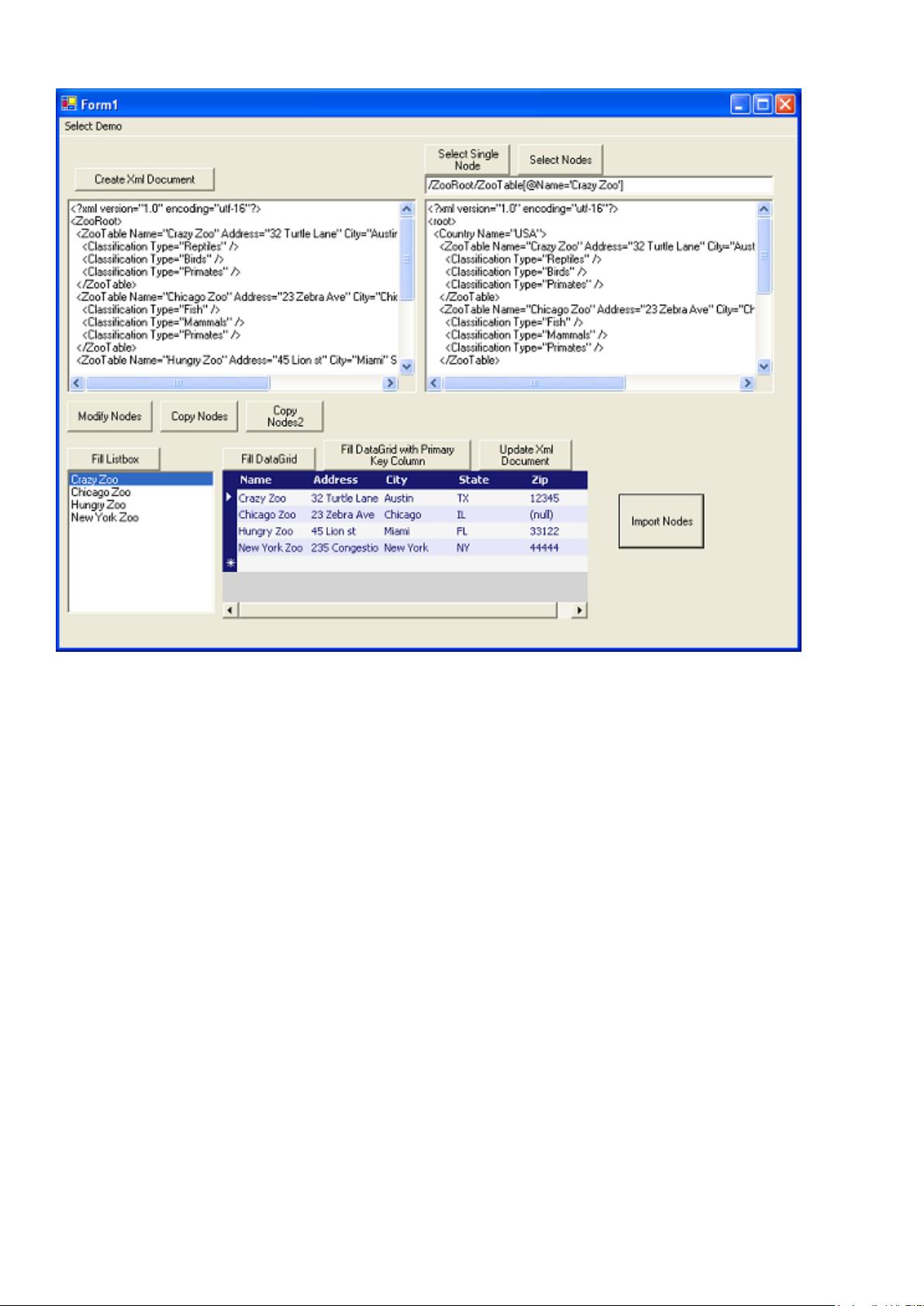
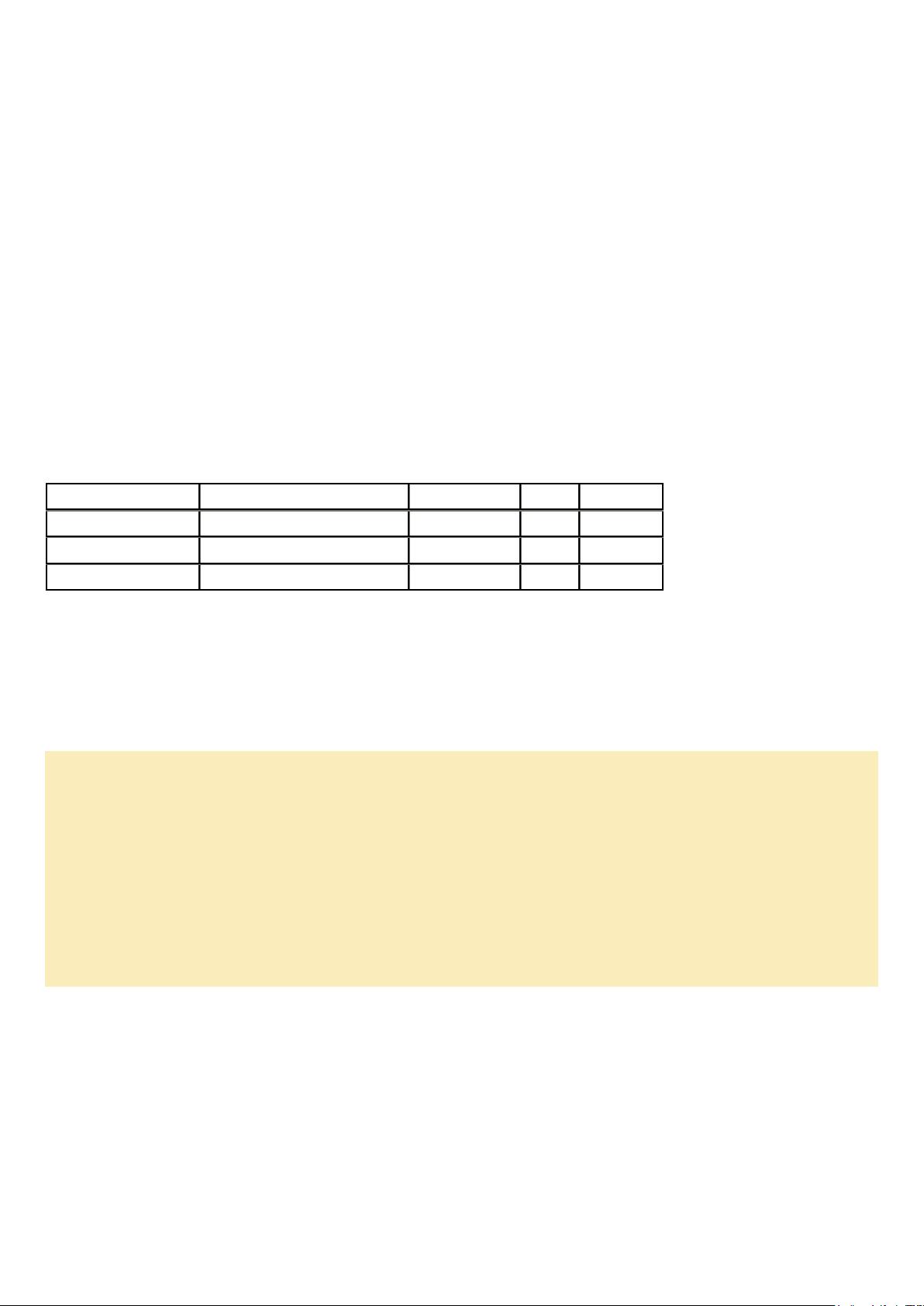
Introduction
After working with a couple of XML projects for the last six months, I am ready to share a class I wrote called
XmlHelper which got me through all the complexity of XML and made my life a lot simpler. If you are a XML
beginner or have no idea about XML, this article should teach you the very basic concepts that you need to create
any type of XML document. If you already have experience in XML, you will probably benefit by using the
methods available in XmlHelper. The article evolves from simple concepts about XML to creation of XML
documents, to DataSource assignment from XML documents to ListBoxes and DataGrids, and finally to
DataSource update from changes made on a DataGrid to update the source XML document.
