package com.cl.controller;
import java.math.BigDecimal;
import java.text.SimpleDateFormat;
import java.text.ParseException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Calendar;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Date;
import java.util.List;
import javax.servlet.http.HttpServletRequest;
import com.cl.utils.ValidatorUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.format.annotation.DateTimeFormat;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import com.baomidou.mybatisplus.mapper.EntityWrapper;
import com.baomidou.mybatisplus.mapper.Wrapper;
import com.cl.annotation.IgnoreAuth;
import com.cl.entity.XueshengchengjiEntity;
import com.cl.entity.view.XueshengchengjiView;
import com.cl.service.XueshengchengjiService;
import com.cl.service.TokenService;
import com.cl.utils.PageUtils;
import com.cl.utils.R;
import com.cl.utils.MPUtil;
import com.cl.utils.CommonUtil;
import java.io.IOException;
/**
* 学生成绩
* 后端接口
* @author
* @email
* @date 2024-03-05 21:53:17
*/
@RestController
@RequestMapping("/xueshengchengji")
public class XueshengchengjiController {
@Autowired
private XueshengchengjiService xueshengchengjiService;
/**
* 后端列表
*/
@RequestMapping("/page")
public R page(@RequestParam Map<String, Object> params,XueshengchengjiEntity xueshengchengji,
@RequestParam(required = false) Double kaoshichengjistart,
@RequestParam(required = false) Double kaoshichengjiend,
HttpServletRequest request){
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("xuesheng")) {
xueshengchengji.setXuehao((String)request.getSession().getAttribute("username"));
}
if(tableName.equals("jiaoshi")) {
xueshengchengji.setJiaoshigonghao((String)request.getSession().getAttribute("username"));
}
