# vproxy
[](https://github.com/wkgcass/vproxy/actions/workflows/ci.yaml)
[中文文档](https://github.com/wkgcass/vproxy/blob/master/README_ZH.md)
## Intro
VProxy is a zero-dependency TCP Loadbalancer based on Java NIO. The project only requires Java 11 to run.
Clone it, compile it, then everything is ready for running.
## Features
1. TCP Loadbalancer with TLS termination
2. HTTP/1.x and HTTP/2 Loadbalancer with `Host` header consideration
3. Other tcp based protocol loadbalancer, such as grpc, dubbo
4. Socks5 server
5. DNS server and customizable A|AAAA records
6. Kubernetes integration
7. Many other standalone extended apps, such as `WebSocksProxyAgent` and `WebSocksProxyServer`
## Make
<details><summary>use pre-built releases</summary>
<br>
See the [release page](https://github.com/wkgcass/vproxy/releases).
#### For linux
Use the latest `vproxy-linux` binary file in release page.
Or
Use the jlink built runtime [here](https://github.com/wkgcass/vproxy/releases/download/1.0.0-BETA-12/vproxy-runtime-linux.tar.gz).
#### For macos
Use the latest `vproxy-macos` binary file in release page.
#### For windows
Java runtime can be found [here](https://adoptium.net/releases.html?variant=openjdk17&jvmVariant=hotspot).
#### For musl
Use the jlink built runtime [here](https://github.com/wkgcass/vproxy/releases/download/1.0.0-BETA-12/vproxy-runtime-musl.tar.gz).
</details>
<details><summary>build prerequisites</summary>
Run:
```shell
make init
```
to initiate submodules and some other init work.
A java agent is required as a patch for gradle.
Copy `misc/modify-gradle-compiler-args-agent.jar` to `~/.gradle/` before compiling this project.
</details>
<details><summary>jar package</summary>
<br>
```
./gradlew clean jar
java -jar build/libs/vproxy.jar -Deploy=HelloWorld
```
</details>
<details><summary>jlink</summary>
<br>
```
make jlink
./build/image/bin/vproxy -Deploy=HelloWorld
```
</details>
<details><summary>docker</summary>
<br>
```
# make docker
docker run -it --rm vproxyio/vproxy -Deploy=HelloWorld
```
</details>
<details><summary>graal native-image</summary>
<br>
```
make image
./vproxy -Deploy=HelloWorld
```
</details>
<details><summary>native fds impl</summary>
<br>
Only macos(bsd)/linux supported.
```
make vfdposix
java -Dvfd=posix -Djava.library.path=./base/src/main/c -jar build/libs/vproxy.jar -Deploy=HelloWorld
```
And there's a special version for windows to support Tap devices: `-Dvfd=windows`, however the normal fds and event loop are stll based on jdk selector channel.
```
make vfdwindows
java -Dvfd=windows -Djava.library.path=./base/src/main/c -jar build/libs/vproxy.jar -Deploy=HelloWorld
```
Windows TAP depends on OpenVPN TAP Driver. MacOS TAP depends on tuntaposx.
MacOS TUN, Linux TAP and TUN has no extra dependencies.
</details>
<details><summary>xdp</summary>
<br>
It's recommended to run a kernel with minimum version 5.10 (or at least 5.4) in order to use xdp support in the switch module.
If using a lower version, you cannot share the same umem with different xdp interfaces.
To build the xdp support, you will need these packages: `apt-get install -y linux-headers-$(uname -r) build-essential libelf-dev clang llvm`, then:
```
make vpxdp
```
Or compile it inside a docker container on a non-Linux platform:
```
make vpxdp-linux
```
</details>
<details><summary>test</summary>
<br>
Run test cases:
```
./gradlew runTest
```
Run test cases in docker:
```
make dockertest
```
Test vswitch, docker network plugin, vpctl, k8s controller:
```shell
# requires virtualbox installed
cd ./misc/auto-setup/
./auto-setup.sh
./auto-verify.sh
```
</details>
<details><summary>ui</summary>
<br>
vproxy provides some ui tools.