Xml notation
I believe it’s always easier to learn with actual examples, so to give you a brief tutorial about XML, I created the
following "ZooTable". By the way, I have no idea about Zoos; it was the first thing that came to my mind when I
decided to give an example:
Name
Address
City
State
Zip
Crazy Zoo
32 Turtle Lane
Austin
Tx
12345
Chicago Zoo
23 Zebra Ave
Chicago
IL
(null)
Hungry Zoo
45 Lion st
Miami
FL
33122
There are two ways to represent this table, the first way which is the easier to read is by using "attributes". The
second way to represent this table using XML is by using "elements".
XmlDocument representation using attributes
<?xml version="1.0" encoding="utf-8" ?>
<ZooRoot>
<ZooTable Name="Crazy Zoo" Address="32 Turtle Lane"
City="Austin" State="TX" Zip="12345" />
<ZooTable Name="Chicago Zoo" Address="23 Zebra Ave"
City="Chicago" State="IL" />
<ZooTable Name="Hungry Zoo" Address="45 Lion st"
City="Miami" State="FL" Zip="33122" />
</ZooRoot>
The field "ZooRoot" is an element and the fields named "ZooTable" are also elements. The fields
inside ZooTable such as "Name", "Address", "City", "State", "Zip" are the "attributes" of element ZooTable.
Note: Each element is also called a node.
When you create an XML document you always need a root element and then inside that element you can place
any number of elements. In this case the root element is "ZooRoot" and the element inside this root is called
"ZooTable". Each column is represented by an attribute that corresponds to the column name.
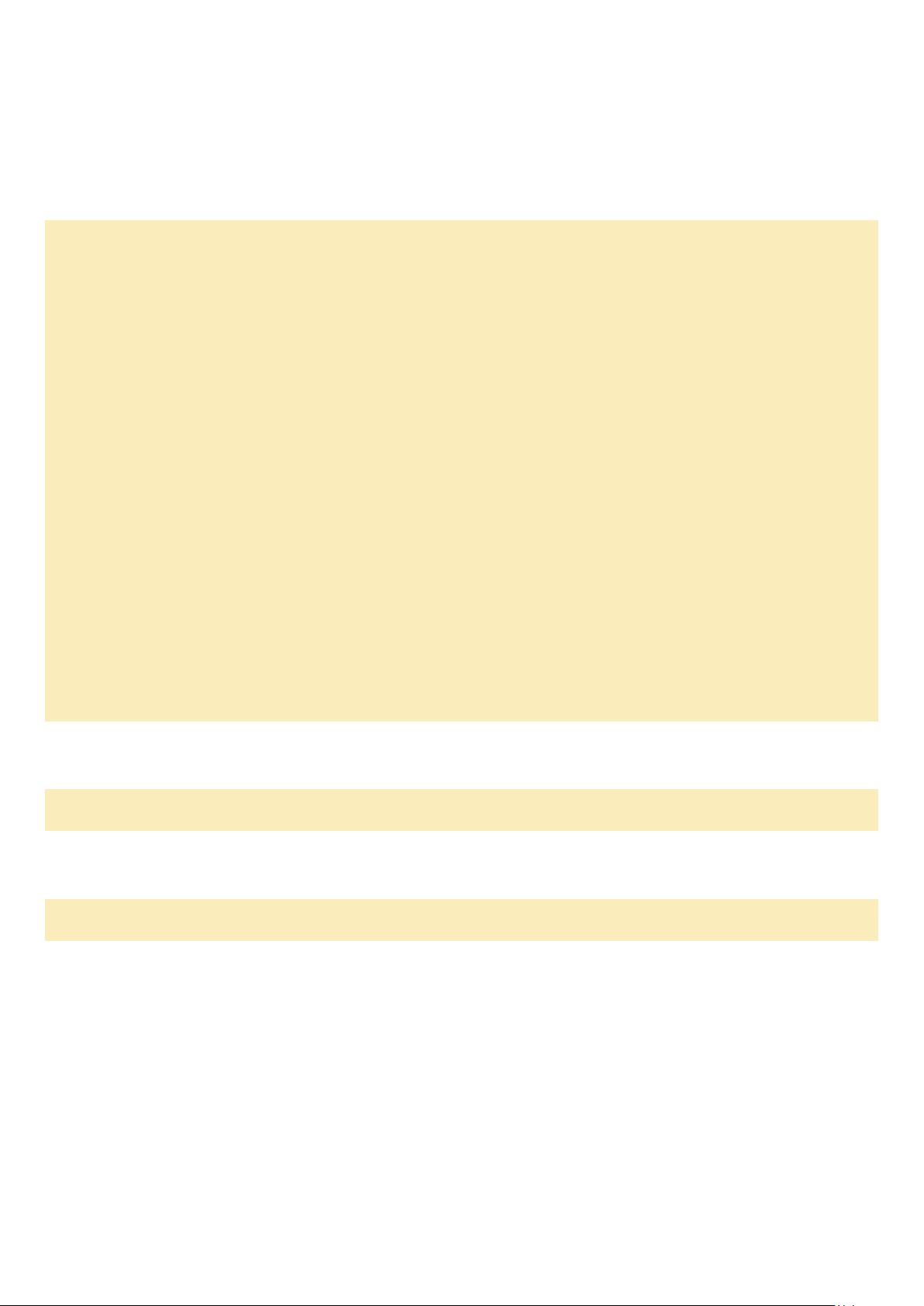
I left the "Zip" field of the second row empty intentionally so you can see how to represent null attributes in XML.
