/*------------------------------------------------------------
HELLOWIN.C -- Displays "Hello, Windows 98!" in client area
(c) Charles Petzold, 1998
------------------------------------------------------------*/
#include <windows.h>
#include <stdlib.h>
#include <time.h>
#include <tchar.h>
#include <math.h>
#include "WinMain.h"
#include "resource.h"
#pragma comment( lib,"winmm.lib")
LRESULT CALLBACK WndProc (HWND, UINT, WPARAM, LPARAM) ;
BOOL CALLBACK WinDlgProc(HWND hDlg, UINT message, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain (HINSTANCE hInstance, HINSTANCE hPrevInstance,
PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT ("Mine sweeper") ;
HWND hwnd ;
MSG msg ;
WNDCLASS wndclass ;
wndclass.style = NULL;
wndclass.lpfnWndProc = WndProc ;
wndclass.cbClsExtra = 0 ;
wndclass.cbWndExtra = 0 ;
wndclass.hInstance = hInstance ;
wndclass.hIcon = LoadIcon (hInstance,MAKEINTRESOURCE(IDI_MINE)) ;
wndclass.hCursor = LoadCursor (NULL, IDC_ARROW) ;
wndclass.hbrBackground = (HBRUSH) GetStockObject (WHITE_BRUSH) ;
wndclass.lpszMenuName = MAKEINTRESOURCE(IDR_MENU);
wndclass.lpszClassName = szAppName ;
if (!RegisterClass (&wndclass))
{
MessageBox (NULL, TEXT ("This program requires Windows NT!"),
szAppName, MB_ICONERROR) ;
return 0 ;
}
UINT uXPos=GetSystemMetrics(SM_CXSCREEN)/2-200;
UINT uYPos=GetSystemMetrics(SM_CYSCREEN)/2-200;
hwnd = CreateWindow (szAppName, // window class name
TEXT ("扫雷"), // window caption
WS_SYSMENU | WS_CAPTION | WS_MINIMIZEBOX, // window style
uXPos, // initial x position
uYPos, // initial y position
CW_USEDEFAULT, // initial x size
CW_USEDEFAULT, // initial y size
NULL, // parent window handle
NULL, // window menu handle
hInstance, // program instance handle
NULL) ; // creation parameters
ShowWindow (hwnd, iCmdShow) ;
//UpdateWindow (hwnd) ;
while (GetMessage (&msg, NULL, 0, 0))
{
TranslateMessage (&msg) ;
DispatchMessage (&msg) ;
}
return msg.wParam ;
}
LRESULT CALLBACK WndProc (HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
static HBITMAP hbmpMine;
static HBITMAP hbmpButton;
static HBITMAP hbmpNumber;
static cxClient,cyClient;
static cxBitMap; //cyBitMap;
static RECT rcClient;
static int iBtnRectLeft,iBtnRectTop=16; //存放笑脸按钮左上角的坐标
static CButtonState strButton_State;
static UINT uNumRectLeft; //时间一栏左边的坐标
static CMineArray minearray[100][100];
static UINT uGameState; //游戏状态,0为等待,1为运行,2失败,3胜利
static int iRow,iCol; //游戏雷区的行数、列数
static int oldrow; //存放LBUTTON_DOWN的历史位置
static int oldcol;
static StrLRDownFlag strLRDownFlag;
static int iRemainBrick; //剩余的(没有被翻开的)方砖的数量
static BOOL ClickInButton; //标记是LButton时否在笑脸内部
static BOOL fLRBtnDown; //标记是否L_R_ButtonDown
static int iSpendTime; //用时
static int iLeaveMineNum; //剩下的雷
static bool bMarkful;
static bool bColorful;
static bool bSoundful;
static HINSTANCE hInstance;
static void* pSndDead=NULL; // 失败提示音
static void* pSndVictory=NULL; // 胜利提示音
static void* pSndClock=NULL; // 时钟提示音
HBITMAP hbitmap;
HDC hdc,hdcMem;
PAINTSTRUCT ps ;
switch (message)
{
case WM_CREATE:
{
hInstance=((LPCREATESTRUCT) lParam)->hInstance;
/*********初始化游戏**********/
LoadConfig(uColMax,
uRowMax,
uMineNum,
uLevel,
uPrimary,
uSecond,
uAdvance,
szPrimary,
szSecond,
szAdvance,
bMarkful,
bColorful,
bSoundful);
LoadBitmap(hInstance,hbmpMine,hbmpButton,hbmpNumber,bColorful);
InitGame(uColMax,
uRowMax,
uMineNum,
iRemainBrick,
minearray,
uGameState,
strButton_State,
fLRBtnDown,
iSpendTime,
iLeaveMineNum);
HMENU hMenu=GetMenu(hwnd);
SetCheckedLevel(hMenu,uLevel);
SetCheckedMark(hMenu,bMarkful);
SetCheckedColor(hMenu,bColorful);
