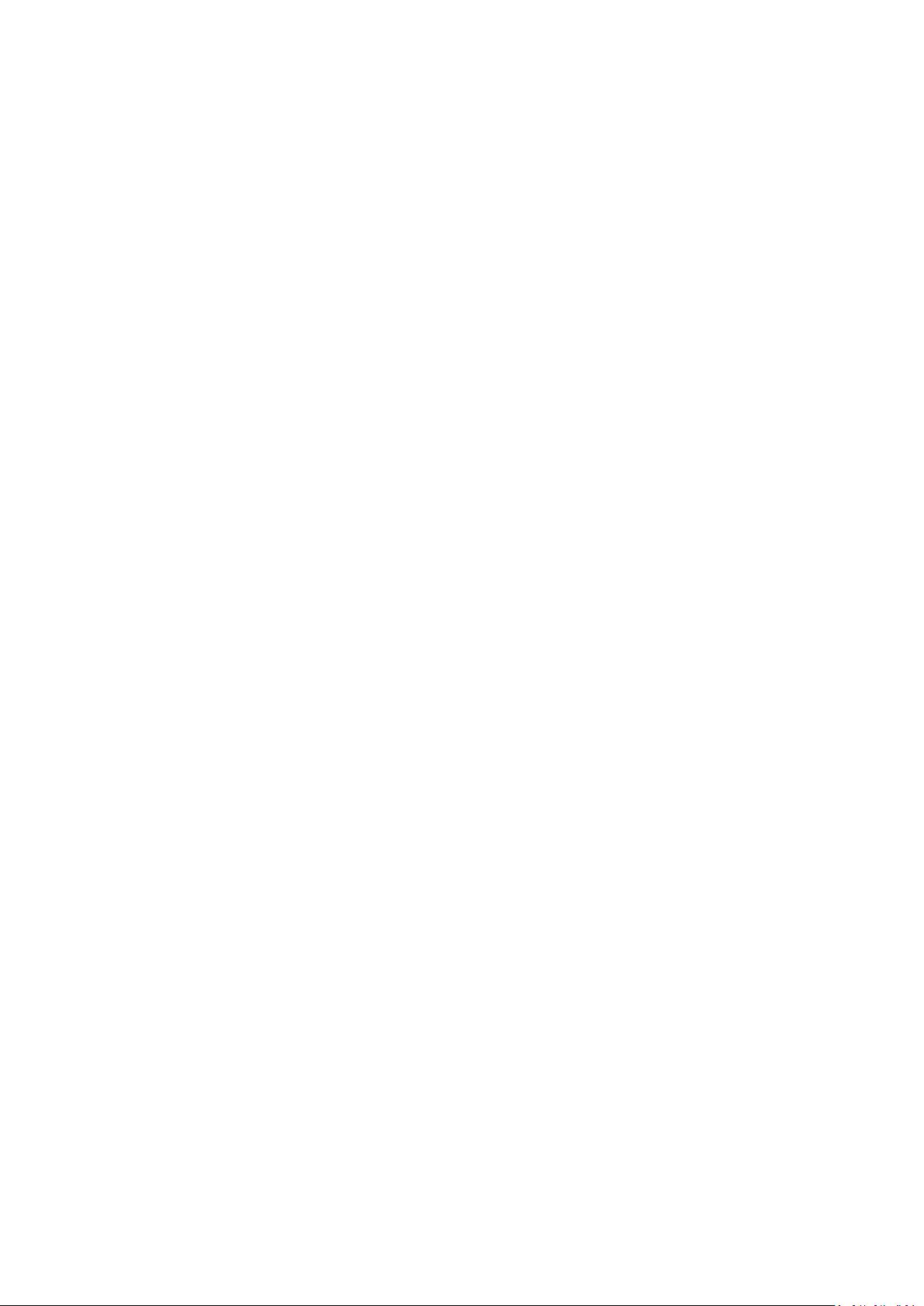
Exceptional C++ Style: New
Engineering Puzzles,
Programming Problems, and
Solutions
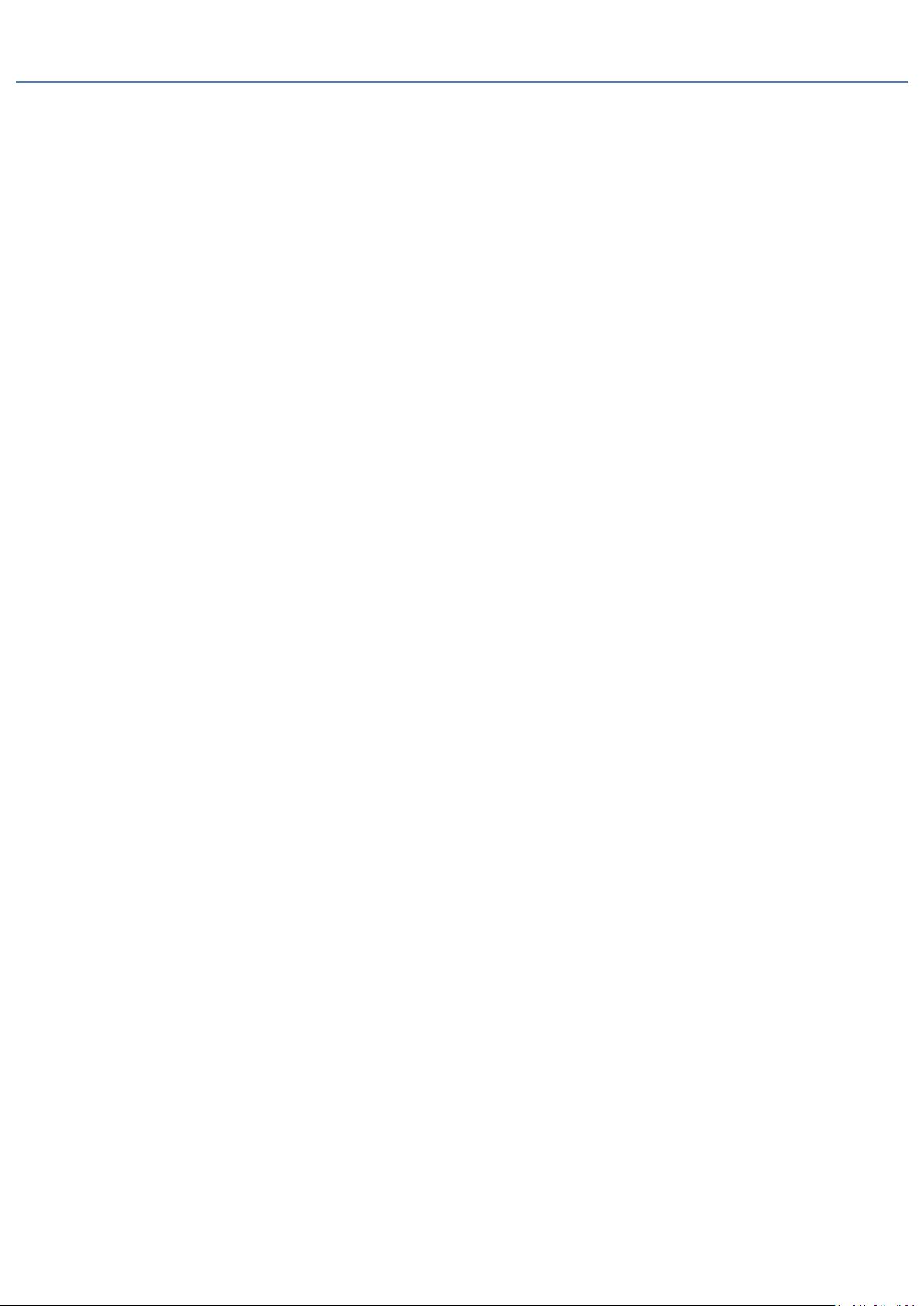
Exceptional C++ Style: New Engineering Puzzles, Programming Problems, and Solutions
1
Table of Contents
1. Table of Contents ...................................................................................................................... 5
2. Preface ...................................................................................................................................... 8
2.1 Style or Substance? ............................................................................................................. 9
2.2 The Exceptional Socrates .................................................................................................. 11
2.3 What I assume You Know ................................................................................................. 13
2.4 How to Read This Book ..................................................................................................... 13
2.5 ##. The Topic of This Item ................................................................................................. 14
2.6 Acknowledgments............................................................................................................. 15
3. Part 1 - Generic Programming and the C++ Standard Library ................................................ 16
3.1 Chapter 1. Uses and Abuses of vector .............................................................................. 17
3.1.1 Solution ...................................................................................................................... 18
3.2 Chapter 2. The String Formatters of Manor Farm, Part 1: sprintf .................................... 25
3.2.1 Solution ...................................................................................................................... 26
3.3 Chapter 3. The String Formatters of Manor Farm, Part 2: Standard (or Blindingly Elegant)
