Crypto++: a C++ Class Library of Cryptographic Schemes
Version 5.5.2 (9/24/2007)
Crypto++ Library is a free C++ class library of cryptographic schemes.
Currently the library contains the following algorithms:
algorithm type name
high speed stream ciphers Panama, Salsa20, Sosemanuk
AES and AES candidates AES (Rijndael), RC6, MARS, Twofish, Serpent,
CAST-256
IDEA, Triple-DES (DES-EDE2 and DES-EDE3),
other block ciphers Camellia, RC5, Blowfish, TEA, XTEA,
Skipjack, SHACAL-2
block cipher modes of operation ECB, CBC, CBC ciphertext stealing (CTS),
CFB, OFB, counter mode (CTR)
message authentication codes VMAC, HMAC, CBC-MAC, DMAC, Two-Track-MAC
SHA-1, SHA-2 (SHA-224, SHA-256, SHA-384, and
hash functions SHA-512), Tiger, WHIRLPOOL, RIPEMD-128,
RIPEMD-256, RIPEMD-160, RIPEMD-320
RSA, DSA, ElGamal, Nyberg-Rueppel (NR),
public-key cryptography Rabin, Rabin-Williams (RW), LUC, LUCELG,
DLIES (variants of DHAES), ESIGN
padding schemes for public-key PKCS#1 v2.0, OAEP, PSS, PSSR, IEEE P1363
systems EMSA2 and EMSA5
Diffie-Hellman (DH), Unified Diffie-Hellman
key agreement schemes (DH2), Menezes-Qu-Vanstone (MQV), LUCDIF,
XTR-DH
elliptic curve cryptography ECDSA, ECNR, ECIES, ECDH, ECMQV
insecure or obsolescent MD2, MD4, MD5, Panama Hash, DES, ARC4, SEAL
algorithms retained for backwards 3.0, WAKE, WAKE-OFB, DESX (DES-XEX3), RC2,
compatibility and historical SAFER, 3-WAY, GOST, SHARK, CAST-128, Square
value
Other features include:
* pseudo random number generators (PRNG): ANSI X9.17 appendix C, RandomPool
* password based key derivation functions: PBKDF1 and PBKDF2 from PKCS #5,
PBKDF from PKCS #12 appendix B
* Shamir's secret sharing scheme and Rabin's information dispersal algorithm
(IDA)
* fast multi-precision integer (bignum) and polynomial operations
* finite field arithmetics, including GF(p) and GF(2^n)
* prime number generation and verification
* useful non-cryptographic algorithms
+ DEFLATE (RFC 1951) compression/decompression with gzip (RFC 1952) and
zlib (RFC 1950) format support
+ hex, base-32, and base-64 coding/decoding
+ 32-bit CRC and Adler32 checksum
* class wrappers for these operating system features (optional):
+ high resolution timers on Windows, Unix, and Mac OS
+ Berkeley and Windows style sockets
+ Windows named pipes
+ /dev/random, /dev/urandom, /dev/srandom
+ Microsoft's CryptGenRandom on Windows
* A high level interface for most of the above, using a filter/pipeline
metaphor
* benchmarks and validation testing
* x86, x86-64 (x64), MMX, and SSE2 assembly code for the most commonly used
algorithms, with run-time CPU feature detection and code selection
* some versions are available in FIPS 140-2 validated form
You are welcome to use it for any purpose without paying me, but see
License.txt for the fine print.
The following compilers are supported for this release. Please visit
http://www.cryptopp.com the most up to date build instructions and porting notes.
* MSVC 6.0 - 2008
* GCC 3.3 - 4.2
* Borland C++Builder 2006 - 2007
* Intel C++ Compiler 9.1 - 10.0
* Sun Studio 11 - 12 (CC 5.8 - 5.9)
*** Important Usage Notes ***
1. If a constructor for A takes a pointer to an object B (except primitive
types such as int and char), then A owns B and will delete B at A's
destruction. If a constructor for A takes a reference to an object B,
then the caller retains ownership of B and should not destroy it until
A no longer needs it.
2. Crypto++ is thread safe at the class level. This means you can use
Crypto++ safely in a multithreaded application, but you must provide
synchronization when multiple threads access a common Crypto++ object.
