/**
******************************************************************************
* @file stm32f10x_tim.c
* @author MCD Application Team
* @version V3.5.0
* @date 11-March-2011
* @brief This file provides all the TIM firmware functions.
******************************************************************************
* @attention
*
* THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS
* WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE
* TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY
* DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING
* FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE
* CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS.
*
* <h2><center>© COPYRIGHT 2011 STMicroelectronics</center></h2>
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f10x_tim.h"
#include "stm32f10x_rcc.h"
/** @addtogroup STM32F10x_StdPeriph_Driver
* @{
*/
/** @defgroup TIM
* @brief TIM driver modules
* @{
*/
/** @defgroup TIM_Private_TypesDefinitions
* @{
*/
/**
* @}
*/
/** @defgroup TIM_Private_Defines
* @{
*/
/* ---------------------- TIM registers bit mask ------------------------ */
#define SMCR_ETR_Mask ((uint16_t)0x00FF)
#define CCMR_Offset ((uint16_t)0x0018)
#define CCER_CCE_Set ((uint16_t)0x0001)
#define CCER_CCNE_Set ((uint16_t)0x0004)
/**
* @}
*/
/** @defgroup TIM_Private_Macros
* @{
*/
/**
* @}
*/
/** @defgroup TIM_Private_Variables
* @{
*/
/**
* @}
*/
/** @defgroup TIM_Private_FunctionPrototypes
* @{
*/
static void TI1_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection,
uint16_t TIM_ICFilter);
static void TI2_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection,
uint16_t TIM_ICFilter);
static void TI3_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection,
uint16_t TIM_ICFilter);
static void TI4_Config(TIM_TypeDef* TIMx, uint16_t TIM_ICPolarity, uint16_t TIM_ICSelection,
uint16_t TIM_ICFilter);
/**
* @}
*/
/** @defgroup TIM_Private_Macros
* @{
*/
/**
* @}
*/
/** @defgroup TIM_Private_Variables
* @{
*/
/**
* @}
*/
/** @defgroup TIM_Private_FunctionPrototypes
* @{
*/
/**
* @}
*/
/** @defgroup TIM_Private_Functions
* @{
*/
/**
* @brief Deinitializes the TIMx peripheral registers to their default reset values.
* @param TIMx: where x can be 1 to 17 to select the TIM peripheral.
* @retval None
*/
void TIM_DeInit(TIM_TypeDef* TIMx)
{
/* Check the parameters */
assert_param(IS_TIM_ALL_PERIPH(TIMx));
if (TIMx == TIM1)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM1, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM1, DISABLE);
}
else if (TIMx == TIM2)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM2, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM2, DISABLE);
}
else if (TIMx == TIM3)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM3, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM3, DISABLE);
}
else if (TIMx == TIM4)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM4, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM4, DISABLE);
}
else if (TIMx == TIM5)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM5, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM5, DISABLE);
}
else if (TIMx == TIM6)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM6, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM6, DISABLE);
}
else if (TIMx == TIM7)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM7, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM7, DISABLE);
}
else if (TIMx == TIM8)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM8, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM8, DISABLE);
}
else if (TIMx == TIM9)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM9, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM9, DISABLE);
}
else if (TIMx == TIM10)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM10, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM10, DISABLE);
}
