package com.ideabobo.util;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.lang.reflect.Field;
import java.sql.Timestamp;
import java.util.Enumeration;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import javax.annotation.Resource;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import com.google.gson.GsonBuilder;
import org.apache.commons.io.FileUtils;
import org.apache.struts2.ServletActionContext;
import org.apache.struts2.dispatcher.SessionMap;
import org.apache.struts2.interceptor.ServletRequestAware;
import org.apache.struts2.interceptor.ServletResponseAware;
import org.apache.struts2.interceptor.SessionAware;
import com.google.gson.Gson;
import com.ideabobo.service.BaseService;
import com.opensymphony.xwork2.ActionSupport;
public class IdeaAction extends ActionSupport implements SessionAware,
ServletRequestAware, ServletResponseAware {
/**
*
*/
private static final long serialVersionUID = 6587353999198278262L;
public HttpServletResponse response;
public HttpServletRequest request;
public SessionMap<String,Object> session;
public Gson gson = new GsonBuilder().serializeNulls().create();
@Override
public void setServletResponse(HttpServletResponse response) {
// TODO Auto-generated method stub
this.response = response;
}
@Override
public void setServletRequest(HttpServletRequest request) {
// TODO Auto-generated method stub
this.request = request;
}
@Override
public void setSession(Map<String, Object> session) {
this.session = (SessionMap<String, Object>) session;
}
public void render(String mesg){
try {
response.setContentType("text/html;charset=UTF-8");
response.getWriter().print(mesg);
response.getWriter().flush();
response.getWriter().close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void renderJsonpString(String mesg){
Map obj = new HashMap();