```shell
./gradlew ui:jar
java -cp ./ui/build/libs/vproxy-ui.jar $mainClassName
```
Current available ui tools:
1. `io.vproxy.ui.calculator.CalculatorMain`: an IPv4 network calculator
</details>
## Aim
* Zero dependency: no dependency other than java and kotlin standard library.
* Simple: keep code simple and clear.
* Modifiable when running: no need to reload for configuration update.
* Fast: performance is one of our main priorities.
* TCP Loadbalancer: we now support TCP and TCP based protocols, also allow your own protocols.
* Kubernetes: integrate vproxy resources into k8s.
* SDN: modifying and forwarding packets with flows and routes.
## How to use
<details><summary>use as a library</summary>
<br>
**gradle**
```groovy
implementation group: 'io.vproxy', name: 'vproxy-adaptor-netty', version: '1.0.0-BETA-12'
// all available artifacts: dep, base, adaptor-netty, adaptor-vertx
```
**maven**
```xml
<dependency>
<groupId>io.vproxy</groupId>
<artifactId>vproxy-adaptor-netty</artifactId>
<version>1.0.0-BETA-12</version>
</dependency>
<!-- all available artifacts: dep, base, adaptor-netty, adaptor-vertx -->
```
**module-info.java**
```java
requires io.vproxy.dep;
requires io.vproxy.base;
requires io.vproxy.adaptor.netty;
requires io.vproxy.adaptor.vertx;
```
**netty**
```java
var acceptelg = new VProxyEventLoopGroup();
var elg = new VProxyEventLoopGroup(4);
var bootstrap = new ServerBootstrap();
bootstrap
.channel(VProxyInetServerSocketChannel.class)
.childHandler(new ChannelInitializer<>() {
@Override
protected void initChannel(Channel ch) {
ChannelPipeline p = ch.pipeline();
p.addLast(new HttpServerCodec());
p.addLast(new HttpHelloWorldServerHandler());
}
});
bootstrap.group(acceptelg, elg);
bootstrap.bind(hostname, port).sync();
```
</details>
<details><summary>use vproxy with kubernetes</summary>
<br>
Add crd, launch vproxy and controller
```
kubectl apply -f https://github.com/vproxy-tools/vpctl/blob/master/misc/crd.yaml
kubectl apply -f https://github.com/vproxy-tools/vpctl/blob/master/misc/k8s-vproxy.yaml
```
Launch the example app
```
kubectl apply -f https://github.com/vproxy-tools/vpctl/blob/master/misc/cr-example.yaml
```
Detailed info can be found [here](https://github.com/vproxy-tools/vpctl/blob/master/README.md).
</details>
<details><summary>vpctl</summary>
<br>
A command line client application is provided to manipulate the vproxy instance. You may see more info in [vpctl repo](https://github.com/vproxy-tools/vpctl).
This tool is fully tested and simple to use. Some examples are provided in the tool repo for reference.
</details>
<details><summary>Simple mode</summary>
<br>
You can start a simple loadbalancer in one command:
```
java -Deploy=Simple -jar vproxy.jar \
bind {port} \
backend {host1:port1,host2:port2} \
[ssl {path of cert1,cert2} {path of key}] \
[protocol {...}] \
```
Use `help` to view the parameters.
</details>
<details><summary>Standard mode</summary>
<br>
Use `help` to view the launching parameters.
After launching, you may use `help`, `man`, `man ${action}`, `man ${resource}`, `man ${resource} ${action}` to check the command manual. Also you can use `System: help` to check the system commands.
After launching vproxy, you may use `System:` to run some system commands, You may create `http-controller`s and `resp-controller`s. Then you can operate the vproxy instance using `curl` or `redis-cli`. You may also operate the vproxy instance directly using standard input (stdin).