else if (TIMx == TIM11)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM11, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM11, DISABLE);
}
else if (TIMx == TIM12)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM12, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM12, DISABLE);
}
else if (TIMx == TIM13)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM13, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM13, DISABLE);
}
else if (TIMx == TIM14)
{
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM14, ENABLE);
RCC_APB1PeriphResetCmd(RCC_APB1Periph_TIM14, DISABLE);
}
else if (TIMx == TIM15)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM15, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM15, DISABLE);
}
else if (TIMx == TIM16)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM16, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM16, DISABLE);
}
else
{
if (TIMx == TIM17)
{
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM17, ENABLE);
RCC_APB2PeriphResetCmd(RCC_APB2Periph_TIM17, DISABLE);
}
}
}
/**
* @brief Initializes the TIMx Time Base Unit peripheral according to
* the specified parameters in the TIM_TimeBaseInitStruct.
* @param TIMx: where x can be 1 to 17 to select the TIM peripheral.
* @param TIM_TimeBaseInitStruct: pointer to a TIM_TimeBaseInitTypeDef
* structure that contains the configuration information for the
* specified TIM peripheral.
* @retval None
*/
void TIM_TimeBaseInit(TIM_TypeDef* TIMx, TIM_TimeBaseInitTypeDef* TIM_TimeBaseInitStruct)
{
uint16_t tmpcr1 = 0;
/* Check the parameters */
assert_param(IS_TIM_ALL_PERIPH(TIMx));
assert_param(IS_TIM_COUNTER_MODE(TIM_TimeBaseInitStruct->TIM_CounterMode));
assert_param(IS_TIM_CKD_DIV(TIM_TimeBaseInitStruct->TIM_ClockDivision));
tmpcr1 = TIMx->CR1;
if((TIMx == TIM1) || (TIMx == TIM8)|| (TIMx == TIM2) || (TIMx == TIM3)||
(TIMx == TIM4) || (TIMx == TIM5))
{
/* Select the Counter Mode */
tmpcr1 &= (uint16_t)(~((uint16_t)(TIM_CR1_DIR | TIM_CR1_CMS)));
tmpcr1 |= (uint32_t)TIM_TimeBaseInitStruct->TIM_CounterMode;
}
if((TIMx != TIM6) && (TIMx != TIM7))
{
/* Set the clock division */
tmpcr1 &= (uint16_t)(~((uint16_t)TIM_CR1_CKD));
tmpcr1 |= (uint32_t)TIM_TimeBaseInitStruct->TIM_ClockDivision;
}
TIMx->CR1 = tmpcr1;
/* Set the Autoreload value */
TIMx->ARR = TIM_TimeBaseInitStruct->TIM_Period ;
/* Set the Prescaler value */
TIMx->PSC = TIM_TimeBaseInitStruct->TIM_Prescaler;
if ((TIMx == TIM1) || (TIMx == TIM8)|| (TIMx == TIM15)|| (TIMx == TIM16) || (TIMx == TIM17))
{
/* Set the Repetition Counter value */
TIMx->RCR = TIM_TimeBaseInitStruct->TIM_RepetitionCounter;
}
/* Generate an update event to reload the Prescaler and the Repetition counter
values immediately */
TIMx->EGR = TIM_PSCReloadMode_Immediate;
}
/**
* @brief Initializes the TIMx Channel1 according to the specified
* parameters in the TIM_OCInitStruct.
* @param TIMx: where x can be 1 to 17 except 6 and 7 to select the TIM peripheral.
* @param TIM_OCInitStru
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
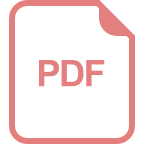
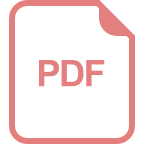
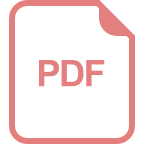
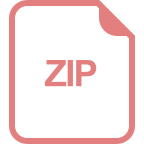
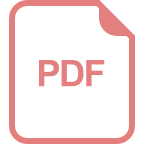
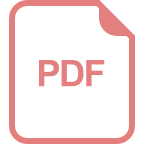
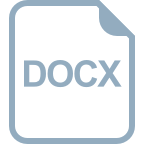
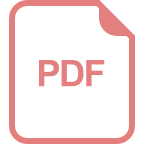
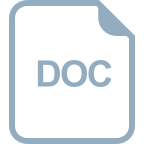
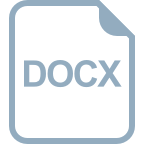
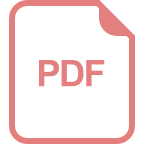
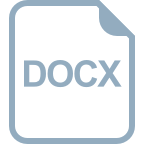
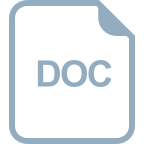
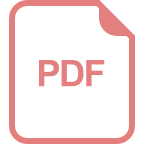
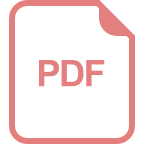
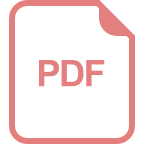
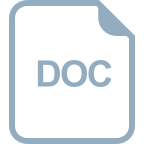
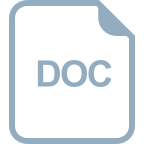
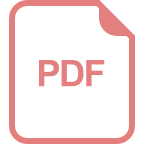
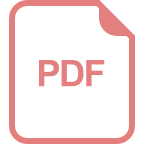
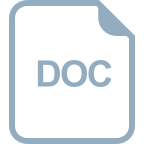
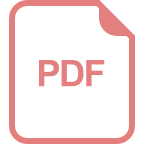
收起资源包目录


