package com.comdosoft.qxg;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Timer;
import java.util.TimerTask;
import com.comdosoft.pojo.DownViewPojo;
import android.app.Activity;
import android.app.DownloadManager;
import android.app.DownloadManager.Request;
import android.database.Cursor;
import android.net.Uri;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.LayoutInflater;
import android.view.View;
import android.view.View.OnClickListener;
import android.webkit.MimeTypeMap;
import android.widget.Button;
import android.widget.LinearLayout;
import android.widget.ProgressBar;
import android.widget.RelativeLayout;
import android.widget.TextView;
import android.widget.Toast;
public class Qixueguan_appActivity extends Activity {
private static int MB_SIZE = 1024 * 1024;
private static int KB_SIZE = 1024;
private LinearLayout mLinearLayout;
private DownloadManager downloadManager;
private List<DownViewPojo> mDownViewList = new ArrayList<DownViewPojo>();
private List<String> mUrlList = new ArrayList<String>();
private List<Map<String, Object>> mData;
private Handler handler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
int msgId = msg.what;
switch (msgId) {
case 1:
DownViewPojo dv = mDownViewList.get(msg.getData().getInt(
"index"));
dv.getPb().setMax(msg.arg2);
dv.getPb().setProgress(msg.arg1);
dv.getSize().setText(
getFileSize(msg.arg1) + "/" + getFileSize(msg.arg2));
dv.getPro().setText(msg.arg1 * 100 / msg.arg2 + "%");
if (msg.arg1 == msg.arg2) {
Toast.makeText(getApplicationContext(),
"文件" + msg.getData().getInt("index") + "下载完成", 0)
.show();
dv.getBn().setText("已下载");
dv.getTimer().cancel();
}
break;
}
}
};
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
initData();
}
private void initData() {
downloadManager = (DownloadManager) getSystemService(DOWNLOAD_SERVICE);
mLinearLayout = (LinearLayout) findViewById(R.id.mLinearLayout);
mUrlList.add("http://music.baidu.com/data/music/file?link=http://zhangmenshiting.baidu.com/data2/music/44804430/733833686400256.mp3?xcode=b6941d7fea0365339abd5bdf376d47f3959a2ceab6004476");
mUrlList.add("http://music.baidu.com/data/music/file?link=http://zhangmenshiting.baidu.com/data2/music/35468028/2355901379365261320.mp3?xcode=bf64667c54c02224b9ce27303f1db07269eae4df0fb501ff");
mUrlList.add("http://music.baidu.com/data/music/file?link=http://zhangmenshiting.baidu.com/data2/music/57129486/2658981379350861256.mp3?xcode=39cb9a27958605ddf9dd2bec60984cad0d620aa2c069e25d");
for (int i = 0; i < mUrlList.size(); i++) {
initView(i);
}
}
public void initView(final int index) {
LayoutInflater inflater = LayoutInflater.from(this);
RelativeLayout relativeLayout = (RelativeLayout) inflater.inflate(
R.layout.item, null);
TextView pro = (TextView) relativeLayout.findViewById(R.id.downPro);
TextView size = (TextView) relativeLayout.findViewById(R.id.downSize);
ProgressBar pb = (ProgressBar) relativeLayout.findViewById(R.id.downPb);
Button bn = (Button) relativeLayout.findViewById(R.id.downBn);
DownViewPojo dv = new DownViewPojo(pro, size, pb, bn);
mDownViewList.add(dv);
bn.setOnClickListener(new OnClickListener() {
public void onClick(View v) {
downCourse(index);
}
});
mLinearLayout.addView(relativeLayout);
}
public void downCourse(final int index) {
// 开始下载
Uri resource = Uri.parse(mUrlList.get(index));
DownloadManager.Request request = new DownloadManager.Request(resource);
request.setAllowedNetworkTypes(Request.NETWORK_MOBILE
| Request.NETWORK_WIFI);
request.setAllowedOverRoaming(false);
// 设置文件类型
MimeTypeMap mimeTypeMap = MimeTypeMap.getSingleton();
String mimeString = mimeTypeMap.getMimeTypeFromExtension(MimeTypeMap
.getFileExtensionFromUrl(mUrlList.get(index)));
request.setMimeType(mimeString);
// 在通知栏中显示
request.setShowRunningNotification(false);
request.setVisibleInDownloadsUi(false);
// sdcard的目录下的download文件夹
request.setDestinationInExternalPublicDir("/download/", index + ".mp3");
final long id = downloadManager.enqueue(request);
Timer t = new Timer();
mDownViewList.get(index).setTimer(t);
t.schedule(new TimerTask() {
@Override
public void run() {
// 保存id
int[] bytesArr = getBytesAndStatus(id);
Bundle bundle = new Bundle();
bundle.putInt("index", index);
Message msg = handler.obtainMessage(1, bytesArr[0],
bytesArr[1], bytesArr[2]);
msg.setData(bundle);
handler.sendMessage(msg);
};
}, 0, 1000);
}
public int[] getBytesAndStatus(long downloadId) {
int[] bytesAndStatus = new int[] { -1, -1, 0 };
DownloadManager.Query query = new DownloadManager.Query()
.setFilterById(downloadId);
Cursor c = null;
try {
c = downloadManager.query(query);
if (c != null && c.moveToFirst()) {
bytesAndStatus[0] = c
.getInt(c
.getColumnIndexOrThrow(DownloadManager.COLUMN_BYTES_DOWNLOADED_SO_FAR));
bytesAndStatus[1] = c
.getInt(c
.getColumnIndexOrThrow(DownloadManager.COLUMN_TOTAL_SIZE_BYTES));
bytesAndStatus[2] = c.getInt(c
.getColumnIndex(DownloadManager.COLUMN_STATUS));
}
} finally {
if (c != null) {
c.close();
}
}
return bytesAndStatus;
}
public String getFileSize(long size) {
if (size <= 0) {
return "0M";
}
if (size >= MB_SIZE) {
return ((((double) (size * 100 / MB_SIZE)) / 100) + "M");
} else if (size >= KB_SIZE) {
return (((double) (size * 100 / KB_SIZE)) / 100 + "K");
} else {
return size + "B";
}
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
Android 多线程下载+UI进度条刷新
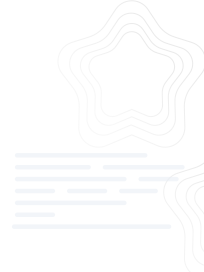
共66个文件
class:40个
xml:8个
png:6个