See [command.md](https://github.com/wkgcass/vproxy/blob/master/doc/command.md) and [api doc](https://github.com/wkgcass/vproxy/blob/master/doc/api.yaml) for more info.
</details>
## Doc
* [how-to-use.md](https://github.com/wkgcass/vproxy/blob/master/doc/how-to-use.md): How to use config file and controllers.
* [api.yaml](https://github.com/wkgcass/vproxy/blob/dev/doc/api.yaml): api doc for http-controller in swagger format.
* [command.md](https://github.com/wkgcass/vproxy/blob/master
没有合适的资源?快使用搜索试试~ 我知道了~
基于Java实现的零依赖的基于NIO的TCP负载均衡器项目源码
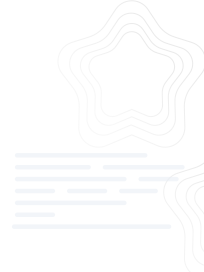
共1233个文件
java:937个
h:64个
kt:46个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 60 浏览量
2023-11-22
10:04:38
上传
评论
收藏 2.47MB ZIP 举报
温馨提示
基于Java实现的零依赖的基于NIO的TCP负载均衡器项目源码 特性 1、TCP和TLS负载均衡 2、HTTP/1.x和HTTP/2负载均衡,支持根据Host分发请求 3、支持其他协议的负载均衡,例如grpc, dubbo 4、Socks5服务 5、DNS服务,支持A|AAAA记录 6、与Kubernetes整合 7、封装好的针对特定场景的应用,例如WebSocksProxyAgent和WebSocksProxyServer
资源推荐
资源详情
资源评论
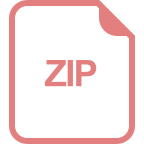
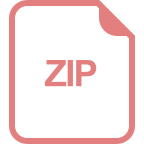