EntityWrapper<XueshengchengjiEntity> ew = new EntityWrapper<XueshengchengjiEntity>();
if(kaoshichengjistart!=null) ew.ge("kaoshichengji", kaoshichengjistart);
if(kaoshichengjiend!=null) ew.le("kaoshichengji", kaoshichengjiend);
PageUtils page = xueshengchengjiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, xueshengchengji), params), params));
return R.ok().put("data", page);
}
/**
* 前端列表
*/
@IgnoreAuth
@RequestMapping("/list")
public R list(@RequestParam Map<String, Object> params,XueshengchengjiEntity xueshengchengji,
@RequestParam(required = false) Double kaoshichengjistart,
@RequestParam(required = false) Double kaoshichengjiend,
HttpServletRequest request){
EntityWrapper<XueshengchengjiEntity> ew = new EntityWrapper<XueshengchengjiEntity>();
if(kaoshichengjistart!=null) ew.ge("kaoshichengji", kaoshichengjistart);
if(kaoshichengjiend!=null) ew.le("kaoshichengji", kaoshichengjiend);
PageUtils page = xueshengchengjiService.queryPage(params, MPUtil.sort(MPUtil.between(MPUtil.likeOrEq(ew, xueshengchengji), params), params));
return R.ok().put("data", page);
}
/**
* 列表
*/
@RequestMapping("/lists")
public R list( XueshengchengjiEntity xueshengchengji){
EntityWrapper<XueshengchengjiEntity> ew = new EntityWrapper<XueshengchengjiEntity>();
ew.allEq(MPUtil.allEQMapPre( xueshengchengji, "xueshengchengji"));
return R.ok().put("data", xueshengchengjiService.selectListView(ew));
}
/**
* 查询
*/
@RequestMapping("/query")
public R query(XueshengchengjiEntity xueshengchengji){
EntityWrapper< XueshengchengjiEntity> ew = new EntityWrapper< XueshengchengjiEntity>();
ew.allEq(MPUtil.allEQMapPre( xueshengchengji, "xueshengchengji"));
XueshengchengjiView xueshengchengjiView = xueshengchengjiService.selectView(ew);