*** MSVC-Specific Information ***
On Windows, Crypto++ can be compiled into 3 forms: a static library
including all algorithms, a DLL with only FIPS Approved algorithms, and
a static library with only algorithms not in the DLL.
(FIPS Approved means Approved according to the FIPS 140-2 standard.)
The DLL may be used by itself, or it may be used together with the second
form of the static library. MSVC project files are included to build
all three forms, and sample applications using each of the three forms
are also included.
To compile Crypto++ with MSVC, open the "cryptest.dsw" (for MSVC 6 and MSVC .NET
2003) or "cryptest.sln" (for MSVC .NET 2005) workspace file and build one or
more of the following projects:
cryptdll - This builds the DLL. Please note that if you wish to use Crypto++
as a FIPS validated module, you must use a pre-built DLL that has undergone
the FIPS validation process instead of building your own.
dlltest - This builds a sample application that only uses the DLL.
cryptest Non-DLL-Import Configuration - This builds the full static library
along with a full test driver.
cryptest DLL-Import Configuration - This builds a static library containing
only algorithms not in the DLL, along with a full test driver that uses
both the DLL and the static library.
To use the Crypto++ DLL in your application, #include "dll.h" before including
any other Crypto++ header files, and place the DLL in the same directory as
your .exe file. dll.h includes the line #pragma comment(lib, "cryptopp")
