/**
* @license Highcharts JS v6.1.1 (2018-06-27)
*
* (c) 2009-2016 Torstein Honsi
*
* License: www.highcharts.com/license
*/
'use strict';
(function (root, factory) {
if (typeof module === 'object' && module.exports) {
module.exports = root.document ?
factory(root) :
factory;
} else {
root.Highcharts = factory(root);
}
}(typeof window !== 'undefined' ? window : this, function (win) {
var Highcharts = (function () {
/**
* (c) 2010-2017 Torstein Honsi
*
* License: www.highcharts.com/license
*/
/* global win, window */
// glob is a temporary fix to allow our es-modules to work.
var glob = typeof win === 'undefined' ? window : win,
doc = glob.document,
SVG_NS = 'http://www.w3.org/2000/svg',
userAgent = (glob.navigator && glob.navigator.userAgent) || '',
svg = (
doc &&
doc.createElementNS &&
!!doc.createElementNS(SVG_NS, 'svg').createSVGRect
),
isMS = /(edge|msie|trident)/i.test(userAgent) && !glob.opera,
isFirefox = userAgent.indexOf('Firefox') !== -1,
isChrome = userAgent.indexOf('Chrome') !== -1,
hasBidiBug = (
isFirefox &&
parseInt(userAgent.split('Firefox/')[1], 10) < 4 // issue #38
);
var Highcharts = glob.Highcharts ? glob.Highcharts.error(16, true) : {
product: 'Highcharts',
version: '6.1.1',
deg2rad: Math.PI * 2 / 360,
doc: doc,
hasBidiBug: hasBidiBug,
hasTouch: doc && doc.documentElement.ontouchstart !== undefined,
isMS: isMS,
isWebKit: userAgent.indexOf('AppleWebKit') !== -1,
isFirefox: isFirefox,
isChrome: isChrome,
isSafari: !isChrome && userAgent.indexOf('Safari') !== -1,
isTouchDevice: /(Mobile|Android|Windows Phone)/.test(userAgent),
SVG_NS: SVG_NS,
chartCount: 0,
seriesTypes: {},
symbolSizes: {},
svg: svg,
win: glob,
marginNames: ['plotTop', 'marginRight', 'marginBottom', 'plotLeft'],
noop: function () {
return undefined;
},
/**
* An array containing the current chart objects in the page. A chart's
* position in the array is preserved throughout the page's lifetime. When
* a chart is destroyed, the array item becomes `undefined`.
* @type {Array<Chart>}
* @memberof Highcharts
*/
charts: []
};
return Highcharts;
}());
(function (H) {
/**
* (c) 2010-2017 Torstein Honsi
*
* License: www.highcharts.com/license
*/
/**
* The Highcharts object is the placeholder for all other members, and various
* utility functions. The most important member of the namespace would be the
* chart constructor.
*
* @example
* var chart = Highcharts.chart('container', { ... });
*
* @namespace Highcharts
*/
H.timers = [];
var charts = H.charts,
doc = H.doc,
win = H.win;
/**
* Provide error messages for debugging, with links to online explanation. This
* function can be overridden to provide custom error handling.
*
* @function #error
* @memberof Highcharts
* @param {Number|String} code - The error code. See [errors.xml]{@link
* https://github.com/highcharts/highcharts/blob/master/errors/errors.xml}
* for available codes. If it is a string, the error message is printed
* directly in the console.
* @param {Boolean} [stop=false] - Whether to throw an error or just log a
* warning in the console.
*
* @sample highcharts/chart/highcharts-error/ Custom error handler
*/
H.error = function (code, stop) {
var msg = H.isNumber(code) ?
'Highcharts error #' + code + ': www.highcharts.com/errors/' + code :
code;
if (stop) {
throw new Error(msg);
}
// else ...
if (win.console) {
console.log(msg); // eslint-disable-line no-console
}
};
/**
* An animator object used internally. One instance applies to one property
* (attribute or style prop) on one element. Animation is always initiated
* through {@link SVGElement#animate}.
