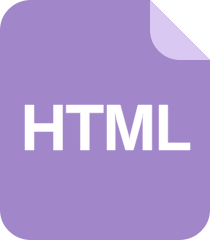
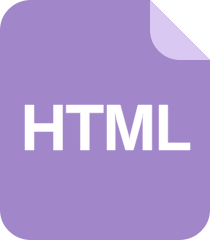
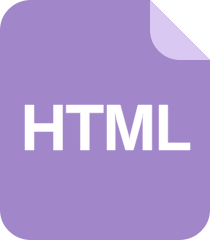
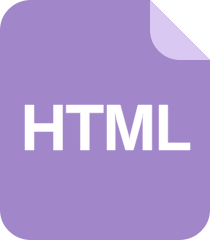
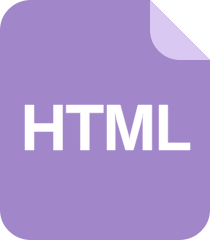
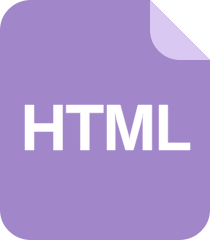
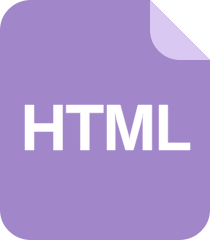
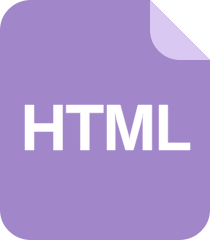
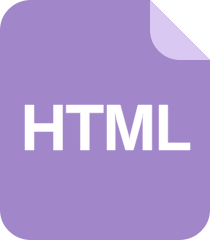
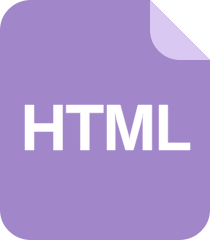
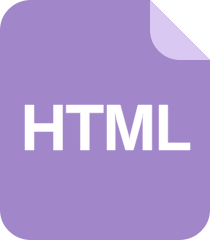
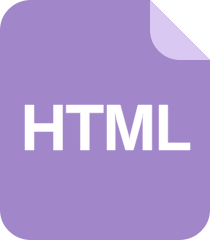
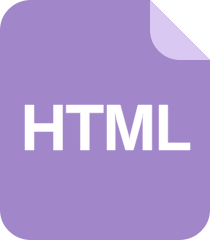
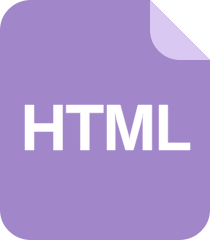
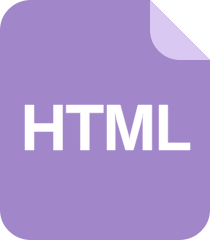
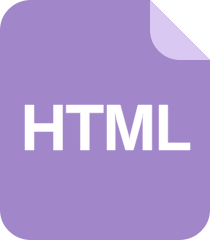
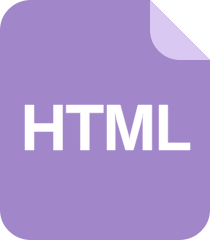
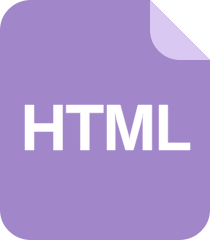
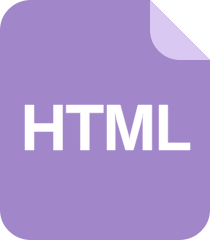
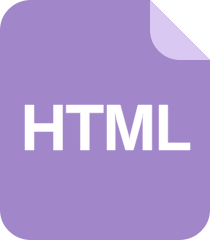
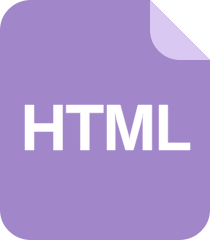
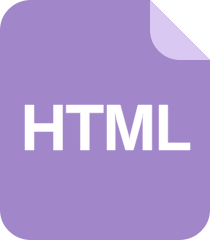
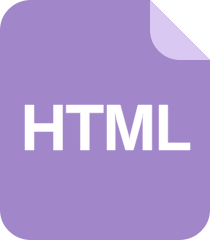
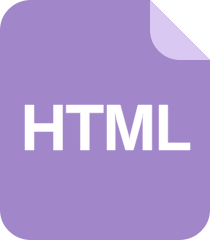
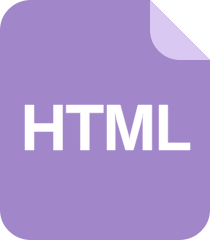
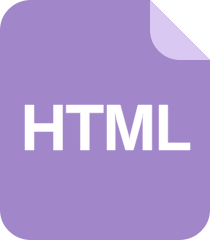