温馨提示
http://blog.csdn.net/sky286753213/article/details/11770299
资源推荐
资源详情
资源评论
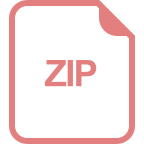
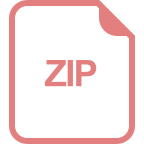
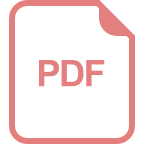
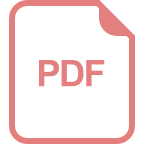
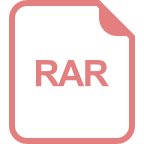
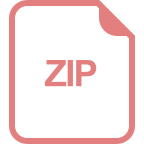
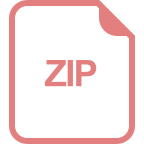
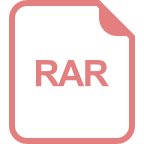
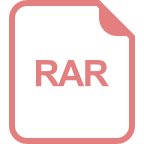
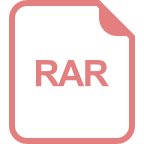
收起资源包目录



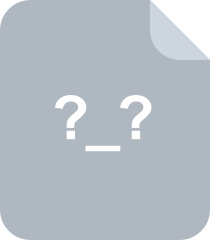


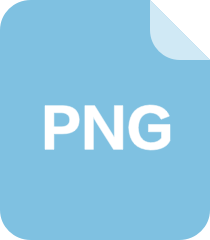

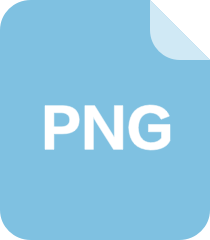

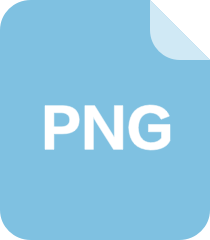
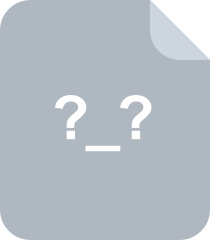




