#!/usr/bin/python -tt
# This program is free software; you can redistribute it and/or modify
# it under the terms of the GNU General Public License as published by
# the Free Software Foundation; either version 2 of the License, or
# (at your option) any later version.
#
# This program is distributed in the hope that it will be useful,
# but WITHOUT ANY WARRANTY; without even the implied warranty of
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
# GNU Library General Public License for more details.
#
# You should have received a copy of the GNU General Public License
# along with this program; if not, write to the Free Software
# Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
# Copyright 2005 Duke University
"""
The Yum RPM software updater.
"""
import os
import os.path
import rpm
import re
import types
import errno
import time
import glob
import fnmatch
import logging
import logging.config
import operator
import yum.i18n
_ = yum.i18n._
P_ = yum.i18n.P_
import config
from config import ParsingError, ConfigParser
import Errors
import rpmsack
import rpmUtils.updates
from rpmUtils.arch import archDifference, canCoinstall, ArchStorage, isMultiLibArch
from rpmUtils.miscutils import compareEVR
import rpmUtils.transaction
import comps
import pkgtag_db
from repos import RepoStorage
import misc
from parser import ConfigPreProcessor, varReplace
import transactioninfo
import urlgrabber
from urlgrabber.grabber import URLGrabber, URLGrabError
from urlgrabber.progress import format_number
from packageSack import packagesNewestByName, packagesNewestByNameArch, ListPackageSack
import depsolve
import plugins
import logginglevels
import yumRepo
import callbacks
import yum.history
import warnings
warnings.simplefilter("ignore", Errors.YumFutureDeprecationWarning)
from packages import parsePackages, comparePoEVR
from packages import YumAvailablePackage, YumLocalPackage, YumInstalledPackage
from packages import YumUrlPackage
from constants import *
from yum.rpmtrans import RPMTransaction,SimpleCliCallBack
from yum.i18n import to_unicode, to_str
import string
from weakref import proxy as weakref
from urlgrabber.grabber import default_grabber
__version__ = '3.2.28'
__version_info__ = tuple([ int(num) for num in __version__.split('.')])
# Setup a default_grabber UA here that says we are yum, done using the global
# so that other API users can easily add to it if they want.
# Don't do it at init time, or we'll get multiple additions if you create
# multiple YumBase() objects.
default_grabber.opts.user_agent += " yum/" + __version__
class _YumPreBaseConf:
"""This is the configuration interface for the YumBase configuration.
So if you want to change if plugins are on/off, or debuglevel/etc.
you tweak it here, and when yb.conf does it's thing ... it happens. """
def __init__(self):
self.fn = '/etc/yum/yum.conf'
self.root = '/'
self.init_plugins = True
self.plugin_types = (plugins.TYPE_CORE,)
self.optparser = None
self.debuglevel = None
self.errorlevel = None
self.disabled_plugins = None
self.enabled_plugins = None
self.syslog_ident = None
self.syslog_facility = None
self.syslog_device = None
self.arch = None
self.releasever = None
self.uuid = None
class _YumPreRepoConf:
"""This is the configuration interface for the repos configuration.
So if you want to change callbacks etc. you tweak it here, and when
yb.repos does it's thing ... it happens. """
def __init__(self):
self.progressbar = None
self.callback = None
self.failure_callback = None
self.interrupt_callback = None
self.confirm_func = None
self.gpg_import_func = None
self.cachedir = None
self.cache = None
class _YumCostExclude:
""" This excludes packages that are in repos. of lower cost than the passed
repo. """
def __init__(self, repo, repos):
self.repo = weakref(repo)
self._repos = weakref(repos)
def __contains__(self, pkgtup):
# (n, a, e, v, r) = pkgtup
for repo in self._repos.listEnabled():
if repo.cost >= self.repo.cost:
break
# searchNevra is a bit slower, although more generic for repos.
# that don't use sqlitesack as the backend ... although they are
# probably screwed anyway.
#
# if repo.sack.searchNevra(n, e, v, r, a):
if pkgtup in repo.sack._pkgtup2pkgs:
return True
return False
class YumBase(depsolve.Depsolve):
"""This is a primary structure and base class. It houses the objects and
methods needed to perform most things in yum. It is almost an abstract
class in that you will need to add your own class above it for most
real use."""