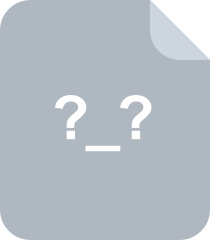

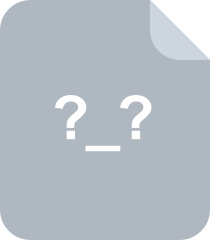
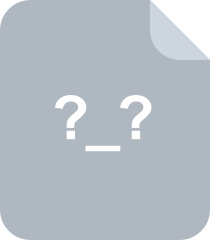
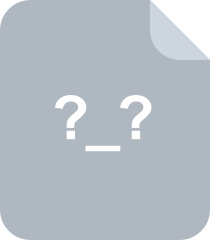
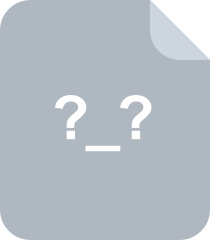
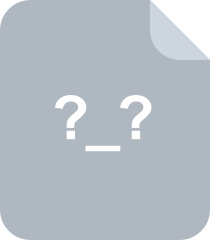
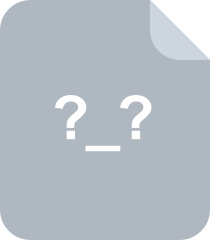