obj.put("info", mesg);
String callbackFunctionName = request.getParameter("callback");
try {
response.setContentType("text/html;charset=UTF-8");
response.getWriter().print(callbackFunctionName+"("+gson.toJson(obj)+")");
response.getWriter().flush();
response.getWriter().close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void renderJsonpObj(Object json){
String callbackFunctionName = request.getParameter("callback");
try {
response.setContentType("text/html;charset=UTF-8");
response.getWriter().print(callbackFunctionName+"("+gson.toJson(json)+")");
response.getWriter().flush();
response.getWriter().close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public String encodeGet(String str){
if(str!=null){
try {
str = new String(str.getBytes("iso8859-1"),"utf-8");
} catch (UnsupportedEncodingException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return str;
}
public String getRequestEncode(String key){
String str = request.getParameter(key);
return encodeGet(str);
}
public Object getByRequest(Object model){
//Object model = c.getInterfaces();
Class c = model.getClass();
Map<String,String> map = new HashMap();
Map<String,String[]> parammap = request.getParameterMap();
for(Map.Entry<String, String[]> entry:parammap.entrySet()){
String[] value = entry.getValue();
if(value.length==1){
map.put(entry.getKey(),value[0]);
}
}
Field[] fields = c.getDeclaredFields();
try {
for(int i=0;i<fields.length;i++){
Field field = fields[i];
field.setAccessible(true);
String fname = field.getName();
String mvalue = map.get(fname);
if(mvalue != null && !mvalue.equals("") && !mvalue.equals("null")){
String ftype = field.getType().getSimpleName();
if(ftype.equals("String")){
field.set(model, mvalue);
}else if(ftype.equals("Integer") || ftype.equals("int")){
field.set(model, Integer.parseInt(mvalue));