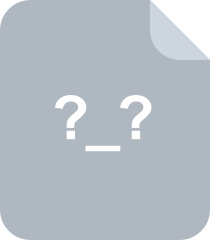

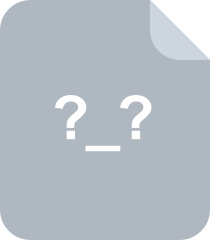
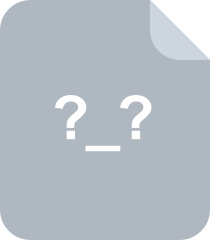
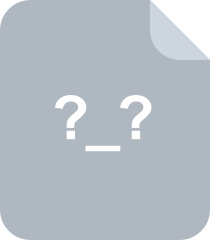
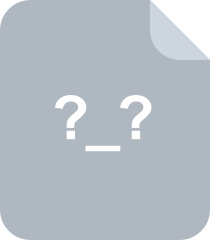
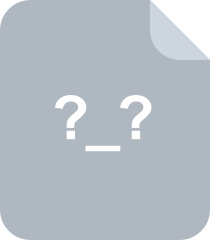
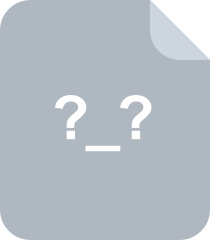
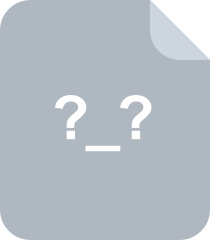
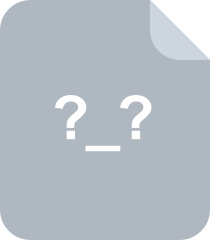
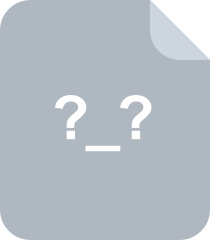
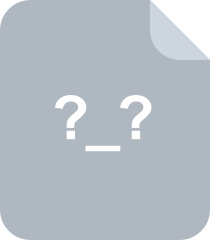
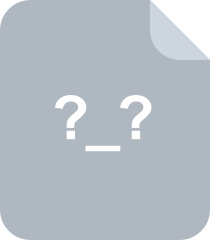
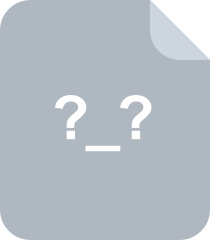
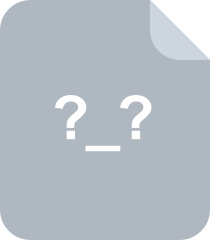
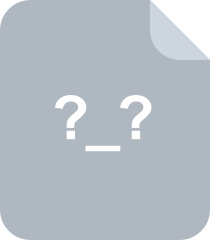


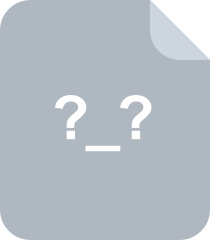
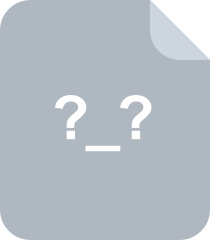
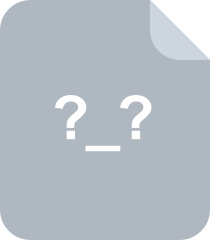
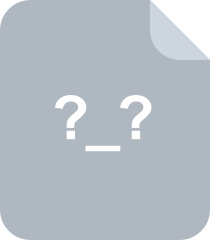
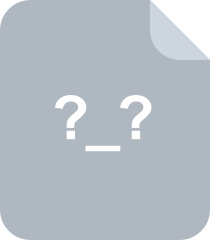
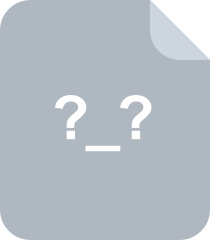

