#include "widget.h"
#include "ui_widget.h"
#include <QDebug>
Widget::Widget(QWidget *parent) :
QWidget(parent),
ui(new Ui::Widget)
{
ui->setupUi(this);
ui->lineEdit->setText("");
keyFom = new SoftKeyDialog(this);
init();
}
Widget::~Widget()
{
delete ui;
}
void Widget::init()
{
QPushButton *btnKey1 = keyFom->findChild<QPushButton *>("key_1");
if(btnKey1)
{
connect(btnKey1,SIGNAL(clicked()),this,SLOT(onKey1()));
}
QPushButton *btnKey2 = keyFom->findChild<QPushButton *>("key_2");
if(btnKey2)
{
connect(btnKey2,SIGNAL(clicked()),this,SLOT(onKey2()));
}
QPushButton *btnKey3 = keyFom->findChild<QPushButton *>("key_3");
if(btnKey3)
{
connect(btnKey3,SIGNAL(clicked()),this,SLOT(onKey3()));
}
QPushButton *btnKey4 = keyFom->findChild<QPushButton *>("key_4");
if(btnKey4)
{
connect(btnKey4,SIGNAL(clicked()),this,SLOT(onKey4()));
}
QPushButton *btnKey5 = keyFom->findChild<QPushButton *>("key_5");
if(btnKey5)
{
connect(btnKey5,SIGNAL(clicked()),this,SLOT(onKey5()));
}
QPushButton *btnKey6 = keyFom->findChild<QPushButton *>("key_6");
if(btnKey6)
{
connect(btnKey6,SIGNAL(clicked()),this,SLOT(onKey6()));
}
QPushButton *btnKey7 = keyFom->findChild<QPushButton *>("key_7");
if(btnKey7)
{
connect(btnKey7,SIGNAL(clicked()),this,SLOT(onKey7()));
}
QPushButton *btnKey8 = keyFom->findChild<QPushButton *>("key_8");
if(btnKey8)
{
connect(btnKey8,SIGNAL(clicked()),this,SLOT(onKey8()));
}
QPushButton *btnKey9 = keyFom->findChild<QPushButton *>("key_9");
if(btnKey9)
{
connect(btnKey9,SIGNAL(clicked()),this,SLOT(onKey9()));
}
QPushButton *btnKey0 = keyFom->findChild<QPushButton *>("key_0");
if(btnKey0)
{
connect(btnKey0,SIGNAL(clicked()),this,SLOT(onKey0()));
}
QPushButton *btnKeyC = keyFom->findChild<QPushButton *>("key_C");
if(btnKeyC)
{
connect(btnKeyC,SIGNAL(clicked()),this,SLOT(onKeyC()));
}