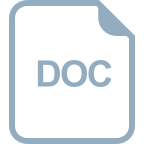
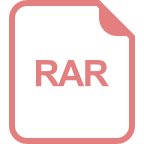
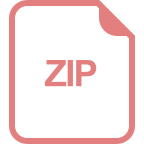
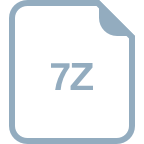
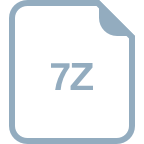
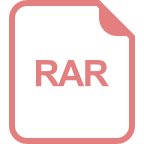
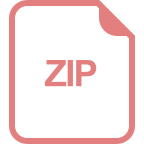
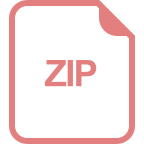
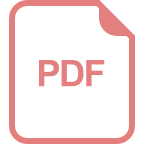
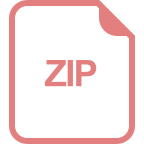
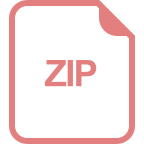
收起资源包目录

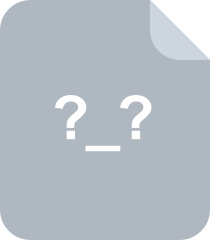
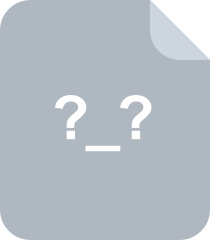
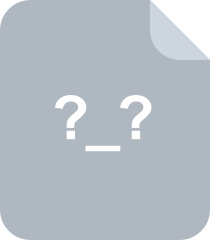
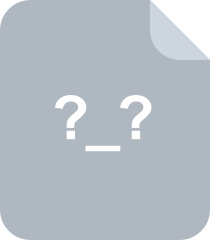
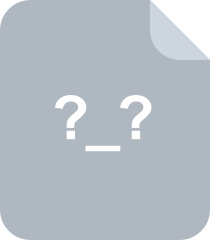
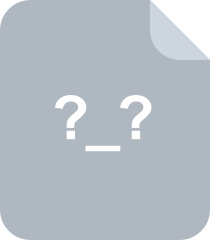
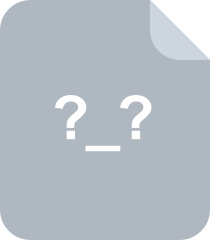
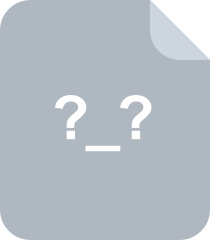
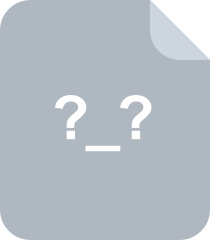
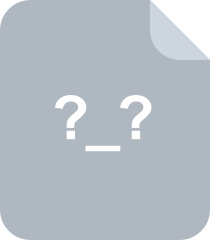
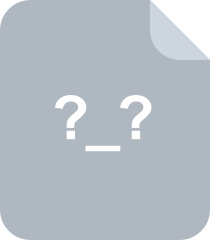
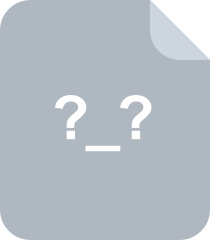
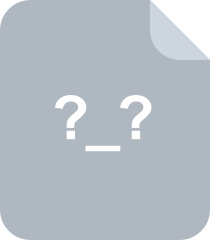
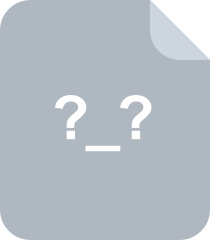
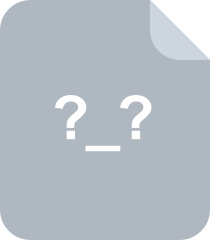
