在Android开发中,RSA是一种广泛使用的非对称加密算法,用于保护敏感数据,如用户密码、个人信息等。本文将深入探讨如何在Android平台上实现RSA加解密和签名操作,同时也会涉及从字符串和PEM文件读取公钥和私钥的方法,并使用Android自带的Base64库进行编码和解码。 我们需要理解RSA算法的基本原理。RSA是基于数论中的大数因子分解难题构建的,它包括一对密钥:公钥和私钥。公钥可以公开,用于加密数据,而私钥必须保密,用于解密数据。此外,私钥也可用于数字签名,验证数据的完整性和来源。 在Android中,我们可以使用Java的`java.security`包来处理RSA密钥对和加密解密操作。我们需要生成一个密钥对,这可以通过`KeyPairGenerator`类实现: ```java KeyPairGenerator keyPairGen = KeyPairGenerator.getInstance("RSA"); keyPairGen.initialize(2048); // 指定密钥长度,如2048位 KeyPair keyPair = keyPairGen.generateKeyPair(); PublicKey publicKey = keyPair.getPublic(); PrivateKey privateKey = keyPair.getPrivate(); ``` 接着,我们可以将公钥和私钥转换为字符串,方便存储和传输。通常,我们会使用Base64编码将密钥转换为ASCII字符串: ```java String publicKeyStr = Base64.encodeToString(publicKey.getEncoded(), Base64.DEFAULT); String privateKeyStr = Base64.encodeToString(privateKey.getEncoded(), Base64.DEFAULT); ``` 要从字符串恢复密钥,我们需要反向解码并重建密钥对象: ```java byte[] publicKeyBytes = Base64.decode(publicKeyStr, Base64.DEFAULT); byte[] privateKeyBytes = Base64.decode(privateKeyStr, Base64.DEFAULT); PublicKey publicKeyFromStr = KeyFactory.getInstance("RSA").generatePublic(new X509EncodedKeySpec(publicKeyBytes)); PrivateKey privateKeyFromStr = KeyFactory.getInstance("RSA").generatePrivate(new PKCS8EncodedKeySpec(privateKeyBytes)); ``` PEM文件是公钥和私钥的常见存储格式,包含Base64编码的密钥和一些元数据。Android也可以读取PEM文件,但需要自定义解析方法,因为Android默认不支持PEM格式。以下是一个简单的示例: ```java public static PrivateKey readPrivateKeyFromPEM(InputStream inputStream) { try { BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream)); String line; StringBuilder pemContent = new StringBuilder(); while ((line = reader.readLine()) != null) { if (line.startsWith("-----BEGIN PRIVATE KEY-----")) { break; } } while ((line = reader.readLine()) != null && !line.startsWith("-----END PRIVATE KEY-----")) { pemContent.append(line.trim()); } byte[] keyBytes = Base64.decode(pemContent.toString(), Base64.DEFAULT); PKCS8EncodedKeySpec keySpec = new PKCS8EncodedKeySpec(keyBytes); return KeyFactory.getInstance("RSA").generatePrivate(keySpec); } catch (Exception e) { throw new RuntimeException("Failed to read private key from PEM", e); } } ``` 对于加密和解密操作,我们可以使用`Cipher`类: ```java Cipher cipher = Cipher.getInstance("RSA/ECB/PKCS1Padding"); // 注意选择合适的填充模式 cipher.init(Cipher.ENCRYPT_MODE, publicKey); byte[] encryptedData = cipher.doFinal(dataToEncrypt); cipher.init(Cipher.DECRYPT_MODE, privateKey); byte[] decryptedData = cipher.doFinal(encryptedData); ``` 数字签名通常用于验证数据未被篡改。使用私钥进行签名,然后用公钥验证: ```java Signature signature = Signature.getInstance("SHA256withRSA"); signature.initSign(privateKey); signature.update(dataToSign); byte[] signedData = signature.sign(); signature.initVerify(publicKey); signature.update(dataToSign); boolean isValid = signature.verify(signedData); ``` Android RSA加解密签名涉及了非对称加密、密钥生成、字符串和PEM文件的读取以及签名验证等多个环节。在实际应用中,开发者需要注意安全实践,如妥善保管私钥,避免密钥泄露。同时,理解并正确使用这些技术能够确保应用程序的数据安全。
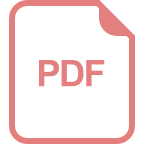
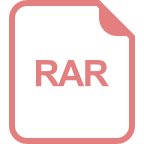
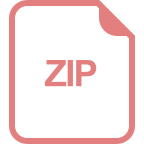
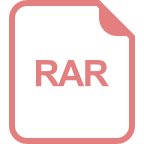
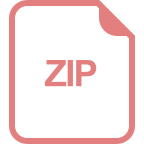
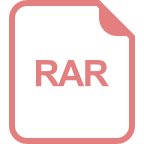
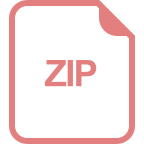
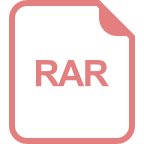
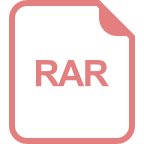
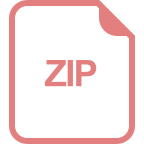
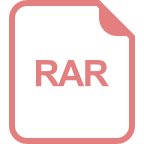
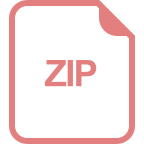
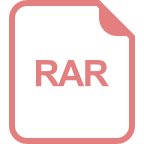
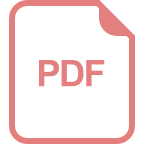
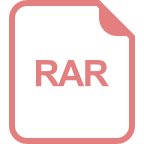
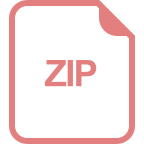
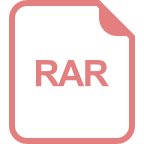
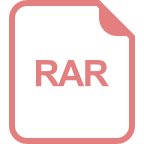
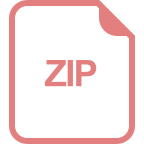
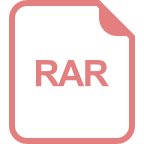
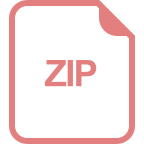
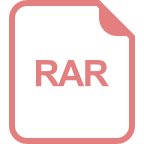
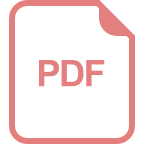
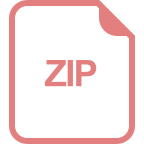