}else if(ftype.equals("Double")){
field.set(model, Double.parseDouble(mvalue));
}else if(ftype.equals("Timestamp")){
Timestamp timestamp = Timestamp.valueOf(mvalue);
field.set(model,timestamp);
}
}
}
}catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return model;
}
public Object getByRequest(Object model,boolean jsonp){
//Object model = c.getInterfaces();
Class c = model.getClass();
Map<String,String> map = new HashMap();
Map<String,String[]> parammap = request.getParameterMap();
for(Map.Entry<String, String[]> entry:parammap.entrySet()){
String[] value = entry.getValue();
if(value.length==1){
map.put(entry.getKey(),value[0]);
}
}
Field[] fields = c.getDeclaredFields();
try {
for(int i=0;i<fields.length;i++){
Field field = fields[i];
field.setAccessible(true);
String fname = field.getName();
String mvalue = map.get(fname);
if(mvalue != null && !mvalue.equals("") && !mvalue.equals("null")){
String ftype = field.getType().getSimpleName();
if(ftype.equals("String")){
if(jsonp){
field.set(model, encodeGet(mvalue));
}else{
field.set(model, mvalue);
}
}else if(ftype.equals("Integer") || ftype.equals("int")){
field.set(model, Integer.parseInt(mvalue));
}else if(ftype.equals("Double")){
field.set(model, Double.parseDouble(mvalue));
}else if(ftype.equals("Timestamp")){
Timestamp timestamp = Timestamp.valueOf(mvalue);
field.set(model,timestamp);
}
}
}
}catch (IllegalAccessException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return model;
}
@Resource
private BaseService baseService;
/*******************************上传相关属性************************/
private File file; //上传的文件
private String fileFileName; //文件名称
private String fileContentType; //文件类型
public String uploadP(){
String realpath = ServletActionContext.getServletContext().getRealPath("/upload");