*
* @constructor Fx
* @memberof Highcharts
* @param {HTMLDOMElement|SVGElement} elem - The element to animate.
* @param {AnimationOptions} options - Animation options.
* @param {string} prop - The single attribute or CSS property to animate.
* @private
*
* @example
* var rect = renderer.rect(0, 0, 10, 10).add();
* rect.animate({ width: 100 });
*/
H.Fx = function (elem, options, prop) {
this.options = options;
this.elem = elem;
this.prop = prop;
};
H.Fx.prototype = {
/**
* Set the current step of a path definition on SVGElement.
*
* @function #dSetter
* @memberof Highcharts.Fx
*/
dSetter: function () {
var start = this.paths[0],
end = this.paths[1],
ret = [],
now = this.now,
i = start.length,
startVal;
// Land on the final path without adjustment points appended in the ends
if (now === 1) {
ret = this.toD;
} else if (i === end.length && now < 1) {
while (i--) {
startVal = parseFloat(start[i]);
ret[i] =
isNaN(startVal) ? // a letter instruction like M or L
end[i] :
now * (parseFloat(end[i] - startVal)) + startVal;
}
// If animation is finished or length not matching, land on right value
} else {
ret = end;
}
this.elem.attr('d', ret, null, true);
},
/**
* Update the element with the current animation step.
*
* @function #update
* @memberof Highcharts.Fx
*/
update: function () {
var elem = this.elem,