QPushButton *btnKeyD = keyFom->findChild<QPushButton *>("key_dot");
if(btnKeyD)
{
connect(btnKeyD,SIGNAL(clicked()),this,SLOT(onKeyDot()));
}
QPushButton *btnKeyDel = keyFom->findChild<QPushButton *>("key_del");
if(btnKeyDel)
{
connect(btnKeyDel,SIGNAL(clicked()),this,SLOT(onKeyDel()));
}
QPushButton *btnKeyE = keyFom->findChild<QPushButton *>("key_enter");
if(btnKeyE)
{
connect(btnKeyE,SIGNAL(clicked()),this,SLOT(onKeyEnter()));
}
}
void Widget::on_pushButton_clicked()
{
ui->lineEdit->setText("");
keyFom->setWindowFlags(Qt::FramelessWindowHint);
keyFom->exec();
QPoint pos = ui->lineEdit->pos();
keyFom->move(QPoint(160,145));
}
void Widget::onKey1()
{
ui->lineEdit->setText(ui->lineEdit->text()+"1");
}
void Widget::onKey2()
{
ui->lineEdit->setText(ui->lineEdit->text()+"2");
}
void Widget::onKey3()
{
ui->lineEdit->setText(ui->lineEdit->text()+"3");
}
void Widget::onKey4()
{
ui->lineEdit->setText(ui->lineEdit->text()+"4");
}
void Widget::onKey5()
{
ui->lineEdit->setText(ui->lineEdit->text()+"5");
}
void Widget::onKey6()
{
ui->lineEdit->setText(ui->lineEdit->text()+"6");
}
void Widget::onKey7()
{
ui->lineEdit->setText(ui->lineEdit->text()+"7");
}
void Widget::onKey8()
{
ui->lineEdit->setText(ui->lineEdit->text()+"8");
}
void Widget::onKey9()
{
ui->lineEdit->setText(ui->lineEdit->text()+"9");
}
void Widget::onKey0()
{
if(ui->lineEdit->text() != "")
{
ui->lineEdit->setText(ui->lineEdit->text()+"0");
}
}
void Widget::onKeydot()
{
//ui->lineEdit->setText(ui->lineEdit->text()+".");
return;
}
void Widget::onKeyC()
{
ui->lineEdit->setText("");
}
void Widget::onKeyDel()
{
if(ui->lineEdit->text() != "")
{
QString text =ui->lineEdit->text();
text.chop(1);
ui->lineEdit->setText(text);
}
}
void Widget::onKeyEnter()
{
keyFom->close();
}
没有合适的资源?快使用搜索试试~ 我知道了~
QT arm显示屏支持数字软键盘
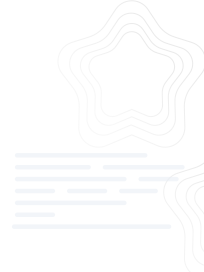