Alternatives ............................................................................................................................. 31
3.3.1 Solution ...................................................................................................................... 32
3.4 Chapter 4. Standard Library Member Functions .............................................................. 43
3.4.1 Solution ...................................................................................................................... 44
3.5 Chapter 5. Flavors of Genericity, Part 1: Covering the Basis [sic] ..................................... 47
3.5.1 Solution ...................................................................................................................... 48
3.6 Chapter 6. Flavors of Genericity, Part 2: Generic Enough? .............................................. 51
3.6.1 Solution ...................................................................................................................... 52
3.7 Chapter 7. Why Not Specialize Function Templates? ....................................................... 58
3.7.1 Solution ...................................................................................................................... 59
3.8 Chapter 8. Befriending Templates .................................................................................... 65
3.8.1 Solution ...................................................................................................................... 67
3.9 Chapter 9. Export Restrictions, Part 1: Fundamentals ...................................................... 77
3.9.1 Solution ...................................................................................................................... 77
3.9.2 A Tale of Two Models ................................................................................................ 78
3.9.3 Illustrating the Issues ................................................................................................. 80
3.9.4 Export InAction [sic] ................................................................................................... 81
3.9.5 Issue the First: Source Exposure ................................................................................ 83
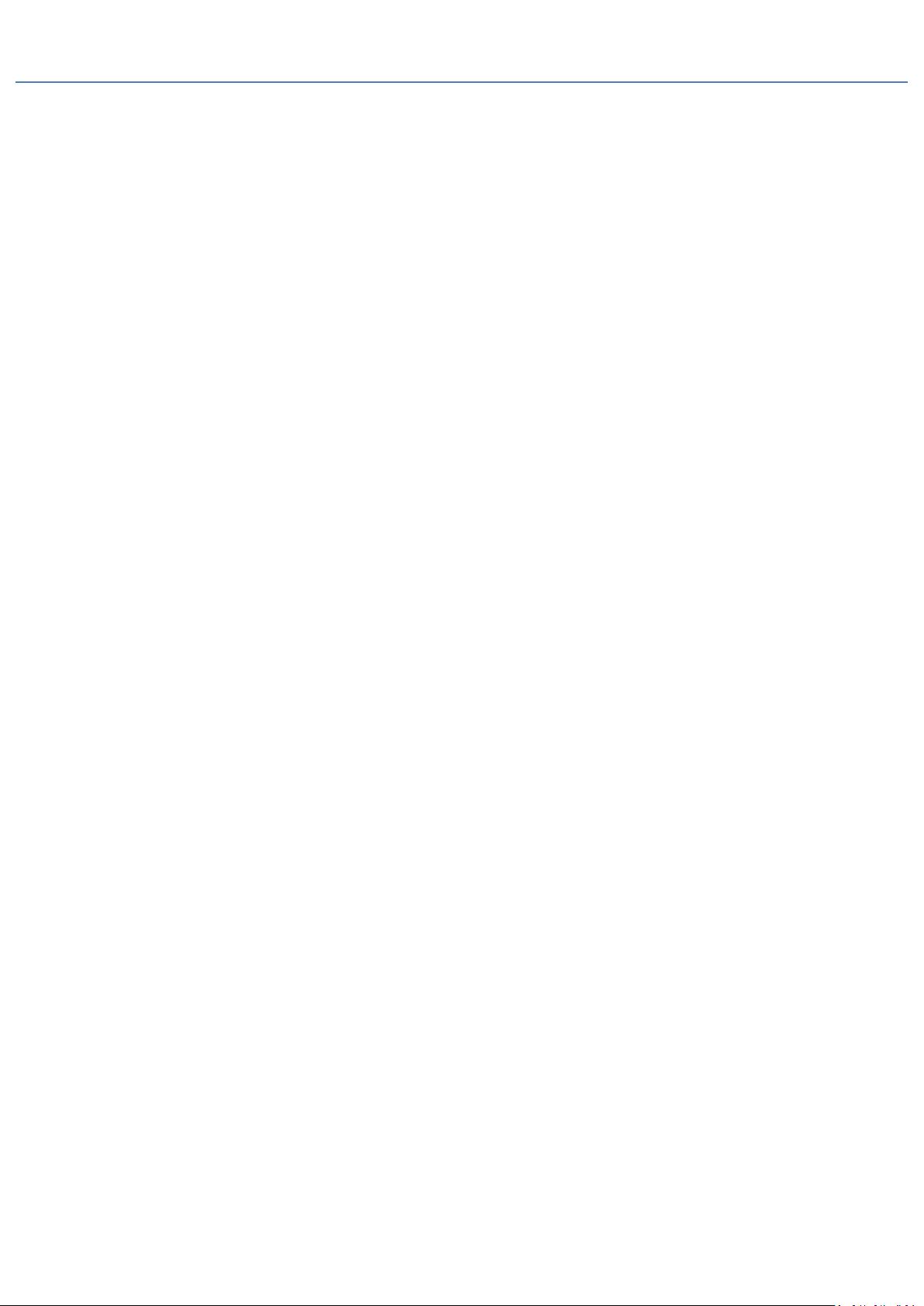
Exceptional C++ Style: New Engineering Puzzles, Programming Problems, and Solutions
2
3.9.6 Issue the Second: Dependencies and Build Times ..................................................... 85
3.9.7 Summary .................................................................................................................... 86
3.10 Chapter 10. Export Restrictions, Part 2: Interactions, Usability Issues, and Guidelines . 87
3.10.1 Solution .................................................................................................................... 88
4. Part 2 - Exception Safety Issues and Techniques .................................................................... 97
4.1 Chapter 11. Try and Catch Me .......................................................................................... 98
4.1.1 Solution ...................................................................................................................... 99
4.2 Chapter 12. Exception Safety: Is It Worth It?.................................................................. 103
4.2.1 Solution .................................................................................................................... 104
4.3 Chapter 13. A Pragmatic Look at Exception Specifications ............................................ 106
4.3.1 Solution .................................................................................................................... 108
5. Part 3 - Class Design, Inheritance, and Polymorphism ......................................................... 116
5.1 Chapter 14. Order, Order! .............................................................................................. 117
5.1.1 Solution .................................................................................................................... 118