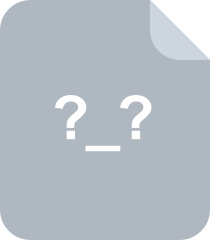
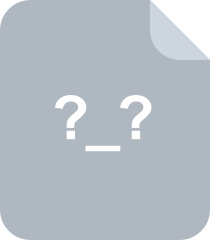
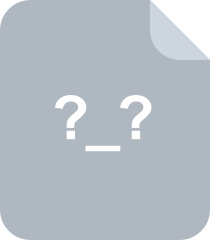
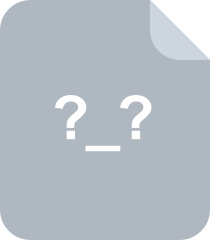
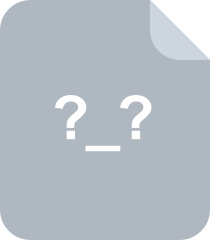
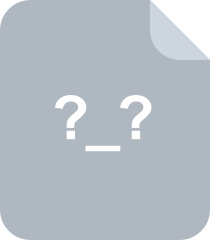
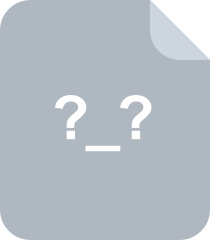
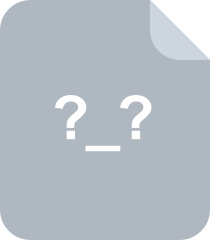
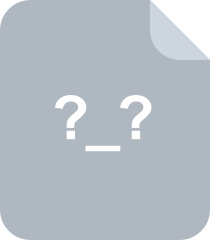
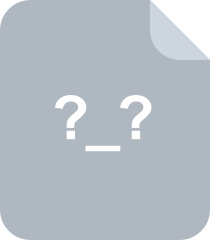
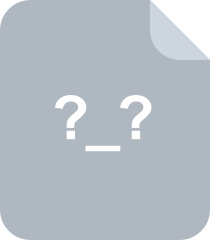
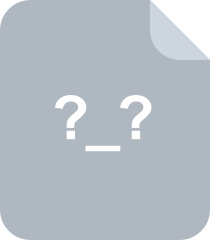
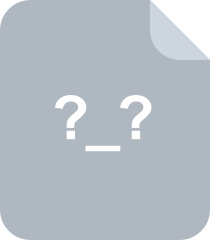
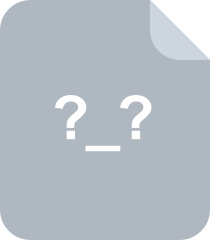
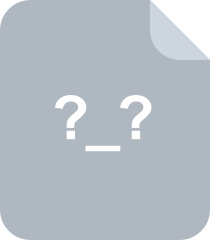
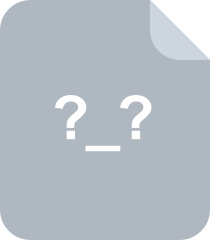
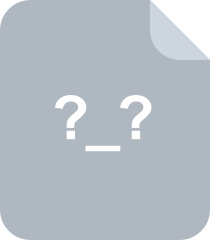
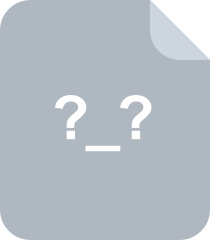
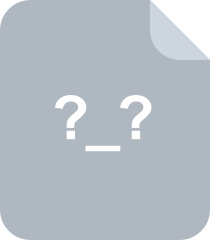
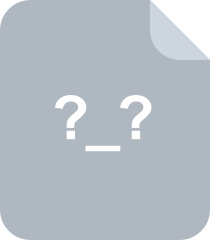
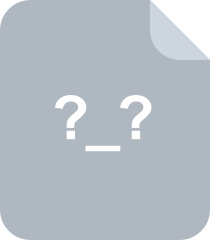
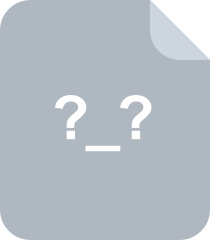
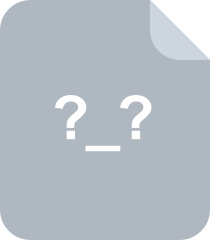