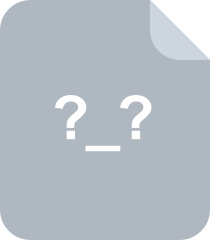
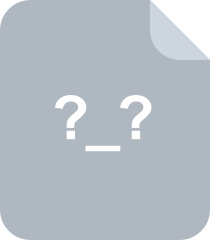
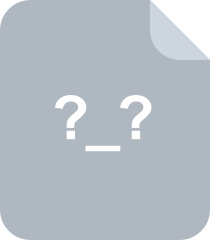
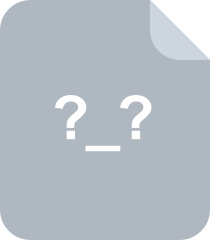
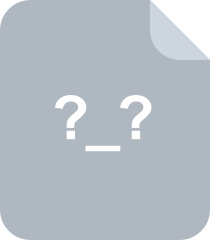
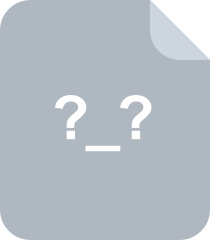
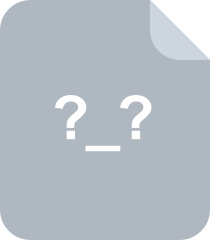
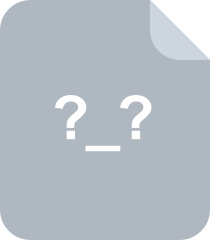
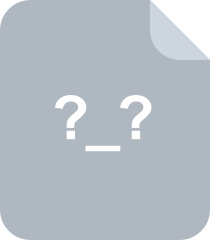
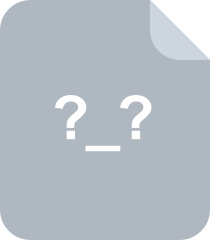
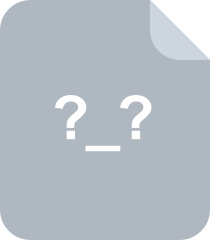
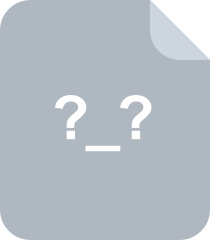
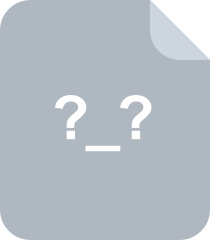
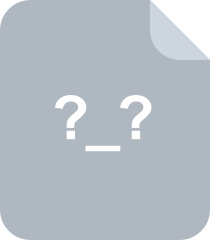
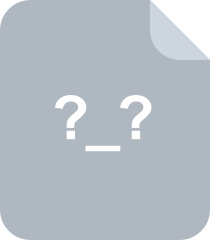
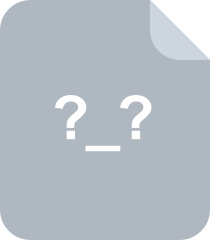
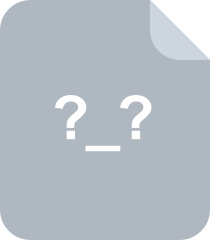
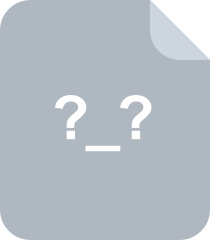
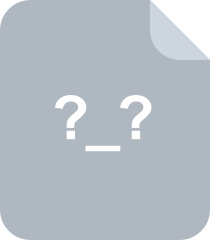
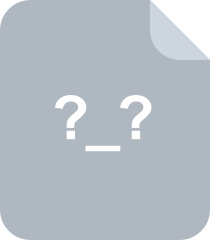
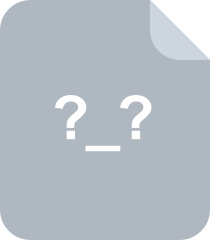
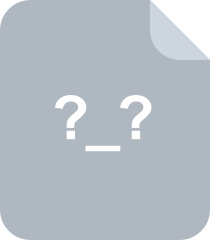
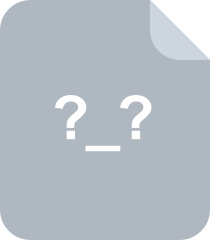
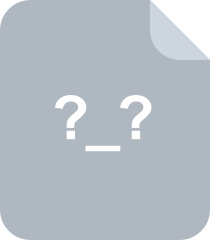
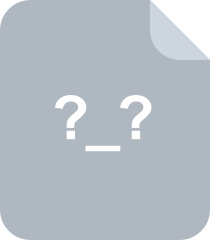