def __init__(self):
depsolve.Depsolve.__init__(self)
self._conf = None
self._tsInfo = None
self._rpmdb = None
self._up = None
self._comps = None
self._history = None
self._pkgSack = None
self._lockfile = None
self._tags = None
self.skipped_packages = [] # packages skip by the skip-broken code
self.logger = logging.getLogger("yum.YumBase")
self.verbose_logger = logging.getLogger("yum.verbose.YumBase")
self._repos = RepoStorage(self)
self.repo_setopts = {} # since we have to use repo_setopts in base and
# not in cli - set it up as empty so no one
# trips over it later
# Start with plugins disabled
self.disablePlugins()
self.localPackages = [] # for local package handling
self.mediagrabber = None
self.arch = ArchStorage()
self.preconf = _YumPreBaseConf()
self.prerepoconf = _YumPreRepoConf()
self.run_with_package_names = set()
def __del__(self):
self.close()
self.closeRpmDB()
self.doUnlock()
def close(self):
# We don't want to create the object, so we test if it's been created
if self._history is not None:
self.history.close()
if self._repos:
self._repos.close()
def _transactionDataFactory(self):
"""Factory method returning TransactionData object"""
return transactioninfo.TransactionData()
def doGenericSetup(self, cache=0):
"""do a default setup for all the normal/necessary yum components,