so you don't have to explicitly list the import library in your project
settings. To use a static library form of Crypto++, specify it as
an additional library to link with in your project settings.
In either case you should check the compiler options to
make sure that the library and your application are using the same C++
run-time libraries and calling conventions.
*** DLL Memory Management ***
Because it's possible for the Crypto++ DLL to delete objects allocated
by the calling application, they must use the same C++ memory heap. Three
methods are provided to achieve this.
1. The calling application can tell Crypto++ what heap to use. This method
is required when the calling application uses a non-standard heap.
2. Crypto++ can tell the calling application what heap to use. This method
is required when the calling application uses a statically linked C++ Run
Time Library. (Method 1 does not work in this case because the Crypto++ DLL
is initialized before the calling application's heap is initialized.)
3. Crypto++ can automatically use the heap provided by the calling application's
dynamically linked C++ Run Time Library. The calling application must
make sure that the dynamically linked C++ Run Time Library is initialized
before Crypto++ is loaded. (At this time it is not clear if it is possible
to control the order in which DLLs are initialized on Windows 9x machines,
so it might be best to avoid using this method.)
When Crypto++ attaches to a new process, it searches all modules loaded
into the process space for exported functions "GetNewAndDeleteForCryptoPP"
and "SetNewAndDeleteFromCryptoPP". If one of these functions is found,
Crypto++ uses methods 1 or 2, respectively, by calling the function.
Otherwise, method 3 is used.
*** GCC-Specific Information ***
A makefile is included for you to compile Crypto++ with GCC. Make sure
you are using GNU Make and GNU ld. The make process will produce two files,
libcryptopp.a and cryptest.exe. Run "c
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
提供处理密码,消息验证,单向hash,公匙加密系统等功能的免费库。<br>Crypto++ 是一个非常专业的C++ 密码学函式库,几乎在密码学里头常见的演算法都可以在Crypto++ <br>找到实作的函式,如:block 与stream ciphers,hash functions,MACs,random number generators,<br>public key 加密...等方法<br><br>vc71环境中编译安装<br> 版本:cryptopp552.zip<br><br>直接通过 cryptest.dsw 相关的库<br><br>头文件在 %cryptopp_root%<br>库文件在 %cryptopp_root%/lib<br><br>头文件添加方法如:<br>#include 需要链接lib库
资源推荐
资源详情
资源评论
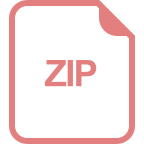
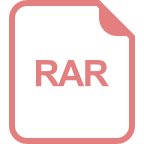
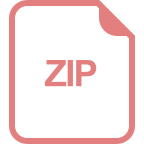
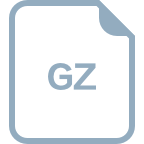
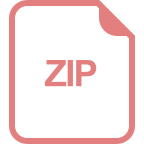
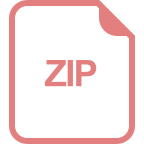
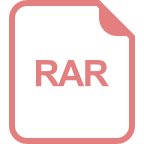
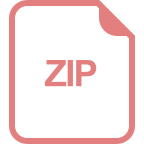
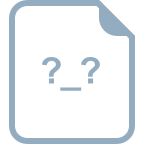
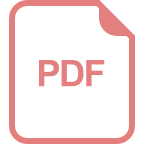
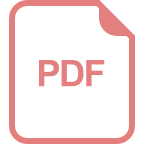
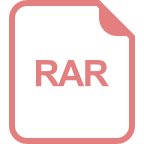
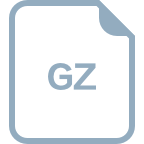
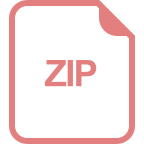
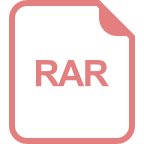
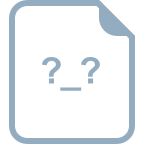
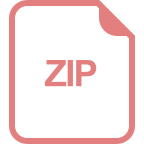