return R.ok("查询学生成绩成功").put("data", xueshengchengjiView);
}
/**
* 后端详情
*/
@RequestMapping("/info/{id}")
public R info(@PathVariable("id") Long id){
XueshengchengjiEntity xueshengchengji = xueshengchengjiService.selectById(id);
xueshengchengji = xueshengchengjiService.selectView(new EntityWrapper<XueshengchengjiEntity>().eq("id", id));
return R.ok().put("data", xueshengchengji);
}
/**
* 前端详情
*/
@IgnoreAuth
@RequestMapping("/detail/{id}")
public R detail(@PathVariable("id") Long id){
XueshengchengjiEntity xueshengchengji = xueshengchengjiService.selectById(id);
xueshengchengji = xueshengchengjiService.selectView(new EntityWrapper<XueshengchengjiEntity>().eq("id", id));
return R.ok().put("data", xueshengchengji);
}
/**
* 后端保存
*/
@RequestMapping("/save")
public R save(@RequestBody XueshengchengjiEntity xueshengchengji, HttpServletRequest request){
xueshengchengji.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(xueshengchengji);
xueshengchengjiService.insert(xueshengchengji);
return R.ok();
}
/**
* 前端保存
*/
@RequestMapping("/add")
public R add(@RequestBody XueshengchengjiEntity xueshengchengji, HttpServletRequest request){
xueshengchengji.setId(new Date().getTime()+new Double(Math.floor(Math.random()*1000)).longValue());
//ValidatorUtils.validateEntity(xueshengchengji);
xueshengchengjiService.insert(xueshengchengji);
return R.ok();
}
/**
* 修改
*/
@RequestMapping("/update")
@Transactional
public R update(@RequestBody XueshengchengjiEntity xueshengchengji, HttpServletRequest request){
//ValidatorUtils.validateEntity(xueshengchengji);
xueshengchengjiService.updateById(xueshengchengji);//全部更新
return R.ok();
}
/**
* 删除
*/
@RequestMapping("/delete")
public R delete(@RequestBody Long[] ids){