System.out.println("realpath: "+realpath);
String fname = "";
if (file != null) {
if(fileFileName!=null && fileFileName.contains(".")){
fname = UUID.randomUUID()+fileFileName.substring(fileFileName.lastIndexOf("."),fileFileName.length());
}else{
fname = UUID.randomUUID()+".jpg";
}
File savefile = new File(new File(realpath), fname);
if (!savefile.getParentFile().exists())
savefile.getParentFile().mkdirs();
try {
FileUtils.copyFile(file, savefile);
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
return fname;
}
public File getFile() {
return file;
}
public void setFile(File file) {
this.file = file;
}
public String getFileFileName() {
return fileFileName;
}
public void setFileFileName(String fileFileName) {
this.fileFileName = fileFileName;
}
public String getFileContentType() {
return fileContentType;
}
public void setFileContentType(String fileContentType) {
this.fileContentType = fileContentType;
}
/*******************************上传相关属性************************/
final String prefix="wct_";
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
(1)设计内容 本项目的面向对象为教学与学习《数据结构与算法》的老师、学生。目的是鼓励学生使用方便快捷的微信小程序,随时随地的进行课程的复习。 学生账户主要功能如下: a)用户登陆、退出 b)“我的”模块:个人信息设置、答题情况统计、错题记录、收藏题库、积分等。 c)“章节练习”模块:根据章节或整门课程进行题目的练习,可选择收藏题库。 d)“闯关答题”模块:可选择就每章或整门课程进行难易度由低到高的闯关答题练习。 教师账户主要功能如下: a)用户登陆、退出 b)“题目发布”模块:可发布题目、编辑题目、删除题目。 c)“题目统计”模块:可按章节统计各章的答题情况、错题率 d)“学习记录”模块:可按班级查看每位同学的做题记录、积分情况、积分排名 e)“我的”模块:个人信息设置、班级设置、名单录入
资源推荐
资源详情
资源评论
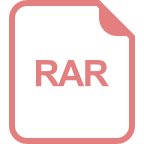
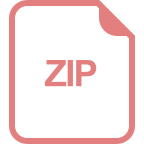
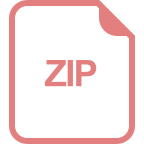
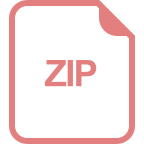
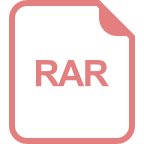
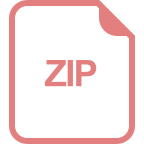
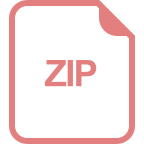
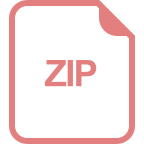
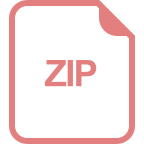