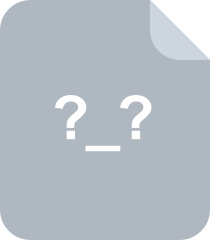
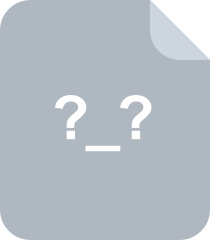
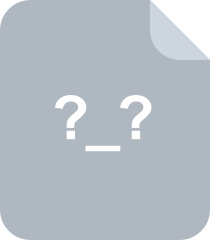
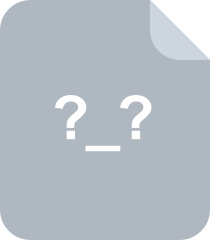
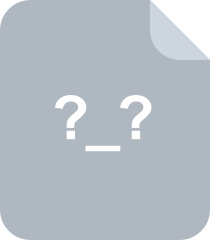
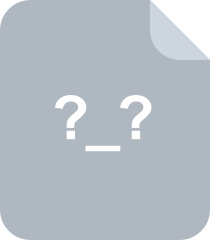
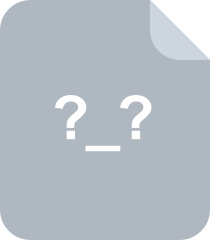
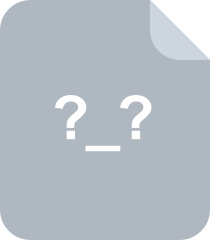
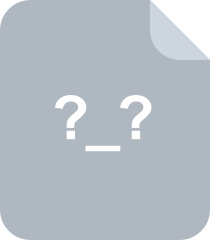
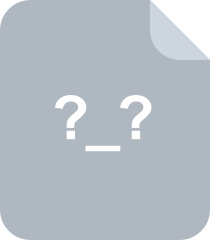
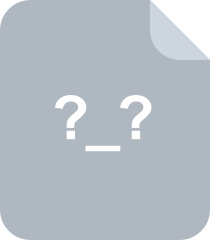
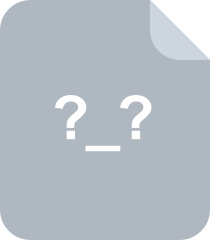
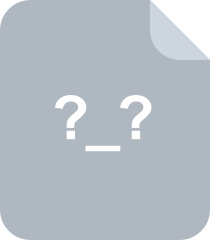
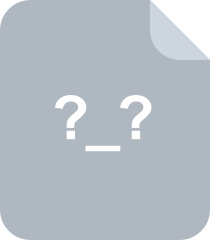
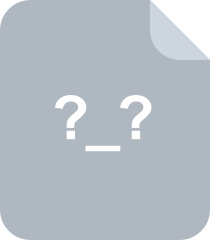
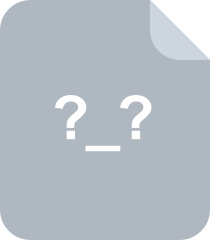
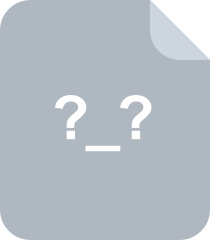
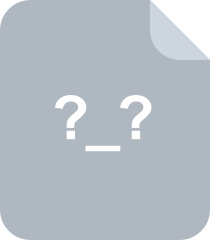
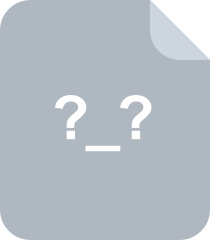
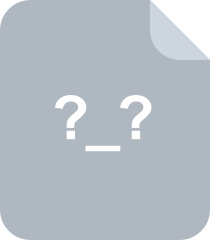
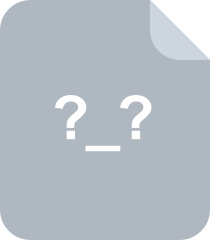
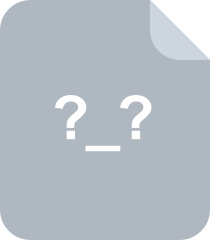
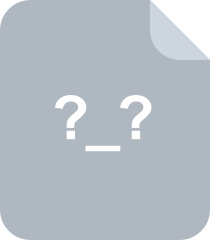
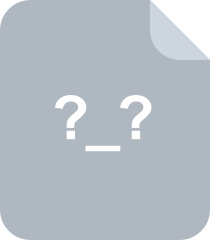
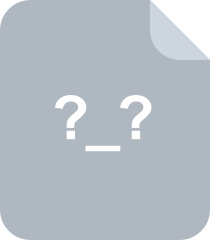
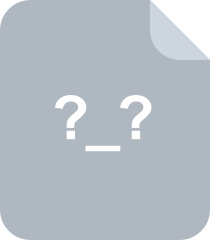