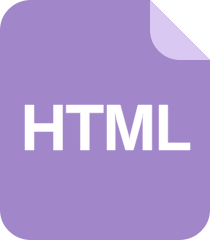
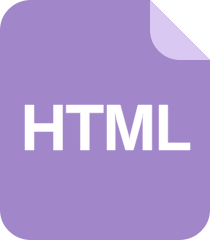
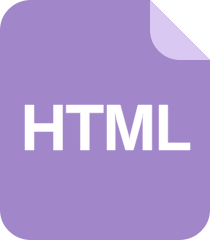
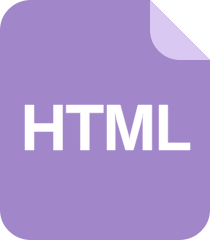
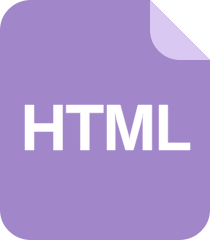
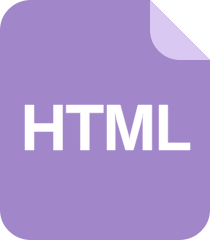
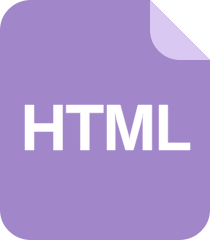
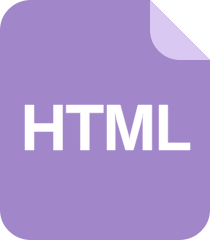
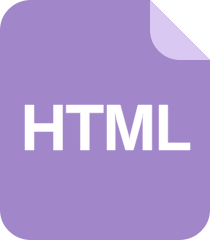
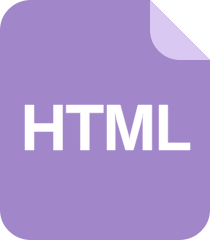
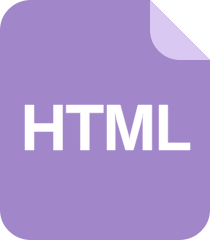
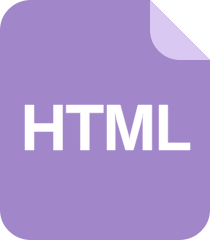
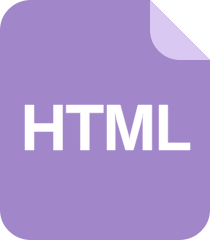
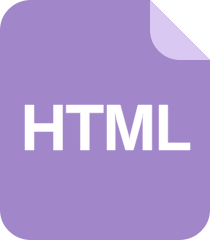
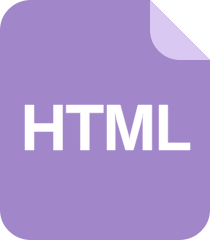
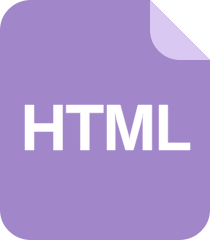
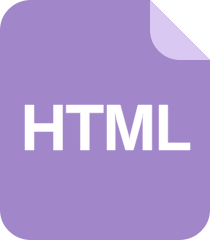
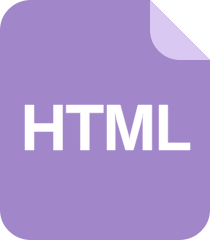
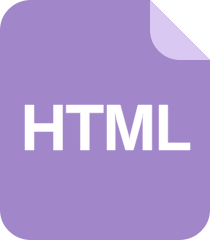
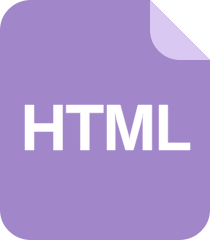
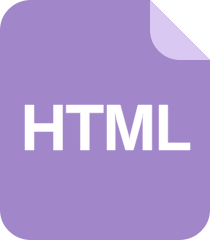
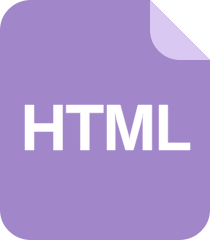
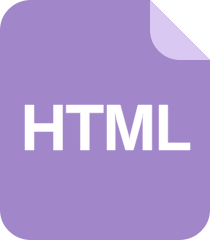
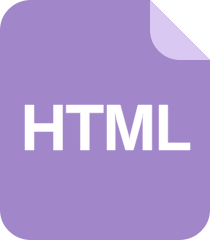
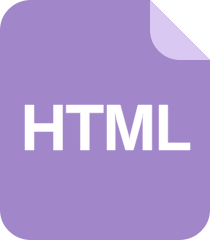
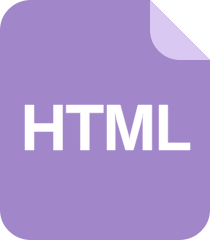