xueshengchengjiService.deleteBatchIds(Arrays.asList(ids));
return R.ok();
}
/**
* (按值统计)
*/
@RequestMapping("/value/{xColumnName}/{yColumnName}")
public R value(@PathVariable("yColumnName") String yColumnName, @PathVariable("xColumnName") String xColumnName,HttpServletRequest request) {
Map<String, Object> params = new HashMap<String, Object>();
params.put("xColumn", xColumnName);
params.put("yColumn", yColumnName);
EntityWrapper<XueshengchengjiEntity> ew = new EntityWrapper<XueshengchengjiEntity>();
String tableName = request.getSession().getAttribute("tableName").toString();
if(tableName.equals("xuesheng")) {
ew.eq("xuehao", (String)request.getSession().getAttribute("username"));
}
if(tableName.equals("jiaoshi")) {
ew.eq("jiaoshigonghao", (String)request.getSession().getAttribute("username"));
}
List<Map<String, Object>> result = xueshengchengjiService.selectValue(params, ew);
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd");
for(Map<String, Object> m : result) {
for(String k : m.keySet()) {
if(m.get(k) instanceof Date) {
m.put(k,
没有合适的资源?快使用搜索试试~ 我知道了~
家校合作平台&java&基于springboot家校合作平台设计与实现
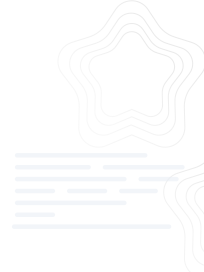
共375个文件
java:94个
vue:85个
png:46个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 158 浏览量
2024-11-09
17:33:24
上传
评论
收藏 12.09MB ZIP 举报
温馨提示
家校合作平台&java&基于springboot家校合作平台设计与实现
资源推荐
资源详情
资源评论
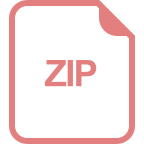
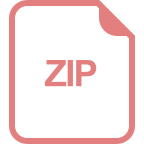
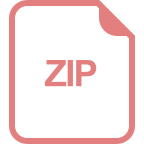
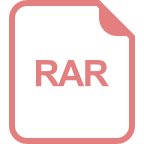
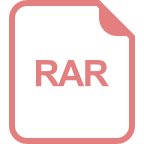
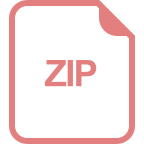
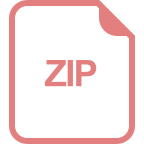
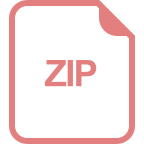
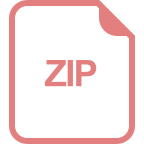