really just a shorthand for testing"""
self.preconf.init_plugins = False
self.conf.cache = cache
def doConfigSetup(self, fn='/etc/yum/yum.conf', root='/', init_plugins=True,
plugin_types=(plugins.TYPE_CORE,), optparser=None, debuglevel=None,
errorlevel=None):
warnings.warn(_('doConfigSetup() will go away in a future version of Yum.\n'),
Errors.YumFutureDeprecationWarning, stacklevel=2)
if hasattr(self, 'preconf'):
self.preconf.fn = fn
self.preconf.root = root
self.preconf.init_plugins = init_plugins
self.preconf.plugin_types = plugin_types
self.preconf.optparser = optparser
self.preconf.debuglevel = debuglevel
self.preconf.errorlevel = errorlevel
return self.conf
def _getConfig(self, **kwargs):
'''
Parse and load Yum's configuration files and call hooks initialise
plugins and logging. Uses self.preconf for pre-configuration,
configuration. '''
# ' xemacs syntax hack
if kwargs:
warnings.warn('Use .preconf instead of passing args to _getConfig')
if self._conf:
return self._conf
conf_st = time.time()
if kwargs:
for arg in ('f
没有合适的资源?快使用搜索试试~ 我知道了~
yum离线安装包,用于RedHat或者CentOS

温馨提示
yum-3.2.28.tar.gz离线安装包,傻瓜式安装, 是一个在Fedora和RedHat以及CentOS中的Shell前端软件包管理器。基于RPM包管理,能够从指定的服务器自动下载RPM包并且安装,可以自动处理依赖性关系,并且一次安装所有依赖的软件包,无须繁琐地操作
资源推荐
资源详情
资源评论
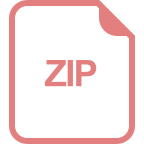