As you see, it’s rather simple, basically a null value in XML is represented by omitting that attribute.
If you have to create a child table of element "ZooTable" then the notation per line is slightly different, for
example if we want to create a child table that contains a classification for the type of animals of each write the
table as follows:
<?xml version="1.0" encoding="utf-8" ?>
<ZooRoot>
<ZooTable Name="Crazy Zoo" Address="32 Turtle Lane"
City="Austin" State="TX" Zip="12345">
<Classification Type="Reptiles" />
<Classification Type="Birds" />
<Classification Type="Primates" />
</ZooTable>
<ZooTable Name="Chicago Zoo" Address="23 Zebra Ave"
City="Chicago" State="IL" >
<Classification Type="Fish" />
<Classification Type="Mammals" />
<Classification Type="Primates" />
</ZooTable>
<ZooTable Name="Hungry Zoo" Address="45 Lion st"
City="Miami" State="FL" Zip="33122" >
<Classification Type="Arachnids" />
<Classification Type="Rodents" />
</ZooTable>
</ZooRoot>
Note that the notation of ZooTable element changed from:
<ElementName attribute1="…" attribute2="…" />
to:
<ElementName attribute1="…" attribute2="…" > </ElementName>
As you can see, it’s pretty simple to add child elements to the existing tables.
XmlDocument representation using elements
When I started learning about XML, I noticed that the preferred notation by Visual Studio was by using "Elements",
so I thought that this was the way we should always represent data; however, as I learned more about XML
notation, I learned that if you are dealing mainly with table representations then you can use
either elements or attributes to represent your XML data; therefore, I usually avoid representing data using
elements because its notation uses more space and it is harder to read if you ever have to debug it. Keep in mind
that although XML representation using attributes is more readable, it is less powerful because an attribute
cannot contain child elements whereas elements can contain any number of child elements.