5.2 Chapter 15. Uses and Abuses of Access Rights ............................................................... 121
5.2.1 Solution .................................................................................................................... 122
5.3 Chapter 16. (Mostly) Private ........................................................................................... 127
5.3.1 Solution .................................................................................................................... 128
5.4 Chapter 17. Encapsulation .............................................................................................. 135
5.4.1 Solution .................................................................................................................... 137
5.5 Chapter 18. Virtuality ...................................................................................................... 145
5.5.1 Solution .................................................................................................................... 146
5.6 Chapter 19. Enforcing Rules for Derived Classes ............................................................ 154
5.6.1 Solution .................................................................................................................... 156
6. Part 4 - Memory and Resource Management ...................................................................... 166
6.1 Chapter 20. Containers in Memory, Part 1: Levels of Memory Management ............... 167
6.1.1 Solution .................................................................................................................... 168
6.2 Chapter 21. Containers in Memory, Part 2: How Big Is It Really?................................... 171
6.2.1 Solution .................................................................................................................... 172
6.3 Chapter 22. To new, Perchance to tHRow, Part 1: The Many Faces of new .................. 178
6.3.1 Solution .................................................................................................................... 180
6.3.2 In-Place, Plain, and Nothrow new ............................................................................ 180
6.3.3 Class-Specific new .................................................................................................... 183
6.3.4 A Name-Hiding Surprise ........................................................................................... 184
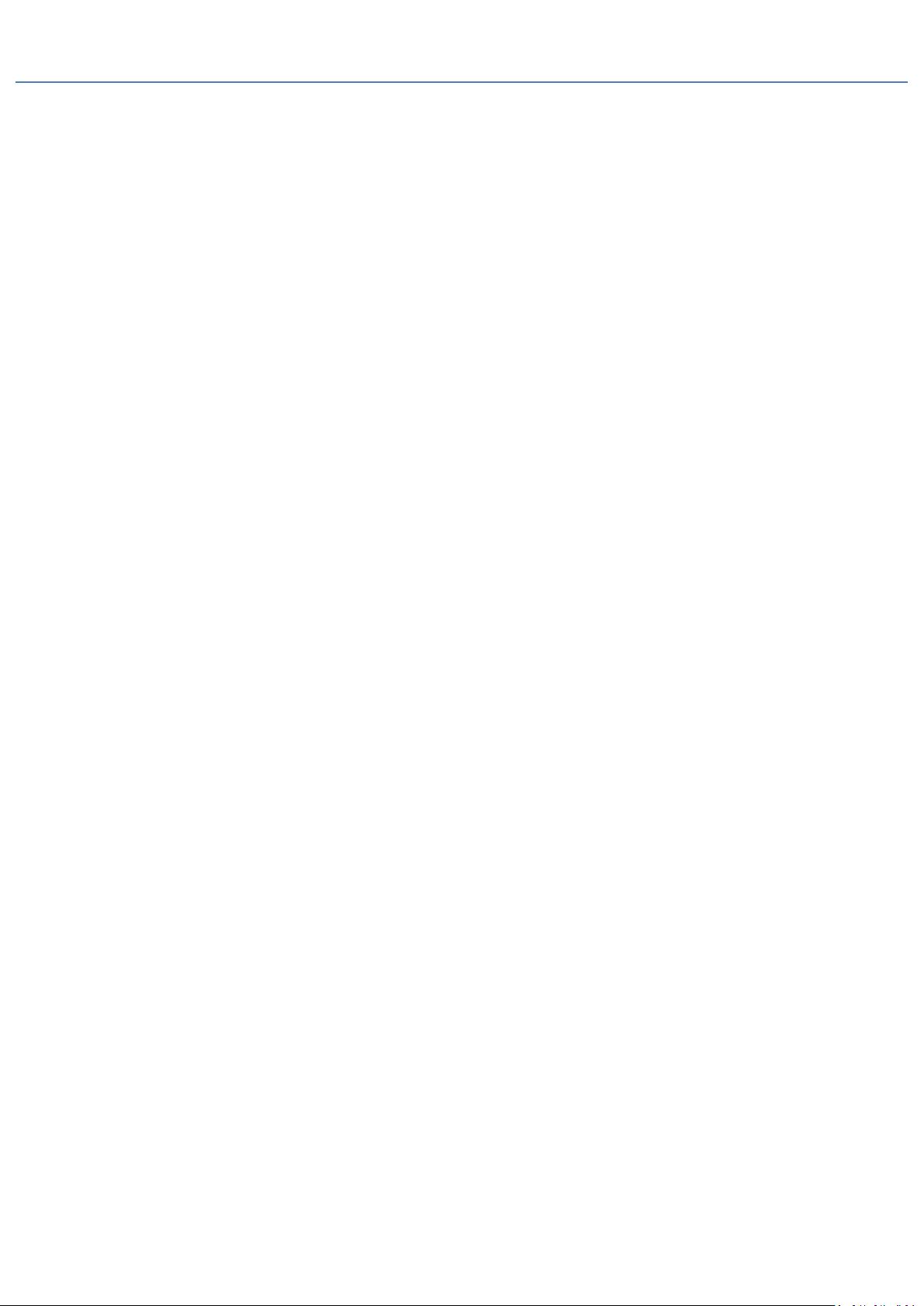
Exceptional C++ Style: New Engineering Puzzles, Programming Problems, and Solutions
3
6.3.5 Summary .................................................................................................................. 188
6.4 Chapter 23. To new, Perchance to tHRow, Part 2: Pragmatic Issues in Memory
Management ......................................................................................................................... 189
6.4.1 Solution .................................................................................................................... 189
7. Part 5 - Optimization and Efficiency ..................................................................................... 196
7.1 Chapter 24. Constant Optimization? .............................................................................. 197
7.1.1 Solution .................................................................................................................... 198
7.2 Chapter 25. inline Redux ................................................................................................. 203
7.2.1 Solution .................................................................................................................... 204
7.3 Chapter 26. Data Formats and Efficiency, Part 1: When Compression Is the Name of the
Game ..................................................................................................................................... 211
7.3.1 Solution .................................................................................................................... 213