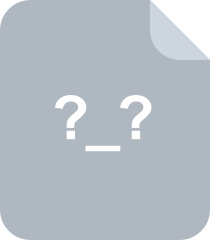
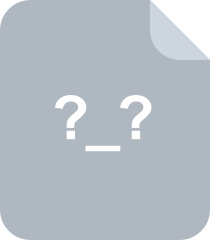
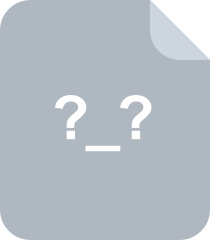
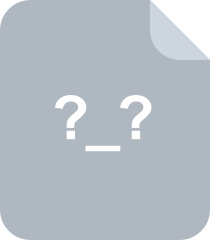
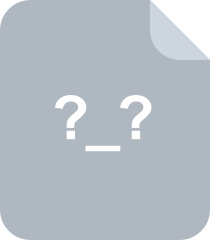
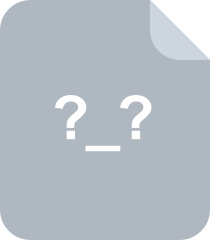
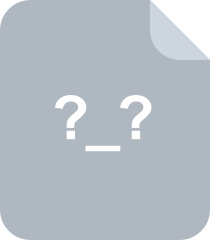
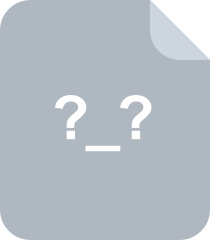
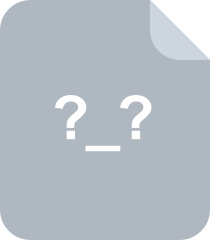
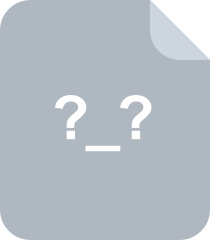
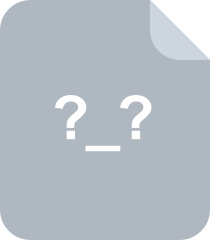
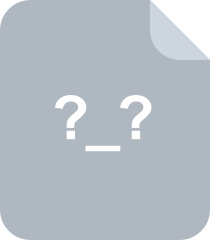
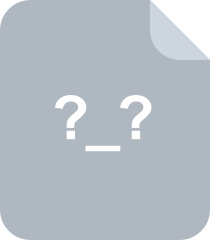
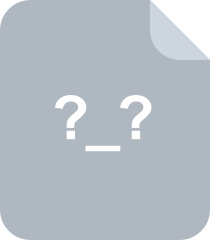
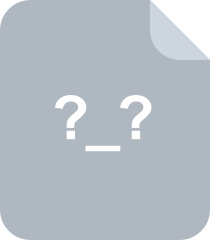
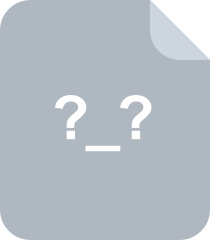
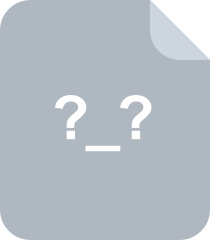
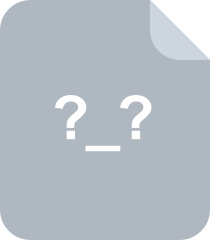
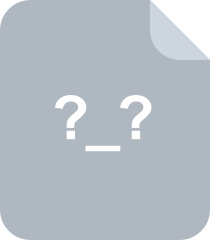
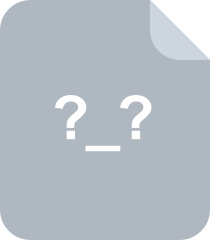
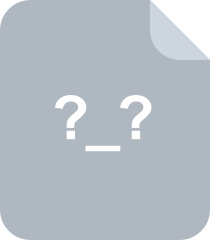
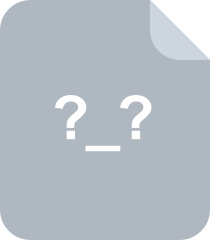
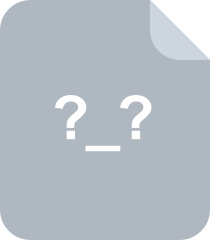
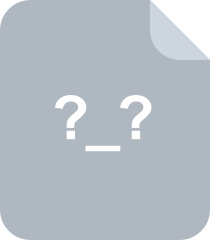
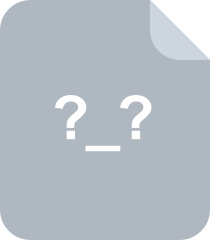
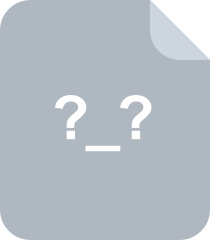