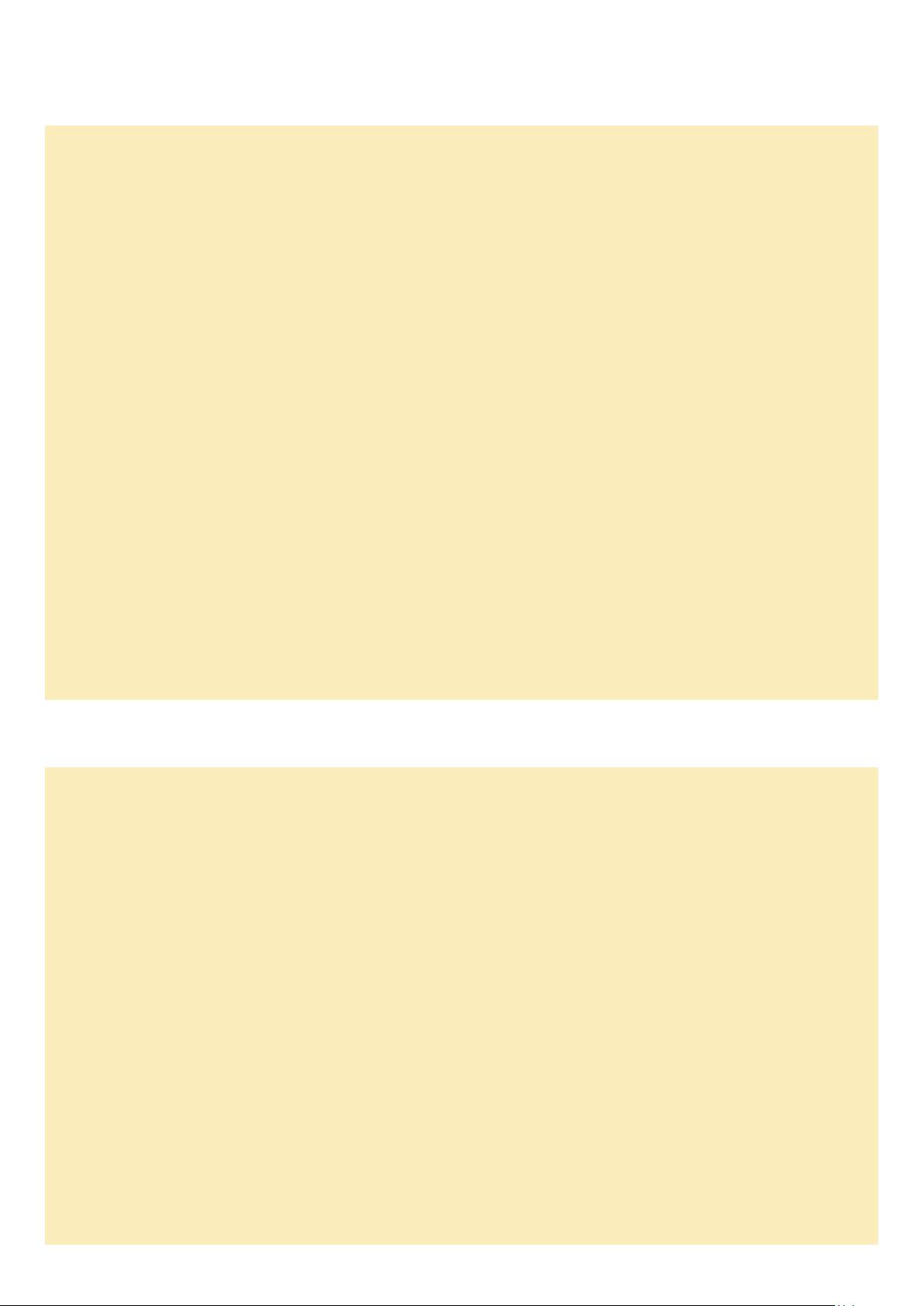
The following representation is to show you the equivalent table using elements instead of attributes. For the
rest of the examples I will use XML notation with attributes.
<?xml version="1.0" encoding="utf-8" ?>
<ZooRoot>
<ZooTable>
<Name>Crazy Zoo</Name>
<Address>32 Turtle Lane</Address>
<City>Austin</City>
<State>TX</State>
<Zip>12345</Zip>
</ZooTable>
<ZooTable>
<Name>Chicago Zoo</Name>
<Address>23 Zebra Ave</Address>
<City>Chicago</City>
<State>IL</State>
</ZooTable>
<ZooTable>
<Name>Hungry Zoo</Name>
<Address>45 Lion st</Address>
<City>Miami</City>
<State>FL</State>
<Zip>33122</Zip>
</ZooTable>
</ZooRoot>
And the following XML representation shows you how to add the "Classification" child nodes using elements:
<?xml version="1.0" encoding="utf-8" ?>
<ZooRoot>
<ZooTable>
<Name>Crazy Zoo</Name>
<Address>32 Turtle Lane</Address>
<City>Austin</City>
<State>TX</State>
<Zip>12345</Zip>
<Classification>
<Type>Reptiles</Type>
<Type>Birds</Type>
<Type>Primates</Type>
</Classification>
</ZooTable>
<ZooTable>
<Name>Chicago Zoo</Name>
<Address>23 Zebra Ave</Address>
<City>Chicago</City>
<State>IL</State>