共21个文件
o:5个
cpp:5个
h:4个

需积分: 50 44 下载量 111 浏览量
2017-06-06
15:35:51
上传
评论 1
收藏 54KB ZIP 举报
温馨提示
QT实现的软键盘,在arm主板上linux系统运行,使用LCD显示屏触发。。。。。。。QT实现的软键盘,在arm主板上linux系统运行,使用LCD显示屏触发
资源推荐
资源详情
资源评论
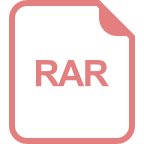
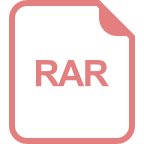
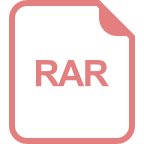
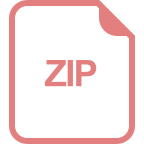
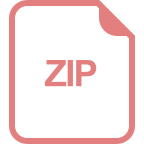
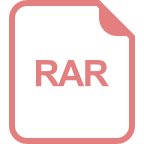
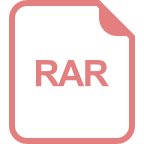
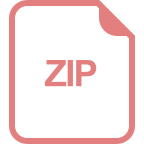
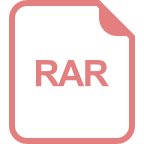
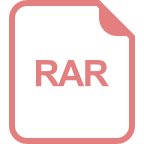
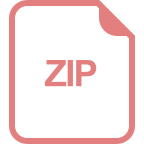
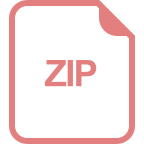
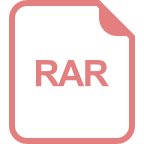
收起资源包目录


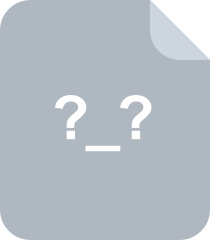
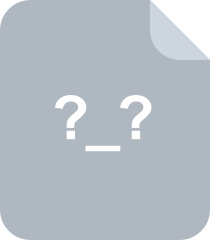
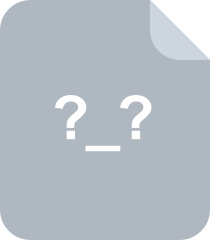
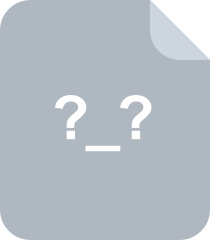
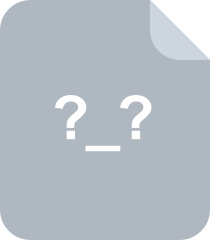
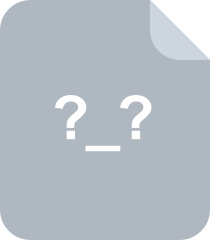
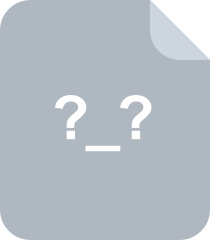
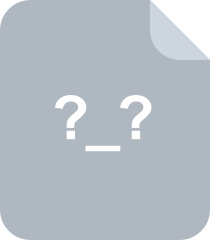
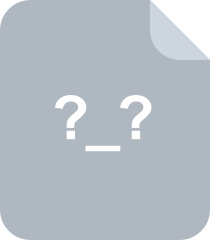
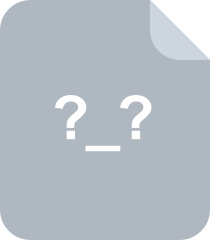
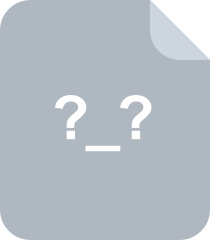
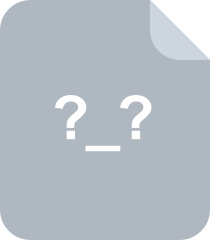
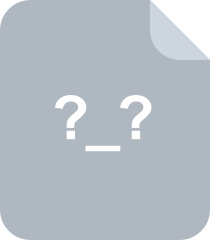
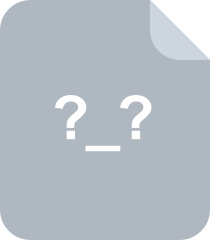
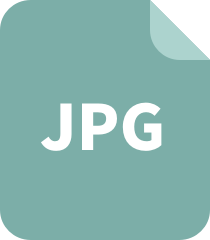
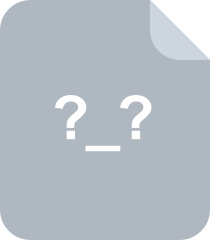
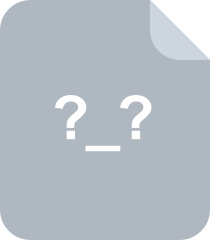
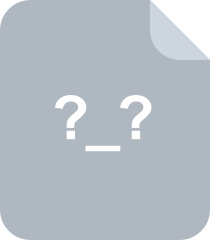
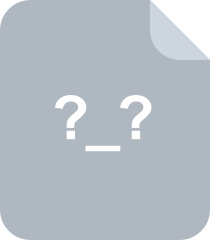
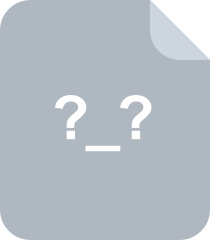
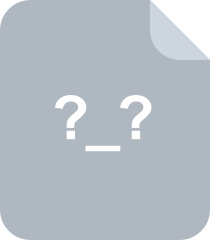
共 21 条
- 1
资源评论
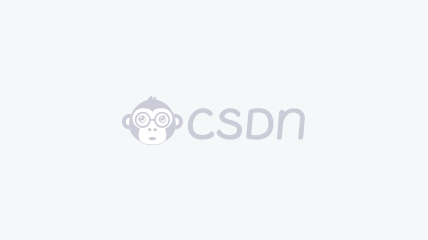

sirius505
- 粉丝: 13
- 资源: 26
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

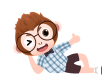
安全验证
文档复制为VIP权益,开通VIP直接复制