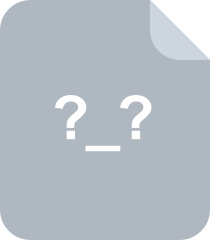
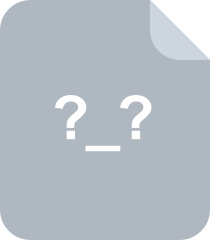
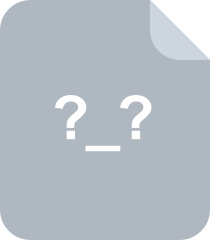
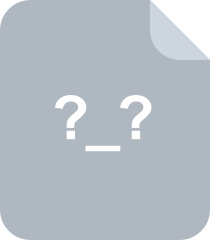
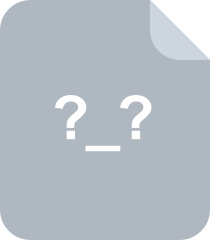
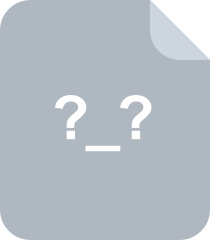
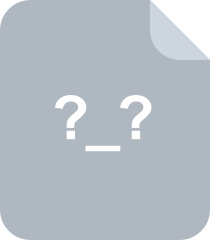
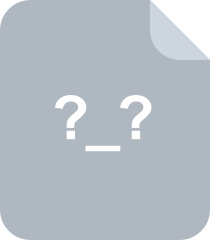
共 1233 条
- 1
- 2
- 3
- 4
- 5
- 6
- 13
资源评论
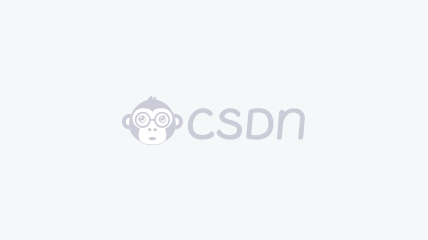

云哲-吉吉2021
- 粉丝: 4054
- 资源: 1128

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

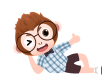
最新资源
- 人和箱子检测2-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 清华大学2022年秋季学期 高等数值分析课程报告
- GEE错误集-Cannot add an object of type <Element> to the map. Might be fixable with an explicit .pdf
- 清华大学2022年秋季学期 高等数值分析课程报告
- 矩阵与线程的对应关系图
- 人体人员检测46-YOLO(v5至v9)、COCO、Darknet、TFRecord数据集合集.rar
- GEMM优化代码实现1
- java实现的堆排序 含代码说明和示例.docx
- 资料阅读器(先下载解压) 5.0.zip
- 人、垃圾、非垃圾检测18-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


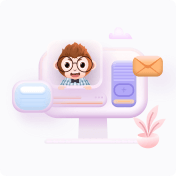
安全验证
文档复制为VIP权益,开通VIP直接复制