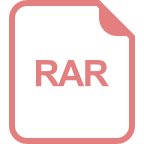
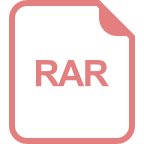
收起资源包目录

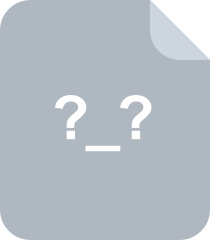
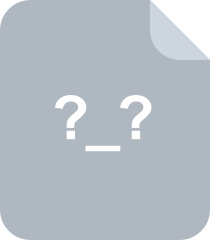
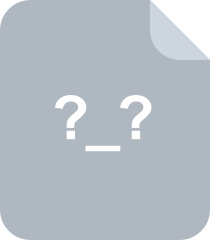
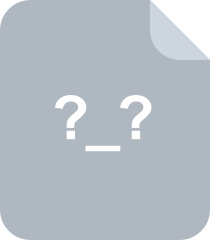
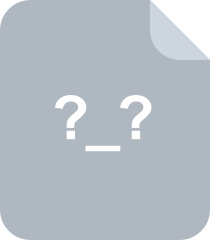
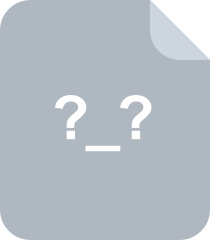
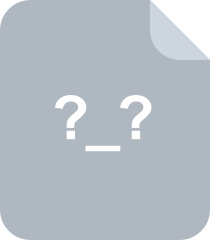
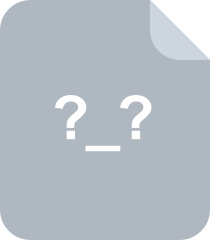
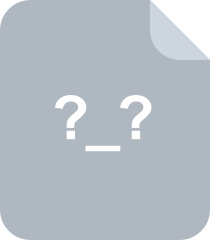
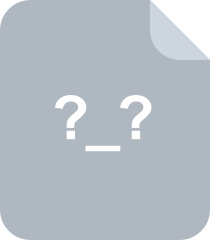
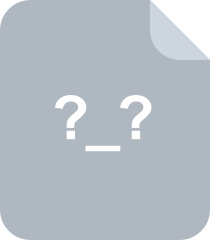
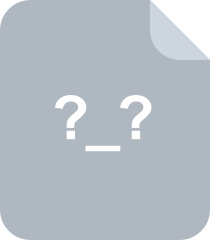
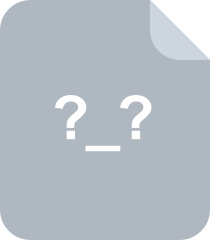
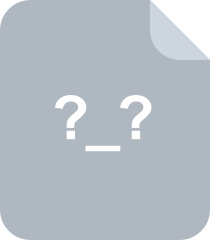
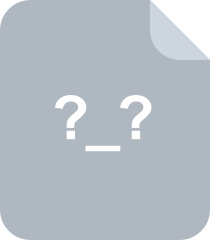
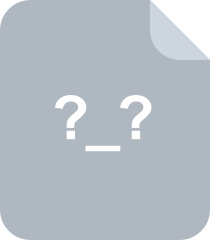
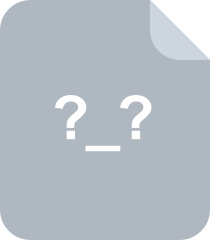
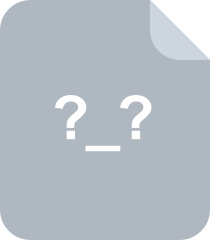
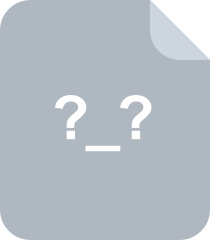
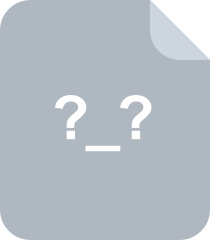
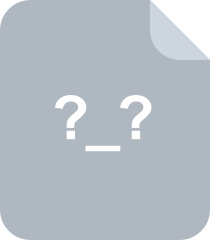
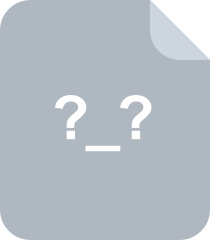
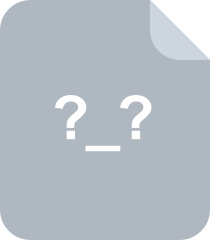
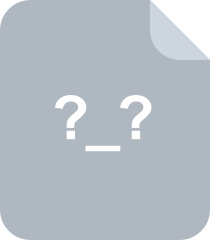
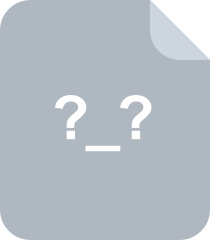
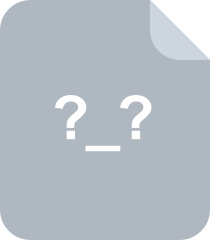
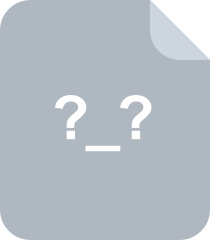
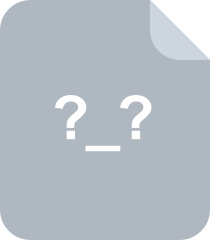
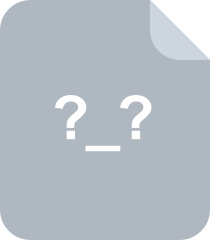
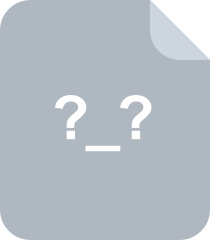
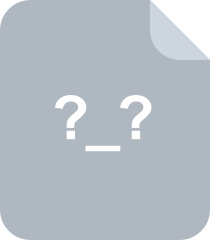
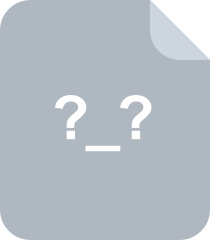
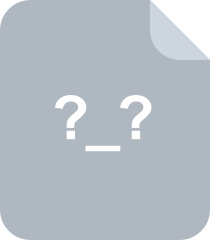
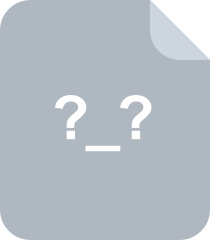
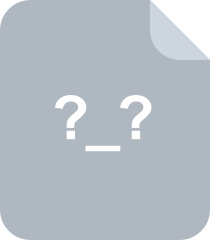
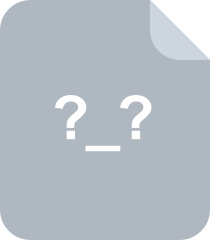