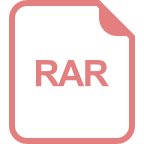
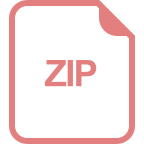
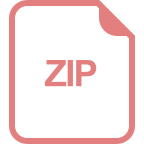
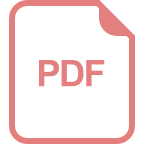
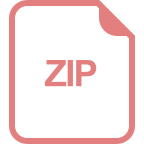
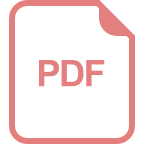
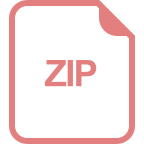
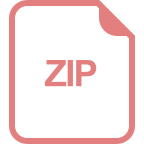
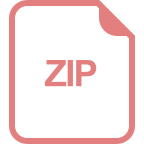
收起资源包目录

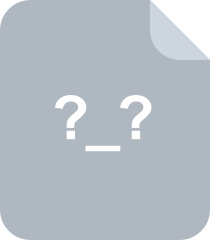
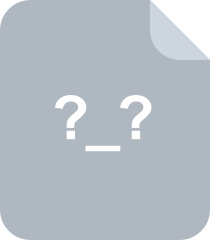
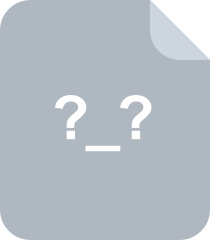
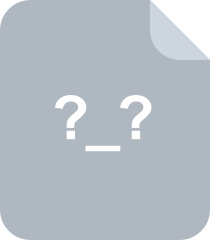
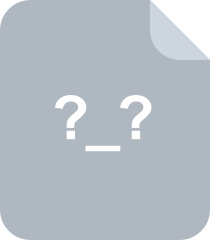
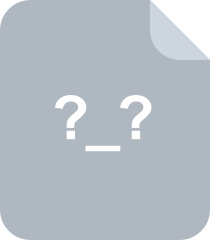
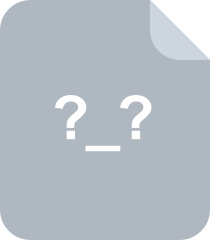
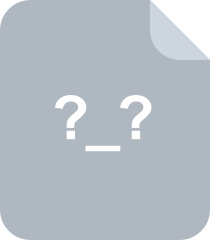
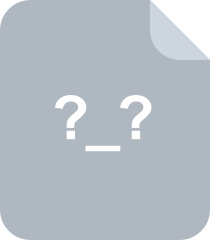
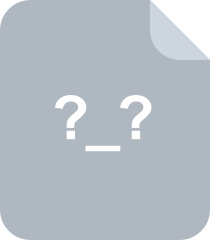
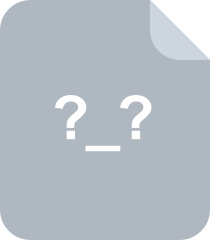
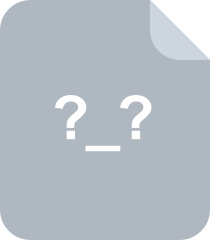
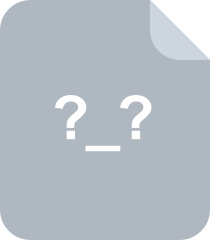
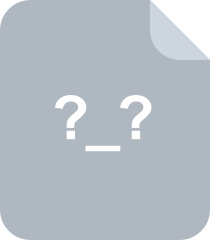
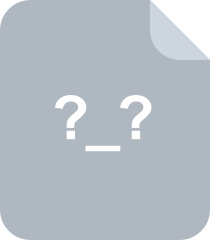
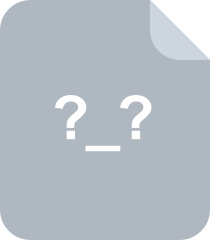
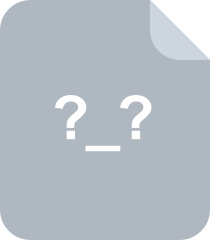
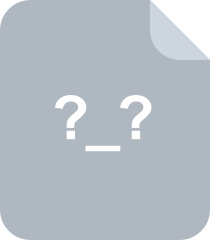
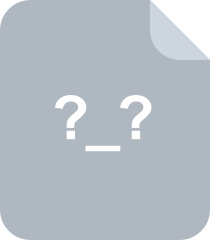
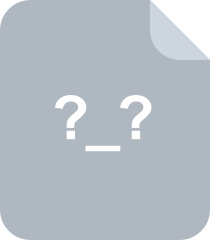
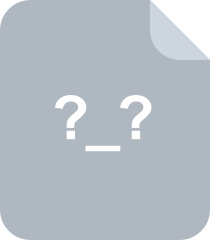
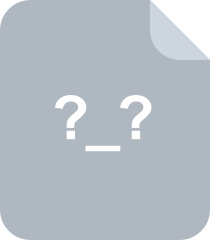
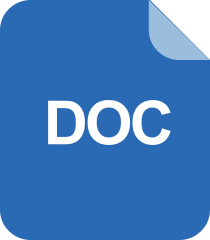
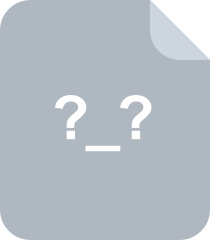
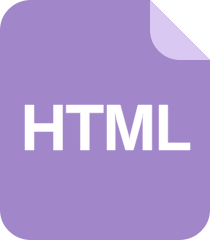
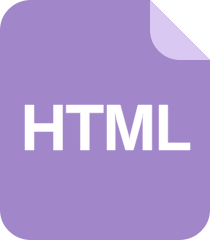
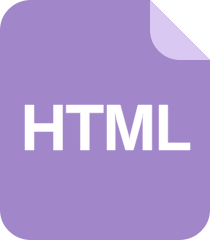
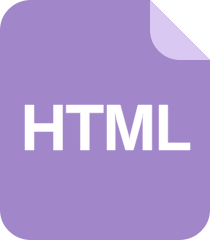
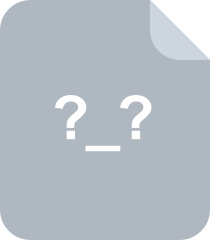
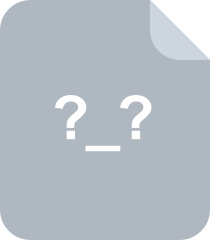
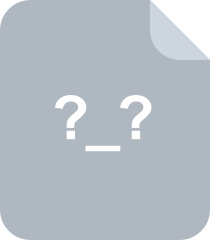
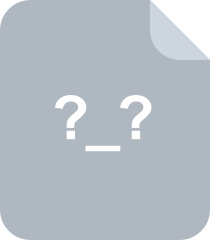
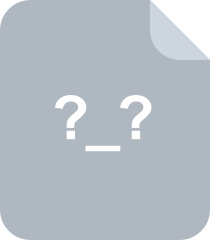