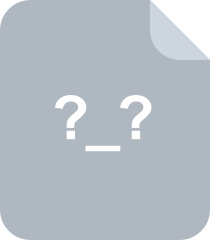
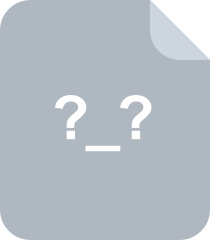
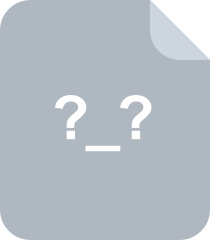
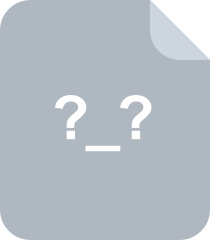
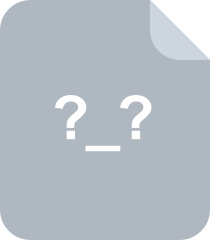
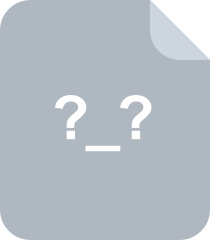
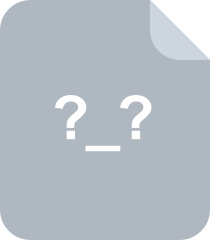
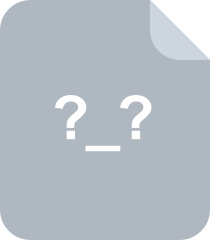
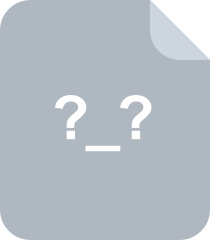
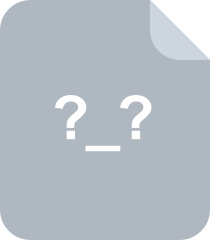
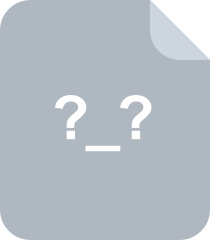
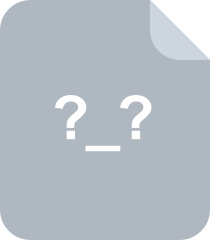
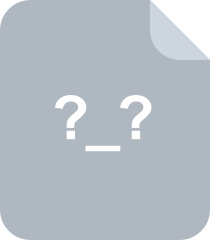
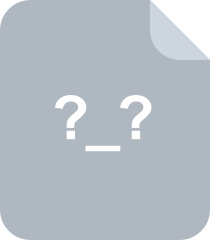
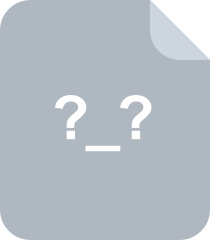
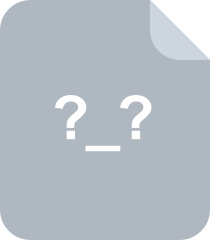
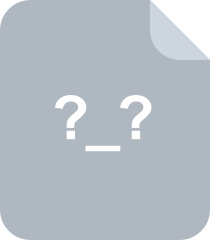
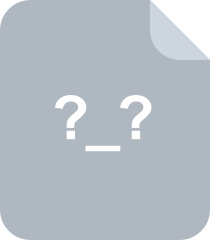
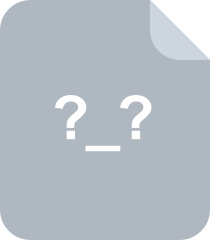
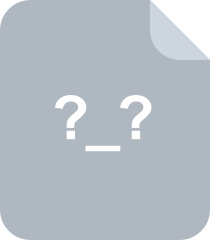
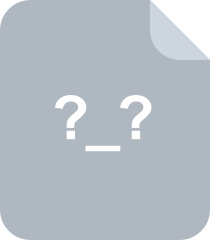
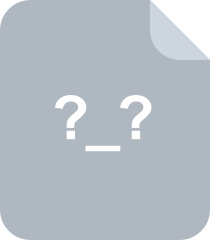
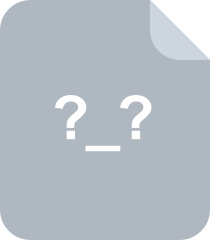

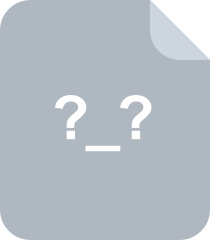
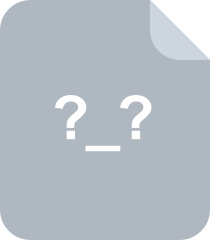
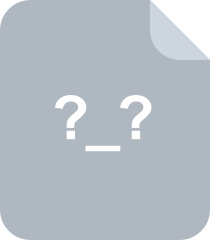
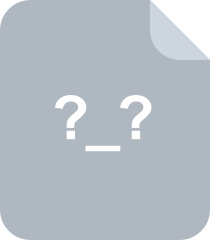
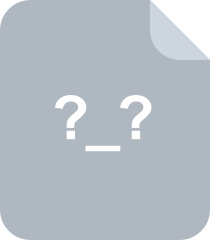