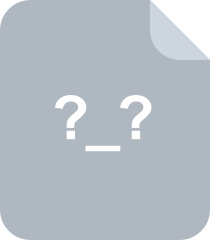
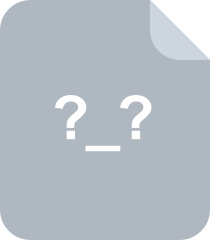
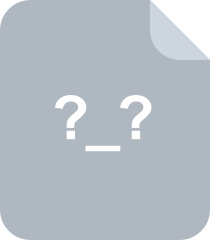
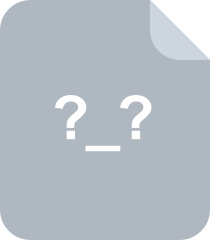
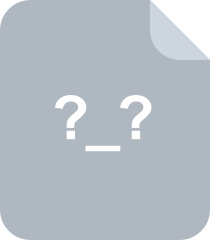
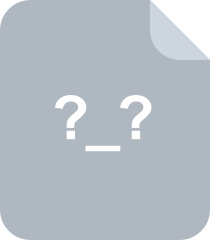
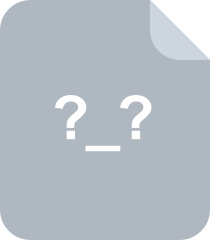
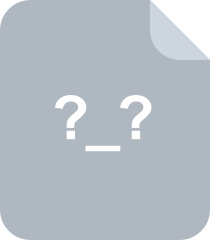
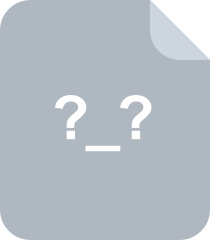
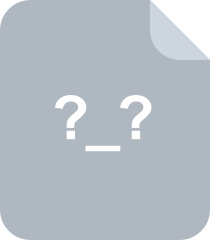
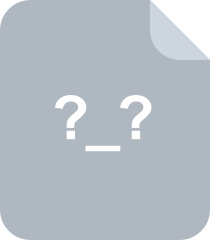
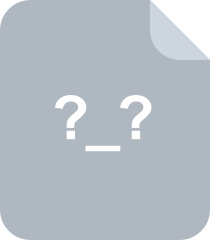
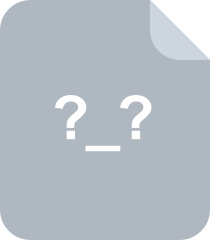
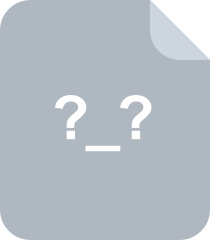
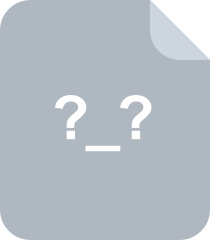
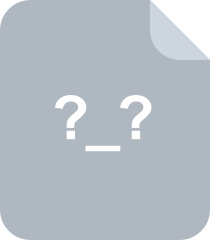
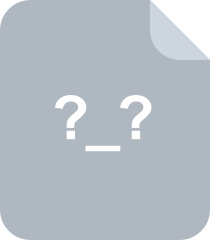
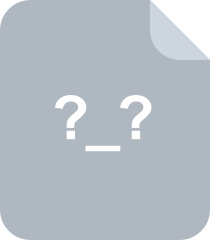
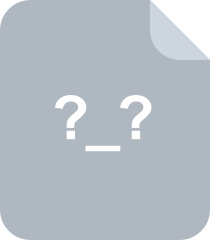
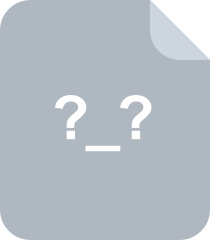
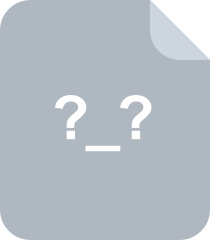
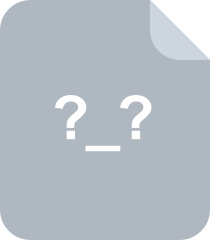
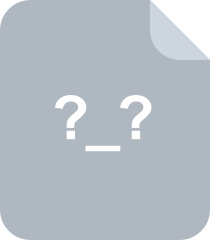
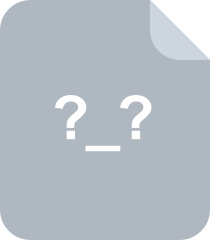
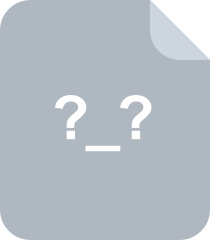
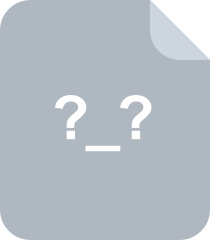
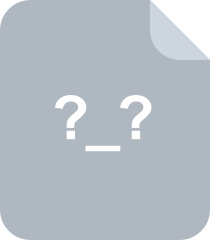
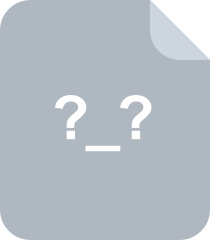
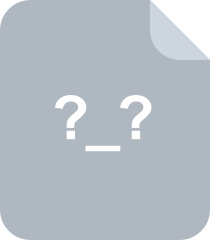
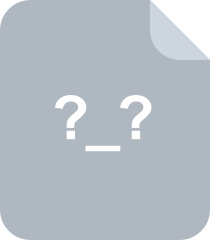
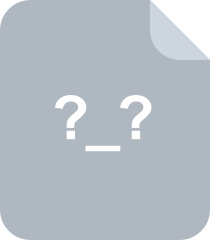
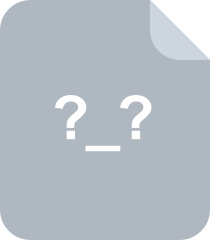
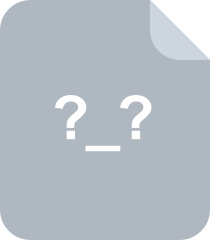
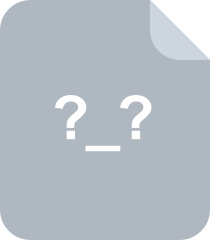
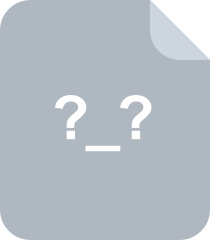
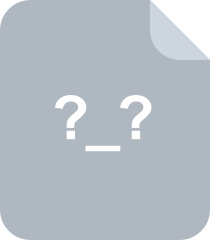
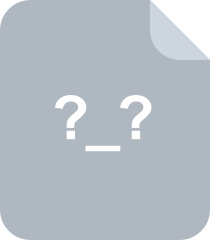
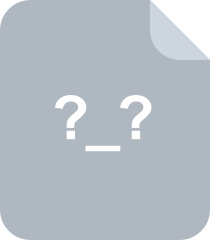