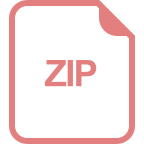
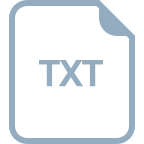
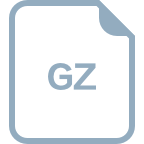
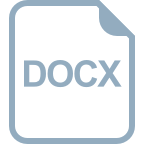
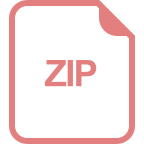
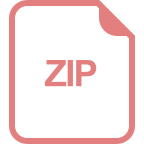
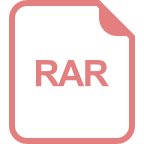
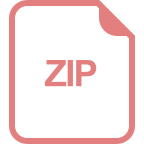
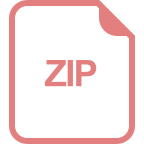
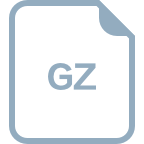
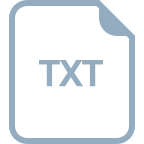
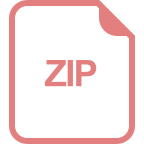
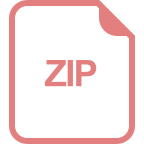
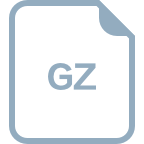
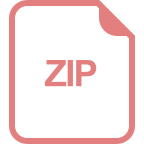
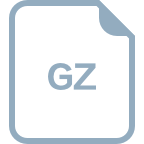
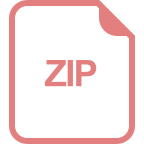
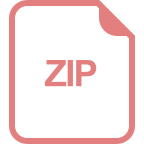
收起资源包目录

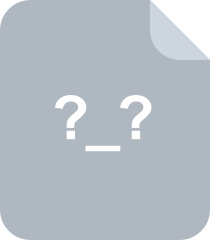
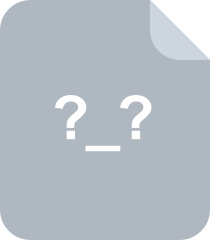
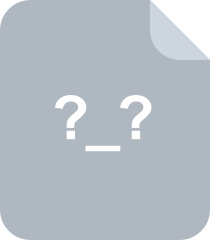
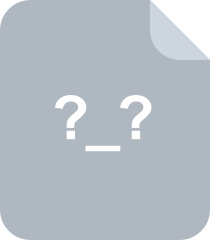
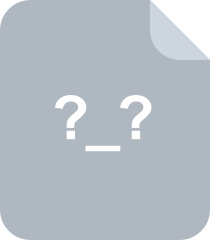
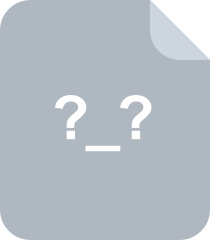
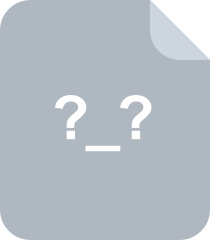
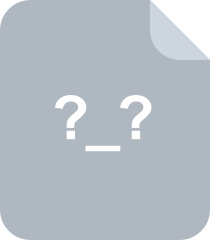
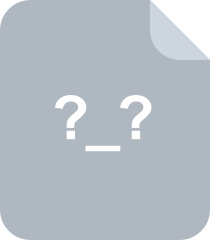
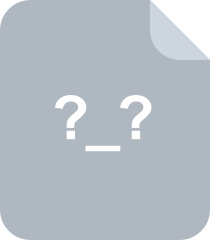
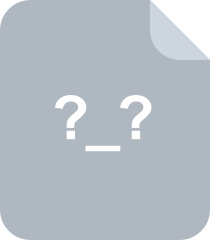
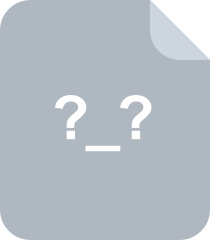
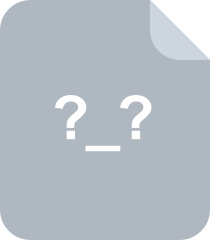