prop = this.prop, // if destroyed, it is null
now = this.now,
step = this.options.step;
// Animation setter defined from outside
if (this[prop + 'Setter']) {
this[prop + 'Setter']();
// Other animations on SVGElement
} else if (elem.attr) {
if (elem.element) {
elem.attr(prop, now, null, true);
}
// HTML styles, raw HTML content like container size
} else {
elem.style[prop] = now + this.unit;
}
if (step) {
step.call(elem, now, this);
}
},
/**
* Run an animation.
*
* @function #run
* @memberof Highcharts.Fx
* @param {Number} from - The current value, value to start from.
* @param {Number} to - The end value, value to land on.
* @param {String} [unit] - The property unit, for example `px`.
*
*/
run: function (from, to, unit) {
var self = this,
options = self.options,
timer = function (gotoEnd) {
return timer.stopped ? false : self.step(gotoEnd);
},
requestAnimationFrame =
win.requestAnimationFrame ||
function (step) {
setTimeout(step, 13);
},
step = function () {
for (var i = 0; i < H.timers.length; i++) {
if (!H.timers[i]()) {
H.timers.splice(i--, 1);
}
}
if (H.timers.length) {
requestAnimationFrame(step);
}
};
if (from === to && !this.elem['forceAnimate:' + this.prop]) {
delete options.curAnim[this.prop];
if (options.complete && H.keys(options.curAnim).length === 0) {