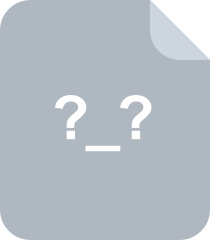
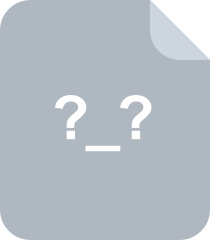
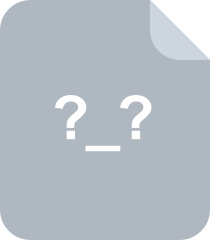
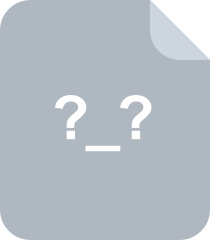
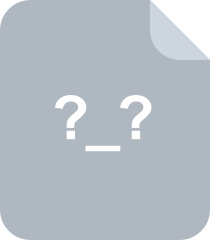
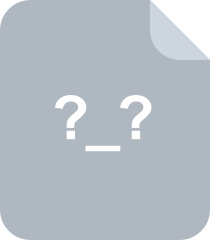
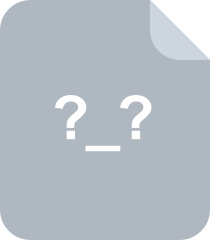
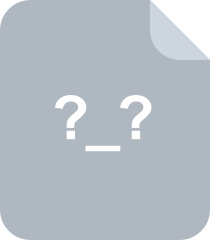
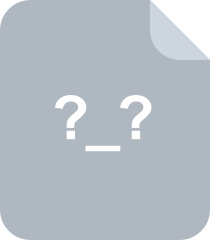
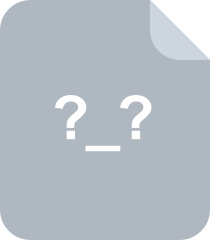
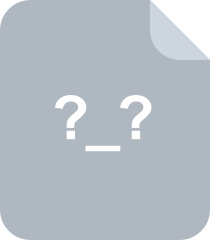
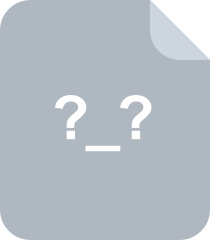
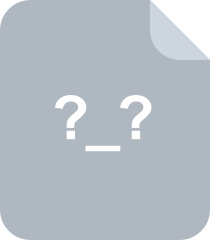
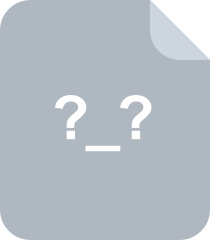
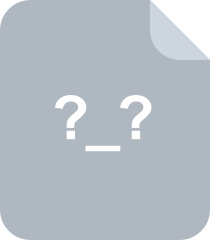
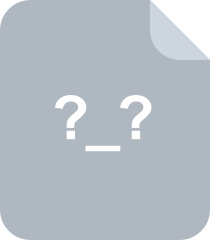
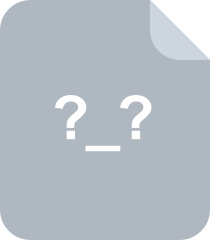
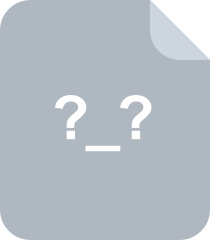
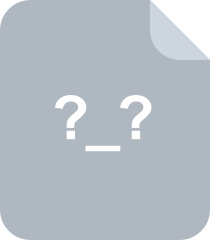
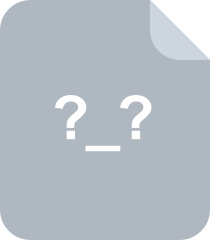
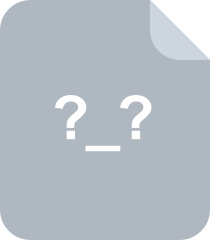
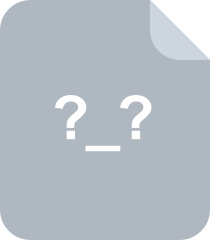
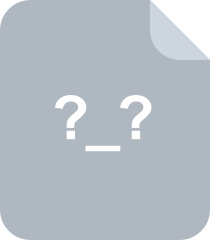
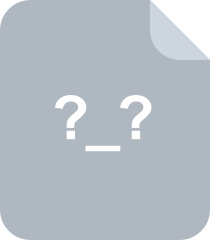
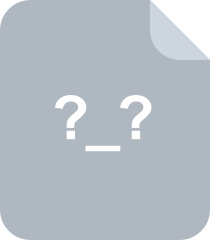
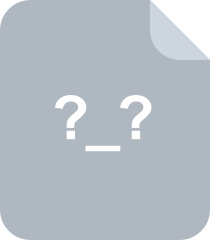
共 354 条
- 1
- 2
- 3
- 4
资源评论
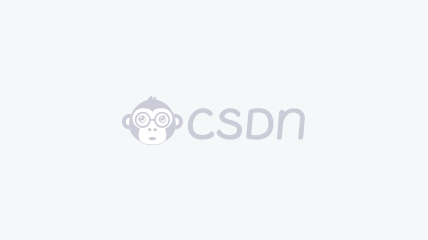
- Ywmet2012-12-17就是我要找的东西,谢谢分享
- hfut_jf2013-10-28不错,可以满足基本密码学实验需求
- dancwolf2012-07-23好东西啊,ida生成库无问题
- baixuefei19882012-05-31编译通过,从别处找了个RSA加密算法的DEMO,成功~好资源!!

sun378554759
- 粉丝: 6
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

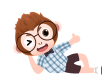
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


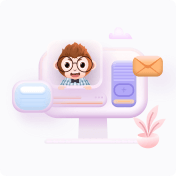
安全验证
文档复制为VIP权益,开通VIP直接复制
