### 链表基础知识 #### 引言 链表是一种常用的数据结构,在计算机科学领域有着广泛的应用。本文档由Nick Parlante撰写,旨在为读者提供关于链表的基础知识和技术细节,包括解释、图表、示例代码和练习。文档不仅适合那些希望理解链表基本原理的人群,同时也适用于那些寻求真实应用中指针密集型代码实例的学习者。 #### 为什么学习链表? 链表之所以值得学习,主要有两个原因:一方面,它们是一种实用的数据结构,可以在实际程序中使用;另一方面,通过链表的学习可以深入了解指针的工作机制。虽然在某些情况下,你可能永远不会在实际项目中直接使用链表,但理解和掌握链表背后的原理对于更好地使用指针至关重要。链表问题通常涉及算法和指针操作的结合,是初学者理解指针概念的理想场所。 #### 链表的定义与结构 链表是一种线性数据结构,它不像数组那样在内存中连续存储元素,而是通过节点(Node)之间的链接来组织数据。每个节点包含两部分:一部分用于存储数据元素(称为数据域),另一部分则包含指向下一个节点的引用(称为指针域或链接域)。 链表的基本类型包括单向链表(Singly Linked List)和双向链表(Doubly Linked List)。在单向链表中,每个节点只包含一个指向其后继节点的指针;而在双向链表中,除了包含指向后继节点的指针外,每个节点还包含一个指向前驱节点的指针,这使得双向链表支持更灵活的遍历方式。 #### 创建与操作链表 创建链表通常包括以下几个步骤: 1. **初始化**:创建一个空的链表,通常通过一个头指针表示。 2. **插入节点**:在链表的不同位置插入新节点,例如头部插入、尾部插入或中间插入。 3. **删除节点**:根据特定条件删除链表中的节点。 4. **遍历链表**:访问链表中的每一个节点。 5. **查找节点**:基于特定条件搜索链表中的节点。 6. **反转链表**:改变链表节点的顺序。 #### 指针与内存管理 为了深入理解链表,必须具备一定的指针和内存管理基础。在链表中,节点是由动态分配的内存块组成,因此需要通过指针来管理和操作这些内存块。理解如何正确地分配和释放内存,以及如何避免内存泄漏等问题,是学习链表不可或缺的一部分。 #### 实践与练习 除了理论知识外,实践也是学习链表的重要组成部分。《链表问题》一文提供了18个不同难度级别的练习题,涵盖了链表操作的各个方面。通过解决这些问题,不仅可以巩固对链表基础知识的理解,还能提高解决问题的能力。 #### 结论 链表作为一种重要的数据结构,对于程序员来说具有不可替代的价值。无论是作为实际项目中的解决方案,还是作为学习指针和内存管理的工具,链表都是必不可少的。通过本篇文章的学习,希望能够帮助读者建立起对链表及其应用场景的深刻认识,并为未来的学习和开发打下坚实的基础。
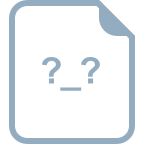
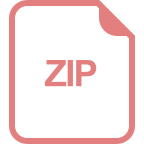
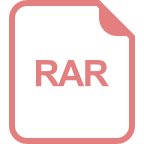
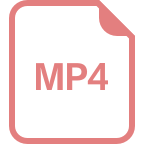
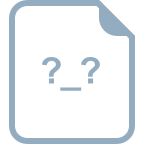
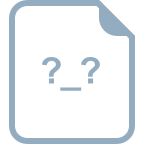
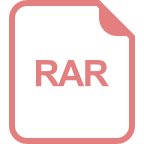
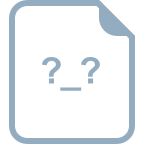
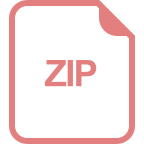
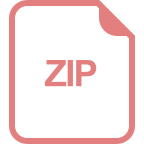
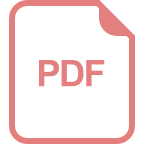
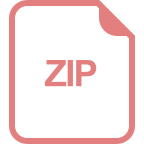
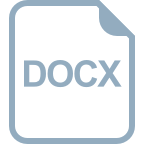
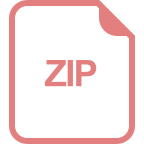
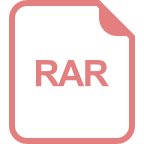
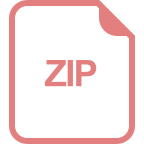