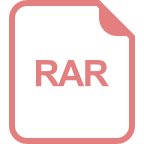
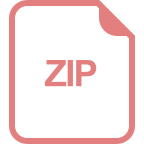
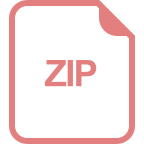
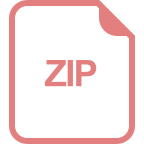
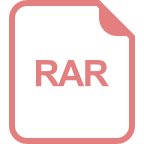
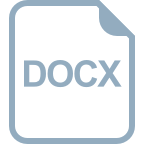
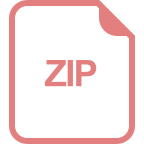
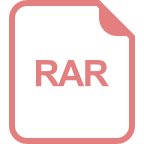
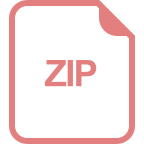
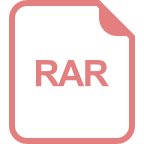
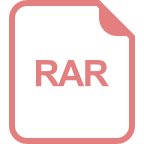
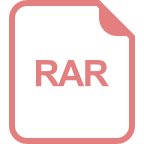
收起资源包目录

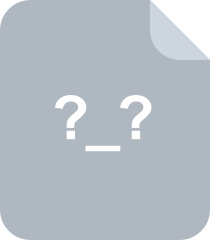
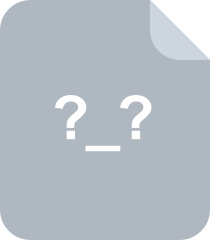
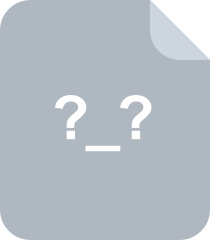
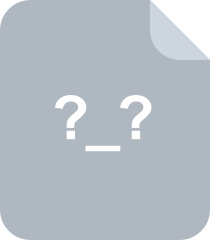
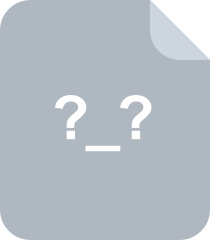
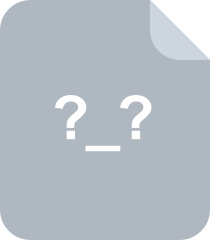
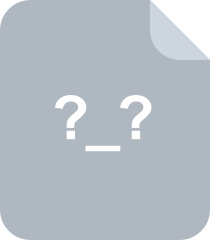
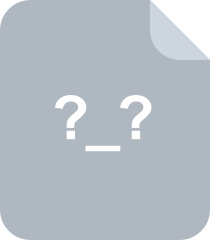
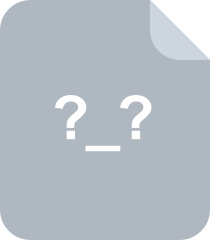
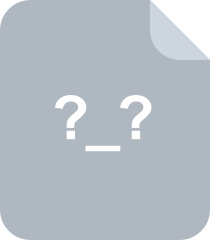
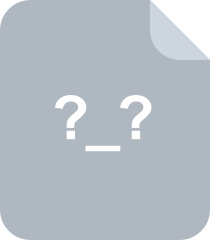
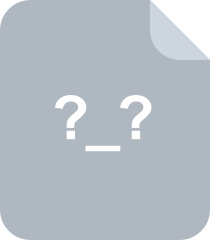
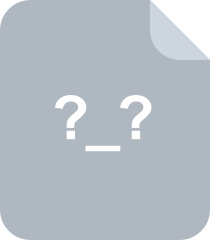
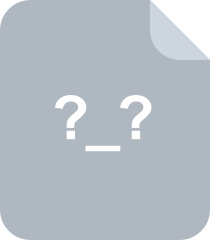
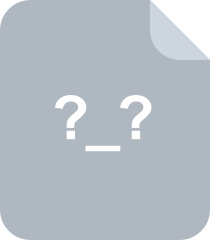
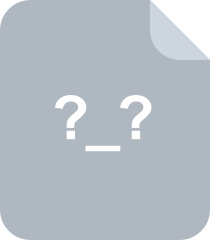
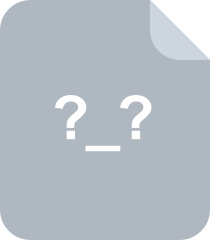
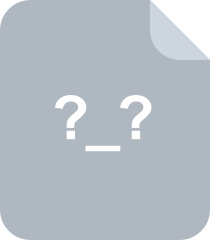
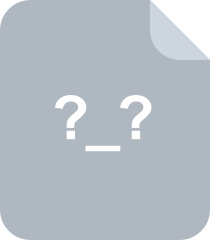
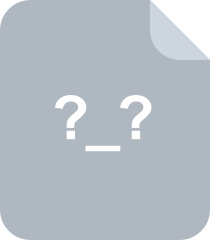
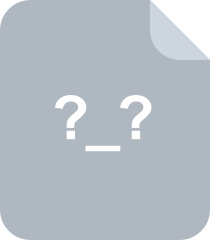
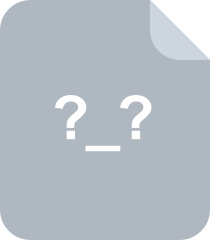
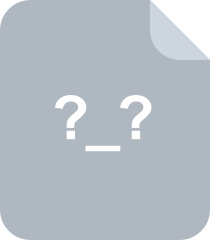
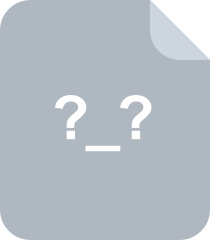
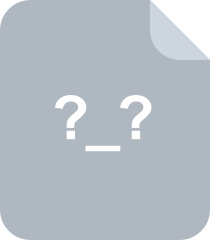
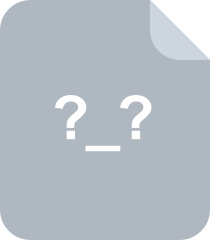
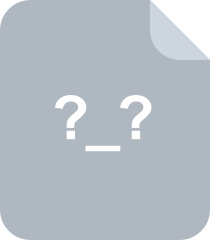
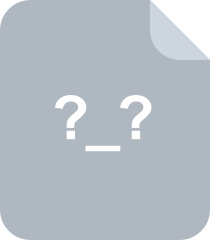
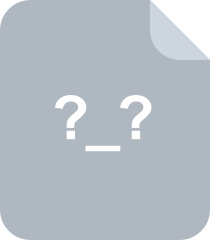
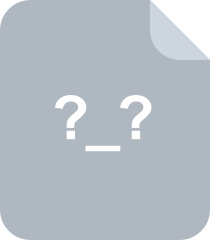
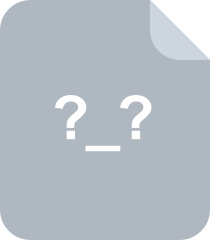
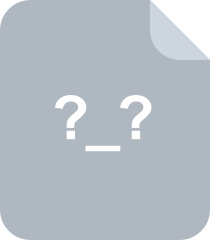