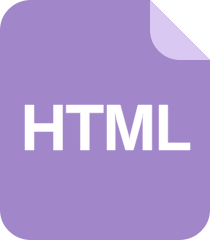
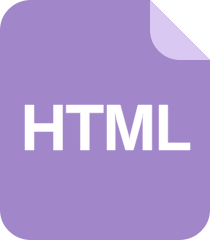
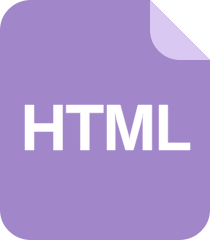
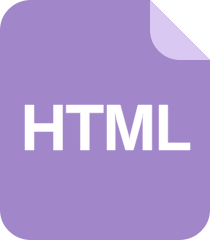
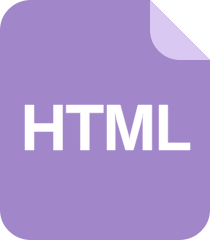
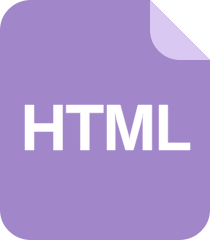
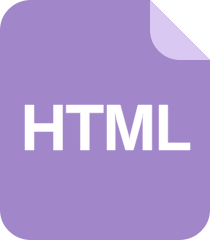
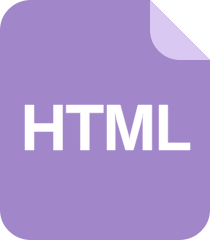
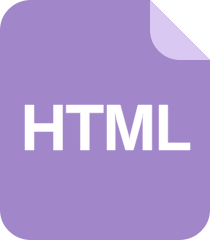
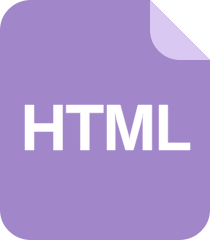
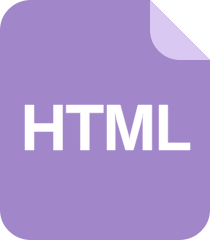
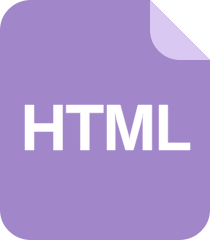
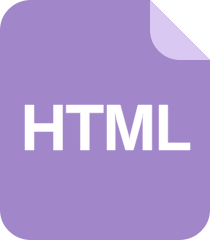
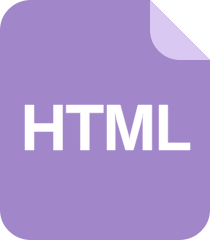
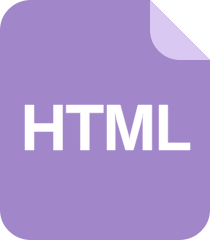
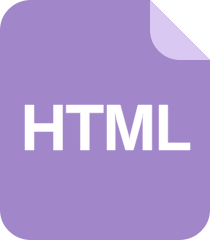
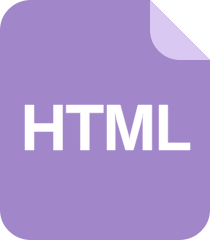
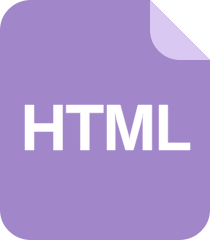
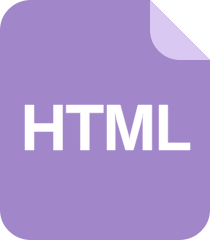
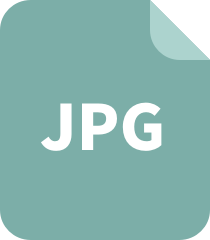
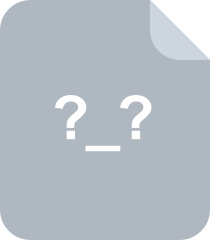
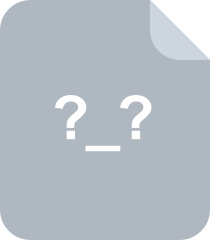
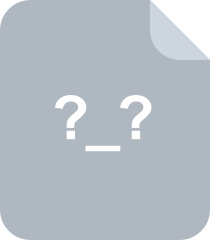
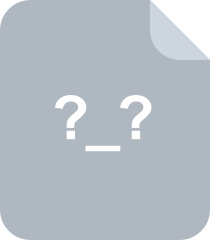
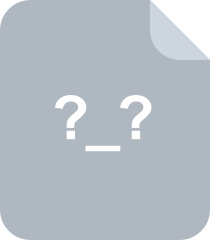
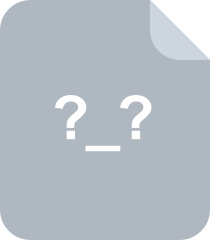