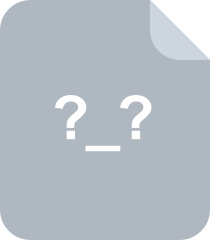
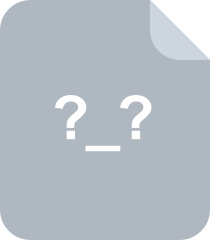
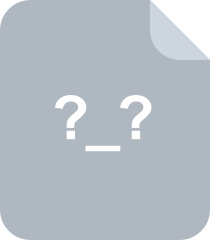
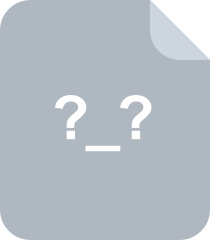
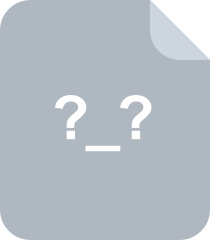
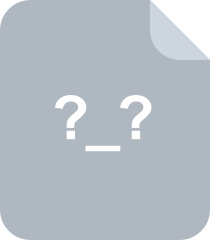
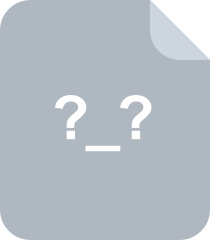
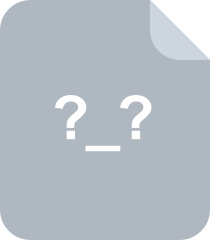
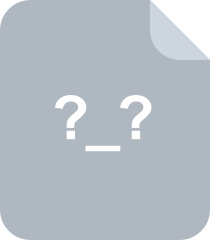
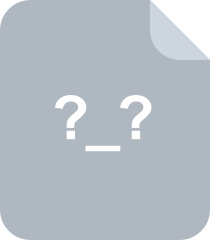
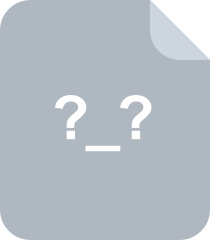
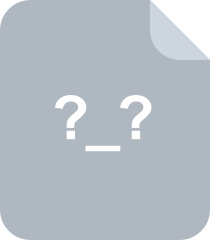
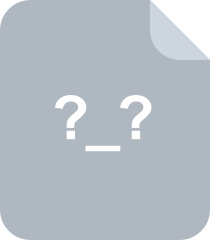
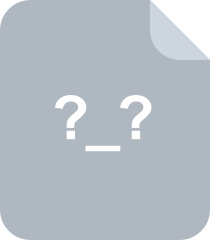
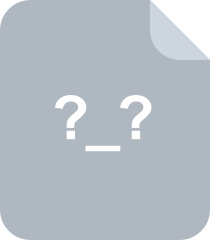
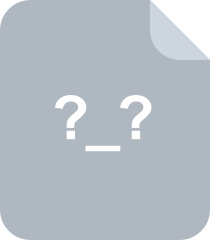
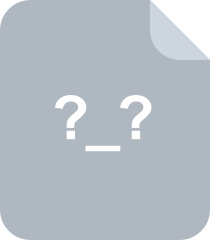
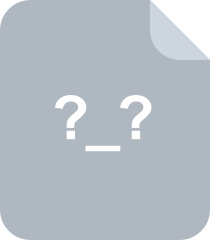
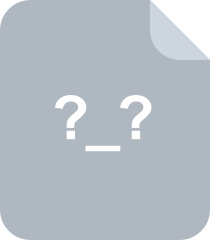
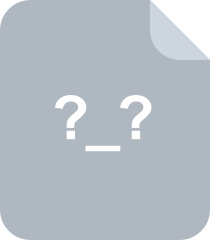
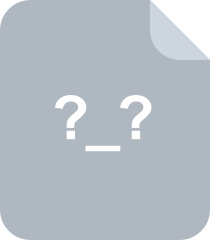
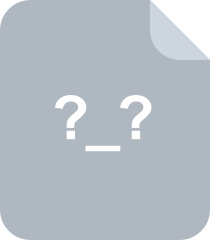
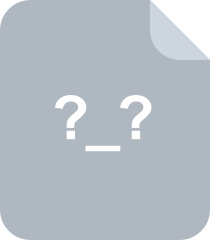
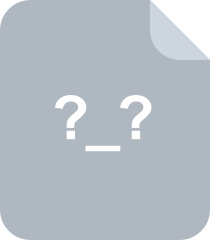
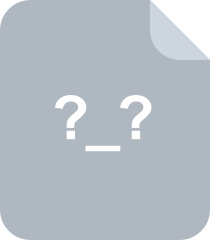
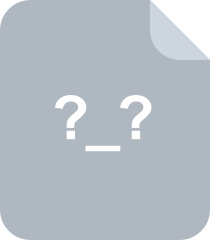
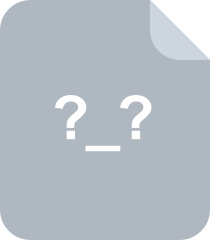
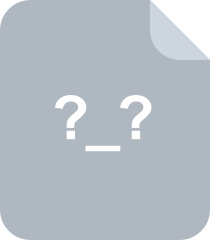
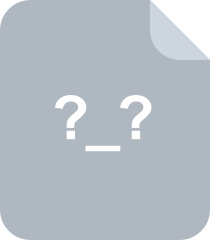
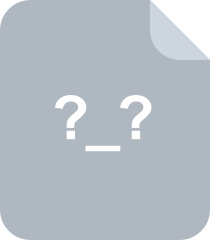
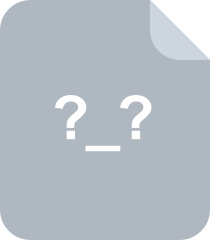