options.complete.call(this.elem);
}
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本篇论文将研究如何使用Python和Django框架,结合sqlite数据库实现一个电子书图书商城网站系统。该系统将包括用户注册、登录、浏览书籍、添加到购物车、下订单等核心功能。 首先,我们需要进行系统需求分析,确定网站的核心功能和用户需求。在这个阶段,我们需要对网站的功能和业务进行详细分析,确定网站的目标用户和核心功能。 其次,我们需要设计网站的数据库架构,包括确定数据表、关系、索引等。在这个阶段,我们需要根据系统需求分析的结果,设计合适的数据模型,以支持网站的核心功能。 接下来,我们需要进行网站的前端设计和开发。在这个阶段,我们需要使用HTML、CSS、JavaScript等前端技术,构建一个美观、易用的用户界面。同时,我们还需要使用Bootstrap等框架,提高网站的响应性和可访问性。 然后,我们需要进行网站的后台设计和开发。在这个阶段,我们需要使用Python和Django框架,构建网站的后台管理系统,以支持网站的各项核心功能。同时,我们还需要使用sqlite数据库,存储和管理网站的数据。 最后,我们需要进行网站的测试和部署。在这个阶段,我们需要对网站进行全面的测试,确保网站的
资源推荐
资源详情
资源评论
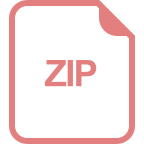
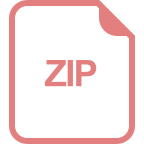
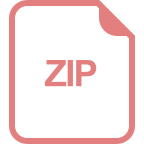
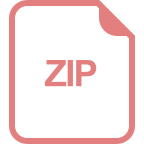
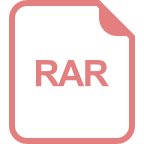
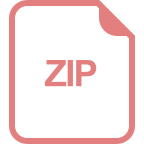
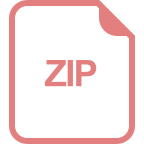
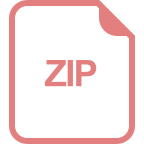
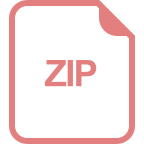
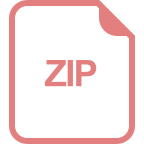
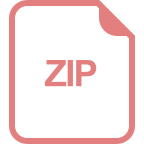
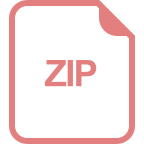
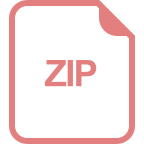
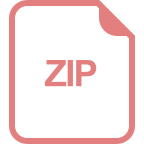
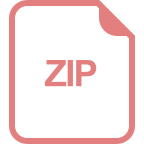
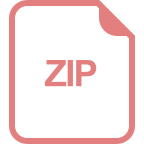
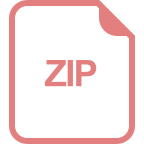