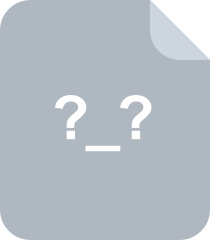
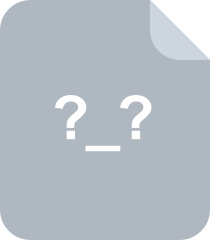
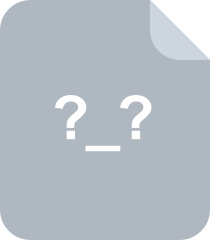
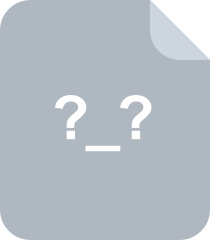
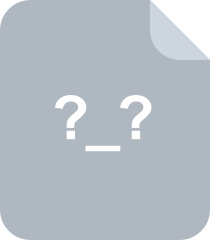
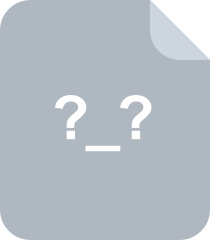
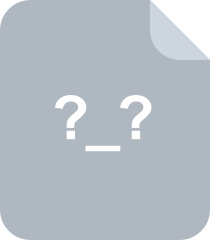
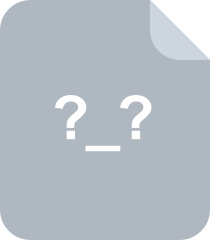
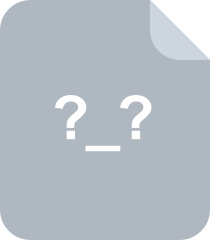
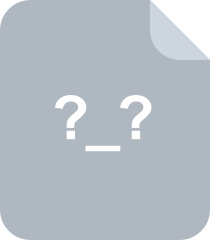
共 632 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
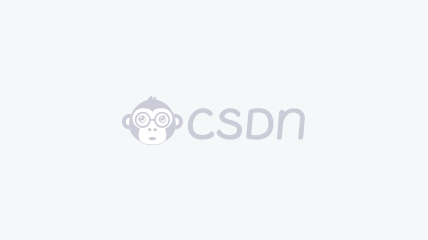

阿国下载
- 粉丝: 620
- 资源: 176
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

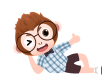
最新资源
- YOLO图片标注xml转txt代码
- 安卓壳可以用于大屏开机打开网址
- paddlepaddle-gpu-2.5.2-cp38-cp38-win-amd64.whl
- Babel Street Analytics Java 客户端库.zip
- 图像处理中的White Patch算法来实现白平衡,MATLAB实现
- 在android studio 中使用jni来进行编程
- 开机自动启动VMWARE workstation16虚拟机
- Python 爬虫:把廖雪峰的教程转换成 PDF 电子书
- 2024 年 Java 开发人员路线图.zip
- matplotlib-3.7.5-cp38-cp38-win-amd64.whl
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


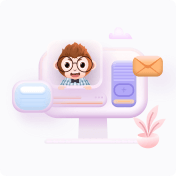
安全验证
文档复制为VIP权益,开通VIP直接复制