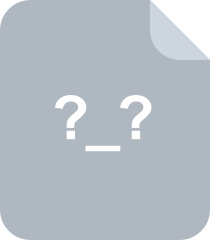
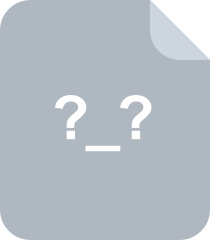
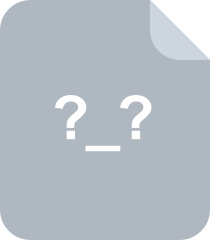
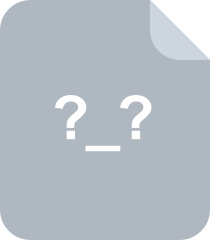
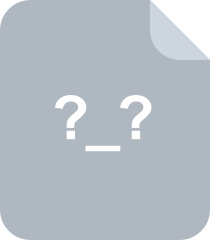
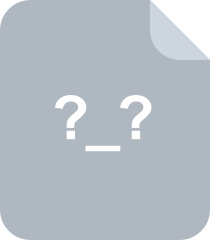
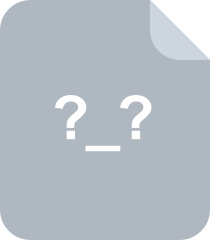
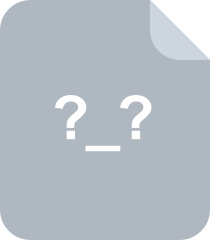
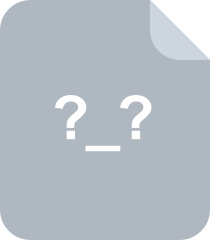
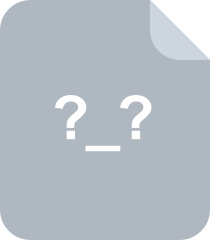
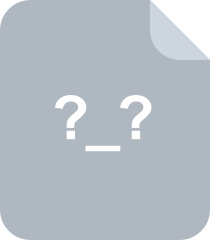
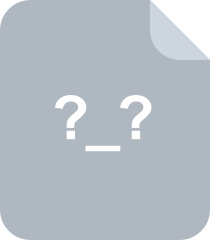
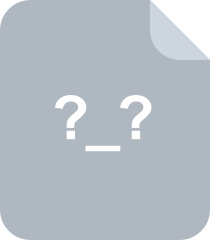
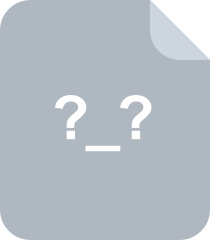
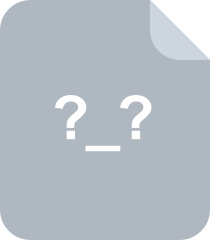
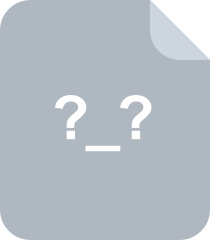
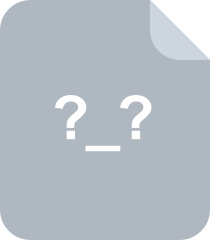
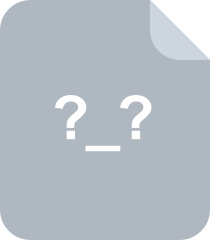
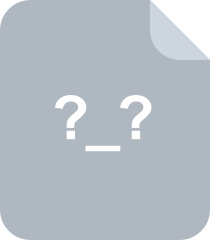
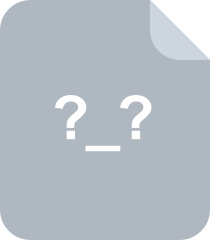
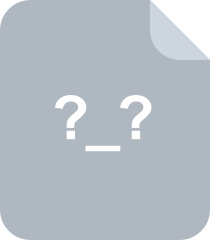
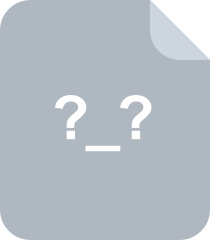
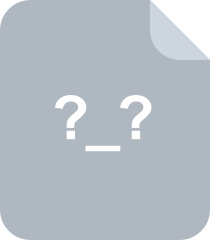
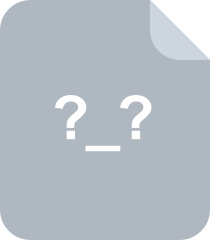
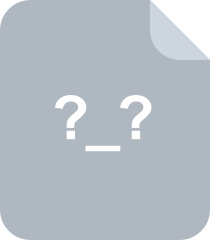
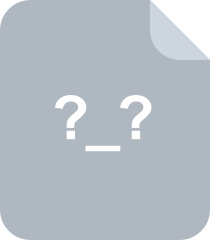
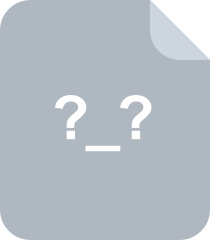
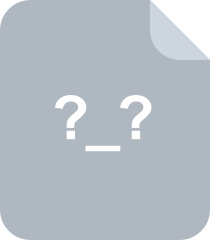
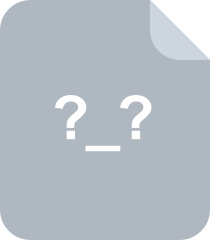
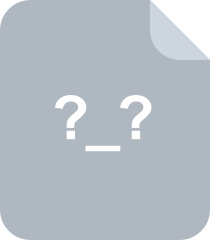
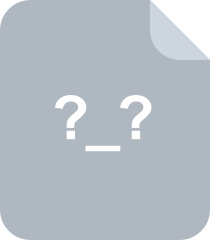
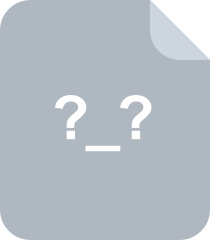