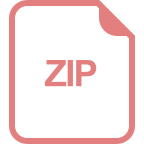
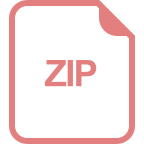
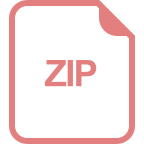
收起资源包目录

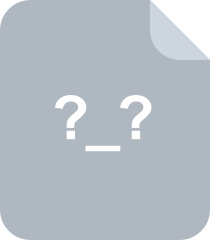
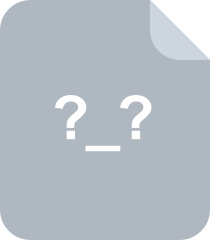
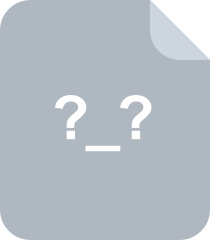
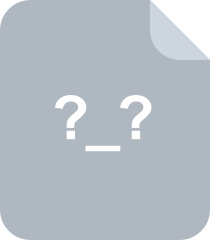
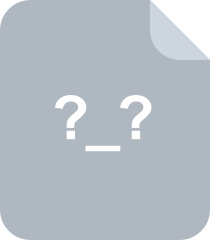
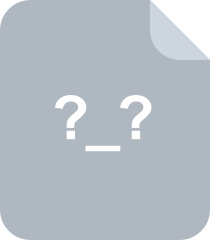
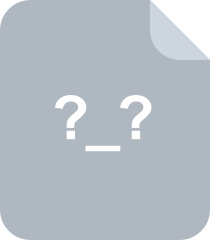
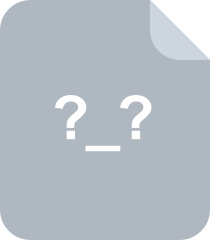
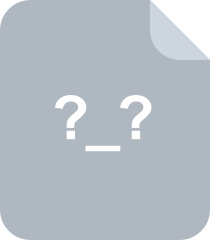
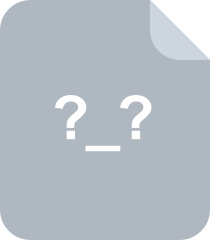
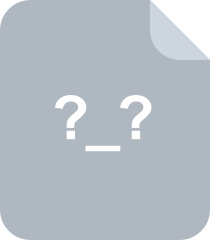
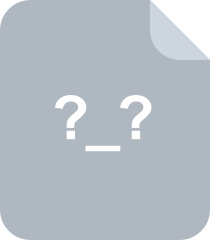
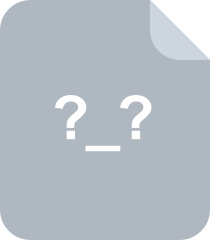
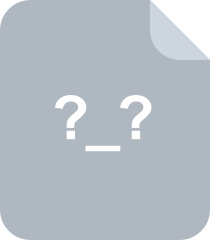
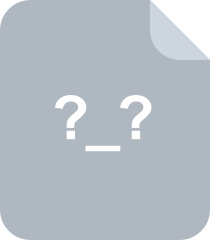
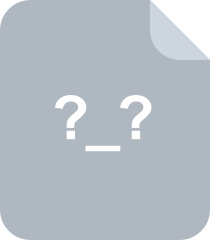
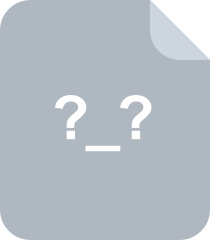
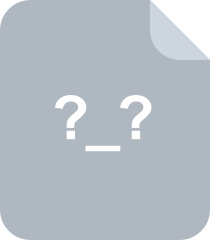
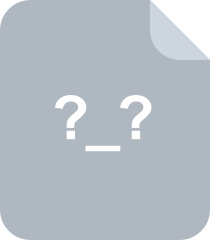
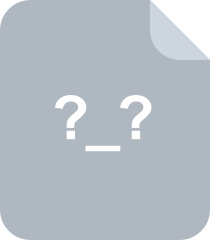
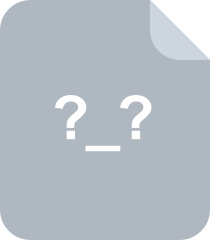
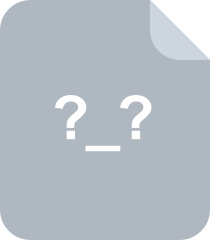
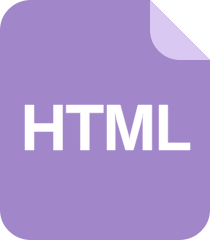
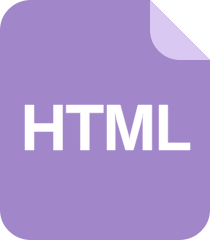
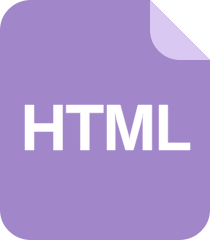
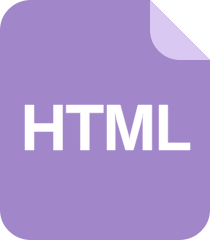
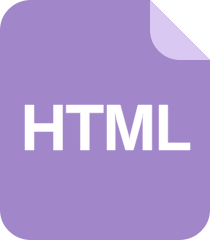
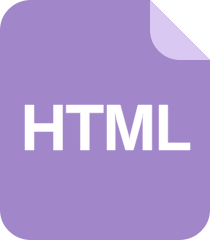
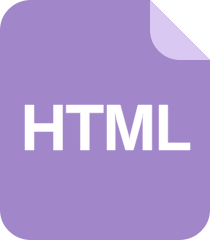