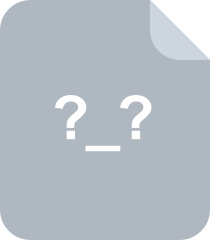
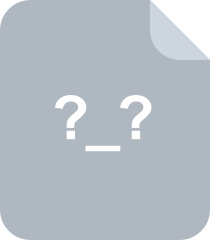

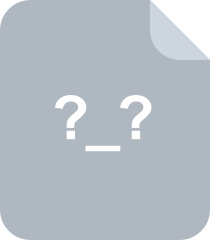
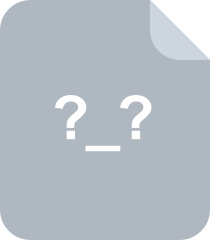

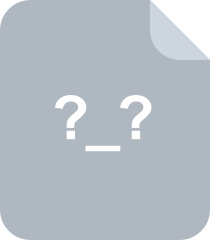
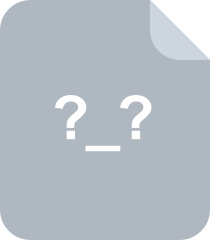
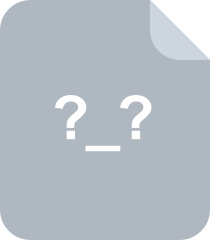
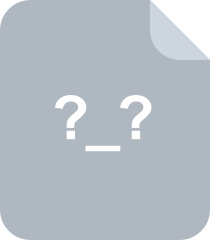
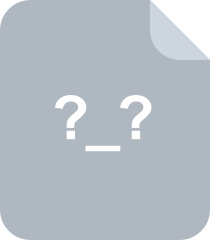
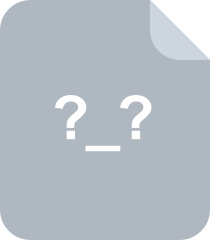
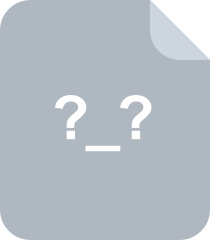
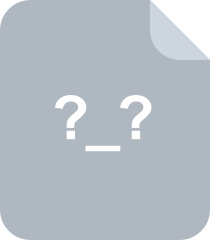
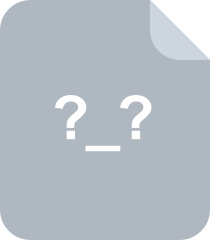
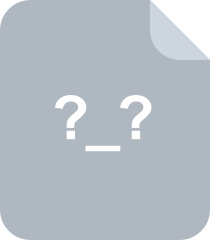
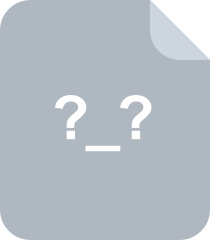
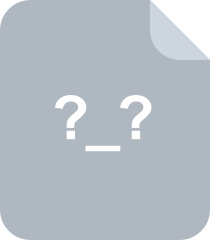
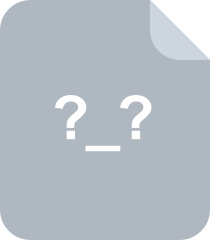
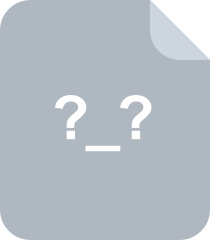

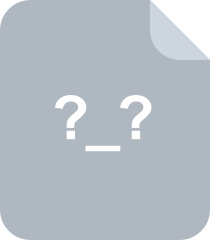
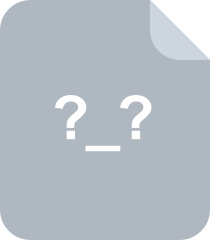