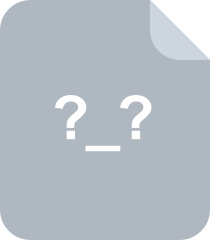
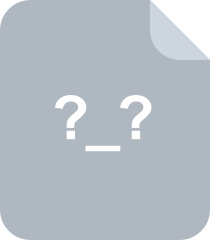
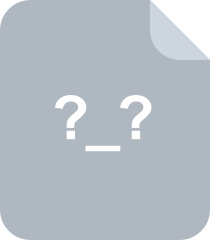
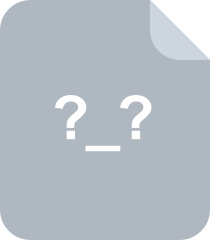
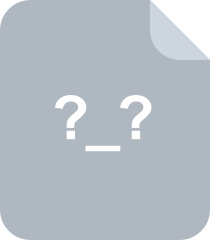
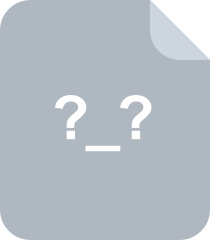
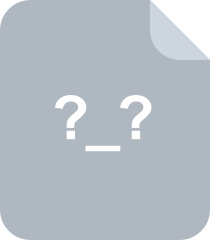
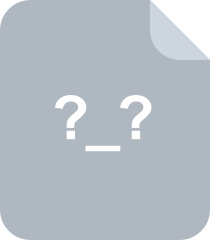
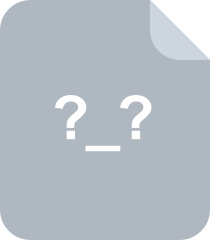
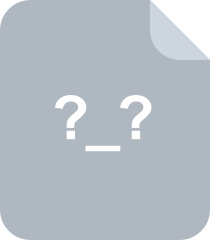
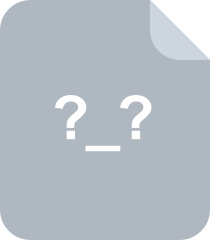
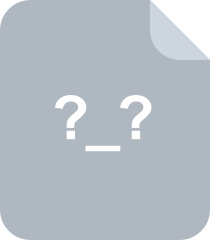
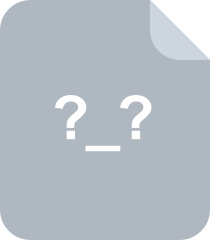
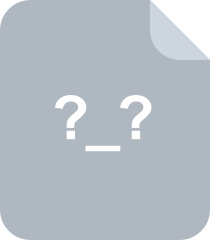
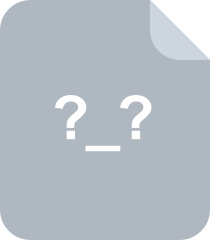
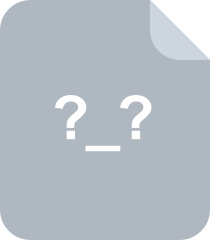
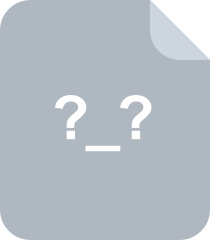
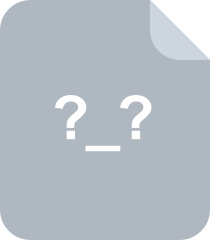
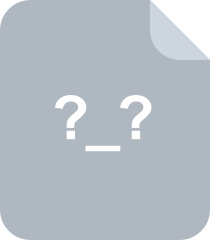
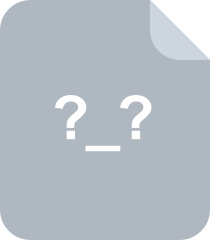
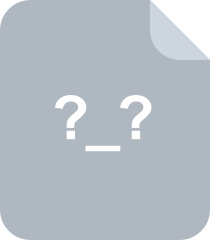
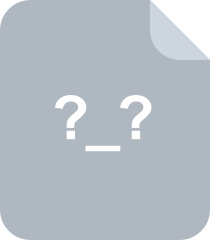
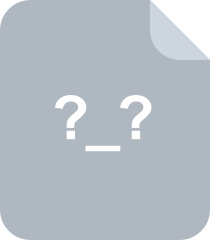
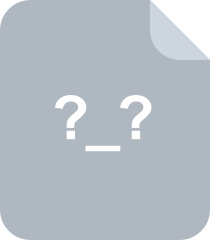
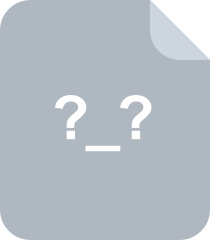
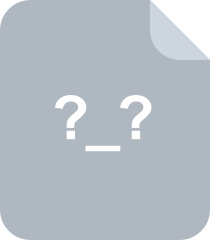
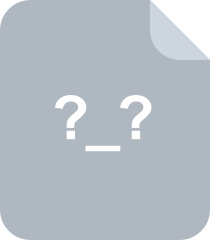
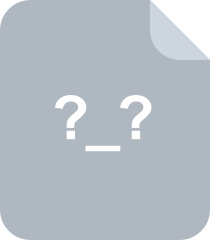
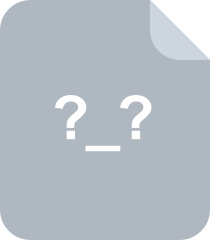
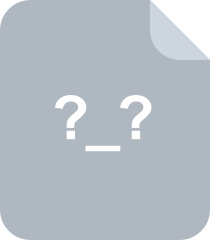
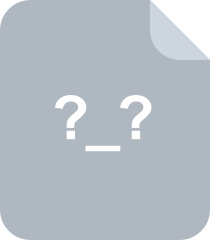
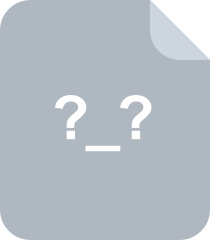
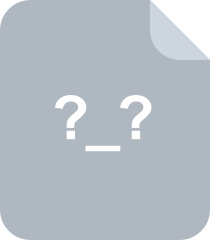
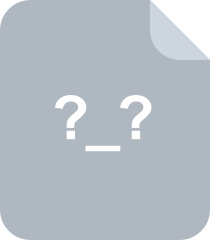
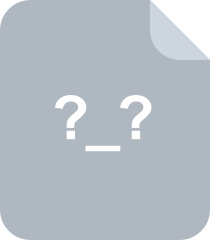
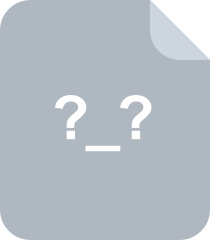
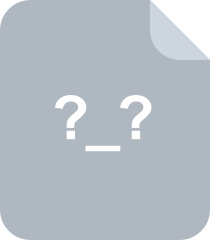
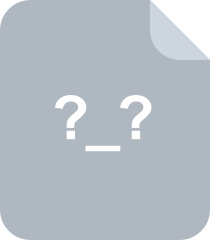
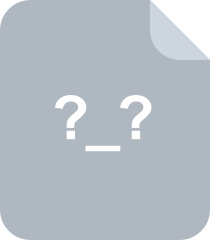
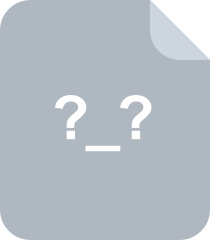
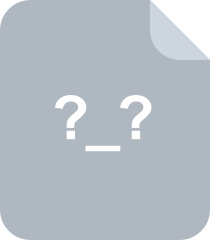
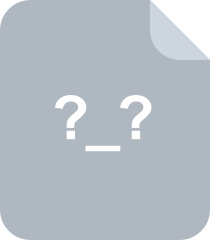
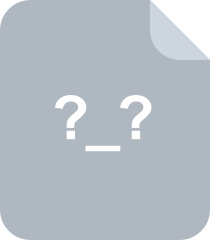
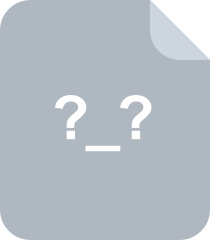