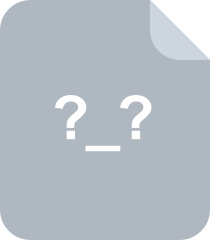
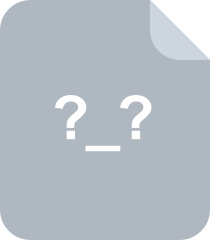
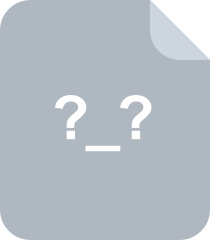
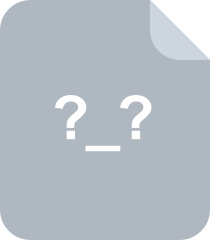
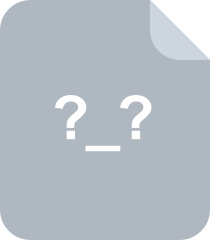
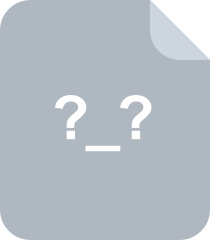
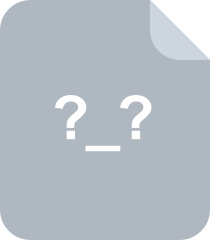
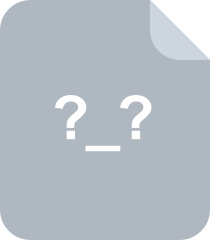
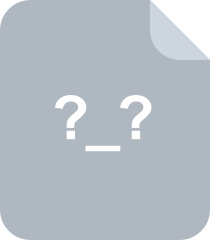
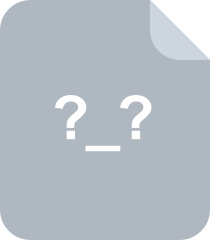
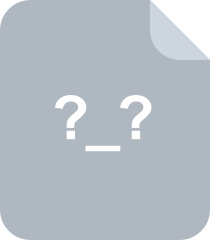
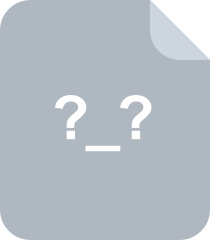
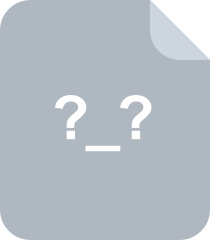
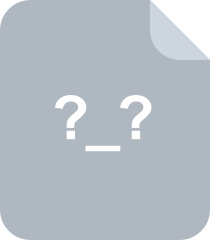
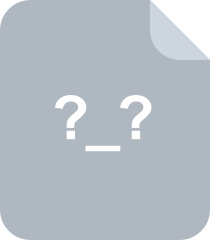
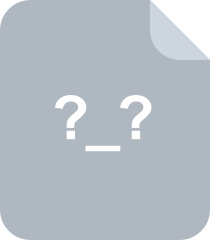
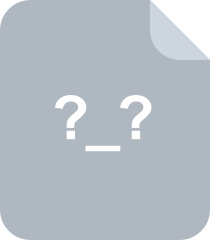
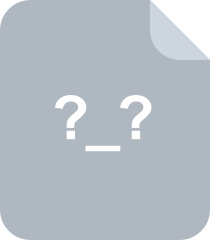
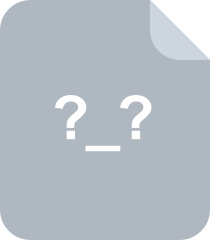
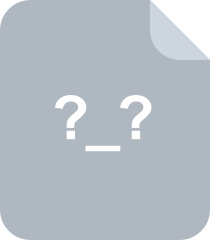
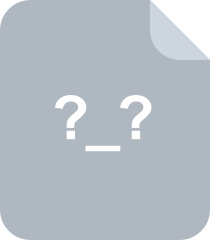
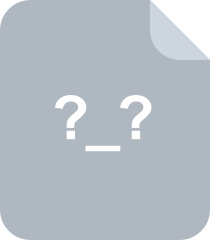
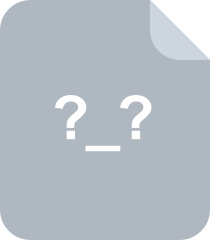
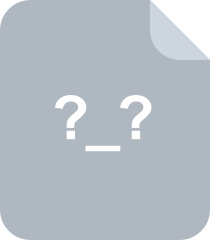
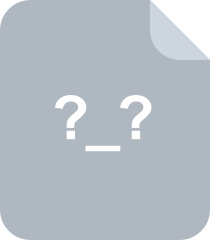
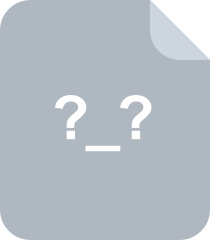
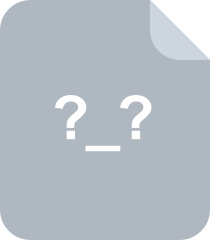
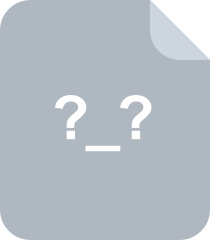
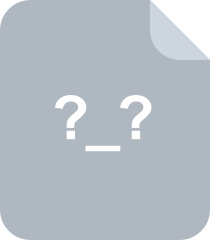
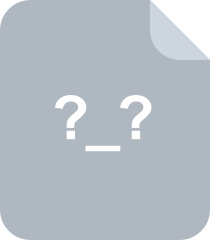
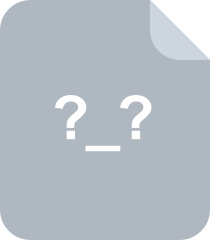
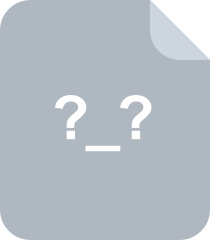
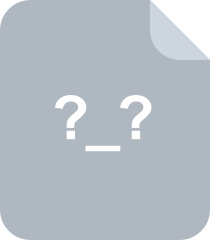
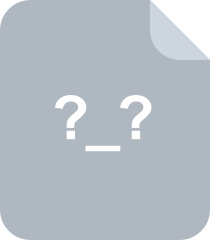
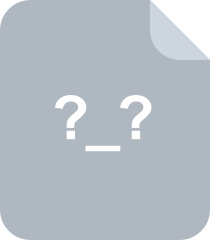
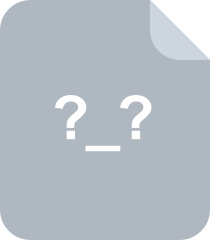
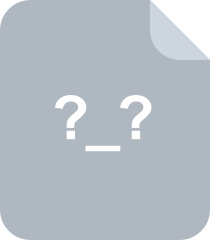
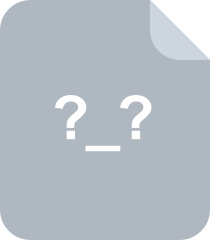
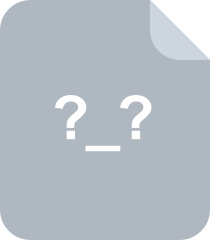
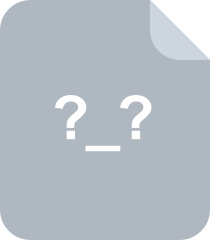
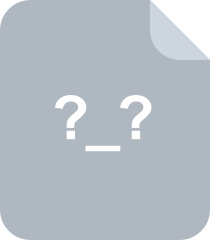
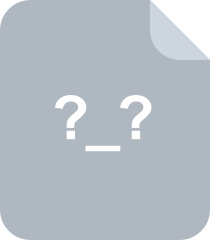