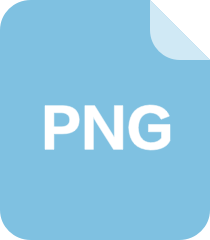

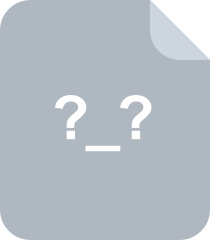

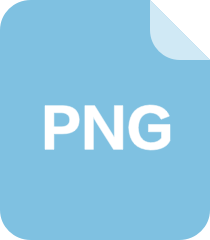

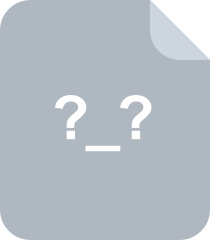
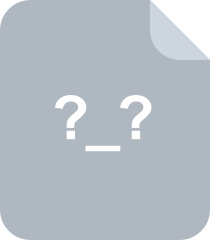
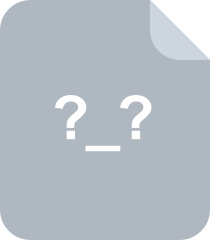

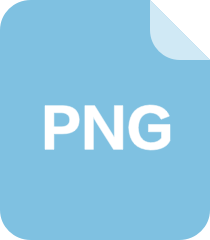

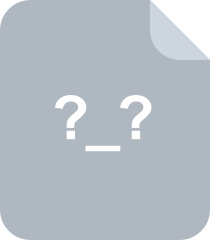
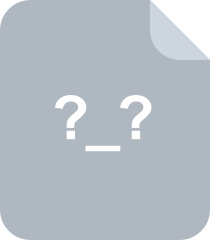
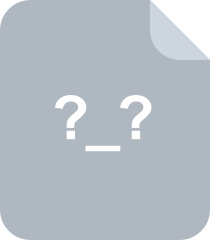
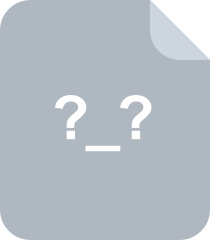





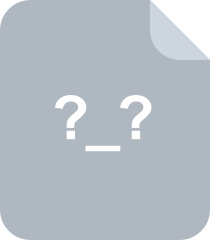
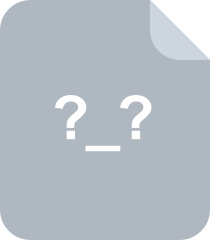

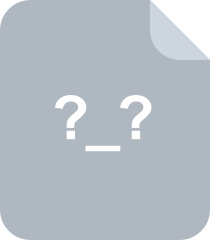




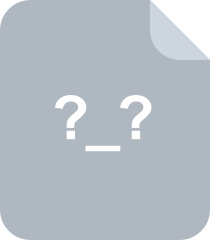

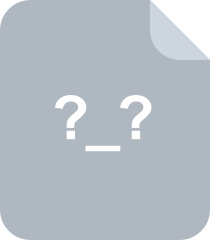
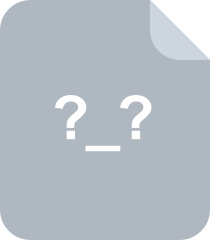
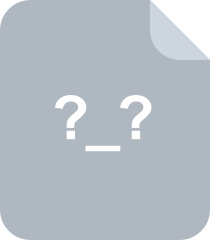
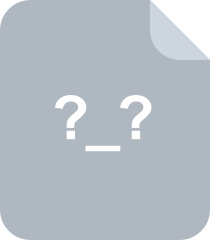
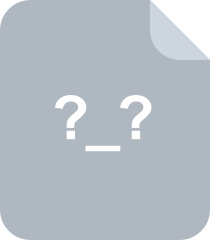
共 66 条
- 1
资源评论
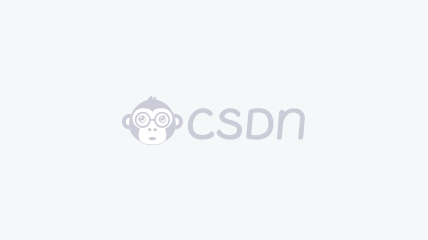
- zjl54392014-03-17不错的例子,学习啦
- andy的逆袭历程2014-06-12很好啊,我自己做了一些噶东,很符合
- nianfanfan2015-08-28不错的例子,对我的开发很有帮助
- huyao80232017-10-31额,就是代码有点过时了

风无形_
- 粉丝: 21
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

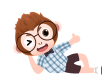
安全验证
文档复制为VIP权益,开通VIP直接复制
