package org.json;
/*
Copyright (c) 2002 JSON.org
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
The Software shall be used for Good, not Evil.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
*/
import java.io.IOException;
import java.io.Writer;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.lang.reflect.Modifier;
import java.util.Collection;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Locale;
import java.util.Map;
import java.util.ResourceBundle;
/**
* A JSONObject is an unordered collection of name/value pairs. Its external
* form is a string wrapped in curly braces with colons between the names and
* values, and commas between the values and names. The internal form is an
* object having <code>get</code> and <code>opt</code> methods for accessing the
* values by name, and <code>put</code> methods for adding or replacing values
* by name. The values can be any of these types: <code>Boolean</code>,
* <code>JSONArray</code>, <code>JSONObject</code>, <code>Number</code>,
* <code>String</code>, or the <code>JSONObject.NULL</code> object. A JSONObject
* constructor can be used to convert an external form JSON text into an
* internal form whose values can be retrieved with the <code>get</code> and
* <code>opt</code> methods, or to convert values into a JSON text using the
* <code>put</code> and <code>toString</code> methods. A <code>get</code> method
* returns a value if one can be found, and throws an exception if one cannot be
* found. An <code>opt</code> method returns a default value instead of throwing
* an exception, and so is useful for obtaining optional values.
* <p>
* The generic <code>get()</code> and <code>opt()</code> methods return an
* object, which you can cast or query for type. There are also typed
* <code>get</code> and <code>opt</code> methods that do type checking and type
* coercion for you. The opt methods differ from the get methods in that they do
* not throw. Instead, they return a specified value, such as null.
* <p>
* The <code>put</code> methods add or replace values in an object. For example,
*
* <pre>
* myString = new JSONObject().put("JSON", "Hello, World!").toString();
* </pre>
*
* produces the string <code>{"JSON": "Hello, World"}</code>.
* <p>
* The texts produced by the <code>toString</code> methods strictly conform to
* the JSON syntax rules. The constructors are more forgiving in the texts they
* will accept:
* <ul>
* <li>An extra <code>,</code> <small>(comma)</small> may appear just
* before the closing brace.</li>
* <li>Strings may be quoted with <code>'</code> <small>(single
* quote)</small>.</li>
* <li>Strings do not need to be quoted at all if they do not begin with a quote
* or single quote, and if they do not contain leading or trailing spaces, and
* if they do not contain any of these characters:
* <code>{ } [ ] / \ : , = ; #</code> and if they do not look like numbers and
* if they are not the reserved words <code>true</code>, <code>false</code>, or
* <code>null</code>.</li>
* <li>Keys can be followed by <code>=</code> or <code>=></code> as well as by
* <code>:</code>.</li>
* <li>Values can be followed by <code>;</code> <small>(semicolon)</small> as
* well as by <code>,</code> <small>(comma)</small>.</li>
* </ul>
*
* @author JSON.org
* @version 2011-11-24
*/
@SuppressWarnings({ "rawtypes", "unchecked" })
public class JSONObject {
/**
* JSONObject.NULL is equivalent to the value that JavaScript calls null,
* whilst Java's null is equivalent to the value that JavaScript calls
* undefined.
*/
private static final class Null {
/**
* There is only intended to be a single instance of the NULL object, so
* the clone method returns itself.
*
* @return NULL.
*/
protected final Object clone() {
return this;
}
/**
* A Null object is equal to the null value and to itself.
*
* @param object
* An object to test for nullness.
* @return true if the object parameter is the JSONObject.NULL object or
* null.
*/
public boolean equals(Object object) {
return object == null || object == this;
}
/**
* Get the "null" string value.
*
* @return The string "null".
*/
public String toString() {
return "null";
}
}
/**
* The map where the JSONObject's properties are kept.
*/
private final Map map;
/**
* It is sometimes more convenient and less ambiguous to have a
* <code>NULL</code> object than to use Java's <code>null</code> value.
* <code>JSONObject.NULL.equals(null)</code> returns <code>true</code>.
* <code>JSONObject.NULL.toString()</code> returns <code>"null"</code>.
*/
public static final Object NULL = new Null();
/**
* Construct an empty JSONObject.
*/
public JSONObject() {
this.map = new HashMap();
}
/**
* Construct a JSONObject from a subset of another JSONObject. An array of
* strings is used to identify the keys that should be copied. Missing keys
* are ignored.
*
* @param jo
* A JSONObject.
* @param names
* An array of strings.
* @throws JSONException
* @exception JSONException
* If a value is a non-finite number or if a name is
* duplicated.
*/
public JSONObject(JSONObject jo, String[] names) {
this();
for (int i = 0; i < names.length; i += 1) {
try {
this.putOnce(names[i], jo.opt(names[i]));
} catch (Exception ignore) {
}
}
}
/**
* Construct a JSONObject from a JSONTokener.
*
* @param x
* A JSONTokener object containing the source string.
* @throws JSONException
* If there is a syntax error in the source string or a
* duplicated key.
*/
public JSONObject(JSONTokener x) throws JSONException {
this();
char c;
String key;
if (x.nextClean() != '{') {
throw x.syntaxError("A JSONObject text must begin with '{'");
}
for (;;) {
c = x.nextClean();
switch (c) {
case 0:
throw x.syntaxError("A JSONObject text must end with '}'");
case '}':
return;
default:
x.back();
key = x.nextValue().toString();
}
// The key is followed by ':'. We will also tolerate '=' or '=>'.
c = x.nextClean();
if (c == '=') {
if (x.next() != '>') {
x.back();
}
} else if (c != ':') {
throw x.syntaxError("Expected a ':' after a key");
}
this.putOnce(key, x.nextValue());
// Pairs are separated by ','. We will also tolerate ';'.
switch (x.nextClean()) {
case ';':
case ',':
if (x.nextClean() == '}') {
return;
}
x.back();
break;
case '}':
return;
default:
throw x.syntaxError("Expected a ',' or '}'");
}
}
}
/**
* Construct a JSONObject from a Map.
*
* @param map
* A map object that can be used to initialize the contents of
* the JSONObject.
* @throws JSONException
*/
public JSONObject(Map map) {

stateCelebrateking
- 粉丝: 90
- 资源: 34
最新资源
- 使用LLVM的高性能零开销可扩展Python编译器.zip
- 使用python pandas库的食谱.zip
- 使用Python创建和修改Word文档.zip
- 使用Python和Jupyter Notebook课程和其他链接深入学习机器学习的免费方法.zip
- 使用python的常见数据分析和机器学习任务.zip
- 使用Python绘制matplotlib.zip
- 使用Python实现更轻的web自动化.zip
- 使用Python类型提示进行数据验证.zip
- 使用简单的Python API构建复杂的用户界面,在终端和web浏览器中运行应用程序.zip
- 适用于Python的Clean Code概念.zip
- 受pythonprompttoolkit的启发,在Go中构建强大的交互式提示符.zip
- 世界上最简单的Python面部识别api和命令行.zip
- 熟悉的asyncio ORM为python构建的关系.zip
- 斯坦福NLP Python库,用于标记化句子分割NER和解析许多人类语言.zip
- 所有3415个LeetCode问题的Python现代C解决方案每周更新.zip
- 它是用Python编写的React.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


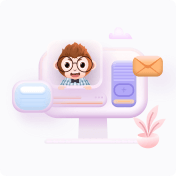