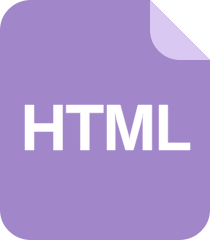
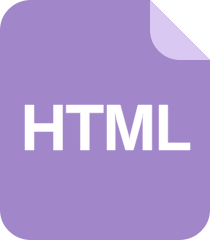
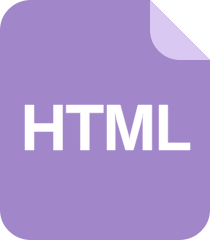
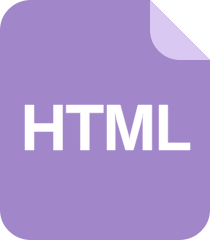
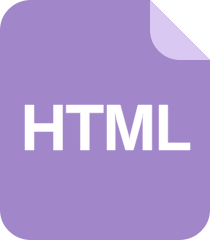
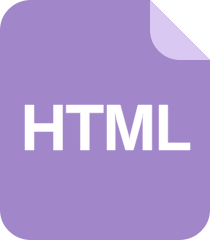
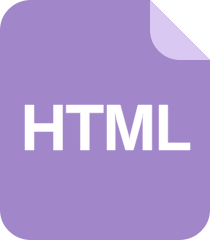
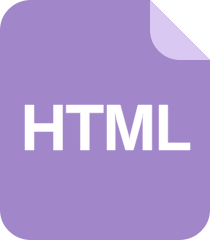
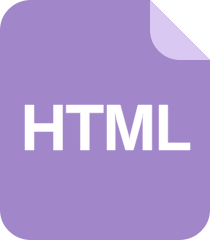
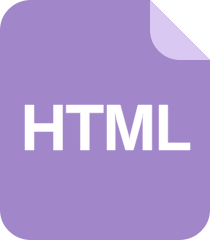
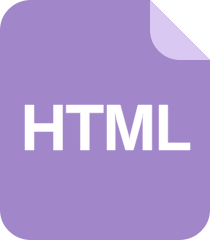
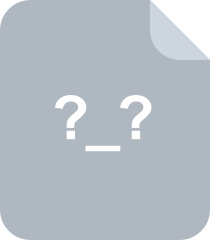
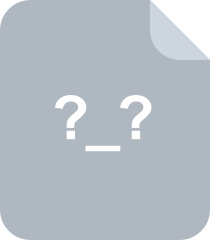
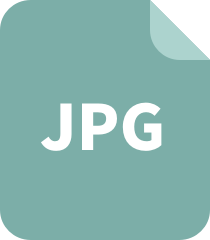
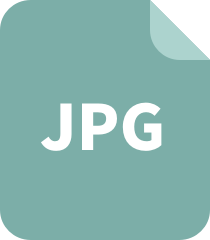
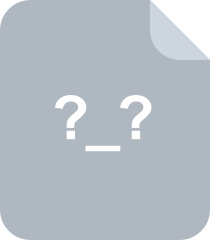
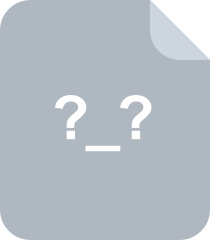
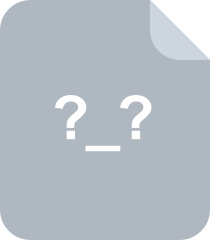
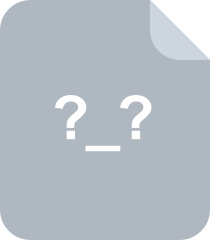
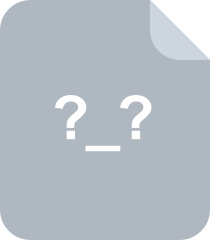
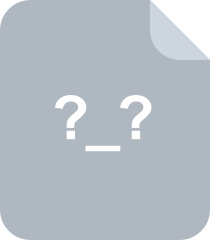
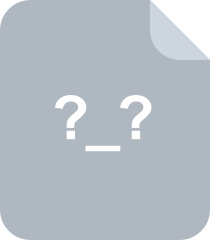
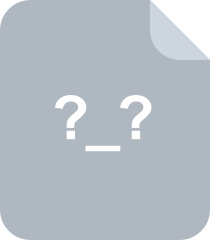
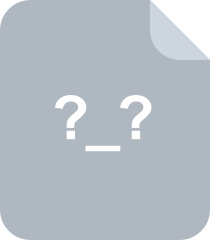
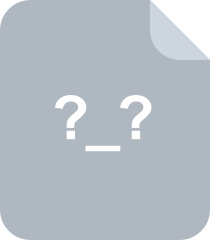
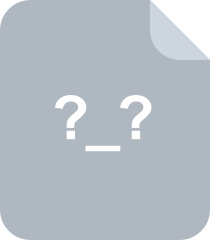
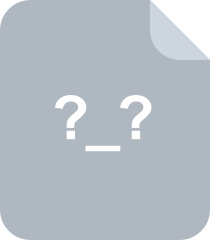
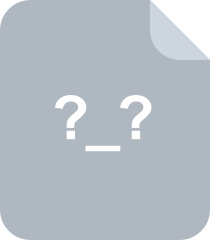
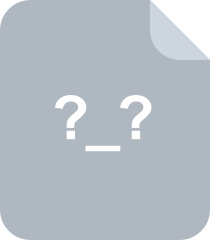
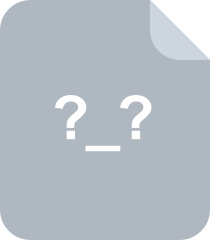
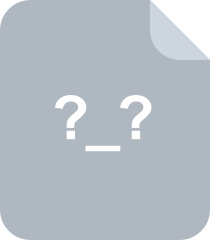
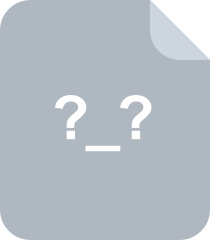