7.4 Chapter 27. Data Formats and Efficiency, Part 2: (Even Less) Bit-Twiddling .................. 216
7.4.1 Solution .................................................................................................................... 217
8. Part 6 - Traps, Pitfalls, and Puzzlers ...................................................................................... 224
8.1 Chapter 28. Keywords That Aren't (or, Comments by Another Name) .......................... 225
8.1.1 Solution .................................................................................................................... 226
8.2 Chapter 29. Is It Initialization? ........................................................................................ 232
8.2.1 Solution .................................................................................................................... 233
8.3 Chapter 30. double or Nothing ....................................................................................... 238
8.3.1 Solution .................................................................................................................... 239
8.4 Chapter 31. Amok Code .................................................................................................. 242
8.4.1 Solution .................................................................................................................... 244
8.5 Chapter 32. Slight Typos? Graphic Language and Other Curiosities .............................. 247
8.5.1 Solution .................................................................................................................... 249
8.6 Chapter 33. Operators, Operators Everywhere .............................................................. 251
8.6.1 Solution .................................................................................................................... 252
9. Part 7 - Style Case Studies ..................................................................................................... 258
9.1 Chapter 34. Index Tables ................................................................................................ 258
9.1.1 Solution .................................................................................................................... 260
9.2 Chapter 35. Generic Callbacks ........................................................................................ 270
9.2.1 Solution .................................................................................................................... 271
9.3 Chapter 36. Construction Unions .................................................................................... 280
9.3.1 Solution .................................................................................................................... 282
9.4 Chapter 37. Monoliths "Unstrung," Part 1: A Look at std::string ................................... 298
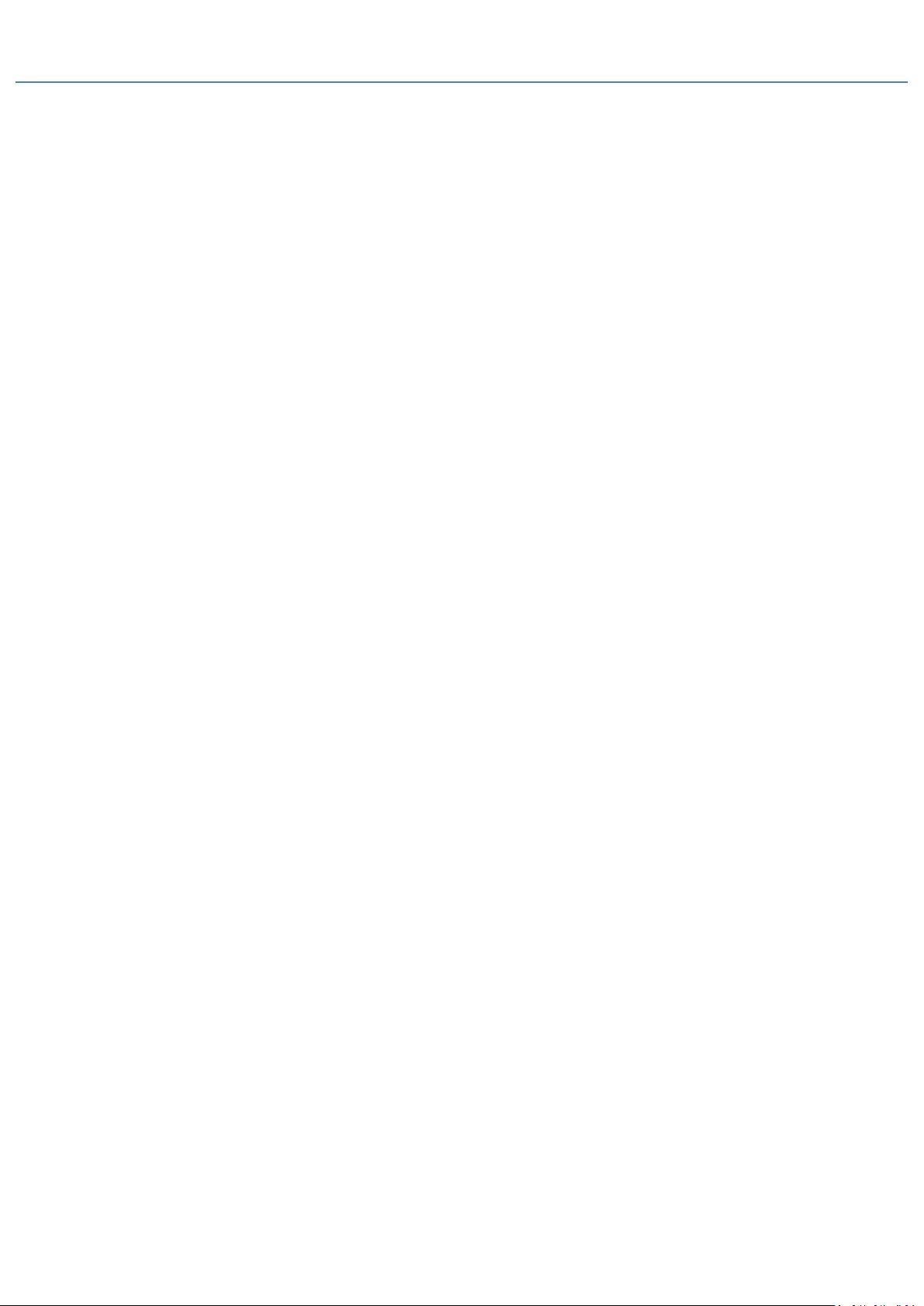
Exceptional C++ Style: New Engineering Puzzles, Programming Problems, and Solutions
4
9.4.1 Solution .................................................................................................................... 299
9.4.2 Summary .................................................................................................................. 304
9.5 Chapter 38. Monoliths "Unstrung," Part 2: Refactoring std::string ................................ 304
9.5.1 Solution .................................................................................................................... 305
9.6 Chapter 39. Monoliths "Unstrung," Part 3: std::string Diminishing ............................... 313
9.6.1 Solution .................................................................................................................... 314
9.7 Chapter 40. Monoliths "Unstrung," Part 4: std::string Redux ........................................ 317
9.7.1 Solution .................................................................................................................... 318
10. Bibliography ........................................................................................................................ 326