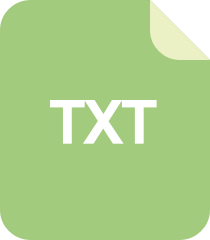
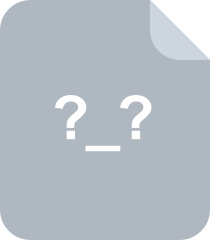
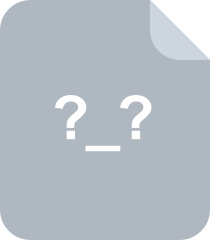
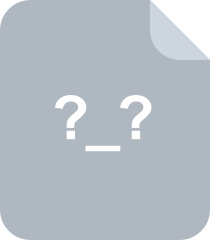
- 1
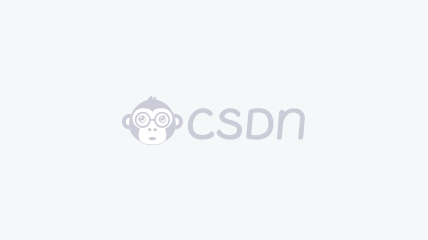

- 粉丝: 0
- 资源: 1
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

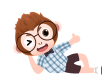
最新资源

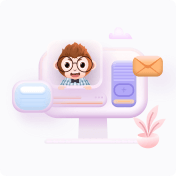