SetCheckedSound(hMenu,bSoundful);
if (bSoundful)
{
LoadWaveSrc(pSndDead,pSndVictory,pSndClock);
}
}
return 0;
case WM_SHOWWINDOW:
{
UINT uWidth = LINE_WIDTH+SHELL_L_START_X*2+4+MINE_WIDTH*uColMax;
UINT uHeight = MINEAREA_UP+MINE_HEIGHT*uRowMax+60;
SetWindowPos(hwnd,HWND_TOPMOST, 0, 0,uWidth ,uHeight,
SWP_NOZORDER | SWP_NOMOVE | SWP_NOCOPYBITS );
/****************得到ClientWindow的坐标******************/
GetClientRect(hwnd,&rcClient);
iBtnRectLeft=rcClient.right/2-12; //笑脸的左边的坐标
uNumRectLeft=rcClient.right-56; //计算显示时间一栏左边的坐标
}
return 0;
case WM_SIZE:
cxClient= LOWORD(lParam);
cyClient= HIWORD(lParam);
return 0;
case WM_PAINT: //先把窗口的重画写好
hdc=BeginPaint(hwnd,&ps);
/************产生ClientDc兼容的内存,并装入Client大小的位图**********/
hdcMem=CreateCompatibleDC(hdc);
hbitmap=CreateCompatibleBitmap(hdc,rcClient.right,rcClient.bottom);
SelectObject(hdcMem,hbitmap);
/*****************用灰色填充ClientWindow******************/
FillSolidRect(hdcMem,&rcClient,COLOR_GRAY);
/*****************显示笑脸按钮************************/
DrawButton(hdcMem,iBtnRectLeft,iBtnRectTop,24,24,hbmpButton,0,24*strButton_State.uState);
/****************显示数字*********************/
DrawNumber(hdcMem,uNumRectLeft,hbmpNumber,iSpendTime,iLeaveMineNum);
/*****************绘制两个3d外壳*****************/
DrawShell(hdcMem,rcClient,uColMax,uRowMax);
/********************贴图(把雷区贴满图片)*****************/
DrawMineArea( hdcMem,hbmpMine,uColMax,uRowMax,minearray);
/********************将hdcMem顶到显示器********************/
BitBlt(hdc,0,0,rcClient.right,rcClient.bottom,hdcMem,0,0,SRCCOPY);
DeleteObject(hbitmap);
DeleteDC(hdcMem);
ReleaseDC(hwnd,hdc);
EndPaint(hwnd,&ps);
return 0 ;
case WM_MOUSEMOVE:
{
int xPos = LOWORD(lParam);
int yPos = HIWORD(lParam);
/*************************************************************/
/***************************左键按下**************************/
/*************************************************************/
if (MK_LBUTTON==wParam)
{
/*************************笑脸的处理**********************************/
/******笑脸区域RECT********/
RECT rect;
rect.left=iBtnRectLeft;
rect.top=iBtnRectTop;
rect.right=iBtnRectLeft+BUTTON_WIDTH;
rect.bottom=iBtnRectTop+BUTTON_HEIGHT;
if (xPos>=iBtnRectLeft && xPos<=iBtnRectLeft+BUTTON_WIDTH && yPos>=iBtnRectTop && yPos<=iBtnRectTop+BUTTON_HEIGHT)
{
if (TRUE==ClickInButton)
{
strButton_State.uState=BUTTON_DOWN;
InvalidateRect(hwnd,&rect,FALSE);
}
}
else
{
if (FALSE==ClickInButton) //确保第一次在笑脸外点击
{
if (GAME_WAITE==uGameState || GAME_RUN==uGameState)
{
strButton_State.uState=BUTTON_CLICK;
InvalidateRect(hwnd,&rect,FALSE);
}
}
else //确保第一次在笑脸内部点击
{
strButton_State.uState=strButton_State.uOldState;
InvalidateRect(hwnd,&rect,FALSE);
}
}
/**************************笑脸区域处理完毕********************************/
/***************************雷区处的处理*******************************/
if (GAME_WAITE==uGameState || GAME_RUN==uGameState)
{
if(FALSE==ClickInButton && FALSE==fLRBtnDown)
OnLButtonDownInMineArea(hwnd,uGameS

suyongsheng
- 粉丝: 2
- 资源: 6
最新资源
- Qu120钢轨的CO2气体保护焊焊接.pdf
- RCC-M2007版与2000版+2002补遗对核级设备焊接过程中热输入要求及差异分析 - .pdf
- QXL锅炉连梁焊接变形的控制与火焰矫正 - .pdf
- RMD焊接工艺隧道管道安装中质量的防控措施.pdf
- RCC-M中的焊接材料评定.pdf
- S31803双相不锈钢球罐制造及焊接技术 - .pdf
- S31803双相不锈钢对接接头焊接工艺参数研究.pdf
- S30408等离子焊接接头组织与性能分析 - .pdf
- S450EW新型耐候钢焊接工艺与低温韧性研究 - .pdf
- S30408不锈钢活性焊接接头微观组织及性能研究.pdf
- S31008(06Cr25Ni20)耐热不锈钢的焊接工艺.pdf
- SA203 Cr.E 的气体容器的焊接工艺评定.pdf
- SA203Gr.D低温钢多道焊焊接性能试验研究.pdf
- SA213-T9合金耐热钢焊接技术.pdf
- SA-204Gr.C的焊接性能与金相组织.pdf
- SA-213T12换热管与SA-387Gr.11CL2管板内孔对接焊接工艺研究.pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


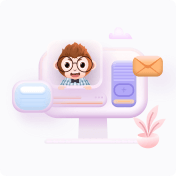