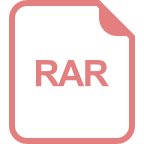
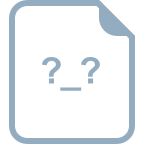
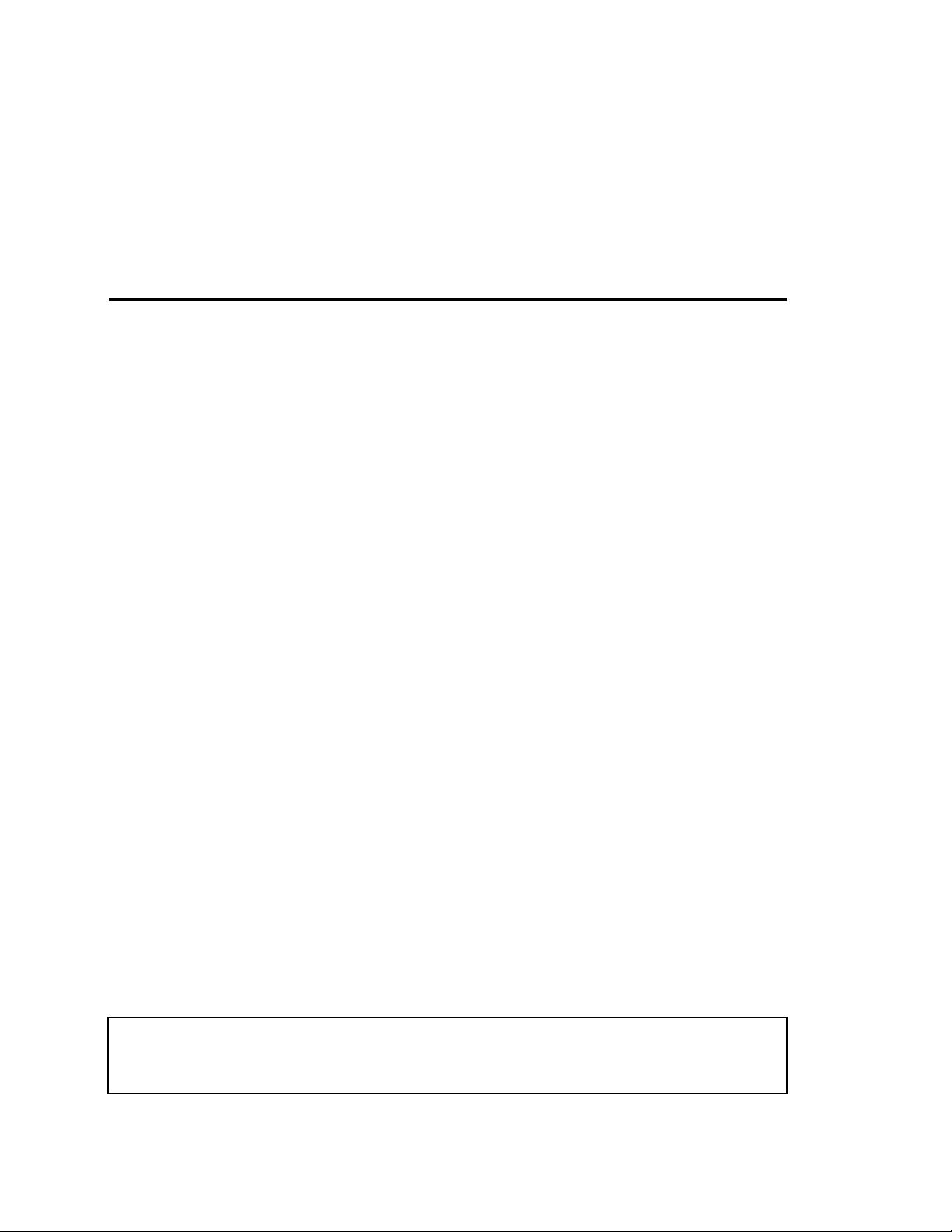
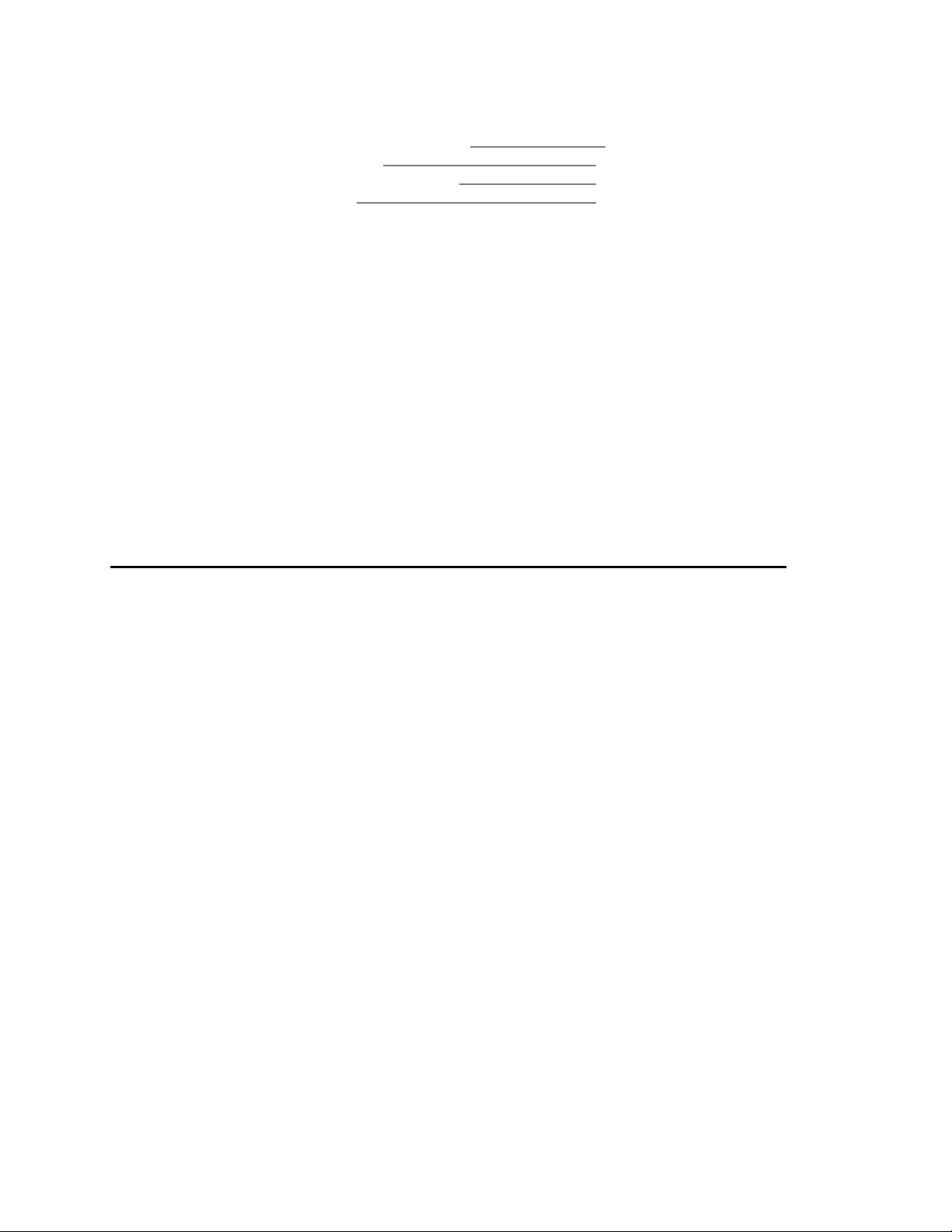
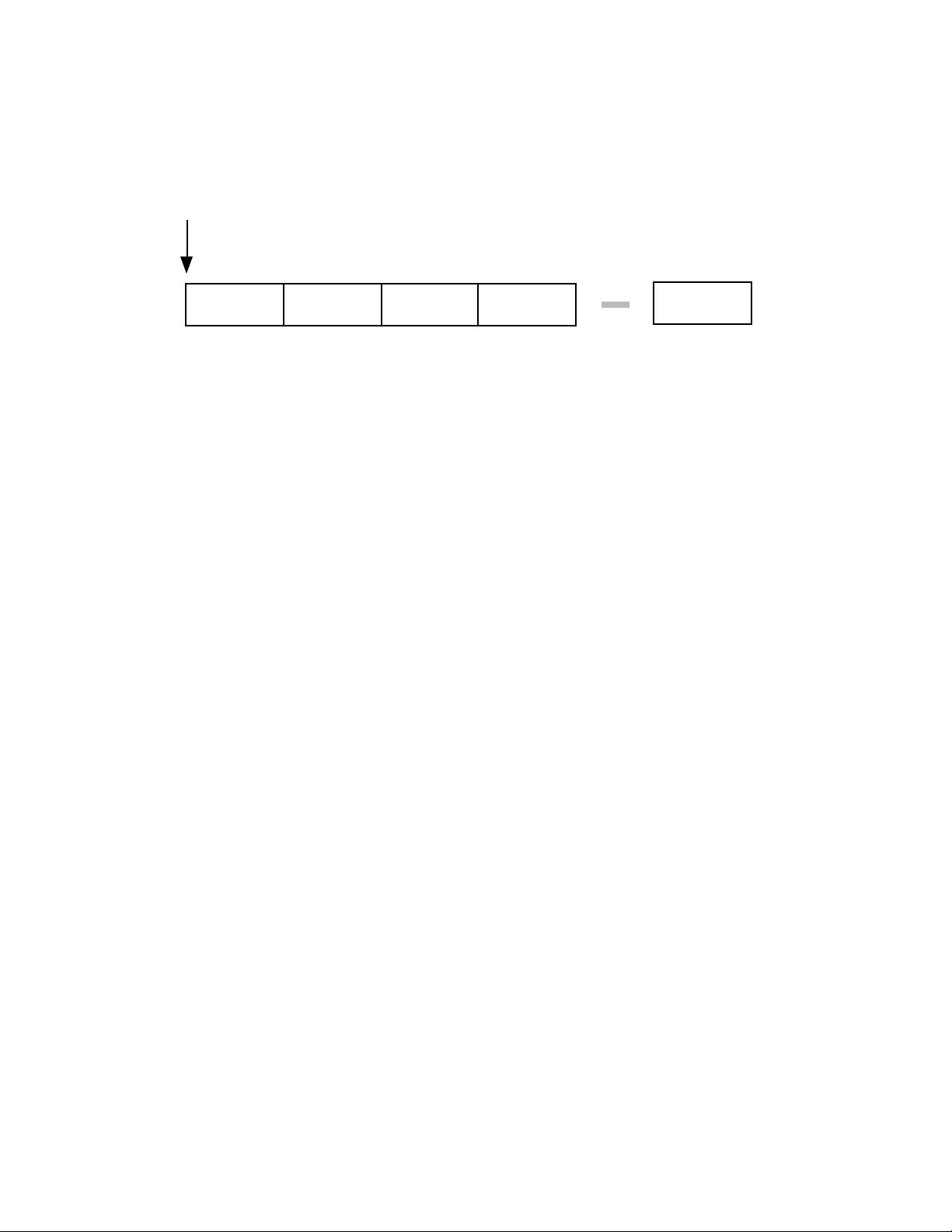
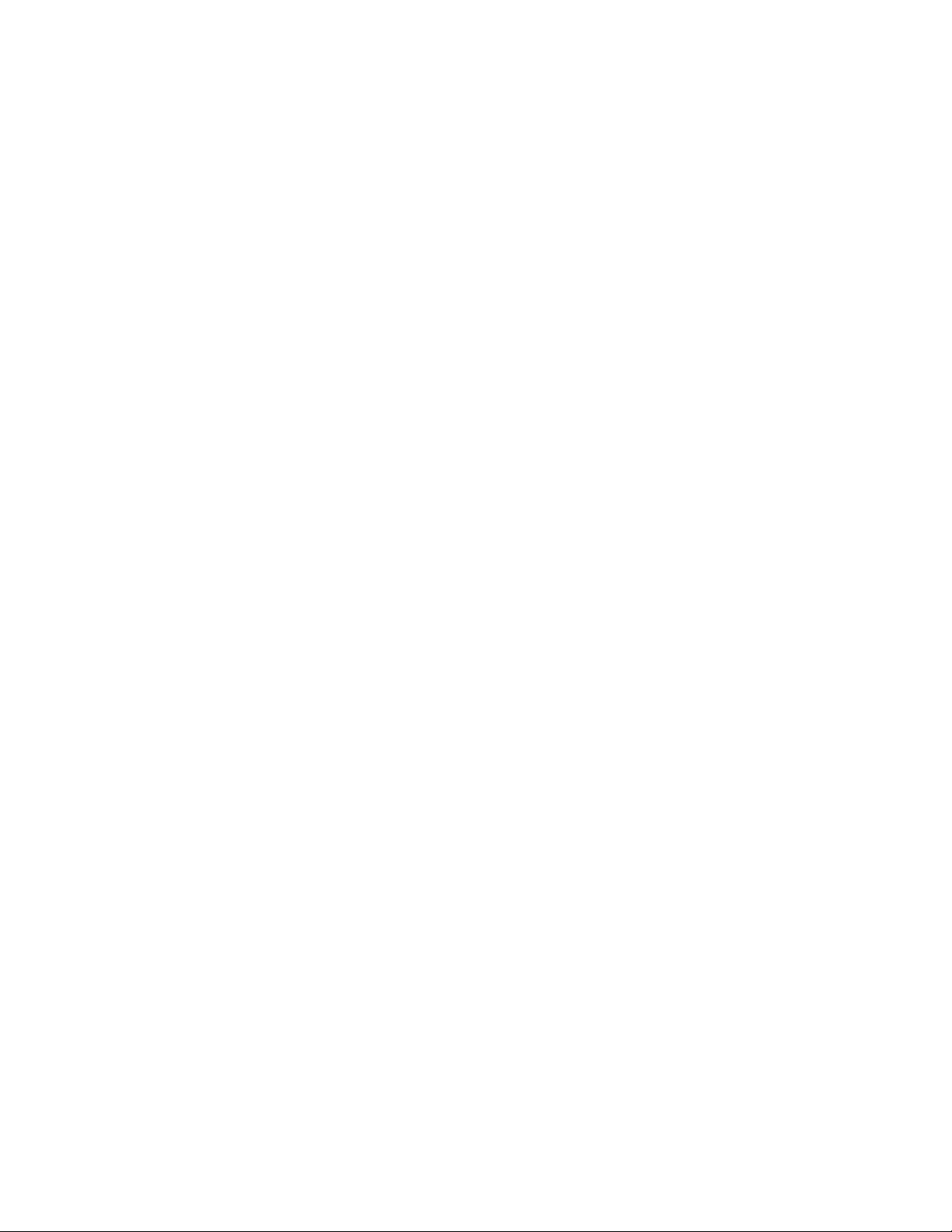
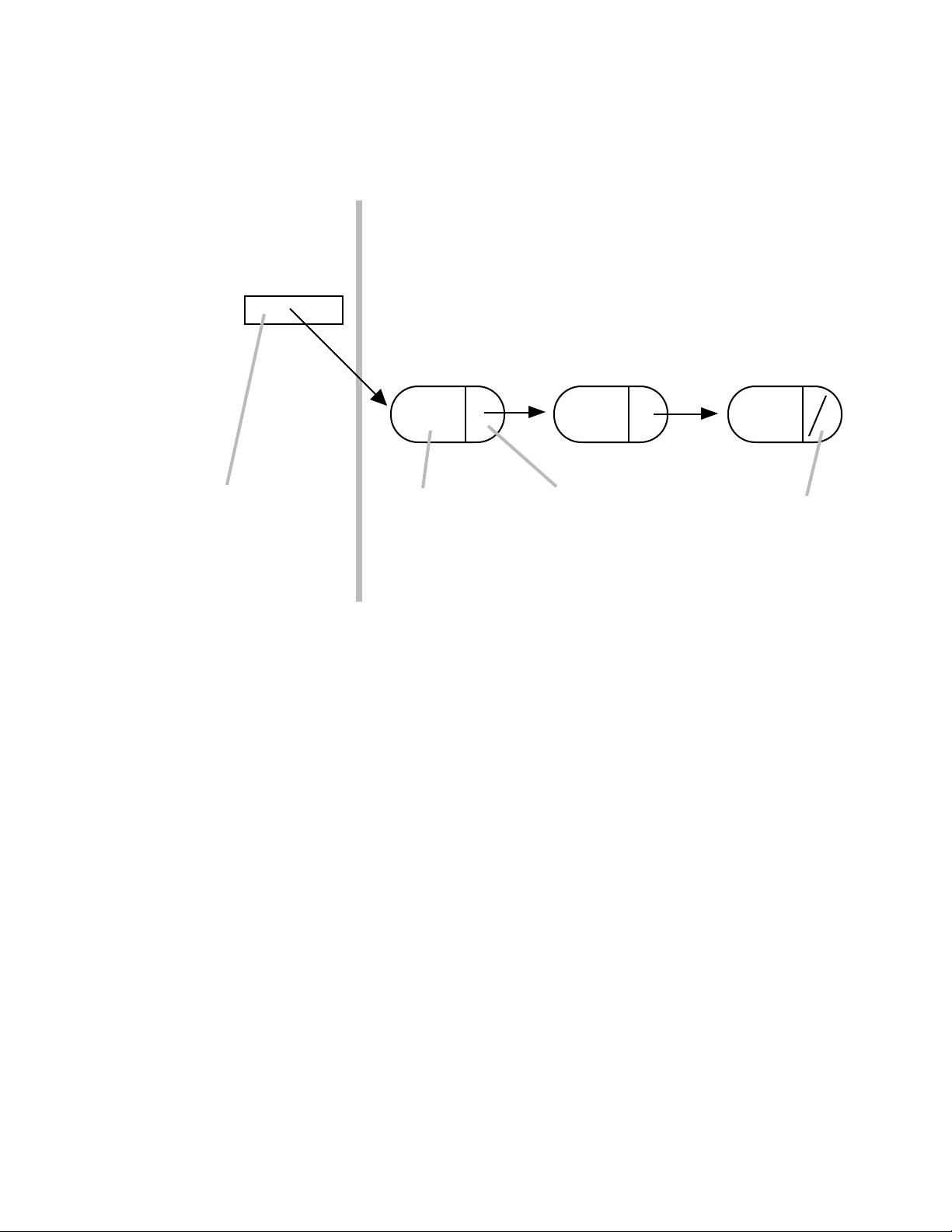
剩余25页未读,继续阅读
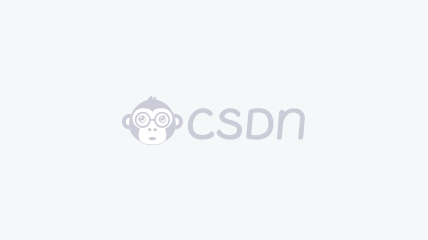
- darlingsun19882012-10-25真的是STANFORD的资料,看了之后还有有些用处的,赞一个

- 粉丝: 35
- 资源: 13
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

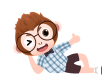
最新资源
- 面部、耳廓损伤损伤程度分级表.docx
- 农资使用情况调查问卷.docx
- 燃气管道施工资质和特种设备安装改造维修委托函.docx
- 食物有毒的鉴定方法.docx
- 市政道路工程联合质量抽检记录表.docx
- 市政道路工程联合质量抽检项目、判定标准、频率或点数.docx
- 视力听力残疾标准.docx
- 视器视力损伤程度分级表.docx
- 收回扣检查报告.docx
- 输液室管理制度、治疗配药室、注射室、处置室感染管理制度、查对制度.docx
- 听器听力损伤程度分级表.docx
- 新生儿评分apgar标准五项、五项体征的打分标准.docx
- 医疗废弃物环境风险评价依据、环境风险分析.docx
- 预防溺水宣传口号.docx
- 招标代理方案评分表.docx
- 职业暴露后的处理流程.docx

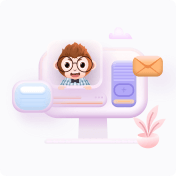