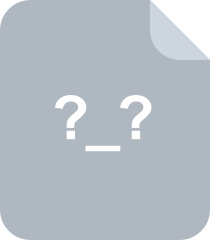
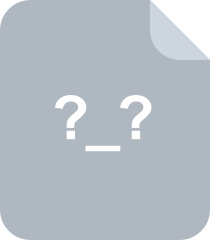
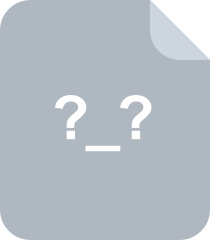
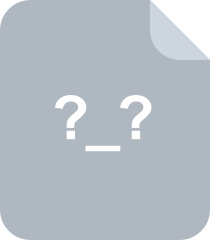
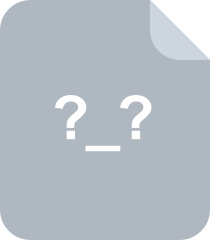
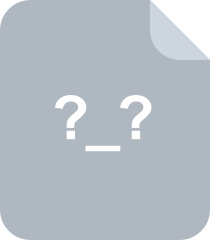
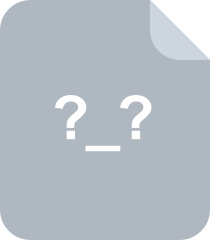
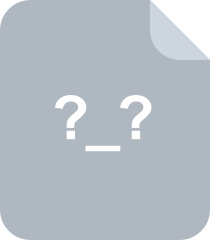
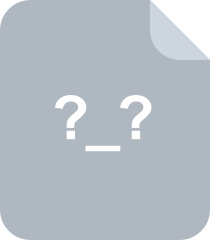
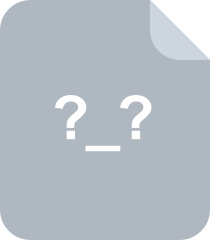
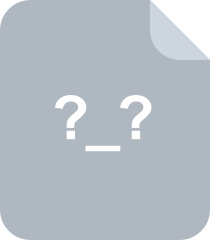
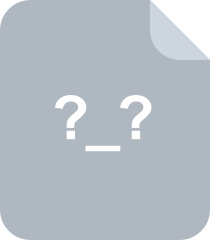
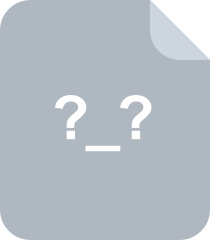
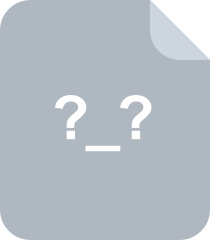
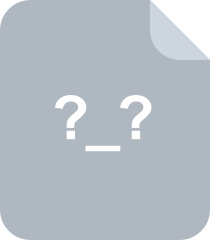
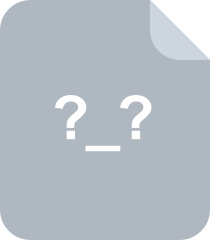
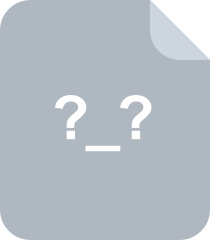
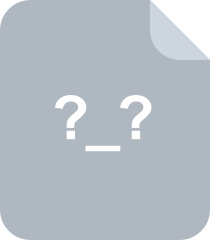
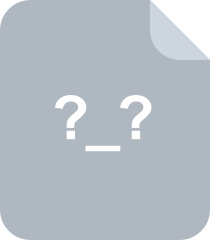
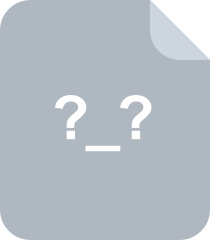
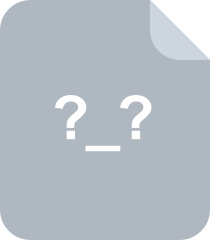
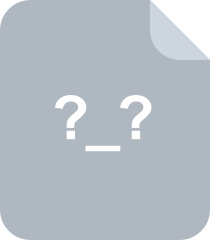
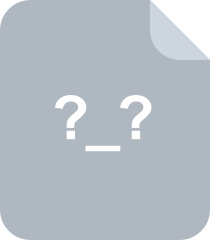
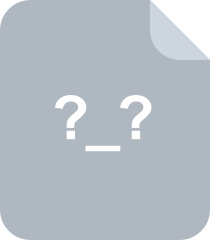
共 123 条
- 1
- 2
资源评论
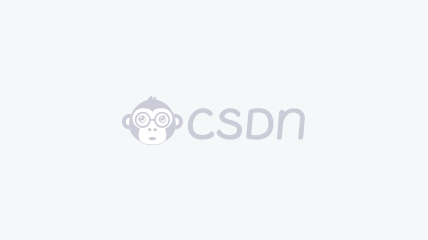
- 梁林張斌2019-03-14https://download.csdn.net/download/qq_33378998/10566158#comment

shanyuan_zlz
- 粉丝: 2
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

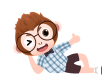
安全验证
文档复制为VIP权益,开通VIP直接复制
