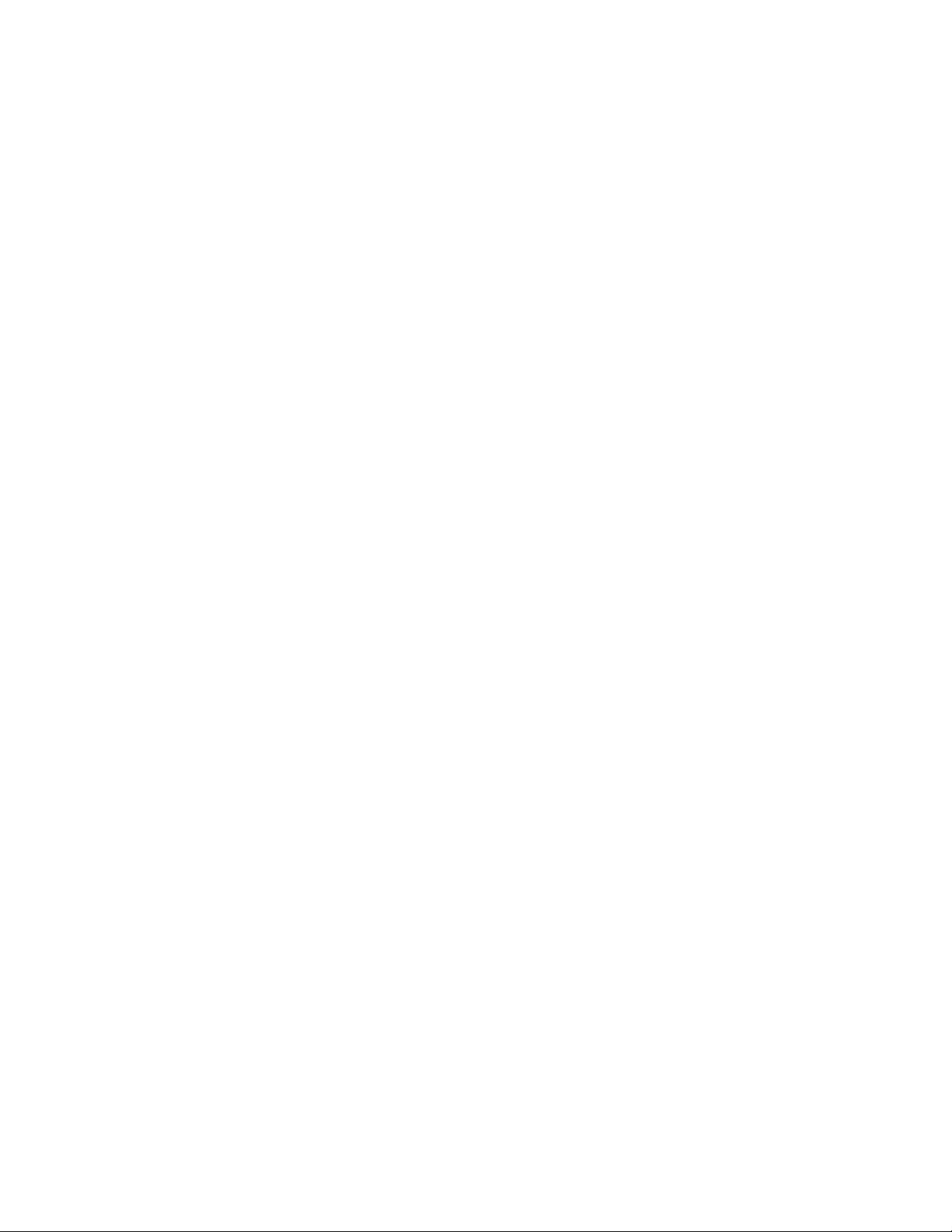
Doc. No. 2RDU00001 Rev C
Date: December 2005
TABLE OF CONTENTS
1 Introduction..............................................................................................................................7
2 Referenced Documents............................................................................................................8
3 General Design......................................................................................................................10
3.1 Coupling & Cohesion....................................................................................................11
3.2 Code Size and Complexity............................................................................................12
4 C++ Coding Standards...........................................................................................................13
4.1 Introduction....................................................................................................................13
4.2 Rules..............................................................................................................................13
4.2.1 Should, Will, and Shall Rules................................................................................13
4.2.2 Breaking Rules.......................................................................................................13
4.2.3 Exceptions to Rules...............................................................................................14
4.3 Terminology...................................................................................................................14
4.4 Environment..................................................................................................................17
4.4.1 Language................................................................................................................17
4.4.2 Character Sets........................................................................................................17
4.4.3 Run-Time Checks..................................................................................................18
4.5 Libraries.........................................................................................................................19
4.5.1 Standard Libraries..................................................................................................19
4.6 Pre-Processing Directives..............................................................................................20
4.6.1 #ifndef and #endif Pre-Processing Directives......................................................20
4.6.2 #define Pre-Processing Directive...........................................................................21
4.6.3 #include Pre-Processing Directive.........................................................................21
4.7 Header Files...................................................................................................................22
4.8 Implementation Files.....................................................................................................23
4.9 Style...............................................................................................................................23
4.9.1 Naming Identifiers.................................................................................................24
4.9.1.1 Naming Classes, Structures, Enumerated types and typedefs...........................25
4.9.1.2 Naming Functions, Variables and Parameters...................................................26
4.9.1.3 Naming Constants and Enumerators.................................................................26
4.9.2 Naming Files..........................................................................................................26
4.9.3 Classes...................................................................................................................27
4.9.4 Functions................................................................................................................27
4.9.5 Blocks....................................................................................................................28
4.9.6 Pointers and References.........................................................................................28
4.9.7 Miscellaneous........................................................................................................28
4.10 Classes...........................................................................................................................29
4.10.1 Class Interfaces......................................................................................................29
4.10.2 Considerations Regarding Access Rights..............................................................29
4.10.3 Member Functions.................................................................................................29
4.10.4 const Member Functions........................................................................................30
4.10.5 Friends...................................................................................................................30
4.10.6 Object Lifetime, Constructors, and Destructors....................................................30
4.10.6.1 Object Lifetime..............................................................................................30
4.10.6.2 Constructors...................................................................................................31
4.10.6.3 Destructors.....................................................................................................32
3