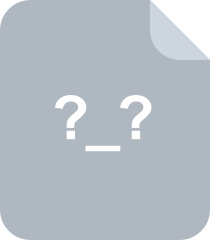
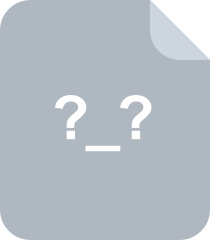
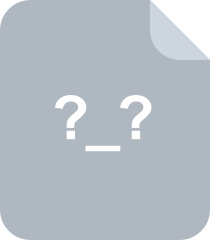
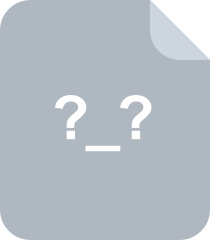
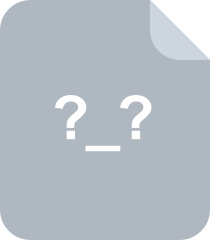
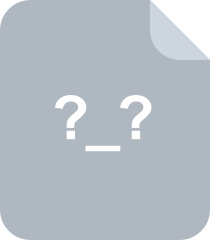
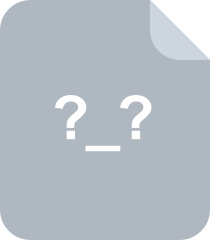
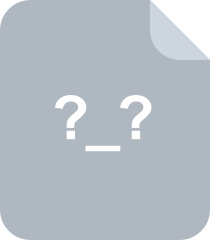
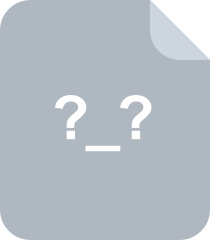
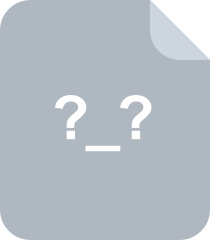
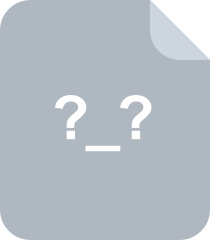
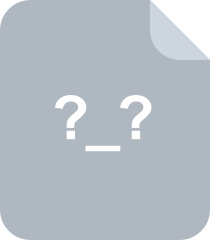
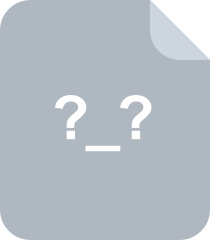
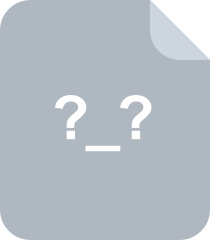
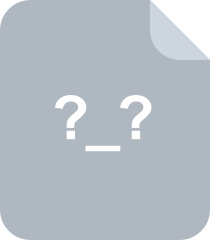
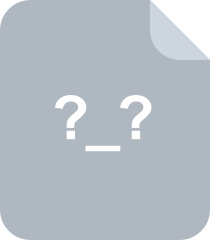
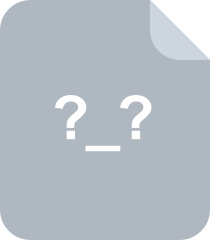
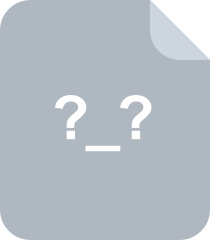
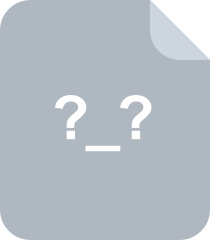
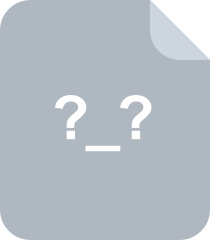
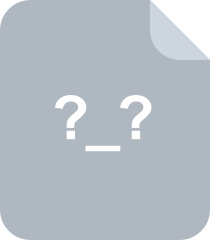
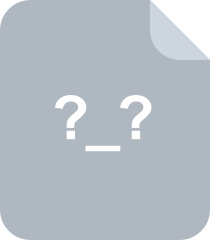
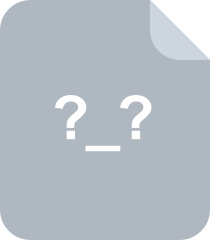
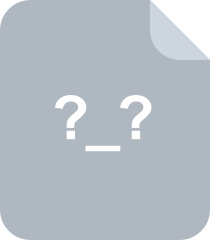
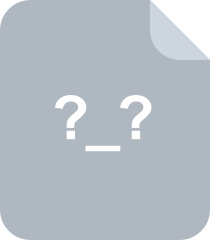
共 375 条
- 1
- 2
- 3
- 4
资源评论
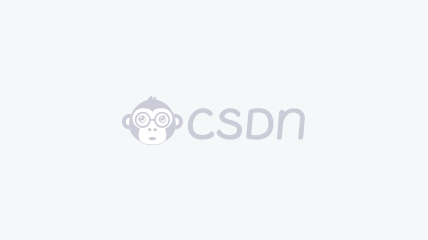

code.song
- 粉丝: 1094
- 资源: 1285
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

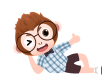
最新资源
- 白色清爽风格的企业网站模板下载.rar
- 白色清新大气的商务企业网站模板下载.rar
- 白色清新风格的HTML5个人简历模板.zip
- 白色清新风格的电商家具商城整站网站源码下载.zip
- 白色清新风格的多用途企业网站模板.rar
- 白色清新风格的工艺品展览馆企业网站模板下载.zip
- 白色清新风格的房地产官网模板下载.zip
- 白色清新风格的工作商务网页CSS模板下载.zip
- 白色清新风格的家具装修设计模板下载.zip
- 白色清新风格的家居公司网站模板下载.zip
- 白色清新风格的陌上花开博客模板下载.zip
- 白色清新风格的家政服务企业网站模板下载.zip
- 白色清新风格的律师法律企业网站模板.zip
- 白色清新风格的皮包设计网站模板下载.zip
- 白色清新风格的甜品网上商城模板.zip
- 白色清新风格的食谱烹饪HTML网站模板.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


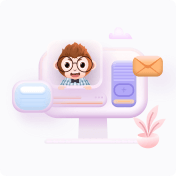
安全验证
文档复制为VIP权益,开通VIP直接复制