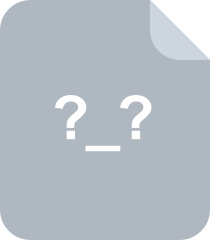



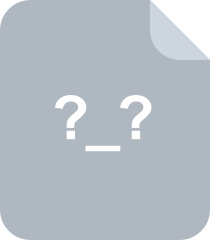
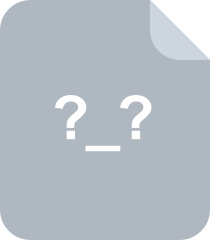

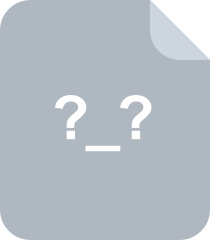
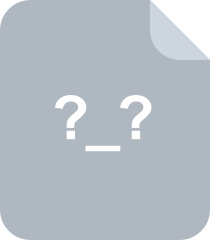
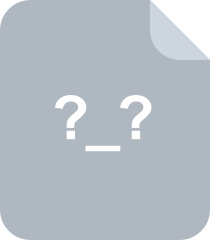
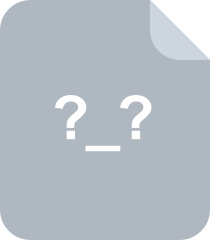
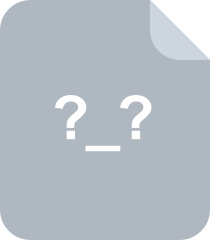
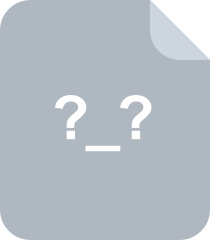
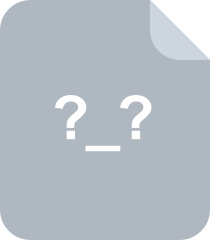
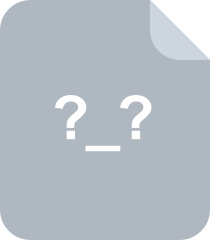
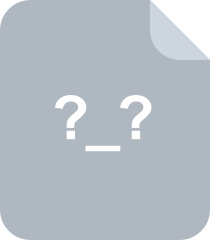


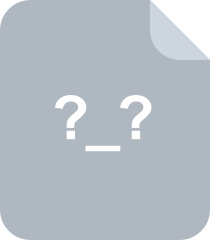
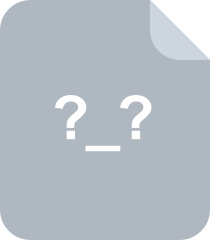

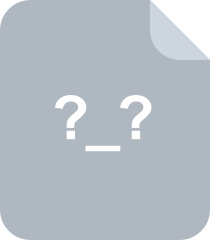
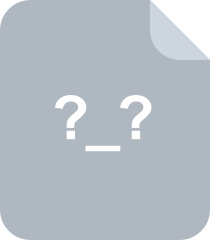

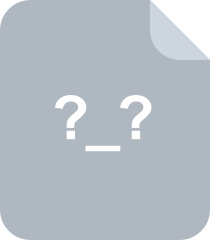
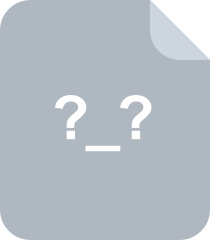
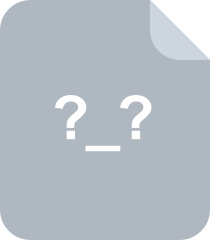