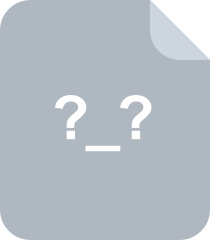
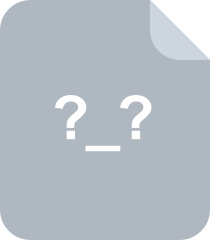
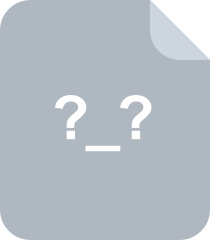
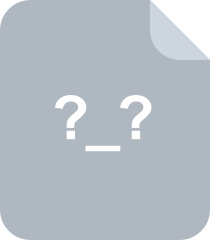
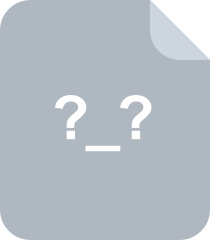
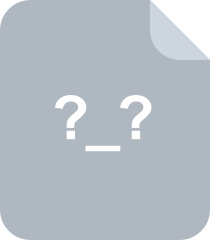
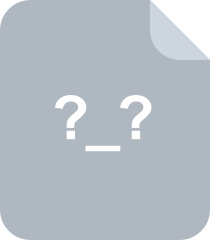
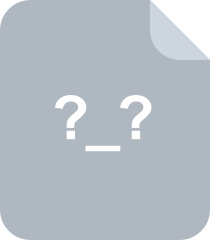
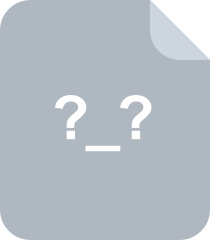
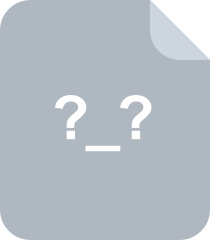
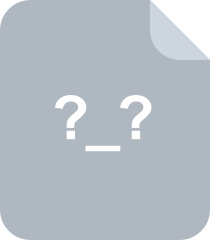
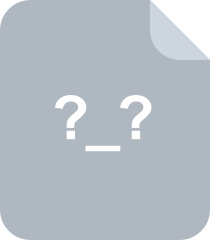
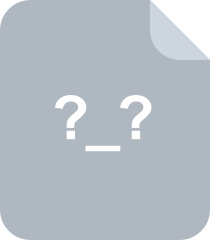
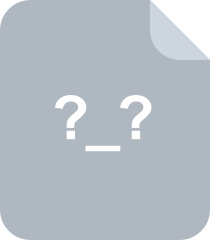
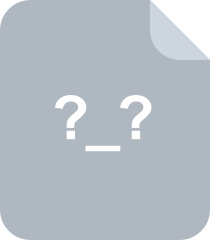
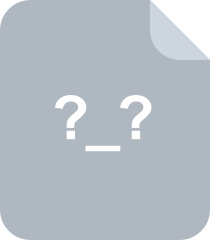
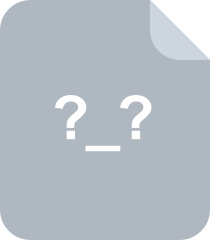
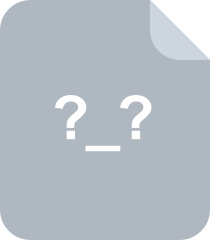
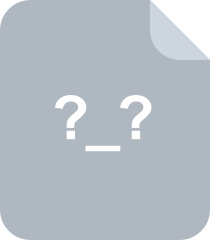
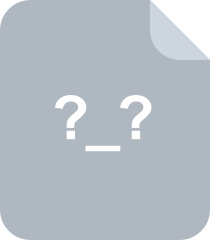
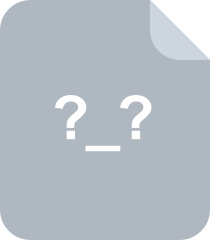
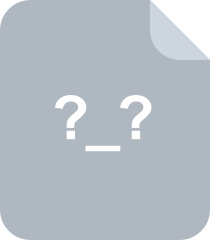
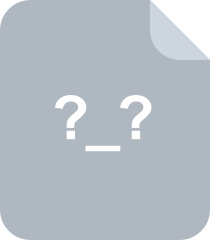
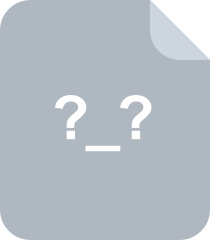
共 1426 条
- 1
- 2
- 3
- 4
- 5
- 6
- 15
资源评论
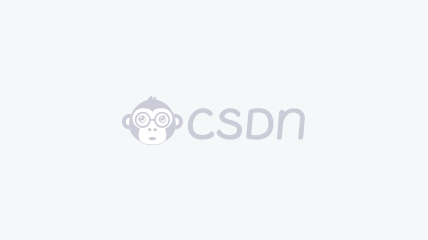

code.song
- 粉丝: 1076
- 资源: 1214

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

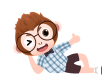
最新资源
- 基于树莓派的3D全息电子宠物嵌入式计算课程设计详细文档+全部资料+高分项目+源码.zip
- 基于指纹识别和指静脉识别技术的嵌入式门禁系统,DSP硬件平台详细文档+全部资料+高分项目+源码.zip
- FGT-80C-v400-build0458-FORTINET.out
- javascript各种算法源代码最全的算法技术资料.zip
- FGT-80C-v400-build0441-FORTINET.out
- 2025元旦倒计时雪花背景特效源码
- python-geohash-0.8.5-cp37-cp37m-win-amd64
- js各种算法源代码最全的算法技术资料.zip
- 实现财富自由的路径PPT
- go语言各种排序算法源代码最全的算法技术资料.zip
- 如何实现财富自由的分析PPT
- 电脑端微信自动锁定2.0
- 个人自我介绍、风采展示PPT
- python语法合集-python语法知识PDF
- Python数据可视化之Seaborn库详解与使用实例
- 俄罗斯大学录取数据集,大学招收数据集(5568行)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


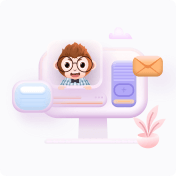
安全验证
文档复制为VIP权益,开通VIP直接复制