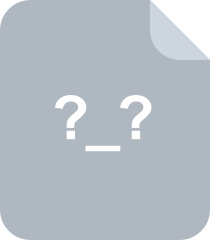
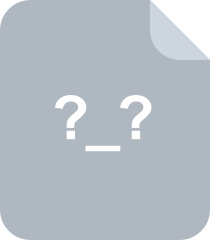
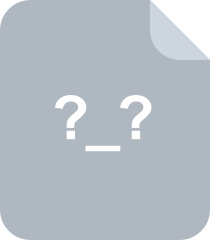
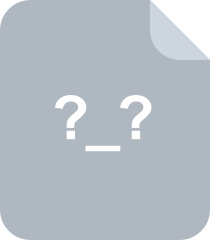
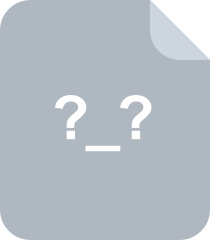
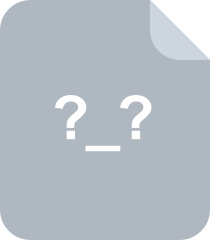
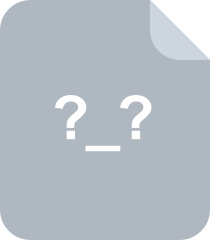
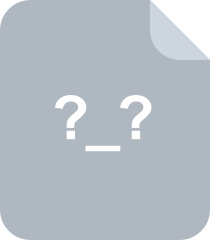
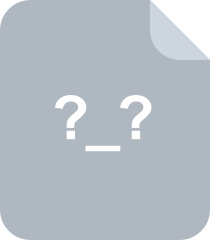
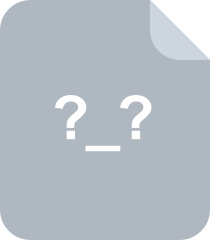
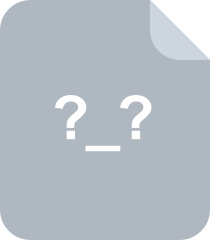
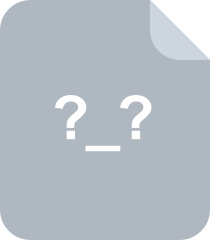
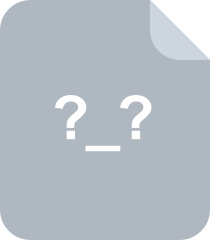
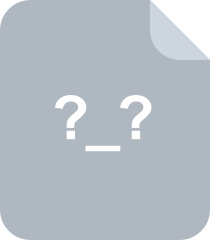
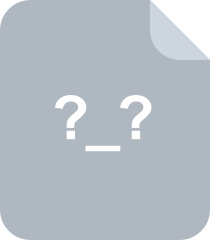
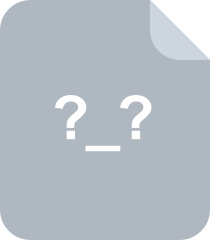
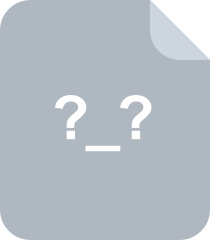
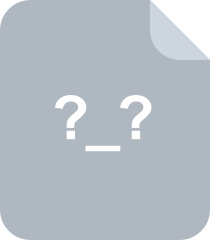
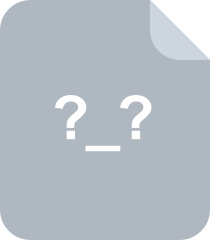
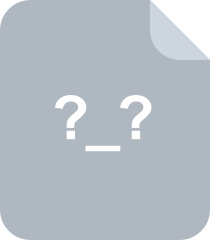
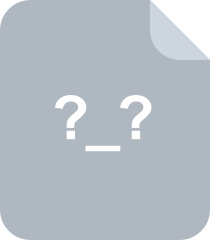
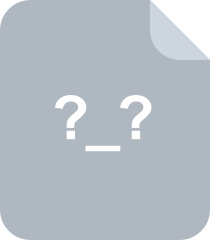
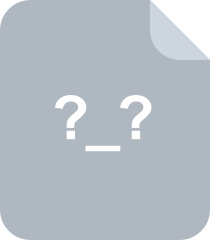
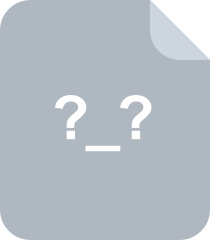
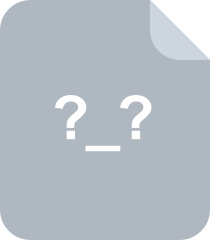
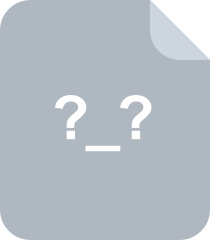
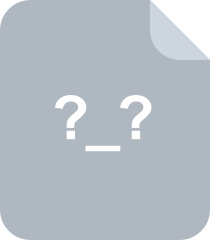
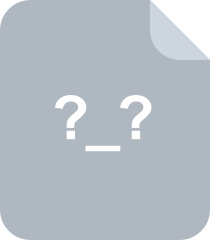
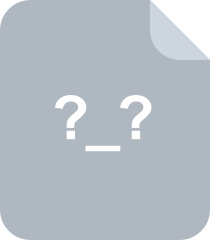
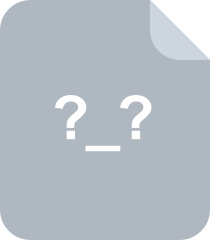
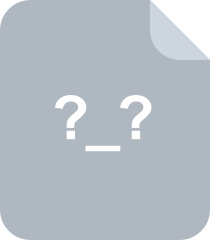
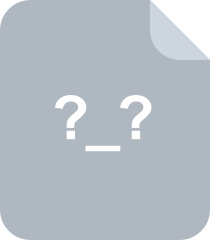