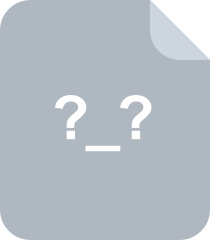
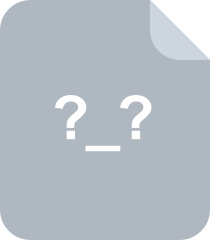
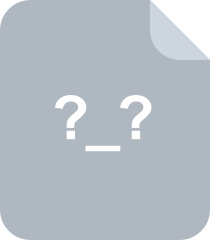

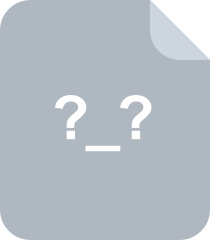
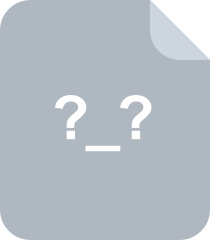

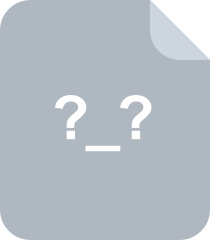
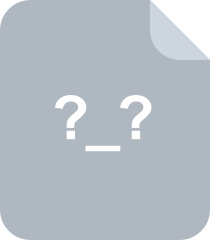

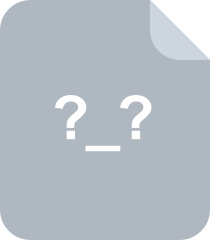
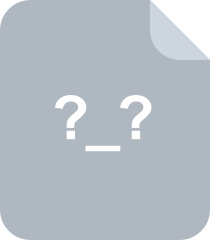

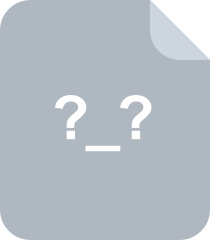
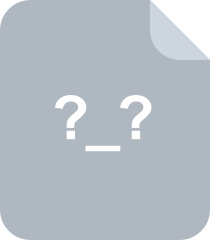

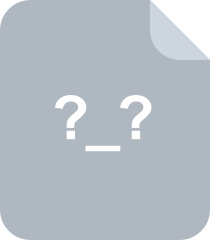
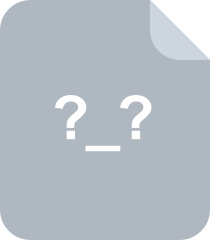

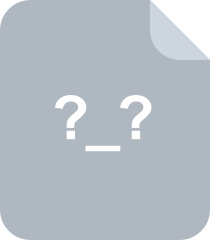
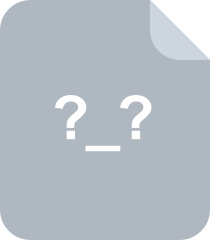

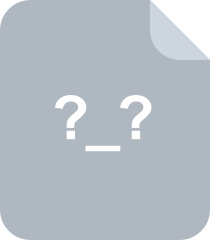
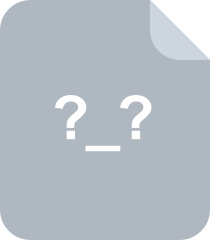
共 94 条
- 1
资源评论
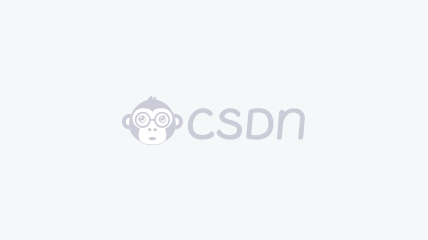

shenminyin
- 粉丝: 153
- 资源: 3
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

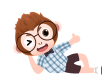
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


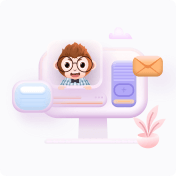
安全验证
文档复制为VIP权益,开通VIP直接复制