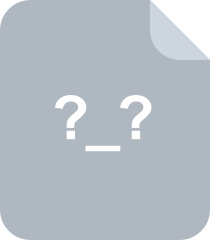
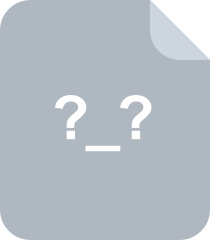
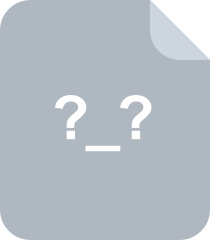
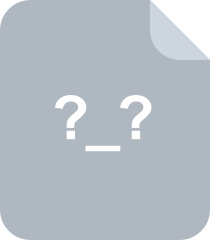
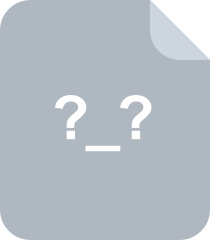
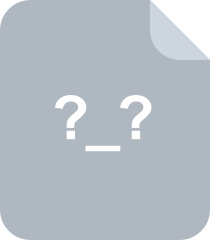
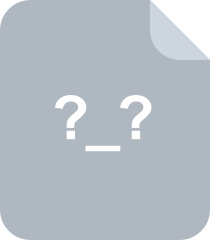
共 608 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
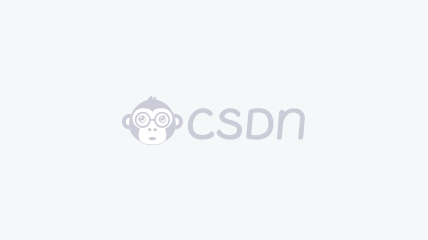
- 不美的阿美2023-07-26:虽然界面不是很华丽,但这个电子书商城网站系统的性能令人满意,确实值得一试。
- 熊比哒2023-07-26:这个电子书商城网站系统确实给我带来了很多方便,可以轻松搜索和购买我想要的图书。
- weixin_357804262023-07-26:这个电子书商城网站系统功能齐全,操作简单易上手。
- 亚赛大人2023-07-26:作为一个初学者,我通过研究这个电子书商城网站系统,对Python Django的应用有了更深入的理解。
- love彤彤2023-07-26:对于学习Python Django的同学来说,这个项目是一个很好的实践材料。

shejizuopin
- 粉丝: 1w+
- 资源: 1300
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

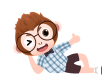
最新资源
- x64dbg-development-2022-09-07-14-52.zip
- 多彩吉安红色旅游网站-JAVA-基于springBoot多彩吉安红色旅游网站的设计与实现
- 本 repo 包含使用新 cv2 接口的 OpenCV-Python 库教程.zip
- 更新框架 (TUF) 的 Python 参考实现.zip
- Qos,GCC,pacing,Nack
- 章节1:Python入门视频
- 无需样板的 Python 类.zip
- ESP32 : 32-bit MCU & 2.4 GHz Wi-Fi & BT/BLE SoCs
- 博物馆文博资源库-JAVA-基于springBoot博物馆文博资源库系统设计与实现
- 旅游网站-JAVA-springboot+vue的桂林旅游网站系统设计与实现
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


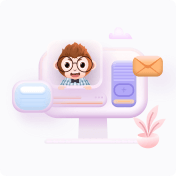
安全验证
文档复制为VIP权益,开通VIP